Git add through python subprocess
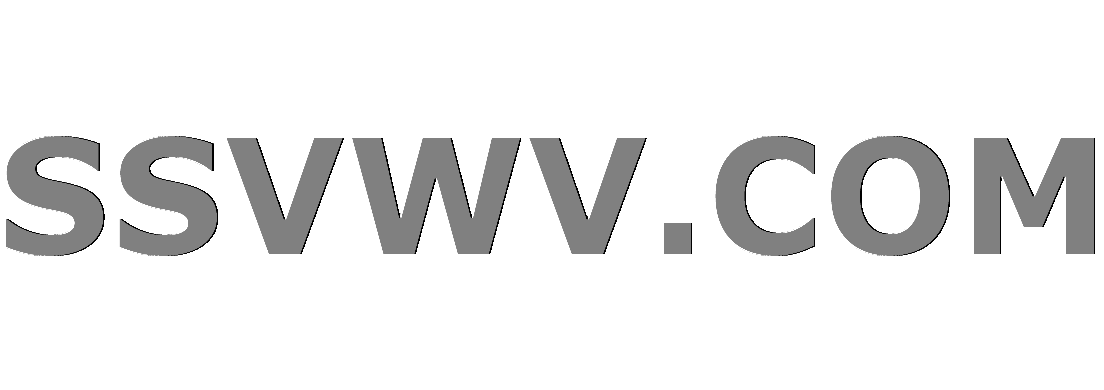
Multi tool use
I am trying to run git commands through python subprocess. I do this by calling the git.exe in the cmd directory of github.
I managed to get most commands working (init, remote, status) but i get an error when calling git add. This is my code so far:
import subprocess
gitPath = 'C:/path/to/git/cmd.exe'
repoPath = 'C:/path/to/my/repo'
repoUrl = 'https://www.github.com/login/repo';
#list to set directory and working tree
dirList = ['--git-dir='+repoPath+'/.git','--work-tree='+repoPath]
#init gitt
subprocess.call([gitPath] + ['init',repoPath]
#add remote
subprocess.call([gitPath] + dirList + ['remote','add','origin',repoUrl])
#Check status, returns files to be commited etc, so a working repo exists there
subprocess.call([gitPath] + dirList + ['status'])
#Adds all files in folder (this returns the error)
subprocess.call([gitPath] + dirList + ['add','.']
The error i get is:
fatal: Not a git repository (or any of the parent directories): .git
So i searched for this error, and most solutions i found were about not being in the right directory. So my guess would also be that. However, i do not know why. Git status returns the correct files in the directory, and i have set --git-dir and --work-tree
If i go to git shell i have no problem adding files, but i cannot find out why things go wrong here.
I am not looking for a solution using pythons git library.
python git github cmd subprocess
add a comment |
I am trying to run git commands through python subprocess. I do this by calling the git.exe in the cmd directory of github.
I managed to get most commands working (init, remote, status) but i get an error when calling git add. This is my code so far:
import subprocess
gitPath = 'C:/path/to/git/cmd.exe'
repoPath = 'C:/path/to/my/repo'
repoUrl = 'https://www.github.com/login/repo';
#list to set directory and working tree
dirList = ['--git-dir='+repoPath+'/.git','--work-tree='+repoPath]
#init gitt
subprocess.call([gitPath] + ['init',repoPath]
#add remote
subprocess.call([gitPath] + dirList + ['remote','add','origin',repoUrl])
#Check status, returns files to be commited etc, so a working repo exists there
subprocess.call([gitPath] + dirList + ['status'])
#Adds all files in folder (this returns the error)
subprocess.call([gitPath] + dirList + ['add','.']
The error i get is:
fatal: Not a git repository (or any of the parent directories): .git
So i searched for this error, and most solutions i found were about not being in the right directory. So my guess would also be that. However, i do not know why. Git status returns the correct files in the directory, and i have set --git-dir and --work-tree
If i go to git shell i have no problem adding files, but i cannot find out why things go wrong here.
I am not looking for a solution using pythons git library.
python git github cmd subprocess
add a comment |
I am trying to run git commands through python subprocess. I do this by calling the git.exe in the cmd directory of github.
I managed to get most commands working (init, remote, status) but i get an error when calling git add. This is my code so far:
import subprocess
gitPath = 'C:/path/to/git/cmd.exe'
repoPath = 'C:/path/to/my/repo'
repoUrl = 'https://www.github.com/login/repo';
#list to set directory and working tree
dirList = ['--git-dir='+repoPath+'/.git','--work-tree='+repoPath]
#init gitt
subprocess.call([gitPath] + ['init',repoPath]
#add remote
subprocess.call([gitPath] + dirList + ['remote','add','origin',repoUrl])
#Check status, returns files to be commited etc, so a working repo exists there
subprocess.call([gitPath] + dirList + ['status'])
#Adds all files in folder (this returns the error)
subprocess.call([gitPath] + dirList + ['add','.']
The error i get is:
fatal: Not a git repository (or any of the parent directories): .git
So i searched for this error, and most solutions i found were about not being in the right directory. So my guess would also be that. However, i do not know why. Git status returns the correct files in the directory, and i have set --git-dir and --work-tree
If i go to git shell i have no problem adding files, but i cannot find out why things go wrong here.
I am not looking for a solution using pythons git library.
python git github cmd subprocess
I am trying to run git commands through python subprocess. I do this by calling the git.exe in the cmd directory of github.
I managed to get most commands working (init, remote, status) but i get an error when calling git add. This is my code so far:
import subprocess
gitPath = 'C:/path/to/git/cmd.exe'
repoPath = 'C:/path/to/my/repo'
repoUrl = 'https://www.github.com/login/repo';
#list to set directory and working tree
dirList = ['--git-dir='+repoPath+'/.git','--work-tree='+repoPath]
#init gitt
subprocess.call([gitPath] + ['init',repoPath]
#add remote
subprocess.call([gitPath] + dirList + ['remote','add','origin',repoUrl])
#Check status, returns files to be commited etc, so a working repo exists there
subprocess.call([gitPath] + dirList + ['status'])
#Adds all files in folder (this returns the error)
subprocess.call([gitPath] + dirList + ['add','.']
The error i get is:
fatal: Not a git repository (or any of the parent directories): .git
So i searched for this error, and most solutions i found were about not being in the right directory. So my guess would also be that. However, i do not know why. Git status returns the correct files in the directory, and i have set --git-dir and --work-tree
If i go to git shell i have no problem adding files, but i cannot find out why things go wrong here.
I am not looking for a solution using pythons git library.
python git github cmd subprocess
python git github cmd subprocess
asked Aug 1 '15 at 22:03
user4493177user4493177
283319
283319
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
You need to specify the working directory.
Functions Popen
, call
, check_call
, and check_output
have a cwd
keyword argument to do so, e.g.:
subprocess.call([gitPath] + dirList + ['add','.'], cwd='/home/me/workdir')
See also Specify working directory for popen
add a comment |
Other than using cwd
Popen's argument, you could also use git's flag -C
:
usage: git [--version] [--help] [-C <path>] [-c name=value]
[--exec-path[=<path>]] [--html-path] [--man-path] [--info-path]
[-p|--paginate|--no-pager] [--no-replace-objects] [--bare]
[--git-dir=<path>] [--work-tree=<path>] [--namespace=<name>]
<command> [<args>]
So that it should be something like
subprocess.Popen('git -C <path>'...)
add a comment |
In Python 2 this works for me.
import subprocess
subprocess.Popen(['git', '--git-dir', '/path/.git', '--work-tree', '/work/dir', 'add', '/that/you/add/file'])
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f31766655%2fgit-add-through-python-subprocess%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
You need to specify the working directory.
Functions Popen
, call
, check_call
, and check_output
have a cwd
keyword argument to do so, e.g.:
subprocess.call([gitPath] + dirList + ['add','.'], cwd='/home/me/workdir')
See also Specify working directory for popen
add a comment |
You need to specify the working directory.
Functions Popen
, call
, check_call
, and check_output
have a cwd
keyword argument to do so, e.g.:
subprocess.call([gitPath] + dirList + ['add','.'], cwd='/home/me/workdir')
See also Specify working directory for popen
add a comment |
You need to specify the working directory.
Functions Popen
, call
, check_call
, and check_output
have a cwd
keyword argument to do so, e.g.:
subprocess.call([gitPath] + dirList + ['add','.'], cwd='/home/me/workdir')
See also Specify working directory for popen
You need to specify the working directory.
Functions Popen
, call
, check_call
, and check_output
have a cwd
keyword argument to do so, e.g.:
subprocess.call([gitPath] + dirList + ['add','.'], cwd='/home/me/workdir')
See also Specify working directory for popen
edited Nov 14 '18 at 18:10
answered Aug 1 '15 at 22:25
fferrifferri
11.7k32251
11.7k32251
add a comment |
add a comment |
Other than using cwd
Popen's argument, you could also use git's flag -C
:
usage: git [--version] [--help] [-C <path>] [-c name=value]
[--exec-path[=<path>]] [--html-path] [--man-path] [--info-path]
[-p|--paginate|--no-pager] [--no-replace-objects] [--bare]
[--git-dir=<path>] [--work-tree=<path>] [--namespace=<name>]
<command> [<args>]
So that it should be something like
subprocess.Popen('git -C <path>'...)
add a comment |
Other than using cwd
Popen's argument, you could also use git's flag -C
:
usage: git [--version] [--help] [-C <path>] [-c name=value]
[--exec-path[=<path>]] [--html-path] [--man-path] [--info-path]
[-p|--paginate|--no-pager] [--no-replace-objects] [--bare]
[--git-dir=<path>] [--work-tree=<path>] [--namespace=<name>]
<command> [<args>]
So that it should be something like
subprocess.Popen('git -C <path>'...)
add a comment |
Other than using cwd
Popen's argument, you could also use git's flag -C
:
usage: git [--version] [--help] [-C <path>] [-c name=value]
[--exec-path[=<path>]] [--html-path] [--man-path] [--info-path]
[-p|--paginate|--no-pager] [--no-replace-objects] [--bare]
[--git-dir=<path>] [--work-tree=<path>] [--namespace=<name>]
<command> [<args>]
So that it should be something like
subprocess.Popen('git -C <path>'...)
Other than using cwd
Popen's argument, you could also use git's flag -C
:
usage: git [--version] [--help] [-C <path>] [-c name=value]
[--exec-path[=<path>]] [--html-path] [--man-path] [--info-path]
[-p|--paginate|--no-pager] [--no-replace-objects] [--bare]
[--git-dir=<path>] [--work-tree=<path>] [--namespace=<name>]
<command> [<args>]
So that it should be something like
subprocess.Popen('git -C <path>'...)
answered Aug 1 '15 at 22:31
Carlo LobranoCarlo Lobrano
1837
1837
add a comment |
add a comment |
In Python 2 this works for me.
import subprocess
subprocess.Popen(['git', '--git-dir', '/path/.git', '--work-tree', '/work/dir', 'add', '/that/you/add/file'])
add a comment |
In Python 2 this works for me.
import subprocess
subprocess.Popen(['git', '--git-dir', '/path/.git', '--work-tree', '/work/dir', 'add', '/that/you/add/file'])
add a comment |
In Python 2 this works for me.
import subprocess
subprocess.Popen(['git', '--git-dir', '/path/.git', '--work-tree', '/work/dir', 'add', '/that/you/add/file'])
In Python 2 this works for me.
import subprocess
subprocess.Popen(['git', '--git-dir', '/path/.git', '--work-tree', '/work/dir', 'add', '/that/you/add/file'])
answered Jun 9 '16 at 22:54


alemolalemol
4,06611624
4,06611624
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f31766655%2fgit-add-through-python-subprocess%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
8L4n3zA1xNbqpf5,Aozi