Axios disconnecting what happens next from user's action when called after clicking a button
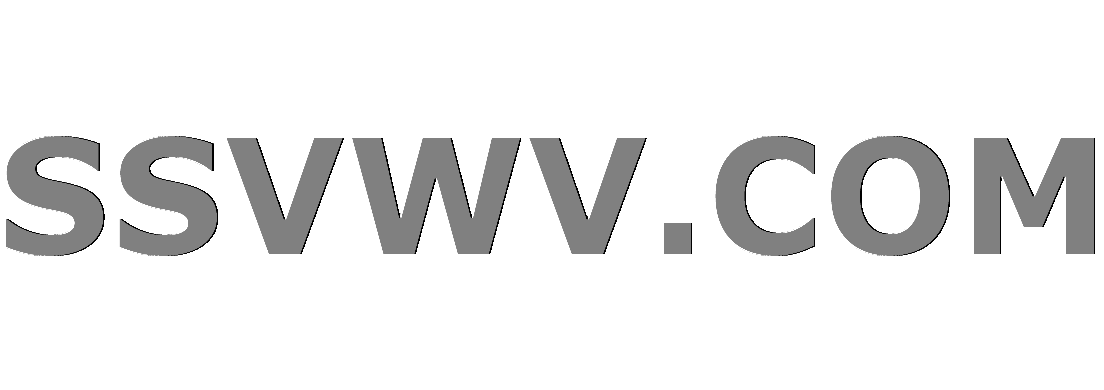
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I have the following code which basically does the following:
When the user clicks the button, an encoded text is sent to an
API
which takes care to decode it. Then the decoded text is set as a query on a Google link which is opened on a new tab.
JAVASCRIPT
// Reference:
// https://base62.io/
let message_encoded = 'T8dgcjRGkZ3aysdN'; // base62('Hello World!'')
let way = 1;
$(function () {
$('.button_test').click(async function() {
let url_api = 'https://api.base62.io/decode';
let response;
switch (way) {
case 1: /* this works */
response = await jQuerySyncPost(url_api, { data: message_encoded });
break;
case 2: /* this DOES NOT work */
response = await fetchSyncPost(url_api, { data: message_encoded });
break;
case 3: /* this DOES NOT work */
response = await axios.post(url_api, { data: message_encoded });
break;
}
alert('Next, let's look on Google for: "' + response.data.decoded + '"');
let message_decoded = response.data.decoded;
let url_google = 'https://www.google.com/search?q='+encodeURIComponent(message_decoded);
openNewTab(url_google);
});
});
function jQuerySyncPost(url, params) {
return new Promise((resolve, reject) => {
$.ajax({
type: 'POST',
url: url,
data: params,
async: false,
success: function (response) {
response = {
data: response
};
resolve(response);
},
error: function (jqXHR, textStatus, errorThrown) {
reject({
jqXHR: jqXHR,
textStatus: textStatus,
errorThrown: errorThrown
});
}
});
});
}
function fetchSyncPost(url, params) {
return new Promise((resolve, reject) => {
let config = {
headers: {
'content-type': 'application/json; charset=UTF-8',
},
body: JSON.stringify(params),
method: 'POST',
};
fetch(url, config)
.then(response => response.json())
.then(response => {
let result = {
data: response,
};
resolve(result);
});
});
}
function openNewTab(url) {
window.open(url, '_blank');
}
HTML
<button class="button_test">Click Here</button>
CSS
.invisible {
visibility: hidden;
}
.button_test {
margin-left: 200px;
margin-top: 100px;
font-size: 50px;
}
The code above is working fine with the jQuery
Ajax call (through custom function):
let response = await syncPost(url_api, { data: message_encoded });
But, in the other hand, if I use axios
, then I get a popup blocked
alert on the browser which prevents the new tab to get opened:
let response = await axios.post(url_api, { data: message_encoded });
Here you have the JSFiddle
: https://jsfiddle.net/otyzadju
where { way=1 }
works, but { way=2, way=3 }
don't.
On both cases the call to the API
via Ajax is done synchronously but the problem is that it seems that axios
is disconnecting what happens next from user's action when called after clicking a button.
Any idea on how to make this work with axios
?
If possible, please, provide your solution on a forked JSFiddle
link.
Thanks!
javascript jquery ajax html5 axios
|
show 3 more comments
I have the following code which basically does the following:
When the user clicks the button, an encoded text is sent to an
API
which takes care to decode it. Then the decoded text is set as a query on a Google link which is opened on a new tab.
JAVASCRIPT
// Reference:
// https://base62.io/
let message_encoded = 'T8dgcjRGkZ3aysdN'; // base62('Hello World!'')
let way = 1;
$(function () {
$('.button_test').click(async function() {
let url_api = 'https://api.base62.io/decode';
let response;
switch (way) {
case 1: /* this works */
response = await jQuerySyncPost(url_api, { data: message_encoded });
break;
case 2: /* this DOES NOT work */
response = await fetchSyncPost(url_api, { data: message_encoded });
break;
case 3: /* this DOES NOT work */
response = await axios.post(url_api, { data: message_encoded });
break;
}
alert('Next, let's look on Google for: "' + response.data.decoded + '"');
let message_decoded = response.data.decoded;
let url_google = 'https://www.google.com/search?q='+encodeURIComponent(message_decoded);
openNewTab(url_google);
});
});
function jQuerySyncPost(url, params) {
return new Promise((resolve, reject) => {
$.ajax({
type: 'POST',
url: url,
data: params,
async: false,
success: function (response) {
response = {
data: response
};
resolve(response);
},
error: function (jqXHR, textStatus, errorThrown) {
reject({
jqXHR: jqXHR,
textStatus: textStatus,
errorThrown: errorThrown
});
}
});
});
}
function fetchSyncPost(url, params) {
return new Promise((resolve, reject) => {
let config = {
headers: {
'content-type': 'application/json; charset=UTF-8',
},
body: JSON.stringify(params),
method: 'POST',
};
fetch(url, config)
.then(response => response.json())
.then(response => {
let result = {
data: response,
};
resolve(result);
});
});
}
function openNewTab(url) {
window.open(url, '_blank');
}
HTML
<button class="button_test">Click Here</button>
CSS
.invisible {
visibility: hidden;
}
.button_test {
margin-left: 200px;
margin-top: 100px;
font-size: 50px;
}
The code above is working fine with the jQuery
Ajax call (through custom function):
let response = await syncPost(url_api, { data: message_encoded });
But, in the other hand, if I use axios
, then I get a popup blocked
alert on the browser which prevents the new tab to get opened:
let response = await axios.post(url_api, { data: message_encoded });
Here you have the JSFiddle
: https://jsfiddle.net/otyzadju
where { way=1 }
works, but { way=2, way=3 }
don't.
On both cases the call to the API
via Ajax is done synchronously but the problem is that it seems that axios
is disconnecting what happens next from user's action when called after clicking a button.
Any idea on how to make this work with axios
?
If possible, please, provide your solution on a forked JSFiddle
link.
Thanks!
javascript jquery ajax html5 axios
This is a bit similar - I hope it helps: stackoverflow.com/questions/4602964/…
– Sparlarva
Nov 17 '18 at 8:55
@Sparlarva that's not similar. They don't useaxios
. My goal is to useAxios
.
– Viewsonic
Nov 17 '18 at 15:45
Okay but you haven't posted a problem using an axios solution.
– Sparlarva
Nov 17 '18 at 18:17
yes I did, just uncomment the line:await axios.post
and comment the line:await syncPost
– Viewsonic
Nov 17 '18 at 18:31
Have you looked in the development console to see what the error is on the browser? I can also see that there are no headers added when you are using axios?
– Sparlarva
Nov 17 '18 at 18:38
|
show 3 more comments
I have the following code which basically does the following:
When the user clicks the button, an encoded text is sent to an
API
which takes care to decode it. Then the decoded text is set as a query on a Google link which is opened on a new tab.
JAVASCRIPT
// Reference:
// https://base62.io/
let message_encoded = 'T8dgcjRGkZ3aysdN'; // base62('Hello World!'')
let way = 1;
$(function () {
$('.button_test').click(async function() {
let url_api = 'https://api.base62.io/decode';
let response;
switch (way) {
case 1: /* this works */
response = await jQuerySyncPost(url_api, { data: message_encoded });
break;
case 2: /* this DOES NOT work */
response = await fetchSyncPost(url_api, { data: message_encoded });
break;
case 3: /* this DOES NOT work */
response = await axios.post(url_api, { data: message_encoded });
break;
}
alert('Next, let's look on Google for: "' + response.data.decoded + '"');
let message_decoded = response.data.decoded;
let url_google = 'https://www.google.com/search?q='+encodeURIComponent(message_decoded);
openNewTab(url_google);
});
});
function jQuerySyncPost(url, params) {
return new Promise((resolve, reject) => {
$.ajax({
type: 'POST',
url: url,
data: params,
async: false,
success: function (response) {
response = {
data: response
};
resolve(response);
},
error: function (jqXHR, textStatus, errorThrown) {
reject({
jqXHR: jqXHR,
textStatus: textStatus,
errorThrown: errorThrown
});
}
});
});
}
function fetchSyncPost(url, params) {
return new Promise((resolve, reject) => {
let config = {
headers: {
'content-type': 'application/json; charset=UTF-8',
},
body: JSON.stringify(params),
method: 'POST',
};
fetch(url, config)
.then(response => response.json())
.then(response => {
let result = {
data: response,
};
resolve(result);
});
});
}
function openNewTab(url) {
window.open(url, '_blank');
}
HTML
<button class="button_test">Click Here</button>
CSS
.invisible {
visibility: hidden;
}
.button_test {
margin-left: 200px;
margin-top: 100px;
font-size: 50px;
}
The code above is working fine with the jQuery
Ajax call (through custom function):
let response = await syncPost(url_api, { data: message_encoded });
But, in the other hand, if I use axios
, then I get a popup blocked
alert on the browser which prevents the new tab to get opened:
let response = await axios.post(url_api, { data: message_encoded });
Here you have the JSFiddle
: https://jsfiddle.net/otyzadju
where { way=1 }
works, but { way=2, way=3 }
don't.
On both cases the call to the API
via Ajax is done synchronously but the problem is that it seems that axios
is disconnecting what happens next from user's action when called after clicking a button.
Any idea on how to make this work with axios
?
If possible, please, provide your solution on a forked JSFiddle
link.
Thanks!
javascript jquery ajax html5 axios
I have the following code which basically does the following:
When the user clicks the button, an encoded text is sent to an
API
which takes care to decode it. Then the decoded text is set as a query on a Google link which is opened on a new tab.
JAVASCRIPT
// Reference:
// https://base62.io/
let message_encoded = 'T8dgcjRGkZ3aysdN'; // base62('Hello World!'')
let way = 1;
$(function () {
$('.button_test').click(async function() {
let url_api = 'https://api.base62.io/decode';
let response;
switch (way) {
case 1: /* this works */
response = await jQuerySyncPost(url_api, { data: message_encoded });
break;
case 2: /* this DOES NOT work */
response = await fetchSyncPost(url_api, { data: message_encoded });
break;
case 3: /* this DOES NOT work */
response = await axios.post(url_api, { data: message_encoded });
break;
}
alert('Next, let's look on Google for: "' + response.data.decoded + '"');
let message_decoded = response.data.decoded;
let url_google = 'https://www.google.com/search?q='+encodeURIComponent(message_decoded);
openNewTab(url_google);
});
});
function jQuerySyncPost(url, params) {
return new Promise((resolve, reject) => {
$.ajax({
type: 'POST',
url: url,
data: params,
async: false,
success: function (response) {
response = {
data: response
};
resolve(response);
},
error: function (jqXHR, textStatus, errorThrown) {
reject({
jqXHR: jqXHR,
textStatus: textStatus,
errorThrown: errorThrown
});
}
});
});
}
function fetchSyncPost(url, params) {
return new Promise((resolve, reject) => {
let config = {
headers: {
'content-type': 'application/json; charset=UTF-8',
},
body: JSON.stringify(params),
method: 'POST',
};
fetch(url, config)
.then(response => response.json())
.then(response => {
let result = {
data: response,
};
resolve(result);
});
});
}
function openNewTab(url) {
window.open(url, '_blank');
}
HTML
<button class="button_test">Click Here</button>
CSS
.invisible {
visibility: hidden;
}
.button_test {
margin-left: 200px;
margin-top: 100px;
font-size: 50px;
}
The code above is working fine with the jQuery
Ajax call (through custom function):
let response = await syncPost(url_api, { data: message_encoded });
But, in the other hand, if I use axios
, then I get a popup blocked
alert on the browser which prevents the new tab to get opened:
let response = await axios.post(url_api, { data: message_encoded });
Here you have the JSFiddle
: https://jsfiddle.net/otyzadju
where { way=1 }
works, but { way=2, way=3 }
don't.
On both cases the call to the API
via Ajax is done synchronously but the problem is that it seems that axios
is disconnecting what happens next from user's action when called after clicking a button.
Any idea on how to make this work with axios
?
If possible, please, provide your solution on a forked JSFiddle
link.
Thanks!
javascript jquery ajax html5 axios
javascript jquery ajax html5 axios
edited Nov 17 '18 at 19:36
Viewsonic
asked Nov 17 '18 at 6:43


ViewsonicViewsonic
1189
1189
This is a bit similar - I hope it helps: stackoverflow.com/questions/4602964/…
– Sparlarva
Nov 17 '18 at 8:55
@Sparlarva that's not similar. They don't useaxios
. My goal is to useAxios
.
– Viewsonic
Nov 17 '18 at 15:45
Okay but you haven't posted a problem using an axios solution.
– Sparlarva
Nov 17 '18 at 18:17
yes I did, just uncomment the line:await axios.post
and comment the line:await syncPost
– Viewsonic
Nov 17 '18 at 18:31
Have you looked in the development console to see what the error is on the browser? I can also see that there are no headers added when you are using axios?
– Sparlarva
Nov 17 '18 at 18:38
|
show 3 more comments
This is a bit similar - I hope it helps: stackoverflow.com/questions/4602964/…
– Sparlarva
Nov 17 '18 at 8:55
@Sparlarva that's not similar. They don't useaxios
. My goal is to useAxios
.
– Viewsonic
Nov 17 '18 at 15:45
Okay but you haven't posted a problem using an axios solution.
– Sparlarva
Nov 17 '18 at 18:17
yes I did, just uncomment the line:await axios.post
and comment the line:await syncPost
– Viewsonic
Nov 17 '18 at 18:31
Have you looked in the development console to see what the error is on the browser? I can also see that there are no headers added when you are using axios?
– Sparlarva
Nov 17 '18 at 18:38
This is a bit similar - I hope it helps: stackoverflow.com/questions/4602964/…
– Sparlarva
Nov 17 '18 at 8:55
This is a bit similar - I hope it helps: stackoverflow.com/questions/4602964/…
– Sparlarva
Nov 17 '18 at 8:55
@Sparlarva that's not similar. They don't use
axios
. My goal is to use Axios
.– Viewsonic
Nov 17 '18 at 15:45
@Sparlarva that's not similar. They don't use
axios
. My goal is to use Axios
.– Viewsonic
Nov 17 '18 at 15:45
Okay but you haven't posted a problem using an axios solution.
– Sparlarva
Nov 17 '18 at 18:17
Okay but you haven't posted a problem using an axios solution.
– Sparlarva
Nov 17 '18 at 18:17
yes I did, just uncomment the line:
await axios.post
and comment the line: await syncPost
– Viewsonic
Nov 17 '18 at 18:31
yes I did, just uncomment the line:
await axios.post
and comment the line: await syncPost
– Viewsonic
Nov 17 '18 at 18:31
Have you looked in the development console to see what the error is on the browser? I can also see that there are no headers added when you are using axios?
– Sparlarva
Nov 17 '18 at 18:38
Have you looked in the development console to see what the error is on the browser? I can also see that there are no headers added when you are using axios?
– Sparlarva
Nov 17 '18 at 18:38
|
show 3 more comments
1 Answer
1
active
oldest
votes
I changed the answer to window.open so it will work with axios.post - it also works with fetch. If you didn't use this originally for a certain reason then please let me know and I can try and update the answer.
function openNewTab(url) {
window.open(url, '_blank')
}
This is a JSfiddle fork with it set to option 2 and the opennewtab function altered.
https://jsfiddle.net/xuywfqzn/
Unfortunatelly I keep getting the popup blocker with:{ way=2, way=3 }
. I ended up using the other code foropenNewTab(...)
I think because I tried wrong parameters withwindow.open(...)
initially. I updated it's code, thanks for that. Beside that, I keep having the issue with the popup blocker. Do you say you don't get the popup blocker?, maybe you disabled it on the browser config? I tried with both:Chrome
andFirefox
and on both browsers I get the popup blocker with ways: 2 and 3. Here is my code updated: jsfiddle.net/otyzadju Thanks!
– Viewsonic
Nov 17 '18 at 19:25
My pop up definitely disappeared even with it enabled i checked but let me look again, give me a little time im just about to have my birthday cake (dont worry its my birthday in 4 days - just at my parents)
– Sparlarva
Nov 17 '18 at 20:43
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53348902%2faxios-disconnecting-what-happens-next-from-users-action-when-called-after-click%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I changed the answer to window.open so it will work with axios.post - it also works with fetch. If you didn't use this originally for a certain reason then please let me know and I can try and update the answer.
function openNewTab(url) {
window.open(url, '_blank')
}
This is a JSfiddle fork with it set to option 2 and the opennewtab function altered.
https://jsfiddle.net/xuywfqzn/
Unfortunatelly I keep getting the popup blocker with:{ way=2, way=3 }
. I ended up using the other code foropenNewTab(...)
I think because I tried wrong parameters withwindow.open(...)
initially. I updated it's code, thanks for that. Beside that, I keep having the issue with the popup blocker. Do you say you don't get the popup blocker?, maybe you disabled it on the browser config? I tried with both:Chrome
andFirefox
and on both browsers I get the popup blocker with ways: 2 and 3. Here is my code updated: jsfiddle.net/otyzadju Thanks!
– Viewsonic
Nov 17 '18 at 19:25
My pop up definitely disappeared even with it enabled i checked but let me look again, give me a little time im just about to have my birthday cake (dont worry its my birthday in 4 days - just at my parents)
– Sparlarva
Nov 17 '18 at 20:43
add a comment |
I changed the answer to window.open so it will work with axios.post - it also works with fetch. If you didn't use this originally for a certain reason then please let me know and I can try and update the answer.
function openNewTab(url) {
window.open(url, '_blank')
}
This is a JSfiddle fork with it set to option 2 and the opennewtab function altered.
https://jsfiddle.net/xuywfqzn/
Unfortunatelly I keep getting the popup blocker with:{ way=2, way=3 }
. I ended up using the other code foropenNewTab(...)
I think because I tried wrong parameters withwindow.open(...)
initially. I updated it's code, thanks for that. Beside that, I keep having the issue with the popup blocker. Do you say you don't get the popup blocker?, maybe you disabled it on the browser config? I tried with both:Chrome
andFirefox
and on both browsers I get the popup blocker with ways: 2 and 3. Here is my code updated: jsfiddle.net/otyzadju Thanks!
– Viewsonic
Nov 17 '18 at 19:25
My pop up definitely disappeared even with it enabled i checked but let me look again, give me a little time im just about to have my birthday cake (dont worry its my birthday in 4 days - just at my parents)
– Sparlarva
Nov 17 '18 at 20:43
add a comment |
I changed the answer to window.open so it will work with axios.post - it also works with fetch. If you didn't use this originally for a certain reason then please let me know and I can try and update the answer.
function openNewTab(url) {
window.open(url, '_blank')
}
This is a JSfiddle fork with it set to option 2 and the opennewtab function altered.
https://jsfiddle.net/xuywfqzn/
I changed the answer to window.open so it will work with axios.post - it also works with fetch. If you didn't use this originally for a certain reason then please let me know and I can try and update the answer.
function openNewTab(url) {
window.open(url, '_blank')
}
This is a JSfiddle fork with it set to option 2 and the opennewtab function altered.
https://jsfiddle.net/xuywfqzn/
answered Nov 17 '18 at 19:08


SparlarvaSparlarva
25711
25711
Unfortunatelly I keep getting the popup blocker with:{ way=2, way=3 }
. I ended up using the other code foropenNewTab(...)
I think because I tried wrong parameters withwindow.open(...)
initially. I updated it's code, thanks for that. Beside that, I keep having the issue with the popup blocker. Do you say you don't get the popup blocker?, maybe you disabled it on the browser config? I tried with both:Chrome
andFirefox
and on both browsers I get the popup blocker with ways: 2 and 3. Here is my code updated: jsfiddle.net/otyzadju Thanks!
– Viewsonic
Nov 17 '18 at 19:25
My pop up definitely disappeared even with it enabled i checked but let me look again, give me a little time im just about to have my birthday cake (dont worry its my birthday in 4 days - just at my parents)
– Sparlarva
Nov 17 '18 at 20:43
add a comment |
Unfortunatelly I keep getting the popup blocker with:{ way=2, way=3 }
. I ended up using the other code foropenNewTab(...)
I think because I tried wrong parameters withwindow.open(...)
initially. I updated it's code, thanks for that. Beside that, I keep having the issue with the popup blocker. Do you say you don't get the popup blocker?, maybe you disabled it on the browser config? I tried with both:Chrome
andFirefox
and on both browsers I get the popup blocker with ways: 2 and 3. Here is my code updated: jsfiddle.net/otyzadju Thanks!
– Viewsonic
Nov 17 '18 at 19:25
My pop up definitely disappeared even with it enabled i checked but let me look again, give me a little time im just about to have my birthday cake (dont worry its my birthday in 4 days - just at my parents)
– Sparlarva
Nov 17 '18 at 20:43
Unfortunatelly I keep getting the popup blocker with:
{ way=2, way=3 }
. I ended up using the other code for openNewTab(...)
I think because I tried wrong parameters with window.open(...)
initially. I updated it's code, thanks for that. Beside that, I keep having the issue with the popup blocker. Do you say you don't get the popup blocker?, maybe you disabled it on the browser config? I tried with both: Chrome
and Firefox
and on both browsers I get the popup blocker with ways: 2 and 3. Here is my code updated: jsfiddle.net/otyzadju Thanks!– Viewsonic
Nov 17 '18 at 19:25
Unfortunatelly I keep getting the popup blocker with:
{ way=2, way=3 }
. I ended up using the other code for openNewTab(...)
I think because I tried wrong parameters with window.open(...)
initially. I updated it's code, thanks for that. Beside that, I keep having the issue with the popup blocker. Do you say you don't get the popup blocker?, maybe you disabled it on the browser config? I tried with both: Chrome
and Firefox
and on both browsers I get the popup blocker with ways: 2 and 3. Here is my code updated: jsfiddle.net/otyzadju Thanks!– Viewsonic
Nov 17 '18 at 19:25
My pop up definitely disappeared even with it enabled i checked but let me look again, give me a little time im just about to have my birthday cake (dont worry its my birthday in 4 days - just at my parents)
– Sparlarva
Nov 17 '18 at 20:43
My pop up definitely disappeared even with it enabled i checked but let me look again, give me a little time im just about to have my birthday cake (dont worry its my birthday in 4 days - just at my parents)
– Sparlarva
Nov 17 '18 at 20:43
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53348902%2faxios-disconnecting-what-happens-next-from-users-action-when-called-after-click%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
VGua,lTzL iylYiycQsxjnIfczlNrJAIKldOuYyk00KaSuV6BQ EAtIgh3,hYVW
This is a bit similar - I hope it helps: stackoverflow.com/questions/4602964/…
– Sparlarva
Nov 17 '18 at 8:55
@Sparlarva that's not similar. They don't use
axios
. My goal is to useAxios
.– Viewsonic
Nov 17 '18 at 15:45
Okay but you haven't posted a problem using an axios solution.
– Sparlarva
Nov 17 '18 at 18:17
yes I did, just uncomment the line:
await axios.post
and comment the line:await syncPost
– Viewsonic
Nov 17 '18 at 18:31
Have you looked in the development console to see what the error is on the browser? I can also see that there are no headers added when you are using axios?
– Sparlarva
Nov 17 '18 at 18:38