Getting arbitrary nested data in single query, with a golang ORM?
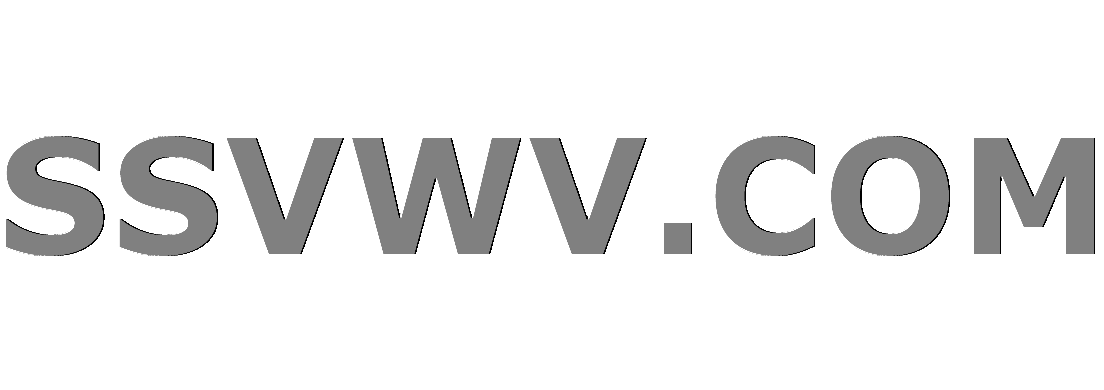
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I am attempting to develop a library in go, to allow the user to specify an arbitrary data structure, and easily set up endpoints that perform CRUD operations on an auto generated postgres database, based on the structure that they define.
For now, I have been using gorm, and am able to have a database automatically generated based on a user-defined set of structs, that support all types of relations (has one, one to many, etc.). I am able to insert, and retrieve the nested structs with preloading.
This is where an efficiency problem lies. Although I am able to put queries in the preloading functions, it still makes multiple queries, as opposed to how Java's hybernate does it, through a single query.
For example, if the user defines:
type Member struct {
ID string
FirstName string
Hometown Hometown `gorm:"ForeignKey:MemberRefer"`
}
type Hometown struct {
ID string
City string
Province string
MemberRefer string
}
Let's say we are trying to query all members with an age > 20, who live in the city of Winnipeg. There may be a million members over the age of 20, but only a handful living in Winnipeg. In a single query, postgres would determine which query to run first, in order to maximize performance.
In gorm however, it will first run the user query:
SELECT * FROM "members" WHERE (age > '20')
, and then run the hometown query: SELECT * FROM "hometowns" WHERE ("member_refer" IN (...)) AND (city = 'Manitoba')
. This would really hurt performance on a large database.
Does anyone know of an orm in golang that works in a single query?
Thanks
sql postgresql go orm go-gorm
add a comment |
I am attempting to develop a library in go, to allow the user to specify an arbitrary data structure, and easily set up endpoints that perform CRUD operations on an auto generated postgres database, based on the structure that they define.
For now, I have been using gorm, and am able to have a database automatically generated based on a user-defined set of structs, that support all types of relations (has one, one to many, etc.). I am able to insert, and retrieve the nested structs with preloading.
This is where an efficiency problem lies. Although I am able to put queries in the preloading functions, it still makes multiple queries, as opposed to how Java's hybernate does it, through a single query.
For example, if the user defines:
type Member struct {
ID string
FirstName string
Hometown Hometown `gorm:"ForeignKey:MemberRefer"`
}
type Hometown struct {
ID string
City string
Province string
MemberRefer string
}
Let's say we are trying to query all members with an age > 20, who live in the city of Winnipeg. There may be a million members over the age of 20, but only a handful living in Winnipeg. In a single query, postgres would determine which query to run first, in order to maximize performance.
In gorm however, it will first run the user query:
SELECT * FROM "members" WHERE (age > '20')
, and then run the hometown query: SELECT * FROM "hometowns" WHERE ("member_refer" IN (...)) AND (city = 'Manitoba')
. This would really hurt performance on a large database.
Does anyone know of an orm in golang that works in a single query?
Thanks
sql postgresql go orm go-gorm
Your question as written "Does anyone know of an orm in golang that works in a single query?" is off-topic for StackOverflow because Questions asking us to recommend or find a book, tool, software library, tutorial or other off-site resource are off-topic for Stack Overflow as they tend to attract opinionated answers and spam.
– Adrian
Nov 16 '18 at 19:45
add a comment |
I am attempting to develop a library in go, to allow the user to specify an arbitrary data structure, and easily set up endpoints that perform CRUD operations on an auto generated postgres database, based on the structure that they define.
For now, I have been using gorm, and am able to have a database automatically generated based on a user-defined set of structs, that support all types of relations (has one, one to many, etc.). I am able to insert, and retrieve the nested structs with preloading.
This is where an efficiency problem lies. Although I am able to put queries in the preloading functions, it still makes multiple queries, as opposed to how Java's hybernate does it, through a single query.
For example, if the user defines:
type Member struct {
ID string
FirstName string
Hometown Hometown `gorm:"ForeignKey:MemberRefer"`
}
type Hometown struct {
ID string
City string
Province string
MemberRefer string
}
Let's say we are trying to query all members with an age > 20, who live in the city of Winnipeg. There may be a million members over the age of 20, but only a handful living in Winnipeg. In a single query, postgres would determine which query to run first, in order to maximize performance.
In gorm however, it will first run the user query:
SELECT * FROM "members" WHERE (age > '20')
, and then run the hometown query: SELECT * FROM "hometowns" WHERE ("member_refer" IN (...)) AND (city = 'Manitoba')
. This would really hurt performance on a large database.
Does anyone know of an orm in golang that works in a single query?
Thanks
sql postgresql go orm go-gorm
I am attempting to develop a library in go, to allow the user to specify an arbitrary data structure, and easily set up endpoints that perform CRUD operations on an auto generated postgres database, based on the structure that they define.
For now, I have been using gorm, and am able to have a database automatically generated based on a user-defined set of structs, that support all types of relations (has one, one to many, etc.). I am able to insert, and retrieve the nested structs with preloading.
This is where an efficiency problem lies. Although I am able to put queries in the preloading functions, it still makes multiple queries, as opposed to how Java's hybernate does it, through a single query.
For example, if the user defines:
type Member struct {
ID string
FirstName string
Hometown Hometown `gorm:"ForeignKey:MemberRefer"`
}
type Hometown struct {
ID string
City string
Province string
MemberRefer string
}
Let's say we are trying to query all members with an age > 20, who live in the city of Winnipeg. There may be a million members over the age of 20, but only a handful living in Winnipeg. In a single query, postgres would determine which query to run first, in order to maximize performance.
In gorm however, it will first run the user query:
SELECT * FROM "members" WHERE (age > '20')
, and then run the hometown query: SELECT * FROM "hometowns" WHERE ("member_refer" IN (...)) AND (city = 'Manitoba')
. This would really hurt performance on a large database.
Does anyone know of an orm in golang that works in a single query?
Thanks
sql postgresql go orm go-gorm
sql postgresql go orm go-gorm
asked Nov 16 '18 at 19:43


robbieperry22robbieperry22
315217
315217
Your question as written "Does anyone know of an orm in golang that works in a single query?" is off-topic for StackOverflow because Questions asking us to recommend or find a book, tool, software library, tutorial or other off-site resource are off-topic for Stack Overflow as they tend to attract opinionated answers and spam.
– Adrian
Nov 16 '18 at 19:45
add a comment |
Your question as written "Does anyone know of an orm in golang that works in a single query?" is off-topic for StackOverflow because Questions asking us to recommend or find a book, tool, software library, tutorial or other off-site resource are off-topic for Stack Overflow as they tend to attract opinionated answers and spam.
– Adrian
Nov 16 '18 at 19:45
Your question as written "Does anyone know of an orm in golang that works in a single query?" is off-topic for StackOverflow because Questions asking us to recommend or find a book, tool, software library, tutorial or other off-site resource are off-topic for Stack Overflow as they tend to attract opinionated answers and spam.
– Adrian
Nov 16 '18 at 19:45
Your question as written "Does anyone know of an orm in golang that works in a single query?" is off-topic for StackOverflow because Questions asking us to recommend or find a book, tool, software library, tutorial or other off-site resource are off-topic for Stack Overflow as they tend to attract opinionated answers and spam.
– Adrian
Nov 16 '18 at 19:45
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53344373%2fgetting-arbitrary-nested-data-in-single-query-with-a-golang-orm%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53344373%2fgetting-arbitrary-nested-data-in-single-query-with-a-golang-orm%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
81F5MkBCxQu,ALBBj
Your question as written "Does anyone know of an orm in golang that works in a single query?" is off-topic for StackOverflow because Questions asking us to recommend or find a book, tool, software library, tutorial or other off-site resource are off-topic for Stack Overflow as they tend to attract opinionated answers and spam.
– Adrian
Nov 16 '18 at 19:45