How to fix java.lang.ArrayIndexOutOfBoundsException: length=1; index=1
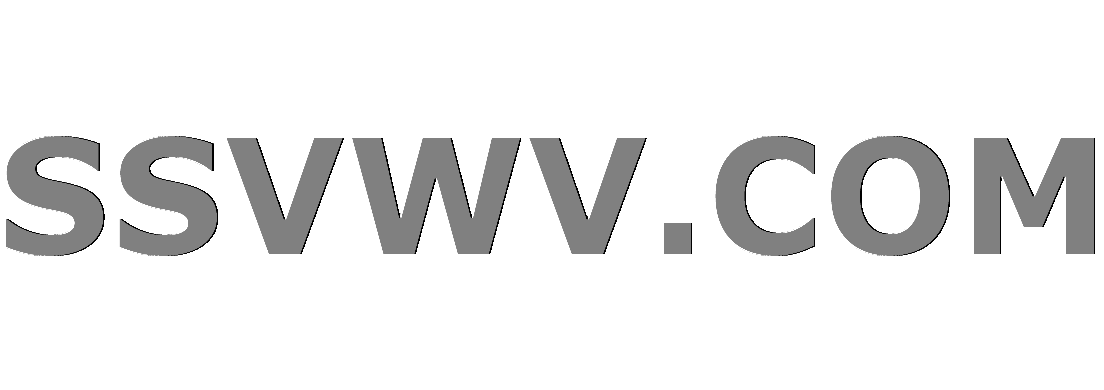
Multi tool use
up vote
2
down vote
favorite
I am creating an android application that consists of a serial communication.I am getting an error called java.lang.ArrayIndexOutOfBoundsException: length=1; index=1 please tell me how to fix it
This is my usb driver:
public class UsbDriver {
private final Context mApplicationContext;
private final UsbManager mUsbManager;
@SuppressWarnings("unused")
private final UsbConnectionHandler mConnectionHandler;
private final int VID;
private final int PID;
protected static final String ACTION_USB_PERMISSION = "ch.serverbox.android.USB";
public static int Device_Exception;
public static UsbDevice Device_Details;
public static UsbEndpoint Data_In_End_Point = null;
public static UsbEndpoint Data_Out_End_Point = null;
public static UsbDeviceConnection USB_Device_Connection;
public UsbDriver(Activity parentActivity,UsbConnectionHandler connectionHandler, int vid, int pid) {
mApplicationContext = parentActivity.getApplicationContext();
mConnectionHandler = connectionHandler;
mUsbManager = (UsbManager) mApplicationContext.getSystemService(Context.USB_SERVICE);
VID = 1027;
PID = 24577;
Device_Exception = 0;
// init();
Check_Devices();
}
private void Check_Devices() {
@SuppressWarnings("unused")
int j=0;
HashMap<String, UsbDevice> devlist = mUsbManager.getDeviceList();
Iterator<UsbDevice> deviter = devlist.values().iterator();
Device_Details = null;
if (devlist.size() != 0) {
while (deviter.hasNext()) {
Device_Details = deviter.next();
if (Device_Details.getVendorId() == VID && Device_Details.getProductId() == PID){
if (!mUsbManager.hasPermission(Device_Details)){
onPermissionDenied(Device_Details);
} else {
UsbDeviceConnection conn = mUsbManager.openDevice(Device_Details);
if (!conn.claimInterface(Device_Details.getInterface(1), true)){
return;
}
conn.controlTransfer(0x21, 34, 0, 0, null, 0, 0);
conn.controlTransfer(0x21, 32, 0, 0,new byte { (byte) 0x80, 0x25 , 0x00, 0x00,0x00, 0x00, 0x08 }, 7, 0);
USB_Device_Connection=conn;
Data_In_End_Point = null;
Data_Out_End_Point = null;
UsbInterface usbIf = Device_Details.getInterface(1);
for (int i = 0; i < usbIf.getEndpointCount(); i++) {
if (usbIf.getEndpoint(i).getType() == UsbConstants.USB_ENDPOINT_XFER_BULK) {
if (usbIf.getEndpoint(i).getDirection() == UsbConstants.USB_DIR_IN)
Data_In_End_Point = usbIf.getEndpoint(i);
else
Data_Out_End_Point = usbIf.getEndpoint(i);
}
}
if (Data_In_End_Point == null || Data_Out_End_Point == null)
Device_Exception = 2;
}
break;
}j++;
}
if (Device_Details == null) {
Device_Exception = 3;
return;
}
}
else {
Device_Exception = 1;
return;
}
}
public void onPermissionDenied(UsbDevice d) {
UsbManager usbman = (UsbManager) mApplicationContext.getSystemService(Context.USB_SERVICE);
PendingIntent pi = PendingIntent.getBroadcast(mApplicationContext, 0, new Intent(ACTION_USB_PERMISSION), 0);
mApplicationContext.registerReceiver(mPermissionReceiver,new IntentFilter(ACTION_USB_PERMISSION));
usbman.requestPermission(d, pi);
}
private class PermissionReceiver extends BroadcastReceiver {
private final IPermissionListener mPermissionListener;
public PermissionReceiver(IPermissionListener permissionListener){
mPermissionListener = permissionListener;
}
@Override
public void onReceive(Context context, Intent intent) {
mApplicationContext.unregisterReceiver(this);
if (intent.getAction().equals(ACTION_USB_PERMISSION)) {
if (!intent.getBooleanExtra(UsbManager.EXTRA_PERMISSION_GRANTED, false)) {
mPermissionListener.onPermissionDenied((UsbDevice) intent.getParcelableExtra(UsbManager.EXTRA_DEVICE));
} else {
l("Permission granted");
UsbDevice dev = (UsbDevice) intent.getParcelableExtra(UsbManager.EXTRA_DEVICE);
if (dev != null){
if (dev.getVendorId() == VID && dev.getProductId() == PID) {
Check_Devices() ;
}
}else{
e("device not present!");
}
}
}
}
}
private BroadcastReceiver mPermissionReceiver = new PermissionReceiver(new IPermissionListener() {
@Override
public void onPermissionDenied(UsbDevice d) {
l("Permission denied on " + d.getDeviceId());
}
});
private static interface IPermissionListener {
void onPermissionDenied(UsbDevice d);
}
public final static String TAG = "USBController";
private void l(Object msg) {
Log.d(TAG, ">==<" + msg.toString() + " >==<");
}
private void e(Object msg) {
Log.e(TAG, ">==< " + msg.toString() + " >==<");
}
}
This is my usb connection handler
public interface UsbConnectionHandler {
void onUsbStopped();
void onErrorLooperRunningAlready();
void onDeviceNotFound();
}
This is my main activity:
public class MainActivity extends Activity {
EditText communication_data;
Button send;
public static final int targetVendorID = 1027;
public static final int targetProductID = 24577;
public UsbManager manager;
public UsbDeviceConnection usbDeviceConnection;
public UsbInterface usbInterfaceFound = null;
public UsbEndpoint endpointOut = null;
public UsbEndpoint endpointIn = null;
public UsbDevice usbdevice,device_details;
public UsbEndpoint listusbendpoint;
private static final int VID = 1027;
private static final int PID = 24577;
@SuppressWarnings("unused")
private static UsbDriver Usb_Driver_class;
ActionBar actionbar;
UsbConnectionHandler connectionHandler;
public static UsbDriver USB_Driver_Child;
public static boolean Communication_Failed,Frame_Ok,Total_Frame_Decoded;
static byte Communication_Byte;
HashMap<String, UsbDevice> devicelist= null;
static byte sample;
static boolean Communication_Ok;
static int Sequence_No,Response_Time;
Thread Receive;
ByteBuffer buffer;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//communication_data = (EditText)findViewById(R.id.editText_comm);
send = (Button)findViewById(R.id.button1_sendin);
send.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Communication_Byte=new byte[1];
if(Check_Devices_Available()==true) {
int Packet_Size = USB_Driver_Child.Data_In_End_Point.getMaxPacketSize();
Toast.makeText(MainActivity.this,""+Packet_Size, Toast.LENGTH_LONG).show();
Receive.start();
Communication_Ok=false;
for(int i=0;(i<5 && Communication_Ok!=true);i++)
Send_Communication_Check_Command();
if(Communication_Ok)
Toast.makeText(MainActivity.this, "Communication Successfully Established", 1000).show();
else
Toast.makeText(MainActivity.this, "Communication Failure", 10000).show();
}
}
private boolean Check_Devices_Available() {
Usb_Driver_class = new UsbDriver(MainActivity.this, connectionHandler, VID, PID);
if(USB_Driver_Child.Device_Exception==0){
if(USB_Driver_Child.USB_Device_Connection==null || USB_Driver_Child.Data_Out_End_Point==null)
return false;
Toast.makeText(MainActivity.this,"Device Found", 1000).show();
return true;
}else if(USB_Driver_Child.Device_Exception==1){
Toast.makeText(MainActivity.this,"No Devices Attached ", Toast.LENGTH_LONG).show();
return false;
}else if(USB_Driver_Child.Device_Exception==2){
Toast.makeText(MainActivity.this,"Device Found,But No End Points", Toast.LENGTH_LONG).show();
return false;
}else if(USB_Driver_Child.Device_Exception==3){
Toast.makeText(MainActivity.this,"Unable to Open Device", Toast.LENGTH_LONG).show();
return false;
}
return false;
}
Thread Receive = new Thread(new Runnable(){
@SuppressWarnings("unused")
@Override
public void run() {
Sequence_No=0;
buffer = ByteBuffer.allocate(64);
sample = new byte[64];
int Frame_Size;
UsbRequest request = new UsbRequest();
int i,j;
byte datarx=new byte[1];
char q;
while (true) {
request.initialize(UsbDriver.USB_Device_Connection, UsbDriver.Data_In_End_Point);
request.queue(buffer, 64);
if (UsbDriver.USB_Device_Connection.requestWait() == request) {
sample=buffer.array();
for(i=0;i<64;i++){
if(sample[i]=='&'){
Communication_Ok=true;
break;
}else if(sample[i]==0x03){
if(sample[0]==0x02)
//Frame_Ok=true;
break;
}
}
if(Frame_Ok==true){
//Frame_Ok=false;
//if(sample[1]==1)
//Coil_No=1;
//else
//Coil_No=2;
//Response_Time= (int)(((sample[2]&0x00FF)<<8) + (sample[3]&0x00FF));
//Total_Frame_Decoded=true;
//sample = null;
}
}
}
}
});
private void Send_Communication_Check_Command() {
long i,j;
Communication_Byte[0]='&';
UsbDriver.USB_Device_Connection.bulkTransfer(UsbDriver.Data_Out_End_Point,Communication_Byte, 1, 0);
for(i=0;(i<1000 && Communication_Ok!=true) ;i++)
for(j=0;(j<1000 && Communication_Ok!=true);j++);
}
});
}
}
This is my logcat:
E/AndroidRuntime(2452): FATAL EXCEPTION: main
E/AndroidRuntime(2452): java.lang.ArrayIndexOutOfBoundsException: length=1; index=1
E/AndroidRuntime(2452): at android.hardware.usb.UsbDevice.getInterface(UsbDevice.java:155)
E/AndroidRuntime(2452): at com.developer.milancomm.UsbDriver.Check_Devices(UsbDriver.java:74)
E/AndroidRuntime(2452): at com.developer.milancomm.UsbDriver.<init>(UsbDriver.java:48)
E/AndroidRuntime(2452): at com.developer.milancomm.MainActivity$1.Check_Devices_Available(MainActivity.java:86)
E/AndroidRuntime(2452): at com.developer.milancomm.MainActivity$1.onClick(MainActivity.java:63)
E/AndroidRuntime(2452): at android.view.View.performClick(View.java:4240)
E/AndroidRuntime(2452): at android.view.View$PerformClick.run(View.java:17721)
E/AndroidRuntime(2452): at android.os.Handler.handleCallback(Handler.java:730)
E/AndroidRuntime(2452): at android.os.Handler.dispatchMessage(Handler.java:92)
E/AndroidRuntime(2452): at android.os.Looper.loop(Looper.java:137)
E/AndroidRuntime(2452): at android.app.ActivityThread.main(ActivityThread.java:5103)
E/AndroidRuntime(2452): at java.lang.reflect.Method.invokeNative(Native Method)
E/AndroidRuntime(2452): at java.lang.reflect.Method.invoke(Method.java:525)
E/AndroidRuntime(2452): at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:737)
E/AndroidRuntime(2452): at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:553)
E/AndroidRuntime(2452): at dalvik.system.NativeStart.main(Native Method)

add a comment |
up vote
2
down vote
favorite
I am creating an android application that consists of a serial communication.I am getting an error called java.lang.ArrayIndexOutOfBoundsException: length=1; index=1 please tell me how to fix it
This is my usb driver:
public class UsbDriver {
private final Context mApplicationContext;
private final UsbManager mUsbManager;
@SuppressWarnings("unused")
private final UsbConnectionHandler mConnectionHandler;
private final int VID;
private final int PID;
protected static final String ACTION_USB_PERMISSION = "ch.serverbox.android.USB";
public static int Device_Exception;
public static UsbDevice Device_Details;
public static UsbEndpoint Data_In_End_Point = null;
public static UsbEndpoint Data_Out_End_Point = null;
public static UsbDeviceConnection USB_Device_Connection;
public UsbDriver(Activity parentActivity,UsbConnectionHandler connectionHandler, int vid, int pid) {
mApplicationContext = parentActivity.getApplicationContext();
mConnectionHandler = connectionHandler;
mUsbManager = (UsbManager) mApplicationContext.getSystemService(Context.USB_SERVICE);
VID = 1027;
PID = 24577;
Device_Exception = 0;
// init();
Check_Devices();
}
private void Check_Devices() {
@SuppressWarnings("unused")
int j=0;
HashMap<String, UsbDevice> devlist = mUsbManager.getDeviceList();
Iterator<UsbDevice> deviter = devlist.values().iterator();
Device_Details = null;
if (devlist.size() != 0) {
while (deviter.hasNext()) {
Device_Details = deviter.next();
if (Device_Details.getVendorId() == VID && Device_Details.getProductId() == PID){
if (!mUsbManager.hasPermission(Device_Details)){
onPermissionDenied(Device_Details);
} else {
UsbDeviceConnection conn = mUsbManager.openDevice(Device_Details);
if (!conn.claimInterface(Device_Details.getInterface(1), true)){
return;
}
conn.controlTransfer(0x21, 34, 0, 0, null, 0, 0);
conn.controlTransfer(0x21, 32, 0, 0,new byte { (byte) 0x80, 0x25 , 0x00, 0x00,0x00, 0x00, 0x08 }, 7, 0);
USB_Device_Connection=conn;
Data_In_End_Point = null;
Data_Out_End_Point = null;
UsbInterface usbIf = Device_Details.getInterface(1);
for (int i = 0; i < usbIf.getEndpointCount(); i++) {
if (usbIf.getEndpoint(i).getType() == UsbConstants.USB_ENDPOINT_XFER_BULK) {
if (usbIf.getEndpoint(i).getDirection() == UsbConstants.USB_DIR_IN)
Data_In_End_Point = usbIf.getEndpoint(i);
else
Data_Out_End_Point = usbIf.getEndpoint(i);
}
}
if (Data_In_End_Point == null || Data_Out_End_Point == null)
Device_Exception = 2;
}
break;
}j++;
}
if (Device_Details == null) {
Device_Exception = 3;
return;
}
}
else {
Device_Exception = 1;
return;
}
}
public void onPermissionDenied(UsbDevice d) {
UsbManager usbman = (UsbManager) mApplicationContext.getSystemService(Context.USB_SERVICE);
PendingIntent pi = PendingIntent.getBroadcast(mApplicationContext, 0, new Intent(ACTION_USB_PERMISSION), 0);
mApplicationContext.registerReceiver(mPermissionReceiver,new IntentFilter(ACTION_USB_PERMISSION));
usbman.requestPermission(d, pi);
}
private class PermissionReceiver extends BroadcastReceiver {
private final IPermissionListener mPermissionListener;
public PermissionReceiver(IPermissionListener permissionListener){
mPermissionListener = permissionListener;
}
@Override
public void onReceive(Context context, Intent intent) {
mApplicationContext.unregisterReceiver(this);
if (intent.getAction().equals(ACTION_USB_PERMISSION)) {
if (!intent.getBooleanExtra(UsbManager.EXTRA_PERMISSION_GRANTED, false)) {
mPermissionListener.onPermissionDenied((UsbDevice) intent.getParcelableExtra(UsbManager.EXTRA_DEVICE));
} else {
l("Permission granted");
UsbDevice dev = (UsbDevice) intent.getParcelableExtra(UsbManager.EXTRA_DEVICE);
if (dev != null){
if (dev.getVendorId() == VID && dev.getProductId() == PID) {
Check_Devices() ;
}
}else{
e("device not present!");
}
}
}
}
}
private BroadcastReceiver mPermissionReceiver = new PermissionReceiver(new IPermissionListener() {
@Override
public void onPermissionDenied(UsbDevice d) {
l("Permission denied on " + d.getDeviceId());
}
});
private static interface IPermissionListener {
void onPermissionDenied(UsbDevice d);
}
public final static String TAG = "USBController";
private void l(Object msg) {
Log.d(TAG, ">==<" + msg.toString() + " >==<");
}
private void e(Object msg) {
Log.e(TAG, ">==< " + msg.toString() + " >==<");
}
}
This is my usb connection handler
public interface UsbConnectionHandler {
void onUsbStopped();
void onErrorLooperRunningAlready();
void onDeviceNotFound();
}
This is my main activity:
public class MainActivity extends Activity {
EditText communication_data;
Button send;
public static final int targetVendorID = 1027;
public static final int targetProductID = 24577;
public UsbManager manager;
public UsbDeviceConnection usbDeviceConnection;
public UsbInterface usbInterfaceFound = null;
public UsbEndpoint endpointOut = null;
public UsbEndpoint endpointIn = null;
public UsbDevice usbdevice,device_details;
public UsbEndpoint listusbendpoint;
private static final int VID = 1027;
private static final int PID = 24577;
@SuppressWarnings("unused")
private static UsbDriver Usb_Driver_class;
ActionBar actionbar;
UsbConnectionHandler connectionHandler;
public static UsbDriver USB_Driver_Child;
public static boolean Communication_Failed,Frame_Ok,Total_Frame_Decoded;
static byte Communication_Byte;
HashMap<String, UsbDevice> devicelist= null;
static byte sample;
static boolean Communication_Ok;
static int Sequence_No,Response_Time;
Thread Receive;
ByteBuffer buffer;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//communication_data = (EditText)findViewById(R.id.editText_comm);
send = (Button)findViewById(R.id.button1_sendin);
send.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Communication_Byte=new byte[1];
if(Check_Devices_Available()==true) {
int Packet_Size = USB_Driver_Child.Data_In_End_Point.getMaxPacketSize();
Toast.makeText(MainActivity.this,""+Packet_Size, Toast.LENGTH_LONG).show();
Receive.start();
Communication_Ok=false;
for(int i=0;(i<5 && Communication_Ok!=true);i++)
Send_Communication_Check_Command();
if(Communication_Ok)
Toast.makeText(MainActivity.this, "Communication Successfully Established", 1000).show();
else
Toast.makeText(MainActivity.this, "Communication Failure", 10000).show();
}
}
private boolean Check_Devices_Available() {
Usb_Driver_class = new UsbDriver(MainActivity.this, connectionHandler, VID, PID);
if(USB_Driver_Child.Device_Exception==0){
if(USB_Driver_Child.USB_Device_Connection==null || USB_Driver_Child.Data_Out_End_Point==null)
return false;
Toast.makeText(MainActivity.this,"Device Found", 1000).show();
return true;
}else if(USB_Driver_Child.Device_Exception==1){
Toast.makeText(MainActivity.this,"No Devices Attached ", Toast.LENGTH_LONG).show();
return false;
}else if(USB_Driver_Child.Device_Exception==2){
Toast.makeText(MainActivity.this,"Device Found,But No End Points", Toast.LENGTH_LONG).show();
return false;
}else if(USB_Driver_Child.Device_Exception==3){
Toast.makeText(MainActivity.this,"Unable to Open Device", Toast.LENGTH_LONG).show();
return false;
}
return false;
}
Thread Receive = new Thread(new Runnable(){
@SuppressWarnings("unused")
@Override
public void run() {
Sequence_No=0;
buffer = ByteBuffer.allocate(64);
sample = new byte[64];
int Frame_Size;
UsbRequest request = new UsbRequest();
int i,j;
byte datarx=new byte[1];
char q;
while (true) {
request.initialize(UsbDriver.USB_Device_Connection, UsbDriver.Data_In_End_Point);
request.queue(buffer, 64);
if (UsbDriver.USB_Device_Connection.requestWait() == request) {
sample=buffer.array();
for(i=0;i<64;i++){
if(sample[i]=='&'){
Communication_Ok=true;
break;
}else if(sample[i]==0x03){
if(sample[0]==0x02)
//Frame_Ok=true;
break;
}
}
if(Frame_Ok==true){
//Frame_Ok=false;
//if(sample[1]==1)
//Coil_No=1;
//else
//Coil_No=2;
//Response_Time= (int)(((sample[2]&0x00FF)<<8) + (sample[3]&0x00FF));
//Total_Frame_Decoded=true;
//sample = null;
}
}
}
}
});
private void Send_Communication_Check_Command() {
long i,j;
Communication_Byte[0]='&';
UsbDriver.USB_Device_Connection.bulkTransfer(UsbDriver.Data_Out_End_Point,Communication_Byte, 1, 0);
for(i=0;(i<1000 && Communication_Ok!=true) ;i++)
for(j=0;(j<1000 && Communication_Ok!=true);j++);
}
});
}
}
This is my logcat:
E/AndroidRuntime(2452): FATAL EXCEPTION: main
E/AndroidRuntime(2452): java.lang.ArrayIndexOutOfBoundsException: length=1; index=1
E/AndroidRuntime(2452): at android.hardware.usb.UsbDevice.getInterface(UsbDevice.java:155)
E/AndroidRuntime(2452): at com.developer.milancomm.UsbDriver.Check_Devices(UsbDriver.java:74)
E/AndroidRuntime(2452): at com.developer.milancomm.UsbDriver.<init>(UsbDriver.java:48)
E/AndroidRuntime(2452): at com.developer.milancomm.MainActivity$1.Check_Devices_Available(MainActivity.java:86)
E/AndroidRuntime(2452): at com.developer.milancomm.MainActivity$1.onClick(MainActivity.java:63)
E/AndroidRuntime(2452): at android.view.View.performClick(View.java:4240)
E/AndroidRuntime(2452): at android.view.View$PerformClick.run(View.java:17721)
E/AndroidRuntime(2452): at android.os.Handler.handleCallback(Handler.java:730)
E/AndroidRuntime(2452): at android.os.Handler.dispatchMessage(Handler.java:92)
E/AndroidRuntime(2452): at android.os.Looper.loop(Looper.java:137)
E/AndroidRuntime(2452): at android.app.ActivityThread.main(ActivityThread.java:5103)
E/AndroidRuntime(2452): at java.lang.reflect.Method.invokeNative(Native Method)
E/AndroidRuntime(2452): at java.lang.reflect.Method.invoke(Method.java:525)
E/AndroidRuntime(2452): at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:737)
E/AndroidRuntime(2452): at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:553)
E/AndroidRuntime(2452): at dalvik.system.NativeStart.main(Native Method)

post logcat in which line it is getting
– No Name
Mar 30 '15 at 11:21
I posted my logcat dude check it @NoName
– RD Division Medequip
Mar 30 '15 at 11:22
Device_Details.getInterface(1)
is the problem. It looks like the underlaying data structure contains only one element,
– Blackbelt
Mar 30 '15 at 11:25
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I am creating an android application that consists of a serial communication.I am getting an error called java.lang.ArrayIndexOutOfBoundsException: length=1; index=1 please tell me how to fix it
This is my usb driver:
public class UsbDriver {
private final Context mApplicationContext;
private final UsbManager mUsbManager;
@SuppressWarnings("unused")
private final UsbConnectionHandler mConnectionHandler;
private final int VID;
private final int PID;
protected static final String ACTION_USB_PERMISSION = "ch.serverbox.android.USB";
public static int Device_Exception;
public static UsbDevice Device_Details;
public static UsbEndpoint Data_In_End_Point = null;
public static UsbEndpoint Data_Out_End_Point = null;
public static UsbDeviceConnection USB_Device_Connection;
public UsbDriver(Activity parentActivity,UsbConnectionHandler connectionHandler, int vid, int pid) {
mApplicationContext = parentActivity.getApplicationContext();
mConnectionHandler = connectionHandler;
mUsbManager = (UsbManager) mApplicationContext.getSystemService(Context.USB_SERVICE);
VID = 1027;
PID = 24577;
Device_Exception = 0;
// init();
Check_Devices();
}
private void Check_Devices() {
@SuppressWarnings("unused")
int j=0;
HashMap<String, UsbDevice> devlist = mUsbManager.getDeviceList();
Iterator<UsbDevice> deviter = devlist.values().iterator();
Device_Details = null;
if (devlist.size() != 0) {
while (deviter.hasNext()) {
Device_Details = deviter.next();
if (Device_Details.getVendorId() == VID && Device_Details.getProductId() == PID){
if (!mUsbManager.hasPermission(Device_Details)){
onPermissionDenied(Device_Details);
} else {
UsbDeviceConnection conn = mUsbManager.openDevice(Device_Details);
if (!conn.claimInterface(Device_Details.getInterface(1), true)){
return;
}
conn.controlTransfer(0x21, 34, 0, 0, null, 0, 0);
conn.controlTransfer(0x21, 32, 0, 0,new byte { (byte) 0x80, 0x25 , 0x00, 0x00,0x00, 0x00, 0x08 }, 7, 0);
USB_Device_Connection=conn;
Data_In_End_Point = null;
Data_Out_End_Point = null;
UsbInterface usbIf = Device_Details.getInterface(1);
for (int i = 0; i < usbIf.getEndpointCount(); i++) {
if (usbIf.getEndpoint(i).getType() == UsbConstants.USB_ENDPOINT_XFER_BULK) {
if (usbIf.getEndpoint(i).getDirection() == UsbConstants.USB_DIR_IN)
Data_In_End_Point = usbIf.getEndpoint(i);
else
Data_Out_End_Point = usbIf.getEndpoint(i);
}
}
if (Data_In_End_Point == null || Data_Out_End_Point == null)
Device_Exception = 2;
}
break;
}j++;
}
if (Device_Details == null) {
Device_Exception = 3;
return;
}
}
else {
Device_Exception = 1;
return;
}
}
public void onPermissionDenied(UsbDevice d) {
UsbManager usbman = (UsbManager) mApplicationContext.getSystemService(Context.USB_SERVICE);
PendingIntent pi = PendingIntent.getBroadcast(mApplicationContext, 0, new Intent(ACTION_USB_PERMISSION), 0);
mApplicationContext.registerReceiver(mPermissionReceiver,new IntentFilter(ACTION_USB_PERMISSION));
usbman.requestPermission(d, pi);
}
private class PermissionReceiver extends BroadcastReceiver {
private final IPermissionListener mPermissionListener;
public PermissionReceiver(IPermissionListener permissionListener){
mPermissionListener = permissionListener;
}
@Override
public void onReceive(Context context, Intent intent) {
mApplicationContext.unregisterReceiver(this);
if (intent.getAction().equals(ACTION_USB_PERMISSION)) {
if (!intent.getBooleanExtra(UsbManager.EXTRA_PERMISSION_GRANTED, false)) {
mPermissionListener.onPermissionDenied((UsbDevice) intent.getParcelableExtra(UsbManager.EXTRA_DEVICE));
} else {
l("Permission granted");
UsbDevice dev = (UsbDevice) intent.getParcelableExtra(UsbManager.EXTRA_DEVICE);
if (dev != null){
if (dev.getVendorId() == VID && dev.getProductId() == PID) {
Check_Devices() ;
}
}else{
e("device not present!");
}
}
}
}
}
private BroadcastReceiver mPermissionReceiver = new PermissionReceiver(new IPermissionListener() {
@Override
public void onPermissionDenied(UsbDevice d) {
l("Permission denied on " + d.getDeviceId());
}
});
private static interface IPermissionListener {
void onPermissionDenied(UsbDevice d);
}
public final static String TAG = "USBController";
private void l(Object msg) {
Log.d(TAG, ">==<" + msg.toString() + " >==<");
}
private void e(Object msg) {
Log.e(TAG, ">==< " + msg.toString() + " >==<");
}
}
This is my usb connection handler
public interface UsbConnectionHandler {
void onUsbStopped();
void onErrorLooperRunningAlready();
void onDeviceNotFound();
}
This is my main activity:
public class MainActivity extends Activity {
EditText communication_data;
Button send;
public static final int targetVendorID = 1027;
public static final int targetProductID = 24577;
public UsbManager manager;
public UsbDeviceConnection usbDeviceConnection;
public UsbInterface usbInterfaceFound = null;
public UsbEndpoint endpointOut = null;
public UsbEndpoint endpointIn = null;
public UsbDevice usbdevice,device_details;
public UsbEndpoint listusbendpoint;
private static final int VID = 1027;
private static final int PID = 24577;
@SuppressWarnings("unused")
private static UsbDriver Usb_Driver_class;
ActionBar actionbar;
UsbConnectionHandler connectionHandler;
public static UsbDriver USB_Driver_Child;
public static boolean Communication_Failed,Frame_Ok,Total_Frame_Decoded;
static byte Communication_Byte;
HashMap<String, UsbDevice> devicelist= null;
static byte sample;
static boolean Communication_Ok;
static int Sequence_No,Response_Time;
Thread Receive;
ByteBuffer buffer;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//communication_data = (EditText)findViewById(R.id.editText_comm);
send = (Button)findViewById(R.id.button1_sendin);
send.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Communication_Byte=new byte[1];
if(Check_Devices_Available()==true) {
int Packet_Size = USB_Driver_Child.Data_In_End_Point.getMaxPacketSize();
Toast.makeText(MainActivity.this,""+Packet_Size, Toast.LENGTH_LONG).show();
Receive.start();
Communication_Ok=false;
for(int i=0;(i<5 && Communication_Ok!=true);i++)
Send_Communication_Check_Command();
if(Communication_Ok)
Toast.makeText(MainActivity.this, "Communication Successfully Established", 1000).show();
else
Toast.makeText(MainActivity.this, "Communication Failure", 10000).show();
}
}
private boolean Check_Devices_Available() {
Usb_Driver_class = new UsbDriver(MainActivity.this, connectionHandler, VID, PID);
if(USB_Driver_Child.Device_Exception==0){
if(USB_Driver_Child.USB_Device_Connection==null || USB_Driver_Child.Data_Out_End_Point==null)
return false;
Toast.makeText(MainActivity.this,"Device Found", 1000).show();
return true;
}else if(USB_Driver_Child.Device_Exception==1){
Toast.makeText(MainActivity.this,"No Devices Attached ", Toast.LENGTH_LONG).show();
return false;
}else if(USB_Driver_Child.Device_Exception==2){
Toast.makeText(MainActivity.this,"Device Found,But No End Points", Toast.LENGTH_LONG).show();
return false;
}else if(USB_Driver_Child.Device_Exception==3){
Toast.makeText(MainActivity.this,"Unable to Open Device", Toast.LENGTH_LONG).show();
return false;
}
return false;
}
Thread Receive = new Thread(new Runnable(){
@SuppressWarnings("unused")
@Override
public void run() {
Sequence_No=0;
buffer = ByteBuffer.allocate(64);
sample = new byte[64];
int Frame_Size;
UsbRequest request = new UsbRequest();
int i,j;
byte datarx=new byte[1];
char q;
while (true) {
request.initialize(UsbDriver.USB_Device_Connection, UsbDriver.Data_In_End_Point);
request.queue(buffer, 64);
if (UsbDriver.USB_Device_Connection.requestWait() == request) {
sample=buffer.array();
for(i=0;i<64;i++){
if(sample[i]=='&'){
Communication_Ok=true;
break;
}else if(sample[i]==0x03){
if(sample[0]==0x02)
//Frame_Ok=true;
break;
}
}
if(Frame_Ok==true){
//Frame_Ok=false;
//if(sample[1]==1)
//Coil_No=1;
//else
//Coil_No=2;
//Response_Time= (int)(((sample[2]&0x00FF)<<8) + (sample[3]&0x00FF));
//Total_Frame_Decoded=true;
//sample = null;
}
}
}
}
});
private void Send_Communication_Check_Command() {
long i,j;
Communication_Byte[0]='&';
UsbDriver.USB_Device_Connection.bulkTransfer(UsbDriver.Data_Out_End_Point,Communication_Byte, 1, 0);
for(i=0;(i<1000 && Communication_Ok!=true) ;i++)
for(j=0;(j<1000 && Communication_Ok!=true);j++);
}
});
}
}
This is my logcat:
E/AndroidRuntime(2452): FATAL EXCEPTION: main
E/AndroidRuntime(2452): java.lang.ArrayIndexOutOfBoundsException: length=1; index=1
E/AndroidRuntime(2452): at android.hardware.usb.UsbDevice.getInterface(UsbDevice.java:155)
E/AndroidRuntime(2452): at com.developer.milancomm.UsbDriver.Check_Devices(UsbDriver.java:74)
E/AndroidRuntime(2452): at com.developer.milancomm.UsbDriver.<init>(UsbDriver.java:48)
E/AndroidRuntime(2452): at com.developer.milancomm.MainActivity$1.Check_Devices_Available(MainActivity.java:86)
E/AndroidRuntime(2452): at com.developer.milancomm.MainActivity$1.onClick(MainActivity.java:63)
E/AndroidRuntime(2452): at android.view.View.performClick(View.java:4240)
E/AndroidRuntime(2452): at android.view.View$PerformClick.run(View.java:17721)
E/AndroidRuntime(2452): at android.os.Handler.handleCallback(Handler.java:730)
E/AndroidRuntime(2452): at android.os.Handler.dispatchMessage(Handler.java:92)
E/AndroidRuntime(2452): at android.os.Looper.loop(Looper.java:137)
E/AndroidRuntime(2452): at android.app.ActivityThread.main(ActivityThread.java:5103)
E/AndroidRuntime(2452): at java.lang.reflect.Method.invokeNative(Native Method)
E/AndroidRuntime(2452): at java.lang.reflect.Method.invoke(Method.java:525)
E/AndroidRuntime(2452): at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:737)
E/AndroidRuntime(2452): at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:553)
E/AndroidRuntime(2452): at dalvik.system.NativeStart.main(Native Method)

I am creating an android application that consists of a serial communication.I am getting an error called java.lang.ArrayIndexOutOfBoundsException: length=1; index=1 please tell me how to fix it
This is my usb driver:
public class UsbDriver {
private final Context mApplicationContext;
private final UsbManager mUsbManager;
@SuppressWarnings("unused")
private final UsbConnectionHandler mConnectionHandler;
private final int VID;
private final int PID;
protected static final String ACTION_USB_PERMISSION = "ch.serverbox.android.USB";
public static int Device_Exception;
public static UsbDevice Device_Details;
public static UsbEndpoint Data_In_End_Point = null;
public static UsbEndpoint Data_Out_End_Point = null;
public static UsbDeviceConnection USB_Device_Connection;
public UsbDriver(Activity parentActivity,UsbConnectionHandler connectionHandler, int vid, int pid) {
mApplicationContext = parentActivity.getApplicationContext();
mConnectionHandler = connectionHandler;
mUsbManager = (UsbManager) mApplicationContext.getSystemService(Context.USB_SERVICE);
VID = 1027;
PID = 24577;
Device_Exception = 0;
// init();
Check_Devices();
}
private void Check_Devices() {
@SuppressWarnings("unused")
int j=0;
HashMap<String, UsbDevice> devlist = mUsbManager.getDeviceList();
Iterator<UsbDevice> deviter = devlist.values().iterator();
Device_Details = null;
if (devlist.size() != 0) {
while (deviter.hasNext()) {
Device_Details = deviter.next();
if (Device_Details.getVendorId() == VID && Device_Details.getProductId() == PID){
if (!mUsbManager.hasPermission(Device_Details)){
onPermissionDenied(Device_Details);
} else {
UsbDeviceConnection conn = mUsbManager.openDevice(Device_Details);
if (!conn.claimInterface(Device_Details.getInterface(1), true)){
return;
}
conn.controlTransfer(0x21, 34, 0, 0, null, 0, 0);
conn.controlTransfer(0x21, 32, 0, 0,new byte { (byte) 0x80, 0x25 , 0x00, 0x00,0x00, 0x00, 0x08 }, 7, 0);
USB_Device_Connection=conn;
Data_In_End_Point = null;
Data_Out_End_Point = null;
UsbInterface usbIf = Device_Details.getInterface(1);
for (int i = 0; i < usbIf.getEndpointCount(); i++) {
if (usbIf.getEndpoint(i).getType() == UsbConstants.USB_ENDPOINT_XFER_BULK) {
if (usbIf.getEndpoint(i).getDirection() == UsbConstants.USB_DIR_IN)
Data_In_End_Point = usbIf.getEndpoint(i);
else
Data_Out_End_Point = usbIf.getEndpoint(i);
}
}
if (Data_In_End_Point == null || Data_Out_End_Point == null)
Device_Exception = 2;
}
break;
}j++;
}
if (Device_Details == null) {
Device_Exception = 3;
return;
}
}
else {
Device_Exception = 1;
return;
}
}
public void onPermissionDenied(UsbDevice d) {
UsbManager usbman = (UsbManager) mApplicationContext.getSystemService(Context.USB_SERVICE);
PendingIntent pi = PendingIntent.getBroadcast(mApplicationContext, 0, new Intent(ACTION_USB_PERMISSION), 0);
mApplicationContext.registerReceiver(mPermissionReceiver,new IntentFilter(ACTION_USB_PERMISSION));
usbman.requestPermission(d, pi);
}
private class PermissionReceiver extends BroadcastReceiver {
private final IPermissionListener mPermissionListener;
public PermissionReceiver(IPermissionListener permissionListener){
mPermissionListener = permissionListener;
}
@Override
public void onReceive(Context context, Intent intent) {
mApplicationContext.unregisterReceiver(this);
if (intent.getAction().equals(ACTION_USB_PERMISSION)) {
if (!intent.getBooleanExtra(UsbManager.EXTRA_PERMISSION_GRANTED, false)) {
mPermissionListener.onPermissionDenied((UsbDevice) intent.getParcelableExtra(UsbManager.EXTRA_DEVICE));
} else {
l("Permission granted");
UsbDevice dev = (UsbDevice) intent.getParcelableExtra(UsbManager.EXTRA_DEVICE);
if (dev != null){
if (dev.getVendorId() == VID && dev.getProductId() == PID) {
Check_Devices() ;
}
}else{
e("device not present!");
}
}
}
}
}
private BroadcastReceiver mPermissionReceiver = new PermissionReceiver(new IPermissionListener() {
@Override
public void onPermissionDenied(UsbDevice d) {
l("Permission denied on " + d.getDeviceId());
}
});
private static interface IPermissionListener {
void onPermissionDenied(UsbDevice d);
}
public final static String TAG = "USBController";
private void l(Object msg) {
Log.d(TAG, ">==<" + msg.toString() + " >==<");
}
private void e(Object msg) {
Log.e(TAG, ">==< " + msg.toString() + " >==<");
}
}
This is my usb connection handler
public interface UsbConnectionHandler {
void onUsbStopped();
void onErrorLooperRunningAlready();
void onDeviceNotFound();
}
This is my main activity:
public class MainActivity extends Activity {
EditText communication_data;
Button send;
public static final int targetVendorID = 1027;
public static final int targetProductID = 24577;
public UsbManager manager;
public UsbDeviceConnection usbDeviceConnection;
public UsbInterface usbInterfaceFound = null;
public UsbEndpoint endpointOut = null;
public UsbEndpoint endpointIn = null;
public UsbDevice usbdevice,device_details;
public UsbEndpoint listusbendpoint;
private static final int VID = 1027;
private static final int PID = 24577;
@SuppressWarnings("unused")
private static UsbDriver Usb_Driver_class;
ActionBar actionbar;
UsbConnectionHandler connectionHandler;
public static UsbDriver USB_Driver_Child;
public static boolean Communication_Failed,Frame_Ok,Total_Frame_Decoded;
static byte Communication_Byte;
HashMap<String, UsbDevice> devicelist= null;
static byte sample;
static boolean Communication_Ok;
static int Sequence_No,Response_Time;
Thread Receive;
ByteBuffer buffer;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//communication_data = (EditText)findViewById(R.id.editText_comm);
send = (Button)findViewById(R.id.button1_sendin);
send.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Communication_Byte=new byte[1];
if(Check_Devices_Available()==true) {
int Packet_Size = USB_Driver_Child.Data_In_End_Point.getMaxPacketSize();
Toast.makeText(MainActivity.this,""+Packet_Size, Toast.LENGTH_LONG).show();
Receive.start();
Communication_Ok=false;
for(int i=0;(i<5 && Communication_Ok!=true);i++)
Send_Communication_Check_Command();
if(Communication_Ok)
Toast.makeText(MainActivity.this, "Communication Successfully Established", 1000).show();
else
Toast.makeText(MainActivity.this, "Communication Failure", 10000).show();
}
}
private boolean Check_Devices_Available() {
Usb_Driver_class = new UsbDriver(MainActivity.this, connectionHandler, VID, PID);
if(USB_Driver_Child.Device_Exception==0){
if(USB_Driver_Child.USB_Device_Connection==null || USB_Driver_Child.Data_Out_End_Point==null)
return false;
Toast.makeText(MainActivity.this,"Device Found", 1000).show();
return true;
}else if(USB_Driver_Child.Device_Exception==1){
Toast.makeText(MainActivity.this,"No Devices Attached ", Toast.LENGTH_LONG).show();
return false;
}else if(USB_Driver_Child.Device_Exception==2){
Toast.makeText(MainActivity.this,"Device Found,But No End Points", Toast.LENGTH_LONG).show();
return false;
}else if(USB_Driver_Child.Device_Exception==3){
Toast.makeText(MainActivity.this,"Unable to Open Device", Toast.LENGTH_LONG).show();
return false;
}
return false;
}
Thread Receive = new Thread(new Runnable(){
@SuppressWarnings("unused")
@Override
public void run() {
Sequence_No=0;
buffer = ByteBuffer.allocate(64);
sample = new byte[64];
int Frame_Size;
UsbRequest request = new UsbRequest();
int i,j;
byte datarx=new byte[1];
char q;
while (true) {
request.initialize(UsbDriver.USB_Device_Connection, UsbDriver.Data_In_End_Point);
request.queue(buffer, 64);
if (UsbDriver.USB_Device_Connection.requestWait() == request) {
sample=buffer.array();
for(i=0;i<64;i++){
if(sample[i]=='&'){
Communication_Ok=true;
break;
}else if(sample[i]==0x03){
if(sample[0]==0x02)
//Frame_Ok=true;
break;
}
}
if(Frame_Ok==true){
//Frame_Ok=false;
//if(sample[1]==1)
//Coil_No=1;
//else
//Coil_No=2;
//Response_Time= (int)(((sample[2]&0x00FF)<<8) + (sample[3]&0x00FF));
//Total_Frame_Decoded=true;
//sample = null;
}
}
}
}
});
private void Send_Communication_Check_Command() {
long i,j;
Communication_Byte[0]='&';
UsbDriver.USB_Device_Connection.bulkTransfer(UsbDriver.Data_Out_End_Point,Communication_Byte, 1, 0);
for(i=0;(i<1000 && Communication_Ok!=true) ;i++)
for(j=0;(j<1000 && Communication_Ok!=true);j++);
}
});
}
}
This is my logcat:
E/AndroidRuntime(2452): FATAL EXCEPTION: main
E/AndroidRuntime(2452): java.lang.ArrayIndexOutOfBoundsException: length=1; index=1
E/AndroidRuntime(2452): at android.hardware.usb.UsbDevice.getInterface(UsbDevice.java:155)
E/AndroidRuntime(2452): at com.developer.milancomm.UsbDriver.Check_Devices(UsbDriver.java:74)
E/AndroidRuntime(2452): at com.developer.milancomm.UsbDriver.<init>(UsbDriver.java:48)
E/AndroidRuntime(2452): at com.developer.milancomm.MainActivity$1.Check_Devices_Available(MainActivity.java:86)
E/AndroidRuntime(2452): at com.developer.milancomm.MainActivity$1.onClick(MainActivity.java:63)
E/AndroidRuntime(2452): at android.view.View.performClick(View.java:4240)
E/AndroidRuntime(2452): at android.view.View$PerformClick.run(View.java:17721)
E/AndroidRuntime(2452): at android.os.Handler.handleCallback(Handler.java:730)
E/AndroidRuntime(2452): at android.os.Handler.dispatchMessage(Handler.java:92)
E/AndroidRuntime(2452): at android.os.Looper.loop(Looper.java:137)
E/AndroidRuntime(2452): at android.app.ActivityThread.main(ActivityThread.java:5103)
E/AndroidRuntime(2452): at java.lang.reflect.Method.invokeNative(Native Method)
E/AndroidRuntime(2452): at java.lang.reflect.Method.invoke(Method.java:525)
E/AndroidRuntime(2452): at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:737)
E/AndroidRuntime(2452): at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:553)
E/AndroidRuntime(2452): at dalvik.system.NativeStart.main(Native Method)


edited Jul 14 '16 at 2:58


W0rmH0le
9,62253549
9,62253549
asked Mar 30 '15 at 11:20
RD Division Medequip
62741127
62741127
post logcat in which line it is getting
– No Name
Mar 30 '15 at 11:21
I posted my logcat dude check it @NoName
– RD Division Medequip
Mar 30 '15 at 11:22
Device_Details.getInterface(1)
is the problem. It looks like the underlaying data structure contains only one element,
– Blackbelt
Mar 30 '15 at 11:25
add a comment |
post logcat in which line it is getting
– No Name
Mar 30 '15 at 11:21
I posted my logcat dude check it @NoName
– RD Division Medequip
Mar 30 '15 at 11:22
Device_Details.getInterface(1)
is the problem. It looks like the underlaying data structure contains only one element,
– Blackbelt
Mar 30 '15 at 11:25
post logcat in which line it is getting
– No Name
Mar 30 '15 at 11:21
post logcat in which line it is getting
– No Name
Mar 30 '15 at 11:21
I posted my logcat dude check it @NoName
– RD Division Medequip
Mar 30 '15 at 11:22
I posted my logcat dude check it @NoName
– RD Division Medequip
Mar 30 '15 at 11:22
Device_Details.getInterface(1)
is the problem. It looks like the underlaying data structure contains only one element,– Blackbelt
Mar 30 '15 at 11:25
Device_Details.getInterface(1)
is the problem. It looks like the underlaying data structure contains only one element,– Blackbelt
Mar 30 '15 at 11:25
add a comment |
3 Answers
3
active
oldest
votes
up vote
8
down vote
accepted
An Array length of 1 means that only the index 0 exist, because in java array indexes start with 0!
Or in other words the Boundaries of an Array are Array.length -1
What is reason behide Array.get(-1) isn't working directly .... -1 means last index ... in that sense??
– Bhuro
Jul 25 '16 at 14:22
1
No not in Java ;)
– Rene M.
Jul 25 '16 at 14:38
add a comment |
up vote
3
down vote
length = 1 means that the array has only 1 element in it, and java is zero based numbering (which means that indexing an array starts from 0 not 1).
since you have only one element in the array, this means that you need to access it with index = 0 , not index = 1
this is what the error trying to tell you.
add a comment |
up vote
1
down vote
The Logcat indicates you are having error at line 74 in UsbDriver Class at:
if (!conn.claimInterface(Device_Details.getInterface(1), true)) { ...}
try replacing with 0 in the place of 1.
I don't know why you hardcoded this with 1.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f29344871%2fhow-to-fix-java-lang-arrayindexoutofboundsexception-length-1-index-1%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
8
down vote
accepted
An Array length of 1 means that only the index 0 exist, because in java array indexes start with 0!
Or in other words the Boundaries of an Array are Array.length -1
What is reason behide Array.get(-1) isn't working directly .... -1 means last index ... in that sense??
– Bhuro
Jul 25 '16 at 14:22
1
No not in Java ;)
– Rene M.
Jul 25 '16 at 14:38
add a comment |
up vote
8
down vote
accepted
An Array length of 1 means that only the index 0 exist, because in java array indexes start with 0!
Or in other words the Boundaries of an Array are Array.length -1
What is reason behide Array.get(-1) isn't working directly .... -1 means last index ... in that sense??
– Bhuro
Jul 25 '16 at 14:22
1
No not in Java ;)
– Rene M.
Jul 25 '16 at 14:38
add a comment |
up vote
8
down vote
accepted
up vote
8
down vote
accepted
An Array length of 1 means that only the index 0 exist, because in java array indexes start with 0!
Or in other words the Boundaries of an Array are Array.length -1
An Array length of 1 means that only the index 0 exist, because in java array indexes start with 0!
Or in other words the Boundaries of an Array are Array.length -1
edited Mar 30 '15 at 11:42
answered Mar 30 '15 at 11:26


Rene M.
2,248721
2,248721
What is reason behide Array.get(-1) isn't working directly .... -1 means last index ... in that sense??
– Bhuro
Jul 25 '16 at 14:22
1
No not in Java ;)
– Rene M.
Jul 25 '16 at 14:38
add a comment |
What is reason behide Array.get(-1) isn't working directly .... -1 means last index ... in that sense??
– Bhuro
Jul 25 '16 at 14:22
1
No not in Java ;)
– Rene M.
Jul 25 '16 at 14:38
What is reason behide Array.get(-1) isn't working directly .... -1 means last index ... in that sense??
– Bhuro
Jul 25 '16 at 14:22
What is reason behide Array.get(-1) isn't working directly .... -1 means last index ... in that sense??
– Bhuro
Jul 25 '16 at 14:22
1
1
No not in Java ;)
– Rene M.
Jul 25 '16 at 14:38
No not in Java ;)
– Rene M.
Jul 25 '16 at 14:38
add a comment |
up vote
3
down vote
length = 1 means that the array has only 1 element in it, and java is zero based numbering (which means that indexing an array starts from 0 not 1).
since you have only one element in the array, this means that you need to access it with index = 0 , not index = 1
this is what the error trying to tell you.
add a comment |
up vote
3
down vote
length = 1 means that the array has only 1 element in it, and java is zero based numbering (which means that indexing an array starts from 0 not 1).
since you have only one element in the array, this means that you need to access it with index = 0 , not index = 1
this is what the error trying to tell you.
add a comment |
up vote
3
down vote
up vote
3
down vote
length = 1 means that the array has only 1 element in it, and java is zero based numbering (which means that indexing an array starts from 0 not 1).
since you have only one element in the array, this means that you need to access it with index = 0 , not index = 1
this is what the error trying to tell you.
length = 1 means that the array has only 1 element in it, and java is zero based numbering (which means that indexing an array starts from 0 not 1).
since you have only one element in the array, this means that you need to access it with index = 0 , not index = 1
this is what the error trying to tell you.
edited Nov 12 at 6:43


Alireza Noorali
1,259531
1,259531
answered Mar 30 '15 at 11:45


Moataz Shawky
393
393
add a comment |
add a comment |
up vote
1
down vote
The Logcat indicates you are having error at line 74 in UsbDriver Class at:
if (!conn.claimInterface(Device_Details.getInterface(1), true)) { ...}
try replacing with 0 in the place of 1.
I don't know why you hardcoded this with 1.
add a comment |
up vote
1
down vote
The Logcat indicates you are having error at line 74 in UsbDriver Class at:
if (!conn.claimInterface(Device_Details.getInterface(1), true)) { ...}
try replacing with 0 in the place of 1.
I don't know why you hardcoded this with 1.
add a comment |
up vote
1
down vote
up vote
1
down vote
The Logcat indicates you are having error at line 74 in UsbDriver Class at:
if (!conn.claimInterface(Device_Details.getInterface(1), true)) { ...}
try replacing with 0 in the place of 1.
I don't know why you hardcoded this with 1.
The Logcat indicates you are having error at line 74 in UsbDriver Class at:
if (!conn.claimInterface(Device_Details.getInterface(1), true)) { ...}
try replacing with 0 in the place of 1.
I don't know why you hardcoded this with 1.
edited Nov 11 at 17:23


Alireza Noorali
1,259531
1,259531
answered Mar 30 '15 at 11:48
syed99
147218
147218
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f29344871%2fhow-to-fix-java-lang-arrayindexoutofboundsexception-length-1-index-1%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
R 8HAhXxSRE41N6 Bf,axAUslP5FrjxE,Gp0s,9eCs
post logcat in which line it is getting
– No Name
Mar 30 '15 at 11:21
I posted my logcat dude check it @NoName
– RD Division Medequip
Mar 30 '15 at 11:22
Device_Details.getInterface(1)
is the problem. It looks like the underlaying data structure contains only one element,– Blackbelt
Mar 30 '15 at 11:25