node js Can't set headers after they are sent
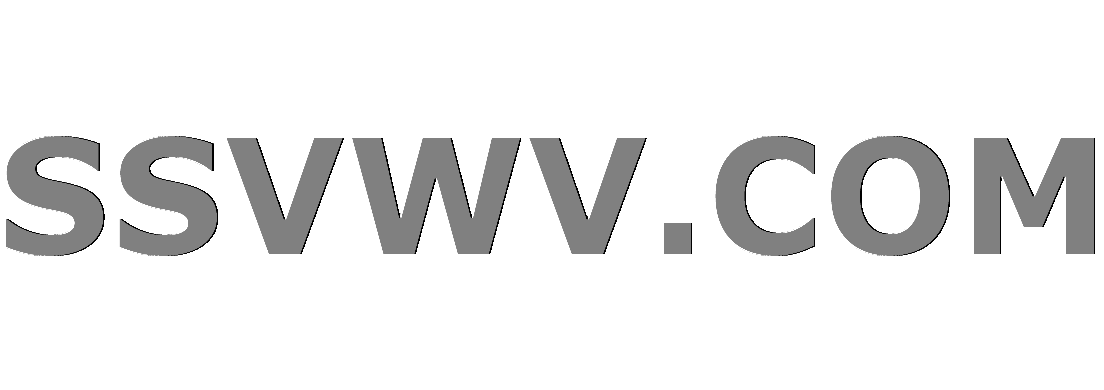
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
i'm new to nodejs i'm trying to make secure route using jwt and passport it work's fine but when i try to verify some information in database using mongoose and returning result i'm getting this error "Can't set headers after they are sent"
here is the code that generate the error (res.status(404).json(errors))
router.get(
'/',
passport.authenticate('jwt', { session: false }),
(req, res) => {
const errors = {};
Profile.findOne({ user: req.user.id })
.then(profile => {
if (!profile) {
errors.noprofile = 'There is no profile for this user';
return res.status(404).json(errors);
}
res.json(profile);
})
.catch(err => console.log(err));
}
);
this is profile model shcema
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const ProfileSchema = new Schema({
user: {
type: Schema.Types.ObjectId,
ref: 'user'
},
handel: {
type: String,
require: true,
max: 40
},
company: {
type: String,
},
webSite: {
type: String,
},
location: {
type: String,
},
});
module.exports = Profile = mongoose.model('profile', ProfileSchema);
this is my server
const express = require('express');
const mongoose = require('mongoose');
const bodyParser = require('body-parser');
const passport = require('passport');
const profile = require('./routes/api/profile');
const app = express();
// passport
app.use(passport.initialize());
// passport config
require('./config/passport')(passport);
// body Parser middleware
app.use(bodyParser.urlencoded({extended:false}));
app.use(bodyParser.json());
//DB config and connection
const db=require('./config/keys').mongoURI;
mongoose.connect(db, { useNewUrlParser: true })
.then(() => console.log("connected"))
.catch(err => console.log(err))
app.use('/api/profile',profile);
const port=process.env.PORT ||5000
app.listen(port,() => console.log('works'))
this is my passport config
const JwtStrategy = require('passport-jwt').Strategy;
const ExtractJwt = require('passport-jwt').ExtractJwt;
const mongoose = require('mongoose');
const User = mongoose.model('user');
const keys = require('./keys');
const opts = {};
opts.jwtFromRequest = ExtractJwt.fromAuthHeaderAsBearerToken();
opts.secretOrKey = keys.secretKey;
module.exports = passport => {
passport.use(
new JwtStrategy(opts, (jwt_payload, done) => {
User.findById(jwt_payload.id)
.then(user => {
if(user){
done(null, user);
}
done(null, false);
}).catch(err => console.log(err));
}))
}
node.js mongoose jwt passport.js
add a comment |
i'm new to nodejs i'm trying to make secure route using jwt and passport it work's fine but when i try to verify some information in database using mongoose and returning result i'm getting this error "Can't set headers after they are sent"
here is the code that generate the error (res.status(404).json(errors))
router.get(
'/',
passport.authenticate('jwt', { session: false }),
(req, res) => {
const errors = {};
Profile.findOne({ user: req.user.id })
.then(profile => {
if (!profile) {
errors.noprofile = 'There is no profile for this user';
return res.status(404).json(errors);
}
res.json(profile);
})
.catch(err => console.log(err));
}
);
this is profile model shcema
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const ProfileSchema = new Schema({
user: {
type: Schema.Types.ObjectId,
ref: 'user'
},
handel: {
type: String,
require: true,
max: 40
},
company: {
type: String,
},
webSite: {
type: String,
},
location: {
type: String,
},
});
module.exports = Profile = mongoose.model('profile', ProfileSchema);
this is my server
const express = require('express');
const mongoose = require('mongoose');
const bodyParser = require('body-parser');
const passport = require('passport');
const profile = require('./routes/api/profile');
const app = express();
// passport
app.use(passport.initialize());
// passport config
require('./config/passport')(passport);
// body Parser middleware
app.use(bodyParser.urlencoded({extended:false}));
app.use(bodyParser.json());
//DB config and connection
const db=require('./config/keys').mongoURI;
mongoose.connect(db, { useNewUrlParser: true })
.then(() => console.log("connected"))
.catch(err => console.log(err))
app.use('/api/profile',profile);
const port=process.env.PORT ||5000
app.listen(port,() => console.log('works'))
this is my passport config
const JwtStrategy = require('passport-jwt').Strategy;
const ExtractJwt = require('passport-jwt').ExtractJwt;
const mongoose = require('mongoose');
const User = mongoose.model('user');
const keys = require('./keys');
const opts = {};
opts.jwtFromRequest = ExtractJwt.fromAuthHeaderAsBearerToken();
opts.secretOrKey = keys.secretKey;
module.exports = passport => {
passport.use(
new JwtStrategy(opts, (jwt_payload, done) => {
User.findById(jwt_payload.id)
.then(user => {
if(user){
done(null, user);
}
done(null, false);
}).catch(err => console.log(err));
}))
}
node.js mongoose jwt passport.js
add a comment |
i'm new to nodejs i'm trying to make secure route using jwt and passport it work's fine but when i try to verify some information in database using mongoose and returning result i'm getting this error "Can't set headers after they are sent"
here is the code that generate the error (res.status(404).json(errors))
router.get(
'/',
passport.authenticate('jwt', { session: false }),
(req, res) => {
const errors = {};
Profile.findOne({ user: req.user.id })
.then(profile => {
if (!profile) {
errors.noprofile = 'There is no profile for this user';
return res.status(404).json(errors);
}
res.json(profile);
})
.catch(err => console.log(err));
}
);
this is profile model shcema
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const ProfileSchema = new Schema({
user: {
type: Schema.Types.ObjectId,
ref: 'user'
},
handel: {
type: String,
require: true,
max: 40
},
company: {
type: String,
},
webSite: {
type: String,
},
location: {
type: String,
},
});
module.exports = Profile = mongoose.model('profile', ProfileSchema);
this is my server
const express = require('express');
const mongoose = require('mongoose');
const bodyParser = require('body-parser');
const passport = require('passport');
const profile = require('./routes/api/profile');
const app = express();
// passport
app.use(passport.initialize());
// passport config
require('./config/passport')(passport);
// body Parser middleware
app.use(bodyParser.urlencoded({extended:false}));
app.use(bodyParser.json());
//DB config and connection
const db=require('./config/keys').mongoURI;
mongoose.connect(db, { useNewUrlParser: true })
.then(() => console.log("connected"))
.catch(err => console.log(err))
app.use('/api/profile',profile);
const port=process.env.PORT ||5000
app.listen(port,() => console.log('works'))
this is my passport config
const JwtStrategy = require('passport-jwt').Strategy;
const ExtractJwt = require('passport-jwt').ExtractJwt;
const mongoose = require('mongoose');
const User = mongoose.model('user');
const keys = require('./keys');
const opts = {};
opts.jwtFromRequest = ExtractJwt.fromAuthHeaderAsBearerToken();
opts.secretOrKey = keys.secretKey;
module.exports = passport => {
passport.use(
new JwtStrategy(opts, (jwt_payload, done) => {
User.findById(jwt_payload.id)
.then(user => {
if(user){
done(null, user);
}
done(null, false);
}).catch(err => console.log(err));
}))
}
node.js mongoose jwt passport.js
i'm new to nodejs i'm trying to make secure route using jwt and passport it work's fine but when i try to verify some information in database using mongoose and returning result i'm getting this error "Can't set headers after they are sent"
here is the code that generate the error (res.status(404).json(errors))
router.get(
'/',
passport.authenticate('jwt', { session: false }),
(req, res) => {
const errors = {};
Profile.findOne({ user: req.user.id })
.then(profile => {
if (!profile) {
errors.noprofile = 'There is no profile for this user';
return res.status(404).json(errors);
}
res.json(profile);
})
.catch(err => console.log(err));
}
);
this is profile model shcema
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const ProfileSchema = new Schema({
user: {
type: Schema.Types.ObjectId,
ref: 'user'
},
handel: {
type: String,
require: true,
max: 40
},
company: {
type: String,
},
webSite: {
type: String,
},
location: {
type: String,
},
});
module.exports = Profile = mongoose.model('profile', ProfileSchema);
this is my server
const express = require('express');
const mongoose = require('mongoose');
const bodyParser = require('body-parser');
const passport = require('passport');
const profile = require('./routes/api/profile');
const app = express();
// passport
app.use(passport.initialize());
// passport config
require('./config/passport')(passport);
// body Parser middleware
app.use(bodyParser.urlencoded({extended:false}));
app.use(bodyParser.json());
//DB config and connection
const db=require('./config/keys').mongoURI;
mongoose.connect(db, { useNewUrlParser: true })
.then(() => console.log("connected"))
.catch(err => console.log(err))
app.use('/api/profile',profile);
const port=process.env.PORT ||5000
app.listen(port,() => console.log('works'))
this is my passport config
const JwtStrategy = require('passport-jwt').Strategy;
const ExtractJwt = require('passport-jwt').ExtractJwt;
const mongoose = require('mongoose');
const User = mongoose.model('user');
const keys = require('./keys');
const opts = {};
opts.jwtFromRequest = ExtractJwt.fromAuthHeaderAsBearerToken();
opts.secretOrKey = keys.secretKey;
module.exports = passport => {
passport.use(
new JwtStrategy(opts, (jwt_payload, done) => {
User.findById(jwt_payload.id)
.then(user => {
if(user){
done(null, user);
}
done(null, false);
}).catch(err => console.log(err));
}))
}
node.js mongoose jwt passport.js
node.js mongoose jwt passport.js
asked Nov 16 '18 at 13:16


Delassi OthmaneDelassi Othmane
33
33
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53338678%2fnode-js-cant-set-headers-after-they-are-sent%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53338678%2fnode-js-cant-set-headers-after-they-are-sent%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
TGErwka9x,2M6F7n YN,23s3s3 i pH40jD bxN,xFyaULmEV,EsB tFfsZ Ud8,EoTDsJmbaW s6J34jbQw8qsljpp