Extend AspNetCore.Identity.EntityFrameworkCore.UserStore (V2.1.3)
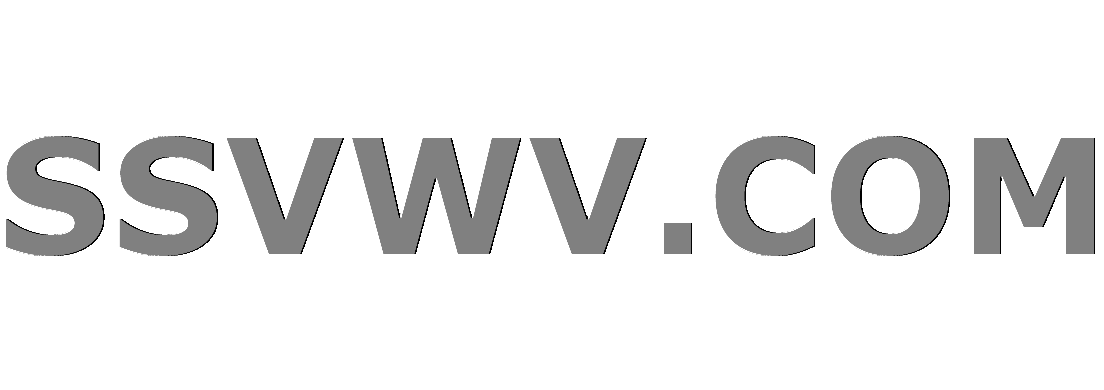
Multi tool use
I simply want to extend the userstore and add extra method. I'm not able to define the correct constructor the customuserstore. How do I define constructor
public class MyCustomUserStore<TUser> : Microsoft.AspNetCore.Identity.EntityFrameworkCore.UserStore<TUser>, IUserLdapStore<TUser>
where TUser : class
{
// constructor goes here
public Task<string> GetDistinguishedNameAsync(TUser user)
{
return Task.FromResult(string.Empty);
}
}
public interface IUserLdapStore<TUser>
where TUser : class
{
/// <summary>
/// When implemented in a derived class, gets the DN that should be used to attempt an LDAP bind for validatio of a user's password.
/// </summary>
/// <param name="user"></param>
/// <returns></returns>
Task<string> GetDistinguishedNameAsync(TUser user);
}
Getting following errors:
The type '`TUser' cannot be used as type parameter 'TUser' in the generic type or method 'UserStore'.
There is no implicit reference conversion from 'TUser' to 'Microsoft.AspNetCore.Identity.IdentityUser'.
'TUser' must be a non-abstract type with a public parameterless constructor in order to use it as parameter 'TUser' in the generic type or method 'UserStore
There is no argument given that corresponds to the required formal parameter 'context' of 'UserStore.UserStore(DbContext, IdentityErrorDescriber)'
c# asp.net-core asp.net-core-identity
add a comment |
I simply want to extend the userstore and add extra method. I'm not able to define the correct constructor the customuserstore. How do I define constructor
public class MyCustomUserStore<TUser> : Microsoft.AspNetCore.Identity.EntityFrameworkCore.UserStore<TUser>, IUserLdapStore<TUser>
where TUser : class
{
// constructor goes here
public Task<string> GetDistinguishedNameAsync(TUser user)
{
return Task.FromResult(string.Empty);
}
}
public interface IUserLdapStore<TUser>
where TUser : class
{
/// <summary>
/// When implemented in a derived class, gets the DN that should be used to attempt an LDAP bind for validatio of a user's password.
/// </summary>
/// <param name="user"></param>
/// <returns></returns>
Task<string> GetDistinguishedNameAsync(TUser user);
}
Getting following errors:
The type '`TUser' cannot be used as type parameter 'TUser' in the generic type or method 'UserStore'.
There is no implicit reference conversion from 'TUser' to 'Microsoft.AspNetCore.Identity.IdentityUser'.
'TUser' must be a non-abstract type with a public parameterless constructor in order to use it as parameter 'TUser' in the generic type or method 'UserStore
There is no argument given that corresponds to the required formal parameter 'context' of 'UserStore.UserStore(DbContext, IdentityErrorDescriber)'
c# asp.net-core asp.net-core-identity
add a comment |
I simply want to extend the userstore and add extra method. I'm not able to define the correct constructor the customuserstore. How do I define constructor
public class MyCustomUserStore<TUser> : Microsoft.AspNetCore.Identity.EntityFrameworkCore.UserStore<TUser>, IUserLdapStore<TUser>
where TUser : class
{
// constructor goes here
public Task<string> GetDistinguishedNameAsync(TUser user)
{
return Task.FromResult(string.Empty);
}
}
public interface IUserLdapStore<TUser>
where TUser : class
{
/// <summary>
/// When implemented in a derived class, gets the DN that should be used to attempt an LDAP bind for validatio of a user's password.
/// </summary>
/// <param name="user"></param>
/// <returns></returns>
Task<string> GetDistinguishedNameAsync(TUser user);
}
Getting following errors:
The type '`TUser' cannot be used as type parameter 'TUser' in the generic type or method 'UserStore'.
There is no implicit reference conversion from 'TUser' to 'Microsoft.AspNetCore.Identity.IdentityUser'.
'TUser' must be a non-abstract type with a public parameterless constructor in order to use it as parameter 'TUser' in the generic type or method 'UserStore
There is no argument given that corresponds to the required formal parameter 'context' of 'UserStore.UserStore(DbContext, IdentityErrorDescriber)'
c# asp.net-core asp.net-core-identity
I simply want to extend the userstore and add extra method. I'm not able to define the correct constructor the customuserstore. How do I define constructor
public class MyCustomUserStore<TUser> : Microsoft.AspNetCore.Identity.EntityFrameworkCore.UserStore<TUser>, IUserLdapStore<TUser>
where TUser : class
{
// constructor goes here
public Task<string> GetDistinguishedNameAsync(TUser user)
{
return Task.FromResult(string.Empty);
}
}
public interface IUserLdapStore<TUser>
where TUser : class
{
/// <summary>
/// When implemented in a derived class, gets the DN that should be used to attempt an LDAP bind for validatio of a user's password.
/// </summary>
/// <param name="user"></param>
/// <returns></returns>
Task<string> GetDistinguishedNameAsync(TUser user);
}
Getting following errors:
The type '`TUser' cannot be used as type parameter 'TUser' in the generic type or method 'UserStore'.
There is no implicit reference conversion from 'TUser' to 'Microsoft.AspNetCore.Identity.IdentityUser'.
'TUser' must be a non-abstract type with a public parameterless constructor in order to use it as parameter 'TUser' in the generic type or method 'UserStore
There is no argument given that corresponds to the required formal parameter 'context' of 'UserStore.UserStore(DbContext, IdentityErrorDescriber)'
c# asp.net-core asp.net-core-identity
c# asp.net-core asp.net-core-identity
edited Nov 15 '18 at 17:36
Kirk Larkin
22k64262
22k64262
asked Nov 15 '18 at 17:01
Abu SufyanAbu Sufyan
6
6
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Going through the error message carefully you seem to be missing to add the constraint for TUser
that forces it to inherit from IdentityUser<string>
. This is an inherited constraint from Microsoft.AspNetCore.Identity.EntityFrameworkCore.UserStore<TUser>
.
Adding that constraint to your custom store is something like:
public class MyCustomUserStore<TUser> : Microsoft.AspNetCore.Identity.EntityFrameworkCore.UserStore<TUser>, IUserLdapStore<TUser>
where TUser : IdentityUser<string>, new()
{
//constructor goes here....
public Task<string> GetDistinguishedNameAsync(TUser user)
{
return Task.FromResult(string.Empty);
}
}
Thanks to @KirkLarkin for pointing the second error I completely overlooked.
There is no argument given that corresponds to the required formal parameter 'context' of 'UserStore.UserStore(DbContext, IdentityErrorDescriber)'
The error is a little cryptic, but it's easily solvable if we provide a constructor for the custom store providing the parameters for the base class:
public MyCustomUserStore(DbContext context, IdentityErrorDescriber describer = null) : base(context, describer)
{
}
Putting all these together, the class should look like this:
public class MyCustomUserStore<TUser> : Microsoft.AspNetCore.Identity.EntityFrameworkCore.UserStore<TUser>, IUserLdapStore<TUser>
where TUser : IdentityUser<string>, new()
{
public MyCustomUserStore(DbContext context, IdentityErrorDescriber describer = null) : base(context, describer)
{
}
public Task<string> GetDistinguishedNameAsync(TUser user)
{
return Task.FromResult(string.Empty);
}
}
Hope this helps!
My TUser is defined as this: public class ApplicationUser : IdentityUser<int>
– Abu Sufyan
Nov 20 '18 at 17:04
@user10658716 if that's the case, it seems that you'll have to do a lot more than just inheriting fromIdentityUser<int>
. You should definitely take a look at these: docs.microsoft.com/en-us/aspnet/core/security/authentication/…, johnatten.com/2014/07/13/….
– Karel Tamayo
Nov 20 '18 at 17:33
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53324505%2fextend-aspnetcore-identity-entityframeworkcore-userstore-v2-1-3%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Going through the error message carefully you seem to be missing to add the constraint for TUser
that forces it to inherit from IdentityUser<string>
. This is an inherited constraint from Microsoft.AspNetCore.Identity.EntityFrameworkCore.UserStore<TUser>
.
Adding that constraint to your custom store is something like:
public class MyCustomUserStore<TUser> : Microsoft.AspNetCore.Identity.EntityFrameworkCore.UserStore<TUser>, IUserLdapStore<TUser>
where TUser : IdentityUser<string>, new()
{
//constructor goes here....
public Task<string> GetDistinguishedNameAsync(TUser user)
{
return Task.FromResult(string.Empty);
}
}
Thanks to @KirkLarkin for pointing the second error I completely overlooked.
There is no argument given that corresponds to the required formal parameter 'context' of 'UserStore.UserStore(DbContext, IdentityErrorDescriber)'
The error is a little cryptic, but it's easily solvable if we provide a constructor for the custom store providing the parameters for the base class:
public MyCustomUserStore(DbContext context, IdentityErrorDescriber describer = null) : base(context, describer)
{
}
Putting all these together, the class should look like this:
public class MyCustomUserStore<TUser> : Microsoft.AspNetCore.Identity.EntityFrameworkCore.UserStore<TUser>, IUserLdapStore<TUser>
where TUser : IdentityUser<string>, new()
{
public MyCustomUserStore(DbContext context, IdentityErrorDescriber describer = null) : base(context, describer)
{
}
public Task<string> GetDistinguishedNameAsync(TUser user)
{
return Task.FromResult(string.Empty);
}
}
Hope this helps!
My TUser is defined as this: public class ApplicationUser : IdentityUser<int>
– Abu Sufyan
Nov 20 '18 at 17:04
@user10658716 if that's the case, it seems that you'll have to do a lot more than just inheriting fromIdentityUser<int>
. You should definitely take a look at these: docs.microsoft.com/en-us/aspnet/core/security/authentication/…, johnatten.com/2014/07/13/….
– Karel Tamayo
Nov 20 '18 at 17:33
add a comment |
Going through the error message carefully you seem to be missing to add the constraint for TUser
that forces it to inherit from IdentityUser<string>
. This is an inherited constraint from Microsoft.AspNetCore.Identity.EntityFrameworkCore.UserStore<TUser>
.
Adding that constraint to your custom store is something like:
public class MyCustomUserStore<TUser> : Microsoft.AspNetCore.Identity.EntityFrameworkCore.UserStore<TUser>, IUserLdapStore<TUser>
where TUser : IdentityUser<string>, new()
{
//constructor goes here....
public Task<string> GetDistinguishedNameAsync(TUser user)
{
return Task.FromResult(string.Empty);
}
}
Thanks to @KirkLarkin for pointing the second error I completely overlooked.
There is no argument given that corresponds to the required formal parameter 'context' of 'UserStore.UserStore(DbContext, IdentityErrorDescriber)'
The error is a little cryptic, but it's easily solvable if we provide a constructor for the custom store providing the parameters for the base class:
public MyCustomUserStore(DbContext context, IdentityErrorDescriber describer = null) : base(context, describer)
{
}
Putting all these together, the class should look like this:
public class MyCustomUserStore<TUser> : Microsoft.AspNetCore.Identity.EntityFrameworkCore.UserStore<TUser>, IUserLdapStore<TUser>
where TUser : IdentityUser<string>, new()
{
public MyCustomUserStore(DbContext context, IdentityErrorDescriber describer = null) : base(context, describer)
{
}
public Task<string> GetDistinguishedNameAsync(TUser user)
{
return Task.FromResult(string.Empty);
}
}
Hope this helps!
My TUser is defined as this: public class ApplicationUser : IdentityUser<int>
– Abu Sufyan
Nov 20 '18 at 17:04
@user10658716 if that's the case, it seems that you'll have to do a lot more than just inheriting fromIdentityUser<int>
. You should definitely take a look at these: docs.microsoft.com/en-us/aspnet/core/security/authentication/…, johnatten.com/2014/07/13/….
– Karel Tamayo
Nov 20 '18 at 17:33
add a comment |
Going through the error message carefully you seem to be missing to add the constraint for TUser
that forces it to inherit from IdentityUser<string>
. This is an inherited constraint from Microsoft.AspNetCore.Identity.EntityFrameworkCore.UserStore<TUser>
.
Adding that constraint to your custom store is something like:
public class MyCustomUserStore<TUser> : Microsoft.AspNetCore.Identity.EntityFrameworkCore.UserStore<TUser>, IUserLdapStore<TUser>
where TUser : IdentityUser<string>, new()
{
//constructor goes here....
public Task<string> GetDistinguishedNameAsync(TUser user)
{
return Task.FromResult(string.Empty);
}
}
Thanks to @KirkLarkin for pointing the second error I completely overlooked.
There is no argument given that corresponds to the required formal parameter 'context' of 'UserStore.UserStore(DbContext, IdentityErrorDescriber)'
The error is a little cryptic, but it's easily solvable if we provide a constructor for the custom store providing the parameters for the base class:
public MyCustomUserStore(DbContext context, IdentityErrorDescriber describer = null) : base(context, describer)
{
}
Putting all these together, the class should look like this:
public class MyCustomUserStore<TUser> : Microsoft.AspNetCore.Identity.EntityFrameworkCore.UserStore<TUser>, IUserLdapStore<TUser>
where TUser : IdentityUser<string>, new()
{
public MyCustomUserStore(DbContext context, IdentityErrorDescriber describer = null) : base(context, describer)
{
}
public Task<string> GetDistinguishedNameAsync(TUser user)
{
return Task.FromResult(string.Empty);
}
}
Hope this helps!
Going through the error message carefully you seem to be missing to add the constraint for TUser
that forces it to inherit from IdentityUser<string>
. This is an inherited constraint from Microsoft.AspNetCore.Identity.EntityFrameworkCore.UserStore<TUser>
.
Adding that constraint to your custom store is something like:
public class MyCustomUserStore<TUser> : Microsoft.AspNetCore.Identity.EntityFrameworkCore.UserStore<TUser>, IUserLdapStore<TUser>
where TUser : IdentityUser<string>, new()
{
//constructor goes here....
public Task<string> GetDistinguishedNameAsync(TUser user)
{
return Task.FromResult(string.Empty);
}
}
Thanks to @KirkLarkin for pointing the second error I completely overlooked.
There is no argument given that corresponds to the required formal parameter 'context' of 'UserStore.UserStore(DbContext, IdentityErrorDescriber)'
The error is a little cryptic, but it's easily solvable if we provide a constructor for the custom store providing the parameters for the base class:
public MyCustomUserStore(DbContext context, IdentityErrorDescriber describer = null) : base(context, describer)
{
}
Putting all these together, the class should look like this:
public class MyCustomUserStore<TUser> : Microsoft.AspNetCore.Identity.EntityFrameworkCore.UserStore<TUser>, IUserLdapStore<TUser>
where TUser : IdentityUser<string>, new()
{
public MyCustomUserStore(DbContext context, IdentityErrorDescriber describer = null) : base(context, describer)
{
}
public Task<string> GetDistinguishedNameAsync(TUser user)
{
return Task.FromResult(string.Empty);
}
}
Hope this helps!
edited Nov 15 '18 at 17:43
answered Nov 15 '18 at 17:23


Karel TamayoKarel Tamayo
2,79721523
2,79721523
My TUser is defined as this: public class ApplicationUser : IdentityUser<int>
– Abu Sufyan
Nov 20 '18 at 17:04
@user10658716 if that's the case, it seems that you'll have to do a lot more than just inheriting fromIdentityUser<int>
. You should definitely take a look at these: docs.microsoft.com/en-us/aspnet/core/security/authentication/…, johnatten.com/2014/07/13/….
– Karel Tamayo
Nov 20 '18 at 17:33
add a comment |
My TUser is defined as this: public class ApplicationUser : IdentityUser<int>
– Abu Sufyan
Nov 20 '18 at 17:04
@user10658716 if that's the case, it seems that you'll have to do a lot more than just inheriting fromIdentityUser<int>
. You should definitely take a look at these: docs.microsoft.com/en-us/aspnet/core/security/authentication/…, johnatten.com/2014/07/13/….
– Karel Tamayo
Nov 20 '18 at 17:33
My TUser is defined as this: public class ApplicationUser : IdentityUser<int>
– Abu Sufyan
Nov 20 '18 at 17:04
My TUser is defined as this: public class ApplicationUser : IdentityUser<int>
– Abu Sufyan
Nov 20 '18 at 17:04
@user10658716 if that's the case, it seems that you'll have to do a lot more than just inheriting from
IdentityUser<int>
. You should definitely take a look at these: docs.microsoft.com/en-us/aspnet/core/security/authentication/…, johnatten.com/2014/07/13/….– Karel Tamayo
Nov 20 '18 at 17:33
@user10658716 if that's the case, it seems that you'll have to do a lot more than just inheriting from
IdentityUser<int>
. You should definitely take a look at these: docs.microsoft.com/en-us/aspnet/core/security/authentication/…, johnatten.com/2014/07/13/….– Karel Tamayo
Nov 20 '18 at 17:33
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53324505%2fextend-aspnetcore-identity-entityframeworkcore-userstore-v2-1-3%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
2LK,7mZO15qtBkhn,UxzCOq,SWHPrvfvVn2PVFg