jQuery infinite loop through each div
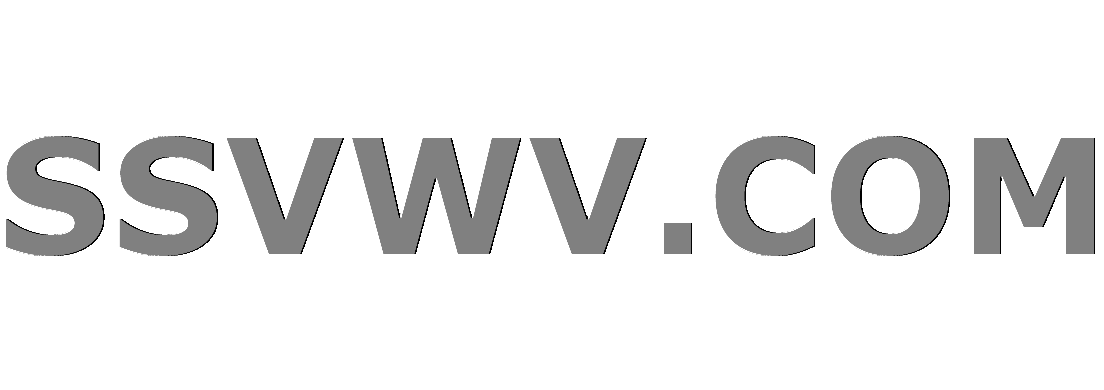
Multi tool use
I have some divs with divs in them, which I want to loop through while removing a class from the current one, then adding a class to the next one.
Then when I reach the last one, I want to go back to the start and do it all over again.
I already have a way of doing this, but it only works for one set of divs, I'm going to be having many sets of divs that need to loop independently.
Here's an example few sets (there would be a random amount of divs in each):
<div class="set">
<div class="current">Item1</div>
<div>Item2</div>
<div>Item3</div>
</div>
<div class="set">
<div class="current">Item1</div>
<div>Item2</div>
<div>Item3</div>
<div>Item4</div>
<div>Item5</div>
</div>
I need to remove that current class, and add it to the next div in each set, and so on.
Here is the code I have that works with one set:
$(function() {
var items = $('.set div').length;
current = 0;
setInterval(function() {
$('.set div').eq(current).removeClass('current');
if (current == items - 1){
current = 0;
} else {
current++;
}
$('.set div').eq(current).addClass('current');
}, 500);
});
jquery infinite-loop
add a comment |
I have some divs with divs in them, which I want to loop through while removing a class from the current one, then adding a class to the next one.
Then when I reach the last one, I want to go back to the start and do it all over again.
I already have a way of doing this, but it only works for one set of divs, I'm going to be having many sets of divs that need to loop independently.
Here's an example few sets (there would be a random amount of divs in each):
<div class="set">
<div class="current">Item1</div>
<div>Item2</div>
<div>Item3</div>
</div>
<div class="set">
<div class="current">Item1</div>
<div>Item2</div>
<div>Item3</div>
<div>Item4</div>
<div>Item5</div>
</div>
I need to remove that current class, and add it to the next div in each set, and so on.
Here is the code I have that works with one set:
$(function() {
var items = $('.set div').length;
current = 0;
setInterval(function() {
$('.set div').eq(current).removeClass('current');
if (current == items - 1){
current = 0;
} else {
current++;
}
$('.set div').eq(current).addClass('current');
}, 500);
});
jquery infinite-loop
posting your current jquery code could help, if you already have a method for doing it to one set of divs usingeach()
for all divs of class "set" should solve the problem, or am I mistaken?
– optimusprime619
Jun 8 '12 at 12:47
would all parent divs haveclass="set"
? and when you say that you'll have "many sets of divs that need to loop independently" are you saying that while all sets have a parent div withclass="set"
, the number of setsn
to work on would be anywhere from 1 ton
?
– jimmym715
Jun 8 '12 at 12:49
add a comment |
I have some divs with divs in them, which I want to loop through while removing a class from the current one, then adding a class to the next one.
Then when I reach the last one, I want to go back to the start and do it all over again.
I already have a way of doing this, but it only works for one set of divs, I'm going to be having many sets of divs that need to loop independently.
Here's an example few sets (there would be a random amount of divs in each):
<div class="set">
<div class="current">Item1</div>
<div>Item2</div>
<div>Item3</div>
</div>
<div class="set">
<div class="current">Item1</div>
<div>Item2</div>
<div>Item3</div>
<div>Item4</div>
<div>Item5</div>
</div>
I need to remove that current class, and add it to the next div in each set, and so on.
Here is the code I have that works with one set:
$(function() {
var items = $('.set div').length;
current = 0;
setInterval(function() {
$('.set div').eq(current).removeClass('current');
if (current == items - 1){
current = 0;
} else {
current++;
}
$('.set div').eq(current).addClass('current');
}, 500);
});
jquery infinite-loop
I have some divs with divs in them, which I want to loop through while removing a class from the current one, then adding a class to the next one.
Then when I reach the last one, I want to go back to the start and do it all over again.
I already have a way of doing this, but it only works for one set of divs, I'm going to be having many sets of divs that need to loop independently.
Here's an example few sets (there would be a random amount of divs in each):
<div class="set">
<div class="current">Item1</div>
<div>Item2</div>
<div>Item3</div>
</div>
<div class="set">
<div class="current">Item1</div>
<div>Item2</div>
<div>Item3</div>
<div>Item4</div>
<div>Item5</div>
</div>
I need to remove that current class, and add it to the next div in each set, and so on.
Here is the code I have that works with one set:
$(function() {
var items = $('.set div').length;
current = 0;
setInterval(function() {
$('.set div').eq(current).removeClass('current');
if (current == items - 1){
current = 0;
} else {
current++;
}
$('.set div').eq(current).addClass('current');
}, 500);
});
jquery infinite-loop
jquery infinite-loop
edited Jun 8 '12 at 12:53
Dentor Uman
asked Jun 8 '12 at 12:43
Dentor UmanDentor Uman
2314
2314
posting your current jquery code could help, if you already have a method for doing it to one set of divs usingeach()
for all divs of class "set" should solve the problem, or am I mistaken?
– optimusprime619
Jun 8 '12 at 12:47
would all parent divs haveclass="set"
? and when you say that you'll have "many sets of divs that need to loop independently" are you saying that while all sets have a parent div withclass="set"
, the number of setsn
to work on would be anywhere from 1 ton
?
– jimmym715
Jun 8 '12 at 12:49
add a comment |
posting your current jquery code could help, if you already have a method for doing it to one set of divs usingeach()
for all divs of class "set" should solve the problem, or am I mistaken?
– optimusprime619
Jun 8 '12 at 12:47
would all parent divs haveclass="set"
? and when you say that you'll have "many sets of divs that need to loop independently" are you saying that while all sets have a parent div withclass="set"
, the number of setsn
to work on would be anywhere from 1 ton
?
– jimmym715
Jun 8 '12 at 12:49
posting your current jquery code could help, if you already have a method for doing it to one set of divs using
each()
for all divs of class "set" should solve the problem, or am I mistaken?– optimusprime619
Jun 8 '12 at 12:47
posting your current jquery code could help, if you already have a method for doing it to one set of divs using
each()
for all divs of class "set" should solve the problem, or am I mistaken?– optimusprime619
Jun 8 '12 at 12:47
would all parent divs have
class="set"
? and when you say that you'll have "many sets of divs that need to loop independently" are you saying that while all sets have a parent div with class="set"
, the number of sets n
to work on would be anywhere from 1 to n
?– jimmym715
Jun 8 '12 at 12:49
would all parent divs have
class="set"
? and when you say that you'll have "many sets of divs that need to loop independently" are you saying that while all sets have a parent div with class="set"
, the number of sets n
to work on would be anywhere from 1 to n
?– jimmym715
Jun 8 '12 at 12:49
add a comment |
3 Answers
3
active
oldest
votes
my take:
http://jsfiddle.net/yyY28/
$('.set').each(function(){
(function($set){
setInterval(function(){
var $cur = $set.find('.current').removeClass('current');
var $next = $cur.next().length?$cur.next():$set.children().eq(0);
$next.addClass('current');
},1000);
})($(this));
});
version 2:
setInterval(function(){
$('.set').each(function(){
var $cur = $(this).find('.current').removeClass('current');
var $next = $cur.next().length?$cur.next():$(this).children().eq(0);
$next.addClass('current');
});
},1000);
That works perfectly, and it's actually less code than my original. Amazing! Thanks!
– Dentor Uman
Jun 8 '12 at 13:10
You assign each.set
a newsetInterval
, so in couple of minutes the sets will run out of synchronous work.
– VisioN
Jun 8 '12 at 13:19
@VisioN good point. I added a second version which can't run out of sync and is more elegant as well. edit: oh it's almost exactly your version now :P
– Andy
Jun 8 '12 at 13:27
@Andy As good as mine :D
– VisioN
Jun 8 '12 at 13:27
add a comment |
Maybe I didn't get you right, but is that you are looking for?
function loop() {
$(".set").each(function() {
var current = $(this).children(".current").removeClass("current");
var i = current.next().length ? current.index() : 0;
current.siblings(":eq(" + i + ")").addClass("current");
});
}
setInterval(loop, 500);
DEMO: http://jsfiddle.net/jGcsh/
add a comment |
try this one:
function changeCurrent(){
$('.set').each(function(){
var currentSubDiv = $(this).children('.current');
currentSubDiv.removeClass('current');
currentSubDiv.next().addClass('current');
})
}
setInterval(changeCurrent,timeInMillis);
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f10949086%2fjquery-infinite-loop-through-each-div%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
my take:
http://jsfiddle.net/yyY28/
$('.set').each(function(){
(function($set){
setInterval(function(){
var $cur = $set.find('.current').removeClass('current');
var $next = $cur.next().length?$cur.next():$set.children().eq(0);
$next.addClass('current');
},1000);
})($(this));
});
version 2:
setInterval(function(){
$('.set').each(function(){
var $cur = $(this).find('.current').removeClass('current');
var $next = $cur.next().length?$cur.next():$(this).children().eq(0);
$next.addClass('current');
});
},1000);
That works perfectly, and it's actually less code than my original. Amazing! Thanks!
– Dentor Uman
Jun 8 '12 at 13:10
You assign each.set
a newsetInterval
, so in couple of minutes the sets will run out of synchronous work.
– VisioN
Jun 8 '12 at 13:19
@VisioN good point. I added a second version which can't run out of sync and is more elegant as well. edit: oh it's almost exactly your version now :P
– Andy
Jun 8 '12 at 13:27
@Andy As good as mine :D
– VisioN
Jun 8 '12 at 13:27
add a comment |
my take:
http://jsfiddle.net/yyY28/
$('.set').each(function(){
(function($set){
setInterval(function(){
var $cur = $set.find('.current').removeClass('current');
var $next = $cur.next().length?$cur.next():$set.children().eq(0);
$next.addClass('current');
},1000);
})($(this));
});
version 2:
setInterval(function(){
$('.set').each(function(){
var $cur = $(this).find('.current').removeClass('current');
var $next = $cur.next().length?$cur.next():$(this).children().eq(0);
$next.addClass('current');
});
},1000);
That works perfectly, and it's actually less code than my original. Amazing! Thanks!
– Dentor Uman
Jun 8 '12 at 13:10
You assign each.set
a newsetInterval
, so in couple of minutes the sets will run out of synchronous work.
– VisioN
Jun 8 '12 at 13:19
@VisioN good point. I added a second version which can't run out of sync and is more elegant as well. edit: oh it's almost exactly your version now :P
– Andy
Jun 8 '12 at 13:27
@Andy As good as mine :D
– VisioN
Jun 8 '12 at 13:27
add a comment |
my take:
http://jsfiddle.net/yyY28/
$('.set').each(function(){
(function($set){
setInterval(function(){
var $cur = $set.find('.current').removeClass('current');
var $next = $cur.next().length?$cur.next():$set.children().eq(0);
$next.addClass('current');
},1000);
})($(this));
});
version 2:
setInterval(function(){
$('.set').each(function(){
var $cur = $(this).find('.current').removeClass('current');
var $next = $cur.next().length?$cur.next():$(this).children().eq(0);
$next.addClass('current');
});
},1000);
my take:
http://jsfiddle.net/yyY28/
$('.set').each(function(){
(function($set){
setInterval(function(){
var $cur = $set.find('.current').removeClass('current');
var $next = $cur.next().length?$cur.next():$set.children().eq(0);
$next.addClass('current');
},1000);
})($(this));
});
version 2:
setInterval(function(){
$('.set').each(function(){
var $cur = $(this).find('.current').removeClass('current');
var $next = $cur.next().length?$cur.next():$(this).children().eq(0);
$next.addClass('current');
});
},1000);
edited Jun 8 '12 at 13:25
answered Jun 8 '12 at 12:54
AndyAndy
25.7k83357
25.7k83357
That works perfectly, and it's actually less code than my original. Amazing! Thanks!
– Dentor Uman
Jun 8 '12 at 13:10
You assign each.set
a newsetInterval
, so in couple of minutes the sets will run out of synchronous work.
– VisioN
Jun 8 '12 at 13:19
@VisioN good point. I added a second version which can't run out of sync and is more elegant as well. edit: oh it's almost exactly your version now :P
– Andy
Jun 8 '12 at 13:27
@Andy As good as mine :D
– VisioN
Jun 8 '12 at 13:27
add a comment |
That works perfectly, and it's actually less code than my original. Amazing! Thanks!
– Dentor Uman
Jun 8 '12 at 13:10
You assign each.set
a newsetInterval
, so in couple of minutes the sets will run out of synchronous work.
– VisioN
Jun 8 '12 at 13:19
@VisioN good point. I added a second version which can't run out of sync and is more elegant as well. edit: oh it's almost exactly your version now :P
– Andy
Jun 8 '12 at 13:27
@Andy As good as mine :D
– VisioN
Jun 8 '12 at 13:27
That works perfectly, and it's actually less code than my original. Amazing! Thanks!
– Dentor Uman
Jun 8 '12 at 13:10
That works perfectly, and it's actually less code than my original. Amazing! Thanks!
– Dentor Uman
Jun 8 '12 at 13:10
You assign each
.set
a new setInterval
, so in couple of minutes the sets will run out of synchronous work.– VisioN
Jun 8 '12 at 13:19
You assign each
.set
a new setInterval
, so in couple of minutes the sets will run out of synchronous work.– VisioN
Jun 8 '12 at 13:19
@VisioN good point. I added a second version which can't run out of sync and is more elegant as well. edit: oh it's almost exactly your version now :P
– Andy
Jun 8 '12 at 13:27
@VisioN good point. I added a second version which can't run out of sync and is more elegant as well. edit: oh it's almost exactly your version now :P
– Andy
Jun 8 '12 at 13:27
@Andy As good as mine :D
– VisioN
Jun 8 '12 at 13:27
@Andy As good as mine :D
– VisioN
Jun 8 '12 at 13:27
add a comment |
Maybe I didn't get you right, but is that you are looking for?
function loop() {
$(".set").each(function() {
var current = $(this).children(".current").removeClass("current");
var i = current.next().length ? current.index() : 0;
current.siblings(":eq(" + i + ")").addClass("current");
});
}
setInterval(loop, 500);
DEMO: http://jsfiddle.net/jGcsh/
add a comment |
Maybe I didn't get you right, but is that you are looking for?
function loop() {
$(".set").each(function() {
var current = $(this).children(".current").removeClass("current");
var i = current.next().length ? current.index() : 0;
current.siblings(":eq(" + i + ")").addClass("current");
});
}
setInterval(loop, 500);
DEMO: http://jsfiddle.net/jGcsh/
add a comment |
Maybe I didn't get you right, but is that you are looking for?
function loop() {
$(".set").each(function() {
var current = $(this).children(".current").removeClass("current");
var i = current.next().length ? current.index() : 0;
current.siblings(":eq(" + i + ")").addClass("current");
});
}
setInterval(loop, 500);
DEMO: http://jsfiddle.net/jGcsh/
Maybe I didn't get you right, but is that you are looking for?
function loop() {
$(".set").each(function() {
var current = $(this).children(".current").removeClass("current");
var i = current.next().length ? current.index() : 0;
current.siblings(":eq(" + i + ")").addClass("current");
});
}
setInterval(loop, 500);
DEMO: http://jsfiddle.net/jGcsh/
answered Jun 8 '12 at 12:55


VisioNVisioN
112k24212229
112k24212229
add a comment |
add a comment |
try this one:
function changeCurrent(){
$('.set').each(function(){
var currentSubDiv = $(this).children('.current');
currentSubDiv.removeClass('current');
currentSubDiv.next().addClass('current');
})
}
setInterval(changeCurrent,timeInMillis);
add a comment |
try this one:
function changeCurrent(){
$('.set').each(function(){
var currentSubDiv = $(this).children('.current');
currentSubDiv.removeClass('current');
currentSubDiv.next().addClass('current');
})
}
setInterval(changeCurrent,timeInMillis);
add a comment |
try this one:
function changeCurrent(){
$('.set').each(function(){
var currentSubDiv = $(this).children('.current');
currentSubDiv.removeClass('current');
currentSubDiv.next().addClass('current');
})
}
setInterval(changeCurrent,timeInMillis);
try this one:
function changeCurrent(){
$('.set').each(function(){
var currentSubDiv = $(this).children('.current');
currentSubDiv.removeClass('current');
currentSubDiv.next().addClass('current');
})
}
setInterval(changeCurrent,timeInMillis);
edited Jun 8 '12 at 13:35
answered Jun 8 '12 at 12:53


Th0rndikeTh0rndike
2,92431331
2,92431331
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f10949086%2fjquery-infinite-loop-through-each-div%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
eEi 16FZ,u675e4OSNO1yp 7TGsrEHEojdq7AU1
posting your current jquery code could help, if you already have a method for doing it to one set of divs using
each()
for all divs of class "set" should solve the problem, or am I mistaken?– optimusprime619
Jun 8 '12 at 12:47
would all parent divs have
class="set"
? and when you say that you'll have "many sets of divs that need to loop independently" are you saying that while all sets have a parent div withclass="set"
, the number of setsn
to work on would be anywhere from 1 ton
?– jimmym715
Jun 8 '12 at 12:49