Creating google api credentials (from service account with scope and delegated account) with oauth2client
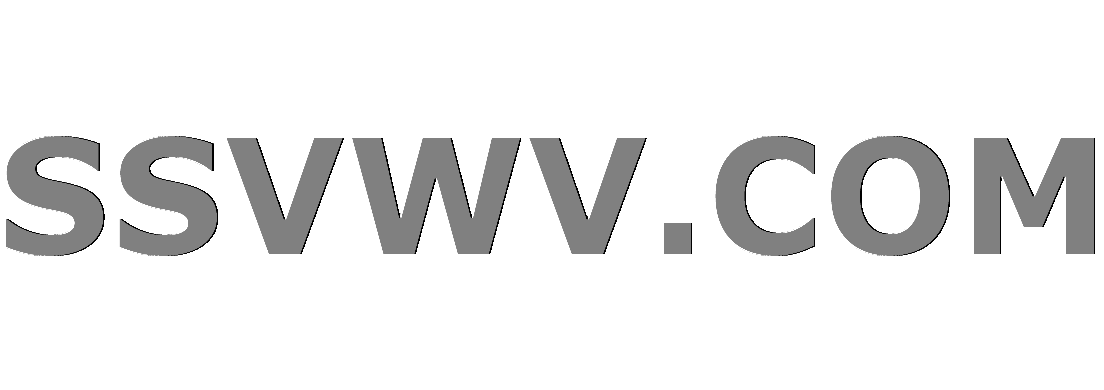
Multi tool use
To access GMail API (and personify calls) I'm using a service account (created from Google Cloud Platform). The json file I have looks like this
{
"type": "service_account",
"project_id": "[PROJECT-ID]",
"private_key_id": "[KEY-ID]"
"private_key": "-----BEGIN PRIVATE KEY-----n[PRIVATE-KEY]n-----END PRIVATE KEY-----n",
"client_email": "[SERVICE-ACCOUNT-EMAIL]",
"client_id": "[CLIENT-ID]",
"auth_uri": "https://accounts.google.com/o/oauth2/auth",
"token_uri": "https://accounts.google.com/o/oauth2/token",
"auth_provider_x509_cert_url": "https://www.googleapis.com/oauth2/v1/certs",
"client_x509_cert_url": "https://www.googleapis.com/robot/v1/metadata/x509/[SERVICE-ACCOUNT-EMAIL]"
}
I'm also using the oauth2client library to make it easier but I can't find a way to create the credentials and then specify a scope and a delegated account.
I tried
from oauth2client import service_account
self._credentials = service_account.ServiceAccountCredentials.from_json(SERVICE_ACCOUNT_JSON_CONTENT)
self._credentials = self._credentials.create_scoped([u'https://www.googleapis.com/auth/gmail.send'])
self._credentials = self._credentials.create_delegated(MY_USER)
self._client = discovery.build(u'gmail', u'v1', credentials=self._credentials)
But I get an error cause it expects a PKCS-8 key.
How can I do that ? (My code runs on App Engine Flex if that helps)
Thanks
google-oauth2 service-accounts app-engine-flexible oauth2client
add a comment |
To access GMail API (and personify calls) I'm using a service account (created from Google Cloud Platform). The json file I have looks like this
{
"type": "service_account",
"project_id": "[PROJECT-ID]",
"private_key_id": "[KEY-ID]"
"private_key": "-----BEGIN PRIVATE KEY-----n[PRIVATE-KEY]n-----END PRIVATE KEY-----n",
"client_email": "[SERVICE-ACCOUNT-EMAIL]",
"client_id": "[CLIENT-ID]",
"auth_uri": "https://accounts.google.com/o/oauth2/auth",
"token_uri": "https://accounts.google.com/o/oauth2/token",
"auth_provider_x509_cert_url": "https://www.googleapis.com/oauth2/v1/certs",
"client_x509_cert_url": "https://www.googleapis.com/robot/v1/metadata/x509/[SERVICE-ACCOUNT-EMAIL]"
}
I'm also using the oauth2client library to make it easier but I can't find a way to create the credentials and then specify a scope and a delegated account.
I tried
from oauth2client import service_account
self._credentials = service_account.ServiceAccountCredentials.from_json(SERVICE_ACCOUNT_JSON_CONTENT)
self._credentials = self._credentials.create_scoped([u'https://www.googleapis.com/auth/gmail.send'])
self._credentials = self._credentials.create_delegated(MY_USER)
self._client = discovery.build(u'gmail', u'v1', credentials=self._credentials)
But I get an error cause it expects a PKCS-8 key.
How can I do that ? (My code runs on App Engine Flex if that helps)
Thanks
google-oauth2 service-accounts app-engine-flexible oauth2client
add a comment |
To access GMail API (and personify calls) I'm using a service account (created from Google Cloud Platform). The json file I have looks like this
{
"type": "service_account",
"project_id": "[PROJECT-ID]",
"private_key_id": "[KEY-ID]"
"private_key": "-----BEGIN PRIVATE KEY-----n[PRIVATE-KEY]n-----END PRIVATE KEY-----n",
"client_email": "[SERVICE-ACCOUNT-EMAIL]",
"client_id": "[CLIENT-ID]",
"auth_uri": "https://accounts.google.com/o/oauth2/auth",
"token_uri": "https://accounts.google.com/o/oauth2/token",
"auth_provider_x509_cert_url": "https://www.googleapis.com/oauth2/v1/certs",
"client_x509_cert_url": "https://www.googleapis.com/robot/v1/metadata/x509/[SERVICE-ACCOUNT-EMAIL]"
}
I'm also using the oauth2client library to make it easier but I can't find a way to create the credentials and then specify a scope and a delegated account.
I tried
from oauth2client import service_account
self._credentials = service_account.ServiceAccountCredentials.from_json(SERVICE_ACCOUNT_JSON_CONTENT)
self._credentials = self._credentials.create_scoped([u'https://www.googleapis.com/auth/gmail.send'])
self._credentials = self._credentials.create_delegated(MY_USER)
self._client = discovery.build(u'gmail', u'v1', credentials=self._credentials)
But I get an error cause it expects a PKCS-8 key.
How can I do that ? (My code runs on App Engine Flex if that helps)
Thanks
google-oauth2 service-accounts app-engine-flexible oauth2client
To access GMail API (and personify calls) I'm using a service account (created from Google Cloud Platform). The json file I have looks like this
{
"type": "service_account",
"project_id": "[PROJECT-ID]",
"private_key_id": "[KEY-ID]"
"private_key": "-----BEGIN PRIVATE KEY-----n[PRIVATE-KEY]n-----END PRIVATE KEY-----n",
"client_email": "[SERVICE-ACCOUNT-EMAIL]",
"client_id": "[CLIENT-ID]",
"auth_uri": "https://accounts.google.com/o/oauth2/auth",
"token_uri": "https://accounts.google.com/o/oauth2/token",
"auth_provider_x509_cert_url": "https://www.googleapis.com/oauth2/v1/certs",
"client_x509_cert_url": "https://www.googleapis.com/robot/v1/metadata/x509/[SERVICE-ACCOUNT-EMAIL]"
}
I'm also using the oauth2client library to make it easier but I can't find a way to create the credentials and then specify a scope and a delegated account.
I tried
from oauth2client import service_account
self._credentials = service_account.ServiceAccountCredentials.from_json(SERVICE_ACCOUNT_JSON_CONTENT)
self._credentials = self._credentials.create_scoped([u'https://www.googleapis.com/auth/gmail.send'])
self._credentials = self._credentials.create_delegated(MY_USER)
self._client = discovery.build(u'gmail', u'v1', credentials=self._credentials)
But I get an error cause it expects a PKCS-8 key.
How can I do that ? (My code runs on App Engine Flex if that helps)
Thanks
google-oauth2 service-accounts app-engine-flexible oauth2client
google-oauth2 service-accounts app-engine-flexible oauth2client
asked Nov 15 '18 at 13:26


Valentin CoudertValentin Coudert
598519
598519
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Finally, since oauth2client is now deprecated in favor of google-auth, I did
from googleapiclient import discovery
from google.oauth2.service_account import Credentials
credentials = Credentials.from_service_account_file(PATH_TO_SERVICE_ACCOUNT_JSON,
scopes=[u'https://www.googleapis.com/auth/gmail.send'])
delegated_credentials = self._credentials.with_subject(MY_USER)
client = discovery.build(u'gmail', u'v1', credentials=delegated_credentials)
and it worked ;-)
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53320537%2fcreating-google-api-credentials-from-service-account-with-scope-and-delegated-a%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Finally, since oauth2client is now deprecated in favor of google-auth, I did
from googleapiclient import discovery
from google.oauth2.service_account import Credentials
credentials = Credentials.from_service_account_file(PATH_TO_SERVICE_ACCOUNT_JSON,
scopes=[u'https://www.googleapis.com/auth/gmail.send'])
delegated_credentials = self._credentials.with_subject(MY_USER)
client = discovery.build(u'gmail', u'v1', credentials=delegated_credentials)
and it worked ;-)
add a comment |
Finally, since oauth2client is now deprecated in favor of google-auth, I did
from googleapiclient import discovery
from google.oauth2.service_account import Credentials
credentials = Credentials.from_service_account_file(PATH_TO_SERVICE_ACCOUNT_JSON,
scopes=[u'https://www.googleapis.com/auth/gmail.send'])
delegated_credentials = self._credentials.with_subject(MY_USER)
client = discovery.build(u'gmail', u'v1', credentials=delegated_credentials)
and it worked ;-)
add a comment |
Finally, since oauth2client is now deprecated in favor of google-auth, I did
from googleapiclient import discovery
from google.oauth2.service_account import Credentials
credentials = Credentials.from_service_account_file(PATH_TO_SERVICE_ACCOUNT_JSON,
scopes=[u'https://www.googleapis.com/auth/gmail.send'])
delegated_credentials = self._credentials.with_subject(MY_USER)
client = discovery.build(u'gmail', u'v1', credentials=delegated_credentials)
and it worked ;-)
Finally, since oauth2client is now deprecated in favor of google-auth, I did
from googleapiclient import discovery
from google.oauth2.service_account import Credentials
credentials = Credentials.from_service_account_file(PATH_TO_SERVICE_ACCOUNT_JSON,
scopes=[u'https://www.googleapis.com/auth/gmail.send'])
delegated_credentials = self._credentials.with_subject(MY_USER)
client = discovery.build(u'gmail', u'v1', credentials=delegated_credentials)
and it worked ;-)
answered Nov 15 '18 at 14:48


Valentin CoudertValentin Coudert
598519
598519
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53320537%2fcreating-google-api-credentials-from-service-account-with-scope-and-delegated-a%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
XZGq,20,8IWvg0rHegWpKeejdRBsy8g 6ZzACOGOH V4xh p353