Select image and gif from Camera roll
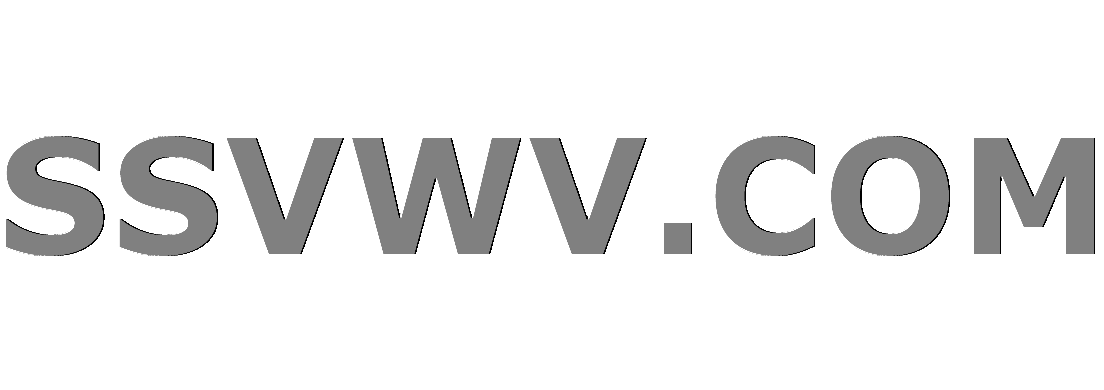
Multi tool use
I'm building an Ionic app with Angular and Firebase.
I want to be able to upload an image and gif to my firebase database, but I've only been able to get image
to work. Also, I don't want videos.
My code is as follows:
takePhoto(sourceType:number) {
const options: CameraOptions = {
quality: 40,
destinationType: this.camera.DestinationType.DATA_URL,
encodingType: this.camera.EncodingType.JPEG,
mediaType: this.camera.MediaType.PICTURE,
correctOrientation: true,
sourceType:sourceType,
}
this.camera.getPicture(options).then((imageData) => {
let base64Image = 'data:image/jpeg;base64,' + imageData;
this.uploadToStorage(base64Image);
}, (err) => {
// Handle error
});
}
uploadToStorage(src) {
this.uploadProgress = true;
let storageRef = firebase.storage().ref();
// Create a timestamp as filename
this.imageFileName = Math.floor(Date.now() / 1000) + "_" + this.userData.uid;
// Create a reference to 'images/todays-date.jpg'
const imageRef = storageRef.child('posts/'+this.imageFileName+'.jpg');
imageRef.putString(src, firebase.storage.StringFormat.DATA_URL).then((snapshot)=> {
snapshot.ref.getDownloadURL().then(downloadURL => {
this.imageURL = downloadURL;
this.uploadProgress = false;
this.uploadSuccess = true;
console.log(this.imageURL)
this.logEvent("Uploaded Image");
});
}, (err) => {
console.log(err)
});
}
But this only allows still images. According to the docs for the Ionic Camera
you can change mediaType: this.camera.MediaType.PICTURE
to mediaType: this.camera.MediaType.ALLMEDIA
but that doesn't work for me. It works when I'm testing on my computer, but not on iOS or Android.
Any ideas how I can allow images and gifs from being selected? Thank you!
javascript cordova ionic-framework ionic2 ionic3
add a comment |
I'm building an Ionic app with Angular and Firebase.
I want to be able to upload an image and gif to my firebase database, but I've only been able to get image
to work. Also, I don't want videos.
My code is as follows:
takePhoto(sourceType:number) {
const options: CameraOptions = {
quality: 40,
destinationType: this.camera.DestinationType.DATA_URL,
encodingType: this.camera.EncodingType.JPEG,
mediaType: this.camera.MediaType.PICTURE,
correctOrientation: true,
sourceType:sourceType,
}
this.camera.getPicture(options).then((imageData) => {
let base64Image = 'data:image/jpeg;base64,' + imageData;
this.uploadToStorage(base64Image);
}, (err) => {
// Handle error
});
}
uploadToStorage(src) {
this.uploadProgress = true;
let storageRef = firebase.storage().ref();
// Create a timestamp as filename
this.imageFileName = Math.floor(Date.now() / 1000) + "_" + this.userData.uid;
// Create a reference to 'images/todays-date.jpg'
const imageRef = storageRef.child('posts/'+this.imageFileName+'.jpg');
imageRef.putString(src, firebase.storage.StringFormat.DATA_URL).then((snapshot)=> {
snapshot.ref.getDownloadURL().then(downloadURL => {
this.imageURL = downloadURL;
this.uploadProgress = false;
this.uploadSuccess = true;
console.log(this.imageURL)
this.logEvent("Uploaded Image");
});
}, (err) => {
console.log(err)
});
}
But this only allows still images. According to the docs for the Ionic Camera
you can change mediaType: this.camera.MediaType.PICTURE
to mediaType: this.camera.MediaType.ALLMEDIA
but that doesn't work for me. It works when I'm testing on my computer, but not on iOS or Android.
Any ideas how I can allow images and gifs from being selected? Thank you!
javascript cordova ionic-framework ionic2 ionic3
Your problem is, your not able choose gif images ? if so, just confirm me, what are the files, that you are able to choose.
– Vasanth
Nov 14 '18 at 10:15
@Vasanth exactly, I can't select gifs. I can select regular images like png and jpeg. If I change mediaType toALLMEDIA
I can select gif, but it doesn't work on android, and I don't want to be able to select video...
– Jane Doe
Nov 14 '18 at 16:31
When you set it to ALLMEDIA, is correct gif file is chosen ? by logging you can check it. If that is working fine, then I will tell you a method to upload the files.
– Vasanth
Nov 15 '18 at 5:17
@Vasanth yes that's fine, but on Android it doesn't let you select any file.. only on iOS it works.
– Jane Doe
Nov 15 '18 at 15:11
If you just want to choose the file from mobile device and don't want take image from camera, then use ionicframework.com/docs/native/file-chooser. That would very straight and all your problems will be solved.
– Vasanth
Nov 16 '18 at 5:33
add a comment |
I'm building an Ionic app with Angular and Firebase.
I want to be able to upload an image and gif to my firebase database, but I've only been able to get image
to work. Also, I don't want videos.
My code is as follows:
takePhoto(sourceType:number) {
const options: CameraOptions = {
quality: 40,
destinationType: this.camera.DestinationType.DATA_URL,
encodingType: this.camera.EncodingType.JPEG,
mediaType: this.camera.MediaType.PICTURE,
correctOrientation: true,
sourceType:sourceType,
}
this.camera.getPicture(options).then((imageData) => {
let base64Image = 'data:image/jpeg;base64,' + imageData;
this.uploadToStorage(base64Image);
}, (err) => {
// Handle error
});
}
uploadToStorage(src) {
this.uploadProgress = true;
let storageRef = firebase.storage().ref();
// Create a timestamp as filename
this.imageFileName = Math.floor(Date.now() / 1000) + "_" + this.userData.uid;
// Create a reference to 'images/todays-date.jpg'
const imageRef = storageRef.child('posts/'+this.imageFileName+'.jpg');
imageRef.putString(src, firebase.storage.StringFormat.DATA_URL).then((snapshot)=> {
snapshot.ref.getDownloadURL().then(downloadURL => {
this.imageURL = downloadURL;
this.uploadProgress = false;
this.uploadSuccess = true;
console.log(this.imageURL)
this.logEvent("Uploaded Image");
});
}, (err) => {
console.log(err)
});
}
But this only allows still images. According to the docs for the Ionic Camera
you can change mediaType: this.camera.MediaType.PICTURE
to mediaType: this.camera.MediaType.ALLMEDIA
but that doesn't work for me. It works when I'm testing on my computer, but not on iOS or Android.
Any ideas how I can allow images and gifs from being selected? Thank you!
javascript cordova ionic-framework ionic2 ionic3
I'm building an Ionic app with Angular and Firebase.
I want to be able to upload an image and gif to my firebase database, but I've only been able to get image
to work. Also, I don't want videos.
My code is as follows:
takePhoto(sourceType:number) {
const options: CameraOptions = {
quality: 40,
destinationType: this.camera.DestinationType.DATA_URL,
encodingType: this.camera.EncodingType.JPEG,
mediaType: this.camera.MediaType.PICTURE,
correctOrientation: true,
sourceType:sourceType,
}
this.camera.getPicture(options).then((imageData) => {
let base64Image = 'data:image/jpeg;base64,' + imageData;
this.uploadToStorage(base64Image);
}, (err) => {
// Handle error
});
}
uploadToStorage(src) {
this.uploadProgress = true;
let storageRef = firebase.storage().ref();
// Create a timestamp as filename
this.imageFileName = Math.floor(Date.now() / 1000) + "_" + this.userData.uid;
// Create a reference to 'images/todays-date.jpg'
const imageRef = storageRef.child('posts/'+this.imageFileName+'.jpg');
imageRef.putString(src, firebase.storage.StringFormat.DATA_URL).then((snapshot)=> {
snapshot.ref.getDownloadURL().then(downloadURL => {
this.imageURL = downloadURL;
this.uploadProgress = false;
this.uploadSuccess = true;
console.log(this.imageURL)
this.logEvent("Uploaded Image");
});
}, (err) => {
console.log(err)
});
}
But this only allows still images. According to the docs for the Ionic Camera
you can change mediaType: this.camera.MediaType.PICTURE
to mediaType: this.camera.MediaType.ALLMEDIA
but that doesn't work for me. It works when I'm testing on my computer, but not on iOS or Android.
Any ideas how I can allow images and gifs from being selected? Thank you!
javascript cordova ionic-framework ionic2 ionic3
javascript cordova ionic-framework ionic2 ionic3
asked Nov 14 '18 at 1:50
Jane DoeJane Doe
7181031
7181031
Your problem is, your not able choose gif images ? if so, just confirm me, what are the files, that you are able to choose.
– Vasanth
Nov 14 '18 at 10:15
@Vasanth exactly, I can't select gifs. I can select regular images like png and jpeg. If I change mediaType toALLMEDIA
I can select gif, but it doesn't work on android, and I don't want to be able to select video...
– Jane Doe
Nov 14 '18 at 16:31
When you set it to ALLMEDIA, is correct gif file is chosen ? by logging you can check it. If that is working fine, then I will tell you a method to upload the files.
– Vasanth
Nov 15 '18 at 5:17
@Vasanth yes that's fine, but on Android it doesn't let you select any file.. only on iOS it works.
– Jane Doe
Nov 15 '18 at 15:11
If you just want to choose the file from mobile device and don't want take image from camera, then use ionicframework.com/docs/native/file-chooser. That would very straight and all your problems will be solved.
– Vasanth
Nov 16 '18 at 5:33
add a comment |
Your problem is, your not able choose gif images ? if so, just confirm me, what are the files, that you are able to choose.
– Vasanth
Nov 14 '18 at 10:15
@Vasanth exactly, I can't select gifs. I can select regular images like png and jpeg. If I change mediaType toALLMEDIA
I can select gif, but it doesn't work on android, and I don't want to be able to select video...
– Jane Doe
Nov 14 '18 at 16:31
When you set it to ALLMEDIA, is correct gif file is chosen ? by logging you can check it. If that is working fine, then I will tell you a method to upload the files.
– Vasanth
Nov 15 '18 at 5:17
@Vasanth yes that's fine, but on Android it doesn't let you select any file.. only on iOS it works.
– Jane Doe
Nov 15 '18 at 15:11
If you just want to choose the file from mobile device and don't want take image from camera, then use ionicframework.com/docs/native/file-chooser. That would very straight and all your problems will be solved.
– Vasanth
Nov 16 '18 at 5:33
Your problem is, your not able choose gif images ? if so, just confirm me, what are the files, that you are able to choose.
– Vasanth
Nov 14 '18 at 10:15
Your problem is, your not able choose gif images ? if so, just confirm me, what are the files, that you are able to choose.
– Vasanth
Nov 14 '18 at 10:15
@Vasanth exactly, I can't select gifs. I can select regular images like png and jpeg. If I change mediaType to
ALLMEDIA
I can select gif, but it doesn't work on android, and I don't want to be able to select video...– Jane Doe
Nov 14 '18 at 16:31
@Vasanth exactly, I can't select gifs. I can select regular images like png and jpeg. If I change mediaType to
ALLMEDIA
I can select gif, but it doesn't work on android, and I don't want to be able to select video...– Jane Doe
Nov 14 '18 at 16:31
When you set it to ALLMEDIA, is correct gif file is chosen ? by logging you can check it. If that is working fine, then I will tell you a method to upload the files.
– Vasanth
Nov 15 '18 at 5:17
When you set it to ALLMEDIA, is correct gif file is chosen ? by logging you can check it. If that is working fine, then I will tell you a method to upload the files.
– Vasanth
Nov 15 '18 at 5:17
@Vasanth yes that's fine, but on Android it doesn't let you select any file.. only on iOS it works.
– Jane Doe
Nov 15 '18 at 15:11
@Vasanth yes that's fine, but on Android it doesn't let you select any file.. only on iOS it works.
– Jane Doe
Nov 15 '18 at 15:11
If you just want to choose the file from mobile device and don't want take image from camera, then use ionicframework.com/docs/native/file-chooser. That would very straight and all your problems will be solved.
– Vasanth
Nov 16 '18 at 5:33
If you just want to choose the file from mobile device and don't want take image from camera, then use ionicframework.com/docs/native/file-chooser. That would very straight and all your problems will be solved.
– Vasanth
Nov 16 '18 at 5:33
add a comment |
1 Answer
1
active
oldest
votes
photos ;
OpenGallery(){
console.log("taktit");
const options: CameraOptions = {
quality: 100,
destinationType: this.camera.DestinationType.DATA_URL,
sourceType: this.camera.PictureSourceType.SAVEDPHOTOALBUM
}
this.camera.getPicture(options).then((imageData) => {
// imageData is either a base64 encoded string or a file URI
// If it's base64:
console.log("taktit");
let base64Image = 'data:image/jpeg;base64,' + imageData;
this.photos.push(base64Image);
this.photos.reverse();
}, (err) => {
// Handle error
}); else { }
}
- Now you can uplode your object photos to your firebase
This doesn't work for gifs :(
– Jane Doe
Nov 17 '18 at 20:05
Convert the gif to base64 and use them , see at google " convert gif to base64 whit javascript
– Youssef Dahmane Jdid
Nov 19 '18 at 12:38
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53292062%2fselect-image-and-gif-from-camera-roll%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
photos ;
OpenGallery(){
console.log("taktit");
const options: CameraOptions = {
quality: 100,
destinationType: this.camera.DestinationType.DATA_URL,
sourceType: this.camera.PictureSourceType.SAVEDPHOTOALBUM
}
this.camera.getPicture(options).then((imageData) => {
// imageData is either a base64 encoded string or a file URI
// If it's base64:
console.log("taktit");
let base64Image = 'data:image/jpeg;base64,' + imageData;
this.photos.push(base64Image);
this.photos.reverse();
}, (err) => {
// Handle error
}); else { }
}
- Now you can uplode your object photos to your firebase
This doesn't work for gifs :(
– Jane Doe
Nov 17 '18 at 20:05
Convert the gif to base64 and use them , see at google " convert gif to base64 whit javascript
– Youssef Dahmane Jdid
Nov 19 '18 at 12:38
add a comment |
photos ;
OpenGallery(){
console.log("taktit");
const options: CameraOptions = {
quality: 100,
destinationType: this.camera.DestinationType.DATA_URL,
sourceType: this.camera.PictureSourceType.SAVEDPHOTOALBUM
}
this.camera.getPicture(options).then((imageData) => {
// imageData is either a base64 encoded string or a file URI
// If it's base64:
console.log("taktit");
let base64Image = 'data:image/jpeg;base64,' + imageData;
this.photos.push(base64Image);
this.photos.reverse();
}, (err) => {
// Handle error
}); else { }
}
- Now you can uplode your object photos to your firebase
This doesn't work for gifs :(
– Jane Doe
Nov 17 '18 at 20:05
Convert the gif to base64 and use them , see at google " convert gif to base64 whit javascript
– Youssef Dahmane Jdid
Nov 19 '18 at 12:38
add a comment |
photos ;
OpenGallery(){
console.log("taktit");
const options: CameraOptions = {
quality: 100,
destinationType: this.camera.DestinationType.DATA_URL,
sourceType: this.camera.PictureSourceType.SAVEDPHOTOALBUM
}
this.camera.getPicture(options).then((imageData) => {
// imageData is either a base64 encoded string or a file URI
// If it's base64:
console.log("taktit");
let base64Image = 'data:image/jpeg;base64,' + imageData;
this.photos.push(base64Image);
this.photos.reverse();
}, (err) => {
// Handle error
}); else { }
}
- Now you can uplode your object photos to your firebase
photos ;
OpenGallery(){
console.log("taktit");
const options: CameraOptions = {
quality: 100,
destinationType: this.camera.DestinationType.DATA_URL,
sourceType: this.camera.PictureSourceType.SAVEDPHOTOALBUM
}
this.camera.getPicture(options).then((imageData) => {
// imageData is either a base64 encoded string or a file URI
// If it's base64:
console.log("taktit");
let base64Image = 'data:image/jpeg;base64,' + imageData;
this.photos.push(base64Image);
this.photos.reverse();
}, (err) => {
// Handle error
}); else { }
}
- Now you can uplode your object photos to your firebase
answered Nov 17 '18 at 16:45
Youssef Dahmane JdidYoussef Dahmane Jdid
65
65
This doesn't work for gifs :(
– Jane Doe
Nov 17 '18 at 20:05
Convert the gif to base64 and use them , see at google " convert gif to base64 whit javascript
– Youssef Dahmane Jdid
Nov 19 '18 at 12:38
add a comment |
This doesn't work for gifs :(
– Jane Doe
Nov 17 '18 at 20:05
Convert the gif to base64 and use them , see at google " convert gif to base64 whit javascript
– Youssef Dahmane Jdid
Nov 19 '18 at 12:38
This doesn't work for gifs :(
– Jane Doe
Nov 17 '18 at 20:05
This doesn't work for gifs :(
– Jane Doe
Nov 17 '18 at 20:05
Convert the gif to base64 and use them , see at google " convert gif to base64 whit javascript
– Youssef Dahmane Jdid
Nov 19 '18 at 12:38
Convert the gif to base64 and use them , see at google " convert gif to base64 whit javascript
– Youssef Dahmane Jdid
Nov 19 '18 at 12:38
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53292062%2fselect-image-and-gif-from-camera-roll%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
jStVX3iEeaj J,4X
Your problem is, your not able choose gif images ? if so, just confirm me, what are the files, that you are able to choose.
– Vasanth
Nov 14 '18 at 10:15
@Vasanth exactly, I can't select gifs. I can select regular images like png and jpeg. If I change mediaType to
ALLMEDIA
I can select gif, but it doesn't work on android, and I don't want to be able to select video...– Jane Doe
Nov 14 '18 at 16:31
When you set it to ALLMEDIA, is correct gif file is chosen ? by logging you can check it. If that is working fine, then I will tell you a method to upload the files.
– Vasanth
Nov 15 '18 at 5:17
@Vasanth yes that's fine, but on Android it doesn't let you select any file.. only on iOS it works.
– Jane Doe
Nov 15 '18 at 15:11
If you just want to choose the file from mobile device and don't want take image from camera, then use ionicframework.com/docs/native/file-chooser. That would very straight and all your problems will be solved.
– Vasanth
Nov 16 '18 at 5:33