Vuex Mutating a state variable not working
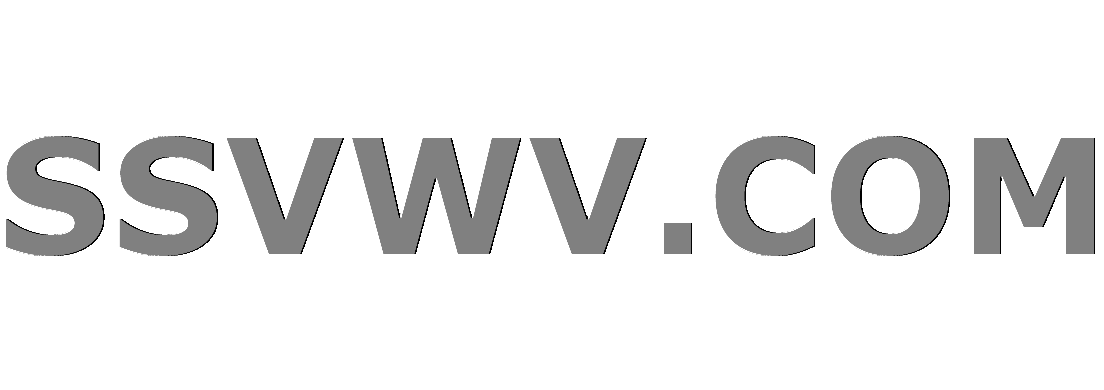
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I'm trying to update my contacts when a different brand is selected. When I select a new brand, the contacts should be updated. So I clear my array of the contacts of the brand and then fill it again.
Somehow though, the clearing of the array doesn't work in my Vuex setup. Is there anyone who knows why?
This is my Store file:
export default {
state: {
brands: Array(), //Might be used later on, if not, remove.
brandsForDropdown: Array(),
brandContactsForDropdown: Array(),
},
getters: {
brands: state => {
return state.brands;
},
brandsForDropdown: state => {
return state.brandsForDropdown
},
brandContactsForDropdown: state => {
return state.brandContactsForDropdown
}
},
actions: {
getBrands({state, commit}) {
Vue.http.get(process.env.VUE_APP_API_SERVER + '/brands').then(response => {
if(response.body.length > 0) {
for (var i = 0; i < response.body.length; i++) {
commit('pushBrands', {"name" : response.body[i].name, "value" : response.body[i].id})
}
}
}, response => {
// error callback
});
},
getBrandContacts({state, commit}, payload) {
//commit('resetBrandContacts')
Vue.http.get(process.env.VUE_APP_API_SERVER + '/brands/contacts/' + payload.value).then(response => {
if(response.body.length > 0) {
let newArray = ;
for (var i = 0; i < response.body.length; i++) {
newArray.push({"name" : response.body[i].firstname + " " + response.body[i].surname, "value" : response.body[i].id})
}
commit('pushBrandContact', newArray)
}
}, response => {
// error callback
});
}
},
mutations: {
pushBrands(state, payload) {
state.brandsForDropdown.push(payload)
},
resetBrands(state) {
state.brandsForDropdown =
},
resetBrandContacts(state) {
state.brandContactsForDropdown =
},
pushBrandContact(state, payload) {
console.log(payload)
state.brandContactsForDropdown = payload
console.log(state.brandContactsForDropdown)
}
}
}
This is my full component code:
<script>
export default {
data () {
return {
productName: null,
productBrand: null,
brands: this.$store.getters.brandsForDropdown,
selectedBrand: null,
brandContacts: this.$store.getters.brandContactsForDropdown,
selectedContacts: null,
}
},
computed: {
},
watch: {
selectedBrand: function() {
if(this.selectedBrand != null) {
this.$store.dispatch('getBrandContacts', this.selectedBrand)
//this.brandContacts = this.$store.getters.brandContactsForDropdown
}
console.log(this.brandContacts);
}
},
methods: {
},
mounted: function() {
this.$store.dispatch('getBrands')
}
}
</script>
And up there is my full Vuex module.
javascript vue.js vuex
|
show 2 more comments
I'm trying to update my contacts when a different brand is selected. When I select a new brand, the contacts should be updated. So I clear my array of the contacts of the brand and then fill it again.
Somehow though, the clearing of the array doesn't work in my Vuex setup. Is there anyone who knows why?
This is my Store file:
export default {
state: {
brands: Array(), //Might be used later on, if not, remove.
brandsForDropdown: Array(),
brandContactsForDropdown: Array(),
},
getters: {
brands: state => {
return state.brands;
},
brandsForDropdown: state => {
return state.brandsForDropdown
},
brandContactsForDropdown: state => {
return state.brandContactsForDropdown
}
},
actions: {
getBrands({state, commit}) {
Vue.http.get(process.env.VUE_APP_API_SERVER + '/brands').then(response => {
if(response.body.length > 0) {
for (var i = 0; i < response.body.length; i++) {
commit('pushBrands', {"name" : response.body[i].name, "value" : response.body[i].id})
}
}
}, response => {
// error callback
});
},
getBrandContacts({state, commit}, payload) {
//commit('resetBrandContacts')
Vue.http.get(process.env.VUE_APP_API_SERVER + '/brands/contacts/' + payload.value).then(response => {
if(response.body.length > 0) {
let newArray = ;
for (var i = 0; i < response.body.length; i++) {
newArray.push({"name" : response.body[i].firstname + " " + response.body[i].surname, "value" : response.body[i].id})
}
commit('pushBrandContact', newArray)
}
}, response => {
// error callback
});
}
},
mutations: {
pushBrands(state, payload) {
state.brandsForDropdown.push(payload)
},
resetBrands(state) {
state.brandsForDropdown =
},
resetBrandContacts(state) {
state.brandContactsForDropdown =
},
pushBrandContact(state, payload) {
console.log(payload)
state.brandContactsForDropdown = payload
console.log(state.brandContactsForDropdown)
}
}
}
This is my full component code:
<script>
export default {
data () {
return {
productName: null,
productBrand: null,
brands: this.$store.getters.brandsForDropdown,
selectedBrand: null,
brandContacts: this.$store.getters.brandContactsForDropdown,
selectedContacts: null,
}
},
computed: {
},
watch: {
selectedBrand: function() {
if(this.selectedBrand != null) {
this.$store.dispatch('getBrandContacts', this.selectedBrand)
//this.brandContacts = this.$store.getters.brandContactsForDropdown
}
console.log(this.brandContacts);
}
},
methods: {
},
mounted: function() {
this.$store.dispatch('getBrands')
}
}
</script>
And up there is my full Vuex module.
javascript vue.js vuex
Is that last line in thedata
method of a component? Because if so,brandContacts
is only getting set once on the component's initialization, and you would need to makebrandContacts
a computed property instead in order for it to reflect any changes to the value ofthis.$store.getters.brandContactsForDropdown
.
– thanksd
Nov 16 '18 at 14:46
The last line is in the data method of my Vue component. It's weird how another value I set IS updated when it changes in Vuex...
– Danoctum
Nov 16 '18 at 14:53
Yeah, without seeing that code it's hard to know what would cause that.
– thanksd
Nov 16 '18 at 15:01
Added the code, hope you can find what's wrong :)
– Danoctum
Nov 16 '18 at 15:14
So I'm assuming thebrands
property is the one you said is getting updated when it changes in vuex. But where is that code?
– thanksd
Nov 16 '18 at 15:20
|
show 2 more comments
I'm trying to update my contacts when a different brand is selected. When I select a new brand, the contacts should be updated. So I clear my array of the contacts of the brand and then fill it again.
Somehow though, the clearing of the array doesn't work in my Vuex setup. Is there anyone who knows why?
This is my Store file:
export default {
state: {
brands: Array(), //Might be used later on, if not, remove.
brandsForDropdown: Array(),
brandContactsForDropdown: Array(),
},
getters: {
brands: state => {
return state.brands;
},
brandsForDropdown: state => {
return state.brandsForDropdown
},
brandContactsForDropdown: state => {
return state.brandContactsForDropdown
}
},
actions: {
getBrands({state, commit}) {
Vue.http.get(process.env.VUE_APP_API_SERVER + '/brands').then(response => {
if(response.body.length > 0) {
for (var i = 0; i < response.body.length; i++) {
commit('pushBrands', {"name" : response.body[i].name, "value" : response.body[i].id})
}
}
}, response => {
// error callback
});
},
getBrandContacts({state, commit}, payload) {
//commit('resetBrandContacts')
Vue.http.get(process.env.VUE_APP_API_SERVER + '/brands/contacts/' + payload.value).then(response => {
if(response.body.length > 0) {
let newArray = ;
for (var i = 0; i < response.body.length; i++) {
newArray.push({"name" : response.body[i].firstname + " " + response.body[i].surname, "value" : response.body[i].id})
}
commit('pushBrandContact', newArray)
}
}, response => {
// error callback
});
}
},
mutations: {
pushBrands(state, payload) {
state.brandsForDropdown.push(payload)
},
resetBrands(state) {
state.brandsForDropdown =
},
resetBrandContacts(state) {
state.brandContactsForDropdown =
},
pushBrandContact(state, payload) {
console.log(payload)
state.brandContactsForDropdown = payload
console.log(state.brandContactsForDropdown)
}
}
}
This is my full component code:
<script>
export default {
data () {
return {
productName: null,
productBrand: null,
brands: this.$store.getters.brandsForDropdown,
selectedBrand: null,
brandContacts: this.$store.getters.brandContactsForDropdown,
selectedContacts: null,
}
},
computed: {
},
watch: {
selectedBrand: function() {
if(this.selectedBrand != null) {
this.$store.dispatch('getBrandContacts', this.selectedBrand)
//this.brandContacts = this.$store.getters.brandContactsForDropdown
}
console.log(this.brandContacts);
}
},
methods: {
},
mounted: function() {
this.$store.dispatch('getBrands')
}
}
</script>
And up there is my full Vuex module.
javascript vue.js vuex
I'm trying to update my contacts when a different brand is selected. When I select a new brand, the contacts should be updated. So I clear my array of the contacts of the brand and then fill it again.
Somehow though, the clearing of the array doesn't work in my Vuex setup. Is there anyone who knows why?
This is my Store file:
export default {
state: {
brands: Array(), //Might be used later on, if not, remove.
brandsForDropdown: Array(),
brandContactsForDropdown: Array(),
},
getters: {
brands: state => {
return state.brands;
},
brandsForDropdown: state => {
return state.brandsForDropdown
},
brandContactsForDropdown: state => {
return state.brandContactsForDropdown
}
},
actions: {
getBrands({state, commit}) {
Vue.http.get(process.env.VUE_APP_API_SERVER + '/brands').then(response => {
if(response.body.length > 0) {
for (var i = 0; i < response.body.length; i++) {
commit('pushBrands', {"name" : response.body[i].name, "value" : response.body[i].id})
}
}
}, response => {
// error callback
});
},
getBrandContacts({state, commit}, payload) {
//commit('resetBrandContacts')
Vue.http.get(process.env.VUE_APP_API_SERVER + '/brands/contacts/' + payload.value).then(response => {
if(response.body.length > 0) {
let newArray = ;
for (var i = 0; i < response.body.length; i++) {
newArray.push({"name" : response.body[i].firstname + " " + response.body[i].surname, "value" : response.body[i].id})
}
commit('pushBrandContact', newArray)
}
}, response => {
// error callback
});
}
},
mutations: {
pushBrands(state, payload) {
state.brandsForDropdown.push(payload)
},
resetBrands(state) {
state.brandsForDropdown =
},
resetBrandContacts(state) {
state.brandContactsForDropdown =
},
pushBrandContact(state, payload) {
console.log(payload)
state.brandContactsForDropdown = payload
console.log(state.brandContactsForDropdown)
}
}
}
This is my full component code:
<script>
export default {
data () {
return {
productName: null,
productBrand: null,
brands: this.$store.getters.brandsForDropdown,
selectedBrand: null,
brandContacts: this.$store.getters.brandContactsForDropdown,
selectedContacts: null,
}
},
computed: {
},
watch: {
selectedBrand: function() {
if(this.selectedBrand != null) {
this.$store.dispatch('getBrandContacts', this.selectedBrand)
//this.brandContacts = this.$store.getters.brandContactsForDropdown
}
console.log(this.brandContacts);
}
},
methods: {
},
mounted: function() {
this.$store.dispatch('getBrands')
}
}
</script>
And up there is my full Vuex module.
javascript vue.js vuex
javascript vue.js vuex
edited Nov 16 '18 at 15:26
Danoctum
asked Nov 16 '18 at 14:41
DanoctumDanoctum
779
779
Is that last line in thedata
method of a component? Because if so,brandContacts
is only getting set once on the component's initialization, and you would need to makebrandContacts
a computed property instead in order for it to reflect any changes to the value ofthis.$store.getters.brandContactsForDropdown
.
– thanksd
Nov 16 '18 at 14:46
The last line is in the data method of my Vue component. It's weird how another value I set IS updated when it changes in Vuex...
– Danoctum
Nov 16 '18 at 14:53
Yeah, without seeing that code it's hard to know what would cause that.
– thanksd
Nov 16 '18 at 15:01
Added the code, hope you can find what's wrong :)
– Danoctum
Nov 16 '18 at 15:14
So I'm assuming thebrands
property is the one you said is getting updated when it changes in vuex. But where is that code?
– thanksd
Nov 16 '18 at 15:20
|
show 2 more comments
Is that last line in thedata
method of a component? Because if so,brandContacts
is only getting set once on the component's initialization, and you would need to makebrandContacts
a computed property instead in order for it to reflect any changes to the value ofthis.$store.getters.brandContactsForDropdown
.
– thanksd
Nov 16 '18 at 14:46
The last line is in the data method of my Vue component. It's weird how another value I set IS updated when it changes in Vuex...
– Danoctum
Nov 16 '18 at 14:53
Yeah, without seeing that code it's hard to know what would cause that.
– thanksd
Nov 16 '18 at 15:01
Added the code, hope you can find what's wrong :)
– Danoctum
Nov 16 '18 at 15:14
So I'm assuming thebrands
property is the one you said is getting updated when it changes in vuex. But where is that code?
– thanksd
Nov 16 '18 at 15:20
Is that last line in the
data
method of a component? Because if so, brandContacts
is only getting set once on the component's initialization, and you would need to make brandContacts
a computed property instead in order for it to reflect any changes to the value of this.$store.getters.brandContactsForDropdown
.– thanksd
Nov 16 '18 at 14:46
Is that last line in the
data
method of a component? Because if so, brandContacts
is only getting set once on the component's initialization, and you would need to make brandContacts
a computed property instead in order for it to reflect any changes to the value of this.$store.getters.brandContactsForDropdown
.– thanksd
Nov 16 '18 at 14:46
The last line is in the data method of my Vue component. It's weird how another value I set IS updated when it changes in Vuex...
– Danoctum
Nov 16 '18 at 14:53
The last line is in the data method of my Vue component. It's weird how another value I set IS updated when it changes in Vuex...
– Danoctum
Nov 16 '18 at 14:53
Yeah, without seeing that code it's hard to know what would cause that.
– thanksd
Nov 16 '18 at 15:01
Yeah, without seeing that code it's hard to know what would cause that.
– thanksd
Nov 16 '18 at 15:01
Added the code, hope you can find what's wrong :)
– Danoctum
Nov 16 '18 at 15:14
Added the code, hope you can find what's wrong :)
– Danoctum
Nov 16 '18 at 15:14
So I'm assuming the
brands
property is the one you said is getting updated when it changes in vuex. But where is that code?– thanksd
Nov 16 '18 at 15:20
So I'm assuming the
brands
property is the one you said is getting updated when it changes in vuex. But where is that code?– thanksd
Nov 16 '18 at 15:20
|
show 2 more comments
1 Answer
1
active
oldest
votes
I assume you reached one of the Gotchas:
I'm not sure if this is the case where you update the array and it will not detect the change, but the best way to update the array would be to place a new array instead of adding to it:
getBrandContacts({state, commit}, payload) {
Vue.http.get(process.env.VUE_APP_API_SERVER + '/brands/contacts/' +
payload.value).then(response => {
if(response.body.length > 0) {
let newArray =
for (var i = 0; i < response.body.length; i++) {
newArray.push({"name" :
response.body[i].firstname + " " + response.body[i].surname, "value" :
response.body[i].id})
}
commit('pushBrandContact', newArray)
}
}, response => {
// error callback
});
}
and in mutations:
pushBrandContact(state, payload) {
state.brandContactsForDropdown = payload
}
It is also not clear how you work with the data inside the component, is it a data? or in computed? sharing this code will help.
You also say the array doesn't clear, what you mean? it have the old values? in the vuex state? on in your component? a lot of info to help is missing.
Update
The data properties are getting updated only on init of the component, and when you manually update it (this.data = newData
), they will not be updated when Vuex is updated.
You need to move the brandContacts: this.$store.getters.brandContactsForDropdown
from the data object to the computed object like this:
brandContacts(){ this.$store.state.brandContactsForDropdown }
This will force vue to update when the brandContactsForDropdown
property in vuex updates.
https://vuejs.org/v2/guide/computed.html
The last line is in the data() of my component. What I mean with the array doesn't clear is that I push contacts to the array, but when a new brand is selected, I want to clear the array to make room for the new contacts of the new brand. The Vuex state should just be cleared, but it seems like it isn't.
– Danoctum
Nov 16 '18 at 14:58
have you tried to replace the whole array as in my answer? did it have the same effect? have you tried moving the line to the computed and set it like this:brandContacts: this.$store.state.brandContactsForDropdown
– Art3mix
Nov 16 '18 at 15:01
I just tried, It seems like the mutation just doesn't want to work.
– Danoctum
Nov 16 '18 at 15:03
The result is just an empty array, like it's initialized.
– Danoctum
Nov 16 '18 at 15:03
how do you know the array is empty? where do you see it?
– Art3mix
Nov 16 '18 at 15:04
|
show 8 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53339991%2fvuex-mutating-a-state-variable-not-working%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I assume you reached one of the Gotchas:
I'm not sure if this is the case where you update the array and it will not detect the change, but the best way to update the array would be to place a new array instead of adding to it:
getBrandContacts({state, commit}, payload) {
Vue.http.get(process.env.VUE_APP_API_SERVER + '/brands/contacts/' +
payload.value).then(response => {
if(response.body.length > 0) {
let newArray =
for (var i = 0; i < response.body.length; i++) {
newArray.push({"name" :
response.body[i].firstname + " " + response.body[i].surname, "value" :
response.body[i].id})
}
commit('pushBrandContact', newArray)
}
}, response => {
// error callback
});
}
and in mutations:
pushBrandContact(state, payload) {
state.brandContactsForDropdown = payload
}
It is also not clear how you work with the data inside the component, is it a data? or in computed? sharing this code will help.
You also say the array doesn't clear, what you mean? it have the old values? in the vuex state? on in your component? a lot of info to help is missing.
Update
The data properties are getting updated only on init of the component, and when you manually update it (this.data = newData
), they will not be updated when Vuex is updated.
You need to move the brandContacts: this.$store.getters.brandContactsForDropdown
from the data object to the computed object like this:
brandContacts(){ this.$store.state.brandContactsForDropdown }
This will force vue to update when the brandContactsForDropdown
property in vuex updates.
https://vuejs.org/v2/guide/computed.html
The last line is in the data() of my component. What I mean with the array doesn't clear is that I push contacts to the array, but when a new brand is selected, I want to clear the array to make room for the new contacts of the new brand. The Vuex state should just be cleared, but it seems like it isn't.
– Danoctum
Nov 16 '18 at 14:58
have you tried to replace the whole array as in my answer? did it have the same effect? have you tried moving the line to the computed and set it like this:brandContacts: this.$store.state.brandContactsForDropdown
– Art3mix
Nov 16 '18 at 15:01
I just tried, It seems like the mutation just doesn't want to work.
– Danoctum
Nov 16 '18 at 15:03
The result is just an empty array, like it's initialized.
– Danoctum
Nov 16 '18 at 15:03
how do you know the array is empty? where do you see it?
– Art3mix
Nov 16 '18 at 15:04
|
show 8 more comments
I assume you reached one of the Gotchas:
I'm not sure if this is the case where you update the array and it will not detect the change, but the best way to update the array would be to place a new array instead of adding to it:
getBrandContacts({state, commit}, payload) {
Vue.http.get(process.env.VUE_APP_API_SERVER + '/brands/contacts/' +
payload.value).then(response => {
if(response.body.length > 0) {
let newArray =
for (var i = 0; i < response.body.length; i++) {
newArray.push({"name" :
response.body[i].firstname + " " + response.body[i].surname, "value" :
response.body[i].id})
}
commit('pushBrandContact', newArray)
}
}, response => {
// error callback
});
}
and in mutations:
pushBrandContact(state, payload) {
state.brandContactsForDropdown = payload
}
It is also not clear how you work with the data inside the component, is it a data? or in computed? sharing this code will help.
You also say the array doesn't clear, what you mean? it have the old values? in the vuex state? on in your component? a lot of info to help is missing.
Update
The data properties are getting updated only on init of the component, and when you manually update it (this.data = newData
), they will not be updated when Vuex is updated.
You need to move the brandContacts: this.$store.getters.brandContactsForDropdown
from the data object to the computed object like this:
brandContacts(){ this.$store.state.brandContactsForDropdown }
This will force vue to update when the brandContactsForDropdown
property in vuex updates.
https://vuejs.org/v2/guide/computed.html
The last line is in the data() of my component. What I mean with the array doesn't clear is that I push contacts to the array, but when a new brand is selected, I want to clear the array to make room for the new contacts of the new brand. The Vuex state should just be cleared, but it seems like it isn't.
– Danoctum
Nov 16 '18 at 14:58
have you tried to replace the whole array as in my answer? did it have the same effect? have you tried moving the line to the computed and set it like this:brandContacts: this.$store.state.brandContactsForDropdown
– Art3mix
Nov 16 '18 at 15:01
I just tried, It seems like the mutation just doesn't want to work.
– Danoctum
Nov 16 '18 at 15:03
The result is just an empty array, like it's initialized.
– Danoctum
Nov 16 '18 at 15:03
how do you know the array is empty? where do you see it?
– Art3mix
Nov 16 '18 at 15:04
|
show 8 more comments
I assume you reached one of the Gotchas:
I'm not sure if this is the case where you update the array and it will not detect the change, but the best way to update the array would be to place a new array instead of adding to it:
getBrandContacts({state, commit}, payload) {
Vue.http.get(process.env.VUE_APP_API_SERVER + '/brands/contacts/' +
payload.value).then(response => {
if(response.body.length > 0) {
let newArray =
for (var i = 0; i < response.body.length; i++) {
newArray.push({"name" :
response.body[i].firstname + " " + response.body[i].surname, "value" :
response.body[i].id})
}
commit('pushBrandContact', newArray)
}
}, response => {
// error callback
});
}
and in mutations:
pushBrandContact(state, payload) {
state.brandContactsForDropdown = payload
}
It is also not clear how you work with the data inside the component, is it a data? or in computed? sharing this code will help.
You also say the array doesn't clear, what you mean? it have the old values? in the vuex state? on in your component? a lot of info to help is missing.
Update
The data properties are getting updated only on init of the component, and when you manually update it (this.data = newData
), they will not be updated when Vuex is updated.
You need to move the brandContacts: this.$store.getters.brandContactsForDropdown
from the data object to the computed object like this:
brandContacts(){ this.$store.state.brandContactsForDropdown }
This will force vue to update when the brandContactsForDropdown
property in vuex updates.
https://vuejs.org/v2/guide/computed.html
I assume you reached one of the Gotchas:
I'm not sure if this is the case where you update the array and it will not detect the change, but the best way to update the array would be to place a new array instead of adding to it:
getBrandContacts({state, commit}, payload) {
Vue.http.get(process.env.VUE_APP_API_SERVER + '/brands/contacts/' +
payload.value).then(response => {
if(response.body.length > 0) {
let newArray =
for (var i = 0; i < response.body.length; i++) {
newArray.push({"name" :
response.body[i].firstname + " " + response.body[i].surname, "value" :
response.body[i].id})
}
commit('pushBrandContact', newArray)
}
}, response => {
// error callback
});
}
and in mutations:
pushBrandContact(state, payload) {
state.brandContactsForDropdown = payload
}
It is also not clear how you work with the data inside the component, is it a data? or in computed? sharing this code will help.
You also say the array doesn't clear, what you mean? it have the old values? in the vuex state? on in your component? a lot of info to help is missing.
Update
The data properties are getting updated only on init of the component, and when you manually update it (this.data = newData
), they will not be updated when Vuex is updated.
You need to move the brandContacts: this.$store.getters.brandContactsForDropdown
from the data object to the computed object like this:
brandContacts(){ this.$store.state.brandContactsForDropdown }
This will force vue to update when the brandContactsForDropdown
property in vuex updates.
https://vuejs.org/v2/guide/computed.html
edited Nov 16 '18 at 15:33
answered Nov 16 '18 at 14:48
Art3mixArt3mix
364110
364110
The last line is in the data() of my component. What I mean with the array doesn't clear is that I push contacts to the array, but when a new brand is selected, I want to clear the array to make room for the new contacts of the new brand. The Vuex state should just be cleared, but it seems like it isn't.
– Danoctum
Nov 16 '18 at 14:58
have you tried to replace the whole array as in my answer? did it have the same effect? have you tried moving the line to the computed and set it like this:brandContacts: this.$store.state.brandContactsForDropdown
– Art3mix
Nov 16 '18 at 15:01
I just tried, It seems like the mutation just doesn't want to work.
– Danoctum
Nov 16 '18 at 15:03
The result is just an empty array, like it's initialized.
– Danoctum
Nov 16 '18 at 15:03
how do you know the array is empty? where do you see it?
– Art3mix
Nov 16 '18 at 15:04
|
show 8 more comments
The last line is in the data() of my component. What I mean with the array doesn't clear is that I push contacts to the array, but when a new brand is selected, I want to clear the array to make room for the new contacts of the new brand. The Vuex state should just be cleared, but it seems like it isn't.
– Danoctum
Nov 16 '18 at 14:58
have you tried to replace the whole array as in my answer? did it have the same effect? have you tried moving the line to the computed and set it like this:brandContacts: this.$store.state.brandContactsForDropdown
– Art3mix
Nov 16 '18 at 15:01
I just tried, It seems like the mutation just doesn't want to work.
– Danoctum
Nov 16 '18 at 15:03
The result is just an empty array, like it's initialized.
– Danoctum
Nov 16 '18 at 15:03
how do you know the array is empty? where do you see it?
– Art3mix
Nov 16 '18 at 15:04
The last line is in the data() of my component. What I mean with the array doesn't clear is that I push contacts to the array, but when a new brand is selected, I want to clear the array to make room for the new contacts of the new brand. The Vuex state should just be cleared, but it seems like it isn't.
– Danoctum
Nov 16 '18 at 14:58
The last line is in the data() of my component. What I mean with the array doesn't clear is that I push contacts to the array, but when a new brand is selected, I want to clear the array to make room for the new contacts of the new brand. The Vuex state should just be cleared, but it seems like it isn't.
– Danoctum
Nov 16 '18 at 14:58
have you tried to replace the whole array as in my answer? did it have the same effect? have you tried moving the line to the computed and set it like this:
brandContacts: this.$store.state.brandContactsForDropdown
– Art3mix
Nov 16 '18 at 15:01
have you tried to replace the whole array as in my answer? did it have the same effect? have you tried moving the line to the computed and set it like this:
brandContacts: this.$store.state.brandContactsForDropdown
– Art3mix
Nov 16 '18 at 15:01
I just tried, It seems like the mutation just doesn't want to work.
– Danoctum
Nov 16 '18 at 15:03
I just tried, It seems like the mutation just doesn't want to work.
– Danoctum
Nov 16 '18 at 15:03
The result is just an empty array, like it's initialized.
– Danoctum
Nov 16 '18 at 15:03
The result is just an empty array, like it's initialized.
– Danoctum
Nov 16 '18 at 15:03
how do you know the array is empty? where do you see it?
– Art3mix
Nov 16 '18 at 15:04
how do you know the array is empty? where do you see it?
– Art3mix
Nov 16 '18 at 15:04
|
show 8 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53339991%2fvuex-mutating-a-state-variable-not-working%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
hZBUA,9B,pn1ckb oNYkfSwc3Aw9gdtz Qbt50j4TzycA98zXXj2FPV W19z wYpU8f7gwtthf1fzVVd,VxzWlzqWZjGjPc
Is that last line in the
data
method of a component? Because if so,brandContacts
is only getting set once on the component's initialization, and you would need to makebrandContacts
a computed property instead in order for it to reflect any changes to the value ofthis.$store.getters.brandContactsForDropdown
.– thanksd
Nov 16 '18 at 14:46
The last line is in the data method of my Vue component. It's weird how another value I set IS updated when it changes in Vuex...
– Danoctum
Nov 16 '18 at 14:53
Yeah, without seeing that code it's hard to know what would cause that.
– thanksd
Nov 16 '18 at 15:01
Added the code, hope you can find what's wrong :)
– Danoctum
Nov 16 '18 at 15:14
So I'm assuming the
brands
property is the one you said is getting updated when it changes in vuex. But where is that code?– thanksd
Nov 16 '18 at 15:20