Attempting to create a rudimentary calculator with C++; Getting a high amount of compilation errors
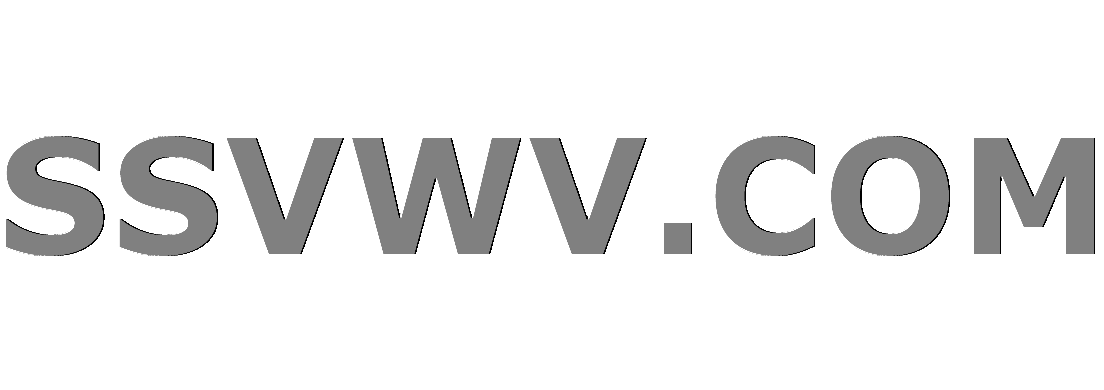
Multi tool use
I am incredibly new to programming and this is my first attempting at doing anything. I believe I had declared everything properly as well as the if statements, however I get multiple compilation errors that do not make any sense, as I cannot parse what they are trying to say is wrong with the code. I am incredibly confused and I want to learn what I am doing wrong.
Here is a list:
1. main.cpp: In function ‘int main()’:
main.cpp:28:19: error: expected primary-expression before ‘answer’
cin >> string answer;
^
2. main.cpp:30:19: error: ‘add’ was not declared in this scope
if (answer == add)
^
3. main.cpp:31:13: error: redeclaration of ‘int add’
int add(answer1,answer2);
^
4. main.cpp:30:19: note: ‘add’ previously declared here
if (answer == add)
^
5. main.cpp:31:13: error: ‘answer1’ was not declared in this scope
int add(answer1,answer2);
^
6. main.cpp:31:21: error: ‘answer2’ was not declared in this scope
int add(answer1,answer2);
^
7. main.cpp:31:28: error: expression list treated as compound expression in initializer [-fpermissive]
int add(answer1,answer2);
^
8. main.cpp:33:5: error: ‘else’ without a previous ‘if’
else if (answer == subtract)
^
9. main.cpp:33:24: error: ‘subtract’ was not declared in this scope
else if (answer == subtract)
^
10. main.cpp:34:18: error: redeclaration of ‘int subtract’
int subtract(answer1,answer2);
^
11. main.cpp:33:24: note: ‘subtract’ previously declared here
else if (answer == subtract)
^
12. main.cpp:34:18: error: ‘answer1’ was not declared in this scope
int subtract(answer1,answer2);
^
13. main.cpp:34:26: error: ‘answer2’ was not declared in this scope
int subtract(answer1,answer2);
^
14. main.cpp:34:33: error: expression list treated as compound expression in initializer [-fpermissive]
int subtract(answer1,answer2);
^
15. main.cpp:36:5: error: ‘else’ without a previous ‘if’
else if (answer == multiply)
^
16. main.cpp:36:24: error: ‘multiply’ was not declared in this scope
else if (answer == multiply)
^
17. main.cpp:37:18: error: redeclaration of ‘int multiply’
int multiply(answer1,answer2);
^
18. main.cpp:36:24: note: ‘multiply’ previously declared here
else if (answer == multiply)
^
19. main.cpp:37:18: error: ‘answer1’ was not declared in this scope
int multiply(answer1,answer2);
^
20. main.cpp:37:26: error: ‘answer2’ was not declared in this scope
int multiply(answer1,answer2);
^
21. main.cpp:37:33: error: expression list treated as compound expression in initializer [-fpermissive]
int multiply(answer1,answer2);
^
22. main.cpp:39:5: error: ‘else’ without a previous ‘if’
else if (answer == divide)
^
23. main.cpp:39:24: error: ‘divide’ was not declared in this scope
else if (answer == divide)
^
24. main.cpp:40:16: error: redeclaration of ‘int divide’
int divide(answer1,answer2);
^
25. main.cpp:39:24: note: ‘divide’ previously declared here
else if (answer == divide)
^
26. main.cpp:40:16: error: ‘answer1’ was not declared in this scope
int divide(answer1,answer2);
^
27. main.cpp:40:24: error: ‘answer2’ was not declared in this scope
int divide(answer1,answer2);
^
28. main.cpp:40:31: error: expression list treated as compound expression in initializer [-fpermissive]
int divide(answer1,answer2);
^
29. main.cpp:42:5: error: ‘else’ without a previous ‘if’
else if
^
30. main.cpp:43:5: error: expected ‘(’ before ‘cout’
cout << "Invalid Option!";
^
31. main.cpp: At global scope:
32. main.cpp:50:14: error: ‘a’ was not declared in this scope
int subtract(a,b);
^
33. main.cpp:50:16: error: ‘b’ was not declared in this scope
int subtract(a,b);
^
34. main.cpp:50:17: error: expression list treated as compound expression in initializer [-fpermissive]
int subtract(a,b);
^
35. main.cpp:51:1: error: expected unqualified-id before ‘{’ token
{
^
36. main.cpp:56:14: error: ‘a’ was not declared in this scope
int multiply(a,b);
^
37. main.cpp:56:16: error: ‘b’ was not declared in this scope
int multiply(a,b);
^
38. main.cpp:56:17: error: expression list treated as compound expression in initializer [-fpermissive]
int multiply(a,b);
^
39. main.cpp:57:1: error: expected unqualified-id before ‘{’ token
{
^
40. main.cpp:62:9: error: ‘a’ was not declared in this scope
int add(a,b);
^
41. main.cpp:62:11: error: ‘b’ was not declared in this scope
int add(a,b);
^
42. main.cpp:62:12: error: expression list treated as compound expression in initializer [-fpermissive]
int add(a,b);
^
43. main.cpp:63:1: error: expected unqualified-id before ‘{’ token
{
^
44. main.cpp:68:12: error: ‘a’ was not declared in this scope
int divide(a,b);
^
45. main.cpp:68:14: error: ‘b’ was not declared in this scope
int divide(a,b);
^
46. main.cpp:68:15: error: expression list treated as compound expression in initializer [-fpermissive]
int divide(a,b);
^
47. main.cpp:69:1: error: expected unqualified-id before ‘{’ token
{
^
Here is the code:
#include <iostream>
using namespace std;
int main()
{
int number1;
int number2;
string answer;
int result;
int a;
int b;
cout << "Enter first number" << endl;
cin >> number1;
cout << "Enter second number" << endl;
cin >> number2;
cout << "What would you like to do?" << endl << "Options: Add, substract,
multiply, divide" << endl;
cin >> string answer;
if (answer == add)
int add(answer1,answer2);
cout << result;
else if (answer == subtract)
int subtract(answer1,answer2);
cout << result;
else if (answer == multiply)
int multiply(answer1,answer2);
cout << result;
else if (answer == divide)
int divide(answer1,answer2);
cout << result;
else if
cout << "Invalid Option!";
return 0;
}
int subtract(a,b);
{
result = a-b;
return result;
}
int multiply(a,b);
{
cout << a*b;
}
int add(a,b);
{
cout << a+b;
}
int divide(a,b);
{
cout << a/b;
}
c++
|
show 1 more comment
I am incredibly new to programming and this is my first attempting at doing anything. I believe I had declared everything properly as well as the if statements, however I get multiple compilation errors that do not make any sense, as I cannot parse what they are trying to say is wrong with the code. I am incredibly confused and I want to learn what I am doing wrong.
Here is a list:
1. main.cpp: In function ‘int main()’:
main.cpp:28:19: error: expected primary-expression before ‘answer’
cin >> string answer;
^
2. main.cpp:30:19: error: ‘add’ was not declared in this scope
if (answer == add)
^
3. main.cpp:31:13: error: redeclaration of ‘int add’
int add(answer1,answer2);
^
4. main.cpp:30:19: note: ‘add’ previously declared here
if (answer == add)
^
5. main.cpp:31:13: error: ‘answer1’ was not declared in this scope
int add(answer1,answer2);
^
6. main.cpp:31:21: error: ‘answer2’ was not declared in this scope
int add(answer1,answer2);
^
7. main.cpp:31:28: error: expression list treated as compound expression in initializer [-fpermissive]
int add(answer1,answer2);
^
8. main.cpp:33:5: error: ‘else’ without a previous ‘if’
else if (answer == subtract)
^
9. main.cpp:33:24: error: ‘subtract’ was not declared in this scope
else if (answer == subtract)
^
10. main.cpp:34:18: error: redeclaration of ‘int subtract’
int subtract(answer1,answer2);
^
11. main.cpp:33:24: note: ‘subtract’ previously declared here
else if (answer == subtract)
^
12. main.cpp:34:18: error: ‘answer1’ was not declared in this scope
int subtract(answer1,answer2);
^
13. main.cpp:34:26: error: ‘answer2’ was not declared in this scope
int subtract(answer1,answer2);
^
14. main.cpp:34:33: error: expression list treated as compound expression in initializer [-fpermissive]
int subtract(answer1,answer2);
^
15. main.cpp:36:5: error: ‘else’ without a previous ‘if’
else if (answer == multiply)
^
16. main.cpp:36:24: error: ‘multiply’ was not declared in this scope
else if (answer == multiply)
^
17. main.cpp:37:18: error: redeclaration of ‘int multiply’
int multiply(answer1,answer2);
^
18. main.cpp:36:24: note: ‘multiply’ previously declared here
else if (answer == multiply)
^
19. main.cpp:37:18: error: ‘answer1’ was not declared in this scope
int multiply(answer1,answer2);
^
20. main.cpp:37:26: error: ‘answer2’ was not declared in this scope
int multiply(answer1,answer2);
^
21. main.cpp:37:33: error: expression list treated as compound expression in initializer [-fpermissive]
int multiply(answer1,answer2);
^
22. main.cpp:39:5: error: ‘else’ without a previous ‘if’
else if (answer == divide)
^
23. main.cpp:39:24: error: ‘divide’ was not declared in this scope
else if (answer == divide)
^
24. main.cpp:40:16: error: redeclaration of ‘int divide’
int divide(answer1,answer2);
^
25. main.cpp:39:24: note: ‘divide’ previously declared here
else if (answer == divide)
^
26. main.cpp:40:16: error: ‘answer1’ was not declared in this scope
int divide(answer1,answer2);
^
27. main.cpp:40:24: error: ‘answer2’ was not declared in this scope
int divide(answer1,answer2);
^
28. main.cpp:40:31: error: expression list treated as compound expression in initializer [-fpermissive]
int divide(answer1,answer2);
^
29. main.cpp:42:5: error: ‘else’ without a previous ‘if’
else if
^
30. main.cpp:43:5: error: expected ‘(’ before ‘cout’
cout << "Invalid Option!";
^
31. main.cpp: At global scope:
32. main.cpp:50:14: error: ‘a’ was not declared in this scope
int subtract(a,b);
^
33. main.cpp:50:16: error: ‘b’ was not declared in this scope
int subtract(a,b);
^
34. main.cpp:50:17: error: expression list treated as compound expression in initializer [-fpermissive]
int subtract(a,b);
^
35. main.cpp:51:1: error: expected unqualified-id before ‘{’ token
{
^
36. main.cpp:56:14: error: ‘a’ was not declared in this scope
int multiply(a,b);
^
37. main.cpp:56:16: error: ‘b’ was not declared in this scope
int multiply(a,b);
^
38. main.cpp:56:17: error: expression list treated as compound expression in initializer [-fpermissive]
int multiply(a,b);
^
39. main.cpp:57:1: error: expected unqualified-id before ‘{’ token
{
^
40. main.cpp:62:9: error: ‘a’ was not declared in this scope
int add(a,b);
^
41. main.cpp:62:11: error: ‘b’ was not declared in this scope
int add(a,b);
^
42. main.cpp:62:12: error: expression list treated as compound expression in initializer [-fpermissive]
int add(a,b);
^
43. main.cpp:63:1: error: expected unqualified-id before ‘{’ token
{
^
44. main.cpp:68:12: error: ‘a’ was not declared in this scope
int divide(a,b);
^
45. main.cpp:68:14: error: ‘b’ was not declared in this scope
int divide(a,b);
^
46. main.cpp:68:15: error: expression list treated as compound expression in initializer [-fpermissive]
int divide(a,b);
^
47. main.cpp:69:1: error: expected unqualified-id before ‘{’ token
{
^
Here is the code:
#include <iostream>
using namespace std;
int main()
{
int number1;
int number2;
string answer;
int result;
int a;
int b;
cout << "Enter first number" << endl;
cin >> number1;
cout << "Enter second number" << endl;
cin >> number2;
cout << "What would you like to do?" << endl << "Options: Add, substract,
multiply, divide" << endl;
cin >> string answer;
if (answer == add)
int add(answer1,answer2);
cout << result;
else if (answer == subtract)
int subtract(answer1,answer2);
cout << result;
else if (answer == multiply)
int multiply(answer1,answer2);
cout << result;
else if (answer == divide)
int divide(answer1,answer2);
cout << result;
else if
cout << "Invalid Option!";
return 0;
}
int subtract(a,b);
{
result = a-b;
return result;
}
int multiply(a,b);
{
cout << a*b;
}
int add(a,b);
{
cout << a+b;
}
int divide(a,b);
{
cout << a/b;
}
c++
6
There is a lot wrong here. Honestly I'd suggest scrapping this and rewriting from the beginning. After every 2-5 lines (whenever you finish an if or some output or a function), compile and run and make sure things are happening the way you expect. When you write up 50+ lines all at once and just hope everything will compile and work at this programming level, you're going to be spending a lot of time scrolling through comipler errors. And once you've dealt with those, things will still probably be broken with your logic.
– scohe001
Nov 15 '18 at 21:56
Good to know, will do. Thanks.
– Pseudoscorpion
Nov 15 '18 at 21:58
(answer == add)
: a key problem is thatadd
needs to be in quotes; otherwise, the compiler interprets it as an object (variable, function, whatever). Hence:error: ‘add’ was not declared in this scope
.
– Daniel R. Livingston
Nov 15 '18 at 22:02
Ohh, I see. That makes sense.
– Pseudoscorpion
Nov 15 '18 at 22:03
1
C++ is not a language to learn by trial and error. C++ has a dark side called Undefined Behaviour which means that serious bugs in your code don't always give compiler errors and throw exceptions. Learn by example and by reading documention.
– alter igel
Nov 16 '18 at 1:06
|
show 1 more comment
I am incredibly new to programming and this is my first attempting at doing anything. I believe I had declared everything properly as well as the if statements, however I get multiple compilation errors that do not make any sense, as I cannot parse what they are trying to say is wrong with the code. I am incredibly confused and I want to learn what I am doing wrong.
Here is a list:
1. main.cpp: In function ‘int main()’:
main.cpp:28:19: error: expected primary-expression before ‘answer’
cin >> string answer;
^
2. main.cpp:30:19: error: ‘add’ was not declared in this scope
if (answer == add)
^
3. main.cpp:31:13: error: redeclaration of ‘int add’
int add(answer1,answer2);
^
4. main.cpp:30:19: note: ‘add’ previously declared here
if (answer == add)
^
5. main.cpp:31:13: error: ‘answer1’ was not declared in this scope
int add(answer1,answer2);
^
6. main.cpp:31:21: error: ‘answer2’ was not declared in this scope
int add(answer1,answer2);
^
7. main.cpp:31:28: error: expression list treated as compound expression in initializer [-fpermissive]
int add(answer1,answer2);
^
8. main.cpp:33:5: error: ‘else’ without a previous ‘if’
else if (answer == subtract)
^
9. main.cpp:33:24: error: ‘subtract’ was not declared in this scope
else if (answer == subtract)
^
10. main.cpp:34:18: error: redeclaration of ‘int subtract’
int subtract(answer1,answer2);
^
11. main.cpp:33:24: note: ‘subtract’ previously declared here
else if (answer == subtract)
^
12. main.cpp:34:18: error: ‘answer1’ was not declared in this scope
int subtract(answer1,answer2);
^
13. main.cpp:34:26: error: ‘answer2’ was not declared in this scope
int subtract(answer1,answer2);
^
14. main.cpp:34:33: error: expression list treated as compound expression in initializer [-fpermissive]
int subtract(answer1,answer2);
^
15. main.cpp:36:5: error: ‘else’ without a previous ‘if’
else if (answer == multiply)
^
16. main.cpp:36:24: error: ‘multiply’ was not declared in this scope
else if (answer == multiply)
^
17. main.cpp:37:18: error: redeclaration of ‘int multiply’
int multiply(answer1,answer2);
^
18. main.cpp:36:24: note: ‘multiply’ previously declared here
else if (answer == multiply)
^
19. main.cpp:37:18: error: ‘answer1’ was not declared in this scope
int multiply(answer1,answer2);
^
20. main.cpp:37:26: error: ‘answer2’ was not declared in this scope
int multiply(answer1,answer2);
^
21. main.cpp:37:33: error: expression list treated as compound expression in initializer [-fpermissive]
int multiply(answer1,answer2);
^
22. main.cpp:39:5: error: ‘else’ without a previous ‘if’
else if (answer == divide)
^
23. main.cpp:39:24: error: ‘divide’ was not declared in this scope
else if (answer == divide)
^
24. main.cpp:40:16: error: redeclaration of ‘int divide’
int divide(answer1,answer2);
^
25. main.cpp:39:24: note: ‘divide’ previously declared here
else if (answer == divide)
^
26. main.cpp:40:16: error: ‘answer1’ was not declared in this scope
int divide(answer1,answer2);
^
27. main.cpp:40:24: error: ‘answer2’ was not declared in this scope
int divide(answer1,answer2);
^
28. main.cpp:40:31: error: expression list treated as compound expression in initializer [-fpermissive]
int divide(answer1,answer2);
^
29. main.cpp:42:5: error: ‘else’ without a previous ‘if’
else if
^
30. main.cpp:43:5: error: expected ‘(’ before ‘cout’
cout << "Invalid Option!";
^
31. main.cpp: At global scope:
32. main.cpp:50:14: error: ‘a’ was not declared in this scope
int subtract(a,b);
^
33. main.cpp:50:16: error: ‘b’ was not declared in this scope
int subtract(a,b);
^
34. main.cpp:50:17: error: expression list treated as compound expression in initializer [-fpermissive]
int subtract(a,b);
^
35. main.cpp:51:1: error: expected unqualified-id before ‘{’ token
{
^
36. main.cpp:56:14: error: ‘a’ was not declared in this scope
int multiply(a,b);
^
37. main.cpp:56:16: error: ‘b’ was not declared in this scope
int multiply(a,b);
^
38. main.cpp:56:17: error: expression list treated as compound expression in initializer [-fpermissive]
int multiply(a,b);
^
39. main.cpp:57:1: error: expected unqualified-id before ‘{’ token
{
^
40. main.cpp:62:9: error: ‘a’ was not declared in this scope
int add(a,b);
^
41. main.cpp:62:11: error: ‘b’ was not declared in this scope
int add(a,b);
^
42. main.cpp:62:12: error: expression list treated as compound expression in initializer [-fpermissive]
int add(a,b);
^
43. main.cpp:63:1: error: expected unqualified-id before ‘{’ token
{
^
44. main.cpp:68:12: error: ‘a’ was not declared in this scope
int divide(a,b);
^
45. main.cpp:68:14: error: ‘b’ was not declared in this scope
int divide(a,b);
^
46. main.cpp:68:15: error: expression list treated as compound expression in initializer [-fpermissive]
int divide(a,b);
^
47. main.cpp:69:1: error: expected unqualified-id before ‘{’ token
{
^
Here is the code:
#include <iostream>
using namespace std;
int main()
{
int number1;
int number2;
string answer;
int result;
int a;
int b;
cout << "Enter first number" << endl;
cin >> number1;
cout << "Enter second number" << endl;
cin >> number2;
cout << "What would you like to do?" << endl << "Options: Add, substract,
multiply, divide" << endl;
cin >> string answer;
if (answer == add)
int add(answer1,answer2);
cout << result;
else if (answer == subtract)
int subtract(answer1,answer2);
cout << result;
else if (answer == multiply)
int multiply(answer1,answer2);
cout << result;
else if (answer == divide)
int divide(answer1,answer2);
cout << result;
else if
cout << "Invalid Option!";
return 0;
}
int subtract(a,b);
{
result = a-b;
return result;
}
int multiply(a,b);
{
cout << a*b;
}
int add(a,b);
{
cout << a+b;
}
int divide(a,b);
{
cout << a/b;
}
c++
I am incredibly new to programming and this is my first attempting at doing anything. I believe I had declared everything properly as well as the if statements, however I get multiple compilation errors that do not make any sense, as I cannot parse what they are trying to say is wrong with the code. I am incredibly confused and I want to learn what I am doing wrong.
Here is a list:
1. main.cpp: In function ‘int main()’:
main.cpp:28:19: error: expected primary-expression before ‘answer’
cin >> string answer;
^
2. main.cpp:30:19: error: ‘add’ was not declared in this scope
if (answer == add)
^
3. main.cpp:31:13: error: redeclaration of ‘int add’
int add(answer1,answer2);
^
4. main.cpp:30:19: note: ‘add’ previously declared here
if (answer == add)
^
5. main.cpp:31:13: error: ‘answer1’ was not declared in this scope
int add(answer1,answer2);
^
6. main.cpp:31:21: error: ‘answer2’ was not declared in this scope
int add(answer1,answer2);
^
7. main.cpp:31:28: error: expression list treated as compound expression in initializer [-fpermissive]
int add(answer1,answer2);
^
8. main.cpp:33:5: error: ‘else’ without a previous ‘if’
else if (answer == subtract)
^
9. main.cpp:33:24: error: ‘subtract’ was not declared in this scope
else if (answer == subtract)
^
10. main.cpp:34:18: error: redeclaration of ‘int subtract’
int subtract(answer1,answer2);
^
11. main.cpp:33:24: note: ‘subtract’ previously declared here
else if (answer == subtract)
^
12. main.cpp:34:18: error: ‘answer1’ was not declared in this scope
int subtract(answer1,answer2);
^
13. main.cpp:34:26: error: ‘answer2’ was not declared in this scope
int subtract(answer1,answer2);
^
14. main.cpp:34:33: error: expression list treated as compound expression in initializer [-fpermissive]
int subtract(answer1,answer2);
^
15. main.cpp:36:5: error: ‘else’ without a previous ‘if’
else if (answer == multiply)
^
16. main.cpp:36:24: error: ‘multiply’ was not declared in this scope
else if (answer == multiply)
^
17. main.cpp:37:18: error: redeclaration of ‘int multiply’
int multiply(answer1,answer2);
^
18. main.cpp:36:24: note: ‘multiply’ previously declared here
else if (answer == multiply)
^
19. main.cpp:37:18: error: ‘answer1’ was not declared in this scope
int multiply(answer1,answer2);
^
20. main.cpp:37:26: error: ‘answer2’ was not declared in this scope
int multiply(answer1,answer2);
^
21. main.cpp:37:33: error: expression list treated as compound expression in initializer [-fpermissive]
int multiply(answer1,answer2);
^
22. main.cpp:39:5: error: ‘else’ without a previous ‘if’
else if (answer == divide)
^
23. main.cpp:39:24: error: ‘divide’ was not declared in this scope
else if (answer == divide)
^
24. main.cpp:40:16: error: redeclaration of ‘int divide’
int divide(answer1,answer2);
^
25. main.cpp:39:24: note: ‘divide’ previously declared here
else if (answer == divide)
^
26. main.cpp:40:16: error: ‘answer1’ was not declared in this scope
int divide(answer1,answer2);
^
27. main.cpp:40:24: error: ‘answer2’ was not declared in this scope
int divide(answer1,answer2);
^
28. main.cpp:40:31: error: expression list treated as compound expression in initializer [-fpermissive]
int divide(answer1,answer2);
^
29. main.cpp:42:5: error: ‘else’ without a previous ‘if’
else if
^
30. main.cpp:43:5: error: expected ‘(’ before ‘cout’
cout << "Invalid Option!";
^
31. main.cpp: At global scope:
32. main.cpp:50:14: error: ‘a’ was not declared in this scope
int subtract(a,b);
^
33. main.cpp:50:16: error: ‘b’ was not declared in this scope
int subtract(a,b);
^
34. main.cpp:50:17: error: expression list treated as compound expression in initializer [-fpermissive]
int subtract(a,b);
^
35. main.cpp:51:1: error: expected unqualified-id before ‘{’ token
{
^
36. main.cpp:56:14: error: ‘a’ was not declared in this scope
int multiply(a,b);
^
37. main.cpp:56:16: error: ‘b’ was not declared in this scope
int multiply(a,b);
^
38. main.cpp:56:17: error: expression list treated as compound expression in initializer [-fpermissive]
int multiply(a,b);
^
39. main.cpp:57:1: error: expected unqualified-id before ‘{’ token
{
^
40. main.cpp:62:9: error: ‘a’ was not declared in this scope
int add(a,b);
^
41. main.cpp:62:11: error: ‘b’ was not declared in this scope
int add(a,b);
^
42. main.cpp:62:12: error: expression list treated as compound expression in initializer [-fpermissive]
int add(a,b);
^
43. main.cpp:63:1: error: expected unqualified-id before ‘{’ token
{
^
44. main.cpp:68:12: error: ‘a’ was not declared in this scope
int divide(a,b);
^
45. main.cpp:68:14: error: ‘b’ was not declared in this scope
int divide(a,b);
^
46. main.cpp:68:15: error: expression list treated as compound expression in initializer [-fpermissive]
int divide(a,b);
^
47. main.cpp:69:1: error: expected unqualified-id before ‘{’ token
{
^
Here is the code:
#include <iostream>
using namespace std;
int main()
{
int number1;
int number2;
string answer;
int result;
int a;
int b;
cout << "Enter first number" << endl;
cin >> number1;
cout << "Enter second number" << endl;
cin >> number2;
cout << "What would you like to do?" << endl << "Options: Add, substract,
multiply, divide" << endl;
cin >> string answer;
if (answer == add)
int add(answer1,answer2);
cout << result;
else if (answer == subtract)
int subtract(answer1,answer2);
cout << result;
else if (answer == multiply)
int multiply(answer1,answer2);
cout << result;
else if (answer == divide)
int divide(answer1,answer2);
cout << result;
else if
cout << "Invalid Option!";
return 0;
}
int subtract(a,b);
{
result = a-b;
return result;
}
int multiply(a,b);
{
cout << a*b;
}
int add(a,b);
{
cout << a+b;
}
int divide(a,b);
{
cout << a/b;
}
c++
c++
edited Nov 15 '18 at 22:00
melpomene
61.8k54994
61.8k54994
asked Nov 15 '18 at 21:52
PseudoscorpionPseudoscorpion
9
9
6
There is a lot wrong here. Honestly I'd suggest scrapping this and rewriting from the beginning. After every 2-5 lines (whenever you finish an if or some output or a function), compile and run and make sure things are happening the way you expect. When you write up 50+ lines all at once and just hope everything will compile and work at this programming level, you're going to be spending a lot of time scrolling through comipler errors. And once you've dealt with those, things will still probably be broken with your logic.
– scohe001
Nov 15 '18 at 21:56
Good to know, will do. Thanks.
– Pseudoscorpion
Nov 15 '18 at 21:58
(answer == add)
: a key problem is thatadd
needs to be in quotes; otherwise, the compiler interprets it as an object (variable, function, whatever). Hence:error: ‘add’ was not declared in this scope
.
– Daniel R. Livingston
Nov 15 '18 at 22:02
Ohh, I see. That makes sense.
– Pseudoscorpion
Nov 15 '18 at 22:03
1
C++ is not a language to learn by trial and error. C++ has a dark side called Undefined Behaviour which means that serious bugs in your code don't always give compiler errors and throw exceptions. Learn by example and by reading documention.
– alter igel
Nov 16 '18 at 1:06
|
show 1 more comment
6
There is a lot wrong here. Honestly I'd suggest scrapping this and rewriting from the beginning. After every 2-5 lines (whenever you finish an if or some output or a function), compile and run and make sure things are happening the way you expect. When you write up 50+ lines all at once and just hope everything will compile and work at this programming level, you're going to be spending a lot of time scrolling through comipler errors. And once you've dealt with those, things will still probably be broken with your logic.
– scohe001
Nov 15 '18 at 21:56
Good to know, will do. Thanks.
– Pseudoscorpion
Nov 15 '18 at 21:58
(answer == add)
: a key problem is thatadd
needs to be in quotes; otherwise, the compiler interprets it as an object (variable, function, whatever). Hence:error: ‘add’ was not declared in this scope
.
– Daniel R. Livingston
Nov 15 '18 at 22:02
Ohh, I see. That makes sense.
– Pseudoscorpion
Nov 15 '18 at 22:03
1
C++ is not a language to learn by trial and error. C++ has a dark side called Undefined Behaviour which means that serious bugs in your code don't always give compiler errors and throw exceptions. Learn by example and by reading documention.
– alter igel
Nov 16 '18 at 1:06
6
6
There is a lot wrong here. Honestly I'd suggest scrapping this and rewriting from the beginning. After every 2-5 lines (whenever you finish an if or some output or a function), compile and run and make sure things are happening the way you expect. When you write up 50+ lines all at once and just hope everything will compile and work at this programming level, you're going to be spending a lot of time scrolling through comipler errors. And once you've dealt with those, things will still probably be broken with your logic.
– scohe001
Nov 15 '18 at 21:56
There is a lot wrong here. Honestly I'd suggest scrapping this and rewriting from the beginning. After every 2-5 lines (whenever you finish an if or some output or a function), compile and run and make sure things are happening the way you expect. When you write up 50+ lines all at once and just hope everything will compile and work at this programming level, you're going to be spending a lot of time scrolling through comipler errors. And once you've dealt with those, things will still probably be broken with your logic.
– scohe001
Nov 15 '18 at 21:56
Good to know, will do. Thanks.
– Pseudoscorpion
Nov 15 '18 at 21:58
Good to know, will do. Thanks.
– Pseudoscorpion
Nov 15 '18 at 21:58
(answer == add)
: a key problem is that add
needs to be in quotes; otherwise, the compiler interprets it as an object (variable, function, whatever). Hence: error: ‘add’ was not declared in this scope
.– Daniel R. Livingston
Nov 15 '18 at 22:02
(answer == add)
: a key problem is that add
needs to be in quotes; otherwise, the compiler interprets it as an object (variable, function, whatever). Hence: error: ‘add’ was not declared in this scope
.– Daniel R. Livingston
Nov 15 '18 at 22:02
Ohh, I see. That makes sense.
– Pseudoscorpion
Nov 15 '18 at 22:03
Ohh, I see. That makes sense.
– Pseudoscorpion
Nov 15 '18 at 22:03
1
1
C++ is not a language to learn by trial and error. C++ has a dark side called Undefined Behaviour which means that serious bugs in your code don't always give compiler errors and throw exceptions. Learn by example and by reading documention.
– alter igel
Nov 16 '18 at 1:06
C++ is not a language to learn by trial and error. C++ has a dark side called Undefined Behaviour which means that serious bugs in your code don't always give compiler errors and throw exceptions. Learn by example and by reading documention.
– alter igel
Nov 16 '18 at 1:06
|
show 1 more comment
3 Answers
3
active
oldest
votes
Instead of cin >> string answer;
use cin >> answer;
. You've a;ready got answer declared, so declaring it here is not necessary (and actually not legal). Other than that your add, subtract, multiply and divide functions are declared too soon. Add declarations or move those function definitions before main. You've got extra ; signs after between function declaration and it's body and you're missing types of functions arguments
int subtract(a,b); // delete ; here and add int (or better float) before a and b
{
result = a-b; // you need to declare result, add type before this line (int or float, float would be better
return result;
}
Also number1 and number2 should be at least floats (or doubles), otherwise you're gonna have a bad time with division operations.
Hit me up if you've got more questions about it.
Thank you so much!
– Pseudoscorpion
Nov 15 '18 at 22:14
Also I've noticed you don't have returns in most of your functions. If you're saying 'int somefunctionname()' you have to have return somewhere. Also you don't need type name before calling your function, but you should catch the result ('result = add(number1, number2)' instead of int add(number1,number2)'). If you want to have more than one line of code executed conditionally you need to surround them with braces. Last if in ie-else ladder is also not correct.
– vrtex
Nov 15 '18 at 22:21
add a comment |
cin >> string answer;
doesn't make sense. string answer
is not an expression, so you can't use it as the right-hand operand of the >>
operator.
You probably meant cin >> answer;
because string answer;
was already declared earlier in the function.
Also, you're missing #include <string>
.
(This answer only covers the first error. I usually only look at the first error message, fix the code, then compile again. In your case there seems to be a lot more wrong with the code. Sorry.)
Don't forget his returns (or lack thereof) in the functions...and the multiline string...and the missing condition in the lastelse if
...and the semicolons after all the function headers in the implementations....and all of the if conditions trying to use variables instead of string literals...I don't think it's even worth trying to fix this.
– scohe001
Nov 15 '18 at 21:58
@scohe001 The multiline string is probably a copy/paste error and not in the original code. The compiler doesn't seem to complain about it.
– melpomene
Nov 15 '18 at 21:59
Fair enough. Swap "and the multiline string..." for "and the way he's trying to call his functions from main..." then :p honestly there's something else that jumps out at me every time I look at it
– scohe001
Nov 15 '18 at 22:01
1
@Pseudoscorpion don't be sorry, everyone's code looks terribad when they first start out! I'm sure you'll look back in as soon as a few weeks and be able to find almost all of your mistakes. The trick is limiting the errors you have to deal with to a minimum by only writing a little at a time before compiling and checking it. I'm sure you'll get better--it just takes practice!!
– scohe001
Nov 15 '18 at 22:04
1
Yeah, you're right ^_^
– Pseudoscorpion
Nov 15 '18 at 22:06
|
show 2 more comments
Don't give up! Try starting from the beginning. Move slowly.
#include <iostream>
#include <string>
/*
This is regarded as bad practice...
From Python's `import this`:
Namespaces are one honking great idea -- let's do more of those!
*/
using namespace std;
// Define your function
int add(int a, int b);
int main()
{
int number1, number2, result;
string answer;
cout << "Enter first number" << endl;
cin >> number1;
cout << "Enter second number" << endl;
cin >> number2;
cout << "What would you like to do?" << endl << "Options: Add, substract, multiply, divide" << endl;
cin >> answer;
cout << "You chose: " << answer << endl;
// Can this handle lowercase "add"? If not, how do you fix it?
if (answer == "Add") {
result = add(number1,number2);
cout << number1 << " + " << number2 << " = " << result << endl;
}
// What about divide, multiply, etc.?
// That's an exercise left to the reader ;)
}
int add(int a, int b) {
return a + b;
}
This produces:
Enter first number
12
Enter second number
12
What would you like to do?
Options: Add, substract, multiply, divide
Add
You chose: Add
12 + 12 = 24
Let me know if there's anything confusing about this code compared to yours and I'll write up a response.
– Daniel R. Livingston
Nov 15 '18 at 22:31
I see what I was doing wrong, not using result and not working functions correctly. I managed to fix everything and do everything the program was intended to do ^_^
– Pseudoscorpion
Nov 15 '18 at 22:44
Awesome, great job! :) Keep at it! ..and make sure to upvote the answers of everyone that helped ;)
– Daniel R. Livingston
Nov 15 '18 at 22:45
Bonus challenge: consider the edge cases. What if someone passes in"abcd"
instead of a number intoresult1
? What will happen then? What if someone writes"AdD"
or"aDD"
or"ADD"
instead of"add"
? Extra extra bonus challenge: can you write a program that doesn't ask for the operation, but instead parses it automatically? i.e. input:5 / 4 -> divide(5,4)
?
– Daniel R. Livingston
Nov 15 '18 at 22:48
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53328399%2fattempting-to-create-a-rudimentary-calculator-with-c-getting-a-high-amount-of%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
Instead of cin >> string answer;
use cin >> answer;
. You've a;ready got answer declared, so declaring it here is not necessary (and actually not legal). Other than that your add, subtract, multiply and divide functions are declared too soon. Add declarations or move those function definitions before main. You've got extra ; signs after between function declaration and it's body and you're missing types of functions arguments
int subtract(a,b); // delete ; here and add int (or better float) before a and b
{
result = a-b; // you need to declare result, add type before this line (int or float, float would be better
return result;
}
Also number1 and number2 should be at least floats (or doubles), otherwise you're gonna have a bad time with division operations.
Hit me up if you've got more questions about it.
Thank you so much!
– Pseudoscorpion
Nov 15 '18 at 22:14
Also I've noticed you don't have returns in most of your functions. If you're saying 'int somefunctionname()' you have to have return somewhere. Also you don't need type name before calling your function, but you should catch the result ('result = add(number1, number2)' instead of int add(number1,number2)'). If you want to have more than one line of code executed conditionally you need to surround them with braces. Last if in ie-else ladder is also not correct.
– vrtex
Nov 15 '18 at 22:21
add a comment |
Instead of cin >> string answer;
use cin >> answer;
. You've a;ready got answer declared, so declaring it here is not necessary (and actually not legal). Other than that your add, subtract, multiply and divide functions are declared too soon. Add declarations or move those function definitions before main. You've got extra ; signs after between function declaration and it's body and you're missing types of functions arguments
int subtract(a,b); // delete ; here and add int (or better float) before a and b
{
result = a-b; // you need to declare result, add type before this line (int or float, float would be better
return result;
}
Also number1 and number2 should be at least floats (or doubles), otherwise you're gonna have a bad time with division operations.
Hit me up if you've got more questions about it.
Thank you so much!
– Pseudoscorpion
Nov 15 '18 at 22:14
Also I've noticed you don't have returns in most of your functions. If you're saying 'int somefunctionname()' you have to have return somewhere. Also you don't need type name before calling your function, but you should catch the result ('result = add(number1, number2)' instead of int add(number1,number2)'). If you want to have more than one line of code executed conditionally you need to surround them with braces. Last if in ie-else ladder is also not correct.
– vrtex
Nov 15 '18 at 22:21
add a comment |
Instead of cin >> string answer;
use cin >> answer;
. You've a;ready got answer declared, so declaring it here is not necessary (and actually not legal). Other than that your add, subtract, multiply and divide functions are declared too soon. Add declarations or move those function definitions before main. You've got extra ; signs after between function declaration and it's body and you're missing types of functions arguments
int subtract(a,b); // delete ; here and add int (or better float) before a and b
{
result = a-b; // you need to declare result, add type before this line (int or float, float would be better
return result;
}
Also number1 and number2 should be at least floats (or doubles), otherwise you're gonna have a bad time with division operations.
Hit me up if you've got more questions about it.
Instead of cin >> string answer;
use cin >> answer;
. You've a;ready got answer declared, so declaring it here is not necessary (and actually not legal). Other than that your add, subtract, multiply and divide functions are declared too soon. Add declarations or move those function definitions before main. You've got extra ; signs after between function declaration and it's body and you're missing types of functions arguments
int subtract(a,b); // delete ; here and add int (or better float) before a and b
{
result = a-b; // you need to declare result, add type before this line (int or float, float would be better
return result;
}
Also number1 and number2 should be at least floats (or doubles), otherwise you're gonna have a bad time with division operations.
Hit me up if you've got more questions about it.
answered Nov 15 '18 at 22:08


vrtexvrtex
1158
1158
Thank you so much!
– Pseudoscorpion
Nov 15 '18 at 22:14
Also I've noticed you don't have returns in most of your functions. If you're saying 'int somefunctionname()' you have to have return somewhere. Also you don't need type name before calling your function, but you should catch the result ('result = add(number1, number2)' instead of int add(number1,number2)'). If you want to have more than one line of code executed conditionally you need to surround them with braces. Last if in ie-else ladder is also not correct.
– vrtex
Nov 15 '18 at 22:21
add a comment |
Thank you so much!
– Pseudoscorpion
Nov 15 '18 at 22:14
Also I've noticed you don't have returns in most of your functions. If you're saying 'int somefunctionname()' you have to have return somewhere. Also you don't need type name before calling your function, but you should catch the result ('result = add(number1, number2)' instead of int add(number1,number2)'). If you want to have more than one line of code executed conditionally you need to surround them with braces. Last if in ie-else ladder is also not correct.
– vrtex
Nov 15 '18 at 22:21
Thank you so much!
– Pseudoscorpion
Nov 15 '18 at 22:14
Thank you so much!
– Pseudoscorpion
Nov 15 '18 at 22:14
Also I've noticed you don't have returns in most of your functions. If you're saying 'int somefunctionname()' you have to have return somewhere. Also you don't need type name before calling your function, but you should catch the result ('result = add(number1, number2)' instead of int add(number1,number2)'). If you want to have more than one line of code executed conditionally you need to surround them with braces. Last if in ie-else ladder is also not correct.
– vrtex
Nov 15 '18 at 22:21
Also I've noticed you don't have returns in most of your functions. If you're saying 'int somefunctionname()' you have to have return somewhere. Also you don't need type name before calling your function, but you should catch the result ('result = add(number1, number2)' instead of int add(number1,number2)'). If you want to have more than one line of code executed conditionally you need to surround them with braces. Last if in ie-else ladder is also not correct.
– vrtex
Nov 15 '18 at 22:21
add a comment |
cin >> string answer;
doesn't make sense. string answer
is not an expression, so you can't use it as the right-hand operand of the >>
operator.
You probably meant cin >> answer;
because string answer;
was already declared earlier in the function.
Also, you're missing #include <string>
.
(This answer only covers the first error. I usually only look at the first error message, fix the code, then compile again. In your case there seems to be a lot more wrong with the code. Sorry.)
Don't forget his returns (or lack thereof) in the functions...and the multiline string...and the missing condition in the lastelse if
...and the semicolons after all the function headers in the implementations....and all of the if conditions trying to use variables instead of string literals...I don't think it's even worth trying to fix this.
– scohe001
Nov 15 '18 at 21:58
@scohe001 The multiline string is probably a copy/paste error and not in the original code. The compiler doesn't seem to complain about it.
– melpomene
Nov 15 '18 at 21:59
Fair enough. Swap "and the multiline string..." for "and the way he's trying to call his functions from main..." then :p honestly there's something else that jumps out at me every time I look at it
– scohe001
Nov 15 '18 at 22:01
1
@Pseudoscorpion don't be sorry, everyone's code looks terribad when they first start out! I'm sure you'll look back in as soon as a few weeks and be able to find almost all of your mistakes. The trick is limiting the errors you have to deal with to a minimum by only writing a little at a time before compiling and checking it. I'm sure you'll get better--it just takes practice!!
– scohe001
Nov 15 '18 at 22:04
1
Yeah, you're right ^_^
– Pseudoscorpion
Nov 15 '18 at 22:06
|
show 2 more comments
cin >> string answer;
doesn't make sense. string answer
is not an expression, so you can't use it as the right-hand operand of the >>
operator.
You probably meant cin >> answer;
because string answer;
was already declared earlier in the function.
Also, you're missing #include <string>
.
(This answer only covers the first error. I usually only look at the first error message, fix the code, then compile again. In your case there seems to be a lot more wrong with the code. Sorry.)
Don't forget his returns (or lack thereof) in the functions...and the multiline string...and the missing condition in the lastelse if
...and the semicolons after all the function headers in the implementations....and all of the if conditions trying to use variables instead of string literals...I don't think it's even worth trying to fix this.
– scohe001
Nov 15 '18 at 21:58
@scohe001 The multiline string is probably a copy/paste error and not in the original code. The compiler doesn't seem to complain about it.
– melpomene
Nov 15 '18 at 21:59
Fair enough. Swap "and the multiline string..." for "and the way he's trying to call his functions from main..." then :p honestly there's something else that jumps out at me every time I look at it
– scohe001
Nov 15 '18 at 22:01
1
@Pseudoscorpion don't be sorry, everyone's code looks terribad when they first start out! I'm sure you'll look back in as soon as a few weeks and be able to find almost all of your mistakes. The trick is limiting the errors you have to deal with to a minimum by only writing a little at a time before compiling and checking it. I'm sure you'll get better--it just takes practice!!
– scohe001
Nov 15 '18 at 22:04
1
Yeah, you're right ^_^
– Pseudoscorpion
Nov 15 '18 at 22:06
|
show 2 more comments
cin >> string answer;
doesn't make sense. string answer
is not an expression, so you can't use it as the right-hand operand of the >>
operator.
You probably meant cin >> answer;
because string answer;
was already declared earlier in the function.
Also, you're missing #include <string>
.
(This answer only covers the first error. I usually only look at the first error message, fix the code, then compile again. In your case there seems to be a lot more wrong with the code. Sorry.)
cin >> string answer;
doesn't make sense. string answer
is not an expression, so you can't use it as the right-hand operand of the >>
operator.
You probably meant cin >> answer;
because string answer;
was already declared earlier in the function.
Also, you're missing #include <string>
.
(This answer only covers the first error. I usually only look at the first error message, fix the code, then compile again. In your case there seems to be a lot more wrong with the code. Sorry.)
answered Nov 15 '18 at 21:56
melpomenemelpomene
61.8k54994
61.8k54994
Don't forget his returns (or lack thereof) in the functions...and the multiline string...and the missing condition in the lastelse if
...and the semicolons after all the function headers in the implementations....and all of the if conditions trying to use variables instead of string literals...I don't think it's even worth trying to fix this.
– scohe001
Nov 15 '18 at 21:58
@scohe001 The multiline string is probably a copy/paste error and not in the original code. The compiler doesn't seem to complain about it.
– melpomene
Nov 15 '18 at 21:59
Fair enough. Swap "and the multiline string..." for "and the way he's trying to call his functions from main..." then :p honestly there's something else that jumps out at me every time I look at it
– scohe001
Nov 15 '18 at 22:01
1
@Pseudoscorpion don't be sorry, everyone's code looks terribad when they first start out! I'm sure you'll look back in as soon as a few weeks and be able to find almost all of your mistakes. The trick is limiting the errors you have to deal with to a minimum by only writing a little at a time before compiling and checking it. I'm sure you'll get better--it just takes practice!!
– scohe001
Nov 15 '18 at 22:04
1
Yeah, you're right ^_^
– Pseudoscorpion
Nov 15 '18 at 22:06
|
show 2 more comments
Don't forget his returns (or lack thereof) in the functions...and the multiline string...and the missing condition in the lastelse if
...and the semicolons after all the function headers in the implementations....and all of the if conditions trying to use variables instead of string literals...I don't think it's even worth trying to fix this.
– scohe001
Nov 15 '18 at 21:58
@scohe001 The multiline string is probably a copy/paste error and not in the original code. The compiler doesn't seem to complain about it.
– melpomene
Nov 15 '18 at 21:59
Fair enough. Swap "and the multiline string..." for "and the way he's trying to call his functions from main..." then :p honestly there's something else that jumps out at me every time I look at it
– scohe001
Nov 15 '18 at 22:01
1
@Pseudoscorpion don't be sorry, everyone's code looks terribad when they first start out! I'm sure you'll look back in as soon as a few weeks and be able to find almost all of your mistakes. The trick is limiting the errors you have to deal with to a minimum by only writing a little at a time before compiling and checking it. I'm sure you'll get better--it just takes practice!!
– scohe001
Nov 15 '18 at 22:04
1
Yeah, you're right ^_^
– Pseudoscorpion
Nov 15 '18 at 22:06
Don't forget his returns (or lack thereof) in the functions...and the multiline string...and the missing condition in the last
else if
...and the semicolons after all the function headers in the implementations....and all of the if conditions trying to use variables instead of string literals...I don't think it's even worth trying to fix this.– scohe001
Nov 15 '18 at 21:58
Don't forget his returns (or lack thereof) in the functions...and the multiline string...and the missing condition in the last
else if
...and the semicolons after all the function headers in the implementations....and all of the if conditions trying to use variables instead of string literals...I don't think it's even worth trying to fix this.– scohe001
Nov 15 '18 at 21:58
@scohe001 The multiline string is probably a copy/paste error and not in the original code. The compiler doesn't seem to complain about it.
– melpomene
Nov 15 '18 at 21:59
@scohe001 The multiline string is probably a copy/paste error and not in the original code. The compiler doesn't seem to complain about it.
– melpomene
Nov 15 '18 at 21:59
Fair enough. Swap "and the multiline string..." for "and the way he's trying to call his functions from main..." then :p honestly there's something else that jumps out at me every time I look at it
– scohe001
Nov 15 '18 at 22:01
Fair enough. Swap "and the multiline string..." for "and the way he's trying to call his functions from main..." then :p honestly there's something else that jumps out at me every time I look at it
– scohe001
Nov 15 '18 at 22:01
1
1
@Pseudoscorpion don't be sorry, everyone's code looks terribad when they first start out! I'm sure you'll look back in as soon as a few weeks and be able to find almost all of your mistakes. The trick is limiting the errors you have to deal with to a minimum by only writing a little at a time before compiling and checking it. I'm sure you'll get better--it just takes practice!!
– scohe001
Nov 15 '18 at 22:04
@Pseudoscorpion don't be sorry, everyone's code looks terribad when they first start out! I'm sure you'll look back in as soon as a few weeks and be able to find almost all of your mistakes. The trick is limiting the errors you have to deal with to a minimum by only writing a little at a time before compiling and checking it. I'm sure you'll get better--it just takes practice!!
– scohe001
Nov 15 '18 at 22:04
1
1
Yeah, you're right ^_^
– Pseudoscorpion
Nov 15 '18 at 22:06
Yeah, you're right ^_^
– Pseudoscorpion
Nov 15 '18 at 22:06
|
show 2 more comments
Don't give up! Try starting from the beginning. Move slowly.
#include <iostream>
#include <string>
/*
This is regarded as bad practice...
From Python's `import this`:
Namespaces are one honking great idea -- let's do more of those!
*/
using namespace std;
// Define your function
int add(int a, int b);
int main()
{
int number1, number2, result;
string answer;
cout << "Enter first number" << endl;
cin >> number1;
cout << "Enter second number" << endl;
cin >> number2;
cout << "What would you like to do?" << endl << "Options: Add, substract, multiply, divide" << endl;
cin >> answer;
cout << "You chose: " << answer << endl;
// Can this handle lowercase "add"? If not, how do you fix it?
if (answer == "Add") {
result = add(number1,number2);
cout << number1 << " + " << number2 << " = " << result << endl;
}
// What about divide, multiply, etc.?
// That's an exercise left to the reader ;)
}
int add(int a, int b) {
return a + b;
}
This produces:
Enter first number
12
Enter second number
12
What would you like to do?
Options: Add, substract, multiply, divide
Add
You chose: Add
12 + 12 = 24
Let me know if there's anything confusing about this code compared to yours and I'll write up a response.
– Daniel R. Livingston
Nov 15 '18 at 22:31
I see what I was doing wrong, not using result and not working functions correctly. I managed to fix everything and do everything the program was intended to do ^_^
– Pseudoscorpion
Nov 15 '18 at 22:44
Awesome, great job! :) Keep at it! ..and make sure to upvote the answers of everyone that helped ;)
– Daniel R. Livingston
Nov 15 '18 at 22:45
Bonus challenge: consider the edge cases. What if someone passes in"abcd"
instead of a number intoresult1
? What will happen then? What if someone writes"AdD"
or"aDD"
or"ADD"
instead of"add"
? Extra extra bonus challenge: can you write a program that doesn't ask for the operation, but instead parses it automatically? i.e. input:5 / 4 -> divide(5,4)
?
– Daniel R. Livingston
Nov 15 '18 at 22:48
add a comment |
Don't give up! Try starting from the beginning. Move slowly.
#include <iostream>
#include <string>
/*
This is regarded as bad practice...
From Python's `import this`:
Namespaces are one honking great idea -- let's do more of those!
*/
using namespace std;
// Define your function
int add(int a, int b);
int main()
{
int number1, number2, result;
string answer;
cout << "Enter first number" << endl;
cin >> number1;
cout << "Enter second number" << endl;
cin >> number2;
cout << "What would you like to do?" << endl << "Options: Add, substract, multiply, divide" << endl;
cin >> answer;
cout << "You chose: " << answer << endl;
// Can this handle lowercase "add"? If not, how do you fix it?
if (answer == "Add") {
result = add(number1,number2);
cout << number1 << " + " << number2 << " = " << result << endl;
}
// What about divide, multiply, etc.?
// That's an exercise left to the reader ;)
}
int add(int a, int b) {
return a + b;
}
This produces:
Enter first number
12
Enter second number
12
What would you like to do?
Options: Add, substract, multiply, divide
Add
You chose: Add
12 + 12 = 24
Let me know if there's anything confusing about this code compared to yours and I'll write up a response.
– Daniel R. Livingston
Nov 15 '18 at 22:31
I see what I was doing wrong, not using result and not working functions correctly. I managed to fix everything and do everything the program was intended to do ^_^
– Pseudoscorpion
Nov 15 '18 at 22:44
Awesome, great job! :) Keep at it! ..and make sure to upvote the answers of everyone that helped ;)
– Daniel R. Livingston
Nov 15 '18 at 22:45
Bonus challenge: consider the edge cases. What if someone passes in"abcd"
instead of a number intoresult1
? What will happen then? What if someone writes"AdD"
or"aDD"
or"ADD"
instead of"add"
? Extra extra bonus challenge: can you write a program that doesn't ask for the operation, but instead parses it automatically? i.e. input:5 / 4 -> divide(5,4)
?
– Daniel R. Livingston
Nov 15 '18 at 22:48
add a comment |
Don't give up! Try starting from the beginning. Move slowly.
#include <iostream>
#include <string>
/*
This is regarded as bad practice...
From Python's `import this`:
Namespaces are one honking great idea -- let's do more of those!
*/
using namespace std;
// Define your function
int add(int a, int b);
int main()
{
int number1, number2, result;
string answer;
cout << "Enter first number" << endl;
cin >> number1;
cout << "Enter second number" << endl;
cin >> number2;
cout << "What would you like to do?" << endl << "Options: Add, substract, multiply, divide" << endl;
cin >> answer;
cout << "You chose: " << answer << endl;
// Can this handle lowercase "add"? If not, how do you fix it?
if (answer == "Add") {
result = add(number1,number2);
cout << number1 << " + " << number2 << " = " << result << endl;
}
// What about divide, multiply, etc.?
// That's an exercise left to the reader ;)
}
int add(int a, int b) {
return a + b;
}
This produces:
Enter first number
12
Enter second number
12
What would you like to do?
Options: Add, substract, multiply, divide
Add
You chose: Add
12 + 12 = 24
Don't give up! Try starting from the beginning. Move slowly.
#include <iostream>
#include <string>
/*
This is regarded as bad practice...
From Python's `import this`:
Namespaces are one honking great idea -- let's do more of those!
*/
using namespace std;
// Define your function
int add(int a, int b);
int main()
{
int number1, number2, result;
string answer;
cout << "Enter first number" << endl;
cin >> number1;
cout << "Enter second number" << endl;
cin >> number2;
cout << "What would you like to do?" << endl << "Options: Add, substract, multiply, divide" << endl;
cin >> answer;
cout << "You chose: " << answer << endl;
// Can this handle lowercase "add"? If not, how do you fix it?
if (answer == "Add") {
result = add(number1,number2);
cout << number1 << " + " << number2 << " = " << result << endl;
}
// What about divide, multiply, etc.?
// That's an exercise left to the reader ;)
}
int add(int a, int b) {
return a + b;
}
This produces:
Enter first number
12
Enter second number
12
What would you like to do?
Options: Add, substract, multiply, divide
Add
You chose: Add
12 + 12 = 24
edited Nov 15 '18 at 22:20
answered Nov 15 '18 at 22:14


Daniel R. LivingstonDaniel R. Livingston
563725
563725
Let me know if there's anything confusing about this code compared to yours and I'll write up a response.
– Daniel R. Livingston
Nov 15 '18 at 22:31
I see what I was doing wrong, not using result and not working functions correctly. I managed to fix everything and do everything the program was intended to do ^_^
– Pseudoscorpion
Nov 15 '18 at 22:44
Awesome, great job! :) Keep at it! ..and make sure to upvote the answers of everyone that helped ;)
– Daniel R. Livingston
Nov 15 '18 at 22:45
Bonus challenge: consider the edge cases. What if someone passes in"abcd"
instead of a number intoresult1
? What will happen then? What if someone writes"AdD"
or"aDD"
or"ADD"
instead of"add"
? Extra extra bonus challenge: can you write a program that doesn't ask for the operation, but instead parses it automatically? i.e. input:5 / 4 -> divide(5,4)
?
– Daniel R. Livingston
Nov 15 '18 at 22:48
add a comment |
Let me know if there's anything confusing about this code compared to yours and I'll write up a response.
– Daniel R. Livingston
Nov 15 '18 at 22:31
I see what I was doing wrong, not using result and not working functions correctly. I managed to fix everything and do everything the program was intended to do ^_^
– Pseudoscorpion
Nov 15 '18 at 22:44
Awesome, great job! :) Keep at it! ..and make sure to upvote the answers of everyone that helped ;)
– Daniel R. Livingston
Nov 15 '18 at 22:45
Bonus challenge: consider the edge cases. What if someone passes in"abcd"
instead of a number intoresult1
? What will happen then? What if someone writes"AdD"
or"aDD"
or"ADD"
instead of"add"
? Extra extra bonus challenge: can you write a program that doesn't ask for the operation, but instead parses it automatically? i.e. input:5 / 4 -> divide(5,4)
?
– Daniel R. Livingston
Nov 15 '18 at 22:48
Let me know if there's anything confusing about this code compared to yours and I'll write up a response.
– Daniel R. Livingston
Nov 15 '18 at 22:31
Let me know if there's anything confusing about this code compared to yours and I'll write up a response.
– Daniel R. Livingston
Nov 15 '18 at 22:31
I see what I was doing wrong, not using result and not working functions correctly. I managed to fix everything and do everything the program was intended to do ^_^
– Pseudoscorpion
Nov 15 '18 at 22:44
I see what I was doing wrong, not using result and not working functions correctly. I managed to fix everything and do everything the program was intended to do ^_^
– Pseudoscorpion
Nov 15 '18 at 22:44
Awesome, great job! :) Keep at it! ..and make sure to upvote the answers of everyone that helped ;)
– Daniel R. Livingston
Nov 15 '18 at 22:45
Awesome, great job! :) Keep at it! ..and make sure to upvote the answers of everyone that helped ;)
– Daniel R. Livingston
Nov 15 '18 at 22:45
Bonus challenge: consider the edge cases. What if someone passes in
"abcd"
instead of a number into result1
? What will happen then? What if someone writes "AdD"
or "aDD"
or "ADD"
instead of "add"
? Extra extra bonus challenge: can you write a program that doesn't ask for the operation, but instead parses it automatically? i.e. input: 5 / 4 -> divide(5,4)
?– Daniel R. Livingston
Nov 15 '18 at 22:48
Bonus challenge: consider the edge cases. What if someone passes in
"abcd"
instead of a number into result1
? What will happen then? What if someone writes "AdD"
or "aDD"
or "ADD"
instead of "add"
? Extra extra bonus challenge: can you write a program that doesn't ask for the operation, but instead parses it automatically? i.e. input: 5 / 4 -> divide(5,4)
?– Daniel R. Livingston
Nov 15 '18 at 22:48
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53328399%2fattempting-to-create-a-rudimentary-calculator-with-c-getting-a-high-amount-of%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Fc DF L,JPY,47fMKSxrSE9w9eVpNfgyLXbXKXXiJaKP3zy0SIR XokTLzEt,V51E
6
There is a lot wrong here. Honestly I'd suggest scrapping this and rewriting from the beginning. After every 2-5 lines (whenever you finish an if or some output or a function), compile and run and make sure things are happening the way you expect. When you write up 50+ lines all at once and just hope everything will compile and work at this programming level, you're going to be spending a lot of time scrolling through comipler errors. And once you've dealt with those, things will still probably be broken with your logic.
– scohe001
Nov 15 '18 at 21:56
Good to know, will do. Thanks.
– Pseudoscorpion
Nov 15 '18 at 21:58
(answer == add)
: a key problem is thatadd
needs to be in quotes; otherwise, the compiler interprets it as an object (variable, function, whatever). Hence:error: ‘add’ was not declared in this scope
.– Daniel R. Livingston
Nov 15 '18 at 22:02
Ohh, I see. That makes sense.
– Pseudoscorpion
Nov 15 '18 at 22:03
1
C++ is not a language to learn by trial and error. C++ has a dark side called Undefined Behaviour which means that serious bugs in your code don't always give compiler errors and throw exceptions. Learn by example and by reading documention.
– alter igel
Nov 16 '18 at 1:06