JPA using @SQLInsert throws Parameter index out of range
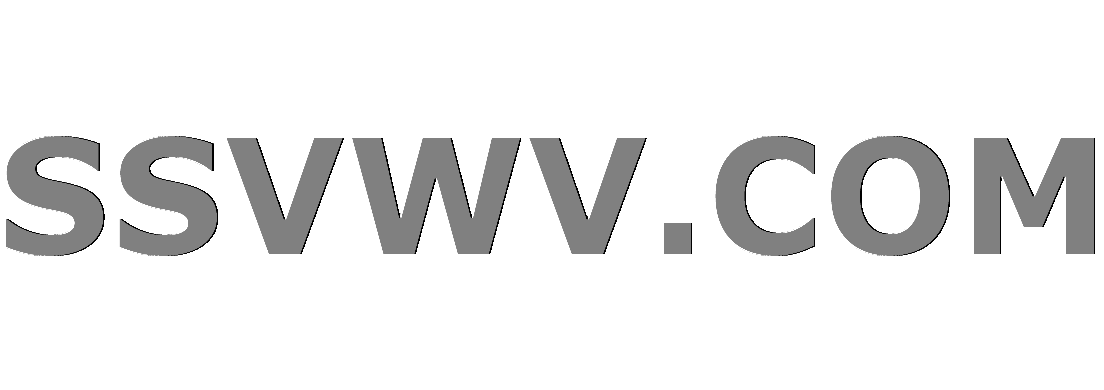
Multi tool use
@Data
@MappedSuperclass
public class BaseModel implements Serializable {
private static final Long serialVersionUID = -1442801573244745790L;
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss")
@Convert(converter = LocalDateTimeConverter.class)
private LocalDateTime createAt = LocalDateTime.now();
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss")
@Convert(converter = LocalDateTimeConverter.class)
private LocalDateTime updateAt = LocalDateTime.now();
}
@Data
@Entity
@Table(name = "tb_vip_code")
@SQLInsert(sql = "insert ignore into tb_vip_code (code, duration) values (?, ?)")
public class VipCode extends BaseModel {
private static final Long serialVersionUID = -4697221755301869573L;
private String code;
private Integer duration;
private Integer status = 0;
private Long userId;
public VipCode() {}
}
@Test
public void addOne() throws Exception {
VipCode vipCode = new VipCode();
vipCode.setCode("123456");
vipCode.setDuration(1);
service.addOne(vipCode);
}
create table tb_vip_code
(
id bigint auto_increment
primary key,
code varchar(20) not null,
status int default '0' not null,
user_id bigint null,
duration int not null,
create_at datetime default CURRENT_TIMESTAMP not null,
update_at timestamp default CURRENT_TIMESTAMP not null,
constraint tb_vip_code_code_uindex unique (code)
);
All code as above, I am trying to use a custom sql to save object, but it throws an exception:
Caused by: java.sql.SQLException: Parameter index out of range (3 > number of parameters, which is 2).
I only have tow parameters, why it says needed three?
java sql hibernate spring-data-jpa parameterbinding
add a comment |
@Data
@MappedSuperclass
public class BaseModel implements Serializable {
private static final Long serialVersionUID = -1442801573244745790L;
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss")
@Convert(converter = LocalDateTimeConverter.class)
private LocalDateTime createAt = LocalDateTime.now();
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss")
@Convert(converter = LocalDateTimeConverter.class)
private LocalDateTime updateAt = LocalDateTime.now();
}
@Data
@Entity
@Table(name = "tb_vip_code")
@SQLInsert(sql = "insert ignore into tb_vip_code (code, duration) values (?, ?)")
public class VipCode extends BaseModel {
private static final Long serialVersionUID = -4697221755301869573L;
private String code;
private Integer duration;
private Integer status = 0;
private Long userId;
public VipCode() {}
}
@Test
public void addOne() throws Exception {
VipCode vipCode = new VipCode();
vipCode.setCode("123456");
vipCode.setDuration(1);
service.addOne(vipCode);
}
create table tb_vip_code
(
id bigint auto_increment
primary key,
code varchar(20) not null,
status int default '0' not null,
user_id bigint null,
duration int not null,
create_at datetime default CURRENT_TIMESTAMP not null,
update_at timestamp default CURRENT_TIMESTAMP not null,
constraint tb_vip_code_code_uindex unique (code)
);
All code as above, I am trying to use a custom sql to save object, but it throws an exception:
Caused by: java.sql.SQLException: Parameter index out of range (3 > number of parameters, which is 2).
I only have tow parameters, why it says needed three?
java sql hibernate spring-data-jpa parameterbinding
add a comment |
@Data
@MappedSuperclass
public class BaseModel implements Serializable {
private static final Long serialVersionUID = -1442801573244745790L;
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss")
@Convert(converter = LocalDateTimeConverter.class)
private LocalDateTime createAt = LocalDateTime.now();
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss")
@Convert(converter = LocalDateTimeConverter.class)
private LocalDateTime updateAt = LocalDateTime.now();
}
@Data
@Entity
@Table(name = "tb_vip_code")
@SQLInsert(sql = "insert ignore into tb_vip_code (code, duration) values (?, ?)")
public class VipCode extends BaseModel {
private static final Long serialVersionUID = -4697221755301869573L;
private String code;
private Integer duration;
private Integer status = 0;
private Long userId;
public VipCode() {}
}
@Test
public void addOne() throws Exception {
VipCode vipCode = new VipCode();
vipCode.setCode("123456");
vipCode.setDuration(1);
service.addOne(vipCode);
}
create table tb_vip_code
(
id bigint auto_increment
primary key,
code varchar(20) not null,
status int default '0' not null,
user_id bigint null,
duration int not null,
create_at datetime default CURRENT_TIMESTAMP not null,
update_at timestamp default CURRENT_TIMESTAMP not null,
constraint tb_vip_code_code_uindex unique (code)
);
All code as above, I am trying to use a custom sql to save object, but it throws an exception:
Caused by: java.sql.SQLException: Parameter index out of range (3 > number of parameters, which is 2).
I only have tow parameters, why it says needed three?
java sql hibernate spring-data-jpa parameterbinding
@Data
@MappedSuperclass
public class BaseModel implements Serializable {
private static final Long serialVersionUID = -1442801573244745790L;
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss")
@Convert(converter = LocalDateTimeConverter.class)
private LocalDateTime createAt = LocalDateTime.now();
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss")
@Convert(converter = LocalDateTimeConverter.class)
private LocalDateTime updateAt = LocalDateTime.now();
}
@Data
@Entity
@Table(name = "tb_vip_code")
@SQLInsert(sql = "insert ignore into tb_vip_code (code, duration) values (?, ?)")
public class VipCode extends BaseModel {
private static final Long serialVersionUID = -4697221755301869573L;
private String code;
private Integer duration;
private Integer status = 0;
private Long userId;
public VipCode() {}
}
@Test
public void addOne() throws Exception {
VipCode vipCode = new VipCode();
vipCode.setCode("123456");
vipCode.setDuration(1);
service.addOne(vipCode);
}
create table tb_vip_code
(
id bigint auto_increment
primary key,
code varchar(20) not null,
status int default '0' not null,
user_id bigint null,
duration int not null,
create_at datetime default CURRENT_TIMESTAMP not null,
update_at timestamp default CURRENT_TIMESTAMP not null,
constraint tb_vip_code_code_uindex unique (code)
);
All code as above, I am trying to use a custom sql to save object, but it throws an exception:
Caused by: java.sql.SQLException: Parameter index out of range (3 > number of parameters, which is 2).
I only have tow parameters, why it says needed three?
java sql hibernate spring-data-jpa parameterbinding
java sql hibernate spring-data-jpa parameterbinding
edited Nov 15 '18 at 6:55
Jens Schauder
46.7k17115240
46.7k17115240
asked Nov 15 '18 at 2:40


林永福林永福
1
1
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
The SQL statement specified by @SQLInsert
gets the fields of your entity bound as parameters.
You have at least 4 attributes in your VipCode
entity directly, and I suspect even more from the BaseModel
class it inherits from. But your SQL statment expects only two parameters.
To fix the problem remove the superfluous attributes from the entity, add parameters for them to the query or mark them as @Transient
.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53311646%2fjpa-using-sqlinsert-throws-parameter-index-out-of-range%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The SQL statement specified by @SQLInsert
gets the fields of your entity bound as parameters.
You have at least 4 attributes in your VipCode
entity directly, and I suspect even more from the BaseModel
class it inherits from. But your SQL statment expects only two parameters.
To fix the problem remove the superfluous attributes from the entity, add parameters for them to the query or mark them as @Transient
.
add a comment |
The SQL statement specified by @SQLInsert
gets the fields of your entity bound as parameters.
You have at least 4 attributes in your VipCode
entity directly, and I suspect even more from the BaseModel
class it inherits from. But your SQL statment expects only two parameters.
To fix the problem remove the superfluous attributes from the entity, add parameters for them to the query or mark them as @Transient
.
add a comment |
The SQL statement specified by @SQLInsert
gets the fields of your entity bound as parameters.
You have at least 4 attributes in your VipCode
entity directly, and I suspect even more from the BaseModel
class it inherits from. But your SQL statment expects only two parameters.
To fix the problem remove the superfluous attributes from the entity, add parameters for them to the query or mark them as @Transient
.
The SQL statement specified by @SQLInsert
gets the fields of your entity bound as parameters.
You have at least 4 attributes in your VipCode
entity directly, and I suspect even more from the BaseModel
class it inherits from. But your SQL statment expects only two parameters.
To fix the problem remove the superfluous attributes from the entity, add parameters for them to the query or mark them as @Transient
.
answered Nov 15 '18 at 6:54
Jens SchauderJens Schauder
46.7k17115240
46.7k17115240
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53311646%2fjpa-using-sqlinsert-throws-parameter-index-out-of-range%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
e95yl,fwFrwqrZhoade