Get Users by Age Using User Birthdate Column From Table
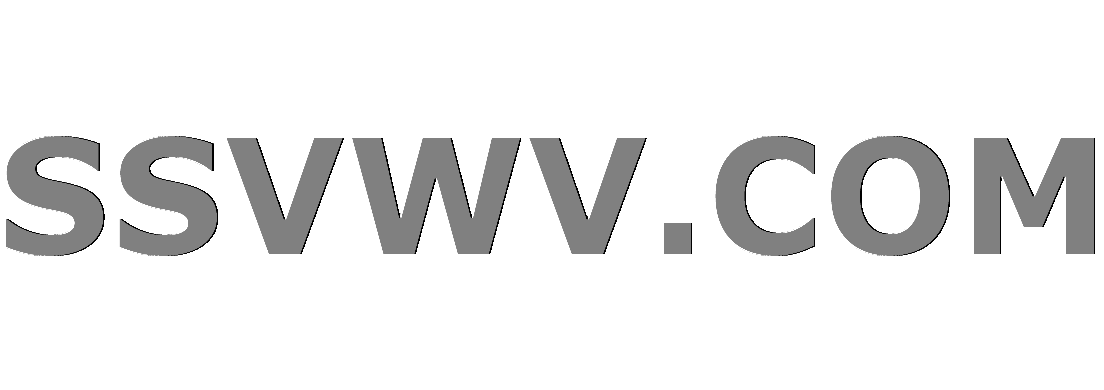
Multi tool use
Brief:
I have user birthdate
column in my users
table. User birthdate has a default date format: Y-m-d
. Also, I have custom mutator to get user age in User
model.
Mutator code:
public function getAgeAttribute($value) {
if($this->birthday !== null) {
$bday = new DateTime("{$this->birthday}");
$today = new Datetime(date('Y-m-d'));
$diff = $today->diff($bday);
return $diff->y;
}
return null;
}
Question:
How I can get users by age using User
model?
laravel eloquent laravel-5.7
add a comment |
Brief:
I have user birthdate
column in my users
table. User birthdate has a default date format: Y-m-d
. Also, I have custom mutator to get user age in User
model.
Mutator code:
public function getAgeAttribute($value) {
if($this->birthday !== null) {
$bday = new DateTime("{$this->birthday}");
$today = new Datetime(date('Y-m-d'));
$diff = $today->diff($bday);
return $diff->y;
}
return null;
}
Question:
How I can get users by age using User
model?
laravel eloquent laravel-5.7
2
what do you meanby age
? you mean that you want to fetch all users greater than 20 years?
– hassan
Oct 29 '18 at 8:11
Yes of course @hassan
– Andreas Hunter
Oct 29 '18 at 8:12
2
what is the column type? datetime/date?
– hassan
Oct 29 '18 at 8:13
1
Column type string. If you think will change the type of field to date from string, I will change it. @hassan
– Andreas Hunter
Oct 29 '18 at 8:16
1
you might want to use a local scope for that.
– duckduckduck
Oct 29 '18 at 9:08
add a comment |
Brief:
I have user birthdate
column in my users
table. User birthdate has a default date format: Y-m-d
. Also, I have custom mutator to get user age in User
model.
Mutator code:
public function getAgeAttribute($value) {
if($this->birthday !== null) {
$bday = new DateTime("{$this->birthday}");
$today = new Datetime(date('Y-m-d'));
$diff = $today->diff($bday);
return $diff->y;
}
return null;
}
Question:
How I can get users by age using User
model?
laravel eloquent laravel-5.7
Brief:
I have user birthdate
column in my users
table. User birthdate has a default date format: Y-m-d
. Also, I have custom mutator to get user age in User
model.
Mutator code:
public function getAgeAttribute($value) {
if($this->birthday !== null) {
$bday = new DateTime("{$this->birthday}");
$today = new Datetime(date('Y-m-d'));
$diff = $today->diff($bday);
return $diff->y;
}
return null;
}
Question:
How I can get users by age using User
model?
laravel eloquent laravel-5.7
laravel eloquent laravel-5.7
edited Nov 14 '18 at 21:56


Karl Hill
2,93412142
2,93412142
asked Oct 29 '18 at 7:59
Andreas HunterAndreas Hunter
745518
745518
2
what do you meanby age
? you mean that you want to fetch all users greater than 20 years?
– hassan
Oct 29 '18 at 8:11
Yes of course @hassan
– Andreas Hunter
Oct 29 '18 at 8:12
2
what is the column type? datetime/date?
– hassan
Oct 29 '18 at 8:13
1
Column type string. If you think will change the type of field to date from string, I will change it. @hassan
– Andreas Hunter
Oct 29 '18 at 8:16
1
you might want to use a local scope for that.
– duckduckduck
Oct 29 '18 at 9:08
add a comment |
2
what do you meanby age
? you mean that you want to fetch all users greater than 20 years?
– hassan
Oct 29 '18 at 8:11
Yes of course @hassan
– Andreas Hunter
Oct 29 '18 at 8:12
2
what is the column type? datetime/date?
– hassan
Oct 29 '18 at 8:13
1
Column type string. If you think will change the type of field to date from string, I will change it. @hassan
– Andreas Hunter
Oct 29 '18 at 8:16
1
you might want to use a local scope for that.
– duckduckduck
Oct 29 '18 at 9:08
2
2
what do you mean
by age
? you mean that you want to fetch all users greater than 20 years?– hassan
Oct 29 '18 at 8:11
what do you mean
by age
? you mean that you want to fetch all users greater than 20 years?– hassan
Oct 29 '18 at 8:11
Yes of course @hassan
– Andreas Hunter
Oct 29 '18 at 8:12
Yes of course @hassan
– Andreas Hunter
Oct 29 '18 at 8:12
2
2
what is the column type? datetime/date?
– hassan
Oct 29 '18 at 8:13
what is the column type? datetime/date?
– hassan
Oct 29 '18 at 8:13
1
1
Column type string. If you think will change the type of field to date from string, I will change it. @hassan
– Andreas Hunter
Oct 29 '18 at 8:16
Column type string. If you think will change the type of field to date from string, I will change it. @hassan
– Andreas Hunter
Oct 29 '18 at 8:16
1
1
you might want to use a local scope for that.
– duckduckduck
Oct 29 '18 at 9:08
you might want to use a local scope for that.
– duckduckduck
Oct 29 '18 at 9:08
add a comment |
4 Answers
4
active
oldest
votes
Just take today's date and substract the number of years. then compare it to birthdays
Users::where('birthday', '<=', date('Y-m-d', strtotime('-20 years')))->get();
adding to this answer, this logic can be enclosed on a local scope defined in the User model.
– duckduckduck
Oct 29 '18 at 9:17
add a comment |
If you're using MySQL there's also this alternative:
$users = User::whereDate('birthdate' , '<', DB::raw("CURDATE()-INTERVAL 20 YEAR"))->get();
add a comment |
You can use Collection
's groupBy
method to group the users based on their age.
$users = User::all()->groupBy(function($user) {
return $user->age;
});
Then, you can get all users for a particular user like this.
$users->get(20);
Hope this is what you are looking for.
add a comment |
i hope this will help you, date()
function is php function which converts a string to datetime.
$users = User::where('birthdate' , '<', date('Y-m-d', strtotime('your input field name')))->get();
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53041087%2fget-users-by-age-using-user-birthdate-column-from-table%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
Just take today's date and substract the number of years. then compare it to birthdays
Users::where('birthday', '<=', date('Y-m-d', strtotime('-20 years')))->get();
adding to this answer, this logic can be enclosed on a local scope defined in the User model.
– duckduckduck
Oct 29 '18 at 9:17
add a comment |
Just take today's date and substract the number of years. then compare it to birthdays
Users::where('birthday', '<=', date('Y-m-d', strtotime('-20 years')))->get();
adding to this answer, this logic can be enclosed on a local scope defined in the User model.
– duckduckduck
Oct 29 '18 at 9:17
add a comment |
Just take today's date and substract the number of years. then compare it to birthdays
Users::where('birthday', '<=', date('Y-m-d', strtotime('-20 years')))->get();
Just take today's date and substract the number of years. then compare it to birthdays
Users::where('birthday', '<=', date('Y-m-d', strtotime('-20 years')))->get();
answered Oct 29 '18 at 8:18


N69SN69S
1,107514
1,107514
adding to this answer, this logic can be enclosed on a local scope defined in the User model.
– duckduckduck
Oct 29 '18 at 9:17
add a comment |
adding to this answer, this logic can be enclosed on a local scope defined in the User model.
– duckduckduck
Oct 29 '18 at 9:17
adding to this answer, this logic can be enclosed on a local scope defined in the User model.
– duckduckduck
Oct 29 '18 at 9:17
adding to this answer, this logic can be enclosed on a local scope defined in the User model.
– duckduckduck
Oct 29 '18 at 9:17
add a comment |
If you're using MySQL there's also this alternative:
$users = User::whereDate('birthdate' , '<', DB::raw("CURDATE()-INTERVAL 20 YEAR"))->get();
add a comment |
If you're using MySQL there's also this alternative:
$users = User::whereDate('birthdate' , '<', DB::raw("CURDATE()-INTERVAL 20 YEAR"))->get();
add a comment |
If you're using MySQL there's also this alternative:
$users = User::whereDate('birthdate' , '<', DB::raw("CURDATE()-INTERVAL 20 YEAR"))->get();
If you're using MySQL there's also this alternative:
$users = User::whereDate('birthdate' , '<', DB::raw("CURDATE()-INTERVAL 20 YEAR"))->get();
answered Oct 29 '18 at 8:46


apokryfosapokryfos
19k43159
19k43159
add a comment |
add a comment |
You can use Collection
's groupBy
method to group the users based on their age.
$users = User::all()->groupBy(function($user) {
return $user->age;
});
Then, you can get all users for a particular user like this.
$users->get(20);
Hope this is what you are looking for.
add a comment |
You can use Collection
's groupBy
method to group the users based on their age.
$users = User::all()->groupBy(function($user) {
return $user->age;
});
Then, you can get all users for a particular user like this.
$users->get(20);
Hope this is what you are looking for.
add a comment |
You can use Collection
's groupBy
method to group the users based on their age.
$users = User::all()->groupBy(function($user) {
return $user->age;
});
Then, you can get all users for a particular user like this.
$users->get(20);
Hope this is what you are looking for.
You can use Collection
's groupBy
method to group the users based on their age.
$users = User::all()->groupBy(function($user) {
return $user->age;
});
Then, you can get all users for a particular user like this.
$users->get(20);
Hope this is what you are looking for.
answered Oct 29 '18 at 8:24
Nabin PaudyalNabin Paudyal
666212
666212
add a comment |
add a comment |
i hope this will help you, date()
function is php function which converts a string to datetime.
$users = User::where('birthdate' , '<', date('Y-m-d', strtotime('your input field name')))->get();
add a comment |
i hope this will help you, date()
function is php function which converts a string to datetime.
$users = User::where('birthdate' , '<', date('Y-m-d', strtotime('your input field name')))->get();
add a comment |
i hope this will help you, date()
function is php function which converts a string to datetime.
$users = User::where('birthdate' , '<', date('Y-m-d', strtotime('your input field name')))->get();
i hope this will help you, date()
function is php function which converts a string to datetime.
$users = User::where('birthdate' , '<', date('Y-m-d', strtotime('your input field name')))->get();
answered Oct 29 '18 at 8:27


Naveed AliNaveed Ali
22916
22916
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53041087%2fget-users-by-age-using-user-birthdate-column-from-table%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
4yht1x2e5IYdh U mRKFm,DQbF4mjSyvMlV B,9ULXg 4csGSRDwQCpv1b TiYke8,Jxq S0VsEAHO8
2
what do you mean
by age
? you mean that you want to fetch all users greater than 20 years?– hassan
Oct 29 '18 at 8:11
Yes of course @hassan
– Andreas Hunter
Oct 29 '18 at 8:12
2
what is the column type? datetime/date?
– hassan
Oct 29 '18 at 8:13
1
Column type string. If you think will change the type of field to date from string, I will change it. @hassan
– Andreas Hunter
Oct 29 '18 at 8:16
1
you might want to use a local scope for that.
– duckduckduck
Oct 29 '18 at 9:08