Send MP3 audio extracted from m3u8 stream to IBM Watson Speech To Text
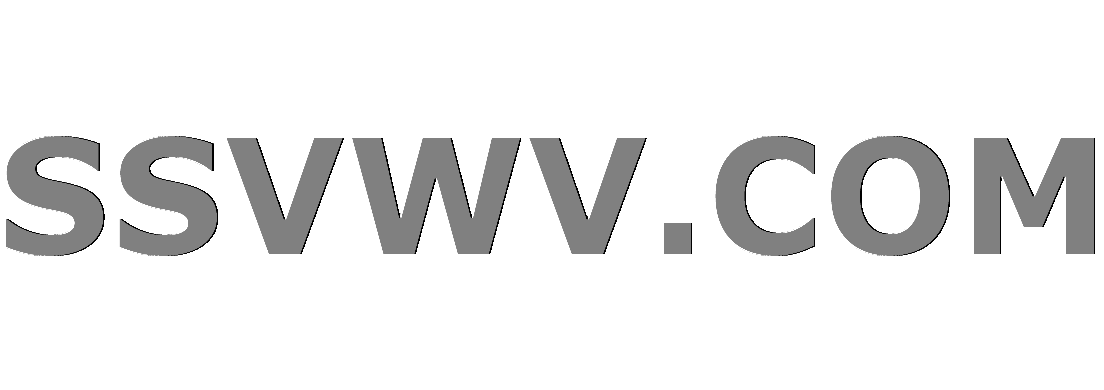
Multi tool use
I'm extracting audio in MP3 format from a M3U8 live url and the final goal is to send the live audio stream to IBM Watson Speech To Text. The m3u8 is obtained by calling an external script via a Process. Then I use FFMPEG script to get the audio in stdout. It works if I save the audio in a file but I don't want to save the extracted audio, I need to send the datas directly to the STT service. So far I proceeded like this:
SpeechToTextService speechToTextService = new SpeechToTextService(sttUsername, sttPassword);
string m3u8Url = "https://something.m3u8";
char buffer = new char[48000];
Process ffmpeg = new ProcessHelper(@"ffmpegffmpeg.exe", $"-v 0 -i {m3u8Url} -acodec mp3 -ac 2 -ar 48000 -f mp3 -");
ffmpeg.Start();
int count;
while ((count = ffmpeg.StandardOutput.Read(buffer, 0, 48000)) > 0)
{
ffmpeg.StandardOutput.Read(buffer, 0, 48000);
var answer = speechToTextService.RecognizeSessionless(
audio: buffer.Select(c => (byte)c).ToArray(),
contentType: "audio/mpeg",
smartFormatting: true,
speakerLabels: false,
model: "en-US_BroadbandModel"
);
// Get answer.ResponseJson, deserializing, clean buffer, etc...
}
When requesting the transcribed audio I'm getting this error:
An unhandled exception of type 'System.AggregateException' occurred in IBM.WatsonDeveloperCloud.SpeechToText.v1.dll: 'One or more errors occurred. (The API query failed with status code BadRequest: Bad Request | x-global-transaction-id: bd6cd203720a70d83b9a03451fe28973 | X-DP-Watson-Tran-ID: bd6cd203720a70d83b9a03451fe28973)'
Inner exceptions found, see $exception in variables window for more details.
Innermost exception IBM.WatsonDeveloperCloud.Http.Exceptions.ServiceResponseException : The API query failed with status code BadRequest: Bad Request | x-global-transaction-id: bd6cd203720a70d83b9a03451fe28973 | X-DP-Watson-Tran-ID: bd6cd203720a70d83b9a03451fe28973
at IBM.WatsonDeveloperCloud.Http.Filters.ErrorFilter.OnResponse(IResponse response, HttpResponseMessage responseMessage)
at IBM.WatsonDeveloperCloud.Http.Request.<GetResponse>d__30.MoveNext()
at System.Runtime.ExceptionServices.ExceptionDispatchInfo.Throw()
at System.Runtime.CompilerServices.TaskAwaiter.HandleNonSuccessAndDebuggerNotification(Task task)
at IBM.WatsonDeveloperCloud.Http.Request.<AsMessage>d__23.MoveNext()
at System.Runtime.ExceptionServices.ExceptionDispatchInfo.Throw()
at System.Runtime.CompilerServices.TaskAwaiter.HandleNonSuccessAndDebuggerNotification(Task task)
at IBM.WatsonDeveloperCloud.Http.Request.<As>d__24`1.MoveNext()
ProcessHelper is just for convenience:
class ProcessHelper : Process
{
private string command;
private string arguments;
public ProcessHelper(string command, string arguments, bool redirectStandardOutput = true)
{
this.command = command;
this.arguments = arguments;
StartInfo = new ProcessStartInfo()
{
FileName = this.command,
Arguments = this.arguments,
UseShellExecute = false,
RedirectStandardOutput = redirectStandardOutput,
CreateNoWindow = true
};
}
}
Pretty sure I'm doing it wrong, I'd love someone to shine a light on this. Thanks.
c# audio ffmpeg ibm-watson m3u8
add a comment |
I'm extracting audio in MP3 format from a M3U8 live url and the final goal is to send the live audio stream to IBM Watson Speech To Text. The m3u8 is obtained by calling an external script via a Process. Then I use FFMPEG script to get the audio in stdout. It works if I save the audio in a file but I don't want to save the extracted audio, I need to send the datas directly to the STT service. So far I proceeded like this:
SpeechToTextService speechToTextService = new SpeechToTextService(sttUsername, sttPassword);
string m3u8Url = "https://something.m3u8";
char buffer = new char[48000];
Process ffmpeg = new ProcessHelper(@"ffmpegffmpeg.exe", $"-v 0 -i {m3u8Url} -acodec mp3 -ac 2 -ar 48000 -f mp3 -");
ffmpeg.Start();
int count;
while ((count = ffmpeg.StandardOutput.Read(buffer, 0, 48000)) > 0)
{
ffmpeg.StandardOutput.Read(buffer, 0, 48000);
var answer = speechToTextService.RecognizeSessionless(
audio: buffer.Select(c => (byte)c).ToArray(),
contentType: "audio/mpeg",
smartFormatting: true,
speakerLabels: false,
model: "en-US_BroadbandModel"
);
// Get answer.ResponseJson, deserializing, clean buffer, etc...
}
When requesting the transcribed audio I'm getting this error:
An unhandled exception of type 'System.AggregateException' occurred in IBM.WatsonDeveloperCloud.SpeechToText.v1.dll: 'One or more errors occurred. (The API query failed with status code BadRequest: Bad Request | x-global-transaction-id: bd6cd203720a70d83b9a03451fe28973 | X-DP-Watson-Tran-ID: bd6cd203720a70d83b9a03451fe28973)'
Inner exceptions found, see $exception in variables window for more details.
Innermost exception IBM.WatsonDeveloperCloud.Http.Exceptions.ServiceResponseException : The API query failed with status code BadRequest: Bad Request | x-global-transaction-id: bd6cd203720a70d83b9a03451fe28973 | X-DP-Watson-Tran-ID: bd6cd203720a70d83b9a03451fe28973
at IBM.WatsonDeveloperCloud.Http.Filters.ErrorFilter.OnResponse(IResponse response, HttpResponseMessage responseMessage)
at IBM.WatsonDeveloperCloud.Http.Request.<GetResponse>d__30.MoveNext()
at System.Runtime.ExceptionServices.ExceptionDispatchInfo.Throw()
at System.Runtime.CompilerServices.TaskAwaiter.HandleNonSuccessAndDebuggerNotification(Task task)
at IBM.WatsonDeveloperCloud.Http.Request.<AsMessage>d__23.MoveNext()
at System.Runtime.ExceptionServices.ExceptionDispatchInfo.Throw()
at System.Runtime.CompilerServices.TaskAwaiter.HandleNonSuccessAndDebuggerNotification(Task task)
at IBM.WatsonDeveloperCloud.Http.Request.<As>d__24`1.MoveNext()
ProcessHelper is just for convenience:
class ProcessHelper : Process
{
private string command;
private string arguments;
public ProcessHelper(string command, string arguments, bool redirectStandardOutput = true)
{
this.command = command;
this.arguments = arguments;
StartInfo = new ProcessStartInfo()
{
FileName = this.command,
Arguments = this.arguments,
UseShellExecute = false,
RedirectStandardOutput = redirectStandardOutput,
CreateNoWindow = true
};
}
}
Pretty sure I'm doing it wrong, I'd love someone to shine a light on this. Thanks.
c# audio ffmpeg ibm-watson m3u8
You may be looking for something mentioned here - stackoverflow.com/questions/46179447/…
– Vidyasagar Machupalli
Nov 16 '18 at 3:04
add a comment |
I'm extracting audio in MP3 format from a M3U8 live url and the final goal is to send the live audio stream to IBM Watson Speech To Text. The m3u8 is obtained by calling an external script via a Process. Then I use FFMPEG script to get the audio in stdout. It works if I save the audio in a file but I don't want to save the extracted audio, I need to send the datas directly to the STT service. So far I proceeded like this:
SpeechToTextService speechToTextService = new SpeechToTextService(sttUsername, sttPassword);
string m3u8Url = "https://something.m3u8";
char buffer = new char[48000];
Process ffmpeg = new ProcessHelper(@"ffmpegffmpeg.exe", $"-v 0 -i {m3u8Url} -acodec mp3 -ac 2 -ar 48000 -f mp3 -");
ffmpeg.Start();
int count;
while ((count = ffmpeg.StandardOutput.Read(buffer, 0, 48000)) > 0)
{
ffmpeg.StandardOutput.Read(buffer, 0, 48000);
var answer = speechToTextService.RecognizeSessionless(
audio: buffer.Select(c => (byte)c).ToArray(),
contentType: "audio/mpeg",
smartFormatting: true,
speakerLabels: false,
model: "en-US_BroadbandModel"
);
// Get answer.ResponseJson, deserializing, clean buffer, etc...
}
When requesting the transcribed audio I'm getting this error:
An unhandled exception of type 'System.AggregateException' occurred in IBM.WatsonDeveloperCloud.SpeechToText.v1.dll: 'One or more errors occurred. (The API query failed with status code BadRequest: Bad Request | x-global-transaction-id: bd6cd203720a70d83b9a03451fe28973 | X-DP-Watson-Tran-ID: bd6cd203720a70d83b9a03451fe28973)'
Inner exceptions found, see $exception in variables window for more details.
Innermost exception IBM.WatsonDeveloperCloud.Http.Exceptions.ServiceResponseException : The API query failed with status code BadRequest: Bad Request | x-global-transaction-id: bd6cd203720a70d83b9a03451fe28973 | X-DP-Watson-Tran-ID: bd6cd203720a70d83b9a03451fe28973
at IBM.WatsonDeveloperCloud.Http.Filters.ErrorFilter.OnResponse(IResponse response, HttpResponseMessage responseMessage)
at IBM.WatsonDeveloperCloud.Http.Request.<GetResponse>d__30.MoveNext()
at System.Runtime.ExceptionServices.ExceptionDispatchInfo.Throw()
at System.Runtime.CompilerServices.TaskAwaiter.HandleNonSuccessAndDebuggerNotification(Task task)
at IBM.WatsonDeveloperCloud.Http.Request.<AsMessage>d__23.MoveNext()
at System.Runtime.ExceptionServices.ExceptionDispatchInfo.Throw()
at System.Runtime.CompilerServices.TaskAwaiter.HandleNonSuccessAndDebuggerNotification(Task task)
at IBM.WatsonDeveloperCloud.Http.Request.<As>d__24`1.MoveNext()
ProcessHelper is just for convenience:
class ProcessHelper : Process
{
private string command;
private string arguments;
public ProcessHelper(string command, string arguments, bool redirectStandardOutput = true)
{
this.command = command;
this.arguments = arguments;
StartInfo = new ProcessStartInfo()
{
FileName = this.command,
Arguments = this.arguments,
UseShellExecute = false,
RedirectStandardOutput = redirectStandardOutput,
CreateNoWindow = true
};
}
}
Pretty sure I'm doing it wrong, I'd love someone to shine a light on this. Thanks.
c# audio ffmpeg ibm-watson m3u8
I'm extracting audio in MP3 format from a M3U8 live url and the final goal is to send the live audio stream to IBM Watson Speech To Text. The m3u8 is obtained by calling an external script via a Process. Then I use FFMPEG script to get the audio in stdout. It works if I save the audio in a file but I don't want to save the extracted audio, I need to send the datas directly to the STT service. So far I proceeded like this:
SpeechToTextService speechToTextService = new SpeechToTextService(sttUsername, sttPassword);
string m3u8Url = "https://something.m3u8";
char buffer = new char[48000];
Process ffmpeg = new ProcessHelper(@"ffmpegffmpeg.exe", $"-v 0 -i {m3u8Url} -acodec mp3 -ac 2 -ar 48000 -f mp3 -");
ffmpeg.Start();
int count;
while ((count = ffmpeg.StandardOutput.Read(buffer, 0, 48000)) > 0)
{
ffmpeg.StandardOutput.Read(buffer, 0, 48000);
var answer = speechToTextService.RecognizeSessionless(
audio: buffer.Select(c => (byte)c).ToArray(),
contentType: "audio/mpeg",
smartFormatting: true,
speakerLabels: false,
model: "en-US_BroadbandModel"
);
// Get answer.ResponseJson, deserializing, clean buffer, etc...
}
When requesting the transcribed audio I'm getting this error:
An unhandled exception of type 'System.AggregateException' occurred in IBM.WatsonDeveloperCloud.SpeechToText.v1.dll: 'One or more errors occurred. (The API query failed with status code BadRequest: Bad Request | x-global-transaction-id: bd6cd203720a70d83b9a03451fe28973 | X-DP-Watson-Tran-ID: bd6cd203720a70d83b9a03451fe28973)'
Inner exceptions found, see $exception in variables window for more details.
Innermost exception IBM.WatsonDeveloperCloud.Http.Exceptions.ServiceResponseException : The API query failed with status code BadRequest: Bad Request | x-global-transaction-id: bd6cd203720a70d83b9a03451fe28973 | X-DP-Watson-Tran-ID: bd6cd203720a70d83b9a03451fe28973
at IBM.WatsonDeveloperCloud.Http.Filters.ErrorFilter.OnResponse(IResponse response, HttpResponseMessage responseMessage)
at IBM.WatsonDeveloperCloud.Http.Request.<GetResponse>d__30.MoveNext()
at System.Runtime.ExceptionServices.ExceptionDispatchInfo.Throw()
at System.Runtime.CompilerServices.TaskAwaiter.HandleNonSuccessAndDebuggerNotification(Task task)
at IBM.WatsonDeveloperCloud.Http.Request.<AsMessage>d__23.MoveNext()
at System.Runtime.ExceptionServices.ExceptionDispatchInfo.Throw()
at System.Runtime.CompilerServices.TaskAwaiter.HandleNonSuccessAndDebuggerNotification(Task task)
at IBM.WatsonDeveloperCloud.Http.Request.<As>d__24`1.MoveNext()
ProcessHelper is just for convenience:
class ProcessHelper : Process
{
private string command;
private string arguments;
public ProcessHelper(string command, string arguments, bool redirectStandardOutput = true)
{
this.command = command;
this.arguments = arguments;
StartInfo = new ProcessStartInfo()
{
FileName = this.command,
Arguments = this.arguments,
UseShellExecute = false,
RedirectStandardOutput = redirectStandardOutput,
CreateNoWindow = true
};
}
}
Pretty sure I'm doing it wrong, I'd love someone to shine a light on this. Thanks.
c# audio ffmpeg ibm-watson m3u8
c# audio ffmpeg ibm-watson m3u8
edited Nov 14 '18 at 15:57
Link69
asked Nov 14 '18 at 15:22


Link69Link69
13
13
You may be looking for something mentioned here - stackoverflow.com/questions/46179447/…
– Vidyasagar Machupalli
Nov 16 '18 at 3:04
add a comment |
You may be looking for something mentioned here - stackoverflow.com/questions/46179447/…
– Vidyasagar Machupalli
Nov 16 '18 at 3:04
You may be looking for something mentioned here - stackoverflow.com/questions/46179447/…
– Vidyasagar Machupalli
Nov 16 '18 at 3:04
You may be looking for something mentioned here - stackoverflow.com/questions/46179447/…
– Vidyasagar Machupalli
Nov 16 '18 at 3:04
add a comment |
1 Answer
1
active
oldest
votes
I still don't know why I can't recognizesessionless my buffer (the second ffmpeg.StandardOutput.Read(buffer, 0, 48000); was a typo btw) but I managed to make it work with websockets like explained there https://gist.github.com/nfriedly/0240e862901474a9447a600e5795d500
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53303499%2fsend-mp3-audio-extracted-from-m3u8-stream-to-ibm-watson-speech-to-text%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I still don't know why I can't recognizesessionless my buffer (the second ffmpeg.StandardOutput.Read(buffer, 0, 48000); was a typo btw) but I managed to make it work with websockets like explained there https://gist.github.com/nfriedly/0240e862901474a9447a600e5795d500
add a comment |
I still don't know why I can't recognizesessionless my buffer (the second ffmpeg.StandardOutput.Read(buffer, 0, 48000); was a typo btw) but I managed to make it work with websockets like explained there https://gist.github.com/nfriedly/0240e862901474a9447a600e5795d500
add a comment |
I still don't know why I can't recognizesessionless my buffer (the second ffmpeg.StandardOutput.Read(buffer, 0, 48000); was a typo btw) but I managed to make it work with websockets like explained there https://gist.github.com/nfriedly/0240e862901474a9447a600e5795d500
I still don't know why I can't recognizesessionless my buffer (the second ffmpeg.StandardOutput.Read(buffer, 0, 48000); was a typo btw) but I managed to make it work with websockets like explained there https://gist.github.com/nfriedly/0240e862901474a9447a600e5795d500
answered Nov 20 '18 at 14:08


Link69Link69
13
13
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53303499%2fsend-mp3-audio-extracted-from-m3u8-stream-to-ibm-watson-speech-to-text%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
SHJIPmeY0t6ij
You may be looking for something mentioned here - stackoverflow.com/questions/46179447/…
– Vidyasagar Machupalli
Nov 16 '18 at 3:04