c++ open gl shader failed compilation
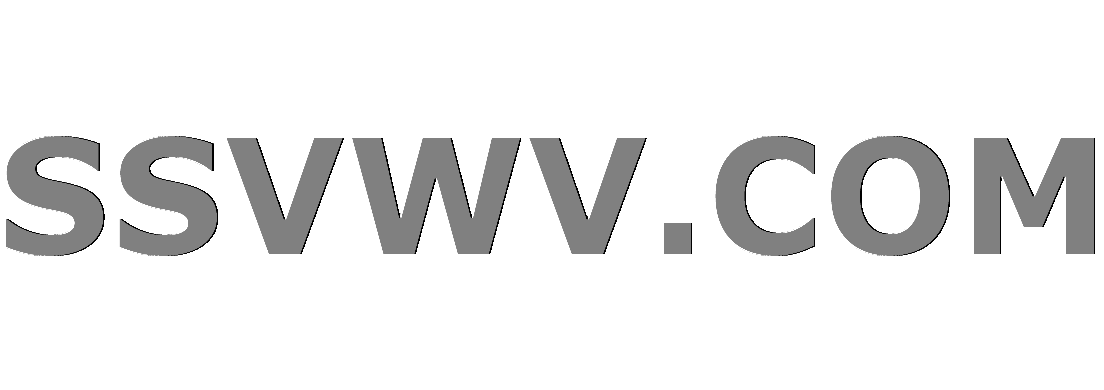
Multi tool use
Im trying to learn a gl in cpp and trying to create some shaders reading its documentation and following a yt tutorial.
It just had a compilation fail.
yestarday it was just printing in console Shader " + filePath + " failed to Compile
Today it printing that :
ERROR: 0:7: 'gl_position' : undeclared identifier
ERROR: 0:7: 'xy' : field selection requires structure, vector, or matrix on left
hand side
ERROR: 0:7: 'assign' : cannot convert from '2-component vector of highp float'
to 'highp float'
ERROR: 0:8: 'z' : field selection requires structure, vector, or matrix on left
hand side
ERROR: 0:9: 'w' : field selection requires structure, vector, or matrix on left
hand side
Shader shaders/colorShading.vert failed to Compile
Enter any key to quit...
this is my shader source code:
#version 130
in vec2 vertexPosition;
void main()
{
gl_position.xy = vertexPosition * 2;
gl_position.z = 0.0;
gl_position.w = 1.0;
}
//////////////////////////////////////////////////////
#include "GLSLProgram.h"
#include "Error.h"
#include <fstream>
#include <vector>
GLSLProgram::GLSLProgram() :_numAttributes(0), _programID(0), _vertexShaderID(0), _fragmentShaderID(0)
{
}
GLSLProgram::~GLSLProgram()
{
}
void GLSLProgram::compileShaders(const std::string& vertexShaderFilePath, const std::string& framgmentShaderFilePath)
{
_vertexShaderID = glCreateShader(GL_VERTEX_SHADER);
if (_vertexShaderID == 0)
{
fatalError("Vertex shader failed to be created!");
}
_fragmentShaderID = glCreateShader(GL_FRAGMENT_SHADER);
if (_fragmentShaderID == 0)
{
fatalError("Fragment shader failed to be created!");
}
compileShader(vertexShaderFilePath, _vertexShaderID);
compileShader(framgmentShaderFilePath, _fragmentShaderID);
}
void GLSLProgram::linkShaders()
{
// Attach Shaders to Program
glAttachShader(_programID, _vertexShaderID);
glAttachShader(_programID, _fragmentShaderID);
// Link the Program.
glLinkProgram(_programID);
GLuint isLinked = 0;
glGetProgramiv(_programID, GL_LINK_STATUS, (int *)&isLinked);
if (GL_FALSE == isLinked) {
GLint maxLength = 0;
glGetProgramiv(_programID, GL_INFO_LOG_LENGTH, &maxLength);
std::vector<char> errorLog(maxLength);
glGetProgramInfoLog(_programID, maxLength, &maxLength, &errorLog[0]);
glDeleteProgram(_programID);
glDeleteShader(_vertexShaderID);
glDeleteShader(_fragmentShaderID);
std::printf("%sn", &(errorLog[0]));
fatalError("Shaders failed to link!");
return;
}
glDetachShader(_programID, _vertexShaderID);
glDetachShader(_programID, _fragmentShaderID);
glDeleteShader(_vertexShaderID);
glDeleteShader(_fragmentShaderID);
}
void GLSLProgram::addAttribute(const std::string& attributeName)
{
glBindAttribLocation(_programID, _numAttributes++, attributeName.c_str());
}
void GLSLProgram::use()
{
glUseProgram(_programID);
for (int i = 0; i < _numAttributes; i++)
{
glEnableVertexAttribArray(i);
}
}
void GLSLProgram::unuse()
{
glUseProgram(0);
for (int i = 0; i < _numAttributes; i++)
{
glDisableVertexAttribArray(i);
}
}
void GLSLProgram::compileShader(const std::string & filePath, GLuint& id)
{
std::ifstream fileptr(filePath);
if (fileptr.fail()) {
// Not sold on this
perror(filePath.c_str());
fatalError("Failed to Open " + filePath);
}
std::string fileContents = "";
std::string line;
while (std::getline(fileptr, line))
{
fileContents += line + "n";
}
fileptr.close();
// This is weird
const GLchar * contentsPtr = fileContents.c_str();
glShaderSource(id, 1, &contentsPtr, 0);
glCompileShader(id);
GLint success = 0;
glGetShaderiv(id, GL_COMPILE_STATUS, &success);
if (success == GL_FALSE) {
GLint maxLength = 0;
glGetShaderiv(id, GL_INFO_LOG_LENGTH, &maxLength);
std::vector<GLchar> errorLog(maxLength);
glGetShaderInfoLog(id, maxLength, &maxLength, &errorLog[0]);
glDeleteShader(id);
std::printf("%sn", &(errorLog[0]));
fatalError("Shader " + filePath + " failed to Compile");
}
}
c++ glsl shader
add a comment |
Im trying to learn a gl in cpp and trying to create some shaders reading its documentation and following a yt tutorial.
It just had a compilation fail.
yestarday it was just printing in console Shader " + filePath + " failed to Compile
Today it printing that :
ERROR: 0:7: 'gl_position' : undeclared identifier
ERROR: 0:7: 'xy' : field selection requires structure, vector, or matrix on left
hand side
ERROR: 0:7: 'assign' : cannot convert from '2-component vector of highp float'
to 'highp float'
ERROR: 0:8: 'z' : field selection requires structure, vector, or matrix on left
hand side
ERROR: 0:9: 'w' : field selection requires structure, vector, or matrix on left
hand side
Shader shaders/colorShading.vert failed to Compile
Enter any key to quit...
this is my shader source code:
#version 130
in vec2 vertexPosition;
void main()
{
gl_position.xy = vertexPosition * 2;
gl_position.z = 0.0;
gl_position.w = 1.0;
}
//////////////////////////////////////////////////////
#include "GLSLProgram.h"
#include "Error.h"
#include <fstream>
#include <vector>
GLSLProgram::GLSLProgram() :_numAttributes(0), _programID(0), _vertexShaderID(0), _fragmentShaderID(0)
{
}
GLSLProgram::~GLSLProgram()
{
}
void GLSLProgram::compileShaders(const std::string& vertexShaderFilePath, const std::string& framgmentShaderFilePath)
{
_vertexShaderID = glCreateShader(GL_VERTEX_SHADER);
if (_vertexShaderID == 0)
{
fatalError("Vertex shader failed to be created!");
}
_fragmentShaderID = glCreateShader(GL_FRAGMENT_SHADER);
if (_fragmentShaderID == 0)
{
fatalError("Fragment shader failed to be created!");
}
compileShader(vertexShaderFilePath, _vertexShaderID);
compileShader(framgmentShaderFilePath, _fragmentShaderID);
}
void GLSLProgram::linkShaders()
{
// Attach Shaders to Program
glAttachShader(_programID, _vertexShaderID);
glAttachShader(_programID, _fragmentShaderID);
// Link the Program.
glLinkProgram(_programID);
GLuint isLinked = 0;
glGetProgramiv(_programID, GL_LINK_STATUS, (int *)&isLinked);
if (GL_FALSE == isLinked) {
GLint maxLength = 0;
glGetProgramiv(_programID, GL_INFO_LOG_LENGTH, &maxLength);
std::vector<char> errorLog(maxLength);
glGetProgramInfoLog(_programID, maxLength, &maxLength, &errorLog[0]);
glDeleteProgram(_programID);
glDeleteShader(_vertexShaderID);
glDeleteShader(_fragmentShaderID);
std::printf("%sn", &(errorLog[0]));
fatalError("Shaders failed to link!");
return;
}
glDetachShader(_programID, _vertexShaderID);
glDetachShader(_programID, _fragmentShaderID);
glDeleteShader(_vertexShaderID);
glDeleteShader(_fragmentShaderID);
}
void GLSLProgram::addAttribute(const std::string& attributeName)
{
glBindAttribLocation(_programID, _numAttributes++, attributeName.c_str());
}
void GLSLProgram::use()
{
glUseProgram(_programID);
for (int i = 0; i < _numAttributes; i++)
{
glEnableVertexAttribArray(i);
}
}
void GLSLProgram::unuse()
{
glUseProgram(0);
for (int i = 0; i < _numAttributes; i++)
{
glDisableVertexAttribArray(i);
}
}
void GLSLProgram::compileShader(const std::string & filePath, GLuint& id)
{
std::ifstream fileptr(filePath);
if (fileptr.fail()) {
// Not sold on this
perror(filePath.c_str());
fatalError("Failed to Open " + filePath);
}
std::string fileContents = "";
std::string line;
while (std::getline(fileptr, line))
{
fileContents += line + "n";
}
fileptr.close();
// This is weird
const GLchar * contentsPtr = fileContents.c_str();
glShaderSource(id, 1, &contentsPtr, 0);
glCompileShader(id);
GLint success = 0;
glGetShaderiv(id, GL_COMPILE_STATUS, &success);
if (success == GL_FALSE) {
GLint maxLength = 0;
glGetShaderiv(id, GL_INFO_LOG_LENGTH, &maxLength);
std::vector<GLchar> errorLog(maxLength);
glGetShaderInfoLog(id, maxLength, &maxLength, &errorLog[0]);
glDeleteShader(id);
std::printf("%sn", &(errorLog[0]));
fatalError("Shader " + filePath + " failed to Compile");
}
}
c++ glsl shader
2
The shader is failing to compile, but we don't have its source code...
– Matthieu Brucher
Nov 16 '18 at 9:29
2
"ERROR: 0:7: 'gl_position' ", it has to begl_Position
, GLSL is case sensitive
– Rabbid76
Nov 16 '18 at 9:37
1
Indeed, just a typo: khronos.org/opengl/wiki/Built-in_Variable_(GLSL)
– Matthieu Brucher
Nov 16 '18 at 9:39
add a comment |
Im trying to learn a gl in cpp and trying to create some shaders reading its documentation and following a yt tutorial.
It just had a compilation fail.
yestarday it was just printing in console Shader " + filePath + " failed to Compile
Today it printing that :
ERROR: 0:7: 'gl_position' : undeclared identifier
ERROR: 0:7: 'xy' : field selection requires structure, vector, or matrix on left
hand side
ERROR: 0:7: 'assign' : cannot convert from '2-component vector of highp float'
to 'highp float'
ERROR: 0:8: 'z' : field selection requires structure, vector, or matrix on left
hand side
ERROR: 0:9: 'w' : field selection requires structure, vector, or matrix on left
hand side
Shader shaders/colorShading.vert failed to Compile
Enter any key to quit...
this is my shader source code:
#version 130
in vec2 vertexPosition;
void main()
{
gl_position.xy = vertexPosition * 2;
gl_position.z = 0.0;
gl_position.w = 1.0;
}
//////////////////////////////////////////////////////
#include "GLSLProgram.h"
#include "Error.h"
#include <fstream>
#include <vector>
GLSLProgram::GLSLProgram() :_numAttributes(0), _programID(0), _vertexShaderID(0), _fragmentShaderID(0)
{
}
GLSLProgram::~GLSLProgram()
{
}
void GLSLProgram::compileShaders(const std::string& vertexShaderFilePath, const std::string& framgmentShaderFilePath)
{
_vertexShaderID = glCreateShader(GL_VERTEX_SHADER);
if (_vertexShaderID == 0)
{
fatalError("Vertex shader failed to be created!");
}
_fragmentShaderID = glCreateShader(GL_FRAGMENT_SHADER);
if (_fragmentShaderID == 0)
{
fatalError("Fragment shader failed to be created!");
}
compileShader(vertexShaderFilePath, _vertexShaderID);
compileShader(framgmentShaderFilePath, _fragmentShaderID);
}
void GLSLProgram::linkShaders()
{
// Attach Shaders to Program
glAttachShader(_programID, _vertexShaderID);
glAttachShader(_programID, _fragmentShaderID);
// Link the Program.
glLinkProgram(_programID);
GLuint isLinked = 0;
glGetProgramiv(_programID, GL_LINK_STATUS, (int *)&isLinked);
if (GL_FALSE == isLinked) {
GLint maxLength = 0;
glGetProgramiv(_programID, GL_INFO_LOG_LENGTH, &maxLength);
std::vector<char> errorLog(maxLength);
glGetProgramInfoLog(_programID, maxLength, &maxLength, &errorLog[0]);
glDeleteProgram(_programID);
glDeleteShader(_vertexShaderID);
glDeleteShader(_fragmentShaderID);
std::printf("%sn", &(errorLog[0]));
fatalError("Shaders failed to link!");
return;
}
glDetachShader(_programID, _vertexShaderID);
glDetachShader(_programID, _fragmentShaderID);
glDeleteShader(_vertexShaderID);
glDeleteShader(_fragmentShaderID);
}
void GLSLProgram::addAttribute(const std::string& attributeName)
{
glBindAttribLocation(_programID, _numAttributes++, attributeName.c_str());
}
void GLSLProgram::use()
{
glUseProgram(_programID);
for (int i = 0; i < _numAttributes; i++)
{
glEnableVertexAttribArray(i);
}
}
void GLSLProgram::unuse()
{
glUseProgram(0);
for (int i = 0; i < _numAttributes; i++)
{
glDisableVertexAttribArray(i);
}
}
void GLSLProgram::compileShader(const std::string & filePath, GLuint& id)
{
std::ifstream fileptr(filePath);
if (fileptr.fail()) {
// Not sold on this
perror(filePath.c_str());
fatalError("Failed to Open " + filePath);
}
std::string fileContents = "";
std::string line;
while (std::getline(fileptr, line))
{
fileContents += line + "n";
}
fileptr.close();
// This is weird
const GLchar * contentsPtr = fileContents.c_str();
glShaderSource(id, 1, &contentsPtr, 0);
glCompileShader(id);
GLint success = 0;
glGetShaderiv(id, GL_COMPILE_STATUS, &success);
if (success == GL_FALSE) {
GLint maxLength = 0;
glGetShaderiv(id, GL_INFO_LOG_LENGTH, &maxLength);
std::vector<GLchar> errorLog(maxLength);
glGetShaderInfoLog(id, maxLength, &maxLength, &errorLog[0]);
glDeleteShader(id);
std::printf("%sn", &(errorLog[0]));
fatalError("Shader " + filePath + " failed to Compile");
}
}
c++ glsl shader
Im trying to learn a gl in cpp and trying to create some shaders reading its documentation and following a yt tutorial.
It just had a compilation fail.
yestarday it was just printing in console Shader " + filePath + " failed to Compile
Today it printing that :
ERROR: 0:7: 'gl_position' : undeclared identifier
ERROR: 0:7: 'xy' : field selection requires structure, vector, or matrix on left
hand side
ERROR: 0:7: 'assign' : cannot convert from '2-component vector of highp float'
to 'highp float'
ERROR: 0:8: 'z' : field selection requires structure, vector, or matrix on left
hand side
ERROR: 0:9: 'w' : field selection requires structure, vector, or matrix on left
hand side
Shader shaders/colorShading.vert failed to Compile
Enter any key to quit...
this is my shader source code:
#version 130
in vec2 vertexPosition;
void main()
{
gl_position.xy = vertexPosition * 2;
gl_position.z = 0.0;
gl_position.w = 1.0;
}
//////////////////////////////////////////////////////
#include "GLSLProgram.h"
#include "Error.h"
#include <fstream>
#include <vector>
GLSLProgram::GLSLProgram() :_numAttributes(0), _programID(0), _vertexShaderID(0), _fragmentShaderID(0)
{
}
GLSLProgram::~GLSLProgram()
{
}
void GLSLProgram::compileShaders(const std::string& vertexShaderFilePath, const std::string& framgmentShaderFilePath)
{
_vertexShaderID = glCreateShader(GL_VERTEX_SHADER);
if (_vertexShaderID == 0)
{
fatalError("Vertex shader failed to be created!");
}
_fragmentShaderID = glCreateShader(GL_FRAGMENT_SHADER);
if (_fragmentShaderID == 0)
{
fatalError("Fragment shader failed to be created!");
}
compileShader(vertexShaderFilePath, _vertexShaderID);
compileShader(framgmentShaderFilePath, _fragmentShaderID);
}
void GLSLProgram::linkShaders()
{
// Attach Shaders to Program
glAttachShader(_programID, _vertexShaderID);
glAttachShader(_programID, _fragmentShaderID);
// Link the Program.
glLinkProgram(_programID);
GLuint isLinked = 0;
glGetProgramiv(_programID, GL_LINK_STATUS, (int *)&isLinked);
if (GL_FALSE == isLinked) {
GLint maxLength = 0;
glGetProgramiv(_programID, GL_INFO_LOG_LENGTH, &maxLength);
std::vector<char> errorLog(maxLength);
glGetProgramInfoLog(_programID, maxLength, &maxLength, &errorLog[0]);
glDeleteProgram(_programID);
glDeleteShader(_vertexShaderID);
glDeleteShader(_fragmentShaderID);
std::printf("%sn", &(errorLog[0]));
fatalError("Shaders failed to link!");
return;
}
glDetachShader(_programID, _vertexShaderID);
glDetachShader(_programID, _fragmentShaderID);
glDeleteShader(_vertexShaderID);
glDeleteShader(_fragmentShaderID);
}
void GLSLProgram::addAttribute(const std::string& attributeName)
{
glBindAttribLocation(_programID, _numAttributes++, attributeName.c_str());
}
void GLSLProgram::use()
{
glUseProgram(_programID);
for (int i = 0; i < _numAttributes; i++)
{
glEnableVertexAttribArray(i);
}
}
void GLSLProgram::unuse()
{
glUseProgram(0);
for (int i = 0; i < _numAttributes; i++)
{
glDisableVertexAttribArray(i);
}
}
void GLSLProgram::compileShader(const std::string & filePath, GLuint& id)
{
std::ifstream fileptr(filePath);
if (fileptr.fail()) {
// Not sold on this
perror(filePath.c_str());
fatalError("Failed to Open " + filePath);
}
std::string fileContents = "";
std::string line;
while (std::getline(fileptr, line))
{
fileContents += line + "n";
}
fileptr.close();
// This is weird
const GLchar * contentsPtr = fileContents.c_str();
glShaderSource(id, 1, &contentsPtr, 0);
glCompileShader(id);
GLint success = 0;
glGetShaderiv(id, GL_COMPILE_STATUS, &success);
if (success == GL_FALSE) {
GLint maxLength = 0;
glGetShaderiv(id, GL_INFO_LOG_LENGTH, &maxLength);
std::vector<GLchar> errorLog(maxLength);
glGetShaderInfoLog(id, maxLength, &maxLength, &errorLog[0]);
glDeleteShader(id);
std::printf("%sn", &(errorLog[0]));
fatalError("Shader " + filePath + " failed to Compile");
}
}
c++ glsl shader
c++ glsl shader
edited Nov 16 '18 at 9:42


Rabbid76
42.4k123353
42.4k123353
asked Nov 16 '18 at 9:28
Unknown NameUnknown Name
14
14
2
The shader is failing to compile, but we don't have its source code...
– Matthieu Brucher
Nov 16 '18 at 9:29
2
"ERROR: 0:7: 'gl_position' ", it has to begl_Position
, GLSL is case sensitive
– Rabbid76
Nov 16 '18 at 9:37
1
Indeed, just a typo: khronos.org/opengl/wiki/Built-in_Variable_(GLSL)
– Matthieu Brucher
Nov 16 '18 at 9:39
add a comment |
2
The shader is failing to compile, but we don't have its source code...
– Matthieu Brucher
Nov 16 '18 at 9:29
2
"ERROR: 0:7: 'gl_position' ", it has to begl_Position
, GLSL is case sensitive
– Rabbid76
Nov 16 '18 at 9:37
1
Indeed, just a typo: khronos.org/opengl/wiki/Built-in_Variable_(GLSL)
– Matthieu Brucher
Nov 16 '18 at 9:39
2
2
The shader is failing to compile, but we don't have its source code...
– Matthieu Brucher
Nov 16 '18 at 9:29
The shader is failing to compile, but we don't have its source code...
– Matthieu Brucher
Nov 16 '18 at 9:29
2
2
"ERROR: 0:7: 'gl_position' ", it has to be
gl_Position
, GLSL is case sensitive– Rabbid76
Nov 16 '18 at 9:37
"ERROR: 0:7: 'gl_position' ", it has to be
gl_Position
, GLSL is case sensitive– Rabbid76
Nov 16 '18 at 9:37
1
1
Indeed, just a typo: khronos.org/opengl/wiki/Built-in_Variable_(GLSL)
– Matthieu Brucher
Nov 16 '18 at 9:39
Indeed, just a typo: khronos.org/opengl/wiki/Built-in_Variable_(GLSL)
– Matthieu Brucher
Nov 16 '18 at 9:39
add a comment |
1 Answer
1
active
oldest
votes
The vertex shader failed to compile, because it has to be gl_Position
instead of gl_position
. GLSL is case sensitive. See Vertex Shader - Outputs:
void main()
{
gl_Position.xy = vertexPosition * 2;
gl_Position.z = 0.0;
gl_Position.w = 1.0;
}
You are right! Thank you. now it says: ERROR: 0:8: 'constructor' : too many arguments Shader shaders/colorShading.frag failed to Compile Enter any key to quit...
– Unknown Name
Nov 16 '18 at 9:40
@IliasKarypidis This is an error in the fragment shader. Pleas show your fragment shader too
– Rabbid76
Nov 16 '18 at 9:44
yes iwrote in vec3(1.0, 0.0, 0,0);
– Unknown Name
Nov 16 '18 at 9:48
now the problem is solved but i get and ms visual c++ runtime library error. debug assertion failed! expression:vector subscrit out of range
– Unknown Name
Nov 16 '18 at 9:49
can anyone help me ?
– Unknown Name
Nov 16 '18 at 10:52
|
show 1 more comment
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53334903%2fc-open-gl-shader-failed-compilation%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The vertex shader failed to compile, because it has to be gl_Position
instead of gl_position
. GLSL is case sensitive. See Vertex Shader - Outputs:
void main()
{
gl_Position.xy = vertexPosition * 2;
gl_Position.z = 0.0;
gl_Position.w = 1.0;
}
You are right! Thank you. now it says: ERROR: 0:8: 'constructor' : too many arguments Shader shaders/colorShading.frag failed to Compile Enter any key to quit...
– Unknown Name
Nov 16 '18 at 9:40
@IliasKarypidis This is an error in the fragment shader. Pleas show your fragment shader too
– Rabbid76
Nov 16 '18 at 9:44
yes iwrote in vec3(1.0, 0.0, 0,0);
– Unknown Name
Nov 16 '18 at 9:48
now the problem is solved but i get and ms visual c++ runtime library error. debug assertion failed! expression:vector subscrit out of range
– Unknown Name
Nov 16 '18 at 9:49
can anyone help me ?
– Unknown Name
Nov 16 '18 at 10:52
|
show 1 more comment
The vertex shader failed to compile, because it has to be gl_Position
instead of gl_position
. GLSL is case sensitive. See Vertex Shader - Outputs:
void main()
{
gl_Position.xy = vertexPosition * 2;
gl_Position.z = 0.0;
gl_Position.w = 1.0;
}
You are right! Thank you. now it says: ERROR: 0:8: 'constructor' : too many arguments Shader shaders/colorShading.frag failed to Compile Enter any key to quit...
– Unknown Name
Nov 16 '18 at 9:40
@IliasKarypidis This is an error in the fragment shader. Pleas show your fragment shader too
– Rabbid76
Nov 16 '18 at 9:44
yes iwrote in vec3(1.0, 0.0, 0,0);
– Unknown Name
Nov 16 '18 at 9:48
now the problem is solved but i get and ms visual c++ runtime library error. debug assertion failed! expression:vector subscrit out of range
– Unknown Name
Nov 16 '18 at 9:49
can anyone help me ?
– Unknown Name
Nov 16 '18 at 10:52
|
show 1 more comment
The vertex shader failed to compile, because it has to be gl_Position
instead of gl_position
. GLSL is case sensitive. See Vertex Shader - Outputs:
void main()
{
gl_Position.xy = vertexPosition * 2;
gl_Position.z = 0.0;
gl_Position.w = 1.0;
}
The vertex shader failed to compile, because it has to be gl_Position
instead of gl_position
. GLSL is case sensitive. See Vertex Shader - Outputs:
void main()
{
gl_Position.xy = vertexPosition * 2;
gl_Position.z = 0.0;
gl_Position.w = 1.0;
}
edited Nov 16 '18 at 9:41
answered Nov 16 '18 at 9:40


Rabbid76Rabbid76
42.4k123353
42.4k123353
You are right! Thank you. now it says: ERROR: 0:8: 'constructor' : too many arguments Shader shaders/colorShading.frag failed to Compile Enter any key to quit...
– Unknown Name
Nov 16 '18 at 9:40
@IliasKarypidis This is an error in the fragment shader. Pleas show your fragment shader too
– Rabbid76
Nov 16 '18 at 9:44
yes iwrote in vec3(1.0, 0.0, 0,0);
– Unknown Name
Nov 16 '18 at 9:48
now the problem is solved but i get and ms visual c++ runtime library error. debug assertion failed! expression:vector subscrit out of range
– Unknown Name
Nov 16 '18 at 9:49
can anyone help me ?
– Unknown Name
Nov 16 '18 at 10:52
|
show 1 more comment
You are right! Thank you. now it says: ERROR: 0:8: 'constructor' : too many arguments Shader shaders/colorShading.frag failed to Compile Enter any key to quit...
– Unknown Name
Nov 16 '18 at 9:40
@IliasKarypidis This is an error in the fragment shader. Pleas show your fragment shader too
– Rabbid76
Nov 16 '18 at 9:44
yes iwrote in vec3(1.0, 0.0, 0,0);
– Unknown Name
Nov 16 '18 at 9:48
now the problem is solved but i get and ms visual c++ runtime library error. debug assertion failed! expression:vector subscrit out of range
– Unknown Name
Nov 16 '18 at 9:49
can anyone help me ?
– Unknown Name
Nov 16 '18 at 10:52
You are right! Thank you. now it says: ERROR: 0:8: 'constructor' : too many arguments Shader shaders/colorShading.frag failed to Compile Enter any key to quit...
– Unknown Name
Nov 16 '18 at 9:40
You are right! Thank you. now it says: ERROR: 0:8: 'constructor' : too many arguments Shader shaders/colorShading.frag failed to Compile Enter any key to quit...
– Unknown Name
Nov 16 '18 at 9:40
@IliasKarypidis This is an error in the fragment shader. Pleas show your fragment shader too
– Rabbid76
Nov 16 '18 at 9:44
@IliasKarypidis This is an error in the fragment shader. Pleas show your fragment shader too
– Rabbid76
Nov 16 '18 at 9:44
yes iwrote in vec3(1.0, 0.0, 0,0);
– Unknown Name
Nov 16 '18 at 9:48
yes iwrote in vec3(1.0, 0.0, 0,0);
– Unknown Name
Nov 16 '18 at 9:48
now the problem is solved but i get and ms visual c++ runtime library error. debug assertion failed! expression:vector subscrit out of range
– Unknown Name
Nov 16 '18 at 9:49
now the problem is solved but i get and ms visual c++ runtime library error. debug assertion failed! expression:vector subscrit out of range
– Unknown Name
Nov 16 '18 at 9:49
can anyone help me ?
– Unknown Name
Nov 16 '18 at 10:52
can anyone help me ?
– Unknown Name
Nov 16 '18 at 10:52
|
show 1 more comment
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53334903%2fc-open-gl-shader-failed-compilation%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
8tLc yx5etACdo1ZcVDK MPySn rJraN
2
The shader is failing to compile, but we don't have its source code...
– Matthieu Brucher
Nov 16 '18 at 9:29
2
"ERROR: 0:7: 'gl_position' ", it has to be
gl_Position
, GLSL is case sensitive– Rabbid76
Nov 16 '18 at 9:37
1
Indeed, just a typo: khronos.org/opengl/wiki/Built-in_Variable_(GLSL)
– Matthieu Brucher
Nov 16 '18 at 9:39