update object property without rewriting
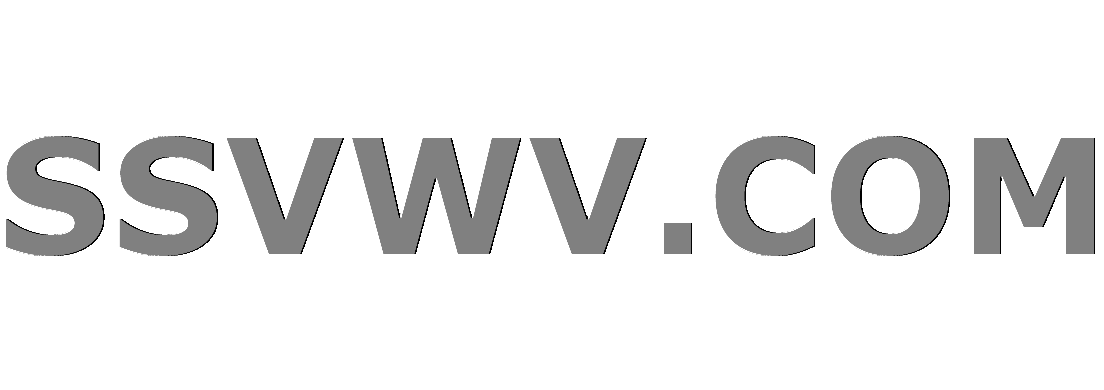
Multi tool use
I have a method which takes two parameters key
for property name and value
for the property value. And when I use it I want to update data[key]
and add as many values there as I call the method setData. I need not rewrite value with the same key
, but update it and add a new one, so the final object can look like this:
{
patients:
formValue: […],
filtersValue: {}
}
current realization:
const data = {}
setData(key, value) {
Object.assign(this.data[key] = {}, value);
}
setData(this.stateName, { firstItemsValue: this.itemsCopy });
setData(this.stateName, { secondItemsValue: this.itemsCopy });
javascript object
add a comment |
I have a method which takes two parameters key
for property name and value
for the property value. And when I use it I want to update data[key]
and add as many values there as I call the method setData. I need not rewrite value with the same key
, but update it and add a new one, so the final object can look like this:
{
patients:
formValue: […],
filtersValue: {}
}
current realization:
const data = {}
setData(key, value) {
Object.assign(this.data[key] = {}, value);
}
setData(this.stateName, { firstItemsValue: this.itemsCopy });
setData(this.stateName, { secondItemsValue: this.itemsCopy });
javascript object
1
what aboutdata[key] = value
?
– Aprillion
Nov 15 '18 at 8:28
Add an input and an output example of your method to make it more clear
– Panos K
Nov 15 '18 at 8:31
@Aprilliondata[key] = value
will rewrite it on every method call
– Bogdan Tushevskyi
Nov 15 '18 at 8:40
add a comment |
I have a method which takes two parameters key
for property name and value
for the property value. And when I use it I want to update data[key]
and add as many values there as I call the method setData. I need not rewrite value with the same key
, but update it and add a new one, so the final object can look like this:
{
patients:
formValue: […],
filtersValue: {}
}
current realization:
const data = {}
setData(key, value) {
Object.assign(this.data[key] = {}, value);
}
setData(this.stateName, { firstItemsValue: this.itemsCopy });
setData(this.stateName, { secondItemsValue: this.itemsCopy });
javascript object
I have a method which takes two parameters key
for property name and value
for the property value. And when I use it I want to update data[key]
and add as many values there as I call the method setData. I need not rewrite value with the same key
, but update it and add a new one, so the final object can look like this:
{
patients:
formValue: […],
filtersValue: {}
}
current realization:
const data = {}
setData(key, value) {
Object.assign(this.data[key] = {}, value);
}
setData(this.stateName, { firstItemsValue: this.itemsCopy });
setData(this.stateName, { secondItemsValue: this.itemsCopy });
javascript object
javascript object
edited Nov 15 '18 at 9:51
Bogdan Tushevskyi
asked Nov 15 '18 at 8:26
Bogdan TushevskyiBogdan Tushevskyi
14814
14814
1
what aboutdata[key] = value
?
– Aprillion
Nov 15 '18 at 8:28
Add an input and an output example of your method to make it more clear
– Panos K
Nov 15 '18 at 8:31
@Aprilliondata[key] = value
will rewrite it on every method call
– Bogdan Tushevskyi
Nov 15 '18 at 8:40
add a comment |
1
what aboutdata[key] = value
?
– Aprillion
Nov 15 '18 at 8:28
Add an input and an output example of your method to make it more clear
– Panos K
Nov 15 '18 at 8:31
@Aprilliondata[key] = value
will rewrite it on every method call
– Bogdan Tushevskyi
Nov 15 '18 at 8:40
1
1
what about
data[key] = value
?– Aprillion
Nov 15 '18 at 8:28
what about
data[key] = value
?– Aprillion
Nov 15 '18 at 8:28
Add an input and an output example of your method to make it more clear
– Panos K
Nov 15 '18 at 8:31
Add an input and an output example of your method to make it more clear
– Panos K
Nov 15 '18 at 8:31
@Aprillion
data[key] = value
will rewrite it on every method call– Bogdan Tushevskyi
Nov 15 '18 at 8:40
@Aprillion
data[key] = value
will rewrite it on every method call– Bogdan Tushevskyi
Nov 15 '18 at 8:40
add a comment |
5 Answers
5
active
oldest
votes
The solution is:
setData(key, value) {
this.data[key] = { ...this.data[key], ...value };
}
spread operator? why not ... ?
– Tornike Shavishvili
Nov 15 '18 at 15:29
@TornikeShavishvili what is the difference between the spread operator and '...'?
– Bogdan Tushevskyi
Nov 16 '18 at 8:42
In your case three dots are spread operator. In javascript three dots '...' have basically two meanings, 1. If used in function declaration it defines "rest prameters", please see following doxumentations: Functions Rest Parameters 2. In other cases it is interpreted as spread operator. Please see Expressions - Spread syntax
– Tornike Shavishvili
Nov 16 '18 at 8:52
@TornikeShavishvili yes, I know this, I don't understand your first question
– Bogdan Tushevskyi
Nov 16 '18 at 8:54
I think my comment confused you. I meant that spread operator is good to use. I have to be more clear next time.
– Tornike Shavishvili
Nov 16 '18 at 8:55
|
show 1 more comment
You have mentioned that you want to add as many values as youi call setData. That sounds like a job for array.
You could use follwoing code:
function setData(targetObj, propName, valueToAdd){
if(typeof targetObj[propName] === 'undefined'){
targetObj[propName] = ;
}
if(Array.isArray(targetObj[propName])){
targetObj[propName].push(valueToAdd);
}else{
console.log('property '+propName +' already exists and is not an array');
}
}
add a comment |
Use like this:
const data = {}
setData(key, value) {
Object.assign(data, {[key]: value});
}
BTW, You can simply set the data like:
setData(key, value) {
data[key] = value
}
1
Is there a reason forObject.assign
over plain bracket notation? (also,key
in your code will refer to the literal stringkey
, not the variable)
– CertainPerformance
Nov 15 '18 at 8:32
you can change it to { [key] : value } and it should compute the value.
– Lloyd
Nov 15 '18 at 8:33
Thanks for the catch.
– Bhojendra Rauniyar
Nov 15 '18 at 8:33
@CertainPerformance Sorry, what do you mean?Is there a reason for Object.assign over plain bracket notation?
– Bhojendra Rauniyar
Nov 15 '18 at 8:34
If you don't need to merge objects, or create a new object or something like that,Object.assign
seems really weird to use over justdata[key] = value
IMO
– CertainPerformance
Nov 15 '18 at 8:36
|
show 2 more comments
What you're trying to do is to implement a HashMap or a Dictionary.
I'd just do it like so:
function setData(key, value)
{
if (data[key])
data[key].push(value)
else
data[key] = [ value ]
}
you are talking about Hashmap/Dictionary (a.k.a. "Object" in JS), but created an Array in your code
– Aprillion
Nov 17 '18 at 11:00
add a comment |
Check this solution
const data = {}
var fun = (key, value)=>{
data[key] = value;
}
fun('kk',123);
console.log(data);
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53315142%2fupdate-object-property-without-rewriting%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
The solution is:
setData(key, value) {
this.data[key] = { ...this.data[key], ...value };
}
spread operator? why not ... ?
– Tornike Shavishvili
Nov 15 '18 at 15:29
@TornikeShavishvili what is the difference between the spread operator and '...'?
– Bogdan Tushevskyi
Nov 16 '18 at 8:42
In your case three dots are spread operator. In javascript three dots '...' have basically two meanings, 1. If used in function declaration it defines "rest prameters", please see following doxumentations: Functions Rest Parameters 2. In other cases it is interpreted as spread operator. Please see Expressions - Spread syntax
– Tornike Shavishvili
Nov 16 '18 at 8:52
@TornikeShavishvili yes, I know this, I don't understand your first question
– Bogdan Tushevskyi
Nov 16 '18 at 8:54
I think my comment confused you. I meant that spread operator is good to use. I have to be more clear next time.
– Tornike Shavishvili
Nov 16 '18 at 8:55
|
show 1 more comment
The solution is:
setData(key, value) {
this.data[key] = { ...this.data[key], ...value };
}
spread operator? why not ... ?
– Tornike Shavishvili
Nov 15 '18 at 15:29
@TornikeShavishvili what is the difference between the spread operator and '...'?
– Bogdan Tushevskyi
Nov 16 '18 at 8:42
In your case three dots are spread operator. In javascript three dots '...' have basically two meanings, 1. If used in function declaration it defines "rest prameters", please see following doxumentations: Functions Rest Parameters 2. In other cases it is interpreted as spread operator. Please see Expressions - Spread syntax
– Tornike Shavishvili
Nov 16 '18 at 8:52
@TornikeShavishvili yes, I know this, I don't understand your first question
– Bogdan Tushevskyi
Nov 16 '18 at 8:54
I think my comment confused you. I meant that spread operator is good to use. I have to be more clear next time.
– Tornike Shavishvili
Nov 16 '18 at 8:55
|
show 1 more comment
The solution is:
setData(key, value) {
this.data[key] = { ...this.data[key], ...value };
}
The solution is:
setData(key, value) {
this.data[key] = { ...this.data[key], ...value };
}
answered Nov 15 '18 at 14:44
Bogdan TushevskyiBogdan Tushevskyi
14814
14814
spread operator? why not ... ?
– Tornike Shavishvili
Nov 15 '18 at 15:29
@TornikeShavishvili what is the difference between the spread operator and '...'?
– Bogdan Tushevskyi
Nov 16 '18 at 8:42
In your case three dots are spread operator. In javascript three dots '...' have basically two meanings, 1. If used in function declaration it defines "rest prameters", please see following doxumentations: Functions Rest Parameters 2. In other cases it is interpreted as spread operator. Please see Expressions - Spread syntax
– Tornike Shavishvili
Nov 16 '18 at 8:52
@TornikeShavishvili yes, I know this, I don't understand your first question
– Bogdan Tushevskyi
Nov 16 '18 at 8:54
I think my comment confused you. I meant that spread operator is good to use. I have to be more clear next time.
– Tornike Shavishvili
Nov 16 '18 at 8:55
|
show 1 more comment
spread operator? why not ... ?
– Tornike Shavishvili
Nov 15 '18 at 15:29
@TornikeShavishvili what is the difference between the spread operator and '...'?
– Bogdan Tushevskyi
Nov 16 '18 at 8:42
In your case three dots are spread operator. In javascript three dots '...' have basically two meanings, 1. If used in function declaration it defines "rest prameters", please see following doxumentations: Functions Rest Parameters 2. In other cases it is interpreted as spread operator. Please see Expressions - Spread syntax
– Tornike Shavishvili
Nov 16 '18 at 8:52
@TornikeShavishvili yes, I know this, I don't understand your first question
– Bogdan Tushevskyi
Nov 16 '18 at 8:54
I think my comment confused you. I meant that spread operator is good to use. I have to be more clear next time.
– Tornike Shavishvili
Nov 16 '18 at 8:55
spread operator? why not ... ?
– Tornike Shavishvili
Nov 15 '18 at 15:29
spread operator? why not ... ?
– Tornike Shavishvili
Nov 15 '18 at 15:29
@TornikeShavishvili what is the difference between the spread operator and '...'?
– Bogdan Tushevskyi
Nov 16 '18 at 8:42
@TornikeShavishvili what is the difference between the spread operator and '...'?
– Bogdan Tushevskyi
Nov 16 '18 at 8:42
In your case three dots are spread operator. In javascript three dots '...' have basically two meanings, 1. If used in function declaration it defines "rest prameters", please see following doxumentations: Functions Rest Parameters 2. In other cases it is interpreted as spread operator. Please see Expressions - Spread syntax
– Tornike Shavishvili
Nov 16 '18 at 8:52
In your case three dots are spread operator. In javascript three dots '...' have basically two meanings, 1. If used in function declaration it defines "rest prameters", please see following doxumentations: Functions Rest Parameters 2. In other cases it is interpreted as spread operator. Please see Expressions - Spread syntax
– Tornike Shavishvili
Nov 16 '18 at 8:52
@TornikeShavishvili yes, I know this, I don't understand your first question
– Bogdan Tushevskyi
Nov 16 '18 at 8:54
@TornikeShavishvili yes, I know this, I don't understand your first question
– Bogdan Tushevskyi
Nov 16 '18 at 8:54
I think my comment confused you. I meant that spread operator is good to use. I have to be more clear next time.
– Tornike Shavishvili
Nov 16 '18 at 8:55
I think my comment confused you. I meant that spread operator is good to use. I have to be more clear next time.
– Tornike Shavishvili
Nov 16 '18 at 8:55
|
show 1 more comment
You have mentioned that you want to add as many values as youi call setData. That sounds like a job for array.
You could use follwoing code:
function setData(targetObj, propName, valueToAdd){
if(typeof targetObj[propName] === 'undefined'){
targetObj[propName] = ;
}
if(Array.isArray(targetObj[propName])){
targetObj[propName].push(valueToAdd);
}else{
console.log('property '+propName +' already exists and is not an array');
}
}
add a comment |
You have mentioned that you want to add as many values as youi call setData. That sounds like a job for array.
You could use follwoing code:
function setData(targetObj, propName, valueToAdd){
if(typeof targetObj[propName] === 'undefined'){
targetObj[propName] = ;
}
if(Array.isArray(targetObj[propName])){
targetObj[propName].push(valueToAdd);
}else{
console.log('property '+propName +' already exists and is not an array');
}
}
add a comment |
You have mentioned that you want to add as many values as youi call setData. That sounds like a job for array.
You could use follwoing code:
function setData(targetObj, propName, valueToAdd){
if(typeof targetObj[propName] === 'undefined'){
targetObj[propName] = ;
}
if(Array.isArray(targetObj[propName])){
targetObj[propName].push(valueToAdd);
}else{
console.log('property '+propName +' already exists and is not an array');
}
}
You have mentioned that you want to add as many values as youi call setData. That sounds like a job for array.
You could use follwoing code:
function setData(targetObj, propName, valueToAdd){
if(typeof targetObj[propName] === 'undefined'){
targetObj[propName] = ;
}
if(Array.isArray(targetObj[propName])){
targetObj[propName].push(valueToAdd);
}else{
console.log('property '+propName +' already exists and is not an array');
}
}
answered Nov 15 '18 at 8:37


Tornike ShavishviliTornike Shavishvili
61021122
61021122
add a comment |
add a comment |
Use like this:
const data = {}
setData(key, value) {
Object.assign(data, {[key]: value});
}
BTW, You can simply set the data like:
setData(key, value) {
data[key] = value
}
1
Is there a reason forObject.assign
over plain bracket notation? (also,key
in your code will refer to the literal stringkey
, not the variable)
– CertainPerformance
Nov 15 '18 at 8:32
you can change it to { [key] : value } and it should compute the value.
– Lloyd
Nov 15 '18 at 8:33
Thanks for the catch.
– Bhojendra Rauniyar
Nov 15 '18 at 8:33
@CertainPerformance Sorry, what do you mean?Is there a reason for Object.assign over plain bracket notation?
– Bhojendra Rauniyar
Nov 15 '18 at 8:34
If you don't need to merge objects, or create a new object or something like that,Object.assign
seems really weird to use over justdata[key] = value
IMO
– CertainPerformance
Nov 15 '18 at 8:36
|
show 2 more comments
Use like this:
const data = {}
setData(key, value) {
Object.assign(data, {[key]: value});
}
BTW, You can simply set the data like:
setData(key, value) {
data[key] = value
}
1
Is there a reason forObject.assign
over plain bracket notation? (also,key
in your code will refer to the literal stringkey
, not the variable)
– CertainPerformance
Nov 15 '18 at 8:32
you can change it to { [key] : value } and it should compute the value.
– Lloyd
Nov 15 '18 at 8:33
Thanks for the catch.
– Bhojendra Rauniyar
Nov 15 '18 at 8:33
@CertainPerformance Sorry, what do you mean?Is there a reason for Object.assign over plain bracket notation?
– Bhojendra Rauniyar
Nov 15 '18 at 8:34
If you don't need to merge objects, or create a new object or something like that,Object.assign
seems really weird to use over justdata[key] = value
IMO
– CertainPerformance
Nov 15 '18 at 8:36
|
show 2 more comments
Use like this:
const data = {}
setData(key, value) {
Object.assign(data, {[key]: value});
}
BTW, You can simply set the data like:
setData(key, value) {
data[key] = value
}
Use like this:
const data = {}
setData(key, value) {
Object.assign(data, {[key]: value});
}
BTW, You can simply set the data like:
setData(key, value) {
data[key] = value
}
edited Nov 15 '18 at 8:38
answered Nov 15 '18 at 8:31


Bhojendra RauniyarBhojendra Rauniyar
51.7k2079128
51.7k2079128
1
Is there a reason forObject.assign
over plain bracket notation? (also,key
in your code will refer to the literal stringkey
, not the variable)
– CertainPerformance
Nov 15 '18 at 8:32
you can change it to { [key] : value } and it should compute the value.
– Lloyd
Nov 15 '18 at 8:33
Thanks for the catch.
– Bhojendra Rauniyar
Nov 15 '18 at 8:33
@CertainPerformance Sorry, what do you mean?Is there a reason for Object.assign over plain bracket notation?
– Bhojendra Rauniyar
Nov 15 '18 at 8:34
If you don't need to merge objects, or create a new object or something like that,Object.assign
seems really weird to use over justdata[key] = value
IMO
– CertainPerformance
Nov 15 '18 at 8:36
|
show 2 more comments
1
Is there a reason forObject.assign
over plain bracket notation? (also,key
in your code will refer to the literal stringkey
, not the variable)
– CertainPerformance
Nov 15 '18 at 8:32
you can change it to { [key] : value } and it should compute the value.
– Lloyd
Nov 15 '18 at 8:33
Thanks for the catch.
– Bhojendra Rauniyar
Nov 15 '18 at 8:33
@CertainPerformance Sorry, what do you mean?Is there a reason for Object.assign over plain bracket notation?
– Bhojendra Rauniyar
Nov 15 '18 at 8:34
If you don't need to merge objects, or create a new object or something like that,Object.assign
seems really weird to use over justdata[key] = value
IMO
– CertainPerformance
Nov 15 '18 at 8:36
1
1
Is there a reason for
Object.assign
over plain bracket notation? (also, key
in your code will refer to the literal string key
, not the variable)– CertainPerformance
Nov 15 '18 at 8:32
Is there a reason for
Object.assign
over plain bracket notation? (also, key
in your code will refer to the literal string key
, not the variable)– CertainPerformance
Nov 15 '18 at 8:32
you can change it to { [key] : value } and it should compute the value.
– Lloyd
Nov 15 '18 at 8:33
you can change it to { [key] : value } and it should compute the value.
– Lloyd
Nov 15 '18 at 8:33
Thanks for the catch.
– Bhojendra Rauniyar
Nov 15 '18 at 8:33
Thanks for the catch.
– Bhojendra Rauniyar
Nov 15 '18 at 8:33
@CertainPerformance Sorry, what do you mean?
Is there a reason for Object.assign over plain bracket notation?
– Bhojendra Rauniyar
Nov 15 '18 at 8:34
@CertainPerformance Sorry, what do you mean?
Is there a reason for Object.assign over plain bracket notation?
– Bhojendra Rauniyar
Nov 15 '18 at 8:34
If you don't need to merge objects, or create a new object or something like that,
Object.assign
seems really weird to use over just data[key] = value
IMO– CertainPerformance
Nov 15 '18 at 8:36
If you don't need to merge objects, or create a new object or something like that,
Object.assign
seems really weird to use over just data[key] = value
IMO– CertainPerformance
Nov 15 '18 at 8:36
|
show 2 more comments
What you're trying to do is to implement a HashMap or a Dictionary.
I'd just do it like so:
function setData(key, value)
{
if (data[key])
data[key].push(value)
else
data[key] = [ value ]
}
you are talking about Hashmap/Dictionary (a.k.a. "Object" in JS), but created an Array in your code
– Aprillion
Nov 17 '18 at 11:00
add a comment |
What you're trying to do is to implement a HashMap or a Dictionary.
I'd just do it like so:
function setData(key, value)
{
if (data[key])
data[key].push(value)
else
data[key] = [ value ]
}
you are talking about Hashmap/Dictionary (a.k.a. "Object" in JS), but created an Array in your code
– Aprillion
Nov 17 '18 at 11:00
add a comment |
What you're trying to do is to implement a HashMap or a Dictionary.
I'd just do it like so:
function setData(key, value)
{
if (data[key])
data[key].push(value)
else
data[key] = [ value ]
}
What you're trying to do is to implement a HashMap or a Dictionary.
I'd just do it like so:
function setData(key, value)
{
if (data[key])
data[key].push(value)
else
data[key] = [ value ]
}
answered Nov 15 '18 at 8:45
AlexLAlexL
813
813
you are talking about Hashmap/Dictionary (a.k.a. "Object" in JS), but created an Array in your code
– Aprillion
Nov 17 '18 at 11:00
add a comment |
you are talking about Hashmap/Dictionary (a.k.a. "Object" in JS), but created an Array in your code
– Aprillion
Nov 17 '18 at 11:00
you are talking about Hashmap/Dictionary (a.k.a. "Object" in JS), but created an Array in your code
– Aprillion
Nov 17 '18 at 11:00
you are talking about Hashmap/Dictionary (a.k.a. "Object" in JS), but created an Array in your code
– Aprillion
Nov 17 '18 at 11:00
add a comment |
Check this solution
const data = {}
var fun = (key, value)=>{
data[key] = value;
}
fun('kk',123);
console.log(data);
add a comment |
Check this solution
const data = {}
var fun = (key, value)=>{
data[key] = value;
}
fun('kk',123);
console.log(data);
add a comment |
Check this solution
const data = {}
var fun = (key, value)=>{
data[key] = value;
}
fun('kk',123);
console.log(data);
Check this solution
const data = {}
var fun = (key, value)=>{
data[key] = value;
}
fun('kk',123);
console.log(data);
const data = {}
var fun = (key, value)=>{
data[key] = value;
}
fun('kk',123);
console.log(data);
const data = {}
var fun = (key, value)=>{
data[key] = value;
}
fun('kk',123);
console.log(data);
answered Nov 15 '18 at 8:49


GAURAVGAURAV
11211
11211
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53315142%2fupdate-object-property-without-rewriting%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
NqXlunxPkcc Zm AvvK,keyGJUe bDG1a5awYcagmEKtkajVMzqH436R1 ViQYpxcSu6cZKwu1K7oRp
1
what about
data[key] = value
?– Aprillion
Nov 15 '18 at 8:28
Add an input and an output example of your method to make it more clear
– Panos K
Nov 15 '18 at 8:31
@Aprillion
data[key] = value
will rewrite it on every method call– Bogdan Tushevskyi
Nov 15 '18 at 8:40