Python 3.7 - Passing parameters to DLL using ctypes
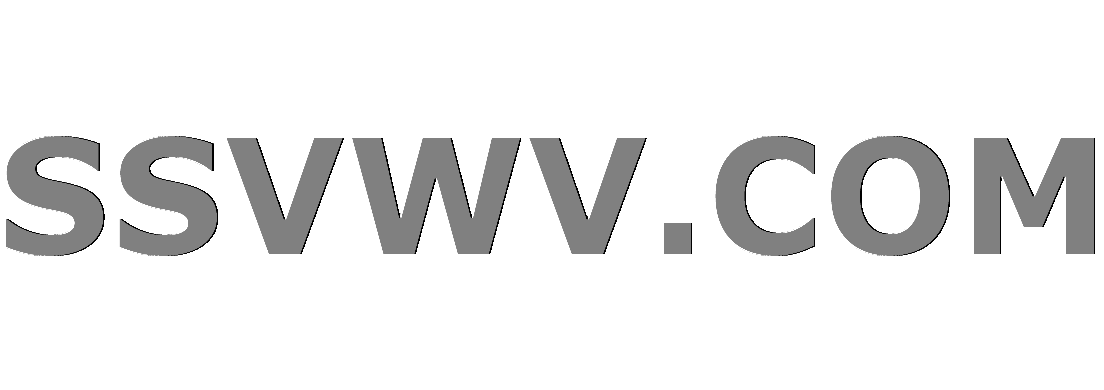
Multi tool use
I have the following code:
num1=10.1234
int1=10
ret=GetOutput(int1,num1)
The function GetOutput is defined as
def GetOutput(int2, num2):
lib = ctypes.WinDLL("mydllname.dll")
prototype = ctypes.WINFUNCTYPE(ctypes.c_int, ctypes.c_int, ctypes.POINTER(ctypes.c_double * 4096))
paramflags = (1, "int2",), (1, "num2",),
GetOutput = prototype(("GetOutput", lib), paramflags)
ret=GetOutput(int2, num2)
return ret
i get the following error and I am requesting your kind help.
LP_c_double_Array_4096 instance instead of float
If I convert num1 as:
num1=list(range(4096))
int1=10
ret=GetOutput(int1,num1)
I get the following error:
LP_c_double_Array_4096 instance instead of list
if I convert the num1 into array as follows:
num1=list(range(4096))
int1=10
for item in num1:
arrayA = array("i", num1)
ret=GetOutput(int1,arrayA)
i get the following errors:
<class 'TypeError'>: expected LP_c_double_Array_4096 instance instead of array.array
Please explain as much as possible as I am new to programming and only have very preliminary/basic understanding of OOPS, lists, tuples, pointers etc and no clue about ctypes.
Thanks
python double ctypes
add a comment |
I have the following code:
num1=10.1234
int1=10
ret=GetOutput(int1,num1)
The function GetOutput is defined as
def GetOutput(int2, num2):
lib = ctypes.WinDLL("mydllname.dll")
prototype = ctypes.WINFUNCTYPE(ctypes.c_int, ctypes.c_int, ctypes.POINTER(ctypes.c_double * 4096))
paramflags = (1, "int2",), (1, "num2",),
GetOutput = prototype(("GetOutput", lib), paramflags)
ret=GetOutput(int2, num2)
return ret
i get the following error and I am requesting your kind help.
LP_c_double_Array_4096 instance instead of float
If I convert num1 as:
num1=list(range(4096))
int1=10
ret=GetOutput(int1,num1)
I get the following error:
LP_c_double_Array_4096 instance instead of list
if I convert the num1 into array as follows:
num1=list(range(4096))
int1=10
for item in num1:
arrayA = array("i", num1)
ret=GetOutput(int1,arrayA)
i get the following errors:
<class 'TypeError'>: expected LP_c_double_Array_4096 instance instead of array.array
Please explain as much as possible as I am new to programming and only have very preliminary/basic understanding of OOPS, lists, tuples, pointers etc and no clue about ctypes.
Thanks
python double ctypes
ctypes can be a little confusing. Are you actually trying to listen to a callback function from you DLL (usingWINFUNCTYPE
)? It seems like you don't actually want to do that, as usually you'd pass a function to theWINFUNCTYPE
object. Can you confirm the signature oflib.GetOutput
?
– 101
Nov 15 '18 at 5:49
What is the C definition ofGetOutput
and what does it do?
– Mark Tolonen
Nov 15 '18 at 7:08
Note: just to avoid confusion don't use the same name (GetOutput) for both functions.
– CristiFati
Nov 15 '18 at 11:40
Thank you all for asking further questions. It made me think and only now I realise that I did not understand the syntax correctly. Briefly, there are two functions and one structure name, all of which are written as "GetOutput". Only the structure needs to be "GetOutput" and the functions can have any other name. I have no way of testing it now from outside office as the code is on a PC connected to an instrument in my office. Will test it out and let you know. Background: I want to send commands to instrument and collect the data from its sensor. Many thanks.
– PK is using Python
Nov 15 '18 at 14:14
add a comment |
I have the following code:
num1=10.1234
int1=10
ret=GetOutput(int1,num1)
The function GetOutput is defined as
def GetOutput(int2, num2):
lib = ctypes.WinDLL("mydllname.dll")
prototype = ctypes.WINFUNCTYPE(ctypes.c_int, ctypes.c_int, ctypes.POINTER(ctypes.c_double * 4096))
paramflags = (1, "int2",), (1, "num2",),
GetOutput = prototype(("GetOutput", lib), paramflags)
ret=GetOutput(int2, num2)
return ret
i get the following error and I am requesting your kind help.
LP_c_double_Array_4096 instance instead of float
If I convert num1 as:
num1=list(range(4096))
int1=10
ret=GetOutput(int1,num1)
I get the following error:
LP_c_double_Array_4096 instance instead of list
if I convert the num1 into array as follows:
num1=list(range(4096))
int1=10
for item in num1:
arrayA = array("i", num1)
ret=GetOutput(int1,arrayA)
i get the following errors:
<class 'TypeError'>: expected LP_c_double_Array_4096 instance instead of array.array
Please explain as much as possible as I am new to programming and only have very preliminary/basic understanding of OOPS, lists, tuples, pointers etc and no clue about ctypes.
Thanks
python double ctypes
I have the following code:
num1=10.1234
int1=10
ret=GetOutput(int1,num1)
The function GetOutput is defined as
def GetOutput(int2, num2):
lib = ctypes.WinDLL("mydllname.dll")
prototype = ctypes.WINFUNCTYPE(ctypes.c_int, ctypes.c_int, ctypes.POINTER(ctypes.c_double * 4096))
paramflags = (1, "int2",), (1, "num2",),
GetOutput = prototype(("GetOutput", lib), paramflags)
ret=GetOutput(int2, num2)
return ret
i get the following error and I am requesting your kind help.
LP_c_double_Array_4096 instance instead of float
If I convert num1 as:
num1=list(range(4096))
int1=10
ret=GetOutput(int1,num1)
I get the following error:
LP_c_double_Array_4096 instance instead of list
if I convert the num1 into array as follows:
num1=list(range(4096))
int1=10
for item in num1:
arrayA = array("i", num1)
ret=GetOutput(int1,arrayA)
i get the following errors:
<class 'TypeError'>: expected LP_c_double_Array_4096 instance instead of array.array
Please explain as much as possible as I am new to programming and only have very preliminary/basic understanding of OOPS, lists, tuples, pointers etc and no clue about ctypes.
Thanks
python double ctypes
python double ctypes
edited Nov 15 '18 at 5:04
PK is using Python
asked Nov 15 '18 at 2:21
PK is using PythonPK is using Python
84
84
ctypes can be a little confusing. Are you actually trying to listen to a callback function from you DLL (usingWINFUNCTYPE
)? It seems like you don't actually want to do that, as usually you'd pass a function to theWINFUNCTYPE
object. Can you confirm the signature oflib.GetOutput
?
– 101
Nov 15 '18 at 5:49
What is the C definition ofGetOutput
and what does it do?
– Mark Tolonen
Nov 15 '18 at 7:08
Note: just to avoid confusion don't use the same name (GetOutput) for both functions.
– CristiFati
Nov 15 '18 at 11:40
Thank you all for asking further questions. It made me think and only now I realise that I did not understand the syntax correctly. Briefly, there are two functions and one structure name, all of which are written as "GetOutput". Only the structure needs to be "GetOutput" and the functions can have any other name. I have no way of testing it now from outside office as the code is on a PC connected to an instrument in my office. Will test it out and let you know. Background: I want to send commands to instrument and collect the data from its sensor. Many thanks.
– PK is using Python
Nov 15 '18 at 14:14
add a comment |
ctypes can be a little confusing. Are you actually trying to listen to a callback function from you DLL (usingWINFUNCTYPE
)? It seems like you don't actually want to do that, as usually you'd pass a function to theWINFUNCTYPE
object. Can you confirm the signature oflib.GetOutput
?
– 101
Nov 15 '18 at 5:49
What is the C definition ofGetOutput
and what does it do?
– Mark Tolonen
Nov 15 '18 at 7:08
Note: just to avoid confusion don't use the same name (GetOutput) for both functions.
– CristiFati
Nov 15 '18 at 11:40
Thank you all for asking further questions. It made me think and only now I realise that I did not understand the syntax correctly. Briefly, there are two functions and one structure name, all of which are written as "GetOutput". Only the structure needs to be "GetOutput" and the functions can have any other name. I have no way of testing it now from outside office as the code is on a PC connected to an instrument in my office. Will test it out and let you know. Background: I want to send commands to instrument and collect the data from its sensor. Many thanks.
– PK is using Python
Nov 15 '18 at 14:14
ctypes can be a little confusing. Are you actually trying to listen to a callback function from you DLL (using
WINFUNCTYPE
)? It seems like you don't actually want to do that, as usually you'd pass a function to the WINFUNCTYPE
object. Can you confirm the signature of lib.GetOutput
?– 101
Nov 15 '18 at 5:49
ctypes can be a little confusing. Are you actually trying to listen to a callback function from you DLL (using
WINFUNCTYPE
)? It seems like you don't actually want to do that, as usually you'd pass a function to the WINFUNCTYPE
object. Can you confirm the signature of lib.GetOutput
?– 101
Nov 15 '18 at 5:49
What is the C definition of
GetOutput
and what does it do?– Mark Tolonen
Nov 15 '18 at 7:08
What is the C definition of
GetOutput
and what does it do?– Mark Tolonen
Nov 15 '18 at 7:08
Note: just to avoid confusion don't use the same name (GetOutput) for both functions.
– CristiFati
Nov 15 '18 at 11:40
Note: just to avoid confusion don't use the same name (GetOutput) for both functions.
– CristiFati
Nov 15 '18 at 11:40
Thank you all for asking further questions. It made me think and only now I realise that I did not understand the syntax correctly. Briefly, there are two functions and one structure name, all of which are written as "GetOutput". Only the structure needs to be "GetOutput" and the functions can have any other name. I have no way of testing it now from outside office as the code is on a PC connected to an instrument in my office. Will test it out and let you know. Background: I want to send commands to instrument and collect the data from its sensor. Many thanks.
– PK is using Python
Nov 15 '18 at 14:14
Thank you all for asking further questions. It made me think and only now I realise that I did not understand the syntax correctly. Briefly, there are two functions and one structure name, all of which are written as "GetOutput". Only the structure needs to be "GetOutput" and the functions can have any other name. I have no way of testing it now from outside office as the code is on a PC connected to an instrument in my office. Will test it out and let you know. Background: I want to send commands to instrument and collect the data from its sensor. Many thanks.
– PK is using Python
Nov 15 '18 at 14:14
add a comment |
1 Answer
1
active
oldest
votes
Thanks all, the following code is working - I have made a mistake in understanding what the dll is doing.
num2 is not an input but an output and hence the paramflags would change. Doing this allows any arbitrary declaration of num1.Since paramflags changed, calling the function Getoutput_Sub requires only one input now. Previously, I got confused by using the same function name.
num1=10.1234
int1=10
ret=GetOutput_Main(int1,num1)
The above is where the function is being called and the below is how the function is defined
def GetOutput_Main(int2, num2):
lib = ctypes.WinDLL("mydllname.dll")
prototype=ctypes.WINFUNCTYPE(ctypes.c_int,ctypes.c_int,ctypes.POINTER(ctypes.c_double * 4096))
paramflags = (1, "int2",), (2, "num2",),
GetOutput_Sub = prototype(("GetOutput", lib),paramflags)
ret=GetOutput_Sub(int2)
return ret
In my comment earlier, I said two functions and a structure. It is actually three functions; two that I am writing and one function (passing a string in quotes) that is standard for the dll.
This still doesn't look quite right. Is it possible to post the C function signature?
– 101
Nov 19 '18 at 2:39
sorry, I do not know how to do that. you are asking for dict/help of something ?
– PK is using Python
Nov 20 '18 at 5:43
Do you have the C source code forGetOutput
?
– 101
Nov 20 '18 at 21:41
No, the dll is provided by equipment manufacturer. Manual is provided about functions and variables.
– PK is using Python
Nov 21 '18 at 3:05
Please see this discussion. stackoverflow.com/questions/53367453/…
– PK is using Python
Nov 21 '18 at 11:15
|
show 2 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53311519%2fpython-3-7-passing-parameters-to-dll-using-ctypes%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks all, the following code is working - I have made a mistake in understanding what the dll is doing.
num2 is not an input but an output and hence the paramflags would change. Doing this allows any arbitrary declaration of num1.Since paramflags changed, calling the function Getoutput_Sub requires only one input now. Previously, I got confused by using the same function name.
num1=10.1234
int1=10
ret=GetOutput_Main(int1,num1)
The above is where the function is being called and the below is how the function is defined
def GetOutput_Main(int2, num2):
lib = ctypes.WinDLL("mydllname.dll")
prototype=ctypes.WINFUNCTYPE(ctypes.c_int,ctypes.c_int,ctypes.POINTER(ctypes.c_double * 4096))
paramflags = (1, "int2",), (2, "num2",),
GetOutput_Sub = prototype(("GetOutput", lib),paramflags)
ret=GetOutput_Sub(int2)
return ret
In my comment earlier, I said two functions and a structure. It is actually three functions; two that I am writing and one function (passing a string in quotes) that is standard for the dll.
This still doesn't look quite right. Is it possible to post the C function signature?
– 101
Nov 19 '18 at 2:39
sorry, I do not know how to do that. you are asking for dict/help of something ?
– PK is using Python
Nov 20 '18 at 5:43
Do you have the C source code forGetOutput
?
– 101
Nov 20 '18 at 21:41
No, the dll is provided by equipment manufacturer. Manual is provided about functions and variables.
– PK is using Python
Nov 21 '18 at 3:05
Please see this discussion. stackoverflow.com/questions/53367453/…
– PK is using Python
Nov 21 '18 at 11:15
|
show 2 more comments
Thanks all, the following code is working - I have made a mistake in understanding what the dll is doing.
num2 is not an input but an output and hence the paramflags would change. Doing this allows any arbitrary declaration of num1.Since paramflags changed, calling the function Getoutput_Sub requires only one input now. Previously, I got confused by using the same function name.
num1=10.1234
int1=10
ret=GetOutput_Main(int1,num1)
The above is where the function is being called and the below is how the function is defined
def GetOutput_Main(int2, num2):
lib = ctypes.WinDLL("mydllname.dll")
prototype=ctypes.WINFUNCTYPE(ctypes.c_int,ctypes.c_int,ctypes.POINTER(ctypes.c_double * 4096))
paramflags = (1, "int2",), (2, "num2",),
GetOutput_Sub = prototype(("GetOutput", lib),paramflags)
ret=GetOutput_Sub(int2)
return ret
In my comment earlier, I said two functions and a structure. It is actually three functions; two that I am writing and one function (passing a string in quotes) that is standard for the dll.
This still doesn't look quite right. Is it possible to post the C function signature?
– 101
Nov 19 '18 at 2:39
sorry, I do not know how to do that. you are asking for dict/help of something ?
– PK is using Python
Nov 20 '18 at 5:43
Do you have the C source code forGetOutput
?
– 101
Nov 20 '18 at 21:41
No, the dll is provided by equipment manufacturer. Manual is provided about functions and variables.
– PK is using Python
Nov 21 '18 at 3:05
Please see this discussion. stackoverflow.com/questions/53367453/…
– PK is using Python
Nov 21 '18 at 11:15
|
show 2 more comments
Thanks all, the following code is working - I have made a mistake in understanding what the dll is doing.
num2 is not an input but an output and hence the paramflags would change. Doing this allows any arbitrary declaration of num1.Since paramflags changed, calling the function Getoutput_Sub requires only one input now. Previously, I got confused by using the same function name.
num1=10.1234
int1=10
ret=GetOutput_Main(int1,num1)
The above is where the function is being called and the below is how the function is defined
def GetOutput_Main(int2, num2):
lib = ctypes.WinDLL("mydllname.dll")
prototype=ctypes.WINFUNCTYPE(ctypes.c_int,ctypes.c_int,ctypes.POINTER(ctypes.c_double * 4096))
paramflags = (1, "int2",), (2, "num2",),
GetOutput_Sub = prototype(("GetOutput", lib),paramflags)
ret=GetOutput_Sub(int2)
return ret
In my comment earlier, I said two functions and a structure. It is actually three functions; two that I am writing and one function (passing a string in quotes) that is standard for the dll.
Thanks all, the following code is working - I have made a mistake in understanding what the dll is doing.
num2 is not an input but an output and hence the paramflags would change. Doing this allows any arbitrary declaration of num1.Since paramflags changed, calling the function Getoutput_Sub requires only one input now. Previously, I got confused by using the same function name.
num1=10.1234
int1=10
ret=GetOutput_Main(int1,num1)
The above is where the function is being called and the below is how the function is defined
def GetOutput_Main(int2, num2):
lib = ctypes.WinDLL("mydllname.dll")
prototype=ctypes.WINFUNCTYPE(ctypes.c_int,ctypes.c_int,ctypes.POINTER(ctypes.c_double * 4096))
paramflags = (1, "int2",), (2, "num2",),
GetOutput_Sub = prototype(("GetOutput", lib),paramflags)
ret=GetOutput_Sub(int2)
return ret
In my comment earlier, I said two functions and a structure. It is actually three functions; two that I am writing and one function (passing a string in quotes) that is standard for the dll.
edited Nov 16 '18 at 6:57
answered Nov 16 '18 at 2:14
PK is using PythonPK is using Python
84
84
This still doesn't look quite right. Is it possible to post the C function signature?
– 101
Nov 19 '18 at 2:39
sorry, I do not know how to do that. you are asking for dict/help of something ?
– PK is using Python
Nov 20 '18 at 5:43
Do you have the C source code forGetOutput
?
– 101
Nov 20 '18 at 21:41
No, the dll is provided by equipment manufacturer. Manual is provided about functions and variables.
– PK is using Python
Nov 21 '18 at 3:05
Please see this discussion. stackoverflow.com/questions/53367453/…
– PK is using Python
Nov 21 '18 at 11:15
|
show 2 more comments
This still doesn't look quite right. Is it possible to post the C function signature?
– 101
Nov 19 '18 at 2:39
sorry, I do not know how to do that. you are asking for dict/help of something ?
– PK is using Python
Nov 20 '18 at 5:43
Do you have the C source code forGetOutput
?
– 101
Nov 20 '18 at 21:41
No, the dll is provided by equipment manufacturer. Manual is provided about functions and variables.
– PK is using Python
Nov 21 '18 at 3:05
Please see this discussion. stackoverflow.com/questions/53367453/…
– PK is using Python
Nov 21 '18 at 11:15
This still doesn't look quite right. Is it possible to post the C function signature?
– 101
Nov 19 '18 at 2:39
This still doesn't look quite right. Is it possible to post the C function signature?
– 101
Nov 19 '18 at 2:39
sorry, I do not know how to do that. you are asking for dict/help of something ?
– PK is using Python
Nov 20 '18 at 5:43
sorry, I do not know how to do that. you are asking for dict/help of something ?
– PK is using Python
Nov 20 '18 at 5:43
Do you have the C source code for
GetOutput
?– 101
Nov 20 '18 at 21:41
Do you have the C source code for
GetOutput
?– 101
Nov 20 '18 at 21:41
No, the dll is provided by equipment manufacturer. Manual is provided about functions and variables.
– PK is using Python
Nov 21 '18 at 3:05
No, the dll is provided by equipment manufacturer. Manual is provided about functions and variables.
– PK is using Python
Nov 21 '18 at 3:05
Please see this discussion. stackoverflow.com/questions/53367453/…
– PK is using Python
Nov 21 '18 at 11:15
Please see this discussion. stackoverflow.com/questions/53367453/…
– PK is using Python
Nov 21 '18 at 11:15
|
show 2 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53311519%2fpython-3-7-passing-parameters-to-dll-using-ctypes%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
W26bXpdk7jmIVR,PY9rGsy9N,ugECAog7pqYb,G 1,pwnt218v MwSMp 9Bt9RjtRmvjqe y0 g g7zZVW0 51,VrUYqz gxBpBi n0BH6pJM
ctypes can be a little confusing. Are you actually trying to listen to a callback function from you DLL (using
WINFUNCTYPE
)? It seems like you don't actually want to do that, as usually you'd pass a function to theWINFUNCTYPE
object. Can you confirm the signature oflib.GetOutput
?– 101
Nov 15 '18 at 5:49
What is the C definition of
GetOutput
and what does it do?– Mark Tolonen
Nov 15 '18 at 7:08
Note: just to avoid confusion don't use the same name (GetOutput) for both functions.
– CristiFati
Nov 15 '18 at 11:40
Thank you all for asking further questions. It made me think and only now I realise that I did not understand the syntax correctly. Briefly, there are two functions and one structure name, all of which are written as "GetOutput". Only the structure needs to be "GetOutput" and the functions can have any other name. I have no way of testing it now from outside office as the code is on a PC connected to an instrument in my office. Will test it out and let you know. Background: I want to send commands to instrument and collect the data from its sensor. Many thanks.
– PK is using Python
Nov 15 '18 at 14:14