React why is this simple array map function not returning anything?
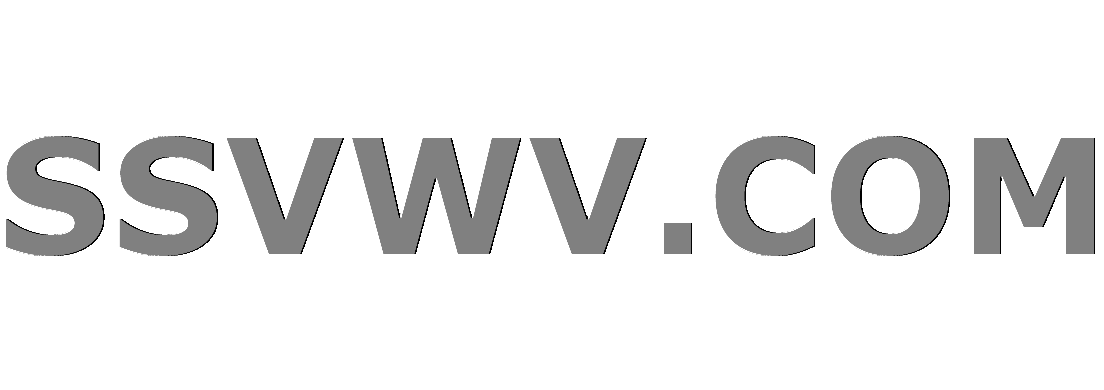
Multi tool use
I have a React component creating a bulleted list for items in an array (called activePath). The array is reporting correctly via console.log, and here is the format:
{device_id: "nlab5320a", port_id: "XGigabitEthernet0/0/3"}
There are six of these in the array. I did an Array.isArray just to confirm that this is a true array and it is. I am trying to map this array and output a bulleted list with the following:
activePath.map((item, i) => (
<li key={i}>{item.device_id}</li>
));
Here is the entire component ...
export default class ServicesInfo extends React.Component {
render() {
const { activePath } = this.props;
const runTable = activePath.map((item, i) => (
<li key={i}>{item.device_id}</li>
));
return (
<div>
<ul>{runTable}</ul>
</div>
);
}
}
The ServicesInfo is the child component. Here is the parent ...
export default class InfoHolder extends React.Component {
constructor(props) {
super(props) {
}
render() {
return(
<div>
<p>Here is a listing of the nodes:</p>
<ServicesInfo />
</div>
)
}
A single bullet with no text is returning. I have been at this project for about 16 hours straight and think I am just missing something simple. Please help!
arrays reactjs
|
show 3 more comments
I have a React component creating a bulleted list for items in an array (called activePath). The array is reporting correctly via console.log, and here is the format:
{device_id: "nlab5320a", port_id: "XGigabitEthernet0/0/3"}
There are six of these in the array. I did an Array.isArray just to confirm that this is a true array and it is. I am trying to map this array and output a bulleted list with the following:
activePath.map((item, i) => (
<li key={i}>{item.device_id}</li>
));
Here is the entire component ...
export default class ServicesInfo extends React.Component {
render() {
const { activePath } = this.props;
const runTable = activePath.map((item, i) => (
<li key={i}>{item.device_id}</li>
));
return (
<div>
<ul>{runTable}</ul>
</div>
);
}
}
The ServicesInfo is the child component. Here is the parent ...
export default class InfoHolder extends React.Component {
constructor(props) {
super(props) {
}
render() {
return(
<div>
<p>Here is a listing of the nodes:</p>
<ServicesInfo />
</div>
)
}
A single bullet with no text is returning. I have been at this project for about 16 hours straight and think I am just missing something simple. Please help!
arrays reactjs
this code you have written inside render function or inside a separate function component.
– Mohammed Ashfaq
Nov 12 at 12:17
1
Could you please include your entire component? The code currently in your question isn't enough to say what might be wrong.
– Tholle
Nov 12 at 12:19
I am running it in the render function with const runTable = activePath.map((item,i) => <li key={i}>{item.device_id}</li> ) and calling it in the return with {runTable}
– CHays412
Nov 12 at 12:21
That's not enough either, sadly. Please include a Minimal, Complete, and Verifiable example or it will be difficult for anyone to help you.
– Tholle
Nov 12 at 12:22
1
You have not passed anyprops
toServicesInfo
in you code snippet above. Thereforethis.props.activePath
is alwaysundefined
inside yourServicesInfo
component.
– dschu
Nov 12 at 12:52
|
show 3 more comments
I have a React component creating a bulleted list for items in an array (called activePath). The array is reporting correctly via console.log, and here is the format:
{device_id: "nlab5320a", port_id: "XGigabitEthernet0/0/3"}
There are six of these in the array. I did an Array.isArray just to confirm that this is a true array and it is. I am trying to map this array and output a bulleted list with the following:
activePath.map((item, i) => (
<li key={i}>{item.device_id}</li>
));
Here is the entire component ...
export default class ServicesInfo extends React.Component {
render() {
const { activePath } = this.props;
const runTable = activePath.map((item, i) => (
<li key={i}>{item.device_id}</li>
));
return (
<div>
<ul>{runTable}</ul>
</div>
);
}
}
The ServicesInfo is the child component. Here is the parent ...
export default class InfoHolder extends React.Component {
constructor(props) {
super(props) {
}
render() {
return(
<div>
<p>Here is a listing of the nodes:</p>
<ServicesInfo />
</div>
)
}
A single bullet with no text is returning. I have been at this project for about 16 hours straight and think I am just missing something simple. Please help!
arrays reactjs
I have a React component creating a bulleted list for items in an array (called activePath). The array is reporting correctly via console.log, and here is the format:
{device_id: "nlab5320a", port_id: "XGigabitEthernet0/0/3"}
There are six of these in the array. I did an Array.isArray just to confirm that this is a true array and it is. I am trying to map this array and output a bulleted list with the following:
activePath.map((item, i) => (
<li key={i}>{item.device_id}</li>
));
Here is the entire component ...
export default class ServicesInfo extends React.Component {
render() {
const { activePath } = this.props;
const runTable = activePath.map((item, i) => (
<li key={i}>{item.device_id}</li>
));
return (
<div>
<ul>{runTable}</ul>
</div>
);
}
}
The ServicesInfo is the child component. Here is the parent ...
export default class InfoHolder extends React.Component {
constructor(props) {
super(props) {
}
render() {
return(
<div>
<p>Here is a listing of the nodes:</p>
<ServicesInfo />
</div>
)
}
A single bullet with no text is returning. I have been at this project for about 16 hours straight and think I am just missing something simple. Please help!
arrays reactjs
arrays reactjs
edited Nov 12 at 12:43
asked Nov 12 at 12:14
CHays412
526
526
this code you have written inside render function or inside a separate function component.
– Mohammed Ashfaq
Nov 12 at 12:17
1
Could you please include your entire component? The code currently in your question isn't enough to say what might be wrong.
– Tholle
Nov 12 at 12:19
I am running it in the render function with const runTable = activePath.map((item,i) => <li key={i}>{item.device_id}</li> ) and calling it in the return with {runTable}
– CHays412
Nov 12 at 12:21
That's not enough either, sadly. Please include a Minimal, Complete, and Verifiable example or it will be difficult for anyone to help you.
– Tholle
Nov 12 at 12:22
1
You have not passed anyprops
toServicesInfo
in you code snippet above. Thereforethis.props.activePath
is alwaysundefined
inside yourServicesInfo
component.
– dschu
Nov 12 at 12:52
|
show 3 more comments
this code you have written inside render function or inside a separate function component.
– Mohammed Ashfaq
Nov 12 at 12:17
1
Could you please include your entire component? The code currently in your question isn't enough to say what might be wrong.
– Tholle
Nov 12 at 12:19
I am running it in the render function with const runTable = activePath.map((item,i) => <li key={i}>{item.device_id}</li> ) and calling it in the return with {runTable}
– CHays412
Nov 12 at 12:21
That's not enough either, sadly. Please include a Minimal, Complete, and Verifiable example or it will be difficult for anyone to help you.
– Tholle
Nov 12 at 12:22
1
You have not passed anyprops
toServicesInfo
in you code snippet above. Thereforethis.props.activePath
is alwaysundefined
inside yourServicesInfo
component.
– dschu
Nov 12 at 12:52
this code you have written inside render function or inside a separate function component.
– Mohammed Ashfaq
Nov 12 at 12:17
this code you have written inside render function or inside a separate function component.
– Mohammed Ashfaq
Nov 12 at 12:17
1
1
Could you please include your entire component? The code currently in your question isn't enough to say what might be wrong.
– Tholle
Nov 12 at 12:19
Could you please include your entire component? The code currently in your question isn't enough to say what might be wrong.
– Tholle
Nov 12 at 12:19
I am running it in the render function with const runTable = activePath.map((item,i) => <li key={i}>{item.device_id}</li> ) and calling it in the return with {runTable}
– CHays412
Nov 12 at 12:21
I am running it in the render function with const runTable = activePath.map((item,i) => <li key={i}>{item.device_id}</li> ) and calling it in the return with {runTable}
– CHays412
Nov 12 at 12:21
That's not enough either, sadly. Please include a Minimal, Complete, and Verifiable example or it will be difficult for anyone to help you.
– Tholle
Nov 12 at 12:22
That's not enough either, sadly. Please include a Minimal, Complete, and Verifiable example or it will be difficult for anyone to help you.
– Tholle
Nov 12 at 12:22
1
1
You have not passed any
props
to ServicesInfo
in you code snippet above. Therefore this.props.activePath
is always undefined
inside your ServicesInfo
component.– dschu
Nov 12 at 12:52
You have not passed any
props
to ServicesInfo
in you code snippet above. Therefore this.props.activePath
is always undefined
inside your ServicesInfo
component.– dschu
Nov 12 at 12:52
|
show 3 more comments
2 Answers
2
active
oldest
votes
In the InfoHolder
you haven't passed activePath
as a prop to the ServicesInfo
component. You have to pass the activePath
like this: <ServicesInfo activePath={activePath} />
Codesandbox
add a comment |
Pass your array in the component.
Also add constructor(props){
to get props from other components.
super(props) {}
export default class InfoHolder extends React.Component {
constructor(props) {
super(props) {
this.state={
objectArray =[{device_id: "nlab5320a", port_id: "XGigabitEthernet0/0/3"}]
}
}
render() {
return(
<div>
<p>Here is a listing of the nodes:</p>
<ServicesInfo activePath={this.state.object} />
</div>
)
}
export default class ServicesInfo extends React.Component {
constructor(props) {
super(props) {
}
render() {
const { activePath } = this.props;
const runTable = activePath.map((item, i) => (
<li key={i}>{item.device_id}</li>
));
return (
<div>
<ul>{runTable}</ul>
</div>
);
}
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53261988%2freact-why-is-this-simple-array-map-function-not-returning-anything%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
In the InfoHolder
you haven't passed activePath
as a prop to the ServicesInfo
component. You have to pass the activePath
like this: <ServicesInfo activePath={activePath} />
Codesandbox
add a comment |
In the InfoHolder
you haven't passed activePath
as a prop to the ServicesInfo
component. You have to pass the activePath
like this: <ServicesInfo activePath={activePath} />
Codesandbox
add a comment |
In the InfoHolder
you haven't passed activePath
as a prop to the ServicesInfo
component. You have to pass the activePath
like this: <ServicesInfo activePath={activePath} />
Codesandbox
In the InfoHolder
you haven't passed activePath
as a prop to the ServicesInfo
component. You have to pass the activePath
like this: <ServicesInfo activePath={activePath} />
Codesandbox
answered Nov 12 at 12:49


im_tsm
711321
711321
add a comment |
add a comment |
Pass your array in the component.
Also add constructor(props){
to get props from other components.
super(props) {}
export default class InfoHolder extends React.Component {
constructor(props) {
super(props) {
this.state={
objectArray =[{device_id: "nlab5320a", port_id: "XGigabitEthernet0/0/3"}]
}
}
render() {
return(
<div>
<p>Here is a listing of the nodes:</p>
<ServicesInfo activePath={this.state.object} />
</div>
)
}
export default class ServicesInfo extends React.Component {
constructor(props) {
super(props) {
}
render() {
const { activePath } = this.props;
const runTable = activePath.map((item, i) => (
<li key={i}>{item.device_id}</li>
));
return (
<div>
<ul>{runTable}</ul>
</div>
);
}
}
add a comment |
Pass your array in the component.
Also add constructor(props){
to get props from other components.
super(props) {}
export default class InfoHolder extends React.Component {
constructor(props) {
super(props) {
this.state={
objectArray =[{device_id: "nlab5320a", port_id: "XGigabitEthernet0/0/3"}]
}
}
render() {
return(
<div>
<p>Here is a listing of the nodes:</p>
<ServicesInfo activePath={this.state.object} />
</div>
)
}
export default class ServicesInfo extends React.Component {
constructor(props) {
super(props) {
}
render() {
const { activePath } = this.props;
const runTable = activePath.map((item, i) => (
<li key={i}>{item.device_id}</li>
));
return (
<div>
<ul>{runTable}</ul>
</div>
);
}
}
add a comment |
Pass your array in the component.
Also add constructor(props){
to get props from other components.
super(props) {}
export default class InfoHolder extends React.Component {
constructor(props) {
super(props) {
this.state={
objectArray =[{device_id: "nlab5320a", port_id: "XGigabitEthernet0/0/3"}]
}
}
render() {
return(
<div>
<p>Here is a listing of the nodes:</p>
<ServicesInfo activePath={this.state.object} />
</div>
)
}
export default class ServicesInfo extends React.Component {
constructor(props) {
super(props) {
}
render() {
const { activePath } = this.props;
const runTable = activePath.map((item, i) => (
<li key={i}>{item.device_id}</li>
));
return (
<div>
<ul>{runTable}</ul>
</div>
);
}
}
Pass your array in the component.
Also add constructor(props){
to get props from other components.
super(props) {}
export default class InfoHolder extends React.Component {
constructor(props) {
super(props) {
this.state={
objectArray =[{device_id: "nlab5320a", port_id: "XGigabitEthernet0/0/3"}]
}
}
render() {
return(
<div>
<p>Here is a listing of the nodes:</p>
<ServicesInfo activePath={this.state.object} />
</div>
)
}
export default class ServicesInfo extends React.Component {
constructor(props) {
super(props) {
}
render() {
const { activePath } = this.props;
const runTable = activePath.map((item, i) => (
<li key={i}>{item.device_id}</li>
));
return (
<div>
<ul>{runTable}</ul>
</div>
);
}
}
answered Nov 13 at 10:48


Yahya Ahmed
1639
1639
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53261988%2freact-why-is-this-simple-array-map-function-not-returning-anything%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
DYiQ PiTiL0wRaN,FOmbVxRlt7U9Q1nTHqlQVw 5rDYZY,9h1yiIV,FPb cO O,Ea2kE dCCH5CkXmSzEJv59qiHavW gLaE2RtnCDXx
this code you have written inside render function or inside a separate function component.
– Mohammed Ashfaq
Nov 12 at 12:17
1
Could you please include your entire component? The code currently in your question isn't enough to say what might be wrong.
– Tholle
Nov 12 at 12:19
I am running it in the render function with const runTable = activePath.map((item,i) => <li key={i}>{item.device_id}</li> ) and calling it in the return with {runTable}
– CHays412
Nov 12 at 12:21
That's not enough either, sadly. Please include a Minimal, Complete, and Verifiable example or it will be difficult for anyone to help you.
– Tholle
Nov 12 at 12:22
1
You have not passed any
props
toServicesInfo
in you code snippet above. Thereforethis.props.activePath
is alwaysundefined
inside yourServicesInfo
component.– dschu
Nov 12 at 12:52