Number of days between dates for two different periods in year gravity forms
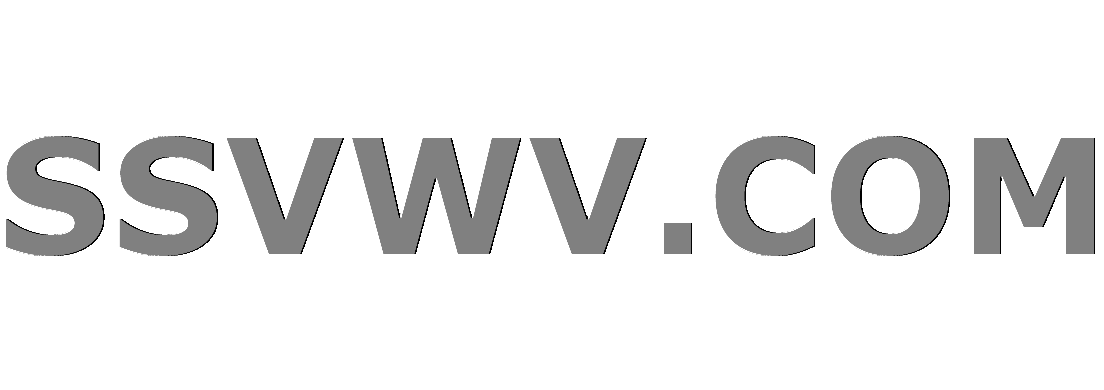
Multi tool use
I've been going on for 2 days try to find solution for gravity forms to find a numbers of days between dates but the problem here is i must find numbers for to different periods in year
year : 365 days
low season : 1 novembre ---> 1 jun
high season : 1 jun ---> 31 october
example : when i give 2 dates 01/10/2018 -----> 01/12/2019
resultat will be :
year : 365days
low season : 31 days
high season : 30
days
in the laste example i have 3 periods year and low season and high
i find solution to give numbers of days total with graity wiz
https://gravitywiz.com/calculate-number-of-days-between-two-dates/
so the answer for me is 426 days
what i can do ?..
<?php /**
* Gravity Wiz // Calculate Number of Days Between Two Gravity Form Date Fields
*
* Allows you to calculated the number of days between two Gravity Form date fields and populate that number into a
* field on your Gravity Form.
*
* @version 1.1
* @author David Smith <david@gravitywiz.com>
* @license GPL-2.0+
* @link http://gravitywiz.com/calculate-number-of-days-between-two-dates/
* @copyright 2013 Gravity Wiz
*/ class GWDayCount {
private static $script_output;
function __construct( $args ) {
extract( wp_parse_args( $args, array(
'form_id' => false,
'start_field_id' => false,
'end_field_id' => false,
'count_field_id' => false,
'include_end_date' => true,
) ) );
$this->form_id = $form_id;
$this->start_field_id = $start_field_id;
$this->end_field_id = $end_field_id;
$this->count_field_id = $count_field_id;
$this->count_adjust = $include_end_date ? 1 : 0;
add_filter( "gform_pre_render_{$form_id}", array( &$this, 'load_form_script') );
add_action( "gform_pre_submission_{$form_id}", array( &$this, 'override_submitted_value') );
}
function load_form_script( $form ) {
// workaround to make this work for < 1.7
$this->form = $form;
add_filter( 'gform_init_scripts_footer', array( &$this, 'add_init_script' ) );
if( self::$script_output )
return $form;
?>
<script type="text/javascript">
(function($){
window.gwdc = function( options ) {
this.options = options;
this.startDateInput = $( '#input_' + this.options.formId + '_' + this.options.startFieldId );
this.endDateInput = $( '#input_' + this.options.formId + '_' + this.options.endFieldId );
this.countInput = $( '#input_' + this.options.formId + '_' + this.options.countFieldId );
this.init = function() {
var gwdc = this;
// add data for "format" for parsing date
gwdc.startDateInput.data( 'format', this.options.startDateFormat );
gwdc.endDateInput.data( 'format', this.options.endDateFormat );
gwdc.populateDayCount();
gwdc.startDateInput.change( function() {
gwdc.populateDayCount();
} );
gwdc.endDateInput.change( function() {
gwdc.populateDayCount();
} );
$( '#ui-datepicker-div' ).hide();
}
this.getDayCount = function() {
var startDate = this.parseDate( this.startDateInput.val(), this.startDateInput.data('format') )
var endDate = this.parseDate( this.endDateInput.val(), this.endDateInput.data('format') );
var dayCount = 0;
if( !this.isValidDate( startDate ) || !this.isValidDate( endDate ) )
return '';
if( startDate > endDate ) {
return 0;
} else {
var diff = endDate - startDate;
dayCount = diff / ( 60 * 60 * 24 * 1000 ); // secs * mins * hours * milliseconds
dayCount = Math.round( dayCount ) + this.options.countAdjust;
return dayCount;
}
}
this.parseDate = function( value, format ) {
if( !value )
return false;
format = format.split('_');
var dateFormat = format[0];
var separators = { slash: '/', dash: '-', dot: '.' };
var separator = format.length > 1 ? separators[format[1]] : separators.slash;
var dateArr = value.split(separator);
switch( dateFormat ) {
case 'mdy':
return new Date( dateArr[2], dateArr[0] - 1, dateArr[1] );
case 'dmy':
return new Date( dateArr[2], dateArr[1] - 1, dateArr[0] );
case 'ymd':
return new Date( dateArr[0], dateArr[1] - 1, dateArr[2] );
}
return false;
}
this.populateDayCount = function() {
this.countInput.val( this.getDayCount() ).change();
}
this.isValidDate = function( date ) {
return !isNaN( Date.parse( date ) );
}
this.init();
}
})(jQuery);
</script>
<?php
self::$script_output = true;
return $form;
}
function add_init_script( $return ) {
$start_field_format = false;
$end_field_format = false;
foreach( $this->form['fields'] as &$field ) {
if( $field['id'] == $this->start_field_id )
$start_field_format = $field['dateFormat'] ? $field['dateFormat'] : 'mdy';
if( $field['id'] == $this->end_field_id )
$end_field_format = $field['dateFormat'] ? $field['dateFormat'] : 'mdy';
}
$script = "new gwdc({
formId: {$this->form['id']},
startFieldId: {$this->start_field_id},
startDateFormat: '$start_field_format',
endFieldId: {$this->end_field_id},
endDateFormat: '$end_field_format',
countFieldId: {$this->count_field_id},
countAdjust: {$this->count_adjust}
});";
$slug = implode( '_', array( 'gw_display_count', $this->start_field_id, $this->end_field_id, $this->count_field_id ) );
GFFormDisplay::add_init_script( $this->form['id'], $slug, GFFormDisplay::ON_PAGE_RENDER, $script );
// remove filter so init script is not output on subsequent forms
remove_filter( 'gform_init_scripts_footer', array( &$this, 'add_init_script' ) );
return $return;
}
function override_submitted_value( $form ) {
$start_date = false;
$end_date = false;
foreach( $form['fields'] as &$field ) {
if( $field['id'] == $this->start_field_id )
$start_date = self::parse_field_date( $field );
if( $field['id'] == $this->end_field_id )
$end_date = self::parse_field_date( $field );
}
if( $start_date > $end_date ) {
$day_count = 0;
} else {
$diff = $end_date - $start_date;
$day_count = $diff / ( 60 * 60 * 24 ); // secs * mins * hours
$day_count = round( $day_count ) + $this->count_adjust;
}
$_POST["input_{$this->count_field_id}"] = $day_count;
}
static function parse_field_date( $field ) {
$date_value = rgpost("input_{$field['id']}");
$date_format = empty( $field['dateFormat'] ) ? 'mdy' : esc_attr( $field['dateFormat'] );
$date_info = GFCommon::parse_date( $date_value, $date_format );
if( empty( $date_info ) )
return false;
return strtotime( "{$date_info['year']}-{$date_info['month']}-{$date_info['day']}" );
}
}
# Configuration
new GWDayCount( array(
'form_id' => 1,
'start_field_id' => 1,
'end_field_id' => 2,
'count_field_id' => 3 ) );
new GWDayCount( array(
'form_id' => 1,
'start_field_id' => 1,
'end_field_id' => 2,
'count_field_id' => 16 ) );
new GWDayCount( array(
'form_id' => 1,
'start_field_id' => 1,
'end_field_id' => 2,
'count_field_id' => 15 ) );
javascript jquery wordpress gravity-forms-plugin gravityforms
add a comment |
I've been going on for 2 days try to find solution for gravity forms to find a numbers of days between dates but the problem here is i must find numbers for to different periods in year
year : 365 days
low season : 1 novembre ---> 1 jun
high season : 1 jun ---> 31 october
example : when i give 2 dates 01/10/2018 -----> 01/12/2019
resultat will be :
year : 365days
low season : 31 days
high season : 30
days
in the laste example i have 3 periods year and low season and high
i find solution to give numbers of days total with graity wiz
https://gravitywiz.com/calculate-number-of-days-between-two-dates/
so the answer for me is 426 days
what i can do ?..
<?php /**
* Gravity Wiz // Calculate Number of Days Between Two Gravity Form Date Fields
*
* Allows you to calculated the number of days between two Gravity Form date fields and populate that number into a
* field on your Gravity Form.
*
* @version 1.1
* @author David Smith <david@gravitywiz.com>
* @license GPL-2.0+
* @link http://gravitywiz.com/calculate-number-of-days-between-two-dates/
* @copyright 2013 Gravity Wiz
*/ class GWDayCount {
private static $script_output;
function __construct( $args ) {
extract( wp_parse_args( $args, array(
'form_id' => false,
'start_field_id' => false,
'end_field_id' => false,
'count_field_id' => false,
'include_end_date' => true,
) ) );
$this->form_id = $form_id;
$this->start_field_id = $start_field_id;
$this->end_field_id = $end_field_id;
$this->count_field_id = $count_field_id;
$this->count_adjust = $include_end_date ? 1 : 0;
add_filter( "gform_pre_render_{$form_id}", array( &$this, 'load_form_script') );
add_action( "gform_pre_submission_{$form_id}", array( &$this, 'override_submitted_value') );
}
function load_form_script( $form ) {
// workaround to make this work for < 1.7
$this->form = $form;
add_filter( 'gform_init_scripts_footer', array( &$this, 'add_init_script' ) );
if( self::$script_output )
return $form;
?>
<script type="text/javascript">
(function($){
window.gwdc = function( options ) {
this.options = options;
this.startDateInput = $( '#input_' + this.options.formId + '_' + this.options.startFieldId );
this.endDateInput = $( '#input_' + this.options.formId + '_' + this.options.endFieldId );
this.countInput = $( '#input_' + this.options.formId + '_' + this.options.countFieldId );
this.init = function() {
var gwdc = this;
// add data for "format" for parsing date
gwdc.startDateInput.data( 'format', this.options.startDateFormat );
gwdc.endDateInput.data( 'format', this.options.endDateFormat );
gwdc.populateDayCount();
gwdc.startDateInput.change( function() {
gwdc.populateDayCount();
} );
gwdc.endDateInput.change( function() {
gwdc.populateDayCount();
} );
$( '#ui-datepicker-div' ).hide();
}
this.getDayCount = function() {
var startDate = this.parseDate( this.startDateInput.val(), this.startDateInput.data('format') )
var endDate = this.parseDate( this.endDateInput.val(), this.endDateInput.data('format') );
var dayCount = 0;
if( !this.isValidDate( startDate ) || !this.isValidDate( endDate ) )
return '';
if( startDate > endDate ) {
return 0;
} else {
var diff = endDate - startDate;
dayCount = diff / ( 60 * 60 * 24 * 1000 ); // secs * mins * hours * milliseconds
dayCount = Math.round( dayCount ) + this.options.countAdjust;
return dayCount;
}
}
this.parseDate = function( value, format ) {
if( !value )
return false;
format = format.split('_');
var dateFormat = format[0];
var separators = { slash: '/', dash: '-', dot: '.' };
var separator = format.length > 1 ? separators[format[1]] : separators.slash;
var dateArr = value.split(separator);
switch( dateFormat ) {
case 'mdy':
return new Date( dateArr[2], dateArr[0] - 1, dateArr[1] );
case 'dmy':
return new Date( dateArr[2], dateArr[1] - 1, dateArr[0] );
case 'ymd':
return new Date( dateArr[0], dateArr[1] - 1, dateArr[2] );
}
return false;
}
this.populateDayCount = function() {
this.countInput.val( this.getDayCount() ).change();
}
this.isValidDate = function( date ) {
return !isNaN( Date.parse( date ) );
}
this.init();
}
})(jQuery);
</script>
<?php
self::$script_output = true;
return $form;
}
function add_init_script( $return ) {
$start_field_format = false;
$end_field_format = false;
foreach( $this->form['fields'] as &$field ) {
if( $field['id'] == $this->start_field_id )
$start_field_format = $field['dateFormat'] ? $field['dateFormat'] : 'mdy';
if( $field['id'] == $this->end_field_id )
$end_field_format = $field['dateFormat'] ? $field['dateFormat'] : 'mdy';
}
$script = "new gwdc({
formId: {$this->form['id']},
startFieldId: {$this->start_field_id},
startDateFormat: '$start_field_format',
endFieldId: {$this->end_field_id},
endDateFormat: '$end_field_format',
countFieldId: {$this->count_field_id},
countAdjust: {$this->count_adjust}
});";
$slug = implode( '_', array( 'gw_display_count', $this->start_field_id, $this->end_field_id, $this->count_field_id ) );
GFFormDisplay::add_init_script( $this->form['id'], $slug, GFFormDisplay::ON_PAGE_RENDER, $script );
// remove filter so init script is not output on subsequent forms
remove_filter( 'gform_init_scripts_footer', array( &$this, 'add_init_script' ) );
return $return;
}
function override_submitted_value( $form ) {
$start_date = false;
$end_date = false;
foreach( $form['fields'] as &$field ) {
if( $field['id'] == $this->start_field_id )
$start_date = self::parse_field_date( $field );
if( $field['id'] == $this->end_field_id )
$end_date = self::parse_field_date( $field );
}
if( $start_date > $end_date ) {
$day_count = 0;
} else {
$diff = $end_date - $start_date;
$day_count = $diff / ( 60 * 60 * 24 ); // secs * mins * hours
$day_count = round( $day_count ) + $this->count_adjust;
}
$_POST["input_{$this->count_field_id}"] = $day_count;
}
static function parse_field_date( $field ) {
$date_value = rgpost("input_{$field['id']}");
$date_format = empty( $field['dateFormat'] ) ? 'mdy' : esc_attr( $field['dateFormat'] );
$date_info = GFCommon::parse_date( $date_value, $date_format );
if( empty( $date_info ) )
return false;
return strtotime( "{$date_info['year']}-{$date_info['month']}-{$date_info['day']}" );
}
}
# Configuration
new GWDayCount( array(
'form_id' => 1,
'start_field_id' => 1,
'end_field_id' => 2,
'count_field_id' => 3 ) );
new GWDayCount( array(
'form_id' => 1,
'start_field_id' => 1,
'end_field_id' => 2,
'count_field_id' => 16 ) );
new GWDayCount( array(
'form_id' => 1,
'start_field_id' => 1,
'end_field_id' => 2,
'count_field_id' => 15 ) );
javascript jquery wordpress gravity-forms-plugin gravityforms
add a comment |
I've been going on for 2 days try to find solution for gravity forms to find a numbers of days between dates but the problem here is i must find numbers for to different periods in year
year : 365 days
low season : 1 novembre ---> 1 jun
high season : 1 jun ---> 31 october
example : when i give 2 dates 01/10/2018 -----> 01/12/2019
resultat will be :
year : 365days
low season : 31 days
high season : 30
days
in the laste example i have 3 periods year and low season and high
i find solution to give numbers of days total with graity wiz
https://gravitywiz.com/calculate-number-of-days-between-two-dates/
so the answer for me is 426 days
what i can do ?..
<?php /**
* Gravity Wiz // Calculate Number of Days Between Two Gravity Form Date Fields
*
* Allows you to calculated the number of days between two Gravity Form date fields and populate that number into a
* field on your Gravity Form.
*
* @version 1.1
* @author David Smith <david@gravitywiz.com>
* @license GPL-2.0+
* @link http://gravitywiz.com/calculate-number-of-days-between-two-dates/
* @copyright 2013 Gravity Wiz
*/ class GWDayCount {
private static $script_output;
function __construct( $args ) {
extract( wp_parse_args( $args, array(
'form_id' => false,
'start_field_id' => false,
'end_field_id' => false,
'count_field_id' => false,
'include_end_date' => true,
) ) );
$this->form_id = $form_id;
$this->start_field_id = $start_field_id;
$this->end_field_id = $end_field_id;
$this->count_field_id = $count_field_id;
$this->count_adjust = $include_end_date ? 1 : 0;
add_filter( "gform_pre_render_{$form_id}", array( &$this, 'load_form_script') );
add_action( "gform_pre_submission_{$form_id}", array( &$this, 'override_submitted_value') );
}
function load_form_script( $form ) {
// workaround to make this work for < 1.7
$this->form = $form;
add_filter( 'gform_init_scripts_footer', array( &$this, 'add_init_script' ) );
if( self::$script_output )
return $form;
?>
<script type="text/javascript">
(function($){
window.gwdc = function( options ) {
this.options = options;
this.startDateInput = $( '#input_' + this.options.formId + '_' + this.options.startFieldId );
this.endDateInput = $( '#input_' + this.options.formId + '_' + this.options.endFieldId );
this.countInput = $( '#input_' + this.options.formId + '_' + this.options.countFieldId );
this.init = function() {
var gwdc = this;
// add data for "format" for parsing date
gwdc.startDateInput.data( 'format', this.options.startDateFormat );
gwdc.endDateInput.data( 'format', this.options.endDateFormat );
gwdc.populateDayCount();
gwdc.startDateInput.change( function() {
gwdc.populateDayCount();
} );
gwdc.endDateInput.change( function() {
gwdc.populateDayCount();
} );
$( '#ui-datepicker-div' ).hide();
}
this.getDayCount = function() {
var startDate = this.parseDate( this.startDateInput.val(), this.startDateInput.data('format') )
var endDate = this.parseDate( this.endDateInput.val(), this.endDateInput.data('format') );
var dayCount = 0;
if( !this.isValidDate( startDate ) || !this.isValidDate( endDate ) )
return '';
if( startDate > endDate ) {
return 0;
} else {
var diff = endDate - startDate;
dayCount = diff / ( 60 * 60 * 24 * 1000 ); // secs * mins * hours * milliseconds
dayCount = Math.round( dayCount ) + this.options.countAdjust;
return dayCount;
}
}
this.parseDate = function( value, format ) {
if( !value )
return false;
format = format.split('_');
var dateFormat = format[0];
var separators = { slash: '/', dash: '-', dot: '.' };
var separator = format.length > 1 ? separators[format[1]] : separators.slash;
var dateArr = value.split(separator);
switch( dateFormat ) {
case 'mdy':
return new Date( dateArr[2], dateArr[0] - 1, dateArr[1] );
case 'dmy':
return new Date( dateArr[2], dateArr[1] - 1, dateArr[0] );
case 'ymd':
return new Date( dateArr[0], dateArr[1] - 1, dateArr[2] );
}
return false;
}
this.populateDayCount = function() {
this.countInput.val( this.getDayCount() ).change();
}
this.isValidDate = function( date ) {
return !isNaN( Date.parse( date ) );
}
this.init();
}
})(jQuery);
</script>
<?php
self::$script_output = true;
return $form;
}
function add_init_script( $return ) {
$start_field_format = false;
$end_field_format = false;
foreach( $this->form['fields'] as &$field ) {
if( $field['id'] == $this->start_field_id )
$start_field_format = $field['dateFormat'] ? $field['dateFormat'] : 'mdy';
if( $field['id'] == $this->end_field_id )
$end_field_format = $field['dateFormat'] ? $field['dateFormat'] : 'mdy';
}
$script = "new gwdc({
formId: {$this->form['id']},
startFieldId: {$this->start_field_id},
startDateFormat: '$start_field_format',
endFieldId: {$this->end_field_id},
endDateFormat: '$end_field_format',
countFieldId: {$this->count_field_id},
countAdjust: {$this->count_adjust}
});";
$slug = implode( '_', array( 'gw_display_count', $this->start_field_id, $this->end_field_id, $this->count_field_id ) );
GFFormDisplay::add_init_script( $this->form['id'], $slug, GFFormDisplay::ON_PAGE_RENDER, $script );
// remove filter so init script is not output on subsequent forms
remove_filter( 'gform_init_scripts_footer', array( &$this, 'add_init_script' ) );
return $return;
}
function override_submitted_value( $form ) {
$start_date = false;
$end_date = false;
foreach( $form['fields'] as &$field ) {
if( $field['id'] == $this->start_field_id )
$start_date = self::parse_field_date( $field );
if( $field['id'] == $this->end_field_id )
$end_date = self::parse_field_date( $field );
}
if( $start_date > $end_date ) {
$day_count = 0;
} else {
$diff = $end_date - $start_date;
$day_count = $diff / ( 60 * 60 * 24 ); // secs * mins * hours
$day_count = round( $day_count ) + $this->count_adjust;
}
$_POST["input_{$this->count_field_id}"] = $day_count;
}
static function parse_field_date( $field ) {
$date_value = rgpost("input_{$field['id']}");
$date_format = empty( $field['dateFormat'] ) ? 'mdy' : esc_attr( $field['dateFormat'] );
$date_info = GFCommon::parse_date( $date_value, $date_format );
if( empty( $date_info ) )
return false;
return strtotime( "{$date_info['year']}-{$date_info['month']}-{$date_info['day']}" );
}
}
# Configuration
new GWDayCount( array(
'form_id' => 1,
'start_field_id' => 1,
'end_field_id' => 2,
'count_field_id' => 3 ) );
new GWDayCount( array(
'form_id' => 1,
'start_field_id' => 1,
'end_field_id' => 2,
'count_field_id' => 16 ) );
new GWDayCount( array(
'form_id' => 1,
'start_field_id' => 1,
'end_field_id' => 2,
'count_field_id' => 15 ) );
javascript jquery wordpress gravity-forms-plugin gravityforms
I've been going on for 2 days try to find solution for gravity forms to find a numbers of days between dates but the problem here is i must find numbers for to different periods in year
year : 365 days
low season : 1 novembre ---> 1 jun
high season : 1 jun ---> 31 october
example : when i give 2 dates 01/10/2018 -----> 01/12/2019
resultat will be :
year : 365days
low season : 31 days
high season : 30
days
in the laste example i have 3 periods year and low season and high
i find solution to give numbers of days total with graity wiz
https://gravitywiz.com/calculate-number-of-days-between-two-dates/
so the answer for me is 426 days
what i can do ?..
<?php /**
* Gravity Wiz // Calculate Number of Days Between Two Gravity Form Date Fields
*
* Allows you to calculated the number of days between two Gravity Form date fields and populate that number into a
* field on your Gravity Form.
*
* @version 1.1
* @author David Smith <david@gravitywiz.com>
* @license GPL-2.0+
* @link http://gravitywiz.com/calculate-number-of-days-between-two-dates/
* @copyright 2013 Gravity Wiz
*/ class GWDayCount {
private static $script_output;
function __construct( $args ) {
extract( wp_parse_args( $args, array(
'form_id' => false,
'start_field_id' => false,
'end_field_id' => false,
'count_field_id' => false,
'include_end_date' => true,
) ) );
$this->form_id = $form_id;
$this->start_field_id = $start_field_id;
$this->end_field_id = $end_field_id;
$this->count_field_id = $count_field_id;
$this->count_adjust = $include_end_date ? 1 : 0;
add_filter( "gform_pre_render_{$form_id}", array( &$this, 'load_form_script') );
add_action( "gform_pre_submission_{$form_id}", array( &$this, 'override_submitted_value') );
}
function load_form_script( $form ) {
// workaround to make this work for < 1.7
$this->form = $form;
add_filter( 'gform_init_scripts_footer', array( &$this, 'add_init_script' ) );
if( self::$script_output )
return $form;
?>
<script type="text/javascript">
(function($){
window.gwdc = function( options ) {
this.options = options;
this.startDateInput = $( '#input_' + this.options.formId + '_' + this.options.startFieldId );
this.endDateInput = $( '#input_' + this.options.formId + '_' + this.options.endFieldId );
this.countInput = $( '#input_' + this.options.formId + '_' + this.options.countFieldId );
this.init = function() {
var gwdc = this;
// add data for "format" for parsing date
gwdc.startDateInput.data( 'format', this.options.startDateFormat );
gwdc.endDateInput.data( 'format', this.options.endDateFormat );
gwdc.populateDayCount();
gwdc.startDateInput.change( function() {
gwdc.populateDayCount();
} );
gwdc.endDateInput.change( function() {
gwdc.populateDayCount();
} );
$( '#ui-datepicker-div' ).hide();
}
this.getDayCount = function() {
var startDate = this.parseDate( this.startDateInput.val(), this.startDateInput.data('format') )
var endDate = this.parseDate( this.endDateInput.val(), this.endDateInput.data('format') );
var dayCount = 0;
if( !this.isValidDate( startDate ) || !this.isValidDate( endDate ) )
return '';
if( startDate > endDate ) {
return 0;
} else {
var diff = endDate - startDate;
dayCount = diff / ( 60 * 60 * 24 * 1000 ); // secs * mins * hours * milliseconds
dayCount = Math.round( dayCount ) + this.options.countAdjust;
return dayCount;
}
}
this.parseDate = function( value, format ) {
if( !value )
return false;
format = format.split('_');
var dateFormat = format[0];
var separators = { slash: '/', dash: '-', dot: '.' };
var separator = format.length > 1 ? separators[format[1]] : separators.slash;
var dateArr = value.split(separator);
switch( dateFormat ) {
case 'mdy':
return new Date( dateArr[2], dateArr[0] - 1, dateArr[1] );
case 'dmy':
return new Date( dateArr[2], dateArr[1] - 1, dateArr[0] );
case 'ymd':
return new Date( dateArr[0], dateArr[1] - 1, dateArr[2] );
}
return false;
}
this.populateDayCount = function() {
this.countInput.val( this.getDayCount() ).change();
}
this.isValidDate = function( date ) {
return !isNaN( Date.parse( date ) );
}
this.init();
}
})(jQuery);
</script>
<?php
self::$script_output = true;
return $form;
}
function add_init_script( $return ) {
$start_field_format = false;
$end_field_format = false;
foreach( $this->form['fields'] as &$field ) {
if( $field['id'] == $this->start_field_id )
$start_field_format = $field['dateFormat'] ? $field['dateFormat'] : 'mdy';
if( $field['id'] == $this->end_field_id )
$end_field_format = $field['dateFormat'] ? $field['dateFormat'] : 'mdy';
}
$script = "new gwdc({
formId: {$this->form['id']},
startFieldId: {$this->start_field_id},
startDateFormat: '$start_field_format',
endFieldId: {$this->end_field_id},
endDateFormat: '$end_field_format',
countFieldId: {$this->count_field_id},
countAdjust: {$this->count_adjust}
});";
$slug = implode( '_', array( 'gw_display_count', $this->start_field_id, $this->end_field_id, $this->count_field_id ) );
GFFormDisplay::add_init_script( $this->form['id'], $slug, GFFormDisplay::ON_PAGE_RENDER, $script );
// remove filter so init script is not output on subsequent forms
remove_filter( 'gform_init_scripts_footer', array( &$this, 'add_init_script' ) );
return $return;
}
function override_submitted_value( $form ) {
$start_date = false;
$end_date = false;
foreach( $form['fields'] as &$field ) {
if( $field['id'] == $this->start_field_id )
$start_date = self::parse_field_date( $field );
if( $field['id'] == $this->end_field_id )
$end_date = self::parse_field_date( $field );
}
if( $start_date > $end_date ) {
$day_count = 0;
} else {
$diff = $end_date - $start_date;
$day_count = $diff / ( 60 * 60 * 24 ); // secs * mins * hours
$day_count = round( $day_count ) + $this->count_adjust;
}
$_POST["input_{$this->count_field_id}"] = $day_count;
}
static function parse_field_date( $field ) {
$date_value = rgpost("input_{$field['id']}");
$date_format = empty( $field['dateFormat'] ) ? 'mdy' : esc_attr( $field['dateFormat'] );
$date_info = GFCommon::parse_date( $date_value, $date_format );
if( empty( $date_info ) )
return false;
return strtotime( "{$date_info['year']}-{$date_info['month']}-{$date_info['day']}" );
}
}
# Configuration
new GWDayCount( array(
'form_id' => 1,
'start_field_id' => 1,
'end_field_id' => 2,
'count_field_id' => 3 ) );
new GWDayCount( array(
'form_id' => 1,
'start_field_id' => 1,
'end_field_id' => 2,
'count_field_id' => 16 ) );
new GWDayCount( array(
'form_id' => 1,
'start_field_id' => 1,
'end_field_id' => 2,
'count_field_id' => 15 ) );
javascript jquery wordpress gravity-forms-plugin gravityforms
javascript jquery wordpress gravity-forms-plugin gravityforms
asked Nov 15 '18 at 16:58
Hassani MohamedHassani Mohamed
1
1
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
If you need to calculate the number 426 in javascript, you can just apply the same logic used in the PHP part.
Using .getTime()
on a javascript Date object gets you unix time.
const start = '2018-10-01';
const end = '2019-12-01';
const MILISECONDS_DAY = 86400000;
const difference_in_days = Math.round(( new Date( end ).getTime() - new Date( start ).getTime() ) / MILISECONDS_DAY );
console.log( difference_in_days );
thnak u bit is Not that Shilly my question is how to to find number of days "low season : 1 novembre ---> 1 jun / high season : 1 jun ---> 31 october " in one year
– Hassani Mohamed
Nov 16 '18 at 8:10
That's the exact same calculation. The number of days in high season is the difference in days between 2018-10-01 and 2018-10-31, and the low season difference between 2019-11-01 until 2019-12-01. If you save the start and end of the seasons as a datetime object as well, you can clone it and only have to change the year to calculate any season you want.
– Shilly
Nov 16 '18 at 8:27
sorry but i'm confused here how i can put this in the code of gravitywiz to give 2 results not just one for one date ?? thank you
– Hassani Mohamed
Nov 17 '18 at 10:28
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53324446%2fnumber-of-days-between-dates-for-two-different-periods-in-year-gravity-forms%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
If you need to calculate the number 426 in javascript, you can just apply the same logic used in the PHP part.
Using .getTime()
on a javascript Date object gets you unix time.
const start = '2018-10-01';
const end = '2019-12-01';
const MILISECONDS_DAY = 86400000;
const difference_in_days = Math.round(( new Date( end ).getTime() - new Date( start ).getTime() ) / MILISECONDS_DAY );
console.log( difference_in_days );
thnak u bit is Not that Shilly my question is how to to find number of days "low season : 1 novembre ---> 1 jun / high season : 1 jun ---> 31 october " in one year
– Hassani Mohamed
Nov 16 '18 at 8:10
That's the exact same calculation. The number of days in high season is the difference in days between 2018-10-01 and 2018-10-31, and the low season difference between 2019-11-01 until 2019-12-01. If you save the start and end of the seasons as a datetime object as well, you can clone it and only have to change the year to calculate any season you want.
– Shilly
Nov 16 '18 at 8:27
sorry but i'm confused here how i can put this in the code of gravitywiz to give 2 results not just one for one date ?? thank you
– Hassani Mohamed
Nov 17 '18 at 10:28
add a comment |
If you need to calculate the number 426 in javascript, you can just apply the same logic used in the PHP part.
Using .getTime()
on a javascript Date object gets you unix time.
const start = '2018-10-01';
const end = '2019-12-01';
const MILISECONDS_DAY = 86400000;
const difference_in_days = Math.round(( new Date( end ).getTime() - new Date( start ).getTime() ) / MILISECONDS_DAY );
console.log( difference_in_days );
thnak u bit is Not that Shilly my question is how to to find number of days "low season : 1 novembre ---> 1 jun / high season : 1 jun ---> 31 october " in one year
– Hassani Mohamed
Nov 16 '18 at 8:10
That's the exact same calculation. The number of days in high season is the difference in days between 2018-10-01 and 2018-10-31, and the low season difference between 2019-11-01 until 2019-12-01. If you save the start and end of the seasons as a datetime object as well, you can clone it and only have to change the year to calculate any season you want.
– Shilly
Nov 16 '18 at 8:27
sorry but i'm confused here how i can put this in the code of gravitywiz to give 2 results not just one for one date ?? thank you
– Hassani Mohamed
Nov 17 '18 at 10:28
add a comment |
If you need to calculate the number 426 in javascript, you can just apply the same logic used in the PHP part.
Using .getTime()
on a javascript Date object gets you unix time.
const start = '2018-10-01';
const end = '2019-12-01';
const MILISECONDS_DAY = 86400000;
const difference_in_days = Math.round(( new Date( end ).getTime() - new Date( start ).getTime() ) / MILISECONDS_DAY );
console.log( difference_in_days );
If you need to calculate the number 426 in javascript, you can just apply the same logic used in the PHP part.
Using .getTime()
on a javascript Date object gets you unix time.
const start = '2018-10-01';
const end = '2019-12-01';
const MILISECONDS_DAY = 86400000;
const difference_in_days = Math.round(( new Date( end ).getTime() - new Date( start ).getTime() ) / MILISECONDS_DAY );
console.log( difference_in_days );
const start = '2018-10-01';
const end = '2019-12-01';
const MILISECONDS_DAY = 86400000;
const difference_in_days = Math.round(( new Date( end ).getTime() - new Date( start ).getTime() ) / MILISECONDS_DAY );
console.log( difference_in_days );
const start = '2018-10-01';
const end = '2019-12-01';
const MILISECONDS_DAY = 86400000;
const difference_in_days = Math.round(( new Date( end ).getTime() - new Date( start ).getTime() ) / MILISECONDS_DAY );
console.log( difference_in_days );
answered Nov 15 '18 at 17:09
ShillyShilly
5,7181717
5,7181717
thnak u bit is Not that Shilly my question is how to to find number of days "low season : 1 novembre ---> 1 jun / high season : 1 jun ---> 31 october " in one year
– Hassani Mohamed
Nov 16 '18 at 8:10
That's the exact same calculation. The number of days in high season is the difference in days between 2018-10-01 and 2018-10-31, and the low season difference between 2019-11-01 until 2019-12-01. If you save the start and end of the seasons as a datetime object as well, you can clone it and only have to change the year to calculate any season you want.
– Shilly
Nov 16 '18 at 8:27
sorry but i'm confused here how i can put this in the code of gravitywiz to give 2 results not just one for one date ?? thank you
– Hassani Mohamed
Nov 17 '18 at 10:28
add a comment |
thnak u bit is Not that Shilly my question is how to to find number of days "low season : 1 novembre ---> 1 jun / high season : 1 jun ---> 31 october " in one year
– Hassani Mohamed
Nov 16 '18 at 8:10
That's the exact same calculation. The number of days in high season is the difference in days between 2018-10-01 and 2018-10-31, and the low season difference between 2019-11-01 until 2019-12-01. If you save the start and end of the seasons as a datetime object as well, you can clone it and only have to change the year to calculate any season you want.
– Shilly
Nov 16 '18 at 8:27
sorry but i'm confused here how i can put this in the code of gravitywiz to give 2 results not just one for one date ?? thank you
– Hassani Mohamed
Nov 17 '18 at 10:28
thnak u bit is Not that Shilly my question is how to to find number of days "low season : 1 novembre ---> 1 jun / high season : 1 jun ---> 31 october " in one year
– Hassani Mohamed
Nov 16 '18 at 8:10
thnak u bit is Not that Shilly my question is how to to find number of days "low season : 1 novembre ---> 1 jun / high season : 1 jun ---> 31 october " in one year
– Hassani Mohamed
Nov 16 '18 at 8:10
That's the exact same calculation. The number of days in high season is the difference in days between 2018-10-01 and 2018-10-31, and the low season difference between 2019-11-01 until 2019-12-01. If you save the start and end of the seasons as a datetime object as well, you can clone it and only have to change the year to calculate any season you want.
– Shilly
Nov 16 '18 at 8:27
That's the exact same calculation. The number of days in high season is the difference in days between 2018-10-01 and 2018-10-31, and the low season difference between 2019-11-01 until 2019-12-01. If you save the start and end of the seasons as a datetime object as well, you can clone it and only have to change the year to calculate any season you want.
– Shilly
Nov 16 '18 at 8:27
sorry but i'm confused here how i can put this in the code of gravitywiz to give 2 results not just one for one date ?? thank you
– Hassani Mohamed
Nov 17 '18 at 10:28
sorry but i'm confused here how i can put this in the code of gravitywiz to give 2 results not just one for one date ?? thank you
– Hassani Mohamed
Nov 17 '18 at 10:28
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53324446%2fnumber-of-days-between-dates-for-two-different-periods-in-year-gravity-forms%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
J95,RwJq5miU9EFp 43kSnuoEo,9tOuyo7DpHX,fQ0R,0TZp5JyY70TZ2H,ItRJAOzK4966,Om4a9L8,1er3Yg6,GPciPHL