Why does click event handler fire immediately upon page load?
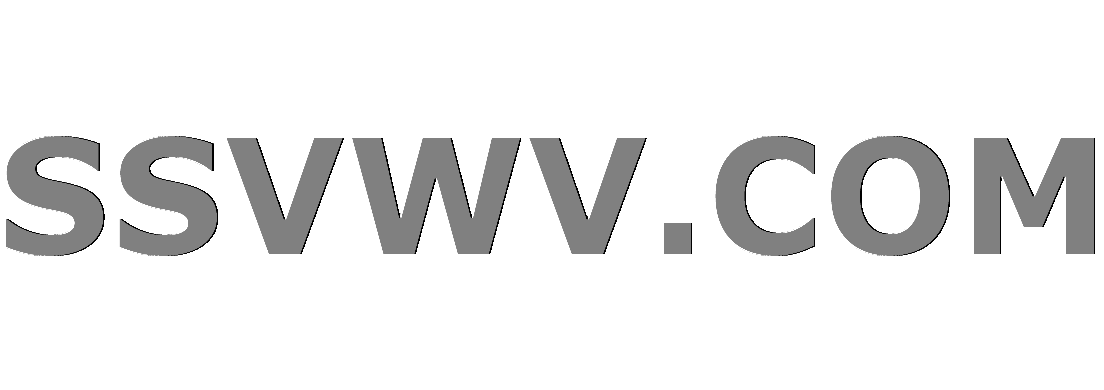
Multi tool use
I playing around with a function that I want to bind to all the links. At the present the function fires when the page loads, instead of when I click on the link.
Here's my code. (I can past in the function showDiv()
if you need to see it.) Can you tell if I'm doing something wrong or stupid here?
$(document).ready(function(){
$('a.test').bind("click", showDiv());
});
Thanks
jquery bind
add a comment |
I playing around with a function that I want to bind to all the links. At the present the function fires when the page loads, instead of when I click on the link.
Here's my code. (I can past in the function showDiv()
if you need to see it.) Can you tell if I'm doing something wrong or stupid here?
$(document).ready(function(){
$('a.test').bind("click", showDiv());
});
Thanks
jquery bind
1
A few notes on how to write code the "jQuery way", usejQuery(function($){ $('a.test').click(showDiv); });
. If jQuery's factory function receives a function it automatically uses it as adocument.ready
callback. Thedocument.ready
callback will be sent the jQuery object as the first parameter, which allows you to aliasjQuery
to$
to prevent any possible multi-library issues. Also, use the event shortcuts for improved readability.
– zzzzBov
Aug 18 '11 at 4:52
By the way: in newer versions.on
should be used stackoverflow.com/questions/11847021/jquery-s-bind-vs-on
– marsze
Nov 14 '18 at 11:22
add a comment |
I playing around with a function that I want to bind to all the links. At the present the function fires when the page loads, instead of when I click on the link.
Here's my code. (I can past in the function showDiv()
if you need to see it.) Can you tell if I'm doing something wrong or stupid here?
$(document).ready(function(){
$('a.test').bind("click", showDiv());
});
Thanks
jquery bind
I playing around with a function that I want to bind to all the links. At the present the function fires when the page loads, instead of when I click on the link.
Here's my code. (I can past in the function showDiv()
if you need to see it.) Can you tell if I'm doing something wrong or stupid here?
$(document).ready(function(){
$('a.test').bind("click", showDiv());
});
Thanks
jquery bind
jquery bind
edited Aug 18 '11 at 4:44
user166390
asked Aug 18 '11 at 4:32
JeffJeff
1,59583158
1,59583158
1
A few notes on how to write code the "jQuery way", usejQuery(function($){ $('a.test').click(showDiv); });
. If jQuery's factory function receives a function it automatically uses it as adocument.ready
callback. Thedocument.ready
callback will be sent the jQuery object as the first parameter, which allows you to aliasjQuery
to$
to prevent any possible multi-library issues. Also, use the event shortcuts for improved readability.
– zzzzBov
Aug 18 '11 at 4:52
By the way: in newer versions.on
should be used stackoverflow.com/questions/11847021/jquery-s-bind-vs-on
– marsze
Nov 14 '18 at 11:22
add a comment |
1
A few notes on how to write code the "jQuery way", usejQuery(function($){ $('a.test').click(showDiv); });
. If jQuery's factory function receives a function it automatically uses it as adocument.ready
callback. Thedocument.ready
callback will be sent the jQuery object as the first parameter, which allows you to aliasjQuery
to$
to prevent any possible multi-library issues. Also, use the event shortcuts for improved readability.
– zzzzBov
Aug 18 '11 at 4:52
By the way: in newer versions.on
should be used stackoverflow.com/questions/11847021/jquery-s-bind-vs-on
– marsze
Nov 14 '18 at 11:22
1
1
A few notes on how to write code the "jQuery way", use
jQuery(function($){ $('a.test').click(showDiv); });
. If jQuery's factory function receives a function it automatically uses it as a document.ready
callback. The document.ready
callback will be sent the jQuery object as the first parameter, which allows you to alias jQuery
to $
to prevent any possible multi-library issues. Also, use the event shortcuts for improved readability.– zzzzBov
Aug 18 '11 at 4:52
A few notes on how to write code the "jQuery way", use
jQuery(function($){ $('a.test').click(showDiv); });
. If jQuery's factory function receives a function it automatically uses it as a document.ready
callback. The document.ready
callback will be sent the jQuery object as the first parameter, which allows you to alias jQuery
to $
to prevent any possible multi-library issues. Also, use the event shortcuts for improved readability.– zzzzBov
Aug 18 '11 at 4:52
By the way: in newer versions
.on
should be used stackoverflow.com/questions/11847021/jquery-s-bind-vs-on– marsze
Nov 14 '18 at 11:22
By the way: in newer versions
.on
should be used stackoverflow.com/questions/11847021/jquery-s-bind-vs-on– marsze
Nov 14 '18 at 11:22
add a comment |
4 Answers
4
active
oldest
votes
You want to pass a reference to a function as a callback, and not the result of function execution:
showDiv()
returns some value; if no return
statement was used, undefined
is returned.
showDiv
is a reference to the function that should be executed.
This should work:
$(document).ready(function(){
$('a.test').bind("click", showDiv);
});
Alternatively, you could use an anonymous function to perform a more advanced function:
...bind('click', function(){
foo.showDiv(a,b,c);
...more code...
});
In some circumstances you may want to use the value returned by a function as a callback:
function function foo(which)
{
function bar()
{
console.log('so very true');
}
function baz()
{
console.log('no way!');
}
return which ? bar : baz;
}
...click( foo( fizz ) );
In this example, foo
is evaluated using fizz
and returns a function that will be assigned as the callback for the click event.
2
In particular,showDiv()
invokes the function-object evaluated from the expressionshowDiv
--showDiv
itself merely evaluates to a function-object (in this instance) which can be used as a callback. (There are no "references" ;-)
– user166390
Aug 18 '11 at 4:40
thanks very much. Problem solved.
– Jeff
Aug 18 '11 at 6:02
+1 This was happening to me and for the life of me I couldn't figure it out, after reading your answer it was obvious.. D'oh!
– MattSizzle
Jun 8 '14 at 1:11
1
I tried every which way, and using the function name alone did not work for me. Finally the anon function worked. Thanks.
– punstress
May 13 '15 at 9:39
1
@MrGuliarte, read the part that starts "Alternatively, you could use an anonymous function to perform a more advanced function"
– zzzzBov
Sep 15 '15 at 2:33
|
show 3 more comments
Looks like you're calling the function showDiv directly there (and binding the return result of showDiv() to the click handler instead of binding it directly.
You want something like
$(document).ready(function() { $('a.test').bind("click", showDiv); });
add a comment |
Use the below line. showDiv()
will call the function rigth away when that line is executed.
$('a.test').bind("click", showDiv);
add a comment |
Change it to: $('a.test').bind("click", showDiv);
(do not put parens around showDiv
since you want to pass the function reference).
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f7102413%2fwhy-does-click-event-handler-fire-immediately-upon-page-load%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
You want to pass a reference to a function as a callback, and not the result of function execution:
showDiv()
returns some value; if no return
statement was used, undefined
is returned.
showDiv
is a reference to the function that should be executed.
This should work:
$(document).ready(function(){
$('a.test').bind("click", showDiv);
});
Alternatively, you could use an anonymous function to perform a more advanced function:
...bind('click', function(){
foo.showDiv(a,b,c);
...more code...
});
In some circumstances you may want to use the value returned by a function as a callback:
function function foo(which)
{
function bar()
{
console.log('so very true');
}
function baz()
{
console.log('no way!');
}
return which ? bar : baz;
}
...click( foo( fizz ) );
In this example, foo
is evaluated using fizz
and returns a function that will be assigned as the callback for the click event.
2
In particular,showDiv()
invokes the function-object evaluated from the expressionshowDiv
--showDiv
itself merely evaluates to a function-object (in this instance) which can be used as a callback. (There are no "references" ;-)
– user166390
Aug 18 '11 at 4:40
thanks very much. Problem solved.
– Jeff
Aug 18 '11 at 6:02
+1 This was happening to me and for the life of me I couldn't figure it out, after reading your answer it was obvious.. D'oh!
– MattSizzle
Jun 8 '14 at 1:11
1
I tried every which way, and using the function name alone did not work for me. Finally the anon function worked. Thanks.
– punstress
May 13 '15 at 9:39
1
@MrGuliarte, read the part that starts "Alternatively, you could use an anonymous function to perform a more advanced function"
– zzzzBov
Sep 15 '15 at 2:33
|
show 3 more comments
You want to pass a reference to a function as a callback, and not the result of function execution:
showDiv()
returns some value; if no return
statement was used, undefined
is returned.
showDiv
is a reference to the function that should be executed.
This should work:
$(document).ready(function(){
$('a.test').bind("click", showDiv);
});
Alternatively, you could use an anonymous function to perform a more advanced function:
...bind('click', function(){
foo.showDiv(a,b,c);
...more code...
});
In some circumstances you may want to use the value returned by a function as a callback:
function function foo(which)
{
function bar()
{
console.log('so very true');
}
function baz()
{
console.log('no way!');
}
return which ? bar : baz;
}
...click( foo( fizz ) );
In this example, foo
is evaluated using fizz
and returns a function that will be assigned as the callback for the click event.
2
In particular,showDiv()
invokes the function-object evaluated from the expressionshowDiv
--showDiv
itself merely evaluates to a function-object (in this instance) which can be used as a callback. (There are no "references" ;-)
– user166390
Aug 18 '11 at 4:40
thanks very much. Problem solved.
– Jeff
Aug 18 '11 at 6:02
+1 This was happening to me and for the life of me I couldn't figure it out, after reading your answer it was obvious.. D'oh!
– MattSizzle
Jun 8 '14 at 1:11
1
I tried every which way, and using the function name alone did not work for me. Finally the anon function worked. Thanks.
– punstress
May 13 '15 at 9:39
1
@MrGuliarte, read the part that starts "Alternatively, you could use an anonymous function to perform a more advanced function"
– zzzzBov
Sep 15 '15 at 2:33
|
show 3 more comments
You want to pass a reference to a function as a callback, and not the result of function execution:
showDiv()
returns some value; if no return
statement was used, undefined
is returned.
showDiv
is a reference to the function that should be executed.
This should work:
$(document).ready(function(){
$('a.test').bind("click", showDiv);
});
Alternatively, you could use an anonymous function to perform a more advanced function:
...bind('click', function(){
foo.showDiv(a,b,c);
...more code...
});
In some circumstances you may want to use the value returned by a function as a callback:
function function foo(which)
{
function bar()
{
console.log('so very true');
}
function baz()
{
console.log('no way!');
}
return which ? bar : baz;
}
...click( foo( fizz ) );
In this example, foo
is evaluated using fizz
and returns a function that will be assigned as the callback for the click event.
You want to pass a reference to a function as a callback, and not the result of function execution:
showDiv()
returns some value; if no return
statement was used, undefined
is returned.
showDiv
is a reference to the function that should be executed.
This should work:
$(document).ready(function(){
$('a.test').bind("click", showDiv);
});
Alternatively, you could use an anonymous function to perform a more advanced function:
...bind('click', function(){
foo.showDiv(a,b,c);
...more code...
});
In some circumstances you may want to use the value returned by a function as a callback:
function function foo(which)
{
function bar()
{
console.log('so very true');
}
function baz()
{
console.log('no way!');
}
return which ? bar : baz;
}
...click( foo( fizz ) );
In this example, foo
is evaluated using fizz
and returns a function that will be assigned as the callback for the click event.
edited Aug 18 '11 at 4:46
answered Aug 18 '11 at 4:36
zzzzBovzzzzBov
131k33262305
131k33262305
2
In particular,showDiv()
invokes the function-object evaluated from the expressionshowDiv
--showDiv
itself merely evaluates to a function-object (in this instance) which can be used as a callback. (There are no "references" ;-)
– user166390
Aug 18 '11 at 4:40
thanks very much. Problem solved.
– Jeff
Aug 18 '11 at 6:02
+1 This was happening to me and for the life of me I couldn't figure it out, after reading your answer it was obvious.. D'oh!
– MattSizzle
Jun 8 '14 at 1:11
1
I tried every which way, and using the function name alone did not work for me. Finally the anon function worked. Thanks.
– punstress
May 13 '15 at 9:39
1
@MrGuliarte, read the part that starts "Alternatively, you could use an anonymous function to perform a more advanced function"
– zzzzBov
Sep 15 '15 at 2:33
|
show 3 more comments
2
In particular,showDiv()
invokes the function-object evaluated from the expressionshowDiv
--showDiv
itself merely evaluates to a function-object (in this instance) which can be used as a callback. (There are no "references" ;-)
– user166390
Aug 18 '11 at 4:40
thanks very much. Problem solved.
– Jeff
Aug 18 '11 at 6:02
+1 This was happening to me and for the life of me I couldn't figure it out, after reading your answer it was obvious.. D'oh!
– MattSizzle
Jun 8 '14 at 1:11
1
I tried every which way, and using the function name alone did not work for me. Finally the anon function worked. Thanks.
– punstress
May 13 '15 at 9:39
1
@MrGuliarte, read the part that starts "Alternatively, you could use an anonymous function to perform a more advanced function"
– zzzzBov
Sep 15 '15 at 2:33
2
2
In particular,
showDiv()
invokes the function-object evaluated from the expression showDiv
-- showDiv
itself merely evaluates to a function-object (in this instance) which can be used as a callback. (There are no "references" ;-)– user166390
Aug 18 '11 at 4:40
In particular,
showDiv()
invokes the function-object evaluated from the expression showDiv
-- showDiv
itself merely evaluates to a function-object (in this instance) which can be used as a callback. (There are no "references" ;-)– user166390
Aug 18 '11 at 4:40
thanks very much. Problem solved.
– Jeff
Aug 18 '11 at 6:02
thanks very much. Problem solved.
– Jeff
Aug 18 '11 at 6:02
+1 This was happening to me and for the life of me I couldn't figure it out, after reading your answer it was obvious.. D'oh!
– MattSizzle
Jun 8 '14 at 1:11
+1 This was happening to me and for the life of me I couldn't figure it out, after reading your answer it was obvious.. D'oh!
– MattSizzle
Jun 8 '14 at 1:11
1
1
I tried every which way, and using the function name alone did not work for me. Finally the anon function worked. Thanks.
– punstress
May 13 '15 at 9:39
I tried every which way, and using the function name alone did not work for me. Finally the anon function worked. Thanks.
– punstress
May 13 '15 at 9:39
1
1
@MrGuliarte, read the part that starts "Alternatively, you could use an anonymous function to perform a more advanced function"
– zzzzBov
Sep 15 '15 at 2:33
@MrGuliarte, read the part that starts "Alternatively, you could use an anonymous function to perform a more advanced function"
– zzzzBov
Sep 15 '15 at 2:33
|
show 3 more comments
Looks like you're calling the function showDiv directly there (and binding the return result of showDiv() to the click handler instead of binding it directly.
You want something like
$(document).ready(function() { $('a.test').bind("click", showDiv); });
add a comment |
Looks like you're calling the function showDiv directly there (and binding the return result of showDiv() to the click handler instead of binding it directly.
You want something like
$(document).ready(function() { $('a.test').bind("click", showDiv); });
add a comment |
Looks like you're calling the function showDiv directly there (and binding the return result of showDiv() to the click handler instead of binding it directly.
You want something like
$(document).ready(function() { $('a.test').bind("click", showDiv); });
Looks like you're calling the function showDiv directly there (and binding the return result of showDiv() to the click handler instead of binding it directly.
You want something like
$(document).ready(function() { $('a.test').bind("click", showDiv); });
answered Aug 18 '11 at 4:35
tjarratttjarratt
1,607917
1,607917
add a comment |
add a comment |
Use the below line. showDiv()
will call the function rigth away when that line is executed.
$('a.test').bind("click", showDiv);
add a comment |
Use the below line. showDiv()
will call the function rigth away when that line is executed.
$('a.test').bind("click", showDiv);
add a comment |
Use the below line. showDiv()
will call the function rigth away when that line is executed.
$('a.test').bind("click", showDiv);
Use the below line. showDiv()
will call the function rigth away when that line is executed.
$('a.test').bind("click", showDiv);
answered Aug 18 '11 at 4:34
ShankarSangoliShankarSangoli
62.8k973118
62.8k973118
add a comment |
add a comment |
Change it to: $('a.test').bind("click", showDiv);
(do not put parens around showDiv
since you want to pass the function reference).
add a comment |
Change it to: $('a.test').bind("click", showDiv);
(do not put parens around showDiv
since you want to pass the function reference).
add a comment |
Change it to: $('a.test').bind("click", showDiv);
(do not put parens around showDiv
since you want to pass the function reference).
Change it to: $('a.test').bind("click", showDiv);
(do not put parens around showDiv
since you want to pass the function reference).
edited Aug 18 '11 at 4:41
answered Aug 18 '11 at 4:34
MrchiefMrchief
59.8k16112171
59.8k16112171
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f7102413%2fwhy-does-click-event-handler-fire-immediately-upon-page-load%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
o sKMv2 6 TAKzqFVmkWW2qd3yX xSTlLV7xV SwYXc,j,bszc11g2auq
1
A few notes on how to write code the "jQuery way", use
jQuery(function($){ $('a.test').click(showDiv); });
. If jQuery's factory function receives a function it automatically uses it as adocument.ready
callback. Thedocument.ready
callback will be sent the jQuery object as the first parameter, which allows you to aliasjQuery
to$
to prevent any possible multi-library issues. Also, use the event shortcuts for improved readability.– zzzzBov
Aug 18 '11 at 4:52
By the way: in newer versions
.on
should be used stackoverflow.com/questions/11847021/jquery-s-bind-vs-on– marsze
Nov 14 '18 at 11:22