How to tell when use has answered iOS Notification Permissions request in React-Native
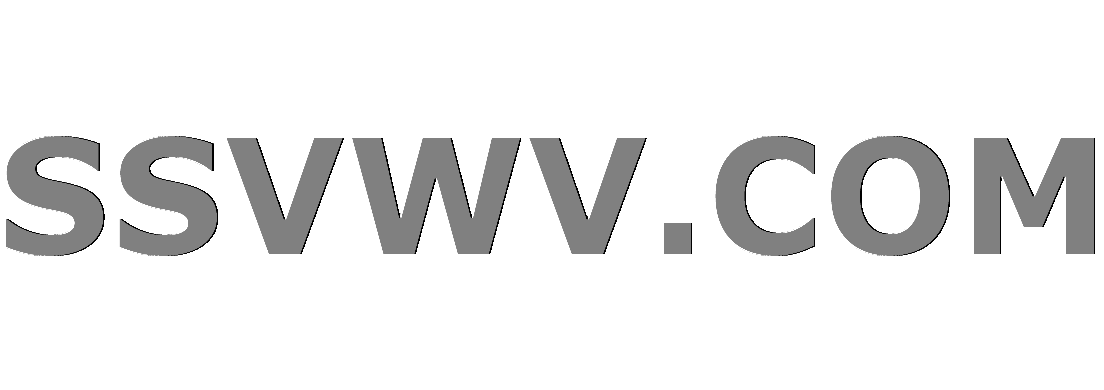
Multi tool use
in iOS, in order to allow push notifications, the user must do 1 of the following 2 options.
answer the original prompt which asks permission for notifications. If they don't do this, you can't bring up the permission request again, so you must redirect them to the settings page.
Go to the settings page and manually change the permissions.
Here's my flow now:
Check permissions: if they have them, move to the next screen.
if they don't, show an alert stating why notifications are important for our app. If they click ok, it shows the real notification screen, if not it just waits to ask later.
If the user already saw the request for notifications screen, I want to show a dialog that asks them to go the settings to allow notifications.
Is there a way of knowing whether the user said yes or no to the original permission box? Is there a way to know when they've answered it?
The way I'm checking now doesn't wait for the user to click on an option in the original dialogue box, so it doesn't wait to check permissions and find s them the exact same as they were before.
is there any way to check whether they've had the request for permissions already and whether they said yes or no?
Here's the code I'm using:
(only relevant imports)
import {PushNotificationIOS} from 'react-native
import PushNotification 'react-native-push-notification'
const requestPermissionsIOS = navigateForward => {
PushNotification.requestPermissions().then( ({alert}) => {
if (!alert) {
settingsAlertIOS(navigateForward)
} else {
configurePushNotification()
scheduleNotifications()
navigateForward()
}
}).catch( err => {
settingsAlertIOS(navigateForward)
})
}
const configurePushNotification = () => {
PushNotification.configure({
onRegister: (token) => {
},
onNotification: (notification) => {
onNotification: notification => handleNotification(notification)
},
permissions: {
alert: true,
badge: true,
sound: true
},
popInitialNotification: true,
requestPermissions: false,
});
}
const settingsAlertIOS = (navigateForward) => {
Alert.alert(
'Go to settings page?',
'In order to receive important notifications, please enable them in the settings page (leaves app)',
[
{text: 'Cancel', onPress: () => {Mixpanel.track('Decline change notifications from settings alert'); scheduleNotifications(); navigateForward()}},
{text: 'Settings', onPress: () => {configurePushNotification(); scheduleNotifications(); navigateForward(); Linking.openURL('app-settings:')}},
]
)
}
ios react-native
add a comment |
in iOS, in order to allow push notifications, the user must do 1 of the following 2 options.
answer the original prompt which asks permission for notifications. If they don't do this, you can't bring up the permission request again, so you must redirect them to the settings page.
Go to the settings page and manually change the permissions.
Here's my flow now:
Check permissions: if they have them, move to the next screen.
if they don't, show an alert stating why notifications are important for our app. If they click ok, it shows the real notification screen, if not it just waits to ask later.
If the user already saw the request for notifications screen, I want to show a dialog that asks them to go the settings to allow notifications.
Is there a way of knowing whether the user said yes or no to the original permission box? Is there a way to know when they've answered it?
The way I'm checking now doesn't wait for the user to click on an option in the original dialogue box, so it doesn't wait to check permissions and find s them the exact same as they were before.
is there any way to check whether they've had the request for permissions already and whether they said yes or no?
Here's the code I'm using:
(only relevant imports)
import {PushNotificationIOS} from 'react-native
import PushNotification 'react-native-push-notification'
const requestPermissionsIOS = navigateForward => {
PushNotification.requestPermissions().then( ({alert}) => {
if (!alert) {
settingsAlertIOS(navigateForward)
} else {
configurePushNotification()
scheduleNotifications()
navigateForward()
}
}).catch( err => {
settingsAlertIOS(navigateForward)
})
}
const configurePushNotification = () => {
PushNotification.configure({
onRegister: (token) => {
},
onNotification: (notification) => {
onNotification: notification => handleNotification(notification)
},
permissions: {
alert: true,
badge: true,
sound: true
},
popInitialNotification: true,
requestPermissions: false,
});
}
const settingsAlertIOS = (navigateForward) => {
Alert.alert(
'Go to settings page?',
'In order to receive important notifications, please enable them in the settings page (leaves app)',
[
{text: 'Cancel', onPress: () => {Mixpanel.track('Decline change notifications from settings alert'); scheduleNotifications(); navigateForward()}},
{text: 'Settings', onPress: () => {configurePushNotification(); scheduleNotifications(); navigateForward(); Linking.openURL('app-settings:')}},
]
)
}
ios react-native
the completion handler you supply torequestAuthorizationWithOptions
is called once they have responded to the dialog
– Paulw11
Nov 14 '18 at 22:08
Ok, I just saw you have tagged react-native, so the process will be slightly different but there must be an equivalent call-back when the user responds to the dialog. Edit your question to show your code
– Paulw11
Nov 14 '18 at 22:11
Can you elaborate whether you're using a 3rd party library with permissions handling (Expo, react-native-permissions etc) or are you using PushNotificationsIOS?
– zvona
Nov 14 '18 at 22:27
I've updated to show my code. I'm using a library and the built in PushNotificationsIOS.
– S S
Nov 14 '18 at 22:40
add a comment |
in iOS, in order to allow push notifications, the user must do 1 of the following 2 options.
answer the original prompt which asks permission for notifications. If they don't do this, you can't bring up the permission request again, so you must redirect them to the settings page.
Go to the settings page and manually change the permissions.
Here's my flow now:
Check permissions: if they have them, move to the next screen.
if they don't, show an alert stating why notifications are important for our app. If they click ok, it shows the real notification screen, if not it just waits to ask later.
If the user already saw the request for notifications screen, I want to show a dialog that asks them to go the settings to allow notifications.
Is there a way of knowing whether the user said yes or no to the original permission box? Is there a way to know when they've answered it?
The way I'm checking now doesn't wait for the user to click on an option in the original dialogue box, so it doesn't wait to check permissions and find s them the exact same as they were before.
is there any way to check whether they've had the request for permissions already and whether they said yes or no?
Here's the code I'm using:
(only relevant imports)
import {PushNotificationIOS} from 'react-native
import PushNotification 'react-native-push-notification'
const requestPermissionsIOS = navigateForward => {
PushNotification.requestPermissions().then( ({alert}) => {
if (!alert) {
settingsAlertIOS(navigateForward)
} else {
configurePushNotification()
scheduleNotifications()
navigateForward()
}
}).catch( err => {
settingsAlertIOS(navigateForward)
})
}
const configurePushNotification = () => {
PushNotification.configure({
onRegister: (token) => {
},
onNotification: (notification) => {
onNotification: notification => handleNotification(notification)
},
permissions: {
alert: true,
badge: true,
sound: true
},
popInitialNotification: true,
requestPermissions: false,
});
}
const settingsAlertIOS = (navigateForward) => {
Alert.alert(
'Go to settings page?',
'In order to receive important notifications, please enable them in the settings page (leaves app)',
[
{text: 'Cancel', onPress: () => {Mixpanel.track('Decline change notifications from settings alert'); scheduleNotifications(); navigateForward()}},
{text: 'Settings', onPress: () => {configurePushNotification(); scheduleNotifications(); navigateForward(); Linking.openURL('app-settings:')}},
]
)
}
ios react-native
in iOS, in order to allow push notifications, the user must do 1 of the following 2 options.
answer the original prompt which asks permission for notifications. If they don't do this, you can't bring up the permission request again, so you must redirect them to the settings page.
Go to the settings page and manually change the permissions.
Here's my flow now:
Check permissions: if they have them, move to the next screen.
if they don't, show an alert stating why notifications are important for our app. If they click ok, it shows the real notification screen, if not it just waits to ask later.
If the user already saw the request for notifications screen, I want to show a dialog that asks them to go the settings to allow notifications.
Is there a way of knowing whether the user said yes or no to the original permission box? Is there a way to know when they've answered it?
The way I'm checking now doesn't wait for the user to click on an option in the original dialogue box, so it doesn't wait to check permissions and find s them the exact same as they were before.
is there any way to check whether they've had the request for permissions already and whether they said yes or no?
Here's the code I'm using:
(only relevant imports)
import {PushNotificationIOS} from 'react-native
import PushNotification 'react-native-push-notification'
const requestPermissionsIOS = navigateForward => {
PushNotification.requestPermissions().then( ({alert}) => {
if (!alert) {
settingsAlertIOS(navigateForward)
} else {
configurePushNotification()
scheduleNotifications()
navigateForward()
}
}).catch( err => {
settingsAlertIOS(navigateForward)
})
}
const configurePushNotification = () => {
PushNotification.configure({
onRegister: (token) => {
},
onNotification: (notification) => {
onNotification: notification => handleNotification(notification)
},
permissions: {
alert: true,
badge: true,
sound: true
},
popInitialNotification: true,
requestPermissions: false,
});
}
const settingsAlertIOS = (navigateForward) => {
Alert.alert(
'Go to settings page?',
'In order to receive important notifications, please enable them in the settings page (leaves app)',
[
{text: 'Cancel', onPress: () => {Mixpanel.track('Decline change notifications from settings alert'); scheduleNotifications(); navigateForward()}},
{text: 'Settings', onPress: () => {configurePushNotification(); scheduleNotifications(); navigateForward(); Linking.openURL('app-settings:')}},
]
)
}
ios react-native
ios react-native
edited Nov 14 '18 at 22:39
S S
asked Nov 14 '18 at 22:01


S SS S
3015
3015
the completion handler you supply torequestAuthorizationWithOptions
is called once they have responded to the dialog
– Paulw11
Nov 14 '18 at 22:08
Ok, I just saw you have tagged react-native, so the process will be slightly different but there must be an equivalent call-back when the user responds to the dialog. Edit your question to show your code
– Paulw11
Nov 14 '18 at 22:11
Can you elaborate whether you're using a 3rd party library with permissions handling (Expo, react-native-permissions etc) or are you using PushNotificationsIOS?
– zvona
Nov 14 '18 at 22:27
I've updated to show my code. I'm using a library and the built in PushNotificationsIOS.
– S S
Nov 14 '18 at 22:40
add a comment |
the completion handler you supply torequestAuthorizationWithOptions
is called once they have responded to the dialog
– Paulw11
Nov 14 '18 at 22:08
Ok, I just saw you have tagged react-native, so the process will be slightly different but there must be an equivalent call-back when the user responds to the dialog. Edit your question to show your code
– Paulw11
Nov 14 '18 at 22:11
Can you elaborate whether you're using a 3rd party library with permissions handling (Expo, react-native-permissions etc) or are you using PushNotificationsIOS?
– zvona
Nov 14 '18 at 22:27
I've updated to show my code. I'm using a library and the built in PushNotificationsIOS.
– S S
Nov 14 '18 at 22:40
the completion handler you supply to
requestAuthorizationWithOptions
is called once they have responded to the dialog– Paulw11
Nov 14 '18 at 22:08
the completion handler you supply to
requestAuthorizationWithOptions
is called once they have responded to the dialog– Paulw11
Nov 14 '18 at 22:08
Ok, I just saw you have tagged react-native, so the process will be slightly different but there must be an equivalent call-back when the user responds to the dialog. Edit your question to show your code
– Paulw11
Nov 14 '18 at 22:11
Ok, I just saw you have tagged react-native, so the process will be slightly different but there must be an equivalent call-back when the user responds to the dialog. Edit your question to show your code
– Paulw11
Nov 14 '18 at 22:11
Can you elaborate whether you're using a 3rd party library with permissions handling (Expo, react-native-permissions etc) or are you using PushNotificationsIOS?
– zvona
Nov 14 '18 at 22:27
Can you elaborate whether you're using a 3rd party library with permissions handling (Expo, react-native-permissions etc) or are you using PushNotificationsIOS?
– zvona
Nov 14 '18 at 22:27
I've updated to show my code. I'm using a library and the built in PushNotificationsIOS.
– S S
Nov 14 '18 at 22:40
I've updated to show my code. I'm using a library and the built in PushNotificationsIOS.
– S S
Nov 14 '18 at 22:40
add a comment |
1 Answer
1
active
oldest
votes
To listen to the user's decision.
PushNotification.requestPermissions().then((response: any) => {
if (response && response.alert !== 0) {
// Allow
return;
}
// Decline
});
Credit - jose920405
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53309384%2fhow-to-tell-when-use-has-answered-ios-notification-permissions-request-in-react%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
To listen to the user's decision.
PushNotification.requestPermissions().then((response: any) => {
if (response && response.alert !== 0) {
// Allow
return;
}
// Decline
});
Credit - jose920405
add a comment |
To listen to the user's decision.
PushNotification.requestPermissions().then((response: any) => {
if (response && response.alert !== 0) {
// Allow
return;
}
// Decline
});
Credit - jose920405
add a comment |
To listen to the user's decision.
PushNotification.requestPermissions().then((response: any) => {
if (response && response.alert !== 0) {
// Allow
return;
}
// Decline
});
Credit - jose920405
To listen to the user's decision.
PushNotification.requestPermissions().then((response: any) => {
if (response && response.alert !== 0) {
// Allow
return;
}
// Decline
});
Credit - jose920405
answered Dec 3 '18 at 19:30
John DoeJohn Doe
705
705
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53309384%2fhow-to-tell-when-use-has-answered-ios-notification-permissions-request-in-react%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
QGX1UTcmU 7F17 gozoRa
the completion handler you supply to
requestAuthorizationWithOptions
is called once they have responded to the dialog– Paulw11
Nov 14 '18 at 22:08
Ok, I just saw you have tagged react-native, so the process will be slightly different but there must be an equivalent call-back when the user responds to the dialog. Edit your question to show your code
– Paulw11
Nov 14 '18 at 22:11
Can you elaborate whether you're using a 3rd party library with permissions handling (Expo, react-native-permissions etc) or are you using PushNotificationsIOS?
– zvona
Nov 14 '18 at 22:27
I've updated to show my code. I'm using a library and the built in PushNotificationsIOS.
– S S
Nov 14 '18 at 22:40