PHP read text file ignore specific tab
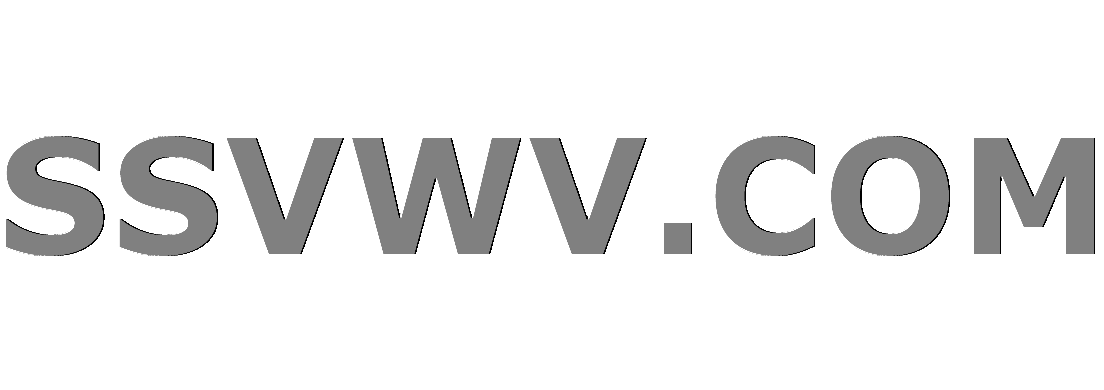
Multi tool use
I have log file data(.txt) here
As you can see on log file that has Date
and Time
content.
And now I have PHP function to get the data from log file.
$fromDateTime = new DateTime('Wed, Sep 19 2018 08:00:00');
$toDateTime = new DateTime('Wed, Sep 19 2018 19:59:00');
$file = file_get_contents('Reject.txt');
$lines = explode("n", $file);
// counter
$rowsintimespan = 0;
// keys should correspond to columns
$keys = [
'Date',
'Time',
'Battery level',
'Piezo sound level',
'Left (Channel 3) light intensity',
'Right (Channel 1) light intensity'
];
$values = array_fill(0, count($keys), 0);
$values = array_combine($keys, $values);
// Do Line-By-Line starting by Line 16 (Array Index 15)
for ($i = 11; $i < count($lines); $i++) {
// if the file is "Tue, Sep 18<tab>2018<tab>23:59:53<tab>"
$dateobj = DateTime::createFromFormat("???,?M?d??Y?H:i:s+", $lines[$i]);
// check if date is in your Timespan
if ($dateobj < $toDateTime && $dateobj > $fromDateTime) {
$rowsintimespan++; // count if in timespan
// get line elements
$lineContent = explode("t", $lines[$i]);
// loop through line elements and count them
$x = 0;
for ($j = 0; $j < count($keys); $j++) {
if (!isset($lineContent[$j])) {
continue;
}
// remember position of last not empty column
if (trim($lineContent[$j]) != '') {
$x = $j;
}
}
if ($x > 0) {
$values[$keys[$x]]++;
}
}
}
// Debug-Output
echo $rowsintimespan;
// Output every column
echo '<pre>';
print_r($values);
And now I don't want Date
and Time
including on counting. So I try to remove both, but the result of count is not in the correct keys
.
My question, is it possible to ignore both keys then the counting is keep correct?
Above image, I try to removed Date
and Time
.
The correct one should be, Battery level = 2
and Piezo sound level = 2
.
php
add a comment |
I have log file data(.txt) here
As you can see on log file that has Date
and Time
content.
And now I have PHP function to get the data from log file.
$fromDateTime = new DateTime('Wed, Sep 19 2018 08:00:00');
$toDateTime = new DateTime('Wed, Sep 19 2018 19:59:00');
$file = file_get_contents('Reject.txt');
$lines = explode("n", $file);
// counter
$rowsintimespan = 0;
// keys should correspond to columns
$keys = [
'Date',
'Time',
'Battery level',
'Piezo sound level',
'Left (Channel 3) light intensity',
'Right (Channel 1) light intensity'
];
$values = array_fill(0, count($keys), 0);
$values = array_combine($keys, $values);
// Do Line-By-Line starting by Line 16 (Array Index 15)
for ($i = 11; $i < count($lines); $i++) {
// if the file is "Tue, Sep 18<tab>2018<tab>23:59:53<tab>"
$dateobj = DateTime::createFromFormat("???,?M?d??Y?H:i:s+", $lines[$i]);
// check if date is in your Timespan
if ($dateobj < $toDateTime && $dateobj > $fromDateTime) {
$rowsintimespan++; // count if in timespan
// get line elements
$lineContent = explode("t", $lines[$i]);
// loop through line elements and count them
$x = 0;
for ($j = 0; $j < count($keys); $j++) {
if (!isset($lineContent[$j])) {
continue;
}
// remember position of last not empty column
if (trim($lineContent[$j]) != '') {
$x = $j;
}
}
if ($x > 0) {
$values[$keys[$x]]++;
}
}
}
// Debug-Output
echo $rowsintimespan;
// Output every column
echo '<pre>';
print_r($values);
And now I don't want Date
and Time
including on counting. So I try to remove both, but the result of count is not in the correct keys
.
My question, is it possible to ignore both keys then the counting is keep correct?
Above image, I try to removed Date
and Time
.
The correct one should be, Battery level = 2
and Piezo sound level = 2
.
php
Your question isn't clear to me. Are you trying to get only the values between a certain timeframe,is that it?
– Pedro Lobito
Nov 13 '18 at 3:39
1
You may find some parts of the code interesting, still, not enough for an answer, as I don't fully understand your question - gist.github.com/x011/a764ee4dab50158725a15180b9c948fa
– Pedro Lobito
Nov 13 '18 at 4:22
add a comment |
I have log file data(.txt) here
As you can see on log file that has Date
and Time
content.
And now I have PHP function to get the data from log file.
$fromDateTime = new DateTime('Wed, Sep 19 2018 08:00:00');
$toDateTime = new DateTime('Wed, Sep 19 2018 19:59:00');
$file = file_get_contents('Reject.txt');
$lines = explode("n", $file);
// counter
$rowsintimespan = 0;
// keys should correspond to columns
$keys = [
'Date',
'Time',
'Battery level',
'Piezo sound level',
'Left (Channel 3) light intensity',
'Right (Channel 1) light intensity'
];
$values = array_fill(0, count($keys), 0);
$values = array_combine($keys, $values);
// Do Line-By-Line starting by Line 16 (Array Index 15)
for ($i = 11; $i < count($lines); $i++) {
// if the file is "Tue, Sep 18<tab>2018<tab>23:59:53<tab>"
$dateobj = DateTime::createFromFormat("???,?M?d??Y?H:i:s+", $lines[$i]);
// check if date is in your Timespan
if ($dateobj < $toDateTime && $dateobj > $fromDateTime) {
$rowsintimespan++; // count if in timespan
// get line elements
$lineContent = explode("t", $lines[$i]);
// loop through line elements and count them
$x = 0;
for ($j = 0; $j < count($keys); $j++) {
if (!isset($lineContent[$j])) {
continue;
}
// remember position of last not empty column
if (trim($lineContent[$j]) != '') {
$x = $j;
}
}
if ($x > 0) {
$values[$keys[$x]]++;
}
}
}
// Debug-Output
echo $rowsintimespan;
// Output every column
echo '<pre>';
print_r($values);
And now I don't want Date
and Time
including on counting. So I try to remove both, but the result of count is not in the correct keys
.
My question, is it possible to ignore both keys then the counting is keep correct?
Above image, I try to removed Date
and Time
.
The correct one should be, Battery level = 2
and Piezo sound level = 2
.
php
I have log file data(.txt) here
As you can see on log file that has Date
and Time
content.
And now I have PHP function to get the data from log file.
$fromDateTime = new DateTime('Wed, Sep 19 2018 08:00:00');
$toDateTime = new DateTime('Wed, Sep 19 2018 19:59:00');
$file = file_get_contents('Reject.txt');
$lines = explode("n", $file);
// counter
$rowsintimespan = 0;
// keys should correspond to columns
$keys = [
'Date',
'Time',
'Battery level',
'Piezo sound level',
'Left (Channel 3) light intensity',
'Right (Channel 1) light intensity'
];
$values = array_fill(0, count($keys), 0);
$values = array_combine($keys, $values);
// Do Line-By-Line starting by Line 16 (Array Index 15)
for ($i = 11; $i < count($lines); $i++) {
// if the file is "Tue, Sep 18<tab>2018<tab>23:59:53<tab>"
$dateobj = DateTime::createFromFormat("???,?M?d??Y?H:i:s+", $lines[$i]);
// check if date is in your Timespan
if ($dateobj < $toDateTime && $dateobj > $fromDateTime) {
$rowsintimespan++; // count if in timespan
// get line elements
$lineContent = explode("t", $lines[$i]);
// loop through line elements and count them
$x = 0;
for ($j = 0; $j < count($keys); $j++) {
if (!isset($lineContent[$j])) {
continue;
}
// remember position of last not empty column
if (trim($lineContent[$j]) != '') {
$x = $j;
}
}
if ($x > 0) {
$values[$keys[$x]]++;
}
}
}
// Debug-Output
echo $rowsintimespan;
// Output every column
echo '<pre>';
print_r($values);
And now I don't want Date
and Time
including on counting. So I try to remove both, but the result of count is not in the correct keys
.
My question, is it possible to ignore both keys then the counting is keep correct?
Above image, I try to removed Date
and Time
.
The correct one should be, Battery level = 2
and Piezo sound level = 2
.
php
php
asked Nov 13 '18 at 1:41


HiDayurie Dave
7041723
7041723
Your question isn't clear to me. Are you trying to get only the values between a certain timeframe,is that it?
– Pedro Lobito
Nov 13 '18 at 3:39
1
You may find some parts of the code interesting, still, not enough for an answer, as I don't fully understand your question - gist.github.com/x011/a764ee4dab50158725a15180b9c948fa
– Pedro Lobito
Nov 13 '18 at 4:22
add a comment |
Your question isn't clear to me. Are you trying to get only the values between a certain timeframe,is that it?
– Pedro Lobito
Nov 13 '18 at 3:39
1
You may find some parts of the code interesting, still, not enough for an answer, as I don't fully understand your question - gist.github.com/x011/a764ee4dab50158725a15180b9c948fa
– Pedro Lobito
Nov 13 '18 at 4:22
Your question isn't clear to me. Are you trying to get only the values between a certain timeframe,is that it?
– Pedro Lobito
Nov 13 '18 at 3:39
Your question isn't clear to me. Are you trying to get only the values between a certain timeframe,is that it?
– Pedro Lobito
Nov 13 '18 at 3:39
1
1
You may find some parts of the code interesting, still, not enough for an answer, as I don't fully understand your question - gist.github.com/x011/a764ee4dab50158725a15180b9c948fa
– Pedro Lobito
Nov 13 '18 at 4:22
You may find some parts of the code interesting, still, not enough for an answer, as I don't fully understand your question - gist.github.com/x011/a764ee4dab50158725a15180b9c948fa
– Pedro Lobito
Nov 13 '18 at 4:22
add a comment |
1 Answer
1
active
oldest
votes
You should subtract the number of columns:
$values[$keys[$x]]++;
→ $values[$keys[$x - 2]]++;
Here is an updated code snippet:
<?php
$fromDateTime = new DateTime('Tue, Nov 13 2018 08:00:00');
$toDateTime = new DateTime('Tue, Nov 13 2018 19:59:00');
$file = file_get_contents('reject_new.txt');
$lines = explode("n", $file);
const IGNORE_COLS = 2; // +++ Introduced const for number of columns to skip
// counter
$rowsintimespan = 0;
// keys should correspond to columns
$keys = [
// 'Date', // +++
// 'Time', // +++
'Battery level',
'Piezo sound level',
'Left (Channel 3) light intensity',
'Right (Channel 1) light intensity'
];
$values = array_fill(0, count($keys), 0);
$values = array_combine($keys, $values);
// Do Line-By-Line starting by Line 12 (Array Index 11)
for ($i = 11; $i < count($lines); $i++) {
// if the file is "Tue, Sep 18 2018<tab>23:59:53<tab>"
$dateobj = DateTime::createFromFormat("???,?M?d??Y?H:i:s+", $lines[$i]);
// check if date is in your Timespan
if ($dateobj < $toDateTime && $dateobj > $fromDateTime) {
$rowsintimespan++; // count if in timespan
// get line elements
$lineContent = explode("t", $lines[$i]);
// loop through line elements and count them
for ($j = IGNORE_COLS; $j < count($keys); $j++) {
if (!isset($lineContent[$j])) {
continue;
}
if (trim($lineContent[$j]) != '') {
$values[$keys[$j - IGNORE_COLS]]++; // −−− Remove redundant $x var
}
}
}
}
// Debug-Output
echo $rowsintimespan . PHP_EOL;
// Output every column
echo '<pre>' . PHP_EOL;
print_r($values);
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53272556%2fphp-read-text-file-ignore-specific-tab%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You should subtract the number of columns:
$values[$keys[$x]]++;
→ $values[$keys[$x - 2]]++;
Here is an updated code snippet:
<?php
$fromDateTime = new DateTime('Tue, Nov 13 2018 08:00:00');
$toDateTime = new DateTime('Tue, Nov 13 2018 19:59:00');
$file = file_get_contents('reject_new.txt');
$lines = explode("n", $file);
const IGNORE_COLS = 2; // +++ Introduced const for number of columns to skip
// counter
$rowsintimespan = 0;
// keys should correspond to columns
$keys = [
// 'Date', // +++
// 'Time', // +++
'Battery level',
'Piezo sound level',
'Left (Channel 3) light intensity',
'Right (Channel 1) light intensity'
];
$values = array_fill(0, count($keys), 0);
$values = array_combine($keys, $values);
// Do Line-By-Line starting by Line 12 (Array Index 11)
for ($i = 11; $i < count($lines); $i++) {
// if the file is "Tue, Sep 18 2018<tab>23:59:53<tab>"
$dateobj = DateTime::createFromFormat("???,?M?d??Y?H:i:s+", $lines[$i]);
// check if date is in your Timespan
if ($dateobj < $toDateTime && $dateobj > $fromDateTime) {
$rowsintimespan++; // count if in timespan
// get line elements
$lineContent = explode("t", $lines[$i]);
// loop through line elements and count them
for ($j = IGNORE_COLS; $j < count($keys); $j++) {
if (!isset($lineContent[$j])) {
continue;
}
if (trim($lineContent[$j]) != '') {
$values[$keys[$j - IGNORE_COLS]]++; // −−− Remove redundant $x var
}
}
}
}
// Debug-Output
echo $rowsintimespan . PHP_EOL;
// Output every column
echo '<pre>' . PHP_EOL;
print_r($values);
add a comment |
You should subtract the number of columns:
$values[$keys[$x]]++;
→ $values[$keys[$x - 2]]++;
Here is an updated code snippet:
<?php
$fromDateTime = new DateTime('Tue, Nov 13 2018 08:00:00');
$toDateTime = new DateTime('Tue, Nov 13 2018 19:59:00');
$file = file_get_contents('reject_new.txt');
$lines = explode("n", $file);
const IGNORE_COLS = 2; // +++ Introduced const for number of columns to skip
// counter
$rowsintimespan = 0;
// keys should correspond to columns
$keys = [
// 'Date', // +++
// 'Time', // +++
'Battery level',
'Piezo sound level',
'Left (Channel 3) light intensity',
'Right (Channel 1) light intensity'
];
$values = array_fill(0, count($keys), 0);
$values = array_combine($keys, $values);
// Do Line-By-Line starting by Line 12 (Array Index 11)
for ($i = 11; $i < count($lines); $i++) {
// if the file is "Tue, Sep 18 2018<tab>23:59:53<tab>"
$dateobj = DateTime::createFromFormat("???,?M?d??Y?H:i:s+", $lines[$i]);
// check if date is in your Timespan
if ($dateobj < $toDateTime && $dateobj > $fromDateTime) {
$rowsintimespan++; // count if in timespan
// get line elements
$lineContent = explode("t", $lines[$i]);
// loop through line elements and count them
for ($j = IGNORE_COLS; $j < count($keys); $j++) {
if (!isset($lineContent[$j])) {
continue;
}
if (trim($lineContent[$j]) != '') {
$values[$keys[$j - IGNORE_COLS]]++; // −−− Remove redundant $x var
}
}
}
}
// Debug-Output
echo $rowsintimespan . PHP_EOL;
// Output every column
echo '<pre>' . PHP_EOL;
print_r($values);
add a comment |
You should subtract the number of columns:
$values[$keys[$x]]++;
→ $values[$keys[$x - 2]]++;
Here is an updated code snippet:
<?php
$fromDateTime = new DateTime('Tue, Nov 13 2018 08:00:00');
$toDateTime = new DateTime('Tue, Nov 13 2018 19:59:00');
$file = file_get_contents('reject_new.txt');
$lines = explode("n", $file);
const IGNORE_COLS = 2; // +++ Introduced const for number of columns to skip
// counter
$rowsintimespan = 0;
// keys should correspond to columns
$keys = [
// 'Date', // +++
// 'Time', // +++
'Battery level',
'Piezo sound level',
'Left (Channel 3) light intensity',
'Right (Channel 1) light intensity'
];
$values = array_fill(0, count($keys), 0);
$values = array_combine($keys, $values);
// Do Line-By-Line starting by Line 12 (Array Index 11)
for ($i = 11; $i < count($lines); $i++) {
// if the file is "Tue, Sep 18 2018<tab>23:59:53<tab>"
$dateobj = DateTime::createFromFormat("???,?M?d??Y?H:i:s+", $lines[$i]);
// check if date is in your Timespan
if ($dateobj < $toDateTime && $dateobj > $fromDateTime) {
$rowsintimespan++; // count if in timespan
// get line elements
$lineContent = explode("t", $lines[$i]);
// loop through line elements and count them
for ($j = IGNORE_COLS; $j < count($keys); $j++) {
if (!isset($lineContent[$j])) {
continue;
}
if (trim($lineContent[$j]) != '') {
$values[$keys[$j - IGNORE_COLS]]++; // −−− Remove redundant $x var
}
}
}
}
// Debug-Output
echo $rowsintimespan . PHP_EOL;
// Output every column
echo '<pre>' . PHP_EOL;
print_r($values);
You should subtract the number of columns:
$values[$keys[$x]]++;
→ $values[$keys[$x - 2]]++;
Here is an updated code snippet:
<?php
$fromDateTime = new DateTime('Tue, Nov 13 2018 08:00:00');
$toDateTime = new DateTime('Tue, Nov 13 2018 19:59:00');
$file = file_get_contents('reject_new.txt');
$lines = explode("n", $file);
const IGNORE_COLS = 2; // +++ Introduced const for number of columns to skip
// counter
$rowsintimespan = 0;
// keys should correspond to columns
$keys = [
// 'Date', // +++
// 'Time', // +++
'Battery level',
'Piezo sound level',
'Left (Channel 3) light intensity',
'Right (Channel 1) light intensity'
];
$values = array_fill(0, count($keys), 0);
$values = array_combine($keys, $values);
// Do Line-By-Line starting by Line 12 (Array Index 11)
for ($i = 11; $i < count($lines); $i++) {
// if the file is "Tue, Sep 18 2018<tab>23:59:53<tab>"
$dateobj = DateTime::createFromFormat("???,?M?d??Y?H:i:s+", $lines[$i]);
// check if date is in your Timespan
if ($dateobj < $toDateTime && $dateobj > $fromDateTime) {
$rowsintimespan++; // count if in timespan
// get line elements
$lineContent = explode("t", $lines[$i]);
// loop through line elements and count them
for ($j = IGNORE_COLS; $j < count($keys); $j++) {
if (!isset($lineContent[$j])) {
continue;
}
if (trim($lineContent[$j]) != '') {
$values[$keys[$j - IGNORE_COLS]]++; // −−− Remove redundant $x var
}
}
}
}
// Debug-Output
echo $rowsintimespan . PHP_EOL;
// Output every column
echo '<pre>' . PHP_EOL;
print_r($values);
answered Nov 13 '18 at 2:50


terales
1,6821526
1,6821526
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53272556%2fphp-read-text-file-ignore-specific-tab%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
EF8 r 3uDQ8tq,ayypJAvZds6 z0 vk,kx0fq6bHmfiRzxKOej4VhRyd1,7ZPGd1wj2b8JdiUW1c3oS
Your question isn't clear to me. Are you trying to get only the values between a certain timeframe,is that it?
– Pedro Lobito
Nov 13 '18 at 3:39
1
You may find some parts of the code interesting, still, not enough for an answer, as I don't fully understand your question - gist.github.com/x011/a764ee4dab50158725a15180b9c948fa
– Pedro Lobito
Nov 13 '18 at 4:22