Fail to autowire dependency in test
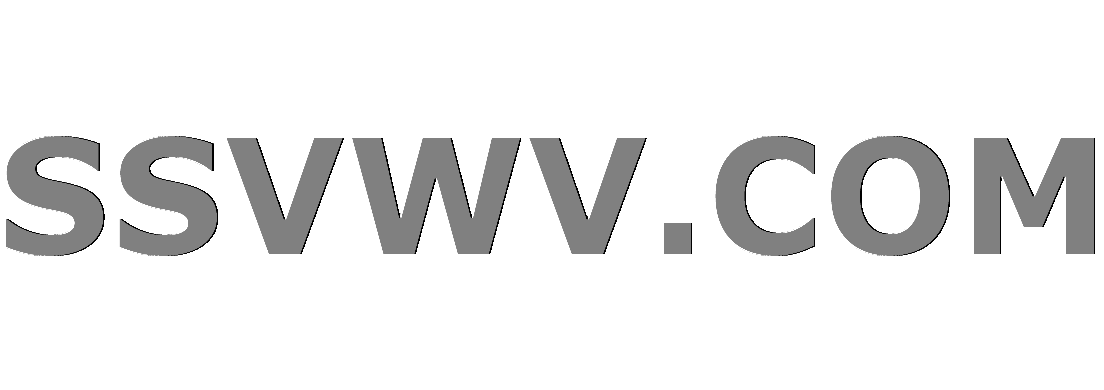
Multi tool use
I'd like to write a Spring integration test to make sure that @Autowired
correctly puts together my classes, but fail.
The test class
@RunWith(SpringRunner.class)
@DataJpaTest
@EntityScan(basePackageClasses = {ClassUnderTest.class, SomRepository.class, SomeEntity.class})
public class ClassUnderTestIT{
@Autowired private InterfaceUnderTest cut;
@Test public void autowires() {
assertThat(cut).isNotNull();
}
}
Should test that this @Service
autowires
@Service
@Transactional
public class ClassUnderTest implements InterfaceUnderTest {
private final SomeRepository repository;
@Autowired
public DefaultWatchlistDataModifier(SomeRepository repository) {
this.repository = repository;
}
}
Paying special attention to the wired dependency
@Repository
@Transactional(readOnly = true)
@Scope(value = BeanDefinition.SCOPE_PROTOTYPE)
public interface SomeRepository extends JpaRepository<SomeEntity, String> {
}
However, all I ever get is the exception
org.springframework.beans.factory.NoSuchBeanDefinitionException:
No qualifying bean of type 'com.[...].InterfaceUnderTest' available:
expected at least 1 bean which qualifies as autowire candidate.
Dependency annotations:
{@org.springframework.beans.factory.annotation.Autowired(required=true)}
Meanwhile, I have experimented with all kinds of additional annotations, such as @ComponentScan
, @EnableJpaRepositories
... whatever I could dig up in the endless number of StackOverflow questions on the topic - all in vain.
spring autowired spring-test
add a comment |
I'd like to write a Spring integration test to make sure that @Autowired
correctly puts together my classes, but fail.
The test class
@RunWith(SpringRunner.class)
@DataJpaTest
@EntityScan(basePackageClasses = {ClassUnderTest.class, SomRepository.class, SomeEntity.class})
public class ClassUnderTestIT{
@Autowired private InterfaceUnderTest cut;
@Test public void autowires() {
assertThat(cut).isNotNull();
}
}
Should test that this @Service
autowires
@Service
@Transactional
public class ClassUnderTest implements InterfaceUnderTest {
private final SomeRepository repository;
@Autowired
public DefaultWatchlistDataModifier(SomeRepository repository) {
this.repository = repository;
}
}
Paying special attention to the wired dependency
@Repository
@Transactional(readOnly = true)
@Scope(value = BeanDefinition.SCOPE_PROTOTYPE)
public interface SomeRepository extends JpaRepository<SomeEntity, String> {
}
However, all I ever get is the exception
org.springframework.beans.factory.NoSuchBeanDefinitionException:
No qualifying bean of type 'com.[...].InterfaceUnderTest' available:
expected at least 1 bean which qualifies as autowire candidate.
Dependency annotations:
{@org.springframework.beans.factory.annotation.Autowired(required=true)}
Meanwhile, I have experimented with all kinds of additional annotations, such as @ComponentScan
, @EnableJpaRepositories
... whatever I could dig up in the endless number of StackOverflow questions on the topic - all in vain.
spring autowired spring-test
@DataJpaTest
only bootstraps JPA related classes. Your class you want to test isn't a JPA class and hence isn't detected. You need an@SpringBootTest
in this case. Also@EntityScan
here doesn't make any sense either.
– M. Deinum
Nov 15 '18 at 8:27
Blimey, you're perfectly right, of course! Mind making this an answer I can vote up?
– Florian
Nov 15 '18 at 8:33
add a comment |
I'd like to write a Spring integration test to make sure that @Autowired
correctly puts together my classes, but fail.
The test class
@RunWith(SpringRunner.class)
@DataJpaTest
@EntityScan(basePackageClasses = {ClassUnderTest.class, SomRepository.class, SomeEntity.class})
public class ClassUnderTestIT{
@Autowired private InterfaceUnderTest cut;
@Test public void autowires() {
assertThat(cut).isNotNull();
}
}
Should test that this @Service
autowires
@Service
@Transactional
public class ClassUnderTest implements InterfaceUnderTest {
private final SomeRepository repository;
@Autowired
public DefaultWatchlistDataModifier(SomeRepository repository) {
this.repository = repository;
}
}
Paying special attention to the wired dependency
@Repository
@Transactional(readOnly = true)
@Scope(value = BeanDefinition.SCOPE_PROTOTYPE)
public interface SomeRepository extends JpaRepository<SomeEntity, String> {
}
However, all I ever get is the exception
org.springframework.beans.factory.NoSuchBeanDefinitionException:
No qualifying bean of type 'com.[...].InterfaceUnderTest' available:
expected at least 1 bean which qualifies as autowire candidate.
Dependency annotations:
{@org.springframework.beans.factory.annotation.Autowired(required=true)}
Meanwhile, I have experimented with all kinds of additional annotations, such as @ComponentScan
, @EnableJpaRepositories
... whatever I could dig up in the endless number of StackOverflow questions on the topic - all in vain.
spring autowired spring-test
I'd like to write a Spring integration test to make sure that @Autowired
correctly puts together my classes, but fail.
The test class
@RunWith(SpringRunner.class)
@DataJpaTest
@EntityScan(basePackageClasses = {ClassUnderTest.class, SomRepository.class, SomeEntity.class})
public class ClassUnderTestIT{
@Autowired private InterfaceUnderTest cut;
@Test public void autowires() {
assertThat(cut).isNotNull();
}
}
Should test that this @Service
autowires
@Service
@Transactional
public class ClassUnderTest implements InterfaceUnderTest {
private final SomeRepository repository;
@Autowired
public DefaultWatchlistDataModifier(SomeRepository repository) {
this.repository = repository;
}
}
Paying special attention to the wired dependency
@Repository
@Transactional(readOnly = true)
@Scope(value = BeanDefinition.SCOPE_PROTOTYPE)
public interface SomeRepository extends JpaRepository<SomeEntity, String> {
}
However, all I ever get is the exception
org.springframework.beans.factory.NoSuchBeanDefinitionException:
No qualifying bean of type 'com.[...].InterfaceUnderTest' available:
expected at least 1 bean which qualifies as autowire candidate.
Dependency annotations:
{@org.springframework.beans.factory.annotation.Autowired(required=true)}
Meanwhile, I have experimented with all kinds of additional annotations, such as @ComponentScan
, @EnableJpaRepositories
... whatever I could dig up in the endless number of StackOverflow questions on the topic - all in vain.
spring autowired spring-test
spring autowired spring-test
asked Nov 15 '18 at 8:25


FlorianFlorian
1,575619
1,575619
@DataJpaTest
only bootstraps JPA related classes. Your class you want to test isn't a JPA class and hence isn't detected. You need an@SpringBootTest
in this case. Also@EntityScan
here doesn't make any sense either.
– M. Deinum
Nov 15 '18 at 8:27
Blimey, you're perfectly right, of course! Mind making this an answer I can vote up?
– Florian
Nov 15 '18 at 8:33
add a comment |
@DataJpaTest
only bootstraps JPA related classes. Your class you want to test isn't a JPA class and hence isn't detected. You need an@SpringBootTest
in this case. Also@EntityScan
here doesn't make any sense either.
– M. Deinum
Nov 15 '18 at 8:27
Blimey, you're perfectly right, of course! Mind making this an answer I can vote up?
– Florian
Nov 15 '18 at 8:33
@DataJpaTest
only bootstraps JPA related classes. Your class you want to test isn't a JPA class and hence isn't detected. You need an @SpringBootTest
in this case. Also @EntityScan
here doesn't make any sense either.– M. Deinum
Nov 15 '18 at 8:27
@DataJpaTest
only bootstraps JPA related classes. Your class you want to test isn't a JPA class and hence isn't detected. You need an @SpringBootTest
in this case. Also @EntityScan
here doesn't make any sense either.– M. Deinum
Nov 15 '18 at 8:27
Blimey, you're perfectly right, of course! Mind making this an answer I can vote up?
– Florian
Nov 15 '18 at 8:33
Blimey, you're perfectly right, of course! Mind making this an answer I can vote up?
– Florian
Nov 15 '18 at 8:33
add a comment |
1 Answer
1
active
oldest
votes
Using [@DataJpaTest
] will only bootstrap the JPA part of your Spring Boot application. As the unit of your test isn't part of that subset it will not be available in the application context.
Either construct it yourself and inject the dependencies or use a full blown @SpringBootTest
instead.
1
Full-blown Spring test it is then. Injecting mocks is exactly what I don't want to do here. On the test class, removed@DataJpaTest
and@EntityScan
and added@SpringBootTest
instead. That fixed it. Thanks!
– Florian
Nov 15 '18 at 9:33
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53315140%2ffail-to-autowire-dependency-in-test%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Using [@DataJpaTest
] will only bootstrap the JPA part of your Spring Boot application. As the unit of your test isn't part of that subset it will not be available in the application context.
Either construct it yourself and inject the dependencies or use a full blown @SpringBootTest
instead.
1
Full-blown Spring test it is then. Injecting mocks is exactly what I don't want to do here. On the test class, removed@DataJpaTest
and@EntityScan
and added@SpringBootTest
instead. That fixed it. Thanks!
– Florian
Nov 15 '18 at 9:33
add a comment |
Using [@DataJpaTest
] will only bootstrap the JPA part of your Spring Boot application. As the unit of your test isn't part of that subset it will not be available in the application context.
Either construct it yourself and inject the dependencies or use a full blown @SpringBootTest
instead.
1
Full-blown Spring test it is then. Injecting mocks is exactly what I don't want to do here. On the test class, removed@DataJpaTest
and@EntityScan
and added@SpringBootTest
instead. That fixed it. Thanks!
– Florian
Nov 15 '18 at 9:33
add a comment |
Using [@DataJpaTest
] will only bootstrap the JPA part of your Spring Boot application. As the unit of your test isn't part of that subset it will not be available in the application context.
Either construct it yourself and inject the dependencies or use a full blown @SpringBootTest
instead.
Using [@DataJpaTest
] will only bootstrap the JPA part of your Spring Boot application. As the unit of your test isn't part of that subset it will not be available in the application context.
Either construct it yourself and inject the dependencies or use a full blown @SpringBootTest
instead.
answered Nov 15 '18 at 9:27


M. DeinumM. Deinum
69.9k13140149
69.9k13140149
1
Full-blown Spring test it is then. Injecting mocks is exactly what I don't want to do here. On the test class, removed@DataJpaTest
and@EntityScan
and added@SpringBootTest
instead. That fixed it. Thanks!
– Florian
Nov 15 '18 at 9:33
add a comment |
1
Full-blown Spring test it is then. Injecting mocks is exactly what I don't want to do here. On the test class, removed@DataJpaTest
and@EntityScan
and added@SpringBootTest
instead. That fixed it. Thanks!
– Florian
Nov 15 '18 at 9:33
1
1
Full-blown Spring test it is then. Injecting mocks is exactly what I don't want to do here. On the test class, removed
@DataJpaTest
and @EntityScan
and added @SpringBootTest
instead. That fixed it. Thanks!– Florian
Nov 15 '18 at 9:33
Full-blown Spring test it is then. Injecting mocks is exactly what I don't want to do here. On the test class, removed
@DataJpaTest
and @EntityScan
and added @SpringBootTest
instead. That fixed it. Thanks!– Florian
Nov 15 '18 at 9:33
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53315140%2ffail-to-autowire-dependency-in-test%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
F,9t8aiPF
@DataJpaTest
only bootstraps JPA related classes. Your class you want to test isn't a JPA class and hence isn't detected. You need an@SpringBootTest
in this case. Also@EntityScan
here doesn't make any sense either.– M. Deinum
Nov 15 '18 at 8:27
Blimey, you're perfectly right, of course! Mind making this an answer I can vote up?
– Florian
Nov 15 '18 at 8:33