How do I connect two different stores to the same action in Redux?
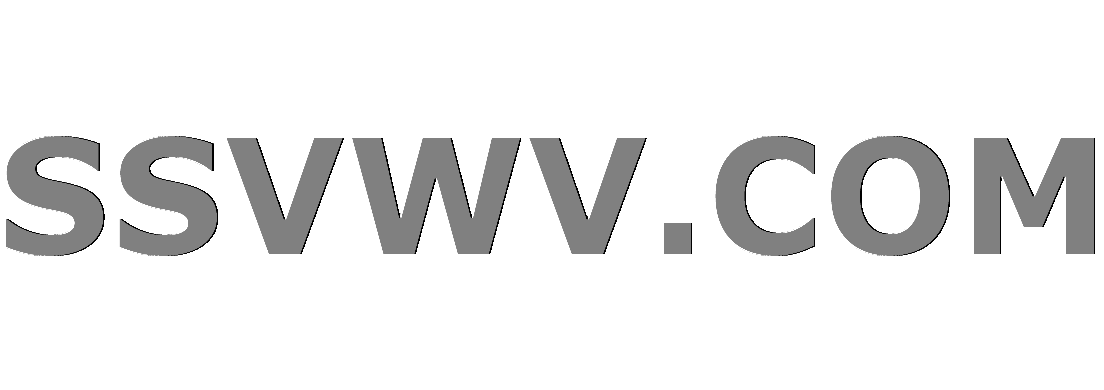
Multi tool use
I have a user store that handles all the user actions, which include fetching user data and updating user information. I've also got a post store that fetches posts users have written and also, posts, updates, and deletes them.
When I update my user information and call my function:
export const putUserDataPending = () => {
return {
type: "PUT_USER_DATA_PENDING"
}
}
export const putUserDataFulfilled = user => {
return {
type: "PUT_USER_DATA_FULFILLED",
user: user
}
}
export const putUserDataRejected = error => {
return {
type: "PUT_USER_DATA_REJECTED",
error: error
}
}
export const putUserData = (username, email, countries, home) => {
const token = localStorage.getItem('token');
return dispatch => {
dispatch(putUserDataPending());
axios.put(
'http://localhost:8000/api/v1/rest-auth/user/',
{
username: username,
email: email,
countries: countries,
home: home
},
{headers: { 'Authorization': `Token ${token}`}}
)
.then(response => {
const user = response.data;
dispatch(putUserDataFulfilled(user));
})
.catch(err => {
dispatch(putUserDataRejected(err));
})
}
}
It rerenders the user profile page, but it doesn't rerender the author information because the state for the posts was never updated. What's the proper way to update any of the author objects in the posts state that may have been updated by updating the user?
Or is it just better to manually rerender the homepage?
My home page MapState is this:
const mapState = state => {
return {
fetched: state.tripReport.fetched,
fetching: state.tripReport.fetching,
tripReports: state.tripReport.tripReports,
showCountryModal: state.modal.showCountryModal,
modalCountry: state.modal.modalCountry
};
}
where tripReports is an array of objects, and some of the authors I want to update.
My editProfile MapState looks like this:
const mapState = state => {
return {
user: state.user.user,
userCountries: state.user.user.countries,
searchedCountry: state.country.country,
showProfileModal: state.modal.showProfileModal,
modalProfile: state.modal.modalProfile
};
}
Where the user object is the object getting updated.
Here is the post reducer:
const initialState = {
fetching: false,
fetched: false,
fetchingTripReports: false,
fetchedTripReports: false,
tripReports: ,
userTripReports: ,
error: null,
}
export default function (state = initialState, action) {
switch (action.type) {
case "FETCH_TRIP_REPORTS_PENDING": {
return {
...state,
fetching: true
}
}
case "FETCH_TRIP_REPORTS_FULFILLED": {
return {
...state,
fetching: false,
fetched: true,
tripReports: action.tripReports
}
}
case "FETCH_TRIP_REPORTS_REJECTED": {
return {
...state,
fetching: false,
fetched: false,
error: action.error
}
}
case "FETCH_USER_TRIP_REPORTS_PENDING": {
return {
...state,
fetchingTripReports: true
}
}
case "FETCH_USER_TRIP_REPORTS_FULFILLED": {
return {
...state,
fetchingTripReports: false,
fetchedTripReports: true,
userTripReports: action.tripReports
}
}
case "FETCH_USER_TRIP_REPORTS_REJECTED": {
return {
...state,
fetchingTripReports: false,
fetchedTripReports: false,
error: action.error
}
}
case "POST_TRIP_REPORTS_PENDING": {
return {
...state,
}
}
case "POST_TRIP_REPORTS_FULFILLED": {
return {
...state,
// The new trip report must be added onto the array, then the array must be sorted by id.
userTripReports: [...state.userTripReports].concat(action.response).sort((a, b) => a.id < b.id),
tripReports: [...state.tripReports].concat(action.response).sort((a, b) => a.id < b.id)
}
}
case "POST_TRIP_REPORTS_REJECTED": {
return {
...state,
error: action.error
}
}
case "DELETE_TRIP_REPORTS_PENDING": {
return {
...state,
}
}
case "DELETE_TRIP_REPORTS_FULFILLED": {
return {
...state,
// The deleted post must be filtered out of the lists.
userTripReports: state.userTripReports.filter(tripReport => tripReport.id !== action.response.id),
tripReports: state.tripReports.filter(tripReport => tripReport.id !== action.response.id)
}
}
case "DELETE_TRIP_REPORTS_REJECTED": {
return {
...state,
error: action.error
}
}
case "UPDATE_TRIP_REPORTS_PENDING": {
return {
...state,
}
}
case "UPDATE_TRIP_REPORTS_FULFILLED": {
return {
...state,
// The old post must be filtered out, the updated post must be added, then the array must be sorted.
userTripReports: [...state.userTripReports].filter(tripReport => tripReport.id !== action.response.id).concat(action.response).sort((a, b) => a.id < b.id),
tripReports: [...state.tripReports].filter(tripReport => tripReport.id !== action.response.id).concat(action.response).sort((a, b) => a.id < b.id),
response: action.response
}
}
case "UPDATE_TRIP_REPORTS_REJECTED": {
return {
...state,
error: action.error
}
}
default:
return state
}
}
and here is the user reducer:
const initialState = {
fetching: false,
fetched: false,
adding: false,
added: false,
user: {},
error: null,
}
/* Reducer Function*/
export default function (state = initialState, action) {
switch (action.type) {
case "FETCH_USER_PENDING": {
return {
...state,
fetching: true
}
}
case "FETCH_USER_FULFILLED": {
return {
...state,
fetching: false,
fetched: true,
user: action.user
}
}
case "FETCH_USER_REJECTED": {
return {
...state,
fetching: false,
fetched: false,
error: action.error
}
}
case "PUT_USER_DATA_PENDING": {
return {
...state,
adding: true,
added: false,
}
}
case "PUT_USER_DATA_FULFILLED": {
return {
...state,
adding: false,
added: true,
user: action.user
}
}
case "PUT_USER_DATA_REJECTED": {
return {
...state,
adding: false,
added: false,
error: action.error
}
}
default:
return state
}
}
Where on the action 'PUT_USER_DATA_FULFILLED' I would like to update the tripReport state to rerender the homepage.
reactjs redux
add a comment |
I have a user store that handles all the user actions, which include fetching user data and updating user information. I've also got a post store that fetches posts users have written and also, posts, updates, and deletes them.
When I update my user information and call my function:
export const putUserDataPending = () => {
return {
type: "PUT_USER_DATA_PENDING"
}
}
export const putUserDataFulfilled = user => {
return {
type: "PUT_USER_DATA_FULFILLED",
user: user
}
}
export const putUserDataRejected = error => {
return {
type: "PUT_USER_DATA_REJECTED",
error: error
}
}
export const putUserData = (username, email, countries, home) => {
const token = localStorage.getItem('token');
return dispatch => {
dispatch(putUserDataPending());
axios.put(
'http://localhost:8000/api/v1/rest-auth/user/',
{
username: username,
email: email,
countries: countries,
home: home
},
{headers: { 'Authorization': `Token ${token}`}}
)
.then(response => {
const user = response.data;
dispatch(putUserDataFulfilled(user));
})
.catch(err => {
dispatch(putUserDataRejected(err));
})
}
}
It rerenders the user profile page, but it doesn't rerender the author information because the state for the posts was never updated. What's the proper way to update any of the author objects in the posts state that may have been updated by updating the user?
Or is it just better to manually rerender the homepage?
My home page MapState is this:
const mapState = state => {
return {
fetched: state.tripReport.fetched,
fetching: state.tripReport.fetching,
tripReports: state.tripReport.tripReports,
showCountryModal: state.modal.showCountryModal,
modalCountry: state.modal.modalCountry
};
}
where tripReports is an array of objects, and some of the authors I want to update.
My editProfile MapState looks like this:
const mapState = state => {
return {
user: state.user.user,
userCountries: state.user.user.countries,
searchedCountry: state.country.country,
showProfileModal: state.modal.showProfileModal,
modalProfile: state.modal.modalProfile
};
}
Where the user object is the object getting updated.
Here is the post reducer:
const initialState = {
fetching: false,
fetched: false,
fetchingTripReports: false,
fetchedTripReports: false,
tripReports: ,
userTripReports: ,
error: null,
}
export default function (state = initialState, action) {
switch (action.type) {
case "FETCH_TRIP_REPORTS_PENDING": {
return {
...state,
fetching: true
}
}
case "FETCH_TRIP_REPORTS_FULFILLED": {
return {
...state,
fetching: false,
fetched: true,
tripReports: action.tripReports
}
}
case "FETCH_TRIP_REPORTS_REJECTED": {
return {
...state,
fetching: false,
fetched: false,
error: action.error
}
}
case "FETCH_USER_TRIP_REPORTS_PENDING": {
return {
...state,
fetchingTripReports: true
}
}
case "FETCH_USER_TRIP_REPORTS_FULFILLED": {
return {
...state,
fetchingTripReports: false,
fetchedTripReports: true,
userTripReports: action.tripReports
}
}
case "FETCH_USER_TRIP_REPORTS_REJECTED": {
return {
...state,
fetchingTripReports: false,
fetchedTripReports: false,
error: action.error
}
}
case "POST_TRIP_REPORTS_PENDING": {
return {
...state,
}
}
case "POST_TRIP_REPORTS_FULFILLED": {
return {
...state,
// The new trip report must be added onto the array, then the array must be sorted by id.
userTripReports: [...state.userTripReports].concat(action.response).sort((a, b) => a.id < b.id),
tripReports: [...state.tripReports].concat(action.response).sort((a, b) => a.id < b.id)
}
}
case "POST_TRIP_REPORTS_REJECTED": {
return {
...state,
error: action.error
}
}
case "DELETE_TRIP_REPORTS_PENDING": {
return {
...state,
}
}
case "DELETE_TRIP_REPORTS_FULFILLED": {
return {
...state,
// The deleted post must be filtered out of the lists.
userTripReports: state.userTripReports.filter(tripReport => tripReport.id !== action.response.id),
tripReports: state.tripReports.filter(tripReport => tripReport.id !== action.response.id)
}
}
case "DELETE_TRIP_REPORTS_REJECTED": {
return {
...state,
error: action.error
}
}
case "UPDATE_TRIP_REPORTS_PENDING": {
return {
...state,
}
}
case "UPDATE_TRIP_REPORTS_FULFILLED": {
return {
...state,
// The old post must be filtered out, the updated post must be added, then the array must be sorted.
userTripReports: [...state.userTripReports].filter(tripReport => tripReport.id !== action.response.id).concat(action.response).sort((a, b) => a.id < b.id),
tripReports: [...state.tripReports].filter(tripReport => tripReport.id !== action.response.id).concat(action.response).sort((a, b) => a.id < b.id),
response: action.response
}
}
case "UPDATE_TRIP_REPORTS_REJECTED": {
return {
...state,
error: action.error
}
}
default:
return state
}
}
and here is the user reducer:
const initialState = {
fetching: false,
fetched: false,
adding: false,
added: false,
user: {},
error: null,
}
/* Reducer Function*/
export default function (state = initialState, action) {
switch (action.type) {
case "FETCH_USER_PENDING": {
return {
...state,
fetching: true
}
}
case "FETCH_USER_FULFILLED": {
return {
...state,
fetching: false,
fetched: true,
user: action.user
}
}
case "FETCH_USER_REJECTED": {
return {
...state,
fetching: false,
fetched: false,
error: action.error
}
}
case "PUT_USER_DATA_PENDING": {
return {
...state,
adding: true,
added: false,
}
}
case "PUT_USER_DATA_FULFILLED": {
return {
...state,
adding: false,
added: true,
user: action.user
}
}
case "PUT_USER_DATA_REJECTED": {
return {
...state,
adding: false,
added: false,
error: action.error
}
}
default:
return state
}
}
Where on the action 'PUT_USER_DATA_FULFILLED' I would like to update the tripReport state to rerender the homepage.
reactjs redux
1
When you say a "posts store" and a "users store", are you actually callingcreateStore()
twice? Or are you using one store with two separate "slice reducers", likecombineReducers({users : usersReducer, posts: postsReducer})
? We recommend using only one store, with multiple slice reducers.
– markerikson
Nov 15 '18 at 16:26
Sorry my post was unclear; I do only have one store with combined reducers, but since they are divided, it's giving me issues with rerendering .
– peter176
Nov 15 '18 at 17:19
Can you show both the reducers and the component'smapState
function?
– markerikson
Nov 15 '18 at 17:34
Yes, I added them to the post
– peter176
Nov 15 '18 at 17:47
add a comment |
I have a user store that handles all the user actions, which include fetching user data and updating user information. I've also got a post store that fetches posts users have written and also, posts, updates, and deletes them.
When I update my user information and call my function:
export const putUserDataPending = () => {
return {
type: "PUT_USER_DATA_PENDING"
}
}
export const putUserDataFulfilled = user => {
return {
type: "PUT_USER_DATA_FULFILLED",
user: user
}
}
export const putUserDataRejected = error => {
return {
type: "PUT_USER_DATA_REJECTED",
error: error
}
}
export const putUserData = (username, email, countries, home) => {
const token = localStorage.getItem('token');
return dispatch => {
dispatch(putUserDataPending());
axios.put(
'http://localhost:8000/api/v1/rest-auth/user/',
{
username: username,
email: email,
countries: countries,
home: home
},
{headers: { 'Authorization': `Token ${token}`}}
)
.then(response => {
const user = response.data;
dispatch(putUserDataFulfilled(user));
})
.catch(err => {
dispatch(putUserDataRejected(err));
})
}
}
It rerenders the user profile page, but it doesn't rerender the author information because the state for the posts was never updated. What's the proper way to update any of the author objects in the posts state that may have been updated by updating the user?
Or is it just better to manually rerender the homepage?
My home page MapState is this:
const mapState = state => {
return {
fetched: state.tripReport.fetched,
fetching: state.tripReport.fetching,
tripReports: state.tripReport.tripReports,
showCountryModal: state.modal.showCountryModal,
modalCountry: state.modal.modalCountry
};
}
where tripReports is an array of objects, and some of the authors I want to update.
My editProfile MapState looks like this:
const mapState = state => {
return {
user: state.user.user,
userCountries: state.user.user.countries,
searchedCountry: state.country.country,
showProfileModal: state.modal.showProfileModal,
modalProfile: state.modal.modalProfile
};
}
Where the user object is the object getting updated.
Here is the post reducer:
const initialState = {
fetching: false,
fetched: false,
fetchingTripReports: false,
fetchedTripReports: false,
tripReports: ,
userTripReports: ,
error: null,
}
export default function (state = initialState, action) {
switch (action.type) {
case "FETCH_TRIP_REPORTS_PENDING": {
return {
...state,
fetching: true
}
}
case "FETCH_TRIP_REPORTS_FULFILLED": {
return {
...state,
fetching: false,
fetched: true,
tripReports: action.tripReports
}
}
case "FETCH_TRIP_REPORTS_REJECTED": {
return {
...state,
fetching: false,
fetched: false,
error: action.error
}
}
case "FETCH_USER_TRIP_REPORTS_PENDING": {
return {
...state,
fetchingTripReports: true
}
}
case "FETCH_USER_TRIP_REPORTS_FULFILLED": {
return {
...state,
fetchingTripReports: false,
fetchedTripReports: true,
userTripReports: action.tripReports
}
}
case "FETCH_USER_TRIP_REPORTS_REJECTED": {
return {
...state,
fetchingTripReports: false,
fetchedTripReports: false,
error: action.error
}
}
case "POST_TRIP_REPORTS_PENDING": {
return {
...state,
}
}
case "POST_TRIP_REPORTS_FULFILLED": {
return {
...state,
// The new trip report must be added onto the array, then the array must be sorted by id.
userTripReports: [...state.userTripReports].concat(action.response).sort((a, b) => a.id < b.id),
tripReports: [...state.tripReports].concat(action.response).sort((a, b) => a.id < b.id)
}
}
case "POST_TRIP_REPORTS_REJECTED": {
return {
...state,
error: action.error
}
}
case "DELETE_TRIP_REPORTS_PENDING": {
return {
...state,
}
}
case "DELETE_TRIP_REPORTS_FULFILLED": {
return {
...state,
// The deleted post must be filtered out of the lists.
userTripReports: state.userTripReports.filter(tripReport => tripReport.id !== action.response.id),
tripReports: state.tripReports.filter(tripReport => tripReport.id !== action.response.id)
}
}
case "DELETE_TRIP_REPORTS_REJECTED": {
return {
...state,
error: action.error
}
}
case "UPDATE_TRIP_REPORTS_PENDING": {
return {
...state,
}
}
case "UPDATE_TRIP_REPORTS_FULFILLED": {
return {
...state,
// The old post must be filtered out, the updated post must be added, then the array must be sorted.
userTripReports: [...state.userTripReports].filter(tripReport => tripReport.id !== action.response.id).concat(action.response).sort((a, b) => a.id < b.id),
tripReports: [...state.tripReports].filter(tripReport => tripReport.id !== action.response.id).concat(action.response).sort((a, b) => a.id < b.id),
response: action.response
}
}
case "UPDATE_TRIP_REPORTS_REJECTED": {
return {
...state,
error: action.error
}
}
default:
return state
}
}
and here is the user reducer:
const initialState = {
fetching: false,
fetched: false,
adding: false,
added: false,
user: {},
error: null,
}
/* Reducer Function*/
export default function (state = initialState, action) {
switch (action.type) {
case "FETCH_USER_PENDING": {
return {
...state,
fetching: true
}
}
case "FETCH_USER_FULFILLED": {
return {
...state,
fetching: false,
fetched: true,
user: action.user
}
}
case "FETCH_USER_REJECTED": {
return {
...state,
fetching: false,
fetched: false,
error: action.error
}
}
case "PUT_USER_DATA_PENDING": {
return {
...state,
adding: true,
added: false,
}
}
case "PUT_USER_DATA_FULFILLED": {
return {
...state,
adding: false,
added: true,
user: action.user
}
}
case "PUT_USER_DATA_REJECTED": {
return {
...state,
adding: false,
added: false,
error: action.error
}
}
default:
return state
}
}
Where on the action 'PUT_USER_DATA_FULFILLED' I would like to update the tripReport state to rerender the homepage.
reactjs redux
I have a user store that handles all the user actions, which include fetching user data and updating user information. I've also got a post store that fetches posts users have written and also, posts, updates, and deletes them.
When I update my user information and call my function:
export const putUserDataPending = () => {
return {
type: "PUT_USER_DATA_PENDING"
}
}
export const putUserDataFulfilled = user => {
return {
type: "PUT_USER_DATA_FULFILLED",
user: user
}
}
export const putUserDataRejected = error => {
return {
type: "PUT_USER_DATA_REJECTED",
error: error
}
}
export const putUserData = (username, email, countries, home) => {
const token = localStorage.getItem('token');
return dispatch => {
dispatch(putUserDataPending());
axios.put(
'http://localhost:8000/api/v1/rest-auth/user/',
{
username: username,
email: email,
countries: countries,
home: home
},
{headers: { 'Authorization': `Token ${token}`}}
)
.then(response => {
const user = response.data;
dispatch(putUserDataFulfilled(user));
})
.catch(err => {
dispatch(putUserDataRejected(err));
})
}
}
It rerenders the user profile page, but it doesn't rerender the author information because the state for the posts was never updated. What's the proper way to update any of the author objects in the posts state that may have been updated by updating the user?
Or is it just better to manually rerender the homepage?
My home page MapState is this:
const mapState = state => {
return {
fetched: state.tripReport.fetched,
fetching: state.tripReport.fetching,
tripReports: state.tripReport.tripReports,
showCountryModal: state.modal.showCountryModal,
modalCountry: state.modal.modalCountry
};
}
where tripReports is an array of objects, and some of the authors I want to update.
My editProfile MapState looks like this:
const mapState = state => {
return {
user: state.user.user,
userCountries: state.user.user.countries,
searchedCountry: state.country.country,
showProfileModal: state.modal.showProfileModal,
modalProfile: state.modal.modalProfile
};
}
Where the user object is the object getting updated.
Here is the post reducer:
const initialState = {
fetching: false,
fetched: false,
fetchingTripReports: false,
fetchedTripReports: false,
tripReports: ,
userTripReports: ,
error: null,
}
export default function (state = initialState, action) {
switch (action.type) {
case "FETCH_TRIP_REPORTS_PENDING": {
return {
...state,
fetching: true
}
}
case "FETCH_TRIP_REPORTS_FULFILLED": {
return {
...state,
fetching: false,
fetched: true,
tripReports: action.tripReports
}
}
case "FETCH_TRIP_REPORTS_REJECTED": {
return {
...state,
fetching: false,
fetched: false,
error: action.error
}
}
case "FETCH_USER_TRIP_REPORTS_PENDING": {
return {
...state,
fetchingTripReports: true
}
}
case "FETCH_USER_TRIP_REPORTS_FULFILLED": {
return {
...state,
fetchingTripReports: false,
fetchedTripReports: true,
userTripReports: action.tripReports
}
}
case "FETCH_USER_TRIP_REPORTS_REJECTED": {
return {
...state,
fetchingTripReports: false,
fetchedTripReports: false,
error: action.error
}
}
case "POST_TRIP_REPORTS_PENDING": {
return {
...state,
}
}
case "POST_TRIP_REPORTS_FULFILLED": {
return {
...state,
// The new trip report must be added onto the array, then the array must be sorted by id.
userTripReports: [...state.userTripReports].concat(action.response).sort((a, b) => a.id < b.id),
tripReports: [...state.tripReports].concat(action.response).sort((a, b) => a.id < b.id)
}
}
case "POST_TRIP_REPORTS_REJECTED": {
return {
...state,
error: action.error
}
}
case "DELETE_TRIP_REPORTS_PENDING": {
return {
...state,
}
}
case "DELETE_TRIP_REPORTS_FULFILLED": {
return {
...state,
// The deleted post must be filtered out of the lists.
userTripReports: state.userTripReports.filter(tripReport => tripReport.id !== action.response.id),
tripReports: state.tripReports.filter(tripReport => tripReport.id !== action.response.id)
}
}
case "DELETE_TRIP_REPORTS_REJECTED": {
return {
...state,
error: action.error
}
}
case "UPDATE_TRIP_REPORTS_PENDING": {
return {
...state,
}
}
case "UPDATE_TRIP_REPORTS_FULFILLED": {
return {
...state,
// The old post must be filtered out, the updated post must be added, then the array must be sorted.
userTripReports: [...state.userTripReports].filter(tripReport => tripReport.id !== action.response.id).concat(action.response).sort((a, b) => a.id < b.id),
tripReports: [...state.tripReports].filter(tripReport => tripReport.id !== action.response.id).concat(action.response).sort((a, b) => a.id < b.id),
response: action.response
}
}
case "UPDATE_TRIP_REPORTS_REJECTED": {
return {
...state,
error: action.error
}
}
default:
return state
}
}
and here is the user reducer:
const initialState = {
fetching: false,
fetched: false,
adding: false,
added: false,
user: {},
error: null,
}
/* Reducer Function*/
export default function (state = initialState, action) {
switch (action.type) {
case "FETCH_USER_PENDING": {
return {
...state,
fetching: true
}
}
case "FETCH_USER_FULFILLED": {
return {
...state,
fetching: false,
fetched: true,
user: action.user
}
}
case "FETCH_USER_REJECTED": {
return {
...state,
fetching: false,
fetched: false,
error: action.error
}
}
case "PUT_USER_DATA_PENDING": {
return {
...state,
adding: true,
added: false,
}
}
case "PUT_USER_DATA_FULFILLED": {
return {
...state,
adding: false,
added: true,
user: action.user
}
}
case "PUT_USER_DATA_REJECTED": {
return {
...state,
adding: false,
added: false,
error: action.error
}
}
default:
return state
}
}
Where on the action 'PUT_USER_DATA_FULFILLED' I would like to update the tripReport state to rerender the homepage.
reactjs redux
reactjs redux
edited Nov 15 '18 at 17:43
peter176
asked Nov 15 '18 at 8:36


peter176peter176
5110
5110
1
When you say a "posts store" and a "users store", are you actually callingcreateStore()
twice? Or are you using one store with two separate "slice reducers", likecombineReducers({users : usersReducer, posts: postsReducer})
? We recommend using only one store, with multiple slice reducers.
– markerikson
Nov 15 '18 at 16:26
Sorry my post was unclear; I do only have one store with combined reducers, but since they are divided, it's giving me issues with rerendering .
– peter176
Nov 15 '18 at 17:19
Can you show both the reducers and the component'smapState
function?
– markerikson
Nov 15 '18 at 17:34
Yes, I added them to the post
– peter176
Nov 15 '18 at 17:47
add a comment |
1
When you say a "posts store" and a "users store", are you actually callingcreateStore()
twice? Or are you using one store with two separate "slice reducers", likecombineReducers({users : usersReducer, posts: postsReducer})
? We recommend using only one store, with multiple slice reducers.
– markerikson
Nov 15 '18 at 16:26
Sorry my post was unclear; I do only have one store with combined reducers, but since they are divided, it's giving me issues with rerendering .
– peter176
Nov 15 '18 at 17:19
Can you show both the reducers and the component'smapState
function?
– markerikson
Nov 15 '18 at 17:34
Yes, I added them to the post
– peter176
Nov 15 '18 at 17:47
1
1
When you say a "posts store" and a "users store", are you actually calling
createStore()
twice? Or are you using one store with two separate "slice reducers", like combineReducers({users : usersReducer, posts: postsReducer})
? We recommend using only one store, with multiple slice reducers.– markerikson
Nov 15 '18 at 16:26
When you say a "posts store" and a "users store", are you actually calling
createStore()
twice? Or are you using one store with two separate "slice reducers", like combineReducers({users : usersReducer, posts: postsReducer})
? We recommend using only one store, with multiple slice reducers.– markerikson
Nov 15 '18 at 16:26
Sorry my post was unclear; I do only have one store with combined reducers, but since they are divided, it's giving me issues with rerendering .
– peter176
Nov 15 '18 at 17:19
Sorry my post was unclear; I do only have one store with combined reducers, but since they are divided, it's giving me issues with rerendering .
– peter176
Nov 15 '18 at 17:19
Can you show both the reducers and the component's
mapState
function?– markerikson
Nov 15 '18 at 17:34
Can you show both the reducers and the component's
mapState
function?– markerikson
Nov 15 '18 at 17:34
Yes, I added them to the post
– peter176
Nov 15 '18 at 17:47
Yes, I added them to the post
– peter176
Nov 15 '18 at 17:47
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53315284%2fhow-do-i-connect-two-different-stores-to-the-same-action-in-redux%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53315284%2fhow-do-i-connect-two-different-stores-to-the-same-action-in-redux%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
TdiT0EC Iq002Zh,KS3ZCHRMRJ0piNHAh0aYFY,8,TFxwC9p4
1
When you say a "posts store" and a "users store", are you actually calling
createStore()
twice? Or are you using one store with two separate "slice reducers", likecombineReducers({users : usersReducer, posts: postsReducer})
? We recommend using only one store, with multiple slice reducers.– markerikson
Nov 15 '18 at 16:26
Sorry my post was unclear; I do only have one store with combined reducers, but since they are divided, it's giving me issues with rerendering .
– peter176
Nov 15 '18 at 17:19
Can you show both the reducers and the component's
mapState
function?– markerikson
Nov 15 '18 at 17:34
Yes, I added them to the post
– peter176
Nov 15 '18 at 17:47