micronaut petstore a code segment from java to groovy
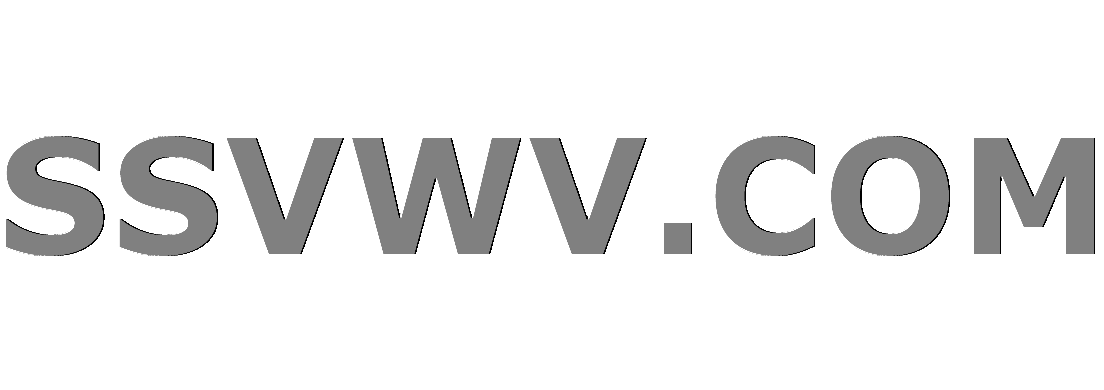
Multi tool use
The actual code is here
private Function<String, Mono<? extends Offer>> keyToOffer(RedisReactiveCommands<String, String> commands) {
return key -> {
Flux<KeyValue<String, String>> values = commands.hmget(key, "price", "description");
Map<String, String> map = new HashMap<>(3);
return values.reduce(map, (all, keyValue) -> {
all.put(keyValue.getKey(), keyValue.getValue());
return all;
})
.map(ConvertibleValues::of)
.flatMap(entries -> {
String description = entries.get("description", String.class).orElseThrow(() -> new IllegalStateException("No description"));
BigDecimal price = entries.get("price", BigDecimal.class).orElseThrow(() -> new IllegalStateException("No price"));
Flowable<Pet> findPetFlowable = petClient.find(key).toFlowable();
return Mono.from(findPetFlowable).map(pet -> new Offer(pet, description, price));
});
};
}
I have tried in a variety of different ways to convert above into groovy and all attempts so far not worked out too well. I wondered if anyone better with groovy could help
My attempt wasn't posted since the code itself firstly returns Ambiguous code block in Intelij and secondly looks totally wrong.
private Function<String, Mono<? extends Orders>> keyToOrder(RedisReactiveCommands<String, String> commands) {
return { key -> {
Flux<KeyValue<String, String>> values = commands.hmget(key, "price", "description");
Map map = [:]
return values.reduce(map, (all, keyValue)= {all.put(keyValue.getKey(), keyValue.getValue());
return all
}).map({entries -> ConvertibleValues.of(entries)})
.flatMap({entries -> {
String description = entries.get("description", String.class).orElseThrow({ new IllegalStateException("No description")});
BigDecimal price = entries.get("price", BigDecimal.class).orElseThrow({new IllegalStateException("No price")});
Flowable<Item> findItemFlowable = itemClient.find(key).toFlowable();
return Mono.from(findItemFlowable).map({item -> new Orders(item, description, price)});
}});
}}
}
When attempting to convert to groovy the biggest struggle appeared to be around:
return values.reduce(map, (all, keyValue)= {all.put(keyValue.getKey(), keyValue.getValue());
return all
This is nothing like what the original java code looked like and really unsure if it would even behave as it should. The issue I had was to find anything aorund RXJAVA Flux .reduce written in groovy out there.
The Ambiguous code block is around this entire flatMap segment at the very bottom
.flatMap({entries -> {
I haven't checked in this change nor did I post it since frankly it was embarrassing.
I have also come across:
http://reactivex.io/documentation/operators/reduce.html#collapseRxGroovy
numbers.reduce({ a, b -> a+b }).
and ended up with:
Map<String, String> map = new HashMap<>(3);
return values.reduce({all, keyValue->
all.put(keyValue.getKey(), keyValue.getValue());
return all
}).map({entries -> ConvertibleValues.of(entries)})
But this again looks wrong and doesn't really match what the java code was doing.
Final edit to suggest I have got Intelij to accept the code as groovy but not quite sure if it is what the java code was actually doing, since declared map is not even used:
private Function<String, Mono<? extends Orders>> keyToOrder(RedisReactiveCommands<String, String> commands) {
Flux<KeyValue<String, String>> values = commands.hmget(key, "price", "description");
Map<String, String> map = new HashMap<>(3);
values.reduce({all, keyValue->
all.put(keyValue.getKey(), keyValue.getValue());
return all
}).map({entries -> ConvertibleValues.of(entries)})
.flatMap({entries -> bindEntry(entries)});
return values.key
}
private Mono<Orders> bindEntry(entries) {
String description = entries.get("description", String.class).orElseThrow({ new IllegalStateException("No description")});
BigDecimal price = entries.get("price", BigDecimal.class).orElseThrow({new IllegalStateException("No price")});
Flowable<Item> findItemFlowable = itemClient.find(key).toFlowable();
return Mono.from(findItemFlowable).map({item -> new Orders(item, description, price)});
}
java groovy rx-java2 micronaut
|
show 1 more comment
The actual code is here
private Function<String, Mono<? extends Offer>> keyToOffer(RedisReactiveCommands<String, String> commands) {
return key -> {
Flux<KeyValue<String, String>> values = commands.hmget(key, "price", "description");
Map<String, String> map = new HashMap<>(3);
return values.reduce(map, (all, keyValue) -> {
all.put(keyValue.getKey(), keyValue.getValue());
return all;
})
.map(ConvertibleValues::of)
.flatMap(entries -> {
String description = entries.get("description", String.class).orElseThrow(() -> new IllegalStateException("No description"));
BigDecimal price = entries.get("price", BigDecimal.class).orElseThrow(() -> new IllegalStateException("No price"));
Flowable<Pet> findPetFlowable = petClient.find(key).toFlowable();
return Mono.from(findPetFlowable).map(pet -> new Offer(pet, description, price));
});
};
}
I have tried in a variety of different ways to convert above into groovy and all attempts so far not worked out too well. I wondered if anyone better with groovy could help
My attempt wasn't posted since the code itself firstly returns Ambiguous code block in Intelij and secondly looks totally wrong.
private Function<String, Mono<? extends Orders>> keyToOrder(RedisReactiveCommands<String, String> commands) {
return { key -> {
Flux<KeyValue<String, String>> values = commands.hmget(key, "price", "description");
Map map = [:]
return values.reduce(map, (all, keyValue)= {all.put(keyValue.getKey(), keyValue.getValue());
return all
}).map({entries -> ConvertibleValues.of(entries)})
.flatMap({entries -> {
String description = entries.get("description", String.class).orElseThrow({ new IllegalStateException("No description")});
BigDecimal price = entries.get("price", BigDecimal.class).orElseThrow({new IllegalStateException("No price")});
Flowable<Item> findItemFlowable = itemClient.find(key).toFlowable();
return Mono.from(findItemFlowable).map({item -> new Orders(item, description, price)});
}});
}}
}
When attempting to convert to groovy the biggest struggle appeared to be around:
return values.reduce(map, (all, keyValue)= {all.put(keyValue.getKey(), keyValue.getValue());
return all
This is nothing like what the original java code looked like and really unsure if it would even behave as it should. The issue I had was to find anything aorund RXJAVA Flux .reduce written in groovy out there.
The Ambiguous code block is around this entire flatMap segment at the very bottom
.flatMap({entries -> {
I haven't checked in this change nor did I post it since frankly it was embarrassing.
I have also come across:
http://reactivex.io/documentation/operators/reduce.html#collapseRxGroovy
numbers.reduce({ a, b -> a+b }).
and ended up with:
Map<String, String> map = new HashMap<>(3);
return values.reduce({all, keyValue->
all.put(keyValue.getKey(), keyValue.getValue());
return all
}).map({entries -> ConvertibleValues.of(entries)})
But this again looks wrong and doesn't really match what the java code was doing.
Final edit to suggest I have got Intelij to accept the code as groovy but not quite sure if it is what the java code was actually doing, since declared map is not even used:
private Function<String, Mono<? extends Orders>> keyToOrder(RedisReactiveCommands<String, String> commands) {
Flux<KeyValue<String, String>> values = commands.hmget(key, "price", "description");
Map<String, String> map = new HashMap<>(3);
values.reduce({all, keyValue->
all.put(keyValue.getKey(), keyValue.getValue());
return all
}).map({entries -> ConvertibleValues.of(entries)})
.flatMap({entries -> bindEntry(entries)});
return values.key
}
private Mono<Orders> bindEntry(entries) {
String description = entries.get("description", String.class).orElseThrow({ new IllegalStateException("No description")});
BigDecimal price = entries.get("price", BigDecimal.class).orElseThrow({new IllegalStateException("No price")});
Flowable<Item> findItemFlowable = itemClient.find(key).toFlowable();
return Mono.from(findItemFlowable).map({item -> new Orders(item, description, price)});
}
java groovy rx-java2 micronaut
I'm not sure what is the question here - do you want us to re-write this class for you?
– Iłya Bursov
Nov 15 '18 at 17:01
Hi llya In short if above can be converted from java to groovy that would be great github.com/vahidhedayati/micronaut-java-groovy-comparison/blob/… - the first link is what I am trying from this code github.com/vahidhedayati/micronaut-java-groovy-comparison/blob/… - Simply trying to understand some of the more complex rxjava statements in java and how to do same under groovy
– Vahid
Nov 15 '18 at 17:03
3
idownvotedbecau.se/itsnotworking
– Iłya Bursov
Nov 15 '18 at 17:07
okay thank you for your input
– Vahid
Nov 15 '18 at 17:09
If you have failing code, then please add your attempt and what errors you get to the question. An potential answer can work from that then.
– cfrick
Nov 15 '18 at 20:03
|
show 1 more comment
The actual code is here
private Function<String, Mono<? extends Offer>> keyToOffer(RedisReactiveCommands<String, String> commands) {
return key -> {
Flux<KeyValue<String, String>> values = commands.hmget(key, "price", "description");
Map<String, String> map = new HashMap<>(3);
return values.reduce(map, (all, keyValue) -> {
all.put(keyValue.getKey(), keyValue.getValue());
return all;
})
.map(ConvertibleValues::of)
.flatMap(entries -> {
String description = entries.get("description", String.class).orElseThrow(() -> new IllegalStateException("No description"));
BigDecimal price = entries.get("price", BigDecimal.class).orElseThrow(() -> new IllegalStateException("No price"));
Flowable<Pet> findPetFlowable = petClient.find(key).toFlowable();
return Mono.from(findPetFlowable).map(pet -> new Offer(pet, description, price));
});
};
}
I have tried in a variety of different ways to convert above into groovy and all attempts so far not worked out too well. I wondered if anyone better with groovy could help
My attempt wasn't posted since the code itself firstly returns Ambiguous code block in Intelij and secondly looks totally wrong.
private Function<String, Mono<? extends Orders>> keyToOrder(RedisReactiveCommands<String, String> commands) {
return { key -> {
Flux<KeyValue<String, String>> values = commands.hmget(key, "price", "description");
Map map = [:]
return values.reduce(map, (all, keyValue)= {all.put(keyValue.getKey(), keyValue.getValue());
return all
}).map({entries -> ConvertibleValues.of(entries)})
.flatMap({entries -> {
String description = entries.get("description", String.class).orElseThrow({ new IllegalStateException("No description")});
BigDecimal price = entries.get("price", BigDecimal.class).orElseThrow({new IllegalStateException("No price")});
Flowable<Item> findItemFlowable = itemClient.find(key).toFlowable();
return Mono.from(findItemFlowable).map({item -> new Orders(item, description, price)});
}});
}}
}
When attempting to convert to groovy the biggest struggle appeared to be around:
return values.reduce(map, (all, keyValue)= {all.put(keyValue.getKey(), keyValue.getValue());
return all
This is nothing like what the original java code looked like and really unsure if it would even behave as it should. The issue I had was to find anything aorund RXJAVA Flux .reduce written in groovy out there.
The Ambiguous code block is around this entire flatMap segment at the very bottom
.flatMap({entries -> {
I haven't checked in this change nor did I post it since frankly it was embarrassing.
I have also come across:
http://reactivex.io/documentation/operators/reduce.html#collapseRxGroovy
numbers.reduce({ a, b -> a+b }).
and ended up with:
Map<String, String> map = new HashMap<>(3);
return values.reduce({all, keyValue->
all.put(keyValue.getKey(), keyValue.getValue());
return all
}).map({entries -> ConvertibleValues.of(entries)})
But this again looks wrong and doesn't really match what the java code was doing.
Final edit to suggest I have got Intelij to accept the code as groovy but not quite sure if it is what the java code was actually doing, since declared map is not even used:
private Function<String, Mono<? extends Orders>> keyToOrder(RedisReactiveCommands<String, String> commands) {
Flux<KeyValue<String, String>> values = commands.hmget(key, "price", "description");
Map<String, String> map = new HashMap<>(3);
values.reduce({all, keyValue->
all.put(keyValue.getKey(), keyValue.getValue());
return all
}).map({entries -> ConvertibleValues.of(entries)})
.flatMap({entries -> bindEntry(entries)});
return values.key
}
private Mono<Orders> bindEntry(entries) {
String description = entries.get("description", String.class).orElseThrow({ new IllegalStateException("No description")});
BigDecimal price = entries.get("price", BigDecimal.class).orElseThrow({new IllegalStateException("No price")});
Flowable<Item> findItemFlowable = itemClient.find(key).toFlowable();
return Mono.from(findItemFlowable).map({item -> new Orders(item, description, price)});
}
java groovy rx-java2 micronaut
The actual code is here
private Function<String, Mono<? extends Offer>> keyToOffer(RedisReactiveCommands<String, String> commands) {
return key -> {
Flux<KeyValue<String, String>> values = commands.hmget(key, "price", "description");
Map<String, String> map = new HashMap<>(3);
return values.reduce(map, (all, keyValue) -> {
all.put(keyValue.getKey(), keyValue.getValue());
return all;
})
.map(ConvertibleValues::of)
.flatMap(entries -> {
String description = entries.get("description", String.class).orElseThrow(() -> new IllegalStateException("No description"));
BigDecimal price = entries.get("price", BigDecimal.class).orElseThrow(() -> new IllegalStateException("No price"));
Flowable<Pet> findPetFlowable = petClient.find(key).toFlowable();
return Mono.from(findPetFlowable).map(pet -> new Offer(pet, description, price));
});
};
}
I have tried in a variety of different ways to convert above into groovy and all attempts so far not worked out too well. I wondered if anyone better with groovy could help
My attempt wasn't posted since the code itself firstly returns Ambiguous code block in Intelij and secondly looks totally wrong.
private Function<String, Mono<? extends Orders>> keyToOrder(RedisReactiveCommands<String, String> commands) {
return { key -> {
Flux<KeyValue<String, String>> values = commands.hmget(key, "price", "description");
Map map = [:]
return values.reduce(map, (all, keyValue)= {all.put(keyValue.getKey(), keyValue.getValue());
return all
}).map({entries -> ConvertibleValues.of(entries)})
.flatMap({entries -> {
String description = entries.get("description", String.class).orElseThrow({ new IllegalStateException("No description")});
BigDecimal price = entries.get("price", BigDecimal.class).orElseThrow({new IllegalStateException("No price")});
Flowable<Item> findItemFlowable = itemClient.find(key).toFlowable();
return Mono.from(findItemFlowable).map({item -> new Orders(item, description, price)});
}});
}}
}
When attempting to convert to groovy the biggest struggle appeared to be around:
return values.reduce(map, (all, keyValue)= {all.put(keyValue.getKey(), keyValue.getValue());
return all
This is nothing like what the original java code looked like and really unsure if it would even behave as it should. The issue I had was to find anything aorund RXJAVA Flux .reduce written in groovy out there.
The Ambiguous code block is around this entire flatMap segment at the very bottom
.flatMap({entries -> {
I haven't checked in this change nor did I post it since frankly it was embarrassing.
I have also come across:
http://reactivex.io/documentation/operators/reduce.html#collapseRxGroovy
numbers.reduce({ a, b -> a+b }).
and ended up with:
Map<String, String> map = new HashMap<>(3);
return values.reduce({all, keyValue->
all.put(keyValue.getKey(), keyValue.getValue());
return all
}).map({entries -> ConvertibleValues.of(entries)})
But this again looks wrong and doesn't really match what the java code was doing.
Final edit to suggest I have got Intelij to accept the code as groovy but not quite sure if it is what the java code was actually doing, since declared map is not even used:
private Function<String, Mono<? extends Orders>> keyToOrder(RedisReactiveCommands<String, String> commands) {
Flux<KeyValue<String, String>> values = commands.hmget(key, "price", "description");
Map<String, String> map = new HashMap<>(3);
values.reduce({all, keyValue->
all.put(keyValue.getKey(), keyValue.getValue());
return all
}).map({entries -> ConvertibleValues.of(entries)})
.flatMap({entries -> bindEntry(entries)});
return values.key
}
private Mono<Orders> bindEntry(entries) {
String description = entries.get("description", String.class).orElseThrow({ new IllegalStateException("No description")});
BigDecimal price = entries.get("price", BigDecimal.class).orElseThrow({new IllegalStateException("No price")});
Flowable<Item> findItemFlowable = itemClient.find(key).toFlowable();
return Mono.from(findItemFlowable).map({item -> new Orders(item, description, price)});
}
java groovy rx-java2 micronaut
java groovy rx-java2 micronaut
edited Nov 16 '18 at 9:56
Vahid
asked Nov 15 '18 at 16:59
VahidVahid
6,30421839
6,30421839
I'm not sure what is the question here - do you want us to re-write this class for you?
– Iłya Bursov
Nov 15 '18 at 17:01
Hi llya In short if above can be converted from java to groovy that would be great github.com/vahidhedayati/micronaut-java-groovy-comparison/blob/… - the first link is what I am trying from this code github.com/vahidhedayati/micronaut-java-groovy-comparison/blob/… - Simply trying to understand some of the more complex rxjava statements in java and how to do same under groovy
– Vahid
Nov 15 '18 at 17:03
3
idownvotedbecau.se/itsnotworking
– Iłya Bursov
Nov 15 '18 at 17:07
okay thank you for your input
– Vahid
Nov 15 '18 at 17:09
If you have failing code, then please add your attempt and what errors you get to the question. An potential answer can work from that then.
– cfrick
Nov 15 '18 at 20:03
|
show 1 more comment
I'm not sure what is the question here - do you want us to re-write this class for you?
– Iłya Bursov
Nov 15 '18 at 17:01
Hi llya In short if above can be converted from java to groovy that would be great github.com/vahidhedayati/micronaut-java-groovy-comparison/blob/… - the first link is what I am trying from this code github.com/vahidhedayati/micronaut-java-groovy-comparison/blob/… - Simply trying to understand some of the more complex rxjava statements in java and how to do same under groovy
– Vahid
Nov 15 '18 at 17:03
3
idownvotedbecau.se/itsnotworking
– Iłya Bursov
Nov 15 '18 at 17:07
okay thank you for your input
– Vahid
Nov 15 '18 at 17:09
If you have failing code, then please add your attempt and what errors you get to the question. An potential answer can work from that then.
– cfrick
Nov 15 '18 at 20:03
I'm not sure what is the question here - do you want us to re-write this class for you?
– Iłya Bursov
Nov 15 '18 at 17:01
I'm not sure what is the question here - do you want us to re-write this class for you?
– Iłya Bursov
Nov 15 '18 at 17:01
Hi llya In short if above can be converted from java to groovy that would be great github.com/vahidhedayati/micronaut-java-groovy-comparison/blob/… - the first link is what I am trying from this code github.com/vahidhedayati/micronaut-java-groovy-comparison/blob/… - Simply trying to understand some of the more complex rxjava statements in java and how to do same under groovy
– Vahid
Nov 15 '18 at 17:03
Hi llya In short if above can be converted from java to groovy that would be great github.com/vahidhedayati/micronaut-java-groovy-comparison/blob/… - the first link is what I am trying from this code github.com/vahidhedayati/micronaut-java-groovy-comparison/blob/… - Simply trying to understand some of the more complex rxjava statements in java and how to do same under groovy
– Vahid
Nov 15 '18 at 17:03
3
3
idownvotedbecau.se/itsnotworking
– Iłya Bursov
Nov 15 '18 at 17:07
idownvotedbecau.se/itsnotworking
– Iłya Bursov
Nov 15 '18 at 17:07
okay thank you for your input
– Vahid
Nov 15 '18 at 17:09
okay thank you for your input
– Vahid
Nov 15 '18 at 17:09
If you have failing code, then please add your attempt and what errors you get to the question. An potential answer can work from that then.
– cfrick
Nov 15 '18 at 20:03
If you have failing code, then please add your attempt and what errors you get to the question. An potential answer can work from that then.
– cfrick
Nov 15 '18 at 20:03
|
show 1 more comment
1 Answer
1
active
oldest
votes
The likely issue you're facing is because Groovy doesn't support Java method references or lambdas.
The first line is returning a lambda
Java: return key -> {
Groovy: return { key - >
That is using a groovy closure that takes the key as the argument.
Other places that use method references need to be converted
Java: .map(ConvertibleValues::of)
Groovy: .map({ values -> ConvertibleValues.of(values) })
It seems like you have most of that worked out, however you specifically asked about the map not being used. That is because you simply aren't passing it to the method.
values.reduce({all, keyValue->
vs
values.reduce(map, {all, keyValue ->
Thank you James. Will give this a try a bit later when I get time.
– Vahid
Nov 17 '18 at 11:52
1
.map(ConvertibleValues::of)
actually corresponds to.map(ConvertibleValues.&of)
in groovy
– injecteer
Nov 20 '18 at 14:26
@injecteer Yes that also works
– James Kleeh
Nov 20 '18 at 17:21
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53324472%2fmicronaut-petstore-a-code-segment-from-java-to-groovy%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The likely issue you're facing is because Groovy doesn't support Java method references or lambdas.
The first line is returning a lambda
Java: return key -> {
Groovy: return { key - >
That is using a groovy closure that takes the key as the argument.
Other places that use method references need to be converted
Java: .map(ConvertibleValues::of)
Groovy: .map({ values -> ConvertibleValues.of(values) })
It seems like you have most of that worked out, however you specifically asked about the map not being used. That is because you simply aren't passing it to the method.
values.reduce({all, keyValue->
vs
values.reduce(map, {all, keyValue ->
Thank you James. Will give this a try a bit later when I get time.
– Vahid
Nov 17 '18 at 11:52
1
.map(ConvertibleValues::of)
actually corresponds to.map(ConvertibleValues.&of)
in groovy
– injecteer
Nov 20 '18 at 14:26
@injecteer Yes that also works
– James Kleeh
Nov 20 '18 at 17:21
add a comment |
The likely issue you're facing is because Groovy doesn't support Java method references or lambdas.
The first line is returning a lambda
Java: return key -> {
Groovy: return { key - >
That is using a groovy closure that takes the key as the argument.
Other places that use method references need to be converted
Java: .map(ConvertibleValues::of)
Groovy: .map({ values -> ConvertibleValues.of(values) })
It seems like you have most of that worked out, however you specifically asked about the map not being used. That is because you simply aren't passing it to the method.
values.reduce({all, keyValue->
vs
values.reduce(map, {all, keyValue ->
Thank you James. Will give this a try a bit later when I get time.
– Vahid
Nov 17 '18 at 11:52
1
.map(ConvertibleValues::of)
actually corresponds to.map(ConvertibleValues.&of)
in groovy
– injecteer
Nov 20 '18 at 14:26
@injecteer Yes that also works
– James Kleeh
Nov 20 '18 at 17:21
add a comment |
The likely issue you're facing is because Groovy doesn't support Java method references or lambdas.
The first line is returning a lambda
Java: return key -> {
Groovy: return { key - >
That is using a groovy closure that takes the key as the argument.
Other places that use method references need to be converted
Java: .map(ConvertibleValues::of)
Groovy: .map({ values -> ConvertibleValues.of(values) })
It seems like you have most of that worked out, however you specifically asked about the map not being used. That is because you simply aren't passing it to the method.
values.reduce({all, keyValue->
vs
values.reduce(map, {all, keyValue ->
The likely issue you're facing is because Groovy doesn't support Java method references or lambdas.
The first line is returning a lambda
Java: return key -> {
Groovy: return { key - >
That is using a groovy closure that takes the key as the argument.
Other places that use method references need to be converted
Java: .map(ConvertibleValues::of)
Groovy: .map({ values -> ConvertibleValues.of(values) })
It seems like you have most of that worked out, however you specifically asked about the map not being used. That is because you simply aren't passing it to the method.
values.reduce({all, keyValue->
vs
values.reduce(map, {all, keyValue ->
answered Nov 16 '18 at 21:39
James KleehJames Kleeh
8,65942452
8,65942452
Thank you James. Will give this a try a bit later when I get time.
– Vahid
Nov 17 '18 at 11:52
1
.map(ConvertibleValues::of)
actually corresponds to.map(ConvertibleValues.&of)
in groovy
– injecteer
Nov 20 '18 at 14:26
@injecteer Yes that also works
– James Kleeh
Nov 20 '18 at 17:21
add a comment |
Thank you James. Will give this a try a bit later when I get time.
– Vahid
Nov 17 '18 at 11:52
1
.map(ConvertibleValues::of)
actually corresponds to.map(ConvertibleValues.&of)
in groovy
– injecteer
Nov 20 '18 at 14:26
@injecteer Yes that also works
– James Kleeh
Nov 20 '18 at 17:21
Thank you James. Will give this a try a bit later when I get time.
– Vahid
Nov 17 '18 at 11:52
Thank you James. Will give this a try a bit later when I get time.
– Vahid
Nov 17 '18 at 11:52
1
1
.map(ConvertibleValues::of)
actually corresponds to .map(ConvertibleValues.&of)
in groovy– injecteer
Nov 20 '18 at 14:26
.map(ConvertibleValues::of)
actually corresponds to .map(ConvertibleValues.&of)
in groovy– injecteer
Nov 20 '18 at 14:26
@injecteer Yes that also works
– James Kleeh
Nov 20 '18 at 17:21
@injecteer Yes that also works
– James Kleeh
Nov 20 '18 at 17:21
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53324472%2fmicronaut-petstore-a-code-segment-from-java-to-groovy%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
nL4TaYwpcTDsy4x92BoOfjOl5ohk d,UUFVe,4ezeXSpvx3MJ,B3PEuA6lIvYpibIShpLLKAP6mWepWrRn N y6QJB,Q,t4sleRG,cGY7
I'm not sure what is the question here - do you want us to re-write this class for you?
– Iłya Bursov
Nov 15 '18 at 17:01
Hi llya In short if above can be converted from java to groovy that would be great github.com/vahidhedayati/micronaut-java-groovy-comparison/blob/… - the first link is what I am trying from this code github.com/vahidhedayati/micronaut-java-groovy-comparison/blob/… - Simply trying to understand some of the more complex rxjava statements in java and how to do same under groovy
– Vahid
Nov 15 '18 at 17:03
3
idownvotedbecau.se/itsnotworking
– Iłya Bursov
Nov 15 '18 at 17:07
okay thank you for your input
– Vahid
Nov 15 '18 at 17:09
If you have failing code, then please add your attempt and what errors you get to the question. An potential answer can work from that then.
– cfrick
Nov 15 '18 at 20:03