C# won't read updated version of SQL variable
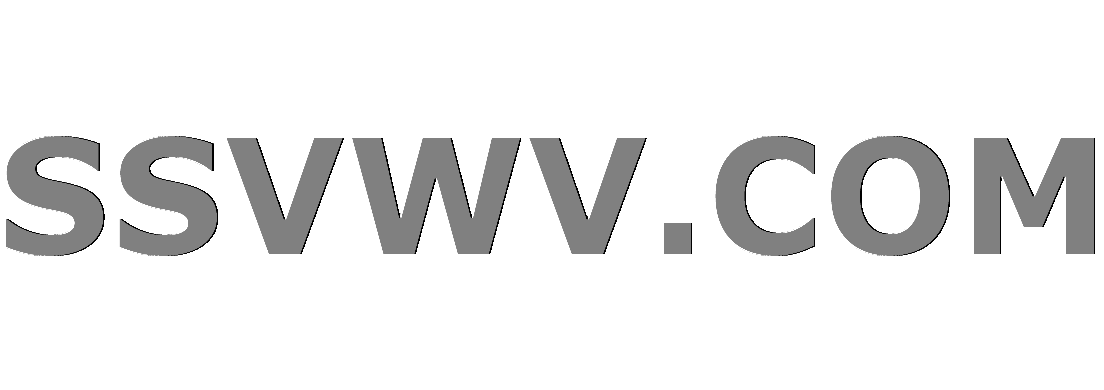
Multi tool use
Resolved - was querying the wrong DB by mistake
sorry for wasting your time! :(
I'm trying to read the results of a series of actions on a SQL variable, this is the query i'm trying to get the value out of:
DECLARE @Count INT = 0
IF EXISTS(SELECT name FROM sys.sysobjects WHERE Name = N'Table1' AND xtype = N'U')
BEGIN
SET @Count = @Count + (SELECT COUNT(*) FROM Table1)
END
IF EXISTS(SELECT name FROM sys.sysobjects WHERE Name = N'Table2' AND xtype = N'U')
BEGIN
SET @Count = @Count + (SELECT COUNT(*) FROM Table2)
END
IF EXISTS(SELECT name FROM sys.sysobjects WHERE Name = N'Table3' AND xtype = N'U')
BEGIN
SET @Count = @Count + (SELECT COUNT(*) FROM Table3)
END
SELECT @Count
Basically it checks if a bunch of tables (which might not exist) have rows in them.
C# Code
List<string> queries = new List<string>();
foreach (var table in tables)
{
queries.Add(string.Format(@"IF EXISTS(SELECT name FROM sys.sysobjects WHERE Name = N'{0}' AND xtype = N'U')
BEGIN
SET @Count = @Count + (SELECT COUNT(*) FROM {0})
END", table.Name.Trim()));
}
var queryString = string.Format(@"DECLARE @Count INT = 0 {0} SELECT @Count
GO", string.Join(" ", queries));
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
//I also tried these methods using Dapper:
// int count = connection.Query<int>(queryString);
// int count = (int)connection.ExecuteScalar(queryString);
SqlCommand cmd = new SqlCommand(queryString, connection);
using (SqlDataReader dr = cmd.ExecuteReader())
{
DataTable dt = new DataTable();
dt.Load(dr);
//dt has one row where the data is 0, not the sum as i want it
}
connection.Close();
}
The Problem
The problem is, no matter how I try to load the result in C# the original value always gets returned (in this case 0, but if i change it in the query the change gets reflected in the result).
I'd like for the returned value to be the sum of all these queries:
(SELECT COUNT(*) FROM X)
What I tried
- Searching online i thought it was a scope problem, so i tried running the query with and without SqlCommand parameters, which didn't change the outcome, maybe i'm not searching the right words
- Adding 'GO' both after the SET commands and at the end
- Trying different ways to parse the data in the ASP.NET application, including using an external library, called Dapper
Putting the query in a stored procedure is not possible unfortunately, anyone has an idea about what to try next? I've been stuck on this for a while...
SQL Server version is SQLServer 2014
c# sql

|
show 8 more comments
Resolved - was querying the wrong DB by mistake
sorry for wasting your time! :(
I'm trying to read the results of a series of actions on a SQL variable, this is the query i'm trying to get the value out of:
DECLARE @Count INT = 0
IF EXISTS(SELECT name FROM sys.sysobjects WHERE Name = N'Table1' AND xtype = N'U')
BEGIN
SET @Count = @Count + (SELECT COUNT(*) FROM Table1)
END
IF EXISTS(SELECT name FROM sys.sysobjects WHERE Name = N'Table2' AND xtype = N'U')
BEGIN
SET @Count = @Count + (SELECT COUNT(*) FROM Table2)
END
IF EXISTS(SELECT name FROM sys.sysobjects WHERE Name = N'Table3' AND xtype = N'U')
BEGIN
SET @Count = @Count + (SELECT COUNT(*) FROM Table3)
END
SELECT @Count
Basically it checks if a bunch of tables (which might not exist) have rows in them.
C# Code
List<string> queries = new List<string>();
foreach (var table in tables)
{
queries.Add(string.Format(@"IF EXISTS(SELECT name FROM sys.sysobjects WHERE Name = N'{0}' AND xtype = N'U')
BEGIN
SET @Count = @Count + (SELECT COUNT(*) FROM {0})
END", table.Name.Trim()));
}
var queryString = string.Format(@"DECLARE @Count INT = 0 {0} SELECT @Count
GO", string.Join(" ", queries));
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
//I also tried these methods using Dapper:
// int count = connection.Query<int>(queryString);
// int count = (int)connection.ExecuteScalar(queryString);
SqlCommand cmd = new SqlCommand(queryString, connection);
using (SqlDataReader dr = cmd.ExecuteReader())
{
DataTable dt = new DataTable();
dt.Load(dr);
//dt has one row where the data is 0, not the sum as i want it
}
connection.Close();
}
The Problem
The problem is, no matter how I try to load the result in C# the original value always gets returned (in this case 0, but if i change it in the query the change gets reflected in the result).
I'd like for the returned value to be the sum of all these queries:
(SELECT COUNT(*) FROM X)
What I tried
- Searching online i thought it was a scope problem, so i tried running the query with and without SqlCommand parameters, which didn't change the outcome, maybe i'm not searching the right words
- Adding 'GO' both after the SET commands and at the end
- Trying different ways to parse the data in the ASP.NET application, including using an external library, called Dapper
Putting the query in a stored procedure is not possible unfortunately, anyone has an idea about what to try next? I've been stuck on this for a while...
SQL Server version is SQLServer 2014
c# sql

1
We need code, please post what you've tried.
– Barr J
Nov 14 '18 at 10:57
In c# you have two commands 1) Insert 2) Update. When using ExecuteNonQuery() you have to check the return value which is the number of rows affected. If you get a return value of zero it means no rows changed. If you are doing an INSERT it means the key already exists in the table and then you have to use UPDATE to change the existing key value. If you are doing UPDATE it means the key doesn't exist in table and then you have to do a INSERT to put a new key into the table.
– jdweng
Nov 14 '18 at 11:02
no matter how I try to load the result in C# the original value always gets returned where is this C# you talk of?
– Liam
Nov 14 '18 at 11:04
I've updated my answer based on the edits...
– Marc Gravell♦
Nov 14 '18 at 11:11
1
Also, make sure you're executing the query on the same database that C# is; wouldn't be the first time I've had someone say "oh, i was testing the query on my prod DB with 4252 rows but I forgot my local debug DB that c# was using is actually empty..."
– Caius Jard
Nov 14 '18 at 11:21
|
show 8 more comments
Resolved - was querying the wrong DB by mistake
sorry for wasting your time! :(
I'm trying to read the results of a series of actions on a SQL variable, this is the query i'm trying to get the value out of:
DECLARE @Count INT = 0
IF EXISTS(SELECT name FROM sys.sysobjects WHERE Name = N'Table1' AND xtype = N'U')
BEGIN
SET @Count = @Count + (SELECT COUNT(*) FROM Table1)
END
IF EXISTS(SELECT name FROM sys.sysobjects WHERE Name = N'Table2' AND xtype = N'U')
BEGIN
SET @Count = @Count + (SELECT COUNT(*) FROM Table2)
END
IF EXISTS(SELECT name FROM sys.sysobjects WHERE Name = N'Table3' AND xtype = N'U')
BEGIN
SET @Count = @Count + (SELECT COUNT(*) FROM Table3)
END
SELECT @Count
Basically it checks if a bunch of tables (which might not exist) have rows in them.
C# Code
List<string> queries = new List<string>();
foreach (var table in tables)
{
queries.Add(string.Format(@"IF EXISTS(SELECT name FROM sys.sysobjects WHERE Name = N'{0}' AND xtype = N'U')
BEGIN
SET @Count = @Count + (SELECT COUNT(*) FROM {0})
END", table.Name.Trim()));
}
var queryString = string.Format(@"DECLARE @Count INT = 0 {0} SELECT @Count
GO", string.Join(" ", queries));
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
//I also tried these methods using Dapper:
// int count = connection.Query<int>(queryString);
// int count = (int)connection.ExecuteScalar(queryString);
SqlCommand cmd = new SqlCommand(queryString, connection);
using (SqlDataReader dr = cmd.ExecuteReader())
{
DataTable dt = new DataTable();
dt.Load(dr);
//dt has one row where the data is 0, not the sum as i want it
}
connection.Close();
}
The Problem
The problem is, no matter how I try to load the result in C# the original value always gets returned (in this case 0, but if i change it in the query the change gets reflected in the result).
I'd like for the returned value to be the sum of all these queries:
(SELECT COUNT(*) FROM X)
What I tried
- Searching online i thought it was a scope problem, so i tried running the query with and without SqlCommand parameters, which didn't change the outcome, maybe i'm not searching the right words
- Adding 'GO' both after the SET commands and at the end
- Trying different ways to parse the data in the ASP.NET application, including using an external library, called Dapper
Putting the query in a stored procedure is not possible unfortunately, anyone has an idea about what to try next? I've been stuck on this for a while...
SQL Server version is SQLServer 2014
c# sql

Resolved - was querying the wrong DB by mistake
sorry for wasting your time! :(
I'm trying to read the results of a series of actions on a SQL variable, this is the query i'm trying to get the value out of:
DECLARE @Count INT = 0
IF EXISTS(SELECT name FROM sys.sysobjects WHERE Name = N'Table1' AND xtype = N'U')
BEGIN
SET @Count = @Count + (SELECT COUNT(*) FROM Table1)
END
IF EXISTS(SELECT name FROM sys.sysobjects WHERE Name = N'Table2' AND xtype = N'U')
BEGIN
SET @Count = @Count + (SELECT COUNT(*) FROM Table2)
END
IF EXISTS(SELECT name FROM sys.sysobjects WHERE Name = N'Table3' AND xtype = N'U')
BEGIN
SET @Count = @Count + (SELECT COUNT(*) FROM Table3)
END
SELECT @Count
Basically it checks if a bunch of tables (which might not exist) have rows in them.
C# Code
List<string> queries = new List<string>();
foreach (var table in tables)
{
queries.Add(string.Format(@"IF EXISTS(SELECT name FROM sys.sysobjects WHERE Name = N'{0}' AND xtype = N'U')
BEGIN
SET @Count = @Count + (SELECT COUNT(*) FROM {0})
END", table.Name.Trim()));
}
var queryString = string.Format(@"DECLARE @Count INT = 0 {0} SELECT @Count
GO", string.Join(" ", queries));
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
//I also tried these methods using Dapper:
// int count = connection.Query<int>(queryString);
// int count = (int)connection.ExecuteScalar(queryString);
SqlCommand cmd = new SqlCommand(queryString, connection);
using (SqlDataReader dr = cmd.ExecuteReader())
{
DataTable dt = new DataTable();
dt.Load(dr);
//dt has one row where the data is 0, not the sum as i want it
}
connection.Close();
}
The Problem
The problem is, no matter how I try to load the result in C# the original value always gets returned (in this case 0, but if i change it in the query the change gets reflected in the result).
I'd like for the returned value to be the sum of all these queries:
(SELECT COUNT(*) FROM X)
What I tried
- Searching online i thought it was a scope problem, so i tried running the query with and without SqlCommand parameters, which didn't change the outcome, maybe i'm not searching the right words
- Adding 'GO' both after the SET commands and at the end
- Trying different ways to parse the data in the ASP.NET application, including using an external library, called Dapper
Putting the query in a stored procedure is not possible unfortunately, anyone has an idea about what to try next? I've been stuck on this for a while...
SQL Server version is SQLServer 2014
c# sql

c# sql

edited Nov 14 '18 at 11:29
HiSpy
asked Nov 14 '18 at 10:55


HiSpyHiSpy
74
74
1
We need code, please post what you've tried.
– Barr J
Nov 14 '18 at 10:57
In c# you have two commands 1) Insert 2) Update. When using ExecuteNonQuery() you have to check the return value which is the number of rows affected. If you get a return value of zero it means no rows changed. If you are doing an INSERT it means the key already exists in the table and then you have to use UPDATE to change the existing key value. If you are doing UPDATE it means the key doesn't exist in table and then you have to do a INSERT to put a new key into the table.
– jdweng
Nov 14 '18 at 11:02
no matter how I try to load the result in C# the original value always gets returned where is this C# you talk of?
– Liam
Nov 14 '18 at 11:04
I've updated my answer based on the edits...
– Marc Gravell♦
Nov 14 '18 at 11:11
1
Also, make sure you're executing the query on the same database that C# is; wouldn't be the first time I've had someone say "oh, i was testing the query on my prod DB with 4252 rows but I forgot my local debug DB that c# was using is actually empty..."
– Caius Jard
Nov 14 '18 at 11:21
|
show 8 more comments
1
We need code, please post what you've tried.
– Barr J
Nov 14 '18 at 10:57
In c# you have two commands 1) Insert 2) Update. When using ExecuteNonQuery() you have to check the return value which is the number of rows affected. If you get a return value of zero it means no rows changed. If you are doing an INSERT it means the key already exists in the table and then you have to use UPDATE to change the existing key value. If you are doing UPDATE it means the key doesn't exist in table and then you have to do a INSERT to put a new key into the table.
– jdweng
Nov 14 '18 at 11:02
no matter how I try to load the result in C# the original value always gets returned where is this C# you talk of?
– Liam
Nov 14 '18 at 11:04
I've updated my answer based on the edits...
– Marc Gravell♦
Nov 14 '18 at 11:11
1
Also, make sure you're executing the query on the same database that C# is; wouldn't be the first time I've had someone say "oh, i was testing the query on my prod DB with 4252 rows but I forgot my local debug DB that c# was using is actually empty..."
– Caius Jard
Nov 14 '18 at 11:21
1
1
We need code, please post what you've tried.
– Barr J
Nov 14 '18 at 10:57
We need code, please post what you've tried.
– Barr J
Nov 14 '18 at 10:57
In c# you have two commands 1) Insert 2) Update. When using ExecuteNonQuery() you have to check the return value which is the number of rows affected. If you get a return value of zero it means no rows changed. If you are doing an INSERT it means the key already exists in the table and then you have to use UPDATE to change the existing key value. If you are doing UPDATE it means the key doesn't exist in table and then you have to do a INSERT to put a new key into the table.
– jdweng
Nov 14 '18 at 11:02
In c# you have two commands 1) Insert 2) Update. When using ExecuteNonQuery() you have to check the return value which is the number of rows affected. If you get a return value of zero it means no rows changed. If you are doing an INSERT it means the key already exists in the table and then you have to use UPDATE to change the existing key value. If you are doing UPDATE it means the key doesn't exist in table and then you have to do a INSERT to put a new key into the table.
– jdweng
Nov 14 '18 at 11:02
no matter how I try to load the result in C# the original value always gets returned where is this C# you talk of?
– Liam
Nov 14 '18 at 11:04
no matter how I try to load the result in C# the original value always gets returned where is this C# you talk of?
– Liam
Nov 14 '18 at 11:04
I've updated my answer based on the edits...
– Marc Gravell♦
Nov 14 '18 at 11:11
I've updated my answer based on the edits...
– Marc Gravell♦
Nov 14 '18 at 11:11
1
1
Also, make sure you're executing the query on the same database that C# is; wouldn't be the first time I've had someone say "oh, i was testing the query on my prod DB with 4252 rows but I forgot my local debug DB that c# was using is actually empty..."
– Caius Jard
Nov 14 '18 at 11:21
Also, make sure you're executing the query on the same database that C# is; wouldn't be the first time I've had someone say "oh, i was testing the query on my prod DB with 4252 rows but I forgot my local debug DB that c# was using is actually empty..."
– Caius Jard
Nov 14 '18 at 11:21
|
show 8 more comments
3 Answers
3
active
oldest
votes
Edit to reflect the question edits:
With the change shown, and:
//dt has one row where the data is 0, not the sum as i want it
then: the answer is zero. You're querying it in an OK way (the DataTable
is massively overkill, but... meh), but: the answer is zero.
I'm pretty sure that if you run the same TSQL in SSMS (in the same database, as the same user): you'll get zero there, too.
Ultimately, the key factor here is: how are you returning the value? In this case, you are doing:
SELECT @Count
which means that it is going to be coming back as a results grid - i.e. it is a query; so: as long as you use a query API, you should be fine. This includes ExecuteScalar
in ADO.NET, and QuerySingle<int>
in dapper (which you mention).
What you can't do is use the return value of ExecuteNonQuery
(Execute
in dapper), as this is an unrelated value (and is usually wrong and/or empty and/or useless - don't use it pretty much ever).
Oh, i dunno.. ExecuteNonQuery on a CRUD is pretty vital for getting optimistic concurrency to work out properly
– Caius Jard
Nov 14 '18 at 11:05
@CaiusJard nothing wrong withExecuteNonQuery
, but the return value is not guaranteed to be set at all; if you want to know a rowcount, for example, then I would strongly recommentselect @@rowcount
- or if you really want to useExecuteNonQuery
,set @someOutArg = @@rowcount
, orreturn @@rowcount
, which is accessed virtually identically to the@someOutArg
API, and is not the return value ofExecuteNonQuery
– Marc Gravell♦
Nov 14 '18 at 11:07
OK.. Though have you ever run an plain oldINSERT/UPDATE/DELETE
query and NOT had ExecuteNonQuery return the number of rows affected?
– Caius Jard
Nov 14 '18 at 11:12
thanks for the reply, unfortunately (int)cmd.ExecuteScalar() still returns 0
– HiSpy
Nov 14 '18 at 11:13
@CaiusJard yes, very often; a: a lot of places make it standard practice toSET NOCOUNT ON
to slightly reduce TDS traffic; b: if tables have triggers, those triggers can cause very confusing results from this
– Marc Gravell♦
Nov 14 '18 at 11:14
|
show 2 more comments
Try this C# (might have minor syntax errors - didn't write/run it in VS). If you still get 0 out of it, you're querying an empty db:
int count = 0;
using (SqlConnection connection = new SqlConnection(connectionString))
{
try{
connection.Open();
foreach (var table in tables)
{
try{
var cmd = "SELECT COUNT(*) FROM " + table;
using(var sc as new SqlCommand(cmd, connection)){
count += (int)sc.ExecuteScalar();
}
}
catch {}
}
}finally{
connection.Close();
}
}
add a comment |
The best way here is to attach your sql to a command, then do
int retVal = cmd.ExecuteScalar();
should get the value back fine - it's one of several ways of doing it though.
ExecuteScalar is for instances where you want to return a single row with a single column, as you have done here.
tried ExecuteScalar, the result is still 0
– HiSpy
Nov 14 '18 at 11:19
have you confirmed zero is not the value in the variable? Try doing SET @COUNT=1; the line before you SELECT it, as a check.
– Cato
Nov 14 '18 at 11:38
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53298550%2fc-sharp-wont-read-updated-version-of-sql-variable%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
Edit to reflect the question edits:
With the change shown, and:
//dt has one row where the data is 0, not the sum as i want it
then: the answer is zero. You're querying it in an OK way (the DataTable
is massively overkill, but... meh), but: the answer is zero.
I'm pretty sure that if you run the same TSQL in SSMS (in the same database, as the same user): you'll get zero there, too.
Ultimately, the key factor here is: how are you returning the value? In this case, you are doing:
SELECT @Count
which means that it is going to be coming back as a results grid - i.e. it is a query; so: as long as you use a query API, you should be fine. This includes ExecuteScalar
in ADO.NET, and QuerySingle<int>
in dapper (which you mention).
What you can't do is use the return value of ExecuteNonQuery
(Execute
in dapper), as this is an unrelated value (and is usually wrong and/or empty and/or useless - don't use it pretty much ever).
Oh, i dunno.. ExecuteNonQuery on a CRUD is pretty vital for getting optimistic concurrency to work out properly
– Caius Jard
Nov 14 '18 at 11:05
@CaiusJard nothing wrong withExecuteNonQuery
, but the return value is not guaranteed to be set at all; if you want to know a rowcount, for example, then I would strongly recommentselect @@rowcount
- or if you really want to useExecuteNonQuery
,set @someOutArg = @@rowcount
, orreturn @@rowcount
, which is accessed virtually identically to the@someOutArg
API, and is not the return value ofExecuteNonQuery
– Marc Gravell♦
Nov 14 '18 at 11:07
OK.. Though have you ever run an plain oldINSERT/UPDATE/DELETE
query and NOT had ExecuteNonQuery return the number of rows affected?
– Caius Jard
Nov 14 '18 at 11:12
thanks for the reply, unfortunately (int)cmd.ExecuteScalar() still returns 0
– HiSpy
Nov 14 '18 at 11:13
@CaiusJard yes, very often; a: a lot of places make it standard practice toSET NOCOUNT ON
to slightly reduce TDS traffic; b: if tables have triggers, those triggers can cause very confusing results from this
– Marc Gravell♦
Nov 14 '18 at 11:14
|
show 2 more comments
Edit to reflect the question edits:
With the change shown, and:
//dt has one row where the data is 0, not the sum as i want it
then: the answer is zero. You're querying it in an OK way (the DataTable
is massively overkill, but... meh), but: the answer is zero.
I'm pretty sure that if you run the same TSQL in SSMS (in the same database, as the same user): you'll get zero there, too.
Ultimately, the key factor here is: how are you returning the value? In this case, you are doing:
SELECT @Count
which means that it is going to be coming back as a results grid - i.e. it is a query; so: as long as you use a query API, you should be fine. This includes ExecuteScalar
in ADO.NET, and QuerySingle<int>
in dapper (which you mention).
What you can't do is use the return value of ExecuteNonQuery
(Execute
in dapper), as this is an unrelated value (and is usually wrong and/or empty and/or useless - don't use it pretty much ever).
Oh, i dunno.. ExecuteNonQuery on a CRUD is pretty vital for getting optimistic concurrency to work out properly
– Caius Jard
Nov 14 '18 at 11:05
@CaiusJard nothing wrong withExecuteNonQuery
, but the return value is not guaranteed to be set at all; if you want to know a rowcount, for example, then I would strongly recommentselect @@rowcount
- or if you really want to useExecuteNonQuery
,set @someOutArg = @@rowcount
, orreturn @@rowcount
, which is accessed virtually identically to the@someOutArg
API, and is not the return value ofExecuteNonQuery
– Marc Gravell♦
Nov 14 '18 at 11:07
OK.. Though have you ever run an plain oldINSERT/UPDATE/DELETE
query and NOT had ExecuteNonQuery return the number of rows affected?
– Caius Jard
Nov 14 '18 at 11:12
thanks for the reply, unfortunately (int)cmd.ExecuteScalar() still returns 0
– HiSpy
Nov 14 '18 at 11:13
@CaiusJard yes, very often; a: a lot of places make it standard practice toSET NOCOUNT ON
to slightly reduce TDS traffic; b: if tables have triggers, those triggers can cause very confusing results from this
– Marc Gravell♦
Nov 14 '18 at 11:14
|
show 2 more comments
Edit to reflect the question edits:
With the change shown, and:
//dt has one row where the data is 0, not the sum as i want it
then: the answer is zero. You're querying it in an OK way (the DataTable
is massively overkill, but... meh), but: the answer is zero.
I'm pretty sure that if you run the same TSQL in SSMS (in the same database, as the same user): you'll get zero there, too.
Ultimately, the key factor here is: how are you returning the value? In this case, you are doing:
SELECT @Count
which means that it is going to be coming back as a results grid - i.e. it is a query; so: as long as you use a query API, you should be fine. This includes ExecuteScalar
in ADO.NET, and QuerySingle<int>
in dapper (which you mention).
What you can't do is use the return value of ExecuteNonQuery
(Execute
in dapper), as this is an unrelated value (and is usually wrong and/or empty and/or useless - don't use it pretty much ever).
Edit to reflect the question edits:
With the change shown, and:
//dt has one row where the data is 0, not the sum as i want it
then: the answer is zero. You're querying it in an OK way (the DataTable
is massively overkill, but... meh), but: the answer is zero.
I'm pretty sure that if you run the same TSQL in SSMS (in the same database, as the same user): you'll get zero there, too.
Ultimately, the key factor here is: how are you returning the value? In this case, you are doing:
SELECT @Count
which means that it is going to be coming back as a results grid - i.e. it is a query; so: as long as you use a query API, you should be fine. This includes ExecuteScalar
in ADO.NET, and QuerySingle<int>
in dapper (which you mention).
What you can't do is use the return value of ExecuteNonQuery
(Execute
in dapper), as this is an unrelated value (and is usually wrong and/or empty and/or useless - don't use it pretty much ever).
edited Nov 14 '18 at 11:12
answered Nov 14 '18 at 11:02


Marc Gravell♦Marc Gravell
783k19521402547
783k19521402547
Oh, i dunno.. ExecuteNonQuery on a CRUD is pretty vital for getting optimistic concurrency to work out properly
– Caius Jard
Nov 14 '18 at 11:05
@CaiusJard nothing wrong withExecuteNonQuery
, but the return value is not guaranteed to be set at all; if you want to know a rowcount, for example, then I would strongly recommentselect @@rowcount
- or if you really want to useExecuteNonQuery
,set @someOutArg = @@rowcount
, orreturn @@rowcount
, which is accessed virtually identically to the@someOutArg
API, and is not the return value ofExecuteNonQuery
– Marc Gravell♦
Nov 14 '18 at 11:07
OK.. Though have you ever run an plain oldINSERT/UPDATE/DELETE
query and NOT had ExecuteNonQuery return the number of rows affected?
– Caius Jard
Nov 14 '18 at 11:12
thanks for the reply, unfortunately (int)cmd.ExecuteScalar() still returns 0
– HiSpy
Nov 14 '18 at 11:13
@CaiusJard yes, very often; a: a lot of places make it standard practice toSET NOCOUNT ON
to slightly reduce TDS traffic; b: if tables have triggers, those triggers can cause very confusing results from this
– Marc Gravell♦
Nov 14 '18 at 11:14
|
show 2 more comments
Oh, i dunno.. ExecuteNonQuery on a CRUD is pretty vital for getting optimistic concurrency to work out properly
– Caius Jard
Nov 14 '18 at 11:05
@CaiusJard nothing wrong withExecuteNonQuery
, but the return value is not guaranteed to be set at all; if you want to know a rowcount, for example, then I would strongly recommentselect @@rowcount
- or if you really want to useExecuteNonQuery
,set @someOutArg = @@rowcount
, orreturn @@rowcount
, which is accessed virtually identically to the@someOutArg
API, and is not the return value ofExecuteNonQuery
– Marc Gravell♦
Nov 14 '18 at 11:07
OK.. Though have you ever run an plain oldINSERT/UPDATE/DELETE
query and NOT had ExecuteNonQuery return the number of rows affected?
– Caius Jard
Nov 14 '18 at 11:12
thanks for the reply, unfortunately (int)cmd.ExecuteScalar() still returns 0
– HiSpy
Nov 14 '18 at 11:13
@CaiusJard yes, very often; a: a lot of places make it standard practice toSET NOCOUNT ON
to slightly reduce TDS traffic; b: if tables have triggers, those triggers can cause very confusing results from this
– Marc Gravell♦
Nov 14 '18 at 11:14
Oh, i dunno.. ExecuteNonQuery on a CRUD is pretty vital for getting optimistic concurrency to work out properly
– Caius Jard
Nov 14 '18 at 11:05
Oh, i dunno.. ExecuteNonQuery on a CRUD is pretty vital for getting optimistic concurrency to work out properly
– Caius Jard
Nov 14 '18 at 11:05
@CaiusJard nothing wrong with
ExecuteNonQuery
, but the return value is not guaranteed to be set at all; if you want to know a rowcount, for example, then I would strongly recomment select @@rowcount
- or if you really want to use ExecuteNonQuery
, set @someOutArg = @@rowcount
, or return @@rowcount
, which is accessed virtually identically to the @someOutArg
API, and is not the return value of ExecuteNonQuery
– Marc Gravell♦
Nov 14 '18 at 11:07
@CaiusJard nothing wrong with
ExecuteNonQuery
, but the return value is not guaranteed to be set at all; if you want to know a rowcount, for example, then I would strongly recomment select @@rowcount
- or if you really want to use ExecuteNonQuery
, set @someOutArg = @@rowcount
, or return @@rowcount
, which is accessed virtually identically to the @someOutArg
API, and is not the return value of ExecuteNonQuery
– Marc Gravell♦
Nov 14 '18 at 11:07
OK.. Though have you ever run an plain old
INSERT/UPDATE/DELETE
query and NOT had ExecuteNonQuery return the number of rows affected?– Caius Jard
Nov 14 '18 at 11:12
OK.. Though have you ever run an plain old
INSERT/UPDATE/DELETE
query and NOT had ExecuteNonQuery return the number of rows affected?– Caius Jard
Nov 14 '18 at 11:12
thanks for the reply, unfortunately (int)cmd.ExecuteScalar() still returns 0
– HiSpy
Nov 14 '18 at 11:13
thanks for the reply, unfortunately (int)cmd.ExecuteScalar() still returns 0
– HiSpy
Nov 14 '18 at 11:13
@CaiusJard yes, very often; a: a lot of places make it standard practice to
SET NOCOUNT ON
to slightly reduce TDS traffic; b: if tables have triggers, those triggers can cause very confusing results from this– Marc Gravell♦
Nov 14 '18 at 11:14
@CaiusJard yes, very often; a: a lot of places make it standard practice to
SET NOCOUNT ON
to slightly reduce TDS traffic; b: if tables have triggers, those triggers can cause very confusing results from this– Marc Gravell♦
Nov 14 '18 at 11:14
|
show 2 more comments
Try this C# (might have minor syntax errors - didn't write/run it in VS). If you still get 0 out of it, you're querying an empty db:
int count = 0;
using (SqlConnection connection = new SqlConnection(connectionString))
{
try{
connection.Open();
foreach (var table in tables)
{
try{
var cmd = "SELECT COUNT(*) FROM " + table;
using(var sc as new SqlCommand(cmd, connection)){
count += (int)sc.ExecuteScalar();
}
}
catch {}
}
}finally{
connection.Close();
}
}
add a comment |
Try this C# (might have minor syntax errors - didn't write/run it in VS). If you still get 0 out of it, you're querying an empty db:
int count = 0;
using (SqlConnection connection = new SqlConnection(connectionString))
{
try{
connection.Open();
foreach (var table in tables)
{
try{
var cmd = "SELECT COUNT(*) FROM " + table;
using(var sc as new SqlCommand(cmd, connection)){
count += (int)sc.ExecuteScalar();
}
}
catch {}
}
}finally{
connection.Close();
}
}
add a comment |
Try this C# (might have minor syntax errors - didn't write/run it in VS). If you still get 0 out of it, you're querying an empty db:
int count = 0;
using (SqlConnection connection = new SqlConnection(connectionString))
{
try{
connection.Open();
foreach (var table in tables)
{
try{
var cmd = "SELECT COUNT(*) FROM " + table;
using(var sc as new SqlCommand(cmd, connection)){
count += (int)sc.ExecuteScalar();
}
}
catch {}
}
}finally{
connection.Close();
}
}
Try this C# (might have minor syntax errors - didn't write/run it in VS). If you still get 0 out of it, you're querying an empty db:
int count = 0;
using (SqlConnection connection = new SqlConnection(connectionString))
{
try{
connection.Open();
foreach (var table in tables)
{
try{
var cmd = "SELECT COUNT(*) FROM " + table;
using(var sc as new SqlCommand(cmd, connection)){
count += (int)sc.ExecuteScalar();
}
}
catch {}
}
}finally{
connection.Close();
}
}
answered Nov 14 '18 at 11:34


Caius JardCaius Jard
11.6k21239
11.6k21239
add a comment |
add a comment |
The best way here is to attach your sql to a command, then do
int retVal = cmd.ExecuteScalar();
should get the value back fine - it's one of several ways of doing it though.
ExecuteScalar is for instances where you want to return a single row with a single column, as you have done here.
tried ExecuteScalar, the result is still 0
– HiSpy
Nov 14 '18 at 11:19
have you confirmed zero is not the value in the variable? Try doing SET @COUNT=1; the line before you SELECT it, as a check.
– Cato
Nov 14 '18 at 11:38
add a comment |
The best way here is to attach your sql to a command, then do
int retVal = cmd.ExecuteScalar();
should get the value back fine - it's one of several ways of doing it though.
ExecuteScalar is for instances where you want to return a single row with a single column, as you have done here.
tried ExecuteScalar, the result is still 0
– HiSpy
Nov 14 '18 at 11:19
have you confirmed zero is not the value in the variable? Try doing SET @COUNT=1; the line before you SELECT it, as a check.
– Cato
Nov 14 '18 at 11:38
add a comment |
The best way here is to attach your sql to a command, then do
int retVal = cmd.ExecuteScalar();
should get the value back fine - it's one of several ways of doing it though.
ExecuteScalar is for instances where you want to return a single row with a single column, as you have done here.
The best way here is to attach your sql to a command, then do
int retVal = cmd.ExecuteScalar();
should get the value back fine - it's one of several ways of doing it though.
ExecuteScalar is for instances where you want to return a single row with a single column, as you have done here.
answered Nov 14 '18 at 11:03
CatoCato
2,912210
2,912210
tried ExecuteScalar, the result is still 0
– HiSpy
Nov 14 '18 at 11:19
have you confirmed zero is not the value in the variable? Try doing SET @COUNT=1; the line before you SELECT it, as a check.
– Cato
Nov 14 '18 at 11:38
add a comment |
tried ExecuteScalar, the result is still 0
– HiSpy
Nov 14 '18 at 11:19
have you confirmed zero is not the value in the variable? Try doing SET @COUNT=1; the line before you SELECT it, as a check.
– Cato
Nov 14 '18 at 11:38
tried ExecuteScalar, the result is still 0
– HiSpy
Nov 14 '18 at 11:19
tried ExecuteScalar, the result is still 0
– HiSpy
Nov 14 '18 at 11:19
have you confirmed zero is not the value in the variable? Try doing SET @COUNT=1; the line before you SELECT it, as a check.
– Cato
Nov 14 '18 at 11:38
have you confirmed zero is not the value in the variable? Try doing SET @COUNT=1; the line before you SELECT it, as a check.
– Cato
Nov 14 '18 at 11:38
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53298550%2fc-sharp-wont-read-updated-version-of-sql-variable%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
q Bslh TpxIIKPa
1
We need code, please post what you've tried.
– Barr J
Nov 14 '18 at 10:57
In c# you have two commands 1) Insert 2) Update. When using ExecuteNonQuery() you have to check the return value which is the number of rows affected. If you get a return value of zero it means no rows changed. If you are doing an INSERT it means the key already exists in the table and then you have to use UPDATE to change the existing key value. If you are doing UPDATE it means the key doesn't exist in table and then you have to do a INSERT to put a new key into the table.
– jdweng
Nov 14 '18 at 11:02
no matter how I try to load the result in C# the original value always gets returned where is this C# you talk of?
– Liam
Nov 14 '18 at 11:04
I've updated my answer based on the edits...
– Marc Gravell♦
Nov 14 '18 at 11:11
1
Also, make sure you're executing the query on the same database that C# is; wouldn't be the first time I've had someone say "oh, i was testing the query on my prod DB with 4252 rows but I forgot my local debug DB that c# was using is actually empty..."
– Caius Jard
Nov 14 '18 at 11:21