How to swap between functions implementation?
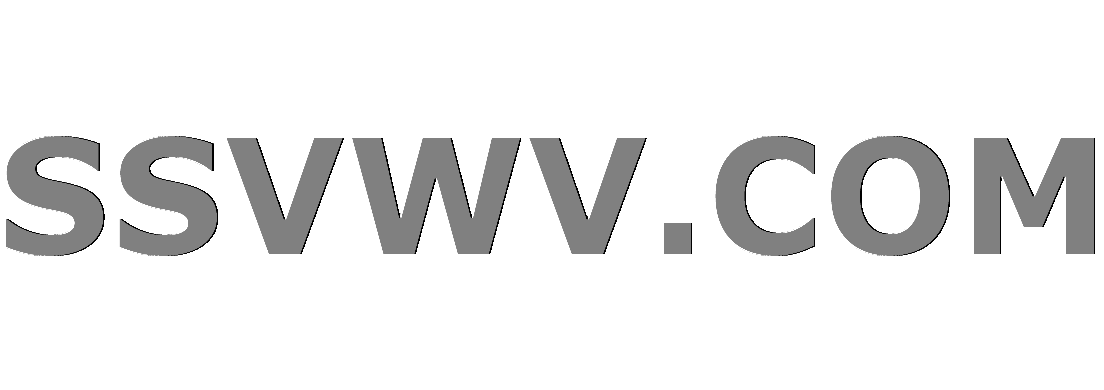
Multi tool use
Is there any way to swap between two functions implementation in C++ ?
Something like this:
void printA(); // print a char
void printB(); // print b char
printA(); // output: a
printB(); // output: b
functionSwap(printA, printB);
printA(); // output: b
printB(); // output: a
I want to use it with the ExitProcess
function.
c++ c++11
add a comment |
Is there any way to swap between two functions implementation in C++ ?
Something like this:
void printA(); // print a char
void printB(); // print b char
printA(); // output: a
printB(); // output: b
functionSwap(printA, printB);
printA(); // output: b
printB(); // output: a
I want to use it with the ExitProcess
function.
c++ c++11
5
This seems to be a possible instance of the XY problem. What is the root goal you're trying to achieve by swappingExitProcess
?
– Andrey Akhmetov
Nov 13 '18 at 14:26
@AndreyAkhmetov I am participating in some kind of competition in which everyone want to make the enemy process stop and there is the option of using DLL injection and using the ExitProcess function in order to make my process exit so I want to avoid the option of others to use the function in my process.
– Mor Ben-Yosef
Nov 13 '18 at 14:31
add a comment |
Is there any way to swap between two functions implementation in C++ ?
Something like this:
void printA(); // print a char
void printB(); // print b char
printA(); // output: a
printB(); // output: b
functionSwap(printA, printB);
printA(); // output: b
printB(); // output: a
I want to use it with the ExitProcess
function.
c++ c++11
Is there any way to swap between two functions implementation in C++ ?
Something like this:
void printA(); // print a char
void printB(); // print b char
printA(); // output: a
printB(); // output: b
functionSwap(printA, printB);
printA(); // output: b
printB(); // output: a
I want to use it with the ExitProcess
function.
c++ c++11
c++ c++11
edited Nov 13 '18 at 14:29
Matthieu Brucher
13.5k32140
13.5k32140
asked Nov 13 '18 at 14:24


Mor Ben-YosefMor Ben-Yosef
537
537
5
This seems to be a possible instance of the XY problem. What is the root goal you're trying to achieve by swappingExitProcess
?
– Andrey Akhmetov
Nov 13 '18 at 14:26
@AndreyAkhmetov I am participating in some kind of competition in which everyone want to make the enemy process stop and there is the option of using DLL injection and using the ExitProcess function in order to make my process exit so I want to avoid the option of others to use the function in my process.
– Mor Ben-Yosef
Nov 13 '18 at 14:31
add a comment |
5
This seems to be a possible instance of the XY problem. What is the root goal you're trying to achieve by swappingExitProcess
?
– Andrey Akhmetov
Nov 13 '18 at 14:26
@AndreyAkhmetov I am participating in some kind of competition in which everyone want to make the enemy process stop and there is the option of using DLL injection and using the ExitProcess function in order to make my process exit so I want to avoid the option of others to use the function in my process.
– Mor Ben-Yosef
Nov 13 '18 at 14:31
5
5
This seems to be a possible instance of the XY problem. What is the root goal you're trying to achieve by swapping
ExitProcess
?– Andrey Akhmetov
Nov 13 '18 at 14:26
This seems to be a possible instance of the XY problem. What is the root goal you're trying to achieve by swapping
ExitProcess
?– Andrey Akhmetov
Nov 13 '18 at 14:26
@AndreyAkhmetov I am participating in some kind of competition in which everyone want to make the enemy process stop and there is the option of using DLL injection and using the ExitProcess function in order to make my process exit so I want to avoid the option of others to use the function in my process.
– Mor Ben-Yosef
Nov 13 '18 at 14:31
@AndreyAkhmetov I am participating in some kind of competition in which everyone want to make the enemy process stop and there is the option of using DLL injection and using the ExitProcess function in order to make my process exit so I want to avoid the option of others to use the function in my process.
– Mor Ben-Yosef
Nov 13 '18 at 14:31
add a comment |
2 Answers
2
active
oldest
votes
You can bind a pointer to both functions in two variables and swap those.
void (*f1)() = printA;
void (*f2)() = printB;
f1(); // output: a
f2(); // output: b
std::swap(f1, f2);
f1(); // output: b
f2(); // output: a
Don't use c function pointers, butstd::function
instead
– hellow
Nov 13 '18 at 14:29
13
@hellow no.std::function
is a heavywieght type-erased container for callable objects. If a function pointer is enough, use that.
– Quentin
Nov 13 '18 at 14:32
add a comment |
You need to wrap them in objects (or pointers to functions):
std::function<void()> myprintA = printA;
std::function<void()> myprintB = printB;
std::swap(myprintA, myprintB);
myprintA();
myprintB();
Otherwise, you are working with symbols themselves, and you can't swap this.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53283181%2fhow-to-swap-between-functions-implementation%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can bind a pointer to both functions in two variables and swap those.
void (*f1)() = printA;
void (*f2)() = printB;
f1(); // output: a
f2(); // output: b
std::swap(f1, f2);
f1(); // output: b
f2(); // output: a
Don't use c function pointers, butstd::function
instead
– hellow
Nov 13 '18 at 14:29
13
@hellow no.std::function
is a heavywieght type-erased container for callable objects. If a function pointer is enough, use that.
– Quentin
Nov 13 '18 at 14:32
add a comment |
You can bind a pointer to both functions in two variables and swap those.
void (*f1)() = printA;
void (*f2)() = printB;
f1(); // output: a
f2(); // output: b
std::swap(f1, f2);
f1(); // output: b
f2(); // output: a
Don't use c function pointers, butstd::function
instead
– hellow
Nov 13 '18 at 14:29
13
@hellow no.std::function
is a heavywieght type-erased container for callable objects. If a function pointer is enough, use that.
– Quentin
Nov 13 '18 at 14:32
add a comment |
You can bind a pointer to both functions in two variables and swap those.
void (*f1)() = printA;
void (*f2)() = printB;
f1(); // output: a
f2(); // output: b
std::swap(f1, f2);
f1(); // output: b
f2(); // output: a
You can bind a pointer to both functions in two variables and swap those.
void (*f1)() = printA;
void (*f2)() = printB;
f1(); // output: a
f2(); // output: b
std::swap(f1, f2);
f1(); // output: b
f2(); // output: a
answered Nov 13 '18 at 14:28


lubgrlubgr
10.4k21745
10.4k21745
Don't use c function pointers, butstd::function
instead
– hellow
Nov 13 '18 at 14:29
13
@hellow no.std::function
is a heavywieght type-erased container for callable objects. If a function pointer is enough, use that.
– Quentin
Nov 13 '18 at 14:32
add a comment |
Don't use c function pointers, butstd::function
instead
– hellow
Nov 13 '18 at 14:29
13
@hellow no.std::function
is a heavywieght type-erased container for callable objects. If a function pointer is enough, use that.
– Quentin
Nov 13 '18 at 14:32
Don't use c function pointers, but
std::function
instead– hellow
Nov 13 '18 at 14:29
Don't use c function pointers, but
std::function
instead– hellow
Nov 13 '18 at 14:29
13
13
@hellow no.
std::function
is a heavywieght type-erased container for callable objects. If a function pointer is enough, use that.– Quentin
Nov 13 '18 at 14:32
@hellow no.
std::function
is a heavywieght type-erased container for callable objects. If a function pointer is enough, use that.– Quentin
Nov 13 '18 at 14:32
add a comment |
You need to wrap them in objects (or pointers to functions):
std::function<void()> myprintA = printA;
std::function<void()> myprintB = printB;
std::swap(myprintA, myprintB);
myprintA();
myprintB();
Otherwise, you are working with symbols themselves, and you can't swap this.
add a comment |
You need to wrap them in objects (or pointers to functions):
std::function<void()> myprintA = printA;
std::function<void()> myprintB = printB;
std::swap(myprintA, myprintB);
myprintA();
myprintB();
Otherwise, you are working with symbols themselves, and you can't swap this.
add a comment |
You need to wrap them in objects (or pointers to functions):
std::function<void()> myprintA = printA;
std::function<void()> myprintB = printB;
std::swap(myprintA, myprintB);
myprintA();
myprintB();
Otherwise, you are working with symbols themselves, and you can't swap this.
You need to wrap them in objects (or pointers to functions):
std::function<void()> myprintA = printA;
std::function<void()> myprintB = printB;
std::swap(myprintA, myprintB);
myprintA();
myprintB();
Otherwise, you are working with symbols themselves, and you can't swap this.
answered Nov 13 '18 at 14:28
Matthieu BrucherMatthieu Brucher
13.5k32140
13.5k32140
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53283181%2fhow-to-swap-between-functions-implementation%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
DY3 C23 2 506ekg
5
This seems to be a possible instance of the XY problem. What is the root goal you're trying to achieve by swapping
ExitProcess
?– Andrey Akhmetov
Nov 13 '18 at 14:26
@AndreyAkhmetov I am participating in some kind of competition in which everyone want to make the enemy process stop and there is the option of using DLL injection and using the ExitProcess function in order to make my process exit so I want to avoid the option of others to use the function in my process.
– Mor Ben-Yosef
Nov 13 '18 at 14:31