How to provide single bean definition for all child class in spring configuration?
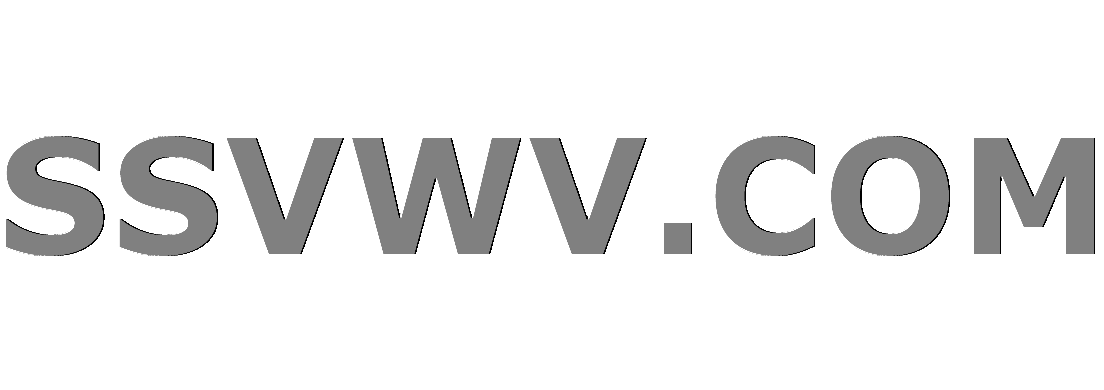
Multi tool use
I am new to spring and exploring it, I want to my spring container to create all objects from a specific package.
Circle Class:
@Component
public class Circle extends AbstractShape {
@Override
public void draw() {
}
@Override
public int calculateArea(int x, int y) {
return (int) (3.14 * x * y);
}
}
Square Class:
@Component
public class Square extends AbstractShape {
@Override
public void draw() {
}
@Override
public int calculateArea(int x, int y) {
return x * y;
}
}
My spring config class which basically create bean defination for me:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class ShapeConfig {
@Bean
public Circle getCircle() {
return new Circle();
}
@Bean
public Square getSquare() {
return new Square();
}
}
I want my bean definition to be created which extends class "AbstractShape", and want to avoid providing one by one bean definition in my config class. Please suggest possible ways to do so.
Thanks in Advance,
Priyank
java spring spring-boot dependency-injection inversion-of-control
add a comment |
I am new to spring and exploring it, I want to my spring container to create all objects from a specific package.
Circle Class:
@Component
public class Circle extends AbstractShape {
@Override
public void draw() {
}
@Override
public int calculateArea(int x, int y) {
return (int) (3.14 * x * y);
}
}
Square Class:
@Component
public class Square extends AbstractShape {
@Override
public void draw() {
}
@Override
public int calculateArea(int x, int y) {
return x * y;
}
}
My spring config class which basically create bean defination for me:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class ShapeConfig {
@Bean
public Circle getCircle() {
return new Circle();
}
@Bean
public Square getSquare() {
return new Square();
}
}
I want my bean definition to be created which extends class "AbstractShape", and want to avoid providing one by one bean definition in my config class. Please suggest possible ways to do so.
Thanks in Advance,
Priyank
java spring spring-boot dependency-injection inversion-of-control
1
I am not sure if I get you right - but why can't you annotate Circle and Square as @Component?
– Andrej Buday
Nov 15 '18 at 17:19
Sorry I missed that part. I already annotated as @Component.
– Priyank Shah
Nov 16 '18 at 5:41
add a comment |
I am new to spring and exploring it, I want to my spring container to create all objects from a specific package.
Circle Class:
@Component
public class Circle extends AbstractShape {
@Override
public void draw() {
}
@Override
public int calculateArea(int x, int y) {
return (int) (3.14 * x * y);
}
}
Square Class:
@Component
public class Square extends AbstractShape {
@Override
public void draw() {
}
@Override
public int calculateArea(int x, int y) {
return x * y;
}
}
My spring config class which basically create bean defination for me:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class ShapeConfig {
@Bean
public Circle getCircle() {
return new Circle();
}
@Bean
public Square getSquare() {
return new Square();
}
}
I want my bean definition to be created which extends class "AbstractShape", and want to avoid providing one by one bean definition in my config class. Please suggest possible ways to do so.
Thanks in Advance,
Priyank
java spring spring-boot dependency-injection inversion-of-control
I am new to spring and exploring it, I want to my spring container to create all objects from a specific package.
Circle Class:
@Component
public class Circle extends AbstractShape {
@Override
public void draw() {
}
@Override
public int calculateArea(int x, int y) {
return (int) (3.14 * x * y);
}
}
Square Class:
@Component
public class Square extends AbstractShape {
@Override
public void draw() {
}
@Override
public int calculateArea(int x, int y) {
return x * y;
}
}
My spring config class which basically create bean defination for me:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class ShapeConfig {
@Bean
public Circle getCircle() {
return new Circle();
}
@Bean
public Square getSquare() {
return new Square();
}
}
I want my bean definition to be created which extends class "AbstractShape", and want to avoid providing one by one bean definition in my config class. Please suggest possible ways to do so.
Thanks in Advance,
Priyank
java spring spring-boot dependency-injection inversion-of-control
java spring spring-boot dependency-injection inversion-of-control
edited Nov 16 '18 at 5:40
Priyank Shah
asked Nov 15 '18 at 17:02
Priyank ShahPriyank Shah
1472518
1472518
1
I am not sure if I get you right - but why can't you annotate Circle and Square as @Component?
– Andrej Buday
Nov 15 '18 at 17:19
Sorry I missed that part. I already annotated as @Component.
– Priyank Shah
Nov 16 '18 at 5:41
add a comment |
1
I am not sure if I get you right - but why can't you annotate Circle and Square as @Component?
– Andrej Buday
Nov 15 '18 at 17:19
Sorry I missed that part. I already annotated as @Component.
– Priyank Shah
Nov 16 '18 at 5:41
1
1
I am not sure if I get you right - but why can't you annotate Circle and Square as @Component?
– Andrej Buday
Nov 15 '18 at 17:19
I am not sure if I get you right - but why can't you annotate Circle and Square as @Component?
– Andrej Buday
Nov 15 '18 at 17:19
Sorry I missed that part. I already annotated as @Component.
– Priyank Shah
Nov 16 '18 at 5:41
Sorry I missed that part. I already annotated as @Component.
– Priyank Shah
Nov 16 '18 at 5:41
add a comment |
2 Answers
2
active
oldest
votes
Annotating the class Circle
and Square
itself with @Component
is much easier for a task like this.
This method is identical to declaring a method in a @Configuration
class, the way you are doing now.
You can try annotating the AbstractShape
class, I don't know from the top of my head if it works
I already tagged "Circle" and "Square" as @Component. and annotating Abstract class is not a good idea. because you can not instantiate an abstract class.
– Priyank Shah
Nov 16 '18 at 5:44
add a comment |
It got resolved by adding @ComponenetScan at configuration.
Thanks for your answers.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53324516%2fhow-to-provide-single-bean-definition-for-all-child-class-in-spring-configuratio%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Annotating the class Circle
and Square
itself with @Component
is much easier for a task like this.
This method is identical to declaring a method in a @Configuration
class, the way you are doing now.
You can try annotating the AbstractShape
class, I don't know from the top of my head if it works
I already tagged "Circle" and "Square" as @Component. and annotating Abstract class is not a good idea. because you can not instantiate an abstract class.
– Priyank Shah
Nov 16 '18 at 5:44
add a comment |
Annotating the class Circle
and Square
itself with @Component
is much easier for a task like this.
This method is identical to declaring a method in a @Configuration
class, the way you are doing now.
You can try annotating the AbstractShape
class, I don't know from the top of my head if it works
I already tagged "Circle" and "Square" as @Component. and annotating Abstract class is not a good idea. because you can not instantiate an abstract class.
– Priyank Shah
Nov 16 '18 at 5:44
add a comment |
Annotating the class Circle
and Square
itself with @Component
is much easier for a task like this.
This method is identical to declaring a method in a @Configuration
class, the way you are doing now.
You can try annotating the AbstractShape
class, I don't know from the top of my head if it works
Annotating the class Circle
and Square
itself with @Component
is much easier for a task like this.
This method is identical to declaring a method in a @Configuration
class, the way you are doing now.
You can try annotating the AbstractShape
class, I don't know from the top of my head if it works
answered Nov 15 '18 at 17:04


László StahorszkiLászló Stahorszki
376212
376212
I already tagged "Circle" and "Square" as @Component. and annotating Abstract class is not a good idea. because you can not instantiate an abstract class.
– Priyank Shah
Nov 16 '18 at 5:44
add a comment |
I already tagged "Circle" and "Square" as @Component. and annotating Abstract class is not a good idea. because you can not instantiate an abstract class.
– Priyank Shah
Nov 16 '18 at 5:44
I already tagged "Circle" and "Square" as @Component. and annotating Abstract class is not a good idea. because you can not instantiate an abstract class.
– Priyank Shah
Nov 16 '18 at 5:44
I already tagged "Circle" and "Square" as @Component. and annotating Abstract class is not a good idea. because you can not instantiate an abstract class.
– Priyank Shah
Nov 16 '18 at 5:44
add a comment |
It got resolved by adding @ComponenetScan at configuration.
Thanks for your answers.
add a comment |
It got resolved by adding @ComponenetScan at configuration.
Thanks for your answers.
add a comment |
It got resolved by adding @ComponenetScan at configuration.
Thanks for your answers.
It got resolved by adding @ComponenetScan at configuration.
Thanks for your answers.
answered Nov 16 '18 at 6:15
Priyank ShahPriyank Shah
1472518
1472518
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53324516%2fhow-to-provide-single-bean-definition-for-all-child-class-in-spring-configuratio%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
7NDbwslT,Rjo m,y,H,Whs2LT,PfZxSH3hq,r8WAzX3qCVzQRIMHfZa18WrM,isHvYgcT NN,b
1
I am not sure if I get you right - but why can't you annotate Circle and Square as @Component?
– Andrej Buday
Nov 15 '18 at 17:19
Sorry I missed that part. I already annotated as @Component.
– Priyank Shah
Nov 16 '18 at 5:41