Seven segment digits clock with the system time in python Tkinter with strftime
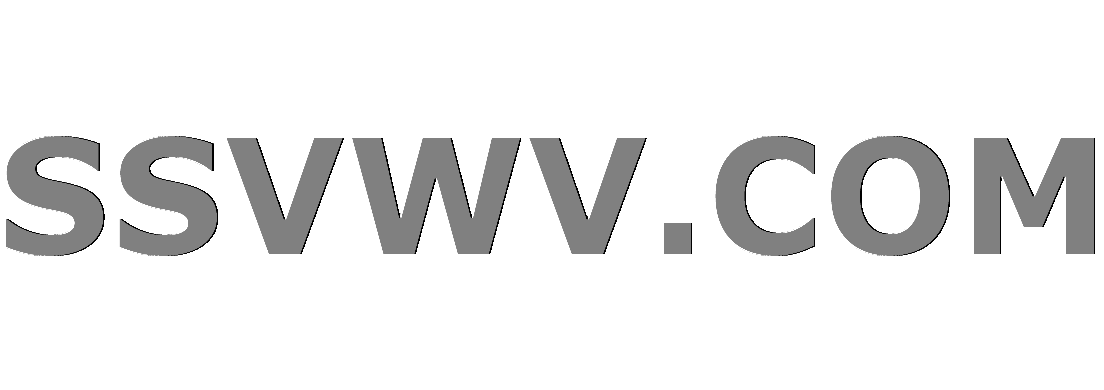
Multi tool use
I need a seven-segment clock with time.strftime
. I have this for the clock:
import sys
from tkinter import *
import time
def tick():
time_string = time.strftime("%M:%S")
clock.config(text=time_string)
clock.after(200, tick)
root = Tk()
clock = Label(root, font = ("times", 250, "bold"), bg="white")
clock.grid(row=0, column=1)
tick()
root.mainloop()
And this for the digits:
offsets = (
(0, 0, 1, 0), # top
(1, 0, 1, 1), # upper right
(1, 1, 1, 2), # lower right
(0, 2, 1, 2), # bottom
(0, 1, 0, 2), # lower left
(0, 0, 0, 1), # upper left
(0, 1, 1, 1), # middle
)
digits = (
(1, 1, 1, 1, 1, 1, 0), # 0
(0, 1, 1, 0, 0, 0, 0), # 1
(1, 1, 0, 1, 1, 0, 1), # 2
(1, 1, 1, 1, 0, 0, 1), # 3
(0, 1, 1, 0, 0, 1, 1), # 4
(1, 0, 1, 1, 0, 1, 1), # 5
(1, 0, 1, 1, 1, 1, 1), # 6
(1, 1, 1, 0, 0, 0, 0), # 7
(1, 1, 1, 1, 1, 1, 1), # 8
(1, 1, 1, 1, 0, 1, 1), # 9
(1, 1, 1, 0, 1, 1, 1), # 10=A
(0, 0, 1, 1, 1, 1, 1), # 11=b
(1, 0, 0, 1, 1, 1, 0), # 12=C
(0, 1, 1, 1, 1, 0, 1), # 13=d
(1, 0, 0, 1, 1, 1, 1), # 14=E
(1, 0, 0, 0, 1, 1, 1), # 15=F
)
class Digit:
def __init__(self, canvas, *, x=10, y=10, length=20, width=3):
self.canvas = canvas
l = length
self.segs =
for x0, y0, x1, y1 in offsets:
self.segs.append(canvas.create_line(
x + x0*l, y + y0*l, x + x1*l, y + y1*l,
width=width, state = 'hidden'))
def show(self, num):
for iid, on in zip(self.segs, digits[num]):
self.canvas.itemconfigure(iid, state = 'normal' if on else 'hidden')
But, I have no idea how to combine them.
Any improvements of the actual code will be appreciated, and if you find that something should be removed or replaced, feel free to do the change. I don't need to use this exact implementation, but this is all I have. If there is a function in a library or something to make the job a hole lot easier, please let me know.
python python-2.7 tkinter
add a comment |
I need a seven-segment clock with time.strftime
. I have this for the clock:
import sys
from tkinter import *
import time
def tick():
time_string = time.strftime("%M:%S")
clock.config(text=time_string)
clock.after(200, tick)
root = Tk()
clock = Label(root, font = ("times", 250, "bold"), bg="white")
clock.grid(row=0, column=1)
tick()
root.mainloop()
And this for the digits:
offsets = (
(0, 0, 1, 0), # top
(1, 0, 1, 1), # upper right
(1, 1, 1, 2), # lower right
(0, 2, 1, 2), # bottom
(0, 1, 0, 2), # lower left
(0, 0, 0, 1), # upper left
(0, 1, 1, 1), # middle
)
digits = (
(1, 1, 1, 1, 1, 1, 0), # 0
(0, 1, 1, 0, 0, 0, 0), # 1
(1, 1, 0, 1, 1, 0, 1), # 2
(1, 1, 1, 1, 0, 0, 1), # 3
(0, 1, 1, 0, 0, 1, 1), # 4
(1, 0, 1, 1, 0, 1, 1), # 5
(1, 0, 1, 1, 1, 1, 1), # 6
(1, 1, 1, 0, 0, 0, 0), # 7
(1, 1, 1, 1, 1, 1, 1), # 8
(1, 1, 1, 1, 0, 1, 1), # 9
(1, 1, 1, 0, 1, 1, 1), # 10=A
(0, 0, 1, 1, 1, 1, 1), # 11=b
(1, 0, 0, 1, 1, 1, 0), # 12=C
(0, 1, 1, 1, 1, 0, 1), # 13=d
(1, 0, 0, 1, 1, 1, 1), # 14=E
(1, 0, 0, 0, 1, 1, 1), # 15=F
)
class Digit:
def __init__(self, canvas, *, x=10, y=10, length=20, width=3):
self.canvas = canvas
l = length
self.segs =
for x0, y0, x1, y1 in offsets:
self.segs.append(canvas.create_line(
x + x0*l, y + y0*l, x + x1*l, y + y1*l,
width=width, state = 'hidden'))
def show(self, num):
for iid, on in zip(self.segs, digits[num]):
self.canvas.itemconfigure(iid, state = 'normal' if on else 'hidden')
But, I have no idea how to combine them.
Any improvements of the actual code will be appreciated, and if you find that something should be removed or replaced, feel free to do the change. I don't need to use this exact implementation, but this is all I have. If there is a function in a library or something to make the job a hole lot easier, please let me know.
python python-2.7 tkinter
Why can you not use the regular time-strafe in tkinter? Are you trying to do something with arduino?
– Mike - SMT
Nov 14 '18 at 16:19
Possible duplicate of Seven segment display in Tkinter
– Mike - SMT
Nov 14 '18 at 16:23
I needed the seven-segment because I have an other opencv script to test a video camera if it recognizes a digital clock. I've got the solution in the answer below though.
– Angelo Catana
Nov 14 '18 at 17:37
add a comment |
I need a seven-segment clock with time.strftime
. I have this for the clock:
import sys
from tkinter import *
import time
def tick():
time_string = time.strftime("%M:%S")
clock.config(text=time_string)
clock.after(200, tick)
root = Tk()
clock = Label(root, font = ("times", 250, "bold"), bg="white")
clock.grid(row=0, column=1)
tick()
root.mainloop()
And this for the digits:
offsets = (
(0, 0, 1, 0), # top
(1, 0, 1, 1), # upper right
(1, 1, 1, 2), # lower right
(0, 2, 1, 2), # bottom
(0, 1, 0, 2), # lower left
(0, 0, 0, 1), # upper left
(0, 1, 1, 1), # middle
)
digits = (
(1, 1, 1, 1, 1, 1, 0), # 0
(0, 1, 1, 0, 0, 0, 0), # 1
(1, 1, 0, 1, 1, 0, 1), # 2
(1, 1, 1, 1, 0, 0, 1), # 3
(0, 1, 1, 0, 0, 1, 1), # 4
(1, 0, 1, 1, 0, 1, 1), # 5
(1, 0, 1, 1, 1, 1, 1), # 6
(1, 1, 1, 0, 0, 0, 0), # 7
(1, 1, 1, 1, 1, 1, 1), # 8
(1, 1, 1, 1, 0, 1, 1), # 9
(1, 1, 1, 0, 1, 1, 1), # 10=A
(0, 0, 1, 1, 1, 1, 1), # 11=b
(1, 0, 0, 1, 1, 1, 0), # 12=C
(0, 1, 1, 1, 1, 0, 1), # 13=d
(1, 0, 0, 1, 1, 1, 1), # 14=E
(1, 0, 0, 0, 1, 1, 1), # 15=F
)
class Digit:
def __init__(self, canvas, *, x=10, y=10, length=20, width=3):
self.canvas = canvas
l = length
self.segs =
for x0, y0, x1, y1 in offsets:
self.segs.append(canvas.create_line(
x + x0*l, y + y0*l, x + x1*l, y + y1*l,
width=width, state = 'hidden'))
def show(self, num):
for iid, on in zip(self.segs, digits[num]):
self.canvas.itemconfigure(iid, state = 'normal' if on else 'hidden')
But, I have no idea how to combine them.
Any improvements of the actual code will be appreciated, and if you find that something should be removed or replaced, feel free to do the change. I don't need to use this exact implementation, but this is all I have. If there is a function in a library or something to make the job a hole lot easier, please let me know.
python python-2.7 tkinter
I need a seven-segment clock with time.strftime
. I have this for the clock:
import sys
from tkinter import *
import time
def tick():
time_string = time.strftime("%M:%S")
clock.config(text=time_string)
clock.after(200, tick)
root = Tk()
clock = Label(root, font = ("times", 250, "bold"), bg="white")
clock.grid(row=0, column=1)
tick()
root.mainloop()
And this for the digits:
offsets = (
(0, 0, 1, 0), # top
(1, 0, 1, 1), # upper right
(1, 1, 1, 2), # lower right
(0, 2, 1, 2), # bottom
(0, 1, 0, 2), # lower left
(0, 0, 0, 1), # upper left
(0, 1, 1, 1), # middle
)
digits = (
(1, 1, 1, 1, 1, 1, 0), # 0
(0, 1, 1, 0, 0, 0, 0), # 1
(1, 1, 0, 1, 1, 0, 1), # 2
(1, 1, 1, 1, 0, 0, 1), # 3
(0, 1, 1, 0, 0, 1, 1), # 4
(1, 0, 1, 1, 0, 1, 1), # 5
(1, 0, 1, 1, 1, 1, 1), # 6
(1, 1, 1, 0, 0, 0, 0), # 7
(1, 1, 1, 1, 1, 1, 1), # 8
(1, 1, 1, 1, 0, 1, 1), # 9
(1, 1, 1, 0, 1, 1, 1), # 10=A
(0, 0, 1, 1, 1, 1, 1), # 11=b
(1, 0, 0, 1, 1, 1, 0), # 12=C
(0, 1, 1, 1, 1, 0, 1), # 13=d
(1, 0, 0, 1, 1, 1, 1), # 14=E
(1, 0, 0, 0, 1, 1, 1), # 15=F
)
class Digit:
def __init__(self, canvas, *, x=10, y=10, length=20, width=3):
self.canvas = canvas
l = length
self.segs =
for x0, y0, x1, y1 in offsets:
self.segs.append(canvas.create_line(
x + x0*l, y + y0*l, x + x1*l, y + y1*l,
width=width, state = 'hidden'))
def show(self, num):
for iid, on in zip(self.segs, digits[num]):
self.canvas.itemconfigure(iid, state = 'normal' if on else 'hidden')
But, I have no idea how to combine them.
Any improvements of the actual code will be appreciated, and if you find that something should be removed or replaced, feel free to do the change. I don't need to use this exact implementation, but this is all I have. If there is a function in a library or something to make the job a hole lot easier, please let me know.
python python-2.7 tkinter
python python-2.7 tkinter
edited Nov 14 '18 at 17:46
Angelo Catana
asked Nov 14 '18 at 16:11


Angelo CatanaAngelo Catana
1514
1514
Why can you not use the regular time-strafe in tkinter? Are you trying to do something with arduino?
– Mike - SMT
Nov 14 '18 at 16:19
Possible duplicate of Seven segment display in Tkinter
– Mike - SMT
Nov 14 '18 at 16:23
I needed the seven-segment because I have an other opencv script to test a video camera if it recognizes a digital clock. I've got the solution in the answer below though.
– Angelo Catana
Nov 14 '18 at 17:37
add a comment |
Why can you not use the regular time-strafe in tkinter? Are you trying to do something with arduino?
– Mike - SMT
Nov 14 '18 at 16:19
Possible duplicate of Seven segment display in Tkinter
– Mike - SMT
Nov 14 '18 at 16:23
I needed the seven-segment because I have an other opencv script to test a video camera if it recognizes a digital clock. I've got the solution in the answer below though.
– Angelo Catana
Nov 14 '18 at 17:37
Why can you not use the regular time-strafe in tkinter? Are you trying to do something with arduino?
– Mike - SMT
Nov 14 '18 at 16:19
Why can you not use the regular time-strafe in tkinter? Are you trying to do something with arduino?
– Mike - SMT
Nov 14 '18 at 16:19
Possible duplicate of Seven segment display in Tkinter
– Mike - SMT
Nov 14 '18 at 16:23
Possible duplicate of Seven segment display in Tkinter
– Mike - SMT
Nov 14 '18 at 16:23
I needed the seven-segment because I have an other opencv script to test a video camera if it recognizes a digital clock. I've got the solution in the answer below though.
– Angelo Catana
Nov 14 '18 at 17:37
I needed the seven-segment because I have an other opencv script to test a video camera if it recognizes a digital clock. I've got the solution in the answer below though.
– Angelo Catana
Nov 14 '18 at 17:37
add a comment |
1 Answer
1
active
oldest
votes
Ok so here is my attempt to convert strftime to a 7 segment clock based on the code you provided.
My goal was to generate a list of canvas's for each digit of the formatted timestrafe string. Then on each lap of the clock simply use the Digit
class to update each canvas item.
Let me know if you have any questions.
import tkinter as tk
import time
# Order 7 segments clockwise from top left, with crossbar last.
# Coordinates of each segment are (x0, y0, x1, y1)
# given as offsets from top left measured in segment lengths.
offsets = (
(0, 0, 1, 0), # top
(1, 0, 1, 1), # upper right
(1, 1, 1, 2), # lower right
(0, 2, 1, 2), # bottom
(0, 1, 0, 2), # lower left
(0, 0, 0, 1), # upper left
(0, 1, 1, 1), # middle
)
# Segments used for each digit; 0, 1 = off, on.
digits = (
(1, 1, 1, 1, 1, 1, 0), # 0
(0, 1, 1, 0, 0, 0, 0), # 1
(1, 1, 0, 1, 1, 0, 1), # 2
(1, 1, 1, 1, 0, 0, 1), # 3
(0, 1, 1, 0, 0, 1, 1), # 4
(1, 0, 1, 1, 0, 1, 1), # 5
(1, 0, 1, 1, 1, 1, 1), # 6
(1, 1, 1, 0, 0, 0, 0), # 7
(1, 1, 1, 1, 1, 1, 1), # 8
(1, 1, 1, 1, 0, 1, 1), # 9
(1, 1, 1, 0, 1, 1, 1), # 10=A
(0, 0, 1, 1, 1, 1, 1), # 11=b
(1, 0, 0, 1, 1, 1, 0), # 12=C
(0, 1, 1, 1, 1, 0, 1), # 13=d
(1, 0, 0, 1, 1, 1, 1), # 14=E
(1, 0, 0, 0, 1, 1, 1), # 15=F
)
class Digit:
def __init__(self, canvas, *, x=10, y=10, l=20, wt=3):
self.canvas = canvas
canvas.delete("all")
self.segs =
for x0, y0, x1, y1 in offsets:
self.segs.append(canvas.create_line(x + x0*l, y + y0*l, x + x1*l, y + y1*l, width=wt, state='hidden'))
def show(self, num):
for iid, on in zip(self.segs, digits[num]):
self.canvas.itemconfigure(iid, state='normal' if on else 'hidden')
def tick():
global canvas_list
for ndex, num in enumerate(time.strftime("%M:%S").replace(':', '')):
Digit(canvas_list[ndex]).show(int(num))
root.after(1000, tick)
root = tk.Tk()
clock_frame = tk.Frame(root)
clock_frame.grid(row=1, column=0)
canvas_list =
time_col = 0
canvas_count = 0
for i in range(5):
if i == 2:
tk.Label(clock_frame, text=":", font = ("times", 40, "bold")).grid(row=0, column=time_col)
time_col += 1
else:
canvas_list.append(tk.Canvas(clock_frame, width=30, height=50))
canvas_list[canvas_count].grid(row=0, column=time_col)
canvas_count += 1
time_col += 1
tick()
root.mainloop()
Keep in mind the OP's code was just looking at min/sec so this clock will reflect that.
Results:
Thank you very much. It works great and it's what i actually needed
– Angelo Catana
Nov 14 '18 at 17:33
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53304437%2fseven-segment-digits-clock-with-the-system-time-in-python-tkinter-with-strftime%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Ok so here is my attempt to convert strftime to a 7 segment clock based on the code you provided.
My goal was to generate a list of canvas's for each digit of the formatted timestrafe string. Then on each lap of the clock simply use the Digit
class to update each canvas item.
Let me know if you have any questions.
import tkinter as tk
import time
# Order 7 segments clockwise from top left, with crossbar last.
# Coordinates of each segment are (x0, y0, x1, y1)
# given as offsets from top left measured in segment lengths.
offsets = (
(0, 0, 1, 0), # top
(1, 0, 1, 1), # upper right
(1, 1, 1, 2), # lower right
(0, 2, 1, 2), # bottom
(0, 1, 0, 2), # lower left
(0, 0, 0, 1), # upper left
(0, 1, 1, 1), # middle
)
# Segments used for each digit; 0, 1 = off, on.
digits = (
(1, 1, 1, 1, 1, 1, 0), # 0
(0, 1, 1, 0, 0, 0, 0), # 1
(1, 1, 0, 1, 1, 0, 1), # 2
(1, 1, 1, 1, 0, 0, 1), # 3
(0, 1, 1, 0, 0, 1, 1), # 4
(1, 0, 1, 1, 0, 1, 1), # 5
(1, 0, 1, 1, 1, 1, 1), # 6
(1, 1, 1, 0, 0, 0, 0), # 7
(1, 1, 1, 1, 1, 1, 1), # 8
(1, 1, 1, 1, 0, 1, 1), # 9
(1, 1, 1, 0, 1, 1, 1), # 10=A
(0, 0, 1, 1, 1, 1, 1), # 11=b
(1, 0, 0, 1, 1, 1, 0), # 12=C
(0, 1, 1, 1, 1, 0, 1), # 13=d
(1, 0, 0, 1, 1, 1, 1), # 14=E
(1, 0, 0, 0, 1, 1, 1), # 15=F
)
class Digit:
def __init__(self, canvas, *, x=10, y=10, l=20, wt=3):
self.canvas = canvas
canvas.delete("all")
self.segs =
for x0, y0, x1, y1 in offsets:
self.segs.append(canvas.create_line(x + x0*l, y + y0*l, x + x1*l, y + y1*l, width=wt, state='hidden'))
def show(self, num):
for iid, on in zip(self.segs, digits[num]):
self.canvas.itemconfigure(iid, state='normal' if on else 'hidden')
def tick():
global canvas_list
for ndex, num in enumerate(time.strftime("%M:%S").replace(':', '')):
Digit(canvas_list[ndex]).show(int(num))
root.after(1000, tick)
root = tk.Tk()
clock_frame = tk.Frame(root)
clock_frame.grid(row=1, column=0)
canvas_list =
time_col = 0
canvas_count = 0
for i in range(5):
if i == 2:
tk.Label(clock_frame, text=":", font = ("times", 40, "bold")).grid(row=0, column=time_col)
time_col += 1
else:
canvas_list.append(tk.Canvas(clock_frame, width=30, height=50))
canvas_list[canvas_count].grid(row=0, column=time_col)
canvas_count += 1
time_col += 1
tick()
root.mainloop()
Keep in mind the OP's code was just looking at min/sec so this clock will reflect that.
Results:
Thank you very much. It works great and it's what i actually needed
– Angelo Catana
Nov 14 '18 at 17:33
add a comment |
Ok so here is my attempt to convert strftime to a 7 segment clock based on the code you provided.
My goal was to generate a list of canvas's for each digit of the formatted timestrafe string. Then on each lap of the clock simply use the Digit
class to update each canvas item.
Let me know if you have any questions.
import tkinter as tk
import time
# Order 7 segments clockwise from top left, with crossbar last.
# Coordinates of each segment are (x0, y0, x1, y1)
# given as offsets from top left measured in segment lengths.
offsets = (
(0, 0, 1, 0), # top
(1, 0, 1, 1), # upper right
(1, 1, 1, 2), # lower right
(0, 2, 1, 2), # bottom
(0, 1, 0, 2), # lower left
(0, 0, 0, 1), # upper left
(0, 1, 1, 1), # middle
)
# Segments used for each digit; 0, 1 = off, on.
digits = (
(1, 1, 1, 1, 1, 1, 0), # 0
(0, 1, 1, 0, 0, 0, 0), # 1
(1, 1, 0, 1, 1, 0, 1), # 2
(1, 1, 1, 1, 0, 0, 1), # 3
(0, 1, 1, 0, 0, 1, 1), # 4
(1, 0, 1, 1, 0, 1, 1), # 5
(1, 0, 1, 1, 1, 1, 1), # 6
(1, 1, 1, 0, 0, 0, 0), # 7
(1, 1, 1, 1, 1, 1, 1), # 8
(1, 1, 1, 1, 0, 1, 1), # 9
(1, 1, 1, 0, 1, 1, 1), # 10=A
(0, 0, 1, 1, 1, 1, 1), # 11=b
(1, 0, 0, 1, 1, 1, 0), # 12=C
(0, 1, 1, 1, 1, 0, 1), # 13=d
(1, 0, 0, 1, 1, 1, 1), # 14=E
(1, 0, 0, 0, 1, 1, 1), # 15=F
)
class Digit:
def __init__(self, canvas, *, x=10, y=10, l=20, wt=3):
self.canvas = canvas
canvas.delete("all")
self.segs =
for x0, y0, x1, y1 in offsets:
self.segs.append(canvas.create_line(x + x0*l, y + y0*l, x + x1*l, y + y1*l, width=wt, state='hidden'))
def show(self, num):
for iid, on in zip(self.segs, digits[num]):
self.canvas.itemconfigure(iid, state='normal' if on else 'hidden')
def tick():
global canvas_list
for ndex, num in enumerate(time.strftime("%M:%S").replace(':', '')):
Digit(canvas_list[ndex]).show(int(num))
root.after(1000, tick)
root = tk.Tk()
clock_frame = tk.Frame(root)
clock_frame.grid(row=1, column=0)
canvas_list =
time_col = 0
canvas_count = 0
for i in range(5):
if i == 2:
tk.Label(clock_frame, text=":", font = ("times", 40, "bold")).grid(row=0, column=time_col)
time_col += 1
else:
canvas_list.append(tk.Canvas(clock_frame, width=30, height=50))
canvas_list[canvas_count].grid(row=0, column=time_col)
canvas_count += 1
time_col += 1
tick()
root.mainloop()
Keep in mind the OP's code was just looking at min/sec so this clock will reflect that.
Results:
Thank you very much. It works great and it's what i actually needed
– Angelo Catana
Nov 14 '18 at 17:33
add a comment |
Ok so here is my attempt to convert strftime to a 7 segment clock based on the code you provided.
My goal was to generate a list of canvas's for each digit of the formatted timestrafe string. Then on each lap of the clock simply use the Digit
class to update each canvas item.
Let me know if you have any questions.
import tkinter as tk
import time
# Order 7 segments clockwise from top left, with crossbar last.
# Coordinates of each segment are (x0, y0, x1, y1)
# given as offsets from top left measured in segment lengths.
offsets = (
(0, 0, 1, 0), # top
(1, 0, 1, 1), # upper right
(1, 1, 1, 2), # lower right
(0, 2, 1, 2), # bottom
(0, 1, 0, 2), # lower left
(0, 0, 0, 1), # upper left
(0, 1, 1, 1), # middle
)
# Segments used for each digit; 0, 1 = off, on.
digits = (
(1, 1, 1, 1, 1, 1, 0), # 0
(0, 1, 1, 0, 0, 0, 0), # 1
(1, 1, 0, 1, 1, 0, 1), # 2
(1, 1, 1, 1, 0, 0, 1), # 3
(0, 1, 1, 0, 0, 1, 1), # 4
(1, 0, 1, 1, 0, 1, 1), # 5
(1, 0, 1, 1, 1, 1, 1), # 6
(1, 1, 1, 0, 0, 0, 0), # 7
(1, 1, 1, 1, 1, 1, 1), # 8
(1, 1, 1, 1, 0, 1, 1), # 9
(1, 1, 1, 0, 1, 1, 1), # 10=A
(0, 0, 1, 1, 1, 1, 1), # 11=b
(1, 0, 0, 1, 1, 1, 0), # 12=C
(0, 1, 1, 1, 1, 0, 1), # 13=d
(1, 0, 0, 1, 1, 1, 1), # 14=E
(1, 0, 0, 0, 1, 1, 1), # 15=F
)
class Digit:
def __init__(self, canvas, *, x=10, y=10, l=20, wt=3):
self.canvas = canvas
canvas.delete("all")
self.segs =
for x0, y0, x1, y1 in offsets:
self.segs.append(canvas.create_line(x + x0*l, y + y0*l, x + x1*l, y + y1*l, width=wt, state='hidden'))
def show(self, num):
for iid, on in zip(self.segs, digits[num]):
self.canvas.itemconfigure(iid, state='normal' if on else 'hidden')
def tick():
global canvas_list
for ndex, num in enumerate(time.strftime("%M:%S").replace(':', '')):
Digit(canvas_list[ndex]).show(int(num))
root.after(1000, tick)
root = tk.Tk()
clock_frame = tk.Frame(root)
clock_frame.grid(row=1, column=0)
canvas_list =
time_col = 0
canvas_count = 0
for i in range(5):
if i == 2:
tk.Label(clock_frame, text=":", font = ("times", 40, "bold")).grid(row=0, column=time_col)
time_col += 1
else:
canvas_list.append(tk.Canvas(clock_frame, width=30, height=50))
canvas_list[canvas_count].grid(row=0, column=time_col)
canvas_count += 1
time_col += 1
tick()
root.mainloop()
Keep in mind the OP's code was just looking at min/sec so this clock will reflect that.
Results:
Ok so here is my attempt to convert strftime to a 7 segment clock based on the code you provided.
My goal was to generate a list of canvas's for each digit of the formatted timestrafe string. Then on each lap of the clock simply use the Digit
class to update each canvas item.
Let me know if you have any questions.
import tkinter as tk
import time
# Order 7 segments clockwise from top left, with crossbar last.
# Coordinates of each segment are (x0, y0, x1, y1)
# given as offsets from top left measured in segment lengths.
offsets = (
(0, 0, 1, 0), # top
(1, 0, 1, 1), # upper right
(1, 1, 1, 2), # lower right
(0, 2, 1, 2), # bottom
(0, 1, 0, 2), # lower left
(0, 0, 0, 1), # upper left
(0, 1, 1, 1), # middle
)
# Segments used for each digit; 0, 1 = off, on.
digits = (
(1, 1, 1, 1, 1, 1, 0), # 0
(0, 1, 1, 0, 0, 0, 0), # 1
(1, 1, 0, 1, 1, 0, 1), # 2
(1, 1, 1, 1, 0, 0, 1), # 3
(0, 1, 1, 0, 0, 1, 1), # 4
(1, 0, 1, 1, 0, 1, 1), # 5
(1, 0, 1, 1, 1, 1, 1), # 6
(1, 1, 1, 0, 0, 0, 0), # 7
(1, 1, 1, 1, 1, 1, 1), # 8
(1, 1, 1, 1, 0, 1, 1), # 9
(1, 1, 1, 0, 1, 1, 1), # 10=A
(0, 0, 1, 1, 1, 1, 1), # 11=b
(1, 0, 0, 1, 1, 1, 0), # 12=C
(0, 1, 1, 1, 1, 0, 1), # 13=d
(1, 0, 0, 1, 1, 1, 1), # 14=E
(1, 0, 0, 0, 1, 1, 1), # 15=F
)
class Digit:
def __init__(self, canvas, *, x=10, y=10, l=20, wt=3):
self.canvas = canvas
canvas.delete("all")
self.segs =
for x0, y0, x1, y1 in offsets:
self.segs.append(canvas.create_line(x + x0*l, y + y0*l, x + x1*l, y + y1*l, width=wt, state='hidden'))
def show(self, num):
for iid, on in zip(self.segs, digits[num]):
self.canvas.itemconfigure(iid, state='normal' if on else 'hidden')
def tick():
global canvas_list
for ndex, num in enumerate(time.strftime("%M:%S").replace(':', '')):
Digit(canvas_list[ndex]).show(int(num))
root.after(1000, tick)
root = tk.Tk()
clock_frame = tk.Frame(root)
clock_frame.grid(row=1, column=0)
canvas_list =
time_col = 0
canvas_count = 0
for i in range(5):
if i == 2:
tk.Label(clock_frame, text=":", font = ("times", 40, "bold")).grid(row=0, column=time_col)
time_col += 1
else:
canvas_list.append(tk.Canvas(clock_frame, width=30, height=50))
canvas_list[canvas_count].grid(row=0, column=time_col)
canvas_count += 1
time_col += 1
tick()
root.mainloop()
Keep in mind the OP's code was just looking at min/sec so this clock will reflect that.
Results:
edited Nov 14 '18 at 17:24
answered Nov 14 '18 at 17:17


Mike - SMTMike - SMT
9,55921434
9,55921434
Thank you very much. It works great and it's what i actually needed
– Angelo Catana
Nov 14 '18 at 17:33
add a comment |
Thank you very much. It works great and it's what i actually needed
– Angelo Catana
Nov 14 '18 at 17:33
Thank you very much. It works great and it's what i actually needed
– Angelo Catana
Nov 14 '18 at 17:33
Thank you very much. It works great and it's what i actually needed
– Angelo Catana
Nov 14 '18 at 17:33
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53304437%2fseven-segment-digits-clock-with-the-system-time-in-python-tkinter-with-strftime%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
3O7u6z 3tZmvregNf3o,wV0kG bct 9B5xIbZ,Z7,hIJdNpWlJ2tUi6B5m
Why can you not use the regular time-strafe in tkinter? Are you trying to do something with arduino?
– Mike - SMT
Nov 14 '18 at 16:19
Possible duplicate of Seven segment display in Tkinter
– Mike - SMT
Nov 14 '18 at 16:23
I needed the seven-segment because I have an other opencv script to test a video camera if it recognizes a digital clock. I've got the solution in the answer below though.
– Angelo Catana
Nov 14 '18 at 17:37