two differents types values at the same column
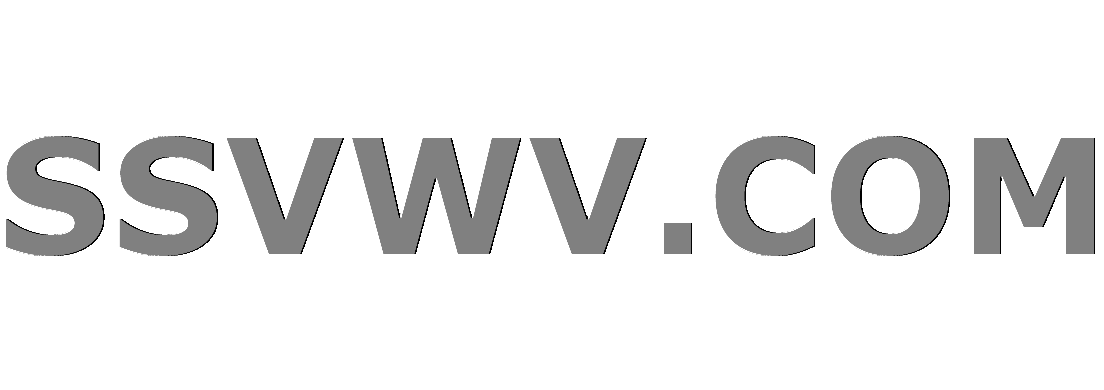
Multi tool use
I have this table which has a column in which I show a quantity. what I want is to show the quantity and the unit of measurement for example
60 meters
10 pieces
20 liters
(the first column)
this is the object that I show in table:
public class VentaDetalle {
private IntegerProperty idVentaDetalle;
private IntegerProperty idMercancia;
private StringProperty nombreMercancia;
private IntegerProperty idVentaGeneral;
private DoubleProperty cantidad;
private DoubleProperty general;
private DoubleProperty mayoreo;
private DoubleProperty subtotal;
public VentaDetalle(int idMercancia, String nombreMercancia, int idVentaGeneral,
Double cantidad, double mayoreo, double general, Double subtotal) {
this.idMercancia = new SimpleIntegerProperty(idMercancia);
this.nombreMercancia = new SimpleStringProperty(nombreMercancia);
this.idVentaGeneral = new SimpleIntegerProperty(idVentaGeneral);
this.cantidad = new SimpleDoubleProperty(cantidad);
this.mayoreo = new SimpleDoubleProperty(mayoreo);
this.general = new SimpleDoubleProperty(general);
this.subtotal = new SimpleDoubleProperty(subtotal);
}
//Metodos atributo: idVentaDetalle
public int getIdVentaDetalle() {
return idVentaDetalle.get();
}
public void setIdVentaDetalle(int idVentaDetalle) {
this.idVentaDetalle = new SimpleIntegerProperty(idVentaDetalle);
}
public IntegerProperty IdVentaDetalleProperty() {
return idVentaDetalle;
}
//Metodos atributo: idMercancia
public int getIdMercancia() {
return idMercancia.get();
}
public void setIdMercancia(int idMercancia) {
this.idMercancia = new SimpleIntegerProperty(idMercancia);
}
public IntegerProperty IdMercanciaProperty() {
return idMercancia;
}
//Metodos atributo: nombreMercancia
public String getNombreMercancia() {
return nombreMercancia.get();
}
public void setNombreMercancia(String nombreMercancia) {
this.nombreMercancia = new SimpleStringProperty(nombreMercancia);
}
public StringProperty NombreMercanciaProperty() {
return nombreMercancia;
}
//Metodos atributo: idVentaGeneral
public int getIdVentaGeneral() {
return idVentaGeneral.get();
}
public void setIdVentaGeneral(int idVentaGeneral) {
this.idVentaGeneral = new SimpleIntegerProperty(idVentaGeneral);
}
public IntegerProperty IdVentaGeneralProperty() {
return idVentaGeneral;
}
//Metodos atributo: cantidad
public Double getCantidad() {
return cantidad.get();
}
public void setCantidad(Double cantidad) {
this.cantidad = new SimpleDoubleProperty(cantidad);
}
public DoubleProperty CantidadProperty() {
return cantidad;
}
//Metodos atributo: general
public Double getMayoreo() {
return mayoreo.get();
}
public void setMayoreo(Double mayoreo) {
this.mayoreo = new SimpleDoubleProperty(mayoreo);
}
public DoubleProperty MayoreoProperty() {
return mayoreo;
}
//Metodos atributo: general
public Double getGeneral() {
return general.get();
}
public void setGeneral(Double general) {
this.general = new SimpleDoubleProperty(general);
}
public DoubleProperty GeneralProperty() {
return general;
}
//Metodos atributo: subtotal
public Double getSubtotal() {
return subtotal.get();
}
public void setSubtotal(Double subtotal) {
this.subtotal = new SimpleDoubleProperty(subtotal);
}
public DoubleProperty SubtotalProperty() {
return subtotal;
and I initialize the column like this:
clmnCantidad.setCellValueFactory(new PropertyValueFactory<VentaDetalle, Double>("cantidad"));
i take the unit from a databse with a table item
the table has name, stock, codebar and unit of measuremen
java javafx
|
show 4 more comments
I have this table which has a column in which I show a quantity. what I want is to show the quantity and the unit of measurement for example
60 meters
10 pieces
20 liters
(the first column)
this is the object that I show in table:
public class VentaDetalle {
private IntegerProperty idVentaDetalle;
private IntegerProperty idMercancia;
private StringProperty nombreMercancia;
private IntegerProperty idVentaGeneral;
private DoubleProperty cantidad;
private DoubleProperty general;
private DoubleProperty mayoreo;
private DoubleProperty subtotal;
public VentaDetalle(int idMercancia, String nombreMercancia, int idVentaGeneral,
Double cantidad, double mayoreo, double general, Double subtotal) {
this.idMercancia = new SimpleIntegerProperty(idMercancia);
this.nombreMercancia = new SimpleStringProperty(nombreMercancia);
this.idVentaGeneral = new SimpleIntegerProperty(idVentaGeneral);
this.cantidad = new SimpleDoubleProperty(cantidad);
this.mayoreo = new SimpleDoubleProperty(mayoreo);
this.general = new SimpleDoubleProperty(general);
this.subtotal = new SimpleDoubleProperty(subtotal);
}
//Metodos atributo: idVentaDetalle
public int getIdVentaDetalle() {
return idVentaDetalle.get();
}
public void setIdVentaDetalle(int idVentaDetalle) {
this.idVentaDetalle = new SimpleIntegerProperty(idVentaDetalle);
}
public IntegerProperty IdVentaDetalleProperty() {
return idVentaDetalle;
}
//Metodos atributo: idMercancia
public int getIdMercancia() {
return idMercancia.get();
}
public void setIdMercancia(int idMercancia) {
this.idMercancia = new SimpleIntegerProperty(idMercancia);
}
public IntegerProperty IdMercanciaProperty() {
return idMercancia;
}
//Metodos atributo: nombreMercancia
public String getNombreMercancia() {
return nombreMercancia.get();
}
public void setNombreMercancia(String nombreMercancia) {
this.nombreMercancia = new SimpleStringProperty(nombreMercancia);
}
public StringProperty NombreMercanciaProperty() {
return nombreMercancia;
}
//Metodos atributo: idVentaGeneral
public int getIdVentaGeneral() {
return idVentaGeneral.get();
}
public void setIdVentaGeneral(int idVentaGeneral) {
this.idVentaGeneral = new SimpleIntegerProperty(idVentaGeneral);
}
public IntegerProperty IdVentaGeneralProperty() {
return idVentaGeneral;
}
//Metodos atributo: cantidad
public Double getCantidad() {
return cantidad.get();
}
public void setCantidad(Double cantidad) {
this.cantidad = new SimpleDoubleProperty(cantidad);
}
public DoubleProperty CantidadProperty() {
return cantidad;
}
//Metodos atributo: general
public Double getMayoreo() {
return mayoreo.get();
}
public void setMayoreo(Double mayoreo) {
this.mayoreo = new SimpleDoubleProperty(mayoreo);
}
public DoubleProperty MayoreoProperty() {
return mayoreo;
}
//Metodos atributo: general
public Double getGeneral() {
return general.get();
}
public void setGeneral(Double general) {
this.general = new SimpleDoubleProperty(general);
}
public DoubleProperty GeneralProperty() {
return general;
}
//Metodos atributo: subtotal
public Double getSubtotal() {
return subtotal.get();
}
public void setSubtotal(Double subtotal) {
this.subtotal = new SimpleDoubleProperty(subtotal);
}
public DoubleProperty SubtotalProperty() {
return subtotal;
and I initialize the column like this:
clmnCantidad.setCellValueFactory(new PropertyValueFactory<VentaDetalle, Double>("cantidad"));
i take the unit from a databse with a table item
the table has name, stock, codebar and unit of measuremen
java javafx
1
Provide some codes so that we know your data structure. How do you determine the unit?
– Jai
Nov 13 '18 at 1:51
i have edited my ask @Jai
– Johnatan De Leon
Nov 13 '18 at 2:34
I'm going to make a guess because there are a lot of non-english here. I'm going to assume "nombre" means name, and this name is used to match against another table in the database to find out which measurement unit the entry uses?
– Jai
Nov 13 '18 at 2:39
Yes i am using two tables of my database item and saleDetail i want to do something like:
– Johnatan De Leon
Nov 13 '18 at 2:46
It may be neater to add the measurement unit property inVentaDetalle
class, and when you are getting the list via SQL, you would do an inner-join SQL query to get the quantity and unit out at the same time. Then you could create a custom cell value factory that does a concatenation of these 2 properties.
– Jai
Nov 13 '18 at 2:50
|
show 4 more comments
I have this table which has a column in which I show a quantity. what I want is to show the quantity and the unit of measurement for example
60 meters
10 pieces
20 liters
(the first column)
this is the object that I show in table:
public class VentaDetalle {
private IntegerProperty idVentaDetalle;
private IntegerProperty idMercancia;
private StringProperty nombreMercancia;
private IntegerProperty idVentaGeneral;
private DoubleProperty cantidad;
private DoubleProperty general;
private DoubleProperty mayoreo;
private DoubleProperty subtotal;
public VentaDetalle(int idMercancia, String nombreMercancia, int idVentaGeneral,
Double cantidad, double mayoreo, double general, Double subtotal) {
this.idMercancia = new SimpleIntegerProperty(idMercancia);
this.nombreMercancia = new SimpleStringProperty(nombreMercancia);
this.idVentaGeneral = new SimpleIntegerProperty(idVentaGeneral);
this.cantidad = new SimpleDoubleProperty(cantidad);
this.mayoreo = new SimpleDoubleProperty(mayoreo);
this.general = new SimpleDoubleProperty(general);
this.subtotal = new SimpleDoubleProperty(subtotal);
}
//Metodos atributo: idVentaDetalle
public int getIdVentaDetalle() {
return idVentaDetalle.get();
}
public void setIdVentaDetalle(int idVentaDetalle) {
this.idVentaDetalle = new SimpleIntegerProperty(idVentaDetalle);
}
public IntegerProperty IdVentaDetalleProperty() {
return idVentaDetalle;
}
//Metodos atributo: idMercancia
public int getIdMercancia() {
return idMercancia.get();
}
public void setIdMercancia(int idMercancia) {
this.idMercancia = new SimpleIntegerProperty(idMercancia);
}
public IntegerProperty IdMercanciaProperty() {
return idMercancia;
}
//Metodos atributo: nombreMercancia
public String getNombreMercancia() {
return nombreMercancia.get();
}
public void setNombreMercancia(String nombreMercancia) {
this.nombreMercancia = new SimpleStringProperty(nombreMercancia);
}
public StringProperty NombreMercanciaProperty() {
return nombreMercancia;
}
//Metodos atributo: idVentaGeneral
public int getIdVentaGeneral() {
return idVentaGeneral.get();
}
public void setIdVentaGeneral(int idVentaGeneral) {
this.idVentaGeneral = new SimpleIntegerProperty(idVentaGeneral);
}
public IntegerProperty IdVentaGeneralProperty() {
return idVentaGeneral;
}
//Metodos atributo: cantidad
public Double getCantidad() {
return cantidad.get();
}
public void setCantidad(Double cantidad) {
this.cantidad = new SimpleDoubleProperty(cantidad);
}
public DoubleProperty CantidadProperty() {
return cantidad;
}
//Metodos atributo: general
public Double getMayoreo() {
return mayoreo.get();
}
public void setMayoreo(Double mayoreo) {
this.mayoreo = new SimpleDoubleProperty(mayoreo);
}
public DoubleProperty MayoreoProperty() {
return mayoreo;
}
//Metodos atributo: general
public Double getGeneral() {
return general.get();
}
public void setGeneral(Double general) {
this.general = new SimpleDoubleProperty(general);
}
public DoubleProperty GeneralProperty() {
return general;
}
//Metodos atributo: subtotal
public Double getSubtotal() {
return subtotal.get();
}
public void setSubtotal(Double subtotal) {
this.subtotal = new SimpleDoubleProperty(subtotal);
}
public DoubleProperty SubtotalProperty() {
return subtotal;
and I initialize the column like this:
clmnCantidad.setCellValueFactory(new PropertyValueFactory<VentaDetalle, Double>("cantidad"));
i take the unit from a databse with a table item
the table has name, stock, codebar and unit of measuremen
java javafx
I have this table which has a column in which I show a quantity. what I want is to show the quantity and the unit of measurement for example
60 meters
10 pieces
20 liters
(the first column)
this is the object that I show in table:
public class VentaDetalle {
private IntegerProperty idVentaDetalle;
private IntegerProperty idMercancia;
private StringProperty nombreMercancia;
private IntegerProperty idVentaGeneral;
private DoubleProperty cantidad;
private DoubleProperty general;
private DoubleProperty mayoreo;
private DoubleProperty subtotal;
public VentaDetalle(int idMercancia, String nombreMercancia, int idVentaGeneral,
Double cantidad, double mayoreo, double general, Double subtotal) {
this.idMercancia = new SimpleIntegerProperty(idMercancia);
this.nombreMercancia = new SimpleStringProperty(nombreMercancia);
this.idVentaGeneral = new SimpleIntegerProperty(idVentaGeneral);
this.cantidad = new SimpleDoubleProperty(cantidad);
this.mayoreo = new SimpleDoubleProperty(mayoreo);
this.general = new SimpleDoubleProperty(general);
this.subtotal = new SimpleDoubleProperty(subtotal);
}
//Metodos atributo: idVentaDetalle
public int getIdVentaDetalle() {
return idVentaDetalle.get();
}
public void setIdVentaDetalle(int idVentaDetalle) {
this.idVentaDetalle = new SimpleIntegerProperty(idVentaDetalle);
}
public IntegerProperty IdVentaDetalleProperty() {
return idVentaDetalle;
}
//Metodos atributo: idMercancia
public int getIdMercancia() {
return idMercancia.get();
}
public void setIdMercancia(int idMercancia) {
this.idMercancia = new SimpleIntegerProperty(idMercancia);
}
public IntegerProperty IdMercanciaProperty() {
return idMercancia;
}
//Metodos atributo: nombreMercancia
public String getNombreMercancia() {
return nombreMercancia.get();
}
public void setNombreMercancia(String nombreMercancia) {
this.nombreMercancia = new SimpleStringProperty(nombreMercancia);
}
public StringProperty NombreMercanciaProperty() {
return nombreMercancia;
}
//Metodos atributo: idVentaGeneral
public int getIdVentaGeneral() {
return idVentaGeneral.get();
}
public void setIdVentaGeneral(int idVentaGeneral) {
this.idVentaGeneral = new SimpleIntegerProperty(idVentaGeneral);
}
public IntegerProperty IdVentaGeneralProperty() {
return idVentaGeneral;
}
//Metodos atributo: cantidad
public Double getCantidad() {
return cantidad.get();
}
public void setCantidad(Double cantidad) {
this.cantidad = new SimpleDoubleProperty(cantidad);
}
public DoubleProperty CantidadProperty() {
return cantidad;
}
//Metodos atributo: general
public Double getMayoreo() {
return mayoreo.get();
}
public void setMayoreo(Double mayoreo) {
this.mayoreo = new SimpleDoubleProperty(mayoreo);
}
public DoubleProperty MayoreoProperty() {
return mayoreo;
}
//Metodos atributo: general
public Double getGeneral() {
return general.get();
}
public void setGeneral(Double general) {
this.general = new SimpleDoubleProperty(general);
}
public DoubleProperty GeneralProperty() {
return general;
}
//Metodos atributo: subtotal
public Double getSubtotal() {
return subtotal.get();
}
public void setSubtotal(Double subtotal) {
this.subtotal = new SimpleDoubleProperty(subtotal);
}
public DoubleProperty SubtotalProperty() {
return subtotal;
and I initialize the column like this:
clmnCantidad.setCellValueFactory(new PropertyValueFactory<VentaDetalle, Double>("cantidad"));
i take the unit from a databse with a table item
the table has name, stock, codebar and unit of measuremen
java javafx
java javafx
edited Nov 13 '18 at 2:33
asked Nov 13 '18 at 1:46
Johnatan De Leon
112
112
1
Provide some codes so that we know your data structure. How do you determine the unit?
– Jai
Nov 13 '18 at 1:51
i have edited my ask @Jai
– Johnatan De Leon
Nov 13 '18 at 2:34
I'm going to make a guess because there are a lot of non-english here. I'm going to assume "nombre" means name, and this name is used to match against another table in the database to find out which measurement unit the entry uses?
– Jai
Nov 13 '18 at 2:39
Yes i am using two tables of my database item and saleDetail i want to do something like:
– Johnatan De Leon
Nov 13 '18 at 2:46
It may be neater to add the measurement unit property inVentaDetalle
class, and when you are getting the list via SQL, you would do an inner-join SQL query to get the quantity and unit out at the same time. Then you could create a custom cell value factory that does a concatenation of these 2 properties.
– Jai
Nov 13 '18 at 2:50
|
show 4 more comments
1
Provide some codes so that we know your data structure. How do you determine the unit?
– Jai
Nov 13 '18 at 1:51
i have edited my ask @Jai
– Johnatan De Leon
Nov 13 '18 at 2:34
I'm going to make a guess because there are a lot of non-english here. I'm going to assume "nombre" means name, and this name is used to match against another table in the database to find out which measurement unit the entry uses?
– Jai
Nov 13 '18 at 2:39
Yes i am using two tables of my database item and saleDetail i want to do something like:
– Johnatan De Leon
Nov 13 '18 at 2:46
It may be neater to add the measurement unit property inVentaDetalle
class, and when you are getting the list via SQL, you would do an inner-join SQL query to get the quantity and unit out at the same time. Then you could create a custom cell value factory that does a concatenation of these 2 properties.
– Jai
Nov 13 '18 at 2:50
1
1
Provide some codes so that we know your data structure. How do you determine the unit?
– Jai
Nov 13 '18 at 1:51
Provide some codes so that we know your data structure. How do you determine the unit?
– Jai
Nov 13 '18 at 1:51
i have edited my ask @Jai
– Johnatan De Leon
Nov 13 '18 at 2:34
i have edited my ask @Jai
– Johnatan De Leon
Nov 13 '18 at 2:34
I'm going to make a guess because there are a lot of non-english here. I'm going to assume "nombre" means name, and this name is used to match against another table in the database to find out which measurement unit the entry uses?
– Jai
Nov 13 '18 at 2:39
I'm going to make a guess because there are a lot of non-english here. I'm going to assume "nombre" means name, and this name is used to match against another table in the database to find out which measurement unit the entry uses?
– Jai
Nov 13 '18 at 2:39
Yes i am using two tables of my database item and saleDetail i want to do something like:
– Johnatan De Leon
Nov 13 '18 at 2:46
Yes i am using two tables of my database item and saleDetail i want to do something like:
– Johnatan De Leon
Nov 13 '18 at 2:46
It may be neater to add the measurement unit property in
VentaDetalle
class, and when you are getting the list via SQL, you would do an inner-join SQL query to get the quantity and unit out at the same time. Then you could create a custom cell value factory that does a concatenation of these 2 properties.– Jai
Nov 13 '18 at 2:50
It may be neater to add the measurement unit property in
VentaDetalle
class, and when you are getting the list via SQL, you would do an inner-join SQL query to get the quantity and unit out at the same time. Then you could create a custom cell value factory that does a concatenation of these 2 properties.– Jai
Nov 13 '18 at 2:50
|
show 4 more comments
1 Answer
1
active
oldest
votes
I'm not going to answer the part on the SQL query - ideally it should be some kind of JOIN
statement that combines the two tables.
There are 2 main approaches when it comes to combine an IntegerProperty
and a StringProperty
.
Approach 1: Make a new property that concatenates them in the Model class
public class VentaDetalle {
private DoubleProperty cantidad;
private StringProperty measurementUnit;
private StringProperty cantidadWithUnit;
// Other properties
// Public getters/setters
public VentaDetalle(......) {
// All the other stuff you did in constructor
cantidadWithUnit.bind(Bindings.concat(cantidad, " ", measurementUnit));
}
}
Then you just need to set the cell value factory:
clmnCantidad.setCellValueFactory(new PropertyValueFactory<>("cantidadWithUnit"));
Approach 2: Concatenate inside the cell value factory callback
clmnCantidad.setCellValueFactory(row ->
Bindings.concat(row.getValue().cantidadProperty(), " ", row.getValue().measurementUnitProperty())
);
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53272599%2ftwo-differents-types-values-at-the-same-column%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I'm not going to answer the part on the SQL query - ideally it should be some kind of JOIN
statement that combines the two tables.
There are 2 main approaches when it comes to combine an IntegerProperty
and a StringProperty
.
Approach 1: Make a new property that concatenates them in the Model class
public class VentaDetalle {
private DoubleProperty cantidad;
private StringProperty measurementUnit;
private StringProperty cantidadWithUnit;
// Other properties
// Public getters/setters
public VentaDetalle(......) {
// All the other stuff you did in constructor
cantidadWithUnit.bind(Bindings.concat(cantidad, " ", measurementUnit));
}
}
Then you just need to set the cell value factory:
clmnCantidad.setCellValueFactory(new PropertyValueFactory<>("cantidadWithUnit"));
Approach 2: Concatenate inside the cell value factory callback
clmnCantidad.setCellValueFactory(row ->
Bindings.concat(row.getValue().cantidadProperty(), " ", row.getValue().measurementUnitProperty())
);
add a comment |
I'm not going to answer the part on the SQL query - ideally it should be some kind of JOIN
statement that combines the two tables.
There are 2 main approaches when it comes to combine an IntegerProperty
and a StringProperty
.
Approach 1: Make a new property that concatenates them in the Model class
public class VentaDetalle {
private DoubleProperty cantidad;
private StringProperty measurementUnit;
private StringProperty cantidadWithUnit;
// Other properties
// Public getters/setters
public VentaDetalle(......) {
// All the other stuff you did in constructor
cantidadWithUnit.bind(Bindings.concat(cantidad, " ", measurementUnit));
}
}
Then you just need to set the cell value factory:
clmnCantidad.setCellValueFactory(new PropertyValueFactory<>("cantidadWithUnit"));
Approach 2: Concatenate inside the cell value factory callback
clmnCantidad.setCellValueFactory(row ->
Bindings.concat(row.getValue().cantidadProperty(), " ", row.getValue().measurementUnitProperty())
);
add a comment |
I'm not going to answer the part on the SQL query - ideally it should be some kind of JOIN
statement that combines the two tables.
There are 2 main approaches when it comes to combine an IntegerProperty
and a StringProperty
.
Approach 1: Make a new property that concatenates them in the Model class
public class VentaDetalle {
private DoubleProperty cantidad;
private StringProperty measurementUnit;
private StringProperty cantidadWithUnit;
// Other properties
// Public getters/setters
public VentaDetalle(......) {
// All the other stuff you did in constructor
cantidadWithUnit.bind(Bindings.concat(cantidad, " ", measurementUnit));
}
}
Then you just need to set the cell value factory:
clmnCantidad.setCellValueFactory(new PropertyValueFactory<>("cantidadWithUnit"));
Approach 2: Concatenate inside the cell value factory callback
clmnCantidad.setCellValueFactory(row ->
Bindings.concat(row.getValue().cantidadProperty(), " ", row.getValue().measurementUnitProperty())
);
I'm not going to answer the part on the SQL query - ideally it should be some kind of JOIN
statement that combines the two tables.
There are 2 main approaches when it comes to combine an IntegerProperty
and a StringProperty
.
Approach 1: Make a new property that concatenates them in the Model class
public class VentaDetalle {
private DoubleProperty cantidad;
private StringProperty measurementUnit;
private StringProperty cantidadWithUnit;
// Other properties
// Public getters/setters
public VentaDetalle(......) {
// All the other stuff you did in constructor
cantidadWithUnit.bind(Bindings.concat(cantidad, " ", measurementUnit));
}
}
Then you just need to set the cell value factory:
clmnCantidad.setCellValueFactory(new PropertyValueFactory<>("cantidadWithUnit"));
Approach 2: Concatenate inside the cell value factory callback
clmnCantidad.setCellValueFactory(row ->
Bindings.concat(row.getValue().cantidadProperty(), " ", row.getValue().measurementUnitProperty())
);
answered Nov 14 '18 at 1:46
Jai
5,72411231
5,72411231
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53272599%2ftwo-differents-types-values-at-the-same-column%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
t3dikJb
1
Provide some codes so that we know your data structure. How do you determine the unit?
– Jai
Nov 13 '18 at 1:51
i have edited my ask @Jai
– Johnatan De Leon
Nov 13 '18 at 2:34
I'm going to make a guess because there are a lot of non-english here. I'm going to assume "nombre" means name, and this name is used to match against another table in the database to find out which measurement unit the entry uses?
– Jai
Nov 13 '18 at 2:39
Yes i am using two tables of my database item and saleDetail i want to do something like:
– Johnatan De Leon
Nov 13 '18 at 2:46
It may be neater to add the measurement unit property in
VentaDetalle
class, and when you are getting the list via SQL, you would do an inner-join SQL query to get the quantity and unit out at the same time. Then you could create a custom cell value factory that does a concatenation of these 2 properties.– Jai
Nov 13 '18 at 2:50