Node.js code works locally but does not work on AWS Lambda
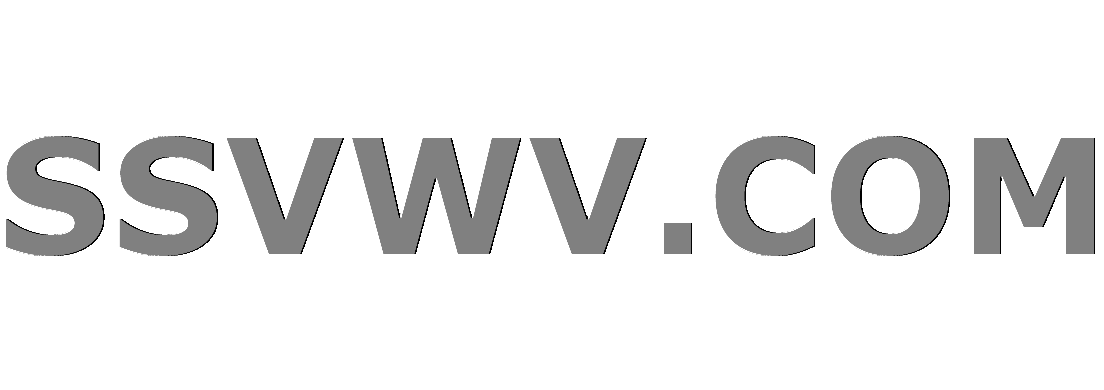
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I have a node.js function for AWS Lambda. It reads a JSON file from an S3 bucket as a stream, parses it and prints the parsed objects to the console. I am using stream-json module for parsing.
It works on my local environment and prints the objects to console. But it does not print the objects to the log streams(CloudWatch) on Lambda. It simply times out after the max duration. It prints other log statements around, but not the object values.
1. Using node.js 6.10 in both environments.
2. callback to the Lambda function is invoked only after the stream ends.
3. Lambda has full access to S3
4. Also tried Promise to wait until streams complete. But no change.
What am I missing? Thank you in advance.
const AWS = require('aws-sdk');
const {parser} = require('stream-json');
const {streamArray} = require('stream-json/streamers/StreamArray');
const {chain} = require('stream-chain');
const S3 = new AWS.S3({ apiVersion: '2006-03-01' });
/** ******************** Lambda Handler *************************** */
exports.handler = (event, context, callback) => {
// Get the object from the event and show its content type
const bucket = event.Records[0].s3.bucket.name;
const key = event.Records[0].s3.object.key;
const params = {
Bucket: bucket,
Key: key
};
console.log("Source: " + bucket +"//" + key);
let s3ReaderStream = S3.getObject(params).createReadStream();
console.log("Setting up pipes");
const pipeline = chain([
s3ReaderStream,
parser(),
streamArray(),
data => {
console.log(data.value);
}
]);
pipeline.on('data', (data) => console.log(data));
pipeline.on('end', () => callback(null, "Stream ended"));
};
node.js aws-lambda node.js-stream
add a comment |
I have a node.js function for AWS Lambda. It reads a JSON file from an S3 bucket as a stream, parses it and prints the parsed objects to the console. I am using stream-json module for parsing.
It works on my local environment and prints the objects to console. But it does not print the objects to the log streams(CloudWatch) on Lambda. It simply times out after the max duration. It prints other log statements around, but not the object values.
1. Using node.js 6.10 in both environments.
2. callback to the Lambda function is invoked only after the stream ends.
3. Lambda has full access to S3
4. Also tried Promise to wait until streams complete. But no change.
What am I missing? Thank you in advance.
const AWS = require('aws-sdk');
const {parser} = require('stream-json');
const {streamArray} = require('stream-json/streamers/StreamArray');
const {chain} = require('stream-chain');
const S3 = new AWS.S3({ apiVersion: '2006-03-01' });
/** ******************** Lambda Handler *************************** */
exports.handler = (event, context, callback) => {
// Get the object from the event and show its content type
const bucket = event.Records[0].s3.bucket.name;
const key = event.Records[0].s3.object.key;
const params = {
Bucket: bucket,
Key: key
};
console.log("Source: " + bucket +"//" + key);
let s3ReaderStream = S3.getObject(params).createReadStream();
console.log("Setting up pipes");
const pipeline = chain([
s3ReaderStream,
parser(),
streamArray(),
data => {
console.log(data.value);
}
]);
pipeline.on('data', (data) => console.log(data));
pipeline.on('end', () => callback(null, "Stream ended"));
};
node.js aws-lambda node.js-stream
What do you see inCloudWatch
- ex: any error string....You maybe assigngetObject
action on your S3 resource for lambda executetion rule.
– hoangdv
Nov 17 '18 at 7:03
No errors. I do see my log statements. Here is what the output looks like: START RequestId: 97899afb-ea79-11e8-8c67-15d57ea60ee7 Version: $LATEST 2018-11-17T15:01:07.573Z 97899afb-ea79-11e8-8c67-15d57ea60ee7 Source: com.lucidmatters.hurdles//HrdlSample.json 2018-11-17T15:01:07.613Z 97899afb-ea79-11e8-8c67-15d57ea60ee7 Setting up pipes END RequestId: 97899afb-ea79-11e8-8c67-15d57ea60ee7 REPORT RequestId: 97899afb-ea79-11e8-8c67-15d57ea60ee7 Duration: 8006.60 ms Billed Duration: 8000 ms Memory Size: 128 MB Max Memory Used: 37 MB ... Task timed out after 8.01 seconds
– Vinay Dhavala
Nov 17 '18 at 15:02
I had assigned a role with full access to S3
– Vinay Dhavala
Nov 17 '18 at 15:06
add a comment |
I have a node.js function for AWS Lambda. It reads a JSON file from an S3 bucket as a stream, parses it and prints the parsed objects to the console. I am using stream-json module for parsing.
It works on my local environment and prints the objects to console. But it does not print the objects to the log streams(CloudWatch) on Lambda. It simply times out after the max duration. It prints other log statements around, but not the object values.
1. Using node.js 6.10 in both environments.
2. callback to the Lambda function is invoked only after the stream ends.
3. Lambda has full access to S3
4. Also tried Promise to wait until streams complete. But no change.
What am I missing? Thank you in advance.
const AWS = require('aws-sdk');
const {parser} = require('stream-json');
const {streamArray} = require('stream-json/streamers/StreamArray');
const {chain} = require('stream-chain');
const S3 = new AWS.S3({ apiVersion: '2006-03-01' });
/** ******************** Lambda Handler *************************** */
exports.handler = (event, context, callback) => {
// Get the object from the event and show its content type
const bucket = event.Records[0].s3.bucket.name;
const key = event.Records[0].s3.object.key;
const params = {
Bucket: bucket,
Key: key
};
console.log("Source: " + bucket +"//" + key);
let s3ReaderStream = S3.getObject(params).createReadStream();
console.log("Setting up pipes");
const pipeline = chain([
s3ReaderStream,
parser(),
streamArray(),
data => {
console.log(data.value);
}
]);
pipeline.on('data', (data) => console.log(data));
pipeline.on('end', () => callback(null, "Stream ended"));
};
node.js aws-lambda node.js-stream
I have a node.js function for AWS Lambda. It reads a JSON file from an S3 bucket as a stream, parses it and prints the parsed objects to the console. I am using stream-json module for parsing.
It works on my local environment and prints the objects to console. But it does not print the objects to the log streams(CloudWatch) on Lambda. It simply times out after the max duration. It prints other log statements around, but not the object values.
1. Using node.js 6.10 in both environments.
2. callback to the Lambda function is invoked only after the stream ends.
3. Lambda has full access to S3
4. Also tried Promise to wait until streams complete. But no change.
What am I missing? Thank you in advance.
const AWS = require('aws-sdk');
const {parser} = require('stream-json');
const {streamArray} = require('stream-json/streamers/StreamArray');
const {chain} = require('stream-chain');
const S3 = new AWS.S3({ apiVersion: '2006-03-01' });
/** ******************** Lambda Handler *************************** */
exports.handler = (event, context, callback) => {
// Get the object from the event and show its content type
const bucket = event.Records[0].s3.bucket.name;
const key = event.Records[0].s3.object.key;
const params = {
Bucket: bucket,
Key: key
};
console.log("Source: " + bucket +"//" + key);
let s3ReaderStream = S3.getObject(params).createReadStream();
console.log("Setting up pipes");
const pipeline = chain([
s3ReaderStream,
parser(),
streamArray(),
data => {
console.log(data.value);
}
]);
pipeline.on('data', (data) => console.log(data));
pipeline.on('end', () => callback(null, "Stream ended"));
};
node.js aws-lambda node.js-stream
node.js aws-lambda node.js-stream
asked Nov 17 '18 at 6:54
Vinay DhavalaVinay Dhavala
164
164
What do you see inCloudWatch
- ex: any error string....You maybe assigngetObject
action on your S3 resource for lambda executetion rule.
– hoangdv
Nov 17 '18 at 7:03
No errors. I do see my log statements. Here is what the output looks like: START RequestId: 97899afb-ea79-11e8-8c67-15d57ea60ee7 Version: $LATEST 2018-11-17T15:01:07.573Z 97899afb-ea79-11e8-8c67-15d57ea60ee7 Source: com.lucidmatters.hurdles//HrdlSample.json 2018-11-17T15:01:07.613Z 97899afb-ea79-11e8-8c67-15d57ea60ee7 Setting up pipes END RequestId: 97899afb-ea79-11e8-8c67-15d57ea60ee7 REPORT RequestId: 97899afb-ea79-11e8-8c67-15d57ea60ee7 Duration: 8006.60 ms Billed Duration: 8000 ms Memory Size: 128 MB Max Memory Used: 37 MB ... Task timed out after 8.01 seconds
– Vinay Dhavala
Nov 17 '18 at 15:02
I had assigned a role with full access to S3
– Vinay Dhavala
Nov 17 '18 at 15:06
add a comment |
What do you see inCloudWatch
- ex: any error string....You maybe assigngetObject
action on your S3 resource for lambda executetion rule.
– hoangdv
Nov 17 '18 at 7:03
No errors. I do see my log statements. Here is what the output looks like: START RequestId: 97899afb-ea79-11e8-8c67-15d57ea60ee7 Version: $LATEST 2018-11-17T15:01:07.573Z 97899afb-ea79-11e8-8c67-15d57ea60ee7 Source: com.lucidmatters.hurdles//HrdlSample.json 2018-11-17T15:01:07.613Z 97899afb-ea79-11e8-8c67-15d57ea60ee7 Setting up pipes END RequestId: 97899afb-ea79-11e8-8c67-15d57ea60ee7 REPORT RequestId: 97899afb-ea79-11e8-8c67-15d57ea60ee7 Duration: 8006.60 ms Billed Duration: 8000 ms Memory Size: 128 MB Max Memory Used: 37 MB ... Task timed out after 8.01 seconds
– Vinay Dhavala
Nov 17 '18 at 15:02
I had assigned a role with full access to S3
– Vinay Dhavala
Nov 17 '18 at 15:06
What do you see in
CloudWatch
- ex: any error string....You maybe assign getObject
action on your S3 resource for lambda executetion rule.– hoangdv
Nov 17 '18 at 7:03
What do you see in
CloudWatch
- ex: any error string....You maybe assign getObject
action on your S3 resource for lambda executetion rule.– hoangdv
Nov 17 '18 at 7:03
No errors. I do see my log statements. Here is what the output looks like: START RequestId: 97899afb-ea79-11e8-8c67-15d57ea60ee7 Version: $LATEST 2018-11-17T15:01:07.573Z 97899afb-ea79-11e8-8c67-15d57ea60ee7 Source: com.lucidmatters.hurdles//HrdlSample.json 2018-11-17T15:01:07.613Z 97899afb-ea79-11e8-8c67-15d57ea60ee7 Setting up pipes END RequestId: 97899afb-ea79-11e8-8c67-15d57ea60ee7 REPORT RequestId: 97899afb-ea79-11e8-8c67-15d57ea60ee7 Duration: 8006.60 ms Billed Duration: 8000 ms Memory Size: 128 MB Max Memory Used: 37 MB ... Task timed out after 8.01 seconds
– Vinay Dhavala
Nov 17 '18 at 15:02
No errors. I do see my log statements. Here is what the output looks like: START RequestId: 97899afb-ea79-11e8-8c67-15d57ea60ee7 Version: $LATEST 2018-11-17T15:01:07.573Z 97899afb-ea79-11e8-8c67-15d57ea60ee7 Source: com.lucidmatters.hurdles//HrdlSample.json 2018-11-17T15:01:07.613Z 97899afb-ea79-11e8-8c67-15d57ea60ee7 Setting up pipes END RequestId: 97899afb-ea79-11e8-8c67-15d57ea60ee7 REPORT RequestId: 97899afb-ea79-11e8-8c67-15d57ea60ee7 Duration: 8006.60 ms Billed Duration: 8000 ms Memory Size: 128 MB Max Memory Used: 37 MB ... Task timed out after 8.01 seconds
– Vinay Dhavala
Nov 17 '18 at 15:02
I had assigned a role with full access to S3
– Vinay Dhavala
Nov 17 '18 at 15:06
I had assigned a role with full access to S3
– Vinay Dhavala
Nov 17 '18 at 15:06
add a comment |
1 Answer
1
active
oldest
votes
I have figured out that it is because my Lambda function is running inside a private VPC.
(I have to run it inside a private VPC because it needs to access my ElastiCache instance. I removed related code when I posted the code, for simplification).
Code can access S3 from my local machine, but not from the private VPC.
There is a process to ensure that S3 is accessible from within your VPC. It is posted here https://aws.amazon.com/premiumsupport/knowledge-center/connect-s3-vpc-endpoint/
Here is another link that explains how you should setup a VPC end point to be able to access AWS resources from within a VPC https://aws.amazon.com/blogs/aws/new-vpc-endpoint-for-amazon-s3/
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53348972%2fnode-js-code-works-locally-but-does-not-work-on-aws-lambda%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I have figured out that it is because my Lambda function is running inside a private VPC.
(I have to run it inside a private VPC because it needs to access my ElastiCache instance. I removed related code when I posted the code, for simplification).
Code can access S3 from my local machine, but not from the private VPC.
There is a process to ensure that S3 is accessible from within your VPC. It is posted here https://aws.amazon.com/premiumsupport/knowledge-center/connect-s3-vpc-endpoint/
Here is another link that explains how you should setup a VPC end point to be able to access AWS resources from within a VPC https://aws.amazon.com/blogs/aws/new-vpc-endpoint-for-amazon-s3/
add a comment |
I have figured out that it is because my Lambda function is running inside a private VPC.
(I have to run it inside a private VPC because it needs to access my ElastiCache instance. I removed related code when I posted the code, for simplification).
Code can access S3 from my local machine, but not from the private VPC.
There is a process to ensure that S3 is accessible from within your VPC. It is posted here https://aws.amazon.com/premiumsupport/knowledge-center/connect-s3-vpc-endpoint/
Here is another link that explains how you should setup a VPC end point to be able to access AWS resources from within a VPC https://aws.amazon.com/blogs/aws/new-vpc-endpoint-for-amazon-s3/
add a comment |
I have figured out that it is because my Lambda function is running inside a private VPC.
(I have to run it inside a private VPC because it needs to access my ElastiCache instance. I removed related code when I posted the code, for simplification).
Code can access S3 from my local machine, but not from the private VPC.
There is a process to ensure that S3 is accessible from within your VPC. It is posted here https://aws.amazon.com/premiumsupport/knowledge-center/connect-s3-vpc-endpoint/
Here is another link that explains how you should setup a VPC end point to be able to access AWS resources from within a VPC https://aws.amazon.com/blogs/aws/new-vpc-endpoint-for-amazon-s3/
I have figured out that it is because my Lambda function is running inside a private VPC.
(I have to run it inside a private VPC because it needs to access my ElastiCache instance. I removed related code when I posted the code, for simplification).
Code can access S3 from my local machine, but not from the private VPC.
There is a process to ensure that S3 is accessible from within your VPC. It is posted here https://aws.amazon.com/premiumsupport/knowledge-center/connect-s3-vpc-endpoint/
Here is another link that explains how you should setup a VPC end point to be able to access AWS resources from within a VPC https://aws.amazon.com/blogs/aws/new-vpc-endpoint-for-amazon-s3/
edited Nov 17 '18 at 17:30
answered Nov 17 '18 at 15:42
Vinay DhavalaVinay Dhavala
164
164
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53348972%2fnode-js-code-works-locally-but-does-not-work-on-aws-lambda%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
E1oFFltu2gSdnO6tiYlKOcQXSZDoLX
What do you see in
CloudWatch
- ex: any error string....You maybe assigngetObject
action on your S3 resource for lambda executetion rule.– hoangdv
Nov 17 '18 at 7:03
No errors. I do see my log statements. Here is what the output looks like: START RequestId: 97899afb-ea79-11e8-8c67-15d57ea60ee7 Version: $LATEST 2018-11-17T15:01:07.573Z 97899afb-ea79-11e8-8c67-15d57ea60ee7 Source: com.lucidmatters.hurdles//HrdlSample.json 2018-11-17T15:01:07.613Z 97899afb-ea79-11e8-8c67-15d57ea60ee7 Setting up pipes END RequestId: 97899afb-ea79-11e8-8c67-15d57ea60ee7 REPORT RequestId: 97899afb-ea79-11e8-8c67-15d57ea60ee7 Duration: 8006.60 ms Billed Duration: 8000 ms Memory Size: 128 MB Max Memory Used: 37 MB ... Task timed out after 8.01 seconds
– Vinay Dhavala
Nov 17 '18 at 15:02
I had assigned a role with full access to S3
– Vinay Dhavala
Nov 17 '18 at 15:06