Can one change templates arguments?
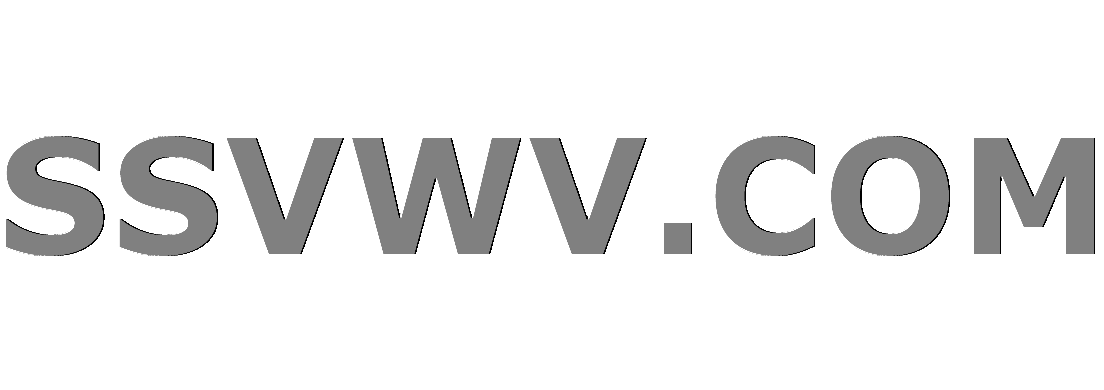
Multi tool use
I have written the following Matrix class:
template <typename T, size_t r, size_t c> //r=rows,c=cols of the Matrix
class Matrix {
public:
size_t row = 0;
size_t col = 0;
T *data;
template <size_t L>
Matrix<T, r, L> operator*(const Matrix<T, c, L> &other) const;
template <size_t r, size_t c>
Matrix &operator=(const Matrix<T, r, c> &other)
// ...
};
I overrode some operators to do some basic arithmetic and everything seems to work properly - however there is an issue which I don't know how to address properly: Given the following lines of code:
Matrix<double, 1, 4> m1(1.2);
Matrix<double, 4, 1> m2(1.2);
Matrix<double, 2, 2> m3; // problematic line
m3 = m1 * m2;
m3
is of type Matrix<double, 2, 2>
, is correctly computed, has one row and one col and carries the 5.76
, but stays as Matrix<double, 2, 2>
. The change of its number of rows and cols is not reflected in its template parameters. Naturally however I would expect the type to be also informative regarding its content.
I don't suppose one cannot turn a Matrix<double, 2, 2>
suddenly into a Matrix<double, 1, 1>
Matrix, but maybe there is a good solution I just cannot think of now.
And replace:
template <size_t r, size_t c> void replace(const Matrix<T, r, c> &other) {
delete data;
row = other.row; //number of rows of a matrix
col = other.col; //number of cols of a matrix
data = new T[col * row]; // data contains all the elements of my matrix
for (size_t i = 0; i < row * col; i++)
data[i] = other.data[i];
}
c++ templates
|
show 5 more comments
I have written the following Matrix class:
template <typename T, size_t r, size_t c> //r=rows,c=cols of the Matrix
class Matrix {
public:
size_t row = 0;
size_t col = 0;
T *data;
template <size_t L>
Matrix<T, r, L> operator*(const Matrix<T, c, L> &other) const;
template <size_t r, size_t c>
Matrix &operator=(const Matrix<T, r, c> &other)
// ...
};
I overrode some operators to do some basic arithmetic and everything seems to work properly - however there is an issue which I don't know how to address properly: Given the following lines of code:
Matrix<double, 1, 4> m1(1.2);
Matrix<double, 4, 1> m2(1.2);
Matrix<double, 2, 2> m3; // problematic line
m3 = m1 * m2;
m3
is of type Matrix<double, 2, 2>
, is correctly computed, has one row and one col and carries the 5.76
, but stays as Matrix<double, 2, 2>
. The change of its number of rows and cols is not reflected in its template parameters. Naturally however I would expect the type to be also informative regarding its content.
I don't suppose one cannot turn a Matrix<double, 2, 2>
suddenly into a Matrix<double, 1, 1>
Matrix, but maybe there is a good solution I just cannot think of now.
And replace:
template <size_t r, size_t c> void replace(const Matrix<T, r, c> &other) {
delete data;
row = other.row; //number of rows of a matrix
col = other.col; //number of cols of a matrix
data = new T[col * row]; // data contains all the elements of my matrix
for (size_t i = 0; i < row * col; i++)
data[i] = other.data[i];
}
c++ templates
1
This is pretty confusing. What exactly did you expectm3
to do? Also isMatrix
self written or fromEigen
?
– Fantastic Mr Fox
Nov 14 '18 at 12:51
2
what does the operator * look like?
– UKMonkey
Nov 14 '18 at 12:52
2
There is no way in normal matrix operations for a 1x4 matrix multiplied by a 4x1 matrix to give a 2x2 matrix. It will (depending on order of operations) give either a 4x4 or a 1x1. Given a 4x4 matrix, I guess you could specify a transformation to a 2x2.
– Peter
Nov 14 '18 at 12:55
2
Storing size information in the type is a very good idea. You just have to adopt initialization andauto
instead of pre-declaring your variables. Alternatively, you can usedecltype(m1 * m2)
.
– Quentin
Nov 14 '18 at 12:57
1
Okay not quite so ludicrous on second read. You could basically create a function to reshape the matrix (you may want two varieties: row-major and column-major). That can be templated and would return an appropriate type to match the assignment.
– paddy
Nov 14 '18 at 13:03
|
show 5 more comments
I have written the following Matrix class:
template <typename T, size_t r, size_t c> //r=rows,c=cols of the Matrix
class Matrix {
public:
size_t row = 0;
size_t col = 0;
T *data;
template <size_t L>
Matrix<T, r, L> operator*(const Matrix<T, c, L> &other) const;
template <size_t r, size_t c>
Matrix &operator=(const Matrix<T, r, c> &other)
// ...
};
I overrode some operators to do some basic arithmetic and everything seems to work properly - however there is an issue which I don't know how to address properly: Given the following lines of code:
Matrix<double, 1, 4> m1(1.2);
Matrix<double, 4, 1> m2(1.2);
Matrix<double, 2, 2> m3; // problematic line
m3 = m1 * m2;
m3
is of type Matrix<double, 2, 2>
, is correctly computed, has one row and one col and carries the 5.76
, but stays as Matrix<double, 2, 2>
. The change of its number of rows and cols is not reflected in its template parameters. Naturally however I would expect the type to be also informative regarding its content.
I don't suppose one cannot turn a Matrix<double, 2, 2>
suddenly into a Matrix<double, 1, 1>
Matrix, but maybe there is a good solution I just cannot think of now.
And replace:
template <size_t r, size_t c> void replace(const Matrix<T, r, c> &other) {
delete data;
row = other.row; //number of rows of a matrix
col = other.col; //number of cols of a matrix
data = new T[col * row]; // data contains all the elements of my matrix
for (size_t i = 0; i < row * col; i++)
data[i] = other.data[i];
}
c++ templates
I have written the following Matrix class:
template <typename T, size_t r, size_t c> //r=rows,c=cols of the Matrix
class Matrix {
public:
size_t row = 0;
size_t col = 0;
T *data;
template <size_t L>
Matrix<T, r, L> operator*(const Matrix<T, c, L> &other) const;
template <size_t r, size_t c>
Matrix &operator=(const Matrix<T, r, c> &other)
// ...
};
I overrode some operators to do some basic arithmetic and everything seems to work properly - however there is an issue which I don't know how to address properly: Given the following lines of code:
Matrix<double, 1, 4> m1(1.2);
Matrix<double, 4, 1> m2(1.2);
Matrix<double, 2, 2> m3; // problematic line
m3 = m1 * m2;
m3
is of type Matrix<double, 2, 2>
, is correctly computed, has one row and one col and carries the 5.76
, but stays as Matrix<double, 2, 2>
. The change of its number of rows and cols is not reflected in its template parameters. Naturally however I would expect the type to be also informative regarding its content.
I don't suppose one cannot turn a Matrix<double, 2, 2>
suddenly into a Matrix<double, 1, 1>
Matrix, but maybe there is a good solution I just cannot think of now.
And replace:
template <size_t r, size_t c> void replace(const Matrix<T, r, c> &other) {
delete data;
row = other.row; //number of rows of a matrix
col = other.col; //number of cols of a matrix
data = new T[col * row]; // data contains all the elements of my matrix
for (size_t i = 0; i < row * col; i++)
data[i] = other.data[i];
}
c++ templates
c++ templates
edited Nov 14 '18 at 13:07
Imago
asked Nov 14 '18 at 12:49
ImagoImago
264315
264315
1
This is pretty confusing. What exactly did you expectm3
to do? Also isMatrix
self written or fromEigen
?
– Fantastic Mr Fox
Nov 14 '18 at 12:51
2
what does the operator * look like?
– UKMonkey
Nov 14 '18 at 12:52
2
There is no way in normal matrix operations for a 1x4 matrix multiplied by a 4x1 matrix to give a 2x2 matrix. It will (depending on order of operations) give either a 4x4 or a 1x1. Given a 4x4 matrix, I guess you could specify a transformation to a 2x2.
– Peter
Nov 14 '18 at 12:55
2
Storing size information in the type is a very good idea. You just have to adopt initialization andauto
instead of pre-declaring your variables. Alternatively, you can usedecltype(m1 * m2)
.
– Quentin
Nov 14 '18 at 12:57
1
Okay not quite so ludicrous on second read. You could basically create a function to reshape the matrix (you may want two varieties: row-major and column-major). That can be templated and would return an appropriate type to match the assignment.
– paddy
Nov 14 '18 at 13:03
|
show 5 more comments
1
This is pretty confusing. What exactly did you expectm3
to do? Also isMatrix
self written or fromEigen
?
– Fantastic Mr Fox
Nov 14 '18 at 12:51
2
what does the operator * look like?
– UKMonkey
Nov 14 '18 at 12:52
2
There is no way in normal matrix operations for a 1x4 matrix multiplied by a 4x1 matrix to give a 2x2 matrix. It will (depending on order of operations) give either a 4x4 or a 1x1. Given a 4x4 matrix, I guess you could specify a transformation to a 2x2.
– Peter
Nov 14 '18 at 12:55
2
Storing size information in the type is a very good idea. You just have to adopt initialization andauto
instead of pre-declaring your variables. Alternatively, you can usedecltype(m1 * m2)
.
– Quentin
Nov 14 '18 at 12:57
1
Okay not quite so ludicrous on second read. You could basically create a function to reshape the matrix (you may want two varieties: row-major and column-major). That can be templated and would return an appropriate type to match the assignment.
– paddy
Nov 14 '18 at 13:03
1
1
This is pretty confusing. What exactly did you expect
m3
to do? Also is Matrix
self written or from Eigen
?– Fantastic Mr Fox
Nov 14 '18 at 12:51
This is pretty confusing. What exactly did you expect
m3
to do? Also is Matrix
self written or from Eigen
?– Fantastic Mr Fox
Nov 14 '18 at 12:51
2
2
what does the operator * look like?
– UKMonkey
Nov 14 '18 at 12:52
what does the operator * look like?
– UKMonkey
Nov 14 '18 at 12:52
2
2
There is no way in normal matrix operations for a 1x4 matrix multiplied by a 4x1 matrix to give a 2x2 matrix. It will (depending on order of operations) give either a 4x4 or a 1x1. Given a 4x4 matrix, I guess you could specify a transformation to a 2x2.
– Peter
Nov 14 '18 at 12:55
There is no way in normal matrix operations for a 1x4 matrix multiplied by a 4x1 matrix to give a 2x2 matrix. It will (depending on order of operations) give either a 4x4 or a 1x1. Given a 4x4 matrix, I guess you could specify a transformation to a 2x2.
– Peter
Nov 14 '18 at 12:55
2
2
Storing size information in the type is a very good idea. You just have to adopt initialization and
auto
instead of pre-declaring your variables. Alternatively, you can use decltype(m1 * m2)
.– Quentin
Nov 14 '18 at 12:57
Storing size information in the type is a very good idea. You just have to adopt initialization and
auto
instead of pre-declaring your variables. Alternatively, you can use decltype(m1 * m2)
.– Quentin
Nov 14 '18 at 12:57
1
1
Okay not quite so ludicrous on second read. You could basically create a function to reshape the matrix (you may want two varieties: row-major and column-major). That can be templated and would return an appropriate type to match the assignment.
– paddy
Nov 14 '18 at 13:03
Okay not quite so ludicrous on second read. You could basically create a function to reshape the matrix (you may want two varieties: row-major and column-major). That can be templated and would return an appropriate type to match the assignment.
– paddy
Nov 14 '18 at 13:03
|
show 5 more comments
1 Answer
1
active
oldest
votes
From your declaration
template <typename T, size_t r, size_t c> //r=rows,c=cols of the Matrix
class Matrix {
public:
template <size_t L>
Matrix<T, r, L> operator*(const Matrix<T, c, L> &other) const;
template <size_t r, size_t c> // BEWARE: shadowing
Matrix &operator=(const Matrix<T, r, c> &other);
// ...
};
we can guess what happens.
Matrix<double, 1, 4> m1(1.2);
Matrix<double, 4, 1> m2(1.2);
m1 * m2; // (1)
(1)
calls Matrix<double, 1, 4>::operator*<1>(Matrix<double, 4, 1> const&)
. It result has then type Matrix<double, 1, 1>
.
Matrix<double, 2, 2> m3;
m3 = /* (2) */ m1 * m2;
(2)
calls Matrix<double, 2, 2>::operator=<1, 1>(Matrix<double, 1, 1> const&)
. This is a problem.
A solution would be to ensure operator=
can only be called with another matrix of the right size:
template <typename T, size_t r, size_t c> //r=rows,c=cols of the Matrix
class Matrix {
public:
template <size_t L>
Matrix<T, r, L> operator*(Matrix<T, c, L> const& other) const;
Matrix &operator=(Matrix const& other);
// ...
};
You could even allow type conversions:
template<class U>
Matrix &operator=(Matrix<U, r, c> const& other);
Finally, you might want to use auto
:
Matrix<double, 1, 4> m1(1.2);
Matrix<double, 4, 1> m2(1.2);
auto m3 = m1 * m2; // m3 is Matrix<double, 1, 1>
That is basically spot on. I like your suggestion to change the =operator and check, if the assignment is valid. Maybe I did view the problem from the wrong angle: If I create a Matrix likem3
, then I already have to know, what it has to look like and what it's going to do. To me it looks now, I cannot work around the auto OR specific in advance what I expect that is going to happen - how the matrix looks like.
– Imago
Nov 14 '18 at 13:23
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53300651%2fcan-one-change-templates-arguments%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
From your declaration
template <typename T, size_t r, size_t c> //r=rows,c=cols of the Matrix
class Matrix {
public:
template <size_t L>
Matrix<T, r, L> operator*(const Matrix<T, c, L> &other) const;
template <size_t r, size_t c> // BEWARE: shadowing
Matrix &operator=(const Matrix<T, r, c> &other);
// ...
};
we can guess what happens.
Matrix<double, 1, 4> m1(1.2);
Matrix<double, 4, 1> m2(1.2);
m1 * m2; // (1)
(1)
calls Matrix<double, 1, 4>::operator*<1>(Matrix<double, 4, 1> const&)
. It result has then type Matrix<double, 1, 1>
.
Matrix<double, 2, 2> m3;
m3 = /* (2) */ m1 * m2;
(2)
calls Matrix<double, 2, 2>::operator=<1, 1>(Matrix<double, 1, 1> const&)
. This is a problem.
A solution would be to ensure operator=
can only be called with another matrix of the right size:
template <typename T, size_t r, size_t c> //r=rows,c=cols of the Matrix
class Matrix {
public:
template <size_t L>
Matrix<T, r, L> operator*(Matrix<T, c, L> const& other) const;
Matrix &operator=(Matrix const& other);
// ...
};
You could even allow type conversions:
template<class U>
Matrix &operator=(Matrix<U, r, c> const& other);
Finally, you might want to use auto
:
Matrix<double, 1, 4> m1(1.2);
Matrix<double, 4, 1> m2(1.2);
auto m3 = m1 * m2; // m3 is Matrix<double, 1, 1>
That is basically spot on. I like your suggestion to change the =operator and check, if the assignment is valid. Maybe I did view the problem from the wrong angle: If I create a Matrix likem3
, then I already have to know, what it has to look like and what it's going to do. To me it looks now, I cannot work around the auto OR specific in advance what I expect that is going to happen - how the matrix looks like.
– Imago
Nov 14 '18 at 13:23
add a comment |
From your declaration
template <typename T, size_t r, size_t c> //r=rows,c=cols of the Matrix
class Matrix {
public:
template <size_t L>
Matrix<T, r, L> operator*(const Matrix<T, c, L> &other) const;
template <size_t r, size_t c> // BEWARE: shadowing
Matrix &operator=(const Matrix<T, r, c> &other);
// ...
};
we can guess what happens.
Matrix<double, 1, 4> m1(1.2);
Matrix<double, 4, 1> m2(1.2);
m1 * m2; // (1)
(1)
calls Matrix<double, 1, 4>::operator*<1>(Matrix<double, 4, 1> const&)
. It result has then type Matrix<double, 1, 1>
.
Matrix<double, 2, 2> m3;
m3 = /* (2) */ m1 * m2;
(2)
calls Matrix<double, 2, 2>::operator=<1, 1>(Matrix<double, 1, 1> const&)
. This is a problem.
A solution would be to ensure operator=
can only be called with another matrix of the right size:
template <typename T, size_t r, size_t c> //r=rows,c=cols of the Matrix
class Matrix {
public:
template <size_t L>
Matrix<T, r, L> operator*(Matrix<T, c, L> const& other) const;
Matrix &operator=(Matrix const& other);
// ...
};
You could even allow type conversions:
template<class U>
Matrix &operator=(Matrix<U, r, c> const& other);
Finally, you might want to use auto
:
Matrix<double, 1, 4> m1(1.2);
Matrix<double, 4, 1> m2(1.2);
auto m3 = m1 * m2; // m3 is Matrix<double, 1, 1>
That is basically spot on. I like your suggestion to change the =operator and check, if the assignment is valid. Maybe I did view the problem from the wrong angle: If I create a Matrix likem3
, then I already have to know, what it has to look like and what it's going to do. To me it looks now, I cannot work around the auto OR specific in advance what I expect that is going to happen - how the matrix looks like.
– Imago
Nov 14 '18 at 13:23
add a comment |
From your declaration
template <typename T, size_t r, size_t c> //r=rows,c=cols of the Matrix
class Matrix {
public:
template <size_t L>
Matrix<T, r, L> operator*(const Matrix<T, c, L> &other) const;
template <size_t r, size_t c> // BEWARE: shadowing
Matrix &operator=(const Matrix<T, r, c> &other);
// ...
};
we can guess what happens.
Matrix<double, 1, 4> m1(1.2);
Matrix<double, 4, 1> m2(1.2);
m1 * m2; // (1)
(1)
calls Matrix<double, 1, 4>::operator*<1>(Matrix<double, 4, 1> const&)
. It result has then type Matrix<double, 1, 1>
.
Matrix<double, 2, 2> m3;
m3 = /* (2) */ m1 * m2;
(2)
calls Matrix<double, 2, 2>::operator=<1, 1>(Matrix<double, 1, 1> const&)
. This is a problem.
A solution would be to ensure operator=
can only be called with another matrix of the right size:
template <typename T, size_t r, size_t c> //r=rows,c=cols of the Matrix
class Matrix {
public:
template <size_t L>
Matrix<T, r, L> operator*(Matrix<T, c, L> const& other) const;
Matrix &operator=(Matrix const& other);
// ...
};
You could even allow type conversions:
template<class U>
Matrix &operator=(Matrix<U, r, c> const& other);
Finally, you might want to use auto
:
Matrix<double, 1, 4> m1(1.2);
Matrix<double, 4, 1> m2(1.2);
auto m3 = m1 * m2; // m3 is Matrix<double, 1, 1>
From your declaration
template <typename T, size_t r, size_t c> //r=rows,c=cols of the Matrix
class Matrix {
public:
template <size_t L>
Matrix<T, r, L> operator*(const Matrix<T, c, L> &other) const;
template <size_t r, size_t c> // BEWARE: shadowing
Matrix &operator=(const Matrix<T, r, c> &other);
// ...
};
we can guess what happens.
Matrix<double, 1, 4> m1(1.2);
Matrix<double, 4, 1> m2(1.2);
m1 * m2; // (1)
(1)
calls Matrix<double, 1, 4>::operator*<1>(Matrix<double, 4, 1> const&)
. It result has then type Matrix<double, 1, 1>
.
Matrix<double, 2, 2> m3;
m3 = /* (2) */ m1 * m2;
(2)
calls Matrix<double, 2, 2>::operator=<1, 1>(Matrix<double, 1, 1> const&)
. This is a problem.
A solution would be to ensure operator=
can only be called with another matrix of the right size:
template <typename T, size_t r, size_t c> //r=rows,c=cols of the Matrix
class Matrix {
public:
template <size_t L>
Matrix<T, r, L> operator*(Matrix<T, c, L> const& other) const;
Matrix &operator=(Matrix const& other);
// ...
};
You could even allow type conversions:
template<class U>
Matrix &operator=(Matrix<U, r, c> const& other);
Finally, you might want to use auto
:
Matrix<double, 1, 4> m1(1.2);
Matrix<double, 4, 1> m2(1.2);
auto m3 = m1 * m2; // m3 is Matrix<double, 1, 1>
answered Nov 14 '18 at 13:12


YSCYSC
23.2k352106
23.2k352106
That is basically spot on. I like your suggestion to change the =operator and check, if the assignment is valid. Maybe I did view the problem from the wrong angle: If I create a Matrix likem3
, then I already have to know, what it has to look like and what it's going to do. To me it looks now, I cannot work around the auto OR specific in advance what I expect that is going to happen - how the matrix looks like.
– Imago
Nov 14 '18 at 13:23
add a comment |
That is basically spot on. I like your suggestion to change the =operator and check, if the assignment is valid. Maybe I did view the problem from the wrong angle: If I create a Matrix likem3
, then I already have to know, what it has to look like and what it's going to do. To me it looks now, I cannot work around the auto OR specific in advance what I expect that is going to happen - how the matrix looks like.
– Imago
Nov 14 '18 at 13:23
That is basically spot on. I like your suggestion to change the =operator and check, if the assignment is valid. Maybe I did view the problem from the wrong angle: If I create a Matrix like
m3
, then I already have to know, what it has to look like and what it's going to do. To me it looks now, I cannot work around the auto OR specific in advance what I expect that is going to happen - how the matrix looks like.– Imago
Nov 14 '18 at 13:23
That is basically spot on. I like your suggestion to change the =operator and check, if the assignment is valid. Maybe I did view the problem from the wrong angle: If I create a Matrix like
m3
, then I already have to know, what it has to look like and what it's going to do. To me it looks now, I cannot work around the auto OR specific in advance what I expect that is going to happen - how the matrix looks like.– Imago
Nov 14 '18 at 13:23
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53300651%2fcan-one-change-templates-arguments%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
L8BBZ WiU,nVYSq,lvawNU4X2kCR,x,dlSW33hiMv6px9lY9
1
This is pretty confusing. What exactly did you expect
m3
to do? Also isMatrix
self written or fromEigen
?– Fantastic Mr Fox
Nov 14 '18 at 12:51
2
what does the operator * look like?
– UKMonkey
Nov 14 '18 at 12:52
2
There is no way in normal matrix operations for a 1x4 matrix multiplied by a 4x1 matrix to give a 2x2 matrix. It will (depending on order of operations) give either a 4x4 or a 1x1. Given a 4x4 matrix, I guess you could specify a transformation to a 2x2.
– Peter
Nov 14 '18 at 12:55
2
Storing size information in the type is a very good idea. You just have to adopt initialization and
auto
instead of pre-declaring your variables. Alternatively, you can usedecltype(m1 * m2)
.– Quentin
Nov 14 '18 at 12:57
1
Okay not quite so ludicrous on second read. You could basically create a function to reshape the matrix (you may want two varieties: row-major and column-major). That can be templated and would return an appropriate type to match the assignment.
– paddy
Nov 14 '18 at 13:03