Why print outside observe block is executed before print(count) inside observe block?
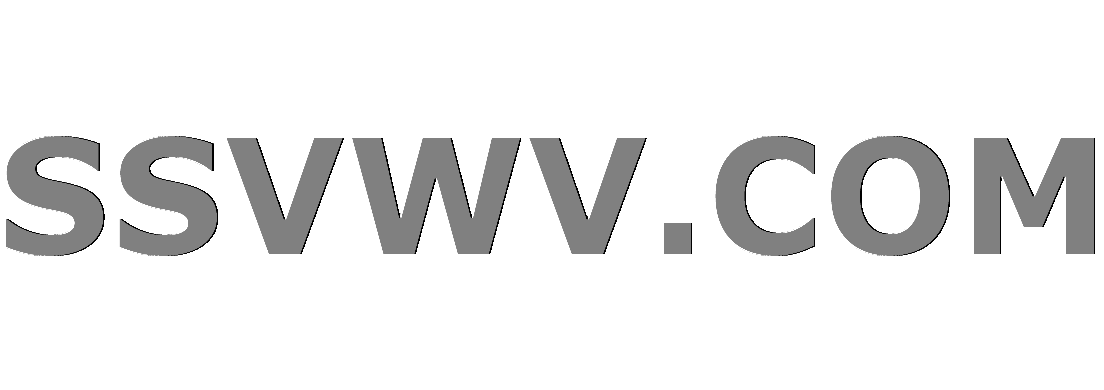
Multi tool use
func teacherExists(teacherName: String) -> Bool
{
var dataBaseRef2: DatabaseReference!
dataBaseRef2 = Database.database().reference()
let teachersTableRef = dataBaseRef2.child("teachers")
self.teachersList.removeAll()
teachersTableRef.observeSingleEvent(of: DataEventType.value, with: { (snapshot) in
// teachersTableRef.observe(.value)
//{
// snapshot in
let teachersNamesDictionary = snapshot.value as? [String: Any] ?? [:]
for(key, _) in teachersNamesDictionary
{
if let teacherDict = teachersNamesDictionary[key] as? [String: Any]
{
if let teacher = Teacher(dictionary: teacherDict)
{
//print(teacher.teacher_name)
self.teachersList.append(teacher.teacher_name)
}
}
}
print(self.teachersList.count)
})
print("Outside (self.teachersList)")
return false
}
ios swift firebase firebase-realtime-database
add a comment |
func teacherExists(teacherName: String) -> Bool
{
var dataBaseRef2: DatabaseReference!
dataBaseRef2 = Database.database().reference()
let teachersTableRef = dataBaseRef2.child("teachers")
self.teachersList.removeAll()
teachersTableRef.observeSingleEvent(of: DataEventType.value, with: { (snapshot) in
// teachersTableRef.observe(.value)
//{
// snapshot in
let teachersNamesDictionary = snapshot.value as? [String: Any] ?? [:]
for(key, _) in teachersNamesDictionary
{
if let teacherDict = teachersNamesDictionary[key] as? [String: Any]
{
if let teacher = Teacher(dictionary: teacherDict)
{
//print(teacher.teacher_name)
self.teachersList.append(teacher.teacher_name)
}
}
}
print(self.teachersList.count)
})
print("Outside (self.teachersList)")
return false
}
ios swift firebase firebase-realtime-database
add a comment |
func teacherExists(teacherName: String) -> Bool
{
var dataBaseRef2: DatabaseReference!
dataBaseRef2 = Database.database().reference()
let teachersTableRef = dataBaseRef2.child("teachers")
self.teachersList.removeAll()
teachersTableRef.observeSingleEvent(of: DataEventType.value, with: { (snapshot) in
// teachersTableRef.observe(.value)
//{
// snapshot in
let teachersNamesDictionary = snapshot.value as? [String: Any] ?? [:]
for(key, _) in teachersNamesDictionary
{
if let teacherDict = teachersNamesDictionary[key] as? [String: Any]
{
if let teacher = Teacher(dictionary: teacherDict)
{
//print(teacher.teacher_name)
self.teachersList.append(teacher.teacher_name)
}
}
}
print(self.teachersList.count)
})
print("Outside (self.teachersList)")
return false
}
ios swift firebase firebase-realtime-database
func teacherExists(teacherName: String) -> Bool
{
var dataBaseRef2: DatabaseReference!
dataBaseRef2 = Database.database().reference()
let teachersTableRef = dataBaseRef2.child("teachers")
self.teachersList.removeAll()
teachersTableRef.observeSingleEvent(of: DataEventType.value, with: { (snapshot) in
// teachersTableRef.observe(.value)
//{
// snapshot in
let teachersNamesDictionary = snapshot.value as? [String: Any] ?? [:]
for(key, _) in teachersNamesDictionary
{
if let teacherDict = teachersNamesDictionary[key] as? [String: Any]
{
if let teacher = Teacher(dictionary: teacherDict)
{
//print(teacher.teacher_name)
self.teachersList.append(teacher.teacher_name)
}
}
}
print(self.teachersList.count)
})
print("Outside (self.teachersList)")
return false
}
ios swift firebase firebase-realtime-database
ios swift firebase firebase-realtime-database
edited Nov 16 '18 at 3:43


Doug Stevenson
81.7k997115
81.7k997115
asked Nov 16 '18 at 3:32


Ashish BhatnagarAshish Bhatnagar
183
183
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
Because Firebase APIs are all asynchronous. It would be bad for your app if they blocked your code path, because that could cause your app to hang indefinitely.
observeSingleEvent
returns immediately, and the passed observer gets invoked some time later, whenever the data is finally ready. Execution continues on the next line, which prints to the console.
so how do I read all the data from firebase server and check for duplicate values?
– Ashish Bhatnagar
Nov 16 '18 at 3:55
or how can I suspend the execution of next statement until the data has been passed by firebase servers?
– Ashish Bhatnagar
Nov 16 '18 at 3:57
Generally speaking, you don't. You deal with the asynchronous APIs by adapting your code to them. If you want something to run after the observer runs, kick off that work inside the observer.
– Doug Stevenson
Nov 16 '18 at 4:30
add a comment |
getting error upon calling teacherExists function
let OKAction = UIAlertAction(title: "OK", style: .default, handler:
{
(action: UIAlertAction!) ->Void in
let textfield = alert.textFields![0] as UITextField
newTeacherName = textfield.text!.uppercased()
if !(newTeacherName.isEmpty)
{
//checking if teacher already exists using function teacherExists
let exists = self.teacherExists(teacherName: newTeacherName, completion:
if exists == true //if duplicate teacher is found
{
let alert = UIAlertController(title: "Duplicate Teacher", message: "Teacher (newTeacherName) has been added earlier", preferredStyle: .alert)
alert.addAction(UIAlertAction(title: "OK", style: .default, handler: nil))
self.present(alert, animated: true, completion: nil)
}
else
{
//add teacher to database here
let dict = ["teacher_name" : newTeacherName]
let newTeacher = Teacher(dictionary: dict)
let tableRef = self.dataBaseRef.child("teachers") //getting reference of node with name teachers
let recordRef = tableRef.childByAutoId() //creating a new record in teachers node
recordRef.setValue(newTeacher!.toDictionary())//adding data to new record in teachers node
}
}
})
help me with calling teacherExists function using completion:
– Ashish Bhatnagar
Nov 16 '18 at 5:26
add a comment |
You can use closure to callback after check for duplicate
func teacherExists(teacherName: String, completion: @escaping ((Bool) -> Void)) -> Void {
var dataBaseRef2: DatabaseReference!
dataBaseRef2 = Database.database().reference()
let teachersTableRef = dataBaseRef2.child("teachers")
self.teachersList.removeAll()
teachersTableRef.observeSingleEvent(of: DataEventType.value, with: { (snapshot) in
let teachersNamesDictionary = snapshot.value as? [String: Any] ?? [:]
for(key, _) in teachersNamesDictionary
{
if let teacherDict = teachersNamesDictionary[key] as? [String: Any]
{
if let teacher = Teacher(dictionary: teacherDict)
{
//print(teacher.teacher_name)
self.teachersList.append(teacher.teacher_name)
}
}
}
let exists = self.teachersList.contains(teacherName)
completion(exists)
})
}
And call function as below
teacherExists(teacherName: newTeacherName) { (exists) in
if exists {
// show alert
} else {
// add new teacher to db
}
}
Hope it help!
I am calling this method from an Alert OK button. But calling this function is giving error. I am not good at using closures.
– Ashish Bhatnagar
Nov 16 '18 at 5:15
awesome man....perfectly worked...this action is vey important for my app as I can't allow duplicate values in the database...you are a life saver...tons of thanks
– Ashish Bhatnagar
Nov 16 '18 at 7:14
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53331022%2fwhy-print-outside-observe-block-is-executed-before-printcount-inside-observe-b%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
Because Firebase APIs are all asynchronous. It would be bad for your app if they blocked your code path, because that could cause your app to hang indefinitely.
observeSingleEvent
returns immediately, and the passed observer gets invoked some time later, whenever the data is finally ready. Execution continues on the next line, which prints to the console.
so how do I read all the data from firebase server and check for duplicate values?
– Ashish Bhatnagar
Nov 16 '18 at 3:55
or how can I suspend the execution of next statement until the data has been passed by firebase servers?
– Ashish Bhatnagar
Nov 16 '18 at 3:57
Generally speaking, you don't. You deal with the asynchronous APIs by adapting your code to them. If you want something to run after the observer runs, kick off that work inside the observer.
– Doug Stevenson
Nov 16 '18 at 4:30
add a comment |
Because Firebase APIs are all asynchronous. It would be bad for your app if they blocked your code path, because that could cause your app to hang indefinitely.
observeSingleEvent
returns immediately, and the passed observer gets invoked some time later, whenever the data is finally ready. Execution continues on the next line, which prints to the console.
so how do I read all the data from firebase server and check for duplicate values?
– Ashish Bhatnagar
Nov 16 '18 at 3:55
or how can I suspend the execution of next statement until the data has been passed by firebase servers?
– Ashish Bhatnagar
Nov 16 '18 at 3:57
Generally speaking, you don't. You deal with the asynchronous APIs by adapting your code to them. If you want something to run after the observer runs, kick off that work inside the observer.
– Doug Stevenson
Nov 16 '18 at 4:30
add a comment |
Because Firebase APIs are all asynchronous. It would be bad for your app if they blocked your code path, because that could cause your app to hang indefinitely.
observeSingleEvent
returns immediately, and the passed observer gets invoked some time later, whenever the data is finally ready. Execution continues on the next line, which prints to the console.
Because Firebase APIs are all asynchronous. It would be bad for your app if they blocked your code path, because that could cause your app to hang indefinitely.
observeSingleEvent
returns immediately, and the passed observer gets invoked some time later, whenever the data is finally ready. Execution continues on the next line, which prints to the console.
answered Nov 16 '18 at 3:45


Doug StevensonDoug Stevenson
81.7k997115
81.7k997115
so how do I read all the data from firebase server and check for duplicate values?
– Ashish Bhatnagar
Nov 16 '18 at 3:55
or how can I suspend the execution of next statement until the data has been passed by firebase servers?
– Ashish Bhatnagar
Nov 16 '18 at 3:57
Generally speaking, you don't. You deal with the asynchronous APIs by adapting your code to them. If you want something to run after the observer runs, kick off that work inside the observer.
– Doug Stevenson
Nov 16 '18 at 4:30
add a comment |
so how do I read all the data from firebase server and check for duplicate values?
– Ashish Bhatnagar
Nov 16 '18 at 3:55
or how can I suspend the execution of next statement until the data has been passed by firebase servers?
– Ashish Bhatnagar
Nov 16 '18 at 3:57
Generally speaking, you don't. You deal with the asynchronous APIs by adapting your code to them. If you want something to run after the observer runs, kick off that work inside the observer.
– Doug Stevenson
Nov 16 '18 at 4:30
so how do I read all the data from firebase server and check for duplicate values?
– Ashish Bhatnagar
Nov 16 '18 at 3:55
so how do I read all the data from firebase server and check for duplicate values?
– Ashish Bhatnagar
Nov 16 '18 at 3:55
or how can I suspend the execution of next statement until the data has been passed by firebase servers?
– Ashish Bhatnagar
Nov 16 '18 at 3:57
or how can I suspend the execution of next statement until the data has been passed by firebase servers?
– Ashish Bhatnagar
Nov 16 '18 at 3:57
Generally speaking, you don't. You deal with the asynchronous APIs by adapting your code to them. If you want something to run after the observer runs, kick off that work inside the observer.
– Doug Stevenson
Nov 16 '18 at 4:30
Generally speaking, you don't. You deal with the asynchronous APIs by adapting your code to them. If you want something to run after the observer runs, kick off that work inside the observer.
– Doug Stevenson
Nov 16 '18 at 4:30
add a comment |
getting error upon calling teacherExists function
let OKAction = UIAlertAction(title: "OK", style: .default, handler:
{
(action: UIAlertAction!) ->Void in
let textfield = alert.textFields![0] as UITextField
newTeacherName = textfield.text!.uppercased()
if !(newTeacherName.isEmpty)
{
//checking if teacher already exists using function teacherExists
let exists = self.teacherExists(teacherName: newTeacherName, completion:
if exists == true //if duplicate teacher is found
{
let alert = UIAlertController(title: "Duplicate Teacher", message: "Teacher (newTeacherName) has been added earlier", preferredStyle: .alert)
alert.addAction(UIAlertAction(title: "OK", style: .default, handler: nil))
self.present(alert, animated: true, completion: nil)
}
else
{
//add teacher to database here
let dict = ["teacher_name" : newTeacherName]
let newTeacher = Teacher(dictionary: dict)
let tableRef = self.dataBaseRef.child("teachers") //getting reference of node with name teachers
let recordRef = tableRef.childByAutoId() //creating a new record in teachers node
recordRef.setValue(newTeacher!.toDictionary())//adding data to new record in teachers node
}
}
})
help me with calling teacherExists function using completion:
– Ashish Bhatnagar
Nov 16 '18 at 5:26
add a comment |
getting error upon calling teacherExists function
let OKAction = UIAlertAction(title: "OK", style: .default, handler:
{
(action: UIAlertAction!) ->Void in
let textfield = alert.textFields![0] as UITextField
newTeacherName = textfield.text!.uppercased()
if !(newTeacherName.isEmpty)
{
//checking if teacher already exists using function teacherExists
let exists = self.teacherExists(teacherName: newTeacherName, completion:
if exists == true //if duplicate teacher is found
{
let alert = UIAlertController(title: "Duplicate Teacher", message: "Teacher (newTeacherName) has been added earlier", preferredStyle: .alert)
alert.addAction(UIAlertAction(title: "OK", style: .default, handler: nil))
self.present(alert, animated: true, completion: nil)
}
else
{
//add teacher to database here
let dict = ["teacher_name" : newTeacherName]
let newTeacher = Teacher(dictionary: dict)
let tableRef = self.dataBaseRef.child("teachers") //getting reference of node with name teachers
let recordRef = tableRef.childByAutoId() //creating a new record in teachers node
recordRef.setValue(newTeacher!.toDictionary())//adding data to new record in teachers node
}
}
})
help me with calling teacherExists function using completion:
– Ashish Bhatnagar
Nov 16 '18 at 5:26
add a comment |
getting error upon calling teacherExists function
let OKAction = UIAlertAction(title: "OK", style: .default, handler:
{
(action: UIAlertAction!) ->Void in
let textfield = alert.textFields![0] as UITextField
newTeacherName = textfield.text!.uppercased()
if !(newTeacherName.isEmpty)
{
//checking if teacher already exists using function teacherExists
let exists = self.teacherExists(teacherName: newTeacherName, completion:
if exists == true //if duplicate teacher is found
{
let alert = UIAlertController(title: "Duplicate Teacher", message: "Teacher (newTeacherName) has been added earlier", preferredStyle: .alert)
alert.addAction(UIAlertAction(title: "OK", style: .default, handler: nil))
self.present(alert, animated: true, completion: nil)
}
else
{
//add teacher to database here
let dict = ["teacher_name" : newTeacherName]
let newTeacher = Teacher(dictionary: dict)
let tableRef = self.dataBaseRef.child("teachers") //getting reference of node with name teachers
let recordRef = tableRef.childByAutoId() //creating a new record in teachers node
recordRef.setValue(newTeacher!.toDictionary())//adding data to new record in teachers node
}
}
})
getting error upon calling teacherExists function
let OKAction = UIAlertAction(title: "OK", style: .default, handler:
{
(action: UIAlertAction!) ->Void in
let textfield = alert.textFields![0] as UITextField
newTeacherName = textfield.text!.uppercased()
if !(newTeacherName.isEmpty)
{
//checking if teacher already exists using function teacherExists
let exists = self.teacherExists(teacherName: newTeacherName, completion:
if exists == true //if duplicate teacher is found
{
let alert = UIAlertController(title: "Duplicate Teacher", message: "Teacher (newTeacherName) has been added earlier", preferredStyle: .alert)
alert.addAction(UIAlertAction(title: "OK", style: .default, handler: nil))
self.present(alert, animated: true, completion: nil)
}
else
{
//add teacher to database here
let dict = ["teacher_name" : newTeacherName]
let newTeacher = Teacher(dictionary: dict)
let tableRef = self.dataBaseRef.child("teachers") //getting reference of node with name teachers
let recordRef = tableRef.childByAutoId() //creating a new record in teachers node
recordRef.setValue(newTeacher!.toDictionary())//adding data to new record in teachers node
}
}
})
answered Nov 16 '18 at 5:20


Ashish BhatnagarAshish Bhatnagar
183
183
help me with calling teacherExists function using completion:
– Ashish Bhatnagar
Nov 16 '18 at 5:26
add a comment |
help me with calling teacherExists function using completion:
– Ashish Bhatnagar
Nov 16 '18 at 5:26
help me with calling teacherExists function using completion:
– Ashish Bhatnagar
Nov 16 '18 at 5:26
help me with calling teacherExists function using completion:
– Ashish Bhatnagar
Nov 16 '18 at 5:26
add a comment |
You can use closure to callback after check for duplicate
func teacherExists(teacherName: String, completion: @escaping ((Bool) -> Void)) -> Void {
var dataBaseRef2: DatabaseReference!
dataBaseRef2 = Database.database().reference()
let teachersTableRef = dataBaseRef2.child("teachers")
self.teachersList.removeAll()
teachersTableRef.observeSingleEvent(of: DataEventType.value, with: { (snapshot) in
let teachersNamesDictionary = snapshot.value as? [String: Any] ?? [:]
for(key, _) in teachersNamesDictionary
{
if let teacherDict = teachersNamesDictionary[key] as? [String: Any]
{
if let teacher = Teacher(dictionary: teacherDict)
{
//print(teacher.teacher_name)
self.teachersList.append(teacher.teacher_name)
}
}
}
let exists = self.teachersList.contains(teacherName)
completion(exists)
})
}
And call function as below
teacherExists(teacherName: newTeacherName) { (exists) in
if exists {
// show alert
} else {
// add new teacher to db
}
}
Hope it help!
I am calling this method from an Alert OK button. But calling this function is giving error. I am not good at using closures.
– Ashish Bhatnagar
Nov 16 '18 at 5:15
awesome man....perfectly worked...this action is vey important for my app as I can't allow duplicate values in the database...you are a life saver...tons of thanks
– Ashish Bhatnagar
Nov 16 '18 at 7:14
add a comment |
You can use closure to callback after check for duplicate
func teacherExists(teacherName: String, completion: @escaping ((Bool) -> Void)) -> Void {
var dataBaseRef2: DatabaseReference!
dataBaseRef2 = Database.database().reference()
let teachersTableRef = dataBaseRef2.child("teachers")
self.teachersList.removeAll()
teachersTableRef.observeSingleEvent(of: DataEventType.value, with: { (snapshot) in
let teachersNamesDictionary = snapshot.value as? [String: Any] ?? [:]
for(key, _) in teachersNamesDictionary
{
if let teacherDict = teachersNamesDictionary[key] as? [String: Any]
{
if let teacher = Teacher(dictionary: teacherDict)
{
//print(teacher.teacher_name)
self.teachersList.append(teacher.teacher_name)
}
}
}
let exists = self.teachersList.contains(teacherName)
completion(exists)
})
}
And call function as below
teacherExists(teacherName: newTeacherName) { (exists) in
if exists {
// show alert
} else {
// add new teacher to db
}
}
Hope it help!
I am calling this method from an Alert OK button. But calling this function is giving error. I am not good at using closures.
– Ashish Bhatnagar
Nov 16 '18 at 5:15
awesome man....perfectly worked...this action is vey important for my app as I can't allow duplicate values in the database...you are a life saver...tons of thanks
– Ashish Bhatnagar
Nov 16 '18 at 7:14
add a comment |
You can use closure to callback after check for duplicate
func teacherExists(teacherName: String, completion: @escaping ((Bool) -> Void)) -> Void {
var dataBaseRef2: DatabaseReference!
dataBaseRef2 = Database.database().reference()
let teachersTableRef = dataBaseRef2.child("teachers")
self.teachersList.removeAll()
teachersTableRef.observeSingleEvent(of: DataEventType.value, with: { (snapshot) in
let teachersNamesDictionary = snapshot.value as? [String: Any] ?? [:]
for(key, _) in teachersNamesDictionary
{
if let teacherDict = teachersNamesDictionary[key] as? [String: Any]
{
if let teacher = Teacher(dictionary: teacherDict)
{
//print(teacher.teacher_name)
self.teachersList.append(teacher.teacher_name)
}
}
}
let exists = self.teachersList.contains(teacherName)
completion(exists)
})
}
And call function as below
teacherExists(teacherName: newTeacherName) { (exists) in
if exists {
// show alert
} else {
// add new teacher to db
}
}
Hope it help!
You can use closure to callback after check for duplicate
func teacherExists(teacherName: String, completion: @escaping ((Bool) -> Void)) -> Void {
var dataBaseRef2: DatabaseReference!
dataBaseRef2 = Database.database().reference()
let teachersTableRef = dataBaseRef2.child("teachers")
self.teachersList.removeAll()
teachersTableRef.observeSingleEvent(of: DataEventType.value, with: { (snapshot) in
let teachersNamesDictionary = snapshot.value as? [String: Any] ?? [:]
for(key, _) in teachersNamesDictionary
{
if let teacherDict = teachersNamesDictionary[key] as? [String: Any]
{
if let teacher = Teacher(dictionary: teacherDict)
{
//print(teacher.teacher_name)
self.teachersList.append(teacher.teacher_name)
}
}
}
let exists = self.teachersList.contains(teacherName)
completion(exists)
})
}
And call function as below
teacherExists(teacherName: newTeacherName) { (exists) in
if exists {
// show alert
} else {
// add new teacher to db
}
}
Hope it help!
edited Nov 16 '18 at 6:03
answered Nov 16 '18 at 4:55


Thao NguyenThao Nguyen
12
12
I am calling this method from an Alert OK button. But calling this function is giving error. I am not good at using closures.
– Ashish Bhatnagar
Nov 16 '18 at 5:15
awesome man....perfectly worked...this action is vey important for my app as I can't allow duplicate values in the database...you are a life saver...tons of thanks
– Ashish Bhatnagar
Nov 16 '18 at 7:14
add a comment |
I am calling this method from an Alert OK button. But calling this function is giving error. I am not good at using closures.
– Ashish Bhatnagar
Nov 16 '18 at 5:15
awesome man....perfectly worked...this action is vey important for my app as I can't allow duplicate values in the database...you are a life saver...tons of thanks
– Ashish Bhatnagar
Nov 16 '18 at 7:14
I am calling this method from an Alert OK button. But calling this function is giving error. I am not good at using closures.
– Ashish Bhatnagar
Nov 16 '18 at 5:15
I am calling this method from an Alert OK button. But calling this function is giving error. I am not good at using closures.
– Ashish Bhatnagar
Nov 16 '18 at 5:15
awesome man....perfectly worked...this action is vey important for my app as I can't allow duplicate values in the database...you are a life saver...tons of thanks
– Ashish Bhatnagar
Nov 16 '18 at 7:14
awesome man....perfectly worked...this action is vey important for my app as I can't allow duplicate values in the database...you are a life saver...tons of thanks
– Ashish Bhatnagar
Nov 16 '18 at 7:14
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53331022%2fwhy-print-outside-observe-block-is-executed-before-printcount-inside-observe-b%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Od33bvlyrQYbtQYnc6,COka1vTOWqIpw,1K