How to get included XML binding in DataBinding
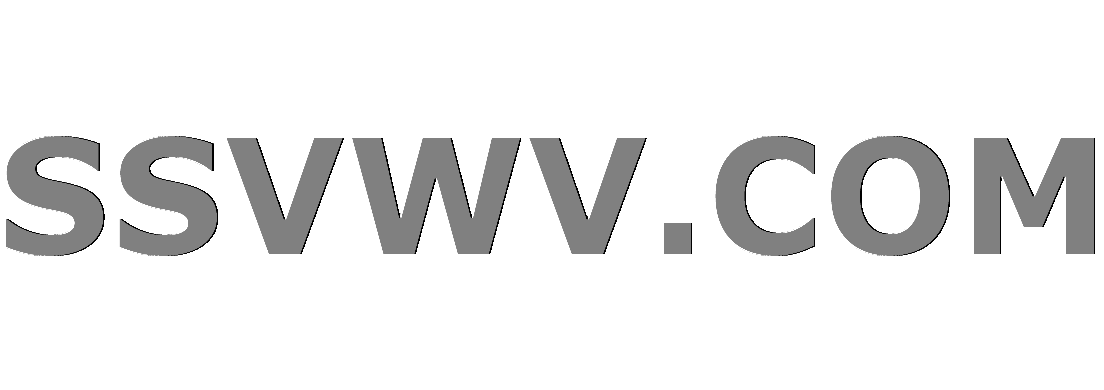
Multi tool use
I have a DataBinding class ActivityMainBinding
where the XMl looks like this
<?xml version="1.0" encoding="utf-8"?>
<layout>
<android.support.design.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/container"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<include layout="@layout/content_main" />
<android.support.design.widget.FloatingActionButton
android:id="@+id/fab"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="bottom|end"
android:layout_margin="@dimen/fab_margin"
app:srcCompat="@drawable/ic_add" />
</android.support.design.widget.CoordinatorLayout>
</layout>
And the content_main.xml is another binding
<?xml version="1.0" encoding="utf-8"?>
<layout>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
tools:showIn="@layout/activity_main">
<android.support.v7.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="0dp"
android:layout_height="0dp"
android:clickable="true"
android:focusable="true"
android:focusableInTouchMode="true"
android:scrollbars="vertical"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</android.support.constraint.ConstraintLayout>
</layout>
Now, in my activity I set the content view like this.
ActivityMainBinding activityMainBinding = DataBindingUtil.setContentView(this, R.layout.activity_main);
Now I can access all XML elemets using this binding. like
Toolbar toolbar = activityMainBinding.toolbar;
but some of my widgets are in the included layout which is ContentMainBinding
Now, how can I create a ContentMainBinding
instance from ActivityMainBinding
?
I tried
contentMainBinding = DataBindingUtil.getBinding(activityMainBinding.container);
But, its crashing with error
ActivityMainBindingImpl cannot be cast to ContentMainBinding

add a comment |
I have a DataBinding class ActivityMainBinding
where the XMl looks like this
<?xml version="1.0" encoding="utf-8"?>
<layout>
<android.support.design.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/container"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<include layout="@layout/content_main" />
<android.support.design.widget.FloatingActionButton
android:id="@+id/fab"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="bottom|end"
android:layout_margin="@dimen/fab_margin"
app:srcCompat="@drawable/ic_add" />
</android.support.design.widget.CoordinatorLayout>
</layout>
And the content_main.xml is another binding
<?xml version="1.0" encoding="utf-8"?>
<layout>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
tools:showIn="@layout/activity_main">
<android.support.v7.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="0dp"
android:layout_height="0dp"
android:clickable="true"
android:focusable="true"
android:focusableInTouchMode="true"
android:scrollbars="vertical"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</android.support.constraint.ConstraintLayout>
</layout>
Now, in my activity I set the content view like this.
ActivityMainBinding activityMainBinding = DataBindingUtil.setContentView(this, R.layout.activity_main);
Now I can access all XML elemets using this binding. like
Toolbar toolbar = activityMainBinding.toolbar;
but some of my widgets are in the included layout which is ContentMainBinding
Now, how can I create a ContentMainBinding
instance from ActivityMainBinding
?
I tried
contentMainBinding = DataBindingUtil.getBinding(activityMainBinding.container);
But, its crashing with error
ActivityMainBindingImpl cannot be cast to ContentMainBinding

add a comment |
I have a DataBinding class ActivityMainBinding
where the XMl looks like this
<?xml version="1.0" encoding="utf-8"?>
<layout>
<android.support.design.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/container"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<include layout="@layout/content_main" />
<android.support.design.widget.FloatingActionButton
android:id="@+id/fab"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="bottom|end"
android:layout_margin="@dimen/fab_margin"
app:srcCompat="@drawable/ic_add" />
</android.support.design.widget.CoordinatorLayout>
</layout>
And the content_main.xml is another binding
<?xml version="1.0" encoding="utf-8"?>
<layout>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
tools:showIn="@layout/activity_main">
<android.support.v7.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="0dp"
android:layout_height="0dp"
android:clickable="true"
android:focusable="true"
android:focusableInTouchMode="true"
android:scrollbars="vertical"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</android.support.constraint.ConstraintLayout>
</layout>
Now, in my activity I set the content view like this.
ActivityMainBinding activityMainBinding = DataBindingUtil.setContentView(this, R.layout.activity_main);
Now I can access all XML elemets using this binding. like
Toolbar toolbar = activityMainBinding.toolbar;
but some of my widgets are in the included layout which is ContentMainBinding
Now, how can I create a ContentMainBinding
instance from ActivityMainBinding
?
I tried
contentMainBinding = DataBindingUtil.getBinding(activityMainBinding.container);
But, its crashing with error
ActivityMainBindingImpl cannot be cast to ContentMainBinding

I have a DataBinding class ActivityMainBinding
where the XMl looks like this
<?xml version="1.0" encoding="utf-8"?>
<layout>
<android.support.design.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/container"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<include layout="@layout/content_main" />
<android.support.design.widget.FloatingActionButton
android:id="@+id/fab"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="bottom|end"
android:layout_margin="@dimen/fab_margin"
app:srcCompat="@drawable/ic_add" />
</android.support.design.widget.CoordinatorLayout>
</layout>
And the content_main.xml is another binding
<?xml version="1.0" encoding="utf-8"?>
<layout>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
tools:showIn="@layout/activity_main">
<android.support.v7.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="0dp"
android:layout_height="0dp"
android:clickable="true"
android:focusable="true"
android:focusableInTouchMode="true"
android:scrollbars="vertical"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</android.support.constraint.ConstraintLayout>
</layout>
Now, in my activity I set the content view like this.
ActivityMainBinding activityMainBinding = DataBindingUtil.setContentView(this, R.layout.activity_main);
Now I can access all XML elemets using this binding. like
Toolbar toolbar = activityMainBinding.toolbar;
but some of my widgets are in the included layout which is ContentMainBinding
Now, how can I create a ContentMainBinding
instance from ActivityMainBinding
?
I tried
contentMainBinding = DataBindingUtil.getBinding(activityMainBinding.container);
But, its crashing with error
ActivityMainBindingImpl cannot be cast to ContentMainBinding


asked Nov 14 '18 at 12:47
doedoe
7010
7010
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
There is no need to add <layout>----</layout>
tag in your content_main.xml file. You need to set id of included layout like :
<?xml version="1.0" encoding="utf-8"?>
<layout>
<android.support.design.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/container"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<include layout="@layoutcontent_main" android:id="@+id/contentMain" />
<android.support.design.widget.FloatingActionButton
android:id="@+id/fab"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="bottom|end"
android:layout_margin="@dimen/fab_margin"
app:srcCompat="@drawable/ic_add" />
</android.support.design.widget.CoordinatorLayout>
</layout>
Then you can directly access included layout view using
ActivityMainBinding activityMainBinding = DataBindingUtil.setContentView(this, R.layout.activity_main);
activityMainBinding.contentMain.recyclerView.setAdapter();
add a comment |
Add id to include tag
<include
android:id="@+id/included"
layout="@layout/content_main"/>
And in code use
activityMainBinding.included.recyclerView
Check that link
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53300598%2fhow-to-get-included-xml-binding-in-databinding%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
There is no need to add <layout>----</layout>
tag in your content_main.xml file. You need to set id of included layout like :
<?xml version="1.0" encoding="utf-8"?>
<layout>
<android.support.design.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/container"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<include layout="@layoutcontent_main" android:id="@+id/contentMain" />
<android.support.design.widget.FloatingActionButton
android:id="@+id/fab"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="bottom|end"
android:layout_margin="@dimen/fab_margin"
app:srcCompat="@drawable/ic_add" />
</android.support.design.widget.CoordinatorLayout>
</layout>
Then you can directly access included layout view using
ActivityMainBinding activityMainBinding = DataBindingUtil.setContentView(this, R.layout.activity_main);
activityMainBinding.contentMain.recyclerView.setAdapter();
add a comment |
There is no need to add <layout>----</layout>
tag in your content_main.xml file. You need to set id of included layout like :
<?xml version="1.0" encoding="utf-8"?>
<layout>
<android.support.design.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/container"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<include layout="@layoutcontent_main" android:id="@+id/contentMain" />
<android.support.design.widget.FloatingActionButton
android:id="@+id/fab"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="bottom|end"
android:layout_margin="@dimen/fab_margin"
app:srcCompat="@drawable/ic_add" />
</android.support.design.widget.CoordinatorLayout>
</layout>
Then you can directly access included layout view using
ActivityMainBinding activityMainBinding = DataBindingUtil.setContentView(this, R.layout.activity_main);
activityMainBinding.contentMain.recyclerView.setAdapter();
add a comment |
There is no need to add <layout>----</layout>
tag in your content_main.xml file. You need to set id of included layout like :
<?xml version="1.0" encoding="utf-8"?>
<layout>
<android.support.design.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/container"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<include layout="@layoutcontent_main" android:id="@+id/contentMain" />
<android.support.design.widget.FloatingActionButton
android:id="@+id/fab"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="bottom|end"
android:layout_margin="@dimen/fab_margin"
app:srcCompat="@drawable/ic_add" />
</android.support.design.widget.CoordinatorLayout>
</layout>
Then you can directly access included layout view using
ActivityMainBinding activityMainBinding = DataBindingUtil.setContentView(this, R.layout.activity_main);
activityMainBinding.contentMain.recyclerView.setAdapter();
There is no need to add <layout>----</layout>
tag in your content_main.xml file. You need to set id of included layout like :
<?xml version="1.0" encoding="utf-8"?>
<layout>
<android.support.design.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/container"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<include layout="@layoutcontent_main" android:id="@+id/contentMain" />
<android.support.design.widget.FloatingActionButton
android:id="@+id/fab"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="bottom|end"
android:layout_margin="@dimen/fab_margin"
app:srcCompat="@drawable/ic_add" />
</android.support.design.widget.CoordinatorLayout>
</layout>
Then you can directly access included layout view using
ActivityMainBinding activityMainBinding = DataBindingUtil.setContentView(this, R.layout.activity_main);
activityMainBinding.contentMain.recyclerView.setAdapter();
answered Nov 14 '18 at 13:03


Paresh RajputParesh Rajput
994
994
add a comment |
add a comment |
Add id to include tag
<include
android:id="@+id/included"
layout="@layout/content_main"/>
And in code use
activityMainBinding.included.recyclerView
Check that link
add a comment |
Add id to include tag
<include
android:id="@+id/included"
layout="@layout/content_main"/>
And in code use
activityMainBinding.included.recyclerView
Check that link
add a comment |
Add id to include tag
<include
android:id="@+id/included"
layout="@layout/content_main"/>
And in code use
activityMainBinding.included.recyclerView
Check that link
Add id to include tag
<include
android:id="@+id/included"
layout="@layout/content_main"/>
And in code use
activityMainBinding.included.recyclerView
Check that link
answered Nov 14 '18 at 13:07


OnixOnix
4578
4578
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53300598%2fhow-to-get-included-xml-binding-in-databinding%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
B,3OXxt,Ci J2D,eDEs5,jnb4nYnqam9LjwS,98k,mb1lE,4VvEJkZ,lMxTYqfl1ZI,P9,MRLj,rXC3vkXv qZUktF Y 0qTn m