Springboot - convert JSON to JAVA
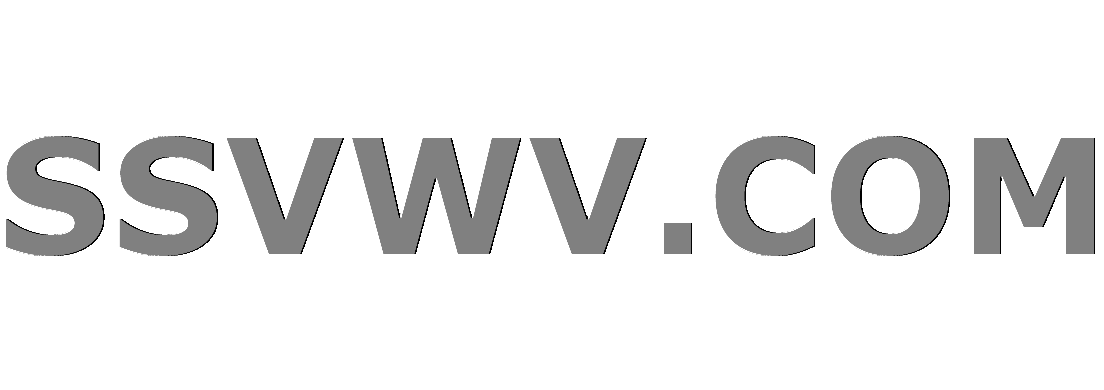
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I need to obtain a currency exchange rate from a rest API into my spring boot application and convert the JSON response to Java class. The API will give me the response as below:-
{
"USD_INR": {
"val": 71.884966
}
}
The code is as shown below:
@RestController
public class MainController {
@Autowired
private RestTemplate restTemplate;
@GetMapping("/hello")
public float hello(){
ExchangeRate exchangeRate = restTemplate.getForObject("https://free.currencyconverterapi.com/api/v6/convert?q=Source_Target&compact=y",ExchangeRate.class);
return exchangeRate.getSource_Target().getVal();
}
}
-
@JsonIgnoreProperties(ignoreUnknown = true)
public class ExchangeRate {
Source_Target SourceTargetObject;
public Source_Target getSource_Target() {
return SourceTargetObject;
}
public void setSource_Target(Source_Target SourceTargetObject) {
this.SourceTargetObject = SourceTargetObject;
}
}
@JsonIgnoreProperties(ignoreUnknown = true)
public class Source_Target {
private float val;
public float getVal() {
return val;
}
public void setVal(float val) {
this.val = val;
}
}
After executing the REST call, I am getting null exception. Nothing is returned. I am not able to figure out the issue here. Please help
json spring-boot resttemplate
add a comment |
I need to obtain a currency exchange rate from a rest API into my spring boot application and convert the JSON response to Java class. The API will give me the response as below:-
{
"USD_INR": {
"val": 71.884966
}
}
The code is as shown below:
@RestController
public class MainController {
@Autowired
private RestTemplate restTemplate;
@GetMapping("/hello")
public float hello(){
ExchangeRate exchangeRate = restTemplate.getForObject("https://free.currencyconverterapi.com/api/v6/convert?q=Source_Target&compact=y",ExchangeRate.class);
return exchangeRate.getSource_Target().getVal();
}
}
-
@JsonIgnoreProperties(ignoreUnknown = true)
public class ExchangeRate {
Source_Target SourceTargetObject;
public Source_Target getSource_Target() {
return SourceTargetObject;
}
public void setSource_Target(Source_Target SourceTargetObject) {
this.SourceTargetObject = SourceTargetObject;
}
}
@JsonIgnoreProperties(ignoreUnknown = true)
public class Source_Target {
private float val;
public float getVal() {
return val;
}
public void setVal(float val) {
this.val = val;
}
}
After executing the REST call, I am getting null exception. Nothing is returned. I am not able to figure out the issue here. Please help
json spring-boot resttemplate
can you post the exactNullPointerException
stack trace to your question?
– rieckpil
Nov 16 '18 at 17:19
add a comment |
I need to obtain a currency exchange rate from a rest API into my spring boot application and convert the JSON response to Java class. The API will give me the response as below:-
{
"USD_INR": {
"val": 71.884966
}
}
The code is as shown below:
@RestController
public class MainController {
@Autowired
private RestTemplate restTemplate;
@GetMapping("/hello")
public float hello(){
ExchangeRate exchangeRate = restTemplate.getForObject("https://free.currencyconverterapi.com/api/v6/convert?q=Source_Target&compact=y",ExchangeRate.class);
return exchangeRate.getSource_Target().getVal();
}
}
-
@JsonIgnoreProperties(ignoreUnknown = true)
public class ExchangeRate {
Source_Target SourceTargetObject;
public Source_Target getSource_Target() {
return SourceTargetObject;
}
public void setSource_Target(Source_Target SourceTargetObject) {
this.SourceTargetObject = SourceTargetObject;
}
}
@JsonIgnoreProperties(ignoreUnknown = true)
public class Source_Target {
private float val;
public float getVal() {
return val;
}
public void setVal(float val) {
this.val = val;
}
}
After executing the REST call, I am getting null exception. Nothing is returned. I am not able to figure out the issue here. Please help
json spring-boot resttemplate
I need to obtain a currency exchange rate from a rest API into my spring boot application and convert the JSON response to Java class. The API will give me the response as below:-
{
"USD_INR": {
"val": 71.884966
}
}
The code is as shown below:
@RestController
public class MainController {
@Autowired
private RestTemplate restTemplate;
@GetMapping("/hello")
public float hello(){
ExchangeRate exchangeRate = restTemplate.getForObject("https://free.currencyconverterapi.com/api/v6/convert?q=Source_Target&compact=y",ExchangeRate.class);
return exchangeRate.getSource_Target().getVal();
}
}
-
@JsonIgnoreProperties(ignoreUnknown = true)
public class ExchangeRate {
Source_Target SourceTargetObject;
public Source_Target getSource_Target() {
return SourceTargetObject;
}
public void setSource_Target(Source_Target SourceTargetObject) {
this.SourceTargetObject = SourceTargetObject;
}
}
@JsonIgnoreProperties(ignoreUnknown = true)
public class Source_Target {
private float val;
public float getVal() {
return val;
}
public void setVal(float val) {
this.val = val;
}
}
After executing the REST call, I am getting null exception. Nothing is returned. I am not able to figure out the issue here. Please help
json spring-boot resttemplate
json spring-boot resttemplate
edited Nov 16 '18 at 23:00


rieckpil
1,3861720
1,3861720
asked Nov 16 '18 at 16:40
Albie AntonyAlbie Antony
111
111
can you post the exactNullPointerException
stack trace to your question?
– rieckpil
Nov 16 '18 at 17:19
add a comment |
can you post the exactNullPointerException
stack trace to your question?
– rieckpil
Nov 16 '18 at 17:19
can you post the exact
NullPointerException
stack trace to your question?– rieckpil
Nov 16 '18 at 17:19
can you post the exact
NullPointerException
stack trace to your question?– rieckpil
Nov 16 '18 at 17:19
add a comment |
3 Answers
3
active
oldest
votes
You can use jsonscheme2pojo for generate java beans from json easily.
JSON:
{
"USD_INR": {
"val": 71.884966
}
}
Corresponding Java bean :
-----------------------------------com.example.Example.java-----------------------------------
package com.example;
import java.io.Serializable;
import java.util.HashMap;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"USD_INR"
})
public class Example implements Serializable
{
@JsonProperty("USD_INR")
private USDINR uSDINR;
@JsonIgnore
private Map<String, Object> additionalProperties = new HashMap<String, Object>();
private final static long serialVersionUID = -932753391825750904L;
@JsonProperty("USD_INR")
public USDINR getUSDINR() {
return uSDINR;
}
@JsonProperty("USD_INR")
public void setUSDINR(USDINR uSDINR) {
this.uSDINR = uSDINR;
}
public Example withUSDINR(USDINR uSDINR) {
this.uSDINR = uSDINR;
return this;
}
@JsonAnyGetter
public Map<String, Object> getAdditionalProperties() {
return this.additionalProperties;
}
@JsonAnySetter
public void setAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
}
public Example withAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
return this;
}
}
-----------------------------------com.example.USDINR.java-----------------------------------
package com.example;
import java.io.Serializable;
import java.util.HashMap;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"val"
})
public class USDINR implements Serializable
{
@JsonProperty("val")
private Double val;
@JsonIgnore
private Map<String, Object> additionalProperties = new HashMap<String, Object>();
private final static long serialVersionUID = -6384976748235176082L;
@JsonProperty("val")
public Double getVal() {
return val;
}
@JsonProperty("val")
public void setVal(Double val) {
this.val = val;
}
public USDINR withVal(Double val) {
this.val = val;
return this;
}
@JsonAnyGetter
public Map<String, Object> getAdditionalProperties() {
return this.additionalProperties;
}
@JsonAnySetter
public void setAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
}
public USDINR withAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
return this;
}
}
@JsonProperty("USD_INR") - Can't give like this because the property changes with different Source & target currencies..
– Albie Antony
Nov 17 '18 at 5:05
add a comment |
Your ExchangeRate class should be:
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonIgnoreProperties(ignoreUnknown = true)
public class ExchangeRate {
@JsonProperty("USD_INR")
Source_Target SourceTargetObject;
public Source_Target getSource_Target() {
return SourceTargetObject;
}
public void setSource_Target(Source_Target SourceTargetObject) {
this.SourceTargetObject = SourceTargetObject;
}
Then your Source_Target class should be:
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonIgnoreProperties(ignoreUnknown = true)
public class Source_Target {
@JsonProperty("val")
private float val;
public float getVal() {
return val;
}
public void setVal(float val) {
this.val = val;
}
}
Please beware of your class naming convention. I would rename Source_Target
to SourceTarget
to follow Java naming convention
@JsonProperty("USD_INR") - Can't give like this because the property changes with different Source & target currencies..
– Albie Antony
Nov 17 '18 at 5:05
add a comment |
You create a custom map:
public class ExchangeRate extends HashMap<String, ExchangeRateValue> implements Serializable {
}
public class ExchangeRateValue implements Serializable {
private float val;
public float getVal() {
return val;
}
public void setVal(float val) {
this.val = val;
}
}
And call it:
ExchangeRate exchangeRate = restTemplate.getForObject("https://free.currencyconverterapi.com/api/v6/convert?q=Source_Target&compact=y", ExchangeRate.class);
float data = exchangeRate.get("USD_INR").getVal();
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53342059%2fspringboot-convert-json-to-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can use jsonscheme2pojo for generate java beans from json easily.
JSON:
{
"USD_INR": {
"val": 71.884966
}
}
Corresponding Java bean :
-----------------------------------com.example.Example.java-----------------------------------
package com.example;
import java.io.Serializable;
import java.util.HashMap;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"USD_INR"
})
public class Example implements Serializable
{
@JsonProperty("USD_INR")
private USDINR uSDINR;
@JsonIgnore
private Map<String, Object> additionalProperties = new HashMap<String, Object>();
private final static long serialVersionUID = -932753391825750904L;
@JsonProperty("USD_INR")
public USDINR getUSDINR() {
return uSDINR;
}
@JsonProperty("USD_INR")
public void setUSDINR(USDINR uSDINR) {
this.uSDINR = uSDINR;
}
public Example withUSDINR(USDINR uSDINR) {
this.uSDINR = uSDINR;
return this;
}
@JsonAnyGetter
public Map<String, Object> getAdditionalProperties() {
return this.additionalProperties;
}
@JsonAnySetter
public void setAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
}
public Example withAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
return this;
}
}
-----------------------------------com.example.USDINR.java-----------------------------------
package com.example;
import java.io.Serializable;
import java.util.HashMap;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"val"
})
public class USDINR implements Serializable
{
@JsonProperty("val")
private Double val;
@JsonIgnore
private Map<String, Object> additionalProperties = new HashMap<String, Object>();
private final static long serialVersionUID = -6384976748235176082L;
@JsonProperty("val")
public Double getVal() {
return val;
}
@JsonProperty("val")
public void setVal(Double val) {
this.val = val;
}
public USDINR withVal(Double val) {
this.val = val;
return this;
}
@JsonAnyGetter
public Map<String, Object> getAdditionalProperties() {
return this.additionalProperties;
}
@JsonAnySetter
public void setAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
}
public USDINR withAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
return this;
}
}
@JsonProperty("USD_INR") - Can't give like this because the property changes with different Source & target currencies..
– Albie Antony
Nov 17 '18 at 5:05
add a comment |
You can use jsonscheme2pojo for generate java beans from json easily.
JSON:
{
"USD_INR": {
"val": 71.884966
}
}
Corresponding Java bean :
-----------------------------------com.example.Example.java-----------------------------------
package com.example;
import java.io.Serializable;
import java.util.HashMap;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"USD_INR"
})
public class Example implements Serializable
{
@JsonProperty("USD_INR")
private USDINR uSDINR;
@JsonIgnore
private Map<String, Object> additionalProperties = new HashMap<String, Object>();
private final static long serialVersionUID = -932753391825750904L;
@JsonProperty("USD_INR")
public USDINR getUSDINR() {
return uSDINR;
}
@JsonProperty("USD_INR")
public void setUSDINR(USDINR uSDINR) {
this.uSDINR = uSDINR;
}
public Example withUSDINR(USDINR uSDINR) {
this.uSDINR = uSDINR;
return this;
}
@JsonAnyGetter
public Map<String, Object> getAdditionalProperties() {
return this.additionalProperties;
}
@JsonAnySetter
public void setAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
}
public Example withAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
return this;
}
}
-----------------------------------com.example.USDINR.java-----------------------------------
package com.example;
import java.io.Serializable;
import java.util.HashMap;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"val"
})
public class USDINR implements Serializable
{
@JsonProperty("val")
private Double val;
@JsonIgnore
private Map<String, Object> additionalProperties = new HashMap<String, Object>();
private final static long serialVersionUID = -6384976748235176082L;
@JsonProperty("val")
public Double getVal() {
return val;
}
@JsonProperty("val")
public void setVal(Double val) {
this.val = val;
}
public USDINR withVal(Double val) {
this.val = val;
return this;
}
@JsonAnyGetter
public Map<String, Object> getAdditionalProperties() {
return this.additionalProperties;
}
@JsonAnySetter
public void setAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
}
public USDINR withAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
return this;
}
}
@JsonProperty("USD_INR") - Can't give like this because the property changes with different Source & target currencies..
– Albie Antony
Nov 17 '18 at 5:05
add a comment |
You can use jsonscheme2pojo for generate java beans from json easily.
JSON:
{
"USD_INR": {
"val": 71.884966
}
}
Corresponding Java bean :
-----------------------------------com.example.Example.java-----------------------------------
package com.example;
import java.io.Serializable;
import java.util.HashMap;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"USD_INR"
})
public class Example implements Serializable
{
@JsonProperty("USD_INR")
private USDINR uSDINR;
@JsonIgnore
private Map<String, Object> additionalProperties = new HashMap<String, Object>();
private final static long serialVersionUID = -932753391825750904L;
@JsonProperty("USD_INR")
public USDINR getUSDINR() {
return uSDINR;
}
@JsonProperty("USD_INR")
public void setUSDINR(USDINR uSDINR) {
this.uSDINR = uSDINR;
}
public Example withUSDINR(USDINR uSDINR) {
this.uSDINR = uSDINR;
return this;
}
@JsonAnyGetter
public Map<String, Object> getAdditionalProperties() {
return this.additionalProperties;
}
@JsonAnySetter
public void setAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
}
public Example withAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
return this;
}
}
-----------------------------------com.example.USDINR.java-----------------------------------
package com.example;
import java.io.Serializable;
import java.util.HashMap;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"val"
})
public class USDINR implements Serializable
{
@JsonProperty("val")
private Double val;
@JsonIgnore
private Map<String, Object> additionalProperties = new HashMap<String, Object>();
private final static long serialVersionUID = -6384976748235176082L;
@JsonProperty("val")
public Double getVal() {
return val;
}
@JsonProperty("val")
public void setVal(Double val) {
this.val = val;
}
public USDINR withVal(Double val) {
this.val = val;
return this;
}
@JsonAnyGetter
public Map<String, Object> getAdditionalProperties() {
return this.additionalProperties;
}
@JsonAnySetter
public void setAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
}
public USDINR withAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
return this;
}
}
You can use jsonscheme2pojo for generate java beans from json easily.
JSON:
{
"USD_INR": {
"val": 71.884966
}
}
Corresponding Java bean :
-----------------------------------com.example.Example.java-----------------------------------
package com.example;
import java.io.Serializable;
import java.util.HashMap;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"USD_INR"
})
public class Example implements Serializable
{
@JsonProperty("USD_INR")
private USDINR uSDINR;
@JsonIgnore
private Map<String, Object> additionalProperties = new HashMap<String, Object>();
private final static long serialVersionUID = -932753391825750904L;
@JsonProperty("USD_INR")
public USDINR getUSDINR() {
return uSDINR;
}
@JsonProperty("USD_INR")
public void setUSDINR(USDINR uSDINR) {
this.uSDINR = uSDINR;
}
public Example withUSDINR(USDINR uSDINR) {
this.uSDINR = uSDINR;
return this;
}
@JsonAnyGetter
public Map<String, Object> getAdditionalProperties() {
return this.additionalProperties;
}
@JsonAnySetter
public void setAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
}
public Example withAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
return this;
}
}
-----------------------------------com.example.USDINR.java-----------------------------------
package com.example;
import java.io.Serializable;
import java.util.HashMap;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"val"
})
public class USDINR implements Serializable
{
@JsonProperty("val")
private Double val;
@JsonIgnore
private Map<String, Object> additionalProperties = new HashMap<String, Object>();
private final static long serialVersionUID = -6384976748235176082L;
@JsonProperty("val")
public Double getVal() {
return val;
}
@JsonProperty("val")
public void setVal(Double val) {
this.val = val;
}
public USDINR withVal(Double val) {
this.val = val;
return this;
}
@JsonAnyGetter
public Map<String, Object> getAdditionalProperties() {
return this.additionalProperties;
}
@JsonAnySetter
public void setAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
}
public USDINR withAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
return this;
}
}
answered Nov 16 '18 at 17:43


Samir AgayarovSamir Agayarov
403310
403310
@JsonProperty("USD_INR") - Can't give like this because the property changes with different Source & target currencies..
– Albie Antony
Nov 17 '18 at 5:05
add a comment |
@JsonProperty("USD_INR") - Can't give like this because the property changes with different Source & target currencies..
– Albie Antony
Nov 17 '18 at 5:05
@JsonProperty("USD_INR") - Can't give like this because the property changes with different Source & target currencies..
– Albie Antony
Nov 17 '18 at 5:05
@JsonProperty("USD_INR") - Can't give like this because the property changes with different Source & target currencies..
– Albie Antony
Nov 17 '18 at 5:05
add a comment |
Your ExchangeRate class should be:
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonIgnoreProperties(ignoreUnknown = true)
public class ExchangeRate {
@JsonProperty("USD_INR")
Source_Target SourceTargetObject;
public Source_Target getSource_Target() {
return SourceTargetObject;
}
public void setSource_Target(Source_Target SourceTargetObject) {
this.SourceTargetObject = SourceTargetObject;
}
Then your Source_Target class should be:
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonIgnoreProperties(ignoreUnknown = true)
public class Source_Target {
@JsonProperty("val")
private float val;
public float getVal() {
return val;
}
public void setVal(float val) {
this.val = val;
}
}
Please beware of your class naming convention. I would rename Source_Target
to SourceTarget
to follow Java naming convention
@JsonProperty("USD_INR") - Can't give like this because the property changes with different Source & target currencies..
– Albie Antony
Nov 17 '18 at 5:05
add a comment |
Your ExchangeRate class should be:
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonIgnoreProperties(ignoreUnknown = true)
public class ExchangeRate {
@JsonProperty("USD_INR")
Source_Target SourceTargetObject;
public Source_Target getSource_Target() {
return SourceTargetObject;
}
public void setSource_Target(Source_Target SourceTargetObject) {
this.SourceTargetObject = SourceTargetObject;
}
Then your Source_Target class should be:
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonIgnoreProperties(ignoreUnknown = true)
public class Source_Target {
@JsonProperty("val")
private float val;
public float getVal() {
return val;
}
public void setVal(float val) {
this.val = val;
}
}
Please beware of your class naming convention. I would rename Source_Target
to SourceTarget
to follow Java naming convention
@JsonProperty("USD_INR") - Can't give like this because the property changes with different Source & target currencies..
– Albie Antony
Nov 17 '18 at 5:05
add a comment |
Your ExchangeRate class should be:
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonIgnoreProperties(ignoreUnknown = true)
public class ExchangeRate {
@JsonProperty("USD_INR")
Source_Target SourceTargetObject;
public Source_Target getSource_Target() {
return SourceTargetObject;
}
public void setSource_Target(Source_Target SourceTargetObject) {
this.SourceTargetObject = SourceTargetObject;
}
Then your Source_Target class should be:
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonIgnoreProperties(ignoreUnknown = true)
public class Source_Target {
@JsonProperty("val")
private float val;
public float getVal() {
return val;
}
public void setVal(float val) {
this.val = val;
}
}
Please beware of your class naming convention. I would rename Source_Target
to SourceTarget
to follow Java naming convention
Your ExchangeRate class should be:
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonIgnoreProperties(ignoreUnknown = true)
public class ExchangeRate {
@JsonProperty("USD_INR")
Source_Target SourceTargetObject;
public Source_Target getSource_Target() {
return SourceTargetObject;
}
public void setSource_Target(Source_Target SourceTargetObject) {
this.SourceTargetObject = SourceTargetObject;
}
Then your Source_Target class should be:
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonIgnoreProperties(ignoreUnknown = true)
public class Source_Target {
@JsonProperty("val")
private float val;
public float getVal() {
return val;
}
public void setVal(float val) {
this.val = val;
}
}
Please beware of your class naming convention. I would rename Source_Target
to SourceTarget
to follow Java naming convention
answered Nov 16 '18 at 17:47
Atika RAtika R
274
274
@JsonProperty("USD_INR") - Can't give like this because the property changes with different Source & target currencies..
– Albie Antony
Nov 17 '18 at 5:05
add a comment |
@JsonProperty("USD_INR") - Can't give like this because the property changes with different Source & target currencies..
– Albie Antony
Nov 17 '18 at 5:05
@JsonProperty("USD_INR") - Can't give like this because the property changes with different Source & target currencies..
– Albie Antony
Nov 17 '18 at 5:05
@JsonProperty("USD_INR") - Can't give like this because the property changes with different Source & target currencies..
– Albie Antony
Nov 17 '18 at 5:05
add a comment |
You create a custom map:
public class ExchangeRate extends HashMap<String, ExchangeRateValue> implements Serializable {
}
public class ExchangeRateValue implements Serializable {
private float val;
public float getVal() {
return val;
}
public void setVal(float val) {
this.val = val;
}
}
And call it:
ExchangeRate exchangeRate = restTemplate.getForObject("https://free.currencyconverterapi.com/api/v6/convert?q=Source_Target&compact=y", ExchangeRate.class);
float data = exchangeRate.get("USD_INR").getVal();
add a comment |
You create a custom map:
public class ExchangeRate extends HashMap<String, ExchangeRateValue> implements Serializable {
}
public class ExchangeRateValue implements Serializable {
private float val;
public float getVal() {
return val;
}
public void setVal(float val) {
this.val = val;
}
}
And call it:
ExchangeRate exchangeRate = restTemplate.getForObject("https://free.currencyconverterapi.com/api/v6/convert?q=Source_Target&compact=y", ExchangeRate.class);
float data = exchangeRate.get("USD_INR").getVal();
add a comment |
You create a custom map:
public class ExchangeRate extends HashMap<String, ExchangeRateValue> implements Serializable {
}
public class ExchangeRateValue implements Serializable {
private float val;
public float getVal() {
return val;
}
public void setVal(float val) {
this.val = val;
}
}
And call it:
ExchangeRate exchangeRate = restTemplate.getForObject("https://free.currencyconverterapi.com/api/v6/convert?q=Source_Target&compact=y", ExchangeRate.class);
float data = exchangeRate.get("USD_INR").getVal();
You create a custom map:
public class ExchangeRate extends HashMap<String, ExchangeRateValue> implements Serializable {
}
public class ExchangeRateValue implements Serializable {
private float val;
public float getVal() {
return val;
}
public void setVal(float val) {
this.val = val;
}
}
And call it:
ExchangeRate exchangeRate = restTemplate.getForObject("https://free.currencyconverterapi.com/api/v6/convert?q=Source_Target&compact=y", ExchangeRate.class);
float data = exchangeRate.get("USD_INR").getVal();
edited Nov 18 '18 at 6:09
answered Nov 18 '18 at 6:01


Jonathan JohxJonathan Johx
1
1
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53342059%2fspringboot-convert-json-to-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Vz,K ndB cMCRKRl50LfK9BDD,hs,MSzqL YHwud5ULE7euCF3QVhvO
can you post the exact
NullPointerException
stack trace to your question?– rieckpil
Nov 16 '18 at 17:19