How to handle kind of nested Promises in NodeJS
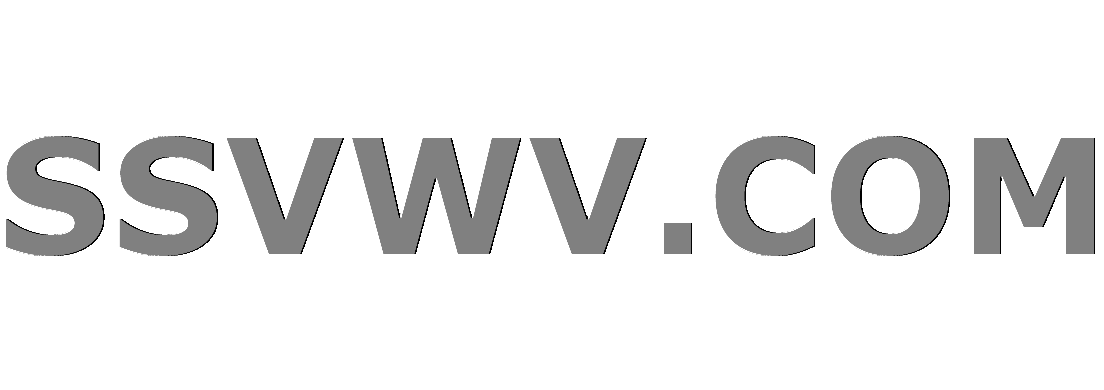
Multi tool use
I'm, relatively new to Promises, so I hope you can help me.
I have the following code:
bcrypt.genSalt(10)
.then((salt) =>{
return bcrypt.hash(newUser.password, salt)
})
.then((hash)=>{
newUser.password = hash;
return mariaDB.pool.getConnection()
})
.then((conn)=>{
conn.beginTransaction()
.then() //here I'm doing some database request
.catch((err)=>{
console.log(err)
return conn.rollback() //where is this Promise handled?
})
})
.catch((err)=>{
res.json({error: err})
})
I receive a newUser object, which I first pass to bcrypt to encrypt my password.
Then I need to make a transaction to my MariaDB database. But is this kind of "nested Promises" correct? Is there a better solution? Where is the promise "return conn.rollback" handled?
Greetings and Thanks!
javascript node.js callback promise nested
add a comment |
I'm, relatively new to Promises, so I hope you can help me.
I have the following code:
bcrypt.genSalt(10)
.then((salt) =>{
return bcrypt.hash(newUser.password, salt)
})
.then((hash)=>{
newUser.password = hash;
return mariaDB.pool.getConnection()
})
.then((conn)=>{
conn.beginTransaction()
.then() //here I'm doing some database request
.catch((err)=>{
console.log(err)
return conn.rollback() //where is this Promise handled?
})
})
.catch((err)=>{
res.json({error: err})
})
I receive a newUser object, which I first pass to bcrypt to encrypt my password.
Then I need to make a transaction to my MariaDB database. But is this kind of "nested Promises" correct? Is there a better solution? Where is the promise "return conn.rollback" handled?
Greetings and Thanks!
javascript node.js callback promise nested
Refactoring this with async/await will be more readable and the code will look like sync but it will behave like async.
– squeekyDave
Nov 14 '18 at 12:10
can you give me s.th. like a pseudo code? How can I change this to async and await?
– mcAngular2
Nov 14 '18 at 12:15
@mcAngular2 you can try using async/await here. Makes life a lot easier!
– Abrar
Nov 14 '18 at 13:30
add a comment |
I'm, relatively new to Promises, so I hope you can help me.
I have the following code:
bcrypt.genSalt(10)
.then((salt) =>{
return bcrypt.hash(newUser.password, salt)
})
.then((hash)=>{
newUser.password = hash;
return mariaDB.pool.getConnection()
})
.then((conn)=>{
conn.beginTransaction()
.then() //here I'm doing some database request
.catch((err)=>{
console.log(err)
return conn.rollback() //where is this Promise handled?
})
})
.catch((err)=>{
res.json({error: err})
})
I receive a newUser object, which I first pass to bcrypt to encrypt my password.
Then I need to make a transaction to my MariaDB database. But is this kind of "nested Promises" correct? Is there a better solution? Where is the promise "return conn.rollback" handled?
Greetings and Thanks!
javascript node.js callback promise nested
I'm, relatively new to Promises, so I hope you can help me.
I have the following code:
bcrypt.genSalt(10)
.then((salt) =>{
return bcrypt.hash(newUser.password, salt)
})
.then((hash)=>{
newUser.password = hash;
return mariaDB.pool.getConnection()
})
.then((conn)=>{
conn.beginTransaction()
.then() //here I'm doing some database request
.catch((err)=>{
console.log(err)
return conn.rollback() //where is this Promise handled?
})
})
.catch((err)=>{
res.json({error: err})
})
I receive a newUser object, which I first pass to bcrypt to encrypt my password.
Then I need to make a transaction to my MariaDB database. But is this kind of "nested Promises" correct? Is there a better solution? Where is the promise "return conn.rollback" handled?
Greetings and Thanks!
javascript node.js callback promise nested
javascript node.js callback promise nested
asked Nov 14 '18 at 11:46
mcAngular2mcAngular2
1169
1169
Refactoring this with async/await will be more readable and the code will look like sync but it will behave like async.
– squeekyDave
Nov 14 '18 at 12:10
can you give me s.th. like a pseudo code? How can I change this to async and await?
– mcAngular2
Nov 14 '18 at 12:15
@mcAngular2 you can try using async/await here. Makes life a lot easier!
– Abrar
Nov 14 '18 at 13:30
add a comment |
Refactoring this with async/await will be more readable and the code will look like sync but it will behave like async.
– squeekyDave
Nov 14 '18 at 12:10
can you give me s.th. like a pseudo code? How can I change this to async and await?
– mcAngular2
Nov 14 '18 at 12:15
@mcAngular2 you can try using async/await here. Makes life a lot easier!
– Abrar
Nov 14 '18 at 13:30
Refactoring this with async/await will be more readable and the code will look like sync but it will behave like async.
– squeekyDave
Nov 14 '18 at 12:10
Refactoring this with async/await will be more readable and the code will look like sync but it will behave like async.
– squeekyDave
Nov 14 '18 at 12:10
can you give me s.th. like a pseudo code? How can I change this to async and await?
– mcAngular2
Nov 14 '18 at 12:15
can you give me s.th. like a pseudo code? How can I change this to async and await?
– mcAngular2
Nov 14 '18 at 12:15
@mcAngular2 you can try using async/await here. Makes life a lot easier!
– Abrar
Nov 14 '18 at 13:30
@mcAngular2 you can try using async/await here. Makes life a lot easier!
– Abrar
Nov 14 '18 at 13:30
add a comment |
4 Answers
4
active
oldest
votes
Do it simple like this:
bcrypt.genSalt(10)
.then(salt => bcrypt.hash(newUser.password, salt))
.then(hash => {
newUser.password = hash;
return mariaDB.pool.getConnection()
})
.then(conn => {
return conn.beginTransaction()
.then(() => {
// here I'm doing some database request
})
.catch( err => {
conn.rollback();
throw new Error(err); // this error will be cathed on bottom catch
});
})
.catch(err => res.json({error: err}))
i will using this thanks
– mcAngular2
Nov 14 '18 at 13:28
@mcAngular2 you are welcome
– аlex dykyі
Nov 14 '18 at 16:58
add a comment |
bcrypt.genSalt(10)
.then((salt) =>{
return bcrypt.hash(newUser.password, salt)
})
.then((hash)=>{
newUser.password = hash;
return mariaDB.pool.getConnection()
})
.then((conn)=>{
return dbops(conn)
})
.catch((err)=>{
res.json({error: err})
})
// added new function db ops
function dbops(conn){
return new Promise(function(resolve,reject){
conn.beginTransaction()
.then((data)=>{
//db stuff
resolve("db stuff done")
}).catch((err)=>{
console.log(err)
conn.rollback()
reject(err)
})
})}
Hope this will help you.
new Promise
is promise construction antipattern. There's already a promise, no need to create a new one. It also doesn't take a promise fromrollback()
into account.
– estus
Nov 14 '18 at 12:20
add a comment |
return conn.rollback() //where is this Promise handled?
is not handled, this is the problem with this snippet. Nested promises should be chained in order to maintain proper control flow, i.e. returned from then
and catch
callbacks:
.then((conn)=>{
return conn.beginTransaction()
...
Nested promise is required because conn
should be available in then
callbacks. A more convenient way to handle this is async..await
, this allows to flatten nested promises:
try {
const salt = await bcrypt.genSalt(10)
const hash = await bcrypt.hash(newUser.password, salt)
newUser.password = hash;
const conn = await mariaDB.pool.getConnection()
try {
conn.beginTransaction()
// ...
} catch (err) {
await conn.rollback()
}
} catch (err) {
res.json({error: err})
}
A good thing would be to rethrow an error after rollback
because it's clear that things went wrong at this point.
How can i change this suing async and await?
– mcAngular2
Nov 14 '18 at 12:12
Something like this. Hope this helps.,
– estus
Nov 14 '18 at 12:19
Just wrap the code above in async function yourFuncName(){}; More about async/await developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– squeekyDave
Nov 14 '18 at 12:21
add a comment |
A rewrite with async/await (making your life easier by getting rid of all those nested promises, thank me later!) would look like this:
try {
const salt = await bcrypt.genSalt(10);
const hash = await bcrypt.hash(newUser.password, salt);
newUser.password = hash;
const conn = await mariaDB.pool.getConnection();
try {
const transaction = await conn.beginTransaction();
// your db calls
} catch (err) {
console.log(err);
return conn.rollback();
}
} catch (err) {
res.json({error: err})
}
Please make sure you wrap this block within an async
function. For example, for self-invoking function use:
(async() => {
// the block of code using await ...
})();
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53299512%2fhow-to-handle-kind-of-nested-promises-in-nodejs%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
Do it simple like this:
bcrypt.genSalt(10)
.then(salt => bcrypt.hash(newUser.password, salt))
.then(hash => {
newUser.password = hash;
return mariaDB.pool.getConnection()
})
.then(conn => {
return conn.beginTransaction()
.then(() => {
// here I'm doing some database request
})
.catch( err => {
conn.rollback();
throw new Error(err); // this error will be cathed on bottom catch
});
})
.catch(err => res.json({error: err}))
i will using this thanks
– mcAngular2
Nov 14 '18 at 13:28
@mcAngular2 you are welcome
– аlex dykyі
Nov 14 '18 at 16:58
add a comment |
Do it simple like this:
bcrypt.genSalt(10)
.then(salt => bcrypt.hash(newUser.password, salt))
.then(hash => {
newUser.password = hash;
return mariaDB.pool.getConnection()
})
.then(conn => {
return conn.beginTransaction()
.then(() => {
// here I'm doing some database request
})
.catch( err => {
conn.rollback();
throw new Error(err); // this error will be cathed on bottom catch
});
})
.catch(err => res.json({error: err}))
i will using this thanks
– mcAngular2
Nov 14 '18 at 13:28
@mcAngular2 you are welcome
– аlex dykyі
Nov 14 '18 at 16:58
add a comment |
Do it simple like this:
bcrypt.genSalt(10)
.then(salt => bcrypt.hash(newUser.password, salt))
.then(hash => {
newUser.password = hash;
return mariaDB.pool.getConnection()
})
.then(conn => {
return conn.beginTransaction()
.then(() => {
// here I'm doing some database request
})
.catch( err => {
conn.rollback();
throw new Error(err); // this error will be cathed on bottom catch
});
})
.catch(err => res.json({error: err}))
Do it simple like this:
bcrypt.genSalt(10)
.then(salt => bcrypt.hash(newUser.password, salt))
.then(hash => {
newUser.password = hash;
return mariaDB.pool.getConnection()
})
.then(conn => {
return conn.beginTransaction()
.then(() => {
// here I'm doing some database request
})
.catch( err => {
conn.rollback();
throw new Error(err); // this error will be cathed on bottom catch
});
})
.catch(err => res.json({error: err}))
edited Nov 20 '18 at 16:03


Yvette Colomb♦
20.3k1470110
20.3k1470110
answered Nov 14 '18 at 12:27


аlex dykyіаlex dykyі
1,7691225
1,7691225
i will using this thanks
– mcAngular2
Nov 14 '18 at 13:28
@mcAngular2 you are welcome
– аlex dykyі
Nov 14 '18 at 16:58
add a comment |
i will using this thanks
– mcAngular2
Nov 14 '18 at 13:28
@mcAngular2 you are welcome
– аlex dykyі
Nov 14 '18 at 16:58
i will using this thanks
– mcAngular2
Nov 14 '18 at 13:28
i will using this thanks
– mcAngular2
Nov 14 '18 at 13:28
@mcAngular2 you are welcome
– аlex dykyі
Nov 14 '18 at 16:58
@mcAngular2 you are welcome
– аlex dykyі
Nov 14 '18 at 16:58
add a comment |
bcrypt.genSalt(10)
.then((salt) =>{
return bcrypt.hash(newUser.password, salt)
})
.then((hash)=>{
newUser.password = hash;
return mariaDB.pool.getConnection()
})
.then((conn)=>{
return dbops(conn)
})
.catch((err)=>{
res.json({error: err})
})
// added new function db ops
function dbops(conn){
return new Promise(function(resolve,reject){
conn.beginTransaction()
.then((data)=>{
//db stuff
resolve("db stuff done")
}).catch((err)=>{
console.log(err)
conn.rollback()
reject(err)
})
})}
Hope this will help you.
new Promise
is promise construction antipattern. There's already a promise, no need to create a new one. It also doesn't take a promise fromrollback()
into account.
– estus
Nov 14 '18 at 12:20
add a comment |
bcrypt.genSalt(10)
.then((salt) =>{
return bcrypt.hash(newUser.password, salt)
})
.then((hash)=>{
newUser.password = hash;
return mariaDB.pool.getConnection()
})
.then((conn)=>{
return dbops(conn)
})
.catch((err)=>{
res.json({error: err})
})
// added new function db ops
function dbops(conn){
return new Promise(function(resolve,reject){
conn.beginTransaction()
.then((data)=>{
//db stuff
resolve("db stuff done")
}).catch((err)=>{
console.log(err)
conn.rollback()
reject(err)
})
})}
Hope this will help you.
new Promise
is promise construction antipattern. There's already a promise, no need to create a new one. It also doesn't take a promise fromrollback()
into account.
– estus
Nov 14 '18 at 12:20
add a comment |
bcrypt.genSalt(10)
.then((salt) =>{
return bcrypt.hash(newUser.password, salt)
})
.then((hash)=>{
newUser.password = hash;
return mariaDB.pool.getConnection()
})
.then((conn)=>{
return dbops(conn)
})
.catch((err)=>{
res.json({error: err})
})
// added new function db ops
function dbops(conn){
return new Promise(function(resolve,reject){
conn.beginTransaction()
.then((data)=>{
//db stuff
resolve("db stuff done")
}).catch((err)=>{
console.log(err)
conn.rollback()
reject(err)
})
})}
Hope this will help you.
bcrypt.genSalt(10)
.then((salt) =>{
return bcrypt.hash(newUser.password, salt)
})
.then((hash)=>{
newUser.password = hash;
return mariaDB.pool.getConnection()
})
.then((conn)=>{
return dbops(conn)
})
.catch((err)=>{
res.json({error: err})
})
// added new function db ops
function dbops(conn){
return new Promise(function(resolve,reject){
conn.beginTransaction()
.then((data)=>{
//db stuff
resolve("db stuff done")
}).catch((err)=>{
console.log(err)
conn.rollback()
reject(err)
})
})}
Hope this will help you.
answered Nov 14 '18 at 12:15


siddhant sankhesiddhant sankhe
31339
31339
new Promise
is promise construction antipattern. There's already a promise, no need to create a new one. It also doesn't take a promise fromrollback()
into account.
– estus
Nov 14 '18 at 12:20
add a comment |
new Promise
is promise construction antipattern. There's already a promise, no need to create a new one. It also doesn't take a promise fromrollback()
into account.
– estus
Nov 14 '18 at 12:20
new Promise
is promise construction antipattern. There's already a promise, no need to create a new one. It also doesn't take a promise from rollback()
into account.– estus
Nov 14 '18 at 12:20
new Promise
is promise construction antipattern. There's already a promise, no need to create a new one. It also doesn't take a promise from rollback()
into account.– estus
Nov 14 '18 at 12:20
add a comment |
return conn.rollback() //where is this Promise handled?
is not handled, this is the problem with this snippet. Nested promises should be chained in order to maintain proper control flow, i.e. returned from then
and catch
callbacks:
.then((conn)=>{
return conn.beginTransaction()
...
Nested promise is required because conn
should be available in then
callbacks. A more convenient way to handle this is async..await
, this allows to flatten nested promises:
try {
const salt = await bcrypt.genSalt(10)
const hash = await bcrypt.hash(newUser.password, salt)
newUser.password = hash;
const conn = await mariaDB.pool.getConnection()
try {
conn.beginTransaction()
// ...
} catch (err) {
await conn.rollback()
}
} catch (err) {
res.json({error: err})
}
A good thing would be to rethrow an error after rollback
because it's clear that things went wrong at this point.
How can i change this suing async and await?
– mcAngular2
Nov 14 '18 at 12:12
Something like this. Hope this helps.,
– estus
Nov 14 '18 at 12:19
Just wrap the code above in async function yourFuncName(){}; More about async/await developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– squeekyDave
Nov 14 '18 at 12:21
add a comment |
return conn.rollback() //where is this Promise handled?
is not handled, this is the problem with this snippet. Nested promises should be chained in order to maintain proper control flow, i.e. returned from then
and catch
callbacks:
.then((conn)=>{
return conn.beginTransaction()
...
Nested promise is required because conn
should be available in then
callbacks. A more convenient way to handle this is async..await
, this allows to flatten nested promises:
try {
const salt = await bcrypt.genSalt(10)
const hash = await bcrypt.hash(newUser.password, salt)
newUser.password = hash;
const conn = await mariaDB.pool.getConnection()
try {
conn.beginTransaction()
// ...
} catch (err) {
await conn.rollback()
}
} catch (err) {
res.json({error: err})
}
A good thing would be to rethrow an error after rollback
because it's clear that things went wrong at this point.
How can i change this suing async and await?
– mcAngular2
Nov 14 '18 at 12:12
Something like this. Hope this helps.,
– estus
Nov 14 '18 at 12:19
Just wrap the code above in async function yourFuncName(){}; More about async/await developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– squeekyDave
Nov 14 '18 at 12:21
add a comment |
return conn.rollback() //where is this Promise handled?
is not handled, this is the problem with this snippet. Nested promises should be chained in order to maintain proper control flow, i.e. returned from then
and catch
callbacks:
.then((conn)=>{
return conn.beginTransaction()
...
Nested promise is required because conn
should be available in then
callbacks. A more convenient way to handle this is async..await
, this allows to flatten nested promises:
try {
const salt = await bcrypt.genSalt(10)
const hash = await bcrypt.hash(newUser.password, salt)
newUser.password = hash;
const conn = await mariaDB.pool.getConnection()
try {
conn.beginTransaction()
// ...
} catch (err) {
await conn.rollback()
}
} catch (err) {
res.json({error: err})
}
A good thing would be to rethrow an error after rollback
because it's clear that things went wrong at this point.
return conn.rollback() //where is this Promise handled?
is not handled, this is the problem with this snippet. Nested promises should be chained in order to maintain proper control flow, i.e. returned from then
and catch
callbacks:
.then((conn)=>{
return conn.beginTransaction()
...
Nested promise is required because conn
should be available in then
callbacks. A more convenient way to handle this is async..await
, this allows to flatten nested promises:
try {
const salt = await bcrypt.genSalt(10)
const hash = await bcrypt.hash(newUser.password, salt)
newUser.password = hash;
const conn = await mariaDB.pool.getConnection()
try {
conn.beginTransaction()
// ...
} catch (err) {
await conn.rollback()
}
} catch (err) {
res.json({error: err})
}
A good thing would be to rethrow an error after rollback
because it's clear that things went wrong at this point.
edited Nov 14 '18 at 12:22
answered Nov 14 '18 at 12:07
estusestus
71.1k22105221
71.1k22105221
How can i change this suing async and await?
– mcAngular2
Nov 14 '18 at 12:12
Something like this. Hope this helps.,
– estus
Nov 14 '18 at 12:19
Just wrap the code above in async function yourFuncName(){}; More about async/await developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– squeekyDave
Nov 14 '18 at 12:21
add a comment |
How can i change this suing async and await?
– mcAngular2
Nov 14 '18 at 12:12
Something like this. Hope this helps.,
– estus
Nov 14 '18 at 12:19
Just wrap the code above in async function yourFuncName(){}; More about async/await developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– squeekyDave
Nov 14 '18 at 12:21
How can i change this suing async and await?
– mcAngular2
Nov 14 '18 at 12:12
How can i change this suing async and await?
– mcAngular2
Nov 14 '18 at 12:12
Something like this. Hope this helps.,
– estus
Nov 14 '18 at 12:19
Something like this. Hope this helps.,
– estus
Nov 14 '18 at 12:19
Just wrap the code above in async function yourFuncName(){}; More about async/await developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– squeekyDave
Nov 14 '18 at 12:21
Just wrap the code above in async function yourFuncName(){}; More about async/await developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– squeekyDave
Nov 14 '18 at 12:21
add a comment |
A rewrite with async/await (making your life easier by getting rid of all those nested promises, thank me later!) would look like this:
try {
const salt = await bcrypt.genSalt(10);
const hash = await bcrypt.hash(newUser.password, salt);
newUser.password = hash;
const conn = await mariaDB.pool.getConnection();
try {
const transaction = await conn.beginTransaction();
// your db calls
} catch (err) {
console.log(err);
return conn.rollback();
}
} catch (err) {
res.json({error: err})
}
Please make sure you wrap this block within an async
function. For example, for self-invoking function use:
(async() => {
// the block of code using await ...
})();
add a comment |
A rewrite with async/await (making your life easier by getting rid of all those nested promises, thank me later!) would look like this:
try {
const salt = await bcrypt.genSalt(10);
const hash = await bcrypt.hash(newUser.password, salt);
newUser.password = hash;
const conn = await mariaDB.pool.getConnection();
try {
const transaction = await conn.beginTransaction();
// your db calls
} catch (err) {
console.log(err);
return conn.rollback();
}
} catch (err) {
res.json({error: err})
}
Please make sure you wrap this block within an async
function. For example, for self-invoking function use:
(async() => {
// the block of code using await ...
})();
add a comment |
A rewrite with async/await (making your life easier by getting rid of all those nested promises, thank me later!) would look like this:
try {
const salt = await bcrypt.genSalt(10);
const hash = await bcrypt.hash(newUser.password, salt);
newUser.password = hash;
const conn = await mariaDB.pool.getConnection();
try {
const transaction = await conn.beginTransaction();
// your db calls
} catch (err) {
console.log(err);
return conn.rollback();
}
} catch (err) {
res.json({error: err})
}
Please make sure you wrap this block within an async
function. For example, for self-invoking function use:
(async() => {
// the block of code using await ...
})();
A rewrite with async/await (making your life easier by getting rid of all those nested promises, thank me later!) would look like this:
try {
const salt = await bcrypt.genSalt(10);
const hash = await bcrypt.hash(newUser.password, salt);
newUser.password = hash;
const conn = await mariaDB.pool.getConnection();
try {
const transaction = await conn.beginTransaction();
// your db calls
} catch (err) {
console.log(err);
return conn.rollback();
}
} catch (err) {
res.json({error: err})
}
Please make sure you wrap this block within an async
function. For example, for self-invoking function use:
(async() => {
// the block of code using await ...
})();
answered Nov 14 '18 at 13:29


AbrarAbrar
7321024
7321024
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53299512%2fhow-to-handle-kind-of-nested-promises-in-nodejs%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
v7kxZnS I blCgK V
Refactoring this with async/await will be more readable and the code will look like sync but it will behave like async.
– squeekyDave
Nov 14 '18 at 12:10
can you give me s.th. like a pseudo code? How can I change this to async and await?
– mcAngular2
Nov 14 '18 at 12:15
@mcAngular2 you can try using async/await here. Makes life a lot easier!
– Abrar
Nov 14 '18 at 13:30