How to sort output of the find command in a script
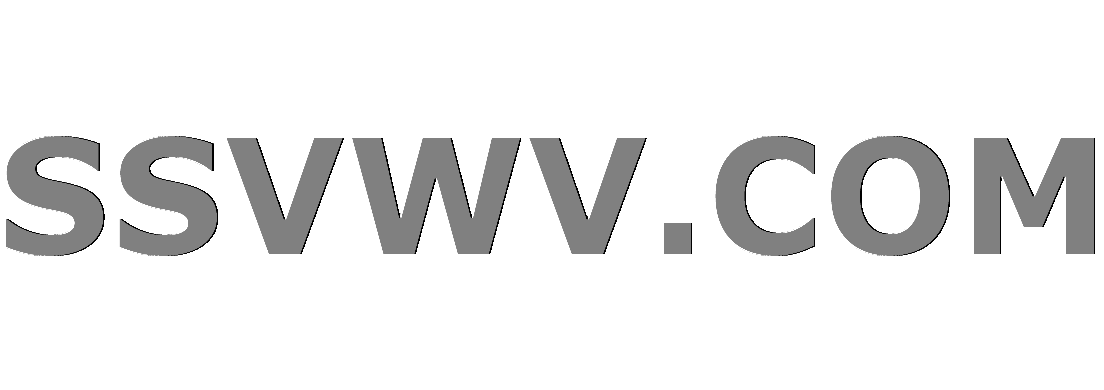
Multi tool use
I have the following simple script to find all directories (at a depth of 2) that were added in the last N days...
#!/bin/bash
DAYS_PRIOR=180
DIR='/mydir'
FILES=`find $DIR -mindepth 2 -maxdepth 2 -type d -mtime -$DAYS_PRIOR -printf '%f\n'`
echo
echo "Files added in the last $DAYS_PRIOR days:"
echo
echo -e $FILES
echo
To get it to add newlines I had to double-escape the printf and use echo -e
. That seems odd to me but it was the only way I could get it to print one directory per line on the output.
Everything works up to this point and I get a list of directories as expected. Now I want to sort the list alphabetically. I tried changing the printf in the find command to...
FILES=`find <xxx> -printf '%f\n' | sort`
however this doesn't sort the directory names. Based on other posts I tried the following..
FILES=`find <xxx> -printf %f\n | sort -t '' | awk -F '' '{print $0; print "\n"}'`
This is very close but leaves an extra space at the start of each line and seems horribly awkward.
Is there a simple method to add a sort to the original find
command?
bash
add a comment |
I have the following simple script to find all directories (at a depth of 2) that were added in the last N days...
#!/bin/bash
DAYS_PRIOR=180
DIR='/mydir'
FILES=`find $DIR -mindepth 2 -maxdepth 2 -type d -mtime -$DAYS_PRIOR -printf '%f\n'`
echo
echo "Files added in the last $DAYS_PRIOR days:"
echo
echo -e $FILES
echo
To get it to add newlines I had to double-escape the printf and use echo -e
. That seems odd to me but it was the only way I could get it to print one directory per line on the output.
Everything works up to this point and I get a list of directories as expected. Now I want to sort the list alphabetically. I tried changing the printf in the find command to...
FILES=`find <xxx> -printf '%f\n' | sort`
however this doesn't sort the directory names. Based on other posts I tried the following..
FILES=`find <xxx> -printf %f\n | sort -t '' | awk -F '' '{print $0; print "\n"}'`
This is very close but leaves an extra space at the start of each line and seems horribly awkward.
Is there a simple method to add a sort to the original find
command?
bash
could you please paste the partial output of first find command?. I don't see issues in my system.
– stack0114106
Nov 14 '18 at 2:05
add a comment |
I have the following simple script to find all directories (at a depth of 2) that were added in the last N days...
#!/bin/bash
DAYS_PRIOR=180
DIR='/mydir'
FILES=`find $DIR -mindepth 2 -maxdepth 2 -type d -mtime -$DAYS_PRIOR -printf '%f\n'`
echo
echo "Files added in the last $DAYS_PRIOR days:"
echo
echo -e $FILES
echo
To get it to add newlines I had to double-escape the printf and use echo -e
. That seems odd to me but it was the only way I could get it to print one directory per line on the output.
Everything works up to this point and I get a list of directories as expected. Now I want to sort the list alphabetically. I tried changing the printf in the find command to...
FILES=`find <xxx> -printf '%f\n' | sort`
however this doesn't sort the directory names. Based on other posts I tried the following..
FILES=`find <xxx> -printf %f\n | sort -t '' | awk -F '' '{print $0; print "\n"}'`
This is very close but leaves an extra space at the start of each line and seems horribly awkward.
Is there a simple method to add a sort to the original find
command?
bash
I have the following simple script to find all directories (at a depth of 2) that were added in the last N days...
#!/bin/bash
DAYS_PRIOR=180
DIR='/mydir'
FILES=`find $DIR -mindepth 2 -maxdepth 2 -type d -mtime -$DAYS_PRIOR -printf '%f\n'`
echo
echo "Files added in the last $DAYS_PRIOR days:"
echo
echo -e $FILES
echo
To get it to add newlines I had to double-escape the printf and use echo -e
. That seems odd to me but it was the only way I could get it to print one directory per line on the output.
Everything works up to this point and I get a list of directories as expected. Now I want to sort the list alphabetically. I tried changing the printf in the find command to...
FILES=`find <xxx> -printf '%f\n' | sort`
however this doesn't sort the directory names. Based on other posts I tried the following..
FILES=`find <xxx> -printf %f\n | sort -t '' | awk -F '' '{print $0; print "\n"}'`
This is very close but leaves an extra space at the start of each line and seems horribly awkward.
Is there a simple method to add a sort to the original find
command?
bash
bash
asked Nov 14 '18 at 1:46
bivouac0bivouac0
1,2381415
1,2381415
could you please paste the partial output of first find command?. I don't see issues in my system.
– stack0114106
Nov 14 '18 at 2:05
add a comment |
could you please paste the partial output of first find command?. I don't see issues in my system.
– stack0114106
Nov 14 '18 at 2:05
could you please paste the partial output of first find command?. I don't see issues in my system.
– stack0114106
Nov 14 '18 at 2:05
could you please paste the partial output of first find command?. I don't see issues in my system.
– stack0114106
Nov 14 '18 at 2:05
add a comment |
1 Answer
1
active
oldest
votes
First: double-quote your variable references! When you use echo -e $FILES
, the variable FILE
's value gets split into "words" based on whitespace (spaces, tabs, and newlines), and then echo
sticks those words back together with spaces between them. This has the effect of converting newlines into spaces. In order to wind up with newlines at the end, you're having to use n
instead of a true newline, and use echo -e
to convert it. Just use real newlines, and put double-quotes around the variable reference to avoid all this mess:
FILES=$(find "$DIR" -mindepth 2 -maxdepth 2 -type d -mtime "-$DAYS_PRIOR" -printf '%fn')
# ...
echo "$FILES"
Note that I put double-quotes around all variable references, since this is almost always a good idea. I also used $( )
instead of backticks -- it's easier to read, and avoids some parsing oddities that backticks have.
Anyway, with this format you're using proper newlines throughout, so piping through sort
should work as expected.
BTW, I'd also recommend switching from uppercase variable names to lower- or mixed-case names, since there are a bunch of all-caps names that have special meanings, and if you accidentally use one of them bad things can happen.
Thanks for the suggestions, but on my system (Ubuntu 18.04) the above prints all directories on the same line, no newline in the output. If I add sort to it, the output is correctly sorted but everything is still on the same line. I also tried this with the double-escape of the -printf %fn but that doesn't add newlines either.
– bivouac0
Nov 14 '18 at 2:36
Oops. Ignore the above comment, I missed something. This does work. Thanks for the input.
– bivouac0
Nov 14 '18 at 2:40
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53292024%2fhow-to-sort-output-of-the-find-command-in-a-script%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
First: double-quote your variable references! When you use echo -e $FILES
, the variable FILE
's value gets split into "words" based on whitespace (spaces, tabs, and newlines), and then echo
sticks those words back together with spaces between them. This has the effect of converting newlines into spaces. In order to wind up with newlines at the end, you're having to use n
instead of a true newline, and use echo -e
to convert it. Just use real newlines, and put double-quotes around the variable reference to avoid all this mess:
FILES=$(find "$DIR" -mindepth 2 -maxdepth 2 -type d -mtime "-$DAYS_PRIOR" -printf '%fn')
# ...
echo "$FILES"
Note that I put double-quotes around all variable references, since this is almost always a good idea. I also used $( )
instead of backticks -- it's easier to read, and avoids some parsing oddities that backticks have.
Anyway, with this format you're using proper newlines throughout, so piping through sort
should work as expected.
BTW, I'd also recommend switching from uppercase variable names to lower- or mixed-case names, since there are a bunch of all-caps names that have special meanings, and if you accidentally use one of them bad things can happen.
Thanks for the suggestions, but on my system (Ubuntu 18.04) the above prints all directories on the same line, no newline in the output. If I add sort to it, the output is correctly sorted but everything is still on the same line. I also tried this with the double-escape of the -printf %fn but that doesn't add newlines either.
– bivouac0
Nov 14 '18 at 2:36
Oops. Ignore the above comment, I missed something. This does work. Thanks for the input.
– bivouac0
Nov 14 '18 at 2:40
add a comment |
First: double-quote your variable references! When you use echo -e $FILES
, the variable FILE
's value gets split into "words" based on whitespace (spaces, tabs, and newlines), and then echo
sticks those words back together with spaces between them. This has the effect of converting newlines into spaces. In order to wind up with newlines at the end, you're having to use n
instead of a true newline, and use echo -e
to convert it. Just use real newlines, and put double-quotes around the variable reference to avoid all this mess:
FILES=$(find "$DIR" -mindepth 2 -maxdepth 2 -type d -mtime "-$DAYS_PRIOR" -printf '%fn')
# ...
echo "$FILES"
Note that I put double-quotes around all variable references, since this is almost always a good idea. I also used $( )
instead of backticks -- it's easier to read, and avoids some parsing oddities that backticks have.
Anyway, with this format you're using proper newlines throughout, so piping through sort
should work as expected.
BTW, I'd also recommend switching from uppercase variable names to lower- or mixed-case names, since there are a bunch of all-caps names that have special meanings, and if you accidentally use one of them bad things can happen.
Thanks for the suggestions, but on my system (Ubuntu 18.04) the above prints all directories on the same line, no newline in the output. If I add sort to it, the output is correctly sorted but everything is still on the same line. I also tried this with the double-escape of the -printf %fn but that doesn't add newlines either.
– bivouac0
Nov 14 '18 at 2:36
Oops. Ignore the above comment, I missed something. This does work. Thanks for the input.
– bivouac0
Nov 14 '18 at 2:40
add a comment |
First: double-quote your variable references! When you use echo -e $FILES
, the variable FILE
's value gets split into "words" based on whitespace (spaces, tabs, and newlines), and then echo
sticks those words back together with spaces between them. This has the effect of converting newlines into spaces. In order to wind up with newlines at the end, you're having to use n
instead of a true newline, and use echo -e
to convert it. Just use real newlines, and put double-quotes around the variable reference to avoid all this mess:
FILES=$(find "$DIR" -mindepth 2 -maxdepth 2 -type d -mtime "-$DAYS_PRIOR" -printf '%fn')
# ...
echo "$FILES"
Note that I put double-quotes around all variable references, since this is almost always a good idea. I also used $( )
instead of backticks -- it's easier to read, and avoids some parsing oddities that backticks have.
Anyway, with this format you're using proper newlines throughout, so piping through sort
should work as expected.
BTW, I'd also recommend switching from uppercase variable names to lower- or mixed-case names, since there are a bunch of all-caps names that have special meanings, and if you accidentally use one of them bad things can happen.
First: double-quote your variable references! When you use echo -e $FILES
, the variable FILE
's value gets split into "words" based on whitespace (spaces, tabs, and newlines), and then echo
sticks those words back together with spaces between them. This has the effect of converting newlines into spaces. In order to wind up with newlines at the end, you're having to use n
instead of a true newline, and use echo -e
to convert it. Just use real newlines, and put double-quotes around the variable reference to avoid all this mess:
FILES=$(find "$DIR" -mindepth 2 -maxdepth 2 -type d -mtime "-$DAYS_PRIOR" -printf '%fn')
# ...
echo "$FILES"
Note that I put double-quotes around all variable references, since this is almost always a good idea. I also used $( )
instead of backticks -- it's easier to read, and avoids some parsing oddities that backticks have.
Anyway, with this format you're using proper newlines throughout, so piping through sort
should work as expected.
BTW, I'd also recommend switching from uppercase variable names to lower- or mixed-case names, since there are a bunch of all-caps names that have special meanings, and if you accidentally use one of them bad things can happen.
edited Nov 14 '18 at 3:09
answered Nov 14 '18 at 2:19
Gordon DavissonGordon Davisson
68.4k97894
68.4k97894
Thanks for the suggestions, but on my system (Ubuntu 18.04) the above prints all directories on the same line, no newline in the output. If I add sort to it, the output is correctly sorted but everything is still on the same line. I also tried this with the double-escape of the -printf %fn but that doesn't add newlines either.
– bivouac0
Nov 14 '18 at 2:36
Oops. Ignore the above comment, I missed something. This does work. Thanks for the input.
– bivouac0
Nov 14 '18 at 2:40
add a comment |
Thanks for the suggestions, but on my system (Ubuntu 18.04) the above prints all directories on the same line, no newline in the output. If I add sort to it, the output is correctly sorted but everything is still on the same line. I also tried this with the double-escape of the -printf %fn but that doesn't add newlines either.
– bivouac0
Nov 14 '18 at 2:36
Oops. Ignore the above comment, I missed something. This does work. Thanks for the input.
– bivouac0
Nov 14 '18 at 2:40
Thanks for the suggestions, but on my system (Ubuntu 18.04) the above prints all directories on the same line, no newline in the output. If I add sort to it, the output is correctly sorted but everything is still on the same line. I also tried this with the double-escape of the -printf %fn but that doesn't add newlines either.
– bivouac0
Nov 14 '18 at 2:36
Thanks for the suggestions, but on my system (Ubuntu 18.04) the above prints all directories on the same line, no newline in the output. If I add sort to it, the output is correctly sorted but everything is still on the same line. I also tried this with the double-escape of the -printf %fn but that doesn't add newlines either.
– bivouac0
Nov 14 '18 at 2:36
Oops. Ignore the above comment, I missed something. This does work. Thanks for the input.
– bivouac0
Nov 14 '18 at 2:40
Oops. Ignore the above comment, I missed something. This does work. Thanks for the input.
– bivouac0
Nov 14 '18 at 2:40
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53292024%2fhow-to-sort-output-of-the-find-command-in-a-script%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
f2ffI N,f8X
could you please paste the partial output of first find command?. I don't see issues in my system.
– stack0114106
Nov 14 '18 at 2:05