How to multicast with ipv6 udp socket in C/C++ on linux?
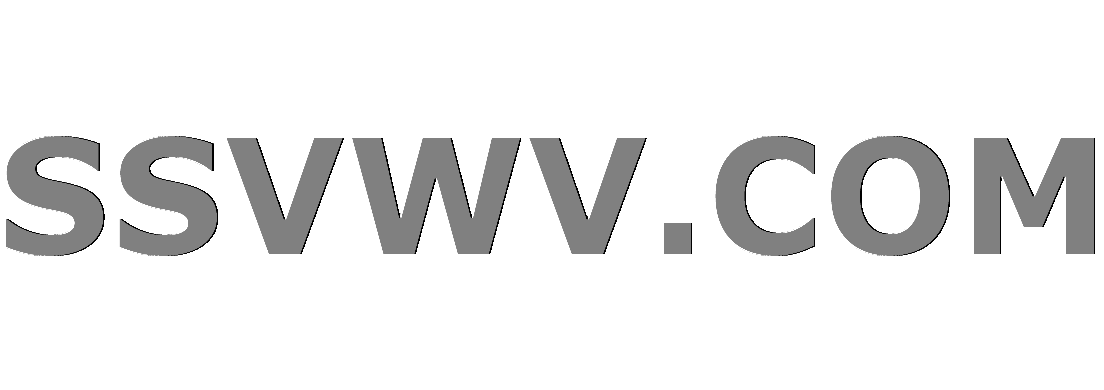
Multi tool use
(English is not my native tongue, don't worry if some sentences are strange ;) ).
I was developing a PONG game and by the way creating some classes to help me managing window, event ... and network because I added a LAN feature to the game but currently you have to enter the address of the one with who you want to play with. And a solution to that was a broadcast (scanning LAN for player). This was easy with ipv4, just use the address 255.255.255.255 but we are in 2017 and provide a feature that works only with ipv4 sucks...
Then I look for a way to broadcast with ipv6 and I learn about multi-cast but this part just get me lost. =(
I use standard libraries on Linux in C++, I found several example of multi-cast that didn't work with me. The best I have done at this time is sending a udp packet from one instance of the program to an other on the same computer.
How can I multi-cast with ipv6 udp socket on Linux in C/C++ ?
The best code found on Internet (I rearranged it) that almost work
(there is client and serv all in one, choice is made by adding 1 or 0 to argv) :
int main(int argc, char const *argv) {
struct sockaddr_in6 groupSock;
int sd = -1;
char databuf[10];
int datalen = sizeof databuf;
/* Create a datagram socket on which to send/receive. */
if((sd = socket(AF_INET6, SOCK_DGRAM, 0)) < 0) {
perror("Opening datagram socket error");
return 1;
} else {
cout << "Opening the datagram socket...OK." << endl;;
}
/* Enable SO_REUSEADDR to allow multiple instances of this */
/* application to receive copies of the multicast datagrams. */
int reuse = 1;
if(setsockopt(sd, SOL_SOCKET, SO_REUSEADDR, (char *)&reuse, sizeof reuse) < 0) {
perror("Setting SO_REUSEADDR error");
close(sd);
return 1;
} else {
cout << "Setting SO_REUSEADDR...OK." << endl;
}
/* Initialize the group sockaddr structure with a */
memset((char *) &groupSock, 0, sizeof groupSock);
groupSock.sin6_family = AF_INET6;
// address of the group
inet_pton(AF_INET6, "ff0e::/16", &groupSock.sin6_addr);
groupSock.sin6_port = htons(4321);
/* Set local interface for outbound multicast datagrams. */
/* The IP address specified must be associated with a local, */
/* multicast capable interface. */
int ifindex = if_nametoindex ("enp3s0");
cout << "ifindex is " << ifindex << endl;
if(setsockopt(sd, IPPROTO_IPV6, IPV6_MULTICAST_IF, &ifindex, sizeof ifindex)) {
perror("Setting local interface error");
return 1;
} else {
cout << "Setting the local interface...OK" << endl;
}
// choice is 0 for sending and 1 for receiving
int choice;
if (argc < 2) {
cout << "missing argv[1]" << endl;
return 1;
}
sscanf (argv[1], "%d", &choice);
// if sending
if (choice == 0) {
memset(databuf, 'a', datalen);
databuf[sizeof databuf - 1] = '';
if (sendto(sd, databuf, datalen, 0, (sockaddr*)&groupSock, sizeof groupSock) < 0) {
cout << "Error in send" << endl;
} else {
cout << "Send okay!" << endl;
}
}
// if receiving
else if (choice == 1) {
groupSock.sin6_addr = in6addr_any;
if(bind(sd, (sockaddr*)&groupSock, sizeof groupSock)) {
perror("Binding datagram socket error");
close(sd);
return 1;
} else {
cout << "Binding datagram socket...OK." << endl;
}
/* Join the multicast group ff0e::/16 on the local */
/* interface. Note that this IP_ADD_MEMBERSHIP option must be */
/* called for each local interface over which the multicast */
/* datagrams are to be received. */
struct ipv6_mreq group;
inet_pton (AF_INET6, "ff0e::", &group.ipv6mr_multiaddr.s6_addr);
group.ipv6mr_interface = ifindex;
if(setsockopt(sd, IPPROTO_IPV6, IPV6_ADD_MEMBERSHIP, (char *)&group, sizeof group) < 0) {
perror("Adding multicast group error");
close(sd);
return 1;
} else {
cout << "Adding multicast group...OK." << endl;
}
if (read(sd, databuf, datalen) < 0) {
perror("Error in read");
} else {
databuf[sizeof databuf - 1] = '';// just for safety
cout << "Read Okay" << endl;
cout << "Message is : " << databuf << endl;
}
}
return 0;
}
Here the address is ff0e:: but I have try with ff01:: and ff02::.
I need help, I have not found any simple documentation about that. Thanks in advance for any answer.
Edit :
Thanks Ron Maupin and Jeremy Friesner for these comments, it helps me.
Edit :
THANKS Jeremy ! Your advice to use ff12::blah:blah(...) instead of ff0e:: works ! Should I write answer to my question to close the thread ?
c++ linux sockets ipv6 multicast
add a comment |
(English is not my native tongue, don't worry if some sentences are strange ;) ).
I was developing a PONG game and by the way creating some classes to help me managing window, event ... and network because I added a LAN feature to the game but currently you have to enter the address of the one with who you want to play with. And a solution to that was a broadcast (scanning LAN for player). This was easy with ipv4, just use the address 255.255.255.255 but we are in 2017 and provide a feature that works only with ipv4 sucks...
Then I look for a way to broadcast with ipv6 and I learn about multi-cast but this part just get me lost. =(
I use standard libraries on Linux in C++, I found several example of multi-cast that didn't work with me. The best I have done at this time is sending a udp packet from one instance of the program to an other on the same computer.
How can I multi-cast with ipv6 udp socket on Linux in C/C++ ?
The best code found on Internet (I rearranged it) that almost work
(there is client and serv all in one, choice is made by adding 1 or 0 to argv) :
int main(int argc, char const *argv) {
struct sockaddr_in6 groupSock;
int sd = -1;
char databuf[10];
int datalen = sizeof databuf;
/* Create a datagram socket on which to send/receive. */
if((sd = socket(AF_INET6, SOCK_DGRAM, 0)) < 0) {
perror("Opening datagram socket error");
return 1;
} else {
cout << "Opening the datagram socket...OK." << endl;;
}
/* Enable SO_REUSEADDR to allow multiple instances of this */
/* application to receive copies of the multicast datagrams. */
int reuse = 1;
if(setsockopt(sd, SOL_SOCKET, SO_REUSEADDR, (char *)&reuse, sizeof reuse) < 0) {
perror("Setting SO_REUSEADDR error");
close(sd);
return 1;
} else {
cout << "Setting SO_REUSEADDR...OK." << endl;
}
/* Initialize the group sockaddr structure with a */
memset((char *) &groupSock, 0, sizeof groupSock);
groupSock.sin6_family = AF_INET6;
// address of the group
inet_pton(AF_INET6, "ff0e::/16", &groupSock.sin6_addr);
groupSock.sin6_port = htons(4321);
/* Set local interface for outbound multicast datagrams. */
/* The IP address specified must be associated with a local, */
/* multicast capable interface. */
int ifindex = if_nametoindex ("enp3s0");
cout << "ifindex is " << ifindex << endl;
if(setsockopt(sd, IPPROTO_IPV6, IPV6_MULTICAST_IF, &ifindex, sizeof ifindex)) {
perror("Setting local interface error");
return 1;
} else {
cout << "Setting the local interface...OK" << endl;
}
// choice is 0 for sending and 1 for receiving
int choice;
if (argc < 2) {
cout << "missing argv[1]" << endl;
return 1;
}
sscanf (argv[1], "%d", &choice);
// if sending
if (choice == 0) {
memset(databuf, 'a', datalen);
databuf[sizeof databuf - 1] = '';
if (sendto(sd, databuf, datalen, 0, (sockaddr*)&groupSock, sizeof groupSock) < 0) {
cout << "Error in send" << endl;
} else {
cout << "Send okay!" << endl;
}
}
// if receiving
else if (choice == 1) {
groupSock.sin6_addr = in6addr_any;
if(bind(sd, (sockaddr*)&groupSock, sizeof groupSock)) {
perror("Binding datagram socket error");
close(sd);
return 1;
} else {
cout << "Binding datagram socket...OK." << endl;
}
/* Join the multicast group ff0e::/16 on the local */
/* interface. Note that this IP_ADD_MEMBERSHIP option must be */
/* called for each local interface over which the multicast */
/* datagrams are to be received. */
struct ipv6_mreq group;
inet_pton (AF_INET6, "ff0e::", &group.ipv6mr_multiaddr.s6_addr);
group.ipv6mr_interface = ifindex;
if(setsockopt(sd, IPPROTO_IPV6, IPV6_ADD_MEMBERSHIP, (char *)&group, sizeof group) < 0) {
perror("Adding multicast group error");
close(sd);
return 1;
} else {
cout << "Adding multicast group...OK." << endl;
}
if (read(sd, databuf, datalen) < 0) {
perror("Error in read");
} else {
databuf[sizeof databuf - 1] = '';// just for safety
cout << "Read Okay" << endl;
cout << "Message is : " << databuf << endl;
}
}
return 0;
}
Here the address is ff0e:: but I have try with ff01:: and ff02::.
I need help, I have not found any simple documentation about that. Thanks in advance for any answer.
Edit :
Thanks Ron Maupin and Jeremy Friesner for these comments, it helps me.
Edit :
THANKS Jeremy ! Your advice to use ff12::blah:blah(...) instead of ff0e:: works ! Should I write answer to my question to close the thread ?
c++ linux sockets ipv6 multicast
2
Even with IPv4, this is a misuse of broadcast, and it is poor network programming. Broadcast should only be used when you need to interrupt every host on a LAN because that is what it does. IPv6 eliminated broadcast because it is often misused. In most cases, you only want to interrupt a subset of hosts on a LAN, and that is multicast. You need to pay attention to the flags and scopes, and pick an unused multicast address. Do not use the All Nodes address unless you really do need to interrupt all the hosts on the LAN.
– Ron Maupin
Jul 24 '17 at 16:24
2
In my experience with IPv6-multicast-over-LAN, a multicast address of the form ff12::blah:blah:blah (pick your own unique values for the blah's) works best. Be aware that the multicast address is interface-specific, so you'll need to specify an interface-index, and if you want your program's multicast to work over all network interfaces, you'll need to join multiple multicast groups (one per network interface; the multicast address can be the same for all interface indices but you need to join each network interface separately and send to each network interface separately)
– Jeremy Friesner
Jul 24 '17 at 17:04
add a comment |
(English is not my native tongue, don't worry if some sentences are strange ;) ).
I was developing a PONG game and by the way creating some classes to help me managing window, event ... and network because I added a LAN feature to the game but currently you have to enter the address of the one with who you want to play with. And a solution to that was a broadcast (scanning LAN for player). This was easy with ipv4, just use the address 255.255.255.255 but we are in 2017 and provide a feature that works only with ipv4 sucks...
Then I look for a way to broadcast with ipv6 and I learn about multi-cast but this part just get me lost. =(
I use standard libraries on Linux in C++, I found several example of multi-cast that didn't work with me. The best I have done at this time is sending a udp packet from one instance of the program to an other on the same computer.
How can I multi-cast with ipv6 udp socket on Linux in C/C++ ?
The best code found on Internet (I rearranged it) that almost work
(there is client and serv all in one, choice is made by adding 1 or 0 to argv) :
int main(int argc, char const *argv) {
struct sockaddr_in6 groupSock;
int sd = -1;
char databuf[10];
int datalen = sizeof databuf;
/* Create a datagram socket on which to send/receive. */
if((sd = socket(AF_INET6, SOCK_DGRAM, 0)) < 0) {
perror("Opening datagram socket error");
return 1;
} else {
cout << "Opening the datagram socket...OK." << endl;;
}
/* Enable SO_REUSEADDR to allow multiple instances of this */
/* application to receive copies of the multicast datagrams. */
int reuse = 1;
if(setsockopt(sd, SOL_SOCKET, SO_REUSEADDR, (char *)&reuse, sizeof reuse) < 0) {
perror("Setting SO_REUSEADDR error");
close(sd);
return 1;
} else {
cout << "Setting SO_REUSEADDR...OK." << endl;
}
/* Initialize the group sockaddr structure with a */
memset((char *) &groupSock, 0, sizeof groupSock);
groupSock.sin6_family = AF_INET6;
// address of the group
inet_pton(AF_INET6, "ff0e::/16", &groupSock.sin6_addr);
groupSock.sin6_port = htons(4321);
/* Set local interface for outbound multicast datagrams. */
/* The IP address specified must be associated with a local, */
/* multicast capable interface. */
int ifindex = if_nametoindex ("enp3s0");
cout << "ifindex is " << ifindex << endl;
if(setsockopt(sd, IPPROTO_IPV6, IPV6_MULTICAST_IF, &ifindex, sizeof ifindex)) {
perror("Setting local interface error");
return 1;
} else {
cout << "Setting the local interface...OK" << endl;
}
// choice is 0 for sending and 1 for receiving
int choice;
if (argc < 2) {
cout << "missing argv[1]" << endl;
return 1;
}
sscanf (argv[1], "%d", &choice);
// if sending
if (choice == 0) {
memset(databuf, 'a', datalen);
databuf[sizeof databuf - 1] = '';
if (sendto(sd, databuf, datalen, 0, (sockaddr*)&groupSock, sizeof groupSock) < 0) {
cout << "Error in send" << endl;
} else {
cout << "Send okay!" << endl;
}
}
// if receiving
else if (choice == 1) {
groupSock.sin6_addr = in6addr_any;
if(bind(sd, (sockaddr*)&groupSock, sizeof groupSock)) {
perror("Binding datagram socket error");
close(sd);
return 1;
} else {
cout << "Binding datagram socket...OK." << endl;
}
/* Join the multicast group ff0e::/16 on the local */
/* interface. Note that this IP_ADD_MEMBERSHIP option must be */
/* called for each local interface over which the multicast */
/* datagrams are to be received. */
struct ipv6_mreq group;
inet_pton (AF_INET6, "ff0e::", &group.ipv6mr_multiaddr.s6_addr);
group.ipv6mr_interface = ifindex;
if(setsockopt(sd, IPPROTO_IPV6, IPV6_ADD_MEMBERSHIP, (char *)&group, sizeof group) < 0) {
perror("Adding multicast group error");
close(sd);
return 1;
} else {
cout << "Adding multicast group...OK." << endl;
}
if (read(sd, databuf, datalen) < 0) {
perror("Error in read");
} else {
databuf[sizeof databuf - 1] = '';// just for safety
cout << "Read Okay" << endl;
cout << "Message is : " << databuf << endl;
}
}
return 0;
}
Here the address is ff0e:: but I have try with ff01:: and ff02::.
I need help, I have not found any simple documentation about that. Thanks in advance for any answer.
Edit :
Thanks Ron Maupin and Jeremy Friesner for these comments, it helps me.
Edit :
THANKS Jeremy ! Your advice to use ff12::blah:blah(...) instead of ff0e:: works ! Should I write answer to my question to close the thread ?
c++ linux sockets ipv6 multicast
(English is not my native tongue, don't worry if some sentences are strange ;) ).
I was developing a PONG game and by the way creating some classes to help me managing window, event ... and network because I added a LAN feature to the game but currently you have to enter the address of the one with who you want to play with. And a solution to that was a broadcast (scanning LAN for player). This was easy with ipv4, just use the address 255.255.255.255 but we are in 2017 and provide a feature that works only with ipv4 sucks...
Then I look for a way to broadcast with ipv6 and I learn about multi-cast but this part just get me lost. =(
I use standard libraries on Linux in C++, I found several example of multi-cast that didn't work with me. The best I have done at this time is sending a udp packet from one instance of the program to an other on the same computer.
How can I multi-cast with ipv6 udp socket on Linux in C/C++ ?
The best code found on Internet (I rearranged it) that almost work
(there is client and serv all in one, choice is made by adding 1 or 0 to argv) :
int main(int argc, char const *argv) {
struct sockaddr_in6 groupSock;
int sd = -1;
char databuf[10];
int datalen = sizeof databuf;
/* Create a datagram socket on which to send/receive. */
if((sd = socket(AF_INET6, SOCK_DGRAM, 0)) < 0) {
perror("Opening datagram socket error");
return 1;
} else {
cout << "Opening the datagram socket...OK." << endl;;
}
/* Enable SO_REUSEADDR to allow multiple instances of this */
/* application to receive copies of the multicast datagrams. */
int reuse = 1;
if(setsockopt(sd, SOL_SOCKET, SO_REUSEADDR, (char *)&reuse, sizeof reuse) < 0) {
perror("Setting SO_REUSEADDR error");
close(sd);
return 1;
} else {
cout << "Setting SO_REUSEADDR...OK." << endl;
}
/* Initialize the group sockaddr structure with a */
memset((char *) &groupSock, 0, sizeof groupSock);
groupSock.sin6_family = AF_INET6;
// address of the group
inet_pton(AF_INET6, "ff0e::/16", &groupSock.sin6_addr);
groupSock.sin6_port = htons(4321);
/* Set local interface for outbound multicast datagrams. */
/* The IP address specified must be associated with a local, */
/* multicast capable interface. */
int ifindex = if_nametoindex ("enp3s0");
cout << "ifindex is " << ifindex << endl;
if(setsockopt(sd, IPPROTO_IPV6, IPV6_MULTICAST_IF, &ifindex, sizeof ifindex)) {
perror("Setting local interface error");
return 1;
} else {
cout << "Setting the local interface...OK" << endl;
}
// choice is 0 for sending and 1 for receiving
int choice;
if (argc < 2) {
cout << "missing argv[1]" << endl;
return 1;
}
sscanf (argv[1], "%d", &choice);
// if sending
if (choice == 0) {
memset(databuf, 'a', datalen);
databuf[sizeof databuf - 1] = '';
if (sendto(sd, databuf, datalen, 0, (sockaddr*)&groupSock, sizeof groupSock) < 0) {
cout << "Error in send" << endl;
} else {
cout << "Send okay!" << endl;
}
}
// if receiving
else if (choice == 1) {
groupSock.sin6_addr = in6addr_any;
if(bind(sd, (sockaddr*)&groupSock, sizeof groupSock)) {
perror("Binding datagram socket error");
close(sd);
return 1;
} else {
cout << "Binding datagram socket...OK." << endl;
}
/* Join the multicast group ff0e::/16 on the local */
/* interface. Note that this IP_ADD_MEMBERSHIP option must be */
/* called for each local interface over which the multicast */
/* datagrams are to be received. */
struct ipv6_mreq group;
inet_pton (AF_INET6, "ff0e::", &group.ipv6mr_multiaddr.s6_addr);
group.ipv6mr_interface = ifindex;
if(setsockopt(sd, IPPROTO_IPV6, IPV6_ADD_MEMBERSHIP, (char *)&group, sizeof group) < 0) {
perror("Adding multicast group error");
close(sd);
return 1;
} else {
cout << "Adding multicast group...OK." << endl;
}
if (read(sd, databuf, datalen) < 0) {
perror("Error in read");
} else {
databuf[sizeof databuf - 1] = '';// just for safety
cout << "Read Okay" << endl;
cout << "Message is : " << databuf << endl;
}
}
return 0;
}
Here the address is ff0e:: but I have try with ff01:: and ff02::.
I need help, I have not found any simple documentation about that. Thanks in advance for any answer.
Edit :
Thanks Ron Maupin and Jeremy Friesner for these comments, it helps me.
Edit :
THANKS Jeremy ! Your advice to use ff12::blah:blah(...) instead of ff0e:: works ! Should I write answer to my question to close the thread ?
c++ linux sockets ipv6 multicast
c++ linux sockets ipv6 multicast
edited Jul 24 '17 at 20:00
uben
asked Jul 24 '17 at 16:14


ubenuben
4218
4218
2
Even with IPv4, this is a misuse of broadcast, and it is poor network programming. Broadcast should only be used when you need to interrupt every host on a LAN because that is what it does. IPv6 eliminated broadcast because it is often misused. In most cases, you only want to interrupt a subset of hosts on a LAN, and that is multicast. You need to pay attention to the flags and scopes, and pick an unused multicast address. Do not use the All Nodes address unless you really do need to interrupt all the hosts on the LAN.
– Ron Maupin
Jul 24 '17 at 16:24
2
In my experience with IPv6-multicast-over-LAN, a multicast address of the form ff12::blah:blah:blah (pick your own unique values for the blah's) works best. Be aware that the multicast address is interface-specific, so you'll need to specify an interface-index, and if you want your program's multicast to work over all network interfaces, you'll need to join multiple multicast groups (one per network interface; the multicast address can be the same for all interface indices but you need to join each network interface separately and send to each network interface separately)
– Jeremy Friesner
Jul 24 '17 at 17:04
add a comment |
2
Even with IPv4, this is a misuse of broadcast, and it is poor network programming. Broadcast should only be used when you need to interrupt every host on a LAN because that is what it does. IPv6 eliminated broadcast because it is often misused. In most cases, you only want to interrupt a subset of hosts on a LAN, and that is multicast. You need to pay attention to the flags and scopes, and pick an unused multicast address. Do not use the All Nodes address unless you really do need to interrupt all the hosts on the LAN.
– Ron Maupin
Jul 24 '17 at 16:24
2
In my experience with IPv6-multicast-over-LAN, a multicast address of the form ff12::blah:blah:blah (pick your own unique values for the blah's) works best. Be aware that the multicast address is interface-specific, so you'll need to specify an interface-index, and if you want your program's multicast to work over all network interfaces, you'll need to join multiple multicast groups (one per network interface; the multicast address can be the same for all interface indices but you need to join each network interface separately and send to each network interface separately)
– Jeremy Friesner
Jul 24 '17 at 17:04
2
2
Even with IPv4, this is a misuse of broadcast, and it is poor network programming. Broadcast should only be used when you need to interrupt every host on a LAN because that is what it does. IPv6 eliminated broadcast because it is often misused. In most cases, you only want to interrupt a subset of hosts on a LAN, and that is multicast. You need to pay attention to the flags and scopes, and pick an unused multicast address. Do not use the All Nodes address unless you really do need to interrupt all the hosts on the LAN.
– Ron Maupin
Jul 24 '17 at 16:24
Even with IPv4, this is a misuse of broadcast, and it is poor network programming. Broadcast should only be used when you need to interrupt every host on a LAN because that is what it does. IPv6 eliminated broadcast because it is often misused. In most cases, you only want to interrupt a subset of hosts on a LAN, and that is multicast. You need to pay attention to the flags and scopes, and pick an unused multicast address. Do not use the All Nodes address unless you really do need to interrupt all the hosts on the LAN.
– Ron Maupin
Jul 24 '17 at 16:24
2
2
In my experience with IPv6-multicast-over-LAN, a multicast address of the form ff12::blah:blah:blah (pick your own unique values for the blah's) works best. Be aware that the multicast address is interface-specific, so you'll need to specify an interface-index, and if you want your program's multicast to work over all network interfaces, you'll need to join multiple multicast groups (one per network interface; the multicast address can be the same for all interface indices but you need to join each network interface separately and send to each network interface separately)
– Jeremy Friesner
Jul 24 '17 at 17:04
In my experience with IPv6-multicast-over-LAN, a multicast address of the form ff12::blah:blah:blah (pick your own unique values for the blah's) works best. Be aware that the multicast address is interface-specific, so you'll need to specify an interface-index, and if you want your program's multicast to work over all network interfaces, you'll need to join multiple multicast groups (one per network interface; the multicast address can be the same for all interface indices but you need to join each network interface separately and send to each network interface separately)
– Jeremy Friesner
Jul 24 '17 at 17:04
add a comment |
1 Answer
1
active
oldest
votes
This code below is right:
The only thing wrong is the address used for the multicast.
Like Jeremy said it, ff0e:: is not correct, I used instead ff12::feed:a:dead:beef and it works.
It is possible to get the name and index of the available interface by using if_nameindex().
Update : I try to remove some code to see if it work without it and I manage to get this :
server :
// OPEN
int fd = socket(AF_INET6, SOCK_DGRAM, 0);
// BIND
struct sockaddr_in6 address = {AF_INET6, htons(4321)};
bind(fd, (struct sockaddr*)&address, sizeof address);
// JOIN MEMBERSHIP
struct ipv6_mreq group;
group.ipv6mr_interface = 0;
inet_pton(AF_INET6, "ff12::1234", &group.ipv6mr_multiaddr);
setsockopt(fd, IPPROTO_IPV6, IPV6_ADD_MEMBERSHIP, &group, sizeof group);
// READ
char buffer[128];
read(fd, buffer, sizeof buffer);
client :
// OPEN
int fd = socket(AF_INET6, SOCK_DGRAM, 0);
// ADDRESS
struct sockaddr_in6 address = {AF_INET6, htons(4321)};
inet_pton(AF_INET6, "ff12::1234", &address.sin6_addr);
// SEND TO
char buffer[128];
strcpy(buffer, "hello world!");
sendto(fd, buffer, sizeof buffer, 0, (struct sockaddr*)&address, sizeof address);
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f45285419%2fhow-to-multicast-with-ipv6-udp-socket-in-c-c-on-linux%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
This code below is right:
The only thing wrong is the address used for the multicast.
Like Jeremy said it, ff0e:: is not correct, I used instead ff12::feed:a:dead:beef and it works.
It is possible to get the name and index of the available interface by using if_nameindex().
Update : I try to remove some code to see if it work without it and I manage to get this :
server :
// OPEN
int fd = socket(AF_INET6, SOCK_DGRAM, 0);
// BIND
struct sockaddr_in6 address = {AF_INET6, htons(4321)};
bind(fd, (struct sockaddr*)&address, sizeof address);
// JOIN MEMBERSHIP
struct ipv6_mreq group;
group.ipv6mr_interface = 0;
inet_pton(AF_INET6, "ff12::1234", &group.ipv6mr_multiaddr);
setsockopt(fd, IPPROTO_IPV6, IPV6_ADD_MEMBERSHIP, &group, sizeof group);
// READ
char buffer[128];
read(fd, buffer, sizeof buffer);
client :
// OPEN
int fd = socket(AF_INET6, SOCK_DGRAM, 0);
// ADDRESS
struct sockaddr_in6 address = {AF_INET6, htons(4321)};
inet_pton(AF_INET6, "ff12::1234", &address.sin6_addr);
// SEND TO
char buffer[128];
strcpy(buffer, "hello world!");
sendto(fd, buffer, sizeof buffer, 0, (struct sockaddr*)&address, sizeof address);
add a comment |
This code below is right:
The only thing wrong is the address used for the multicast.
Like Jeremy said it, ff0e:: is not correct, I used instead ff12::feed:a:dead:beef and it works.
It is possible to get the name and index of the available interface by using if_nameindex().
Update : I try to remove some code to see if it work without it and I manage to get this :
server :
// OPEN
int fd = socket(AF_INET6, SOCK_DGRAM, 0);
// BIND
struct sockaddr_in6 address = {AF_INET6, htons(4321)};
bind(fd, (struct sockaddr*)&address, sizeof address);
// JOIN MEMBERSHIP
struct ipv6_mreq group;
group.ipv6mr_interface = 0;
inet_pton(AF_INET6, "ff12::1234", &group.ipv6mr_multiaddr);
setsockopt(fd, IPPROTO_IPV6, IPV6_ADD_MEMBERSHIP, &group, sizeof group);
// READ
char buffer[128];
read(fd, buffer, sizeof buffer);
client :
// OPEN
int fd = socket(AF_INET6, SOCK_DGRAM, 0);
// ADDRESS
struct sockaddr_in6 address = {AF_INET6, htons(4321)};
inet_pton(AF_INET6, "ff12::1234", &address.sin6_addr);
// SEND TO
char buffer[128];
strcpy(buffer, "hello world!");
sendto(fd, buffer, sizeof buffer, 0, (struct sockaddr*)&address, sizeof address);
add a comment |
This code below is right:
The only thing wrong is the address used for the multicast.
Like Jeremy said it, ff0e:: is not correct, I used instead ff12::feed:a:dead:beef and it works.
It is possible to get the name and index of the available interface by using if_nameindex().
Update : I try to remove some code to see if it work without it and I manage to get this :
server :
// OPEN
int fd = socket(AF_INET6, SOCK_DGRAM, 0);
// BIND
struct sockaddr_in6 address = {AF_INET6, htons(4321)};
bind(fd, (struct sockaddr*)&address, sizeof address);
// JOIN MEMBERSHIP
struct ipv6_mreq group;
group.ipv6mr_interface = 0;
inet_pton(AF_INET6, "ff12::1234", &group.ipv6mr_multiaddr);
setsockopt(fd, IPPROTO_IPV6, IPV6_ADD_MEMBERSHIP, &group, sizeof group);
// READ
char buffer[128];
read(fd, buffer, sizeof buffer);
client :
// OPEN
int fd = socket(AF_INET6, SOCK_DGRAM, 0);
// ADDRESS
struct sockaddr_in6 address = {AF_INET6, htons(4321)};
inet_pton(AF_INET6, "ff12::1234", &address.sin6_addr);
// SEND TO
char buffer[128];
strcpy(buffer, "hello world!");
sendto(fd, buffer, sizeof buffer, 0, (struct sockaddr*)&address, sizeof address);
This code below is right:
The only thing wrong is the address used for the multicast.
Like Jeremy said it, ff0e:: is not correct, I used instead ff12::feed:a:dead:beef and it works.
It is possible to get the name and index of the available interface by using if_nameindex().
Update : I try to remove some code to see if it work without it and I manage to get this :
server :
// OPEN
int fd = socket(AF_INET6, SOCK_DGRAM, 0);
// BIND
struct sockaddr_in6 address = {AF_INET6, htons(4321)};
bind(fd, (struct sockaddr*)&address, sizeof address);
// JOIN MEMBERSHIP
struct ipv6_mreq group;
group.ipv6mr_interface = 0;
inet_pton(AF_INET6, "ff12::1234", &group.ipv6mr_multiaddr);
setsockopt(fd, IPPROTO_IPV6, IPV6_ADD_MEMBERSHIP, &group, sizeof group);
// READ
char buffer[128];
read(fd, buffer, sizeof buffer);
client :
// OPEN
int fd = socket(AF_INET6, SOCK_DGRAM, 0);
// ADDRESS
struct sockaddr_in6 address = {AF_INET6, htons(4321)};
inet_pton(AF_INET6, "ff12::1234", &address.sin6_addr);
// SEND TO
char buffer[128];
strcpy(buffer, "hello world!");
sendto(fd, buffer, sizeof buffer, 0, (struct sockaddr*)&address, sizeof address);
edited Jul 29 '17 at 14:06
answered Jul 25 '17 at 7:45


ubenuben
4218
4218
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f45285419%2fhow-to-multicast-with-ipv6-udp-socket-in-c-c-on-linux%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
dCVbLPtgOl7uSwGx6s3oyTACXzPoSvXLTB6,06Vr43qXGrCEWArgCuq,Febix7vgnN1 NS2jfMY2Eu
2
Even with IPv4, this is a misuse of broadcast, and it is poor network programming. Broadcast should only be used when you need to interrupt every host on a LAN because that is what it does. IPv6 eliminated broadcast because it is often misused. In most cases, you only want to interrupt a subset of hosts on a LAN, and that is multicast. You need to pay attention to the flags and scopes, and pick an unused multicast address. Do not use the All Nodes address unless you really do need to interrupt all the hosts on the LAN.
– Ron Maupin
Jul 24 '17 at 16:24
2
In my experience with IPv6-multicast-over-LAN, a multicast address of the form ff12::blah:blah:blah (pick your own unique values for the blah's) works best. Be aware that the multicast address is interface-specific, so you'll need to specify an interface-index, and if you want your program's multicast to work over all network interfaces, you'll need to join multiple multicast groups (one per network interface; the multicast address can be the same for all interface indices but you need to join each network interface separately and send to each network interface separately)
– Jeremy Friesner
Jul 24 '17 at 17:04