Unable to get form data after setState
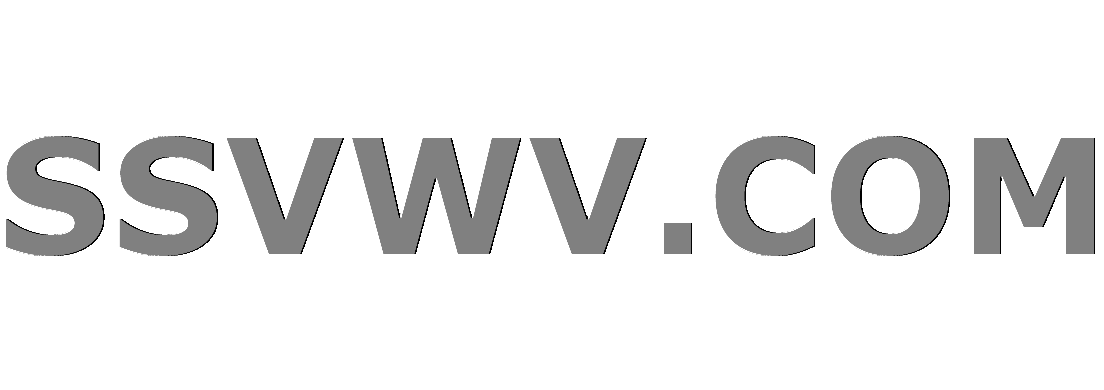
Multi tool use
up vote
-1
down vote
favorite
I am trying to send form data after submitting to the firebase
database using redux
in my react application, but I am unable to send the state so I tried tracking form data by console.log
but the form data is empty. I am able to console log params
but not this.state
Below is the code.
I am using redux
in this app. createUser
is my action
import React, { Component } from "react";
import { connect } from "react-redux";
import { createUser } from "../store/actions/userActions";
class UserForm extends Component {
constructor(props) {
super(props);
this.state = { form: , alert: false, alertData: {} };
this.submitMessage = this.submitMessage.bind(this);
}
submitMessage = e => {
e.preventDefault();
const params = {
name: this.inputName.value,
email: this.inputEmail.value,
city: this.inputCity.value,
age: this.inputAge.value
};
this.setState({ form: params });
console.log(params);
console.log("-----------");
console.log(this.state);
this.props.createUser(this.state);
};
render() {
return (
<div className="container">
<div
className="row"
>
User
</div>
<div className="container" style={{ padding: `10px 0px` }} />
<div className="row">
<div className="col-sm-4">
<h2>Contact Form</h2>
<form onSubmit={this.submitMessage} ref="contactForm">
<div className="form-group">
<label htmlFor="name">Name</label>
<input
type="text"
className="form-control"
id="name"
placeholder="Name"
ref={name => (this.inputName = name)}
/>
</div>
<div className="form-group">
<label htmlFor="emai1">Email</label>
<input
type="email"
className="form-control"
id="email"
placeholder="Email"
ref={email => (this.inputEmail = email)}
/>
</div>
<div className="form-group">
<label htmlFor="city">City</label>
<select
className="form-control"
id="city"
ref={city => (this.inputCity = city)}
>
<option value="India">India</option>
<option value="USA">USA</option>
<option value="UK">UK</option>
<option value="Japan">Japan</option>
<option value="Germany">Germany</option>
</select>
</div>
<div className="form-group">
<label htmlFor="age">Age</label>
<input
type="number"
className="form-control"
id="age"
placeholder="Age"
ref={age => (this.inputAge = age)}
/>
</div>
<button type="submit" className="btn btn-primary">
Send
</button>
</form>
</div>
</div>
</div>
);
}
}
const mapStateToProps = state => {
return {};
};
const mapDispatchToProps = dispatch => {
return {
createUser: user => dispatch(createUser)
};
};
export default connect(
mapStateToProps,
mapDispatchToProps
)(UserForm);
javascript reactjs react-redux console.log
add a comment |
up vote
-1
down vote
favorite
I am trying to send form data after submitting to the firebase
database using redux
in my react application, but I am unable to send the state so I tried tracking form data by console.log
but the form data is empty. I am able to console log params
but not this.state
Below is the code.
I am using redux
in this app. createUser
is my action
import React, { Component } from "react";
import { connect } from "react-redux";
import { createUser } from "../store/actions/userActions";
class UserForm extends Component {
constructor(props) {
super(props);
this.state = { form: , alert: false, alertData: {} };
this.submitMessage = this.submitMessage.bind(this);
}
submitMessage = e => {
e.preventDefault();
const params = {
name: this.inputName.value,
email: this.inputEmail.value,
city: this.inputCity.value,
age: this.inputAge.value
};
this.setState({ form: params });
console.log(params);
console.log("-----------");
console.log(this.state);
this.props.createUser(this.state);
};
render() {
return (
<div className="container">
<div
className="row"
>
User
</div>
<div className="container" style={{ padding: `10px 0px` }} />
<div className="row">
<div className="col-sm-4">
<h2>Contact Form</h2>
<form onSubmit={this.submitMessage} ref="contactForm">
<div className="form-group">
<label htmlFor="name">Name</label>
<input
type="text"
className="form-control"
id="name"
placeholder="Name"
ref={name => (this.inputName = name)}
/>
</div>
<div className="form-group">
<label htmlFor="emai1">Email</label>
<input
type="email"
className="form-control"
id="email"
placeholder="Email"
ref={email => (this.inputEmail = email)}
/>
</div>
<div className="form-group">
<label htmlFor="city">City</label>
<select
className="form-control"
id="city"
ref={city => (this.inputCity = city)}
>
<option value="India">India</option>
<option value="USA">USA</option>
<option value="UK">UK</option>
<option value="Japan">Japan</option>
<option value="Germany">Germany</option>
</select>
</div>
<div className="form-group">
<label htmlFor="age">Age</label>
<input
type="number"
className="form-control"
id="age"
placeholder="Age"
ref={age => (this.inputAge = age)}
/>
</div>
<button type="submit" className="btn btn-primary">
Send
</button>
</form>
</div>
</div>
</div>
);
}
}
const mapStateToProps = state => {
return {};
};
const mapDispatchToProps = dispatch => {
return {
createUser: user => dispatch(createUser)
};
};
export default connect(
mapStateToProps,
mapDispatchToProps
)(UserForm);
javascript reactjs react-redux console.log
i think you initialize your form state as array and you are setting an object into it.
– Rahul Dudharejiya
Nov 12 at 6:59
callback in setState got me data. Now that you mentioned that I declared array and used object in submit function
– Abhishek Konnur
Nov 12 at 7:11
add a comment |
up vote
-1
down vote
favorite
up vote
-1
down vote
favorite
I am trying to send form data after submitting to the firebase
database using redux
in my react application, but I am unable to send the state so I tried tracking form data by console.log
but the form data is empty. I am able to console log params
but not this.state
Below is the code.
I am using redux
in this app. createUser
is my action
import React, { Component } from "react";
import { connect } from "react-redux";
import { createUser } from "../store/actions/userActions";
class UserForm extends Component {
constructor(props) {
super(props);
this.state = { form: , alert: false, alertData: {} };
this.submitMessage = this.submitMessage.bind(this);
}
submitMessage = e => {
e.preventDefault();
const params = {
name: this.inputName.value,
email: this.inputEmail.value,
city: this.inputCity.value,
age: this.inputAge.value
};
this.setState({ form: params });
console.log(params);
console.log("-----------");
console.log(this.state);
this.props.createUser(this.state);
};
render() {
return (
<div className="container">
<div
className="row"
>
User
</div>
<div className="container" style={{ padding: `10px 0px` }} />
<div className="row">
<div className="col-sm-4">
<h2>Contact Form</h2>
<form onSubmit={this.submitMessage} ref="contactForm">
<div className="form-group">
<label htmlFor="name">Name</label>
<input
type="text"
className="form-control"
id="name"
placeholder="Name"
ref={name => (this.inputName = name)}
/>
</div>
<div className="form-group">
<label htmlFor="emai1">Email</label>
<input
type="email"
className="form-control"
id="email"
placeholder="Email"
ref={email => (this.inputEmail = email)}
/>
</div>
<div className="form-group">
<label htmlFor="city">City</label>
<select
className="form-control"
id="city"
ref={city => (this.inputCity = city)}
>
<option value="India">India</option>
<option value="USA">USA</option>
<option value="UK">UK</option>
<option value="Japan">Japan</option>
<option value="Germany">Germany</option>
</select>
</div>
<div className="form-group">
<label htmlFor="age">Age</label>
<input
type="number"
className="form-control"
id="age"
placeholder="Age"
ref={age => (this.inputAge = age)}
/>
</div>
<button type="submit" className="btn btn-primary">
Send
</button>
</form>
</div>
</div>
</div>
);
}
}
const mapStateToProps = state => {
return {};
};
const mapDispatchToProps = dispatch => {
return {
createUser: user => dispatch(createUser)
};
};
export default connect(
mapStateToProps,
mapDispatchToProps
)(UserForm);
javascript reactjs react-redux console.log
I am trying to send form data after submitting to the firebase
database using redux
in my react application, but I am unable to send the state so I tried tracking form data by console.log
but the form data is empty. I am able to console log params
but not this.state
Below is the code.
I am using redux
in this app. createUser
is my action
import React, { Component } from "react";
import { connect } from "react-redux";
import { createUser } from "../store/actions/userActions";
class UserForm extends Component {
constructor(props) {
super(props);
this.state = { form: , alert: false, alertData: {} };
this.submitMessage = this.submitMessage.bind(this);
}
submitMessage = e => {
e.preventDefault();
const params = {
name: this.inputName.value,
email: this.inputEmail.value,
city: this.inputCity.value,
age: this.inputAge.value
};
this.setState({ form: params });
console.log(params);
console.log("-----------");
console.log(this.state);
this.props.createUser(this.state);
};
render() {
return (
<div className="container">
<div
className="row"
>
User
</div>
<div className="container" style={{ padding: `10px 0px` }} />
<div className="row">
<div className="col-sm-4">
<h2>Contact Form</h2>
<form onSubmit={this.submitMessage} ref="contactForm">
<div className="form-group">
<label htmlFor="name">Name</label>
<input
type="text"
className="form-control"
id="name"
placeholder="Name"
ref={name => (this.inputName = name)}
/>
</div>
<div className="form-group">
<label htmlFor="emai1">Email</label>
<input
type="email"
className="form-control"
id="email"
placeholder="Email"
ref={email => (this.inputEmail = email)}
/>
</div>
<div className="form-group">
<label htmlFor="city">City</label>
<select
className="form-control"
id="city"
ref={city => (this.inputCity = city)}
>
<option value="India">India</option>
<option value="USA">USA</option>
<option value="UK">UK</option>
<option value="Japan">Japan</option>
<option value="Germany">Germany</option>
</select>
</div>
<div className="form-group">
<label htmlFor="age">Age</label>
<input
type="number"
className="form-control"
id="age"
placeholder="Age"
ref={age => (this.inputAge = age)}
/>
</div>
<button type="submit" className="btn btn-primary">
Send
</button>
</form>
</div>
</div>
</div>
);
}
}
const mapStateToProps = state => {
return {};
};
const mapDispatchToProps = dispatch => {
return {
createUser: user => dispatch(createUser)
};
};
export default connect(
mapStateToProps,
mapDispatchToProps
)(UserForm);
javascript reactjs react-redux console.log
javascript reactjs react-redux console.log
edited Nov 12 at 9:32
ProgrammerPer
461511
461511
asked Nov 12 at 6:56


Abhishek Konnur
7411
7411
i think you initialize your form state as array and you are setting an object into it.
– Rahul Dudharejiya
Nov 12 at 6:59
callback in setState got me data. Now that you mentioned that I declared array and used object in submit function
– Abhishek Konnur
Nov 12 at 7:11
add a comment |
i think you initialize your form state as array and you are setting an object into it.
– Rahul Dudharejiya
Nov 12 at 6:59
callback in setState got me data. Now that you mentioned that I declared array and used object in submit function
– Abhishek Konnur
Nov 12 at 7:11
i think you initialize your form state as array and you are setting an object into it.
– Rahul Dudharejiya
Nov 12 at 6:59
i think you initialize your form state as array and you are setting an object into it.
– Rahul Dudharejiya
Nov 12 at 6:59
callback in setState got me data. Now that you mentioned that I declared array and used object in submit function
– Abhishek Konnur
Nov 12 at 7:11
callback in setState got me data. Now that you mentioned that I declared array and used object in submit function
– Abhishek Konnur
Nov 12 at 7:11
add a comment |
3 Answers
3
active
oldest
votes
up vote
0
down vote
accepted
There is you are missing two things,
Set state is async in nature so after set state, you must use call back to access it.
You are not passing form data ie. Params to createUser
Solution :
import React, { Component } from "react";
import { connect } from "react-redux";
import { createUser } from "../store/actions/userActions";
class UserForm extends Component {
constructor(props) {
super(props);
this.state = { form: , alert: false, alertData: {} };
this.submitMessage = this.submitMessage.bind(this);
}
submitMessage = e => {
e.preventDefault();
const params = {
name: this.inputName.value,
email: this.inputEmail.value,
city: this.inputCity.value,
age: this.inputAge.value
};
this.setState({ form: params },()=>{
this.props.createUser(this.state);
});
};
render() {
return (
<div className="container">
<div
className="row"
>
User
</div>
<div className="container" style={{ padding: `10px 0px` }} />
<div className="row">
<div className="col-sm-4">
<h2>Contact Form</h2>
<form onSubmit={this.submitMessage} ref="contactForm">
<div className="form-group">
<label htmlFor="name">Name</label>
<input
type="text"
className="form-control"
id="name"
placeholder="Name"
ref={name => (this.inputName = name)}
/>
</div>
<div className="form-group">
<label htmlFor="emai1">Email</label>
<input
type="email"
className="form-control"
id="email"
placeholder="Email"
ref={email => (this.inputEmail = email)}
/>
</div>
<div className="form-group">
<label htmlFor="city">City</label>
<select
className="form-control"
id="city"
ref={city => (this.inputCity = city)}
>
<option value="India">India</option>
<option value="USA">USA</option>
<option value="UK">UK</option>
<option value="Japan">Japan</option>
<option value="Germany">Germany</option>
</select>
</div>
<div className="form-group">
<label htmlFor="age">Age</label>
<input
type="number"
className="form-control"
id="age"
placeholder="Age"
ref={age => (this.inputAge = age)}
/>
</div>
<button type="submit" className="btn btn-primary">
Send
</button>
</form>
</div>
</div>
</div>
);
}
}
const mapStateToProps = state => {
return {};
};
const mapDispatchToProps = dispatch => {
return {
createUser: user => dispatch(createUser(user))
};
};
export default connect(
mapStateToProps,
mapDispatchToProps
)(UserForm);
This works fine for me but unable to get the sent data into my firebase. As I can see mapDispatchToProps is correct.
– Abhishek Konnur
Nov 12 at 7:28
add a comment |
up vote
1
down vote
Due to setState
is asynchronous you can access the changed state in a callback which you can pass as a second param to setState
method. Please read more about setState
submitMessage = e => {
e.preventDefault();
const params = {
name: this.inputName.value,
email: this.inputEmail.value,
city: this.inputCity.value,
age: this.inputAge.value
};
this.setState({ form: params }, () => {
console.log(params);
console.log("-----------");
console.log(this.state);
this.props.createUser(this.state);
});
};
This worked for me
– Abhishek Konnur
Nov 12 at 7:11
add a comment |
up vote
1
down vote
You call createUser
before setState is finished. Why use the state anyway? I would suggest:
submitMessage = e => {
e.preventDefault();
const params = {
name: this.inputName.value,
email: this.inputEmail.value,
city: this.inputCity.value,
age: this.inputAge.value
};
this.props.createUser(params);
};
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53257197%2funable-to-get-form-data-after-setstate%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
There is you are missing two things,
Set state is async in nature so after set state, you must use call back to access it.
You are not passing form data ie. Params to createUser
Solution :
import React, { Component } from "react";
import { connect } from "react-redux";
import { createUser } from "../store/actions/userActions";
class UserForm extends Component {
constructor(props) {
super(props);
this.state = { form: , alert: false, alertData: {} };
this.submitMessage = this.submitMessage.bind(this);
}
submitMessage = e => {
e.preventDefault();
const params = {
name: this.inputName.value,
email: this.inputEmail.value,
city: this.inputCity.value,
age: this.inputAge.value
};
this.setState({ form: params },()=>{
this.props.createUser(this.state);
});
};
render() {
return (
<div className="container">
<div
className="row"
>
User
</div>
<div className="container" style={{ padding: `10px 0px` }} />
<div className="row">
<div className="col-sm-4">
<h2>Contact Form</h2>
<form onSubmit={this.submitMessage} ref="contactForm">
<div className="form-group">
<label htmlFor="name">Name</label>
<input
type="text"
className="form-control"
id="name"
placeholder="Name"
ref={name => (this.inputName = name)}
/>
</div>
<div className="form-group">
<label htmlFor="emai1">Email</label>
<input
type="email"
className="form-control"
id="email"
placeholder="Email"
ref={email => (this.inputEmail = email)}
/>
</div>
<div className="form-group">
<label htmlFor="city">City</label>
<select
className="form-control"
id="city"
ref={city => (this.inputCity = city)}
>
<option value="India">India</option>
<option value="USA">USA</option>
<option value="UK">UK</option>
<option value="Japan">Japan</option>
<option value="Germany">Germany</option>
</select>
</div>
<div className="form-group">
<label htmlFor="age">Age</label>
<input
type="number"
className="form-control"
id="age"
placeholder="Age"
ref={age => (this.inputAge = age)}
/>
</div>
<button type="submit" className="btn btn-primary">
Send
</button>
</form>
</div>
</div>
</div>
);
}
}
const mapStateToProps = state => {
return {};
};
const mapDispatchToProps = dispatch => {
return {
createUser: user => dispatch(createUser(user))
};
};
export default connect(
mapStateToProps,
mapDispatchToProps
)(UserForm);
This works fine for me but unable to get the sent data into my firebase. As I can see mapDispatchToProps is correct.
– Abhishek Konnur
Nov 12 at 7:28
add a comment |
up vote
0
down vote
accepted
There is you are missing two things,
Set state is async in nature so after set state, you must use call back to access it.
You are not passing form data ie. Params to createUser
Solution :
import React, { Component } from "react";
import { connect } from "react-redux";
import { createUser } from "../store/actions/userActions";
class UserForm extends Component {
constructor(props) {
super(props);
this.state = { form: , alert: false, alertData: {} };
this.submitMessage = this.submitMessage.bind(this);
}
submitMessage = e => {
e.preventDefault();
const params = {
name: this.inputName.value,
email: this.inputEmail.value,
city: this.inputCity.value,
age: this.inputAge.value
};
this.setState({ form: params },()=>{
this.props.createUser(this.state);
});
};
render() {
return (
<div className="container">
<div
className="row"
>
User
</div>
<div className="container" style={{ padding: `10px 0px` }} />
<div className="row">
<div className="col-sm-4">
<h2>Contact Form</h2>
<form onSubmit={this.submitMessage} ref="contactForm">
<div className="form-group">
<label htmlFor="name">Name</label>
<input
type="text"
className="form-control"
id="name"
placeholder="Name"
ref={name => (this.inputName = name)}
/>
</div>
<div className="form-group">
<label htmlFor="emai1">Email</label>
<input
type="email"
className="form-control"
id="email"
placeholder="Email"
ref={email => (this.inputEmail = email)}
/>
</div>
<div className="form-group">
<label htmlFor="city">City</label>
<select
className="form-control"
id="city"
ref={city => (this.inputCity = city)}
>
<option value="India">India</option>
<option value="USA">USA</option>
<option value="UK">UK</option>
<option value="Japan">Japan</option>
<option value="Germany">Germany</option>
</select>
</div>
<div className="form-group">
<label htmlFor="age">Age</label>
<input
type="number"
className="form-control"
id="age"
placeholder="Age"
ref={age => (this.inputAge = age)}
/>
</div>
<button type="submit" className="btn btn-primary">
Send
</button>
</form>
</div>
</div>
</div>
);
}
}
const mapStateToProps = state => {
return {};
};
const mapDispatchToProps = dispatch => {
return {
createUser: user => dispatch(createUser(user))
};
};
export default connect(
mapStateToProps,
mapDispatchToProps
)(UserForm);
This works fine for me but unable to get the sent data into my firebase. As I can see mapDispatchToProps is correct.
– Abhishek Konnur
Nov 12 at 7:28
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
There is you are missing two things,
Set state is async in nature so after set state, you must use call back to access it.
You are not passing form data ie. Params to createUser
Solution :
import React, { Component } from "react";
import { connect } from "react-redux";
import { createUser } from "../store/actions/userActions";
class UserForm extends Component {
constructor(props) {
super(props);
this.state = { form: , alert: false, alertData: {} };
this.submitMessage = this.submitMessage.bind(this);
}
submitMessage = e => {
e.preventDefault();
const params = {
name: this.inputName.value,
email: this.inputEmail.value,
city: this.inputCity.value,
age: this.inputAge.value
};
this.setState({ form: params },()=>{
this.props.createUser(this.state);
});
};
render() {
return (
<div className="container">
<div
className="row"
>
User
</div>
<div className="container" style={{ padding: `10px 0px` }} />
<div className="row">
<div className="col-sm-4">
<h2>Contact Form</h2>
<form onSubmit={this.submitMessage} ref="contactForm">
<div className="form-group">
<label htmlFor="name">Name</label>
<input
type="text"
className="form-control"
id="name"
placeholder="Name"
ref={name => (this.inputName = name)}
/>
</div>
<div className="form-group">
<label htmlFor="emai1">Email</label>
<input
type="email"
className="form-control"
id="email"
placeholder="Email"
ref={email => (this.inputEmail = email)}
/>
</div>
<div className="form-group">
<label htmlFor="city">City</label>
<select
className="form-control"
id="city"
ref={city => (this.inputCity = city)}
>
<option value="India">India</option>
<option value="USA">USA</option>
<option value="UK">UK</option>
<option value="Japan">Japan</option>
<option value="Germany">Germany</option>
</select>
</div>
<div className="form-group">
<label htmlFor="age">Age</label>
<input
type="number"
className="form-control"
id="age"
placeholder="Age"
ref={age => (this.inputAge = age)}
/>
</div>
<button type="submit" className="btn btn-primary">
Send
</button>
</form>
</div>
</div>
</div>
);
}
}
const mapStateToProps = state => {
return {};
};
const mapDispatchToProps = dispatch => {
return {
createUser: user => dispatch(createUser(user))
};
};
export default connect(
mapStateToProps,
mapDispatchToProps
)(UserForm);
There is you are missing two things,
Set state is async in nature so after set state, you must use call back to access it.
You are not passing form data ie. Params to createUser
Solution :
import React, { Component } from "react";
import { connect } from "react-redux";
import { createUser } from "../store/actions/userActions";
class UserForm extends Component {
constructor(props) {
super(props);
this.state = { form: , alert: false, alertData: {} };
this.submitMessage = this.submitMessage.bind(this);
}
submitMessage = e => {
e.preventDefault();
const params = {
name: this.inputName.value,
email: this.inputEmail.value,
city: this.inputCity.value,
age: this.inputAge.value
};
this.setState({ form: params },()=>{
this.props.createUser(this.state);
});
};
render() {
return (
<div className="container">
<div
className="row"
>
User
</div>
<div className="container" style={{ padding: `10px 0px` }} />
<div className="row">
<div className="col-sm-4">
<h2>Contact Form</h2>
<form onSubmit={this.submitMessage} ref="contactForm">
<div className="form-group">
<label htmlFor="name">Name</label>
<input
type="text"
className="form-control"
id="name"
placeholder="Name"
ref={name => (this.inputName = name)}
/>
</div>
<div className="form-group">
<label htmlFor="emai1">Email</label>
<input
type="email"
className="form-control"
id="email"
placeholder="Email"
ref={email => (this.inputEmail = email)}
/>
</div>
<div className="form-group">
<label htmlFor="city">City</label>
<select
className="form-control"
id="city"
ref={city => (this.inputCity = city)}
>
<option value="India">India</option>
<option value="USA">USA</option>
<option value="UK">UK</option>
<option value="Japan">Japan</option>
<option value="Germany">Germany</option>
</select>
</div>
<div className="form-group">
<label htmlFor="age">Age</label>
<input
type="number"
className="form-control"
id="age"
placeholder="Age"
ref={age => (this.inputAge = age)}
/>
</div>
<button type="submit" className="btn btn-primary">
Send
</button>
</form>
</div>
</div>
</div>
);
}
}
const mapStateToProps = state => {
return {};
};
const mapDispatchToProps = dispatch => {
return {
createUser: user => dispatch(createUser(user))
};
};
export default connect(
mapStateToProps,
mapDispatchToProps
)(UserForm);
answered Nov 12 at 7:06


RANVIR GORAI
65547
65547
This works fine for me but unable to get the sent data into my firebase. As I can see mapDispatchToProps is correct.
– Abhishek Konnur
Nov 12 at 7:28
add a comment |
This works fine for me but unable to get the sent data into my firebase. As I can see mapDispatchToProps is correct.
– Abhishek Konnur
Nov 12 at 7:28
This works fine for me but unable to get the sent data into my firebase. As I can see mapDispatchToProps is correct.
– Abhishek Konnur
Nov 12 at 7:28
This works fine for me but unable to get the sent data into my firebase. As I can see mapDispatchToProps is correct.
– Abhishek Konnur
Nov 12 at 7:28
add a comment |
up vote
1
down vote
Due to setState
is asynchronous you can access the changed state in a callback which you can pass as a second param to setState
method. Please read more about setState
submitMessage = e => {
e.preventDefault();
const params = {
name: this.inputName.value,
email: this.inputEmail.value,
city: this.inputCity.value,
age: this.inputAge.value
};
this.setState({ form: params }, () => {
console.log(params);
console.log("-----------");
console.log(this.state);
this.props.createUser(this.state);
});
};
This worked for me
– Abhishek Konnur
Nov 12 at 7:11
add a comment |
up vote
1
down vote
Due to setState
is asynchronous you can access the changed state in a callback which you can pass as a second param to setState
method. Please read more about setState
submitMessage = e => {
e.preventDefault();
const params = {
name: this.inputName.value,
email: this.inputEmail.value,
city: this.inputCity.value,
age: this.inputAge.value
};
this.setState({ form: params }, () => {
console.log(params);
console.log("-----------");
console.log(this.state);
this.props.createUser(this.state);
});
};
This worked for me
– Abhishek Konnur
Nov 12 at 7:11
add a comment |
up vote
1
down vote
up vote
1
down vote
Due to setState
is asynchronous you can access the changed state in a callback which you can pass as a second param to setState
method. Please read more about setState
submitMessage = e => {
e.preventDefault();
const params = {
name: this.inputName.value,
email: this.inputEmail.value,
city: this.inputCity.value,
age: this.inputAge.value
};
this.setState({ form: params }, () => {
console.log(params);
console.log("-----------");
console.log(this.state);
this.props.createUser(this.state);
});
};
Due to setState
is asynchronous you can access the changed state in a callback which you can pass as a second param to setState
method. Please read more about setState
submitMessage = e => {
e.preventDefault();
const params = {
name: this.inputName.value,
email: this.inputEmail.value,
city: this.inputCity.value,
age: this.inputAge.value
};
this.setState({ form: params }, () => {
console.log(params);
console.log("-----------");
console.log(this.state);
this.props.createUser(this.state);
});
};
answered Nov 12 at 6:59
falinsky
3,35731942
3,35731942
This worked for me
– Abhishek Konnur
Nov 12 at 7:11
add a comment |
This worked for me
– Abhishek Konnur
Nov 12 at 7:11
This worked for me
– Abhishek Konnur
Nov 12 at 7:11
This worked for me
– Abhishek Konnur
Nov 12 at 7:11
add a comment |
up vote
1
down vote
You call createUser
before setState is finished. Why use the state anyway? I would suggest:
submitMessage = e => {
e.preventDefault();
const params = {
name: this.inputName.value,
email: this.inputEmail.value,
city: this.inputCity.value,
age: this.inputAge.value
};
this.props.createUser(params);
};
add a comment |
up vote
1
down vote
You call createUser
before setState is finished. Why use the state anyway? I would suggest:
submitMessage = e => {
e.preventDefault();
const params = {
name: this.inputName.value,
email: this.inputEmail.value,
city: this.inputCity.value,
age: this.inputAge.value
};
this.props.createUser(params);
};
add a comment |
up vote
1
down vote
up vote
1
down vote
You call createUser
before setState is finished. Why use the state anyway? I would suggest:
submitMessage = e => {
e.preventDefault();
const params = {
name: this.inputName.value,
email: this.inputEmail.value,
city: this.inputCity.value,
age: this.inputAge.value
};
this.props.createUser(params);
};
You call createUser
before setState is finished. Why use the state anyway? I would suggest:
submitMessage = e => {
e.preventDefault();
const params = {
name: this.inputName.value,
email: this.inputEmail.value,
city: this.inputCity.value,
age: this.inputAge.value
};
this.props.createUser(params);
};
answered Nov 12 at 7:06
Juust
407
407
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53257197%2funable-to-get-form-data-after-setstate%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
RtBwwZI8z
i think you initialize your form state as array and you are setting an object into it.
– Rahul Dudharejiya
Nov 12 at 6:59
callback in setState got me data. Now that you mentioned that I declared array and used object in submit function
– Abhishek Konnur
Nov 12 at 7:11