Default value in POJO field incase of data type mismatch during JSON deserialization
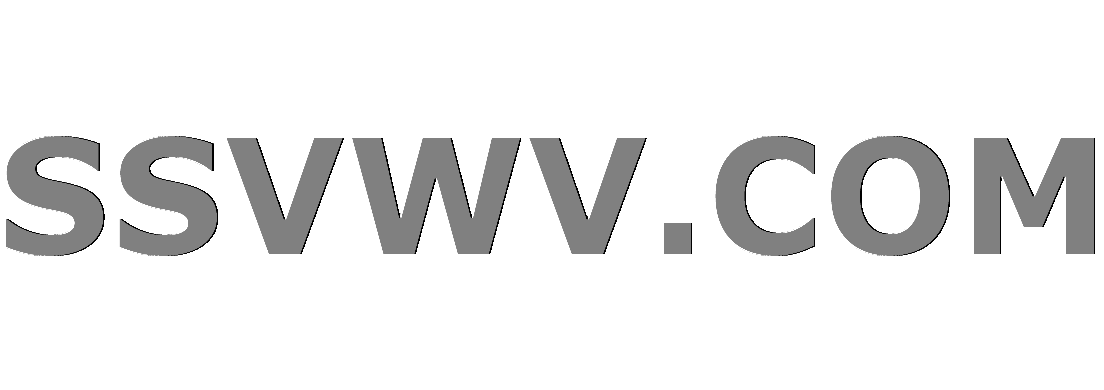
Multi tool use
When we deserialize JSON into POJO, JACKSON throws a Mismatch Input exception in case of data type mismatch, which is expected.
So my question is, "Is there any feature flag available in Jackson or Gson, to set the default value for mismatched field during deserialization?"
I understand that we can implement the custom deserializer for such fields, where random input value can be expected.
But is this already supported using some flag in any serialization deserialization java library?
Sample Code:
import com.fasterxml.jackson.databind.ObjectMapper;
import lombok.Data;
import java.io.IOException;
public class JsonSample {
@Data
static class Address {
String houseAddress;
}
@Data
static class InnerJsonClass {
Integer integerField;
Address address;
}
public static void main(String args) {
String jsonInput = "{"integerField": 1,"address":"hello"}";
try {
ObjectMapper objectMapper = new ObjectMapper();
InnerJsonClass object = objectMapper.readValue(jsonInput, InnerJsonClass.class);
System.out.println(object.toString());
} catch (IOException e) {
e.printStackTrace();
}
}}
Exception:
com.fasterxml.jackson.databind.exc.MismatchedInputException: Cannot construct instance of com.paytm.logistics.JsonSample$Address
(although at least one Creator exists): no String-argument constructor/factory method to deserialize from String value ('hello')
at [Source: (String)"{"integerField": 1,"address":"hello"}"; line: 1, column: 30] (through reference chain: com.paytm.logistics.JsonSample$InnerJsonClass["address"])
at com.fasterxml.jackson.databind.exc.MismatchedInputException.from(MismatchedInputException.java:63)
at com.fasterxml.jackson.databind.DeserializationContext.reportInputMismatch(DeserializationContext.java:1342)
at com.fasterxml.jackson.databind.DeserializationContext.handleMissingInstantiator(DeserializationContext.java:1031)
at com.fasterxml.jackson.databind.deser.ValueInstantiator._createFromStringFallbacks(ValueInstantiator.java:371)
at com.fasterxml.jackson.databind.deser.std.StdValueInstantiator.createFromString(StdValueInstantiator.java:323)
at com.fasterxml.jackson.databind.deser.BeanDeserializerBase.deserializeFromString(BeanDeserializerBase.java:1366)
at com.fasterxml.jackson.databind.deser.BeanDeserializer._deserializeOther(BeanDeserializer.java:171)
at com.fasterxml.jackson.databind.deser.BeanDeserializer.deserialize(BeanDeserializer.java:161)
at com.fasterxml.jackson.databind.deser.impl.MethodProperty.deserializeAndSet(MethodProperty.java:127)
at com.fasterxml.jackson.databind.deser.BeanDeserializer.vanillaDeserialize(BeanDeserializer.java:288)
at com.fasterxml.jackson.databind.deser.BeanDeserializer.deserialize(BeanDeserializer.java:151)
at com.fasterxml.jackson.databind.ObjectMapper._readMapAndClose(ObjectMapper.java:4001)
at com.fasterxml.jackson.databind.ObjectMapper.readValue(ObjectMapper.java:2992)
at com.paytm.logistics.JsonSample.main(JsonSample.java:25)
java json java-8
add a comment |
When we deserialize JSON into POJO, JACKSON throws a Mismatch Input exception in case of data type mismatch, which is expected.
So my question is, "Is there any feature flag available in Jackson or Gson, to set the default value for mismatched field during deserialization?"
I understand that we can implement the custom deserializer for such fields, where random input value can be expected.
But is this already supported using some flag in any serialization deserialization java library?
Sample Code:
import com.fasterxml.jackson.databind.ObjectMapper;
import lombok.Data;
import java.io.IOException;
public class JsonSample {
@Data
static class Address {
String houseAddress;
}
@Data
static class InnerJsonClass {
Integer integerField;
Address address;
}
public static void main(String args) {
String jsonInput = "{"integerField": 1,"address":"hello"}";
try {
ObjectMapper objectMapper = new ObjectMapper();
InnerJsonClass object = objectMapper.readValue(jsonInput, InnerJsonClass.class);
System.out.println(object.toString());
} catch (IOException e) {
e.printStackTrace();
}
}}
Exception:
com.fasterxml.jackson.databind.exc.MismatchedInputException: Cannot construct instance of com.paytm.logistics.JsonSample$Address
(although at least one Creator exists): no String-argument constructor/factory method to deserialize from String value ('hello')
at [Source: (String)"{"integerField": 1,"address":"hello"}"; line: 1, column: 30] (through reference chain: com.paytm.logistics.JsonSample$InnerJsonClass["address"])
at com.fasterxml.jackson.databind.exc.MismatchedInputException.from(MismatchedInputException.java:63)
at com.fasterxml.jackson.databind.DeserializationContext.reportInputMismatch(DeserializationContext.java:1342)
at com.fasterxml.jackson.databind.DeserializationContext.handleMissingInstantiator(DeserializationContext.java:1031)
at com.fasterxml.jackson.databind.deser.ValueInstantiator._createFromStringFallbacks(ValueInstantiator.java:371)
at com.fasterxml.jackson.databind.deser.std.StdValueInstantiator.createFromString(StdValueInstantiator.java:323)
at com.fasterxml.jackson.databind.deser.BeanDeserializerBase.deserializeFromString(BeanDeserializerBase.java:1366)
at com.fasterxml.jackson.databind.deser.BeanDeserializer._deserializeOther(BeanDeserializer.java:171)
at com.fasterxml.jackson.databind.deser.BeanDeserializer.deserialize(BeanDeserializer.java:161)
at com.fasterxml.jackson.databind.deser.impl.MethodProperty.deserializeAndSet(MethodProperty.java:127)
at com.fasterxml.jackson.databind.deser.BeanDeserializer.vanillaDeserialize(BeanDeserializer.java:288)
at com.fasterxml.jackson.databind.deser.BeanDeserializer.deserialize(BeanDeserializer.java:151)
at com.fasterxml.jackson.databind.ObjectMapper._readMapAndClose(ObjectMapper.java:4001)
at com.fasterxml.jackson.databind.ObjectMapper.readValue(ObjectMapper.java:2992)
at com.paytm.logistics.JsonSample.main(JsonSample.java:25)
java json java-8
I'm not sure this exists but you could try to set the default value in the POJO's constructor/initializer so that if Jackson doesn't set that value you get the default. Then you'd need to tell the parser to ignore properties that don't match - I'm not sure there's such a feature in Jackson or any other library though.
– Thomas
Nov 16 '18 at 10:56
Alternatively you might be able to provide a custom yet generic deserializer, i.e. one that delegates to the standard deserializers but handles the exceptions. If that's possible (it's just an idea and I'm not able to verify it atm) you should be able to provide information on the default value via some custom annotation as well.
– Thomas
Nov 16 '18 at 10:59
@Thomas, Yes, that will be the next step, which is implementing my own generic custom deserializer.
– Yogesh
Nov 16 '18 at 11:01
A third thing you could try might be to use@JsonTypeInfo
along with a custom resolver. That way you might able to tell Jackson that you could either have anAddress
or aString
and in the latter case "resolve" the string to some default value or an address with the string parsed ashouseAddress
- again the disclaimer: that's just an idea and would need to be tested or verified.
– Thomas
Nov 16 '18 at 11:03
@Thomas, I will try and get back on this. Thanks!
– Yogesh
Nov 16 '18 at 11:07
add a comment |
When we deserialize JSON into POJO, JACKSON throws a Mismatch Input exception in case of data type mismatch, which is expected.
So my question is, "Is there any feature flag available in Jackson or Gson, to set the default value for mismatched field during deserialization?"
I understand that we can implement the custom deserializer for such fields, where random input value can be expected.
But is this already supported using some flag in any serialization deserialization java library?
Sample Code:
import com.fasterxml.jackson.databind.ObjectMapper;
import lombok.Data;
import java.io.IOException;
public class JsonSample {
@Data
static class Address {
String houseAddress;
}
@Data
static class InnerJsonClass {
Integer integerField;
Address address;
}
public static void main(String args) {
String jsonInput = "{"integerField": 1,"address":"hello"}";
try {
ObjectMapper objectMapper = new ObjectMapper();
InnerJsonClass object = objectMapper.readValue(jsonInput, InnerJsonClass.class);
System.out.println(object.toString());
} catch (IOException e) {
e.printStackTrace();
}
}}
Exception:
com.fasterxml.jackson.databind.exc.MismatchedInputException: Cannot construct instance of com.paytm.logistics.JsonSample$Address
(although at least one Creator exists): no String-argument constructor/factory method to deserialize from String value ('hello')
at [Source: (String)"{"integerField": 1,"address":"hello"}"; line: 1, column: 30] (through reference chain: com.paytm.logistics.JsonSample$InnerJsonClass["address"])
at com.fasterxml.jackson.databind.exc.MismatchedInputException.from(MismatchedInputException.java:63)
at com.fasterxml.jackson.databind.DeserializationContext.reportInputMismatch(DeserializationContext.java:1342)
at com.fasterxml.jackson.databind.DeserializationContext.handleMissingInstantiator(DeserializationContext.java:1031)
at com.fasterxml.jackson.databind.deser.ValueInstantiator._createFromStringFallbacks(ValueInstantiator.java:371)
at com.fasterxml.jackson.databind.deser.std.StdValueInstantiator.createFromString(StdValueInstantiator.java:323)
at com.fasterxml.jackson.databind.deser.BeanDeserializerBase.deserializeFromString(BeanDeserializerBase.java:1366)
at com.fasterxml.jackson.databind.deser.BeanDeserializer._deserializeOther(BeanDeserializer.java:171)
at com.fasterxml.jackson.databind.deser.BeanDeserializer.deserialize(BeanDeserializer.java:161)
at com.fasterxml.jackson.databind.deser.impl.MethodProperty.deserializeAndSet(MethodProperty.java:127)
at com.fasterxml.jackson.databind.deser.BeanDeserializer.vanillaDeserialize(BeanDeserializer.java:288)
at com.fasterxml.jackson.databind.deser.BeanDeserializer.deserialize(BeanDeserializer.java:151)
at com.fasterxml.jackson.databind.ObjectMapper._readMapAndClose(ObjectMapper.java:4001)
at com.fasterxml.jackson.databind.ObjectMapper.readValue(ObjectMapper.java:2992)
at com.paytm.logistics.JsonSample.main(JsonSample.java:25)
java json java-8
When we deserialize JSON into POJO, JACKSON throws a Mismatch Input exception in case of data type mismatch, which is expected.
So my question is, "Is there any feature flag available in Jackson or Gson, to set the default value for mismatched field during deserialization?"
I understand that we can implement the custom deserializer for such fields, where random input value can be expected.
But is this already supported using some flag in any serialization deserialization java library?
Sample Code:
import com.fasterxml.jackson.databind.ObjectMapper;
import lombok.Data;
import java.io.IOException;
public class JsonSample {
@Data
static class Address {
String houseAddress;
}
@Data
static class InnerJsonClass {
Integer integerField;
Address address;
}
public static void main(String args) {
String jsonInput = "{"integerField": 1,"address":"hello"}";
try {
ObjectMapper objectMapper = new ObjectMapper();
InnerJsonClass object = objectMapper.readValue(jsonInput, InnerJsonClass.class);
System.out.println(object.toString());
} catch (IOException e) {
e.printStackTrace();
}
}}
Exception:
com.fasterxml.jackson.databind.exc.MismatchedInputException: Cannot construct instance of com.paytm.logistics.JsonSample$Address
(although at least one Creator exists): no String-argument constructor/factory method to deserialize from String value ('hello')
at [Source: (String)"{"integerField": 1,"address":"hello"}"; line: 1, column: 30] (through reference chain: com.paytm.logistics.JsonSample$InnerJsonClass["address"])
at com.fasterxml.jackson.databind.exc.MismatchedInputException.from(MismatchedInputException.java:63)
at com.fasterxml.jackson.databind.DeserializationContext.reportInputMismatch(DeserializationContext.java:1342)
at com.fasterxml.jackson.databind.DeserializationContext.handleMissingInstantiator(DeserializationContext.java:1031)
at com.fasterxml.jackson.databind.deser.ValueInstantiator._createFromStringFallbacks(ValueInstantiator.java:371)
at com.fasterxml.jackson.databind.deser.std.StdValueInstantiator.createFromString(StdValueInstantiator.java:323)
at com.fasterxml.jackson.databind.deser.BeanDeserializerBase.deserializeFromString(BeanDeserializerBase.java:1366)
at com.fasterxml.jackson.databind.deser.BeanDeserializer._deserializeOther(BeanDeserializer.java:171)
at com.fasterxml.jackson.databind.deser.BeanDeserializer.deserialize(BeanDeserializer.java:161)
at com.fasterxml.jackson.databind.deser.impl.MethodProperty.deserializeAndSet(MethodProperty.java:127)
at com.fasterxml.jackson.databind.deser.BeanDeserializer.vanillaDeserialize(BeanDeserializer.java:288)
at com.fasterxml.jackson.databind.deser.BeanDeserializer.deserialize(BeanDeserializer.java:151)
at com.fasterxml.jackson.databind.ObjectMapper._readMapAndClose(ObjectMapper.java:4001)
at com.fasterxml.jackson.databind.ObjectMapper.readValue(ObjectMapper.java:2992)
at com.paytm.logistics.JsonSample.main(JsonSample.java:25)
java json java-8
java json java-8
asked Nov 16 '18 at 10:48


YogeshYogesh
100111
100111
I'm not sure this exists but you could try to set the default value in the POJO's constructor/initializer so that if Jackson doesn't set that value you get the default. Then you'd need to tell the parser to ignore properties that don't match - I'm not sure there's such a feature in Jackson or any other library though.
– Thomas
Nov 16 '18 at 10:56
Alternatively you might be able to provide a custom yet generic deserializer, i.e. one that delegates to the standard deserializers but handles the exceptions. If that's possible (it's just an idea and I'm not able to verify it atm) you should be able to provide information on the default value via some custom annotation as well.
– Thomas
Nov 16 '18 at 10:59
@Thomas, Yes, that will be the next step, which is implementing my own generic custom deserializer.
– Yogesh
Nov 16 '18 at 11:01
A third thing you could try might be to use@JsonTypeInfo
along with a custom resolver. That way you might able to tell Jackson that you could either have anAddress
or aString
and in the latter case "resolve" the string to some default value or an address with the string parsed ashouseAddress
- again the disclaimer: that's just an idea and would need to be tested or verified.
– Thomas
Nov 16 '18 at 11:03
@Thomas, I will try and get back on this. Thanks!
– Yogesh
Nov 16 '18 at 11:07
add a comment |
I'm not sure this exists but you could try to set the default value in the POJO's constructor/initializer so that if Jackson doesn't set that value you get the default. Then you'd need to tell the parser to ignore properties that don't match - I'm not sure there's such a feature in Jackson or any other library though.
– Thomas
Nov 16 '18 at 10:56
Alternatively you might be able to provide a custom yet generic deserializer, i.e. one that delegates to the standard deserializers but handles the exceptions. If that's possible (it's just an idea and I'm not able to verify it atm) you should be able to provide information on the default value via some custom annotation as well.
– Thomas
Nov 16 '18 at 10:59
@Thomas, Yes, that will be the next step, which is implementing my own generic custom deserializer.
– Yogesh
Nov 16 '18 at 11:01
A third thing you could try might be to use@JsonTypeInfo
along with a custom resolver. That way you might able to tell Jackson that you could either have anAddress
or aString
and in the latter case "resolve" the string to some default value or an address with the string parsed ashouseAddress
- again the disclaimer: that's just an idea and would need to be tested or verified.
– Thomas
Nov 16 '18 at 11:03
@Thomas, I will try and get back on this. Thanks!
– Yogesh
Nov 16 '18 at 11:07
I'm not sure this exists but you could try to set the default value in the POJO's constructor/initializer so that if Jackson doesn't set that value you get the default. Then you'd need to tell the parser to ignore properties that don't match - I'm not sure there's such a feature in Jackson or any other library though.
– Thomas
Nov 16 '18 at 10:56
I'm not sure this exists but you could try to set the default value in the POJO's constructor/initializer so that if Jackson doesn't set that value you get the default. Then you'd need to tell the parser to ignore properties that don't match - I'm not sure there's such a feature in Jackson or any other library though.
– Thomas
Nov 16 '18 at 10:56
Alternatively you might be able to provide a custom yet generic deserializer, i.e. one that delegates to the standard deserializers but handles the exceptions. If that's possible (it's just an idea and I'm not able to verify it atm) you should be able to provide information on the default value via some custom annotation as well.
– Thomas
Nov 16 '18 at 10:59
Alternatively you might be able to provide a custom yet generic deserializer, i.e. one that delegates to the standard deserializers but handles the exceptions. If that's possible (it's just an idea and I'm not able to verify it atm) you should be able to provide information on the default value via some custom annotation as well.
– Thomas
Nov 16 '18 at 10:59
@Thomas, Yes, that will be the next step, which is implementing my own generic custom deserializer.
– Yogesh
Nov 16 '18 at 11:01
@Thomas, Yes, that will be the next step, which is implementing my own generic custom deserializer.
– Yogesh
Nov 16 '18 at 11:01
A third thing you could try might be to use
@JsonTypeInfo
along with a custom resolver. That way you might able to tell Jackson that you could either have an Address
or a String
and in the latter case "resolve" the string to some default value or an address with the string parsed as houseAddress
- again the disclaimer: that's just an idea and would need to be tested or verified.– Thomas
Nov 16 '18 at 11:03
A third thing you could try might be to use
@JsonTypeInfo
along with a custom resolver. That way you might able to tell Jackson that you could either have an Address
or a String
and in the latter case "resolve" the string to some default value or an address with the string parsed as houseAddress
- again the disclaimer: that's just an idea and would need to be tested or verified.– Thomas
Nov 16 '18 at 11:03
@Thomas, I will try and get back on this. Thanks!
– Yogesh
Nov 16 '18 at 11:07
@Thomas, I will try and get back on this. Thanks!
– Yogesh
Nov 16 '18 at 11:07
add a comment |
2 Answers
2
active
oldest
votes
Address is a object, not a String.
String jsonInput = "{"integerField": 1,"address":{"houseAddress": "hello"}}";
Try this
You missed the point: the OP wants to handle the case where the caller supplies a string instead of an object, i.e. use a default address or null then.
– Thomas
Nov 16 '18 at 10:55
add a comment |
Your jsoninput is not in correct format it should be like below:
objectMapper will only convert value in specified object when json and pojo declaration mapping are same.
String jsonInput = "{"integerField": 1,"address":{"houseAddress": "hello"}}";
or if you don't want to change string json then you have to use code like below
@Data
static class InnerJsonClass {
Integer integerField;
String address;
}
You missed the point: the OP wants to handle the case where the caller supplies a string instead of an object, i.e. use a default address or null then.
– Thomas
Nov 16 '18 at 10:56
add a comment |
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53336315%2fdefault-value-in-pojo-field-incase-of-data-type-mismatch-during-json-deserializa%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Address is a object, not a String.
String jsonInput = "{"integerField": 1,"address":{"houseAddress": "hello"}}";
Try this
You missed the point: the OP wants to handle the case where the caller supplies a string instead of an object, i.e. use a default address or null then.
– Thomas
Nov 16 '18 at 10:55
add a comment |
Address is a object, not a String.
String jsonInput = "{"integerField": 1,"address":{"houseAddress": "hello"}}";
Try this
You missed the point: the OP wants to handle the case where the caller supplies a string instead of an object, i.e. use a default address or null then.
– Thomas
Nov 16 '18 at 10:55
add a comment |
Address is a object, not a String.
String jsonInput = "{"integerField": 1,"address":{"houseAddress": "hello"}}";
Try this
Address is a object, not a String.
String jsonInput = "{"integerField": 1,"address":{"houseAddress": "hello"}}";
Try this
answered Nov 16 '18 at 10:53
darshakatdarshakat
391111
391111
You missed the point: the OP wants to handle the case where the caller supplies a string instead of an object, i.e. use a default address or null then.
– Thomas
Nov 16 '18 at 10:55
add a comment |
You missed the point: the OP wants to handle the case where the caller supplies a string instead of an object, i.e. use a default address or null then.
– Thomas
Nov 16 '18 at 10:55
You missed the point: the OP wants to handle the case where the caller supplies a string instead of an object, i.e. use a default address or null then.
– Thomas
Nov 16 '18 at 10:55
You missed the point: the OP wants to handle the case where the caller supplies a string instead of an object, i.e. use a default address or null then.
– Thomas
Nov 16 '18 at 10:55
add a comment |
Your jsoninput is not in correct format it should be like below:
objectMapper will only convert value in specified object when json and pojo declaration mapping are same.
String jsonInput = "{"integerField": 1,"address":{"houseAddress": "hello"}}";
or if you don't want to change string json then you have to use code like below
@Data
static class InnerJsonClass {
Integer integerField;
String address;
}
You missed the point: the OP wants to handle the case where the caller supplies a string instead of an object, i.e. use a default address or null then.
– Thomas
Nov 16 '18 at 10:56
add a comment |
Your jsoninput is not in correct format it should be like below:
objectMapper will only convert value in specified object when json and pojo declaration mapping are same.
String jsonInput = "{"integerField": 1,"address":{"houseAddress": "hello"}}";
or if you don't want to change string json then you have to use code like below
@Data
static class InnerJsonClass {
Integer integerField;
String address;
}
You missed the point: the OP wants to handle the case where the caller supplies a string instead of an object, i.e. use a default address or null then.
– Thomas
Nov 16 '18 at 10:56
add a comment |
Your jsoninput is not in correct format it should be like below:
objectMapper will only convert value in specified object when json and pojo declaration mapping are same.
String jsonInput = "{"integerField": 1,"address":{"houseAddress": "hello"}}";
or if you don't want to change string json then you have to use code like below
@Data
static class InnerJsonClass {
Integer integerField;
String address;
}
Your jsoninput is not in correct format it should be like below:
objectMapper will only convert value in specified object when json and pojo declaration mapping are same.
String jsonInput = "{"integerField": 1,"address":{"houseAddress": "hello"}}";
or if you don't want to change string json then you have to use code like below
@Data
static class InnerJsonClass {
Integer integerField;
String address;
}
edited Nov 16 '18 at 10:56
answered Nov 16 '18 at 10:55
GauravRai1512GauravRai1512
629112
629112
You missed the point: the OP wants to handle the case where the caller supplies a string instead of an object, i.e. use a default address or null then.
– Thomas
Nov 16 '18 at 10:56
add a comment |
You missed the point: the OP wants to handle the case where the caller supplies a string instead of an object, i.e. use a default address or null then.
– Thomas
Nov 16 '18 at 10:56
You missed the point: the OP wants to handle the case where the caller supplies a string instead of an object, i.e. use a default address or null then.
– Thomas
Nov 16 '18 at 10:56
You missed the point: the OP wants to handle the case where the caller supplies a string instead of an object, i.e. use a default address or null then.
– Thomas
Nov 16 '18 at 10:56
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53336315%2fdefault-value-in-pojo-field-incase-of-data-type-mismatch-during-json-deserializa%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
pQ Lpny5 UBW1,AvpgNIDPbtUTR2HuXg7v8,z417Id9M9K ijZ
I'm not sure this exists but you could try to set the default value in the POJO's constructor/initializer so that if Jackson doesn't set that value you get the default. Then you'd need to tell the parser to ignore properties that don't match - I'm not sure there's such a feature in Jackson or any other library though.
– Thomas
Nov 16 '18 at 10:56
Alternatively you might be able to provide a custom yet generic deserializer, i.e. one that delegates to the standard deserializers but handles the exceptions. If that's possible (it's just an idea and I'm not able to verify it atm) you should be able to provide information on the default value via some custom annotation as well.
– Thomas
Nov 16 '18 at 10:59
@Thomas, Yes, that will be the next step, which is implementing my own generic custom deserializer.
– Yogesh
Nov 16 '18 at 11:01
A third thing you could try might be to use
@JsonTypeInfo
along with a custom resolver. That way you might able to tell Jackson that you could either have anAddress
or aString
and in the latter case "resolve" the string to some default value or an address with the string parsed ashouseAddress
- again the disclaimer: that's just an idea and would need to be tested or verified.– Thomas
Nov 16 '18 at 11:03
@Thomas, I will try and get back on this. Thanks!
– Yogesh
Nov 16 '18 at 11:07