X,Y line offset without self intersection
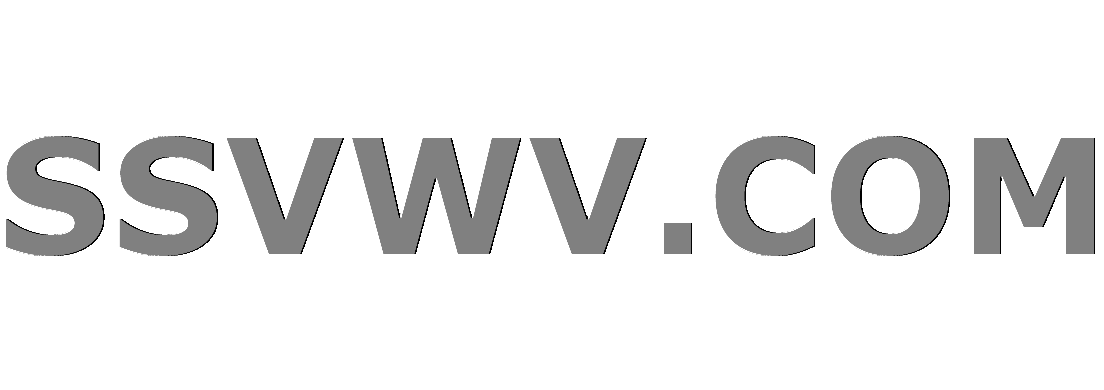
Multi tool use
I am trying to correct this C# code which should create an offset line without self intersection. It calculates its offset from probed points(x,y coordinates) and an inputted offset distance. The Y offset component works but the X does not. Any help or insight would be infinitely appreciated.
Here is the section of code that executes this process.
private void OffsetData(double R)
{
double Increment = 0.01;
X2 = (double)X.Clone(); // copy original
// apply a circle to every measured point and
// push neighbor points out beyond the circle
for (int i = 0; i < Npts; i++)
{
// offset forward
double k = i + Increment;
while (k < Npts - 1)
{
// determine how far away the neighbor is in Y
double dy = (k - i) * DeltaY;
// compute extent outward in X
double d = R * R - dy * dy;
if (d < 0) break; // beyond circel radius - no need to go further
double dx = Math.Sqrt(d);
//interpolate to find X[k] where K is a fraction
int ki = (int)k;
double Xk = X[ki] + (X[ki + 1] - X[ki]) * (k - ki);
// if x value is within circle push out
if (X2[i] < Xk + dx) X2[i] = Xk + dx;
k += Increment;
}
// offset backward
k = i - Increment;
while (k > 0)
{
// determine how far away the neighbor is in Y
double dy = (k - i) * DeltaY;
// compute extent outward in X
double d = R * R - dy * dy;
if (d < 0) break;
// beyond circle radius - no need to go further
double dx = Math.Sqrt(d);
//interpolate to find X[k] where K is a fraction
int ki = (int)k;
double Xk = X[ki] + (X[ki + 1] - X[ki]) * (k - ki);
// if x value is within circle push out
if (X2[i] < Xk + dx) X2[i] = Xk + dx;
k -= Increment;
}
}
}
c#
add a comment |
I am trying to correct this C# code which should create an offset line without self intersection. It calculates its offset from probed points(x,y coordinates) and an inputted offset distance. The Y offset component works but the X does not. Any help or insight would be infinitely appreciated.
Here is the section of code that executes this process.
private void OffsetData(double R)
{
double Increment = 0.01;
X2 = (double)X.Clone(); // copy original
// apply a circle to every measured point and
// push neighbor points out beyond the circle
for (int i = 0; i < Npts; i++)
{
// offset forward
double k = i + Increment;
while (k < Npts - 1)
{
// determine how far away the neighbor is in Y
double dy = (k - i) * DeltaY;
// compute extent outward in X
double d = R * R - dy * dy;
if (d < 0) break; // beyond circel radius - no need to go further
double dx = Math.Sqrt(d);
//interpolate to find X[k] where K is a fraction
int ki = (int)k;
double Xk = X[ki] + (X[ki + 1] - X[ki]) * (k - ki);
// if x value is within circle push out
if (X2[i] < Xk + dx) X2[i] = Xk + dx;
k += Increment;
}
// offset backward
k = i - Increment;
while (k > 0)
{
// determine how far away the neighbor is in Y
double dy = (k - i) * DeltaY;
// compute extent outward in X
double d = R * R - dy * dy;
if (d < 0) break;
// beyond circle radius - no need to go further
double dx = Math.Sqrt(d);
//interpolate to find X[k] where K is a fraction
int ki = (int)k;
double Xk = X[ki] + (X[ki + 1] - X[ki]) * (k - ki);
// if x value is within circle push out
if (X2[i] < Xk + dx) X2[i] = Xk + dx;
k -= Increment;
}
}
}
c#
Distance = sqrt(x^2 + y^2) You have a minus instead of a plus. #
– jdweng
Nov 15 '18 at 22:16
Thank you jdweng I will make that adjustment immediately and let you know how I fare. Hope you have a great week!
– CanOr1
Nov 19 '18 at 16:28
Thanks for the help jdweng unfortunately the offset didn't respond as expected to the change. We did resolve the issue although don't understand why the change we made had the effect it did. We simply removed a line of code which gave an initial 0 x,y reference and a space at the end of the code and it worked fine after that. Thanks for your time and hope you have a good one. Cheers!
– CanOr1
Nov 20 '18 at 15:03
add a comment |
I am trying to correct this C# code which should create an offset line without self intersection. It calculates its offset from probed points(x,y coordinates) and an inputted offset distance. The Y offset component works but the X does not. Any help or insight would be infinitely appreciated.
Here is the section of code that executes this process.
private void OffsetData(double R)
{
double Increment = 0.01;
X2 = (double)X.Clone(); // copy original
// apply a circle to every measured point and
// push neighbor points out beyond the circle
for (int i = 0; i < Npts; i++)
{
// offset forward
double k = i + Increment;
while (k < Npts - 1)
{
// determine how far away the neighbor is in Y
double dy = (k - i) * DeltaY;
// compute extent outward in X
double d = R * R - dy * dy;
if (d < 0) break; // beyond circel radius - no need to go further
double dx = Math.Sqrt(d);
//interpolate to find X[k] where K is a fraction
int ki = (int)k;
double Xk = X[ki] + (X[ki + 1] - X[ki]) * (k - ki);
// if x value is within circle push out
if (X2[i] < Xk + dx) X2[i] = Xk + dx;
k += Increment;
}
// offset backward
k = i - Increment;
while (k > 0)
{
// determine how far away the neighbor is in Y
double dy = (k - i) * DeltaY;
// compute extent outward in X
double d = R * R - dy * dy;
if (d < 0) break;
// beyond circle radius - no need to go further
double dx = Math.Sqrt(d);
//interpolate to find X[k] where K is a fraction
int ki = (int)k;
double Xk = X[ki] + (X[ki + 1] - X[ki]) * (k - ki);
// if x value is within circle push out
if (X2[i] < Xk + dx) X2[i] = Xk + dx;
k -= Increment;
}
}
}
c#
I am trying to correct this C# code which should create an offset line without self intersection. It calculates its offset from probed points(x,y coordinates) and an inputted offset distance. The Y offset component works but the X does not. Any help or insight would be infinitely appreciated.
Here is the section of code that executes this process.
private void OffsetData(double R)
{
double Increment = 0.01;
X2 = (double)X.Clone(); // copy original
// apply a circle to every measured point and
// push neighbor points out beyond the circle
for (int i = 0; i < Npts; i++)
{
// offset forward
double k = i + Increment;
while (k < Npts - 1)
{
// determine how far away the neighbor is in Y
double dy = (k - i) * DeltaY;
// compute extent outward in X
double d = R * R - dy * dy;
if (d < 0) break; // beyond circel radius - no need to go further
double dx = Math.Sqrt(d);
//interpolate to find X[k] where K is a fraction
int ki = (int)k;
double Xk = X[ki] + (X[ki + 1] - X[ki]) * (k - ki);
// if x value is within circle push out
if (X2[i] < Xk + dx) X2[i] = Xk + dx;
k += Increment;
}
// offset backward
k = i - Increment;
while (k > 0)
{
// determine how far away the neighbor is in Y
double dy = (k - i) * DeltaY;
// compute extent outward in X
double d = R * R - dy * dy;
if (d < 0) break;
// beyond circle radius - no need to go further
double dx = Math.Sqrt(d);
//interpolate to find X[k] where K is a fraction
int ki = (int)k;
double Xk = X[ki] + (X[ki + 1] - X[ki]) * (k - ki);
// if x value is within circle push out
if (X2[i] < Xk + dx) X2[i] = Xk + dx;
k -= Increment;
}
}
}
c#
c#
asked Nov 15 '18 at 22:00
CanOr1CanOr1
11
11
Distance = sqrt(x^2 + y^2) You have a minus instead of a plus. #
– jdweng
Nov 15 '18 at 22:16
Thank you jdweng I will make that adjustment immediately and let you know how I fare. Hope you have a great week!
– CanOr1
Nov 19 '18 at 16:28
Thanks for the help jdweng unfortunately the offset didn't respond as expected to the change. We did resolve the issue although don't understand why the change we made had the effect it did. We simply removed a line of code which gave an initial 0 x,y reference and a space at the end of the code and it worked fine after that. Thanks for your time and hope you have a good one. Cheers!
– CanOr1
Nov 20 '18 at 15:03
add a comment |
Distance = sqrt(x^2 + y^2) You have a minus instead of a plus. #
– jdweng
Nov 15 '18 at 22:16
Thank you jdweng I will make that adjustment immediately and let you know how I fare. Hope you have a great week!
– CanOr1
Nov 19 '18 at 16:28
Thanks for the help jdweng unfortunately the offset didn't respond as expected to the change. We did resolve the issue although don't understand why the change we made had the effect it did. We simply removed a line of code which gave an initial 0 x,y reference and a space at the end of the code and it worked fine after that. Thanks for your time and hope you have a good one. Cheers!
– CanOr1
Nov 20 '18 at 15:03
Distance = sqrt(x^2 + y^2) You have a minus instead of a plus. #
– jdweng
Nov 15 '18 at 22:16
Distance = sqrt(x^2 + y^2) You have a minus instead of a plus. #
– jdweng
Nov 15 '18 at 22:16
Thank you jdweng I will make that adjustment immediately and let you know how I fare. Hope you have a great week!
– CanOr1
Nov 19 '18 at 16:28
Thank you jdweng I will make that adjustment immediately and let you know how I fare. Hope you have a great week!
– CanOr1
Nov 19 '18 at 16:28
Thanks for the help jdweng unfortunately the offset didn't respond as expected to the change. We did resolve the issue although don't understand why the change we made had the effect it did. We simply removed a line of code which gave an initial 0 x,y reference and a space at the end of the code and it worked fine after that. Thanks for your time and hope you have a good one. Cheers!
– CanOr1
Nov 20 '18 at 15:03
Thanks for the help jdweng unfortunately the offset didn't respond as expected to the change. We did resolve the issue although don't understand why the change we made had the effect it did. We simply removed a line of code which gave an initial 0 x,y reference and a space at the end of the code and it worked fine after that. Thanks for your time and hope you have a good one. Cheers!
– CanOr1
Nov 20 '18 at 15:03
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53328501%2fx-y-line-offset-without-self-intersection%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53328501%2fx-y-line-offset-without-self-intersection%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
7S1cW,uBZxn,0ZKoh
Distance = sqrt(x^2 + y^2) You have a minus instead of a plus. #
– jdweng
Nov 15 '18 at 22:16
Thank you jdweng I will make that adjustment immediately and let you know how I fare. Hope you have a great week!
– CanOr1
Nov 19 '18 at 16:28
Thanks for the help jdweng unfortunately the offset didn't respond as expected to the change. We did resolve the issue although don't understand why the change we made had the effect it did. We simply removed a line of code which gave an initial 0 x,y reference and a space at the end of the code and it worked fine after that. Thanks for your time and hope you have a good one. Cheers!
– CanOr1
Nov 20 '18 at 15:03