how do you create jumping in pygame for a sprite
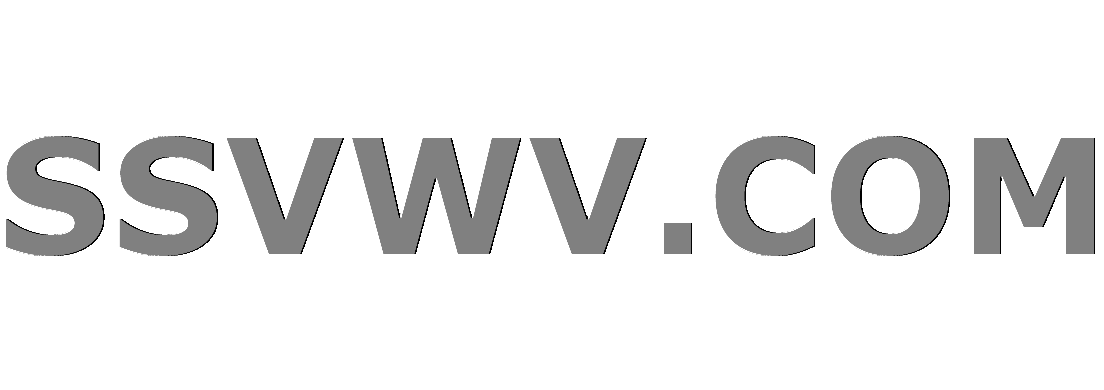
Multi tool use
I am struggling with finding a way to make the sprite jump in pygame. when i basically run the program now the sprite goes straight to the top of the screen and i just want it to do a normal jump. the jump is in a class and is a function. I have used KEYDOWN
to check when its pressed to move it downwards.
import pygame
import random
import sys
pygame.init()
screen = pygame.display.set_mode((1280, 720))
clock = pygame.time.Clock() # A clock to limit the frame rate.
pygame.display.set_caption("this game")
class Background:
picture = pygame.image.load("C:/images/dunes.jpg").convert()
picture = pygame.transform.scale(picture, (1280, 720))
def __init__(self, x, y):
self.xpos = x
self.ypos = y
def draw(self):
# Blit the picture onto the screen surface.
# `self.picture` not just `picture`.
screen.blit(self.picture, (self.xpos, self.ypos))
class Monster:
picture = pygame.image.load("C:/pics/hammerhood.png").convert_alpha()
picture = pygame.transform.scale(picture, (200, 200))
def __init__(self, x, y):
self.xpos = x
self.ypos = y
# If you want to move continuously you need to set these
# attributes to the desired speed and then add them to
# self.xpos and self.ypos in an update method that should
# be called once each frame.
self.speed_x = 0
self.speed_y = 0
def update(self):
# Call this method each frame to update the positions.
self.xpos += self.speed_x
self.ypos += self.speed_y
# Not necessary anymore.
def move_left(self):
self.xpos -= 5 # -= not = -5 (augmented assignment).
def move_right(self):
self.xpos += 5 # += not = +5 (augmented assignment).
def jump(self): #vvvvvvv this is the part i do not know how to fix
# What do you want to do here?
#for x in range(1, 10):
#self.ypos -= 1 # -= not =-
# pygame.display.show() # There's no show method.
#for x in range(1, 10):
#self.ypos += 1
# pygame.display.show()
def draw(self):
screen.blit(self.picture, (self.xpos, self.ypos))
class Enemy: # Use upper camelcase names for classes.
picture = pygame.image.load("C:/pics/dangler_fish.png").convert_alpha()
picture = pygame.transform.scale(picture, (200, 200))
def __init__(self, x, y):
self.xpos = x
self.ypos = y
def teleport(self):
self.xpos = random.randint(1, 1280)
self.ypos= random.randint(1, 720)
def draw(self):
screen.blit(self.picture, (self.xpos, self.ypos))
# Create the instances before the while loop.
ice = Background(0, 0) # I pass 0, 0 so that it fills the whole screen.
hammerhood = Monster(200, 500)
fish = Enemy(0, 0)
while True:
# Handle events.
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# Check if the `event.type` is KEYDOWN first.
elif event.type == pygame.KEYDOWN:
# Then check which `event.key` was pressed.
if event.key == pygame.K_d:
#hammerhood.move_right()
hammerhood.speed_x = 5
elif event.key == pygame.K_a:
#hammerhood.move_left()
hammerhood.speed_x = -5
elif event.key == pygame.K_w:
hammerhood.jump()
elif event.type == pygame.KEYUP:
# Stop moving when the keys are released.
if event.key == pygame.K_d and hammerhood.speed_x > 0:
hammerhood.speed_x = 0
elif event.key == pygame.K_a and hammerhood.speed_x < 0:
hammerhood.speed_x = 0
# Update the game.
hammerhood.update()
fish.teleport()
if hammerhood.xpos == 1280 or hammerhood.xpos == 0:
hammerhood.speed_x = 0
# Draw everything.
ice.draw() # Blit the background to clear the screen.
hammerhood.draw()
fish.draw()
pygame.display.flip()
clock.tick(60) # Limit the frame rate to 60 FPS.
python pygame sprite
add a comment |
I am struggling with finding a way to make the sprite jump in pygame. when i basically run the program now the sprite goes straight to the top of the screen and i just want it to do a normal jump. the jump is in a class and is a function. I have used KEYDOWN
to check when its pressed to move it downwards.
import pygame
import random
import sys
pygame.init()
screen = pygame.display.set_mode((1280, 720))
clock = pygame.time.Clock() # A clock to limit the frame rate.
pygame.display.set_caption("this game")
class Background:
picture = pygame.image.load("C:/images/dunes.jpg").convert()
picture = pygame.transform.scale(picture, (1280, 720))
def __init__(self, x, y):
self.xpos = x
self.ypos = y
def draw(self):
# Blit the picture onto the screen surface.
# `self.picture` not just `picture`.
screen.blit(self.picture, (self.xpos, self.ypos))
class Monster:
picture = pygame.image.load("C:/pics/hammerhood.png").convert_alpha()
picture = pygame.transform.scale(picture, (200, 200))
def __init__(self, x, y):
self.xpos = x
self.ypos = y
# If you want to move continuously you need to set these
# attributes to the desired speed and then add them to
# self.xpos and self.ypos in an update method that should
# be called once each frame.
self.speed_x = 0
self.speed_y = 0
def update(self):
# Call this method each frame to update the positions.
self.xpos += self.speed_x
self.ypos += self.speed_y
# Not necessary anymore.
def move_left(self):
self.xpos -= 5 # -= not = -5 (augmented assignment).
def move_right(self):
self.xpos += 5 # += not = +5 (augmented assignment).
def jump(self): #vvvvvvv this is the part i do not know how to fix
# What do you want to do here?
#for x in range(1, 10):
#self.ypos -= 1 # -= not =-
# pygame.display.show() # There's no show method.
#for x in range(1, 10):
#self.ypos += 1
# pygame.display.show()
def draw(self):
screen.blit(self.picture, (self.xpos, self.ypos))
class Enemy: # Use upper camelcase names for classes.
picture = pygame.image.load("C:/pics/dangler_fish.png").convert_alpha()
picture = pygame.transform.scale(picture, (200, 200))
def __init__(self, x, y):
self.xpos = x
self.ypos = y
def teleport(self):
self.xpos = random.randint(1, 1280)
self.ypos= random.randint(1, 720)
def draw(self):
screen.blit(self.picture, (self.xpos, self.ypos))
# Create the instances before the while loop.
ice = Background(0, 0) # I pass 0, 0 so that it fills the whole screen.
hammerhood = Monster(200, 500)
fish = Enemy(0, 0)
while True:
# Handle events.
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# Check if the `event.type` is KEYDOWN first.
elif event.type == pygame.KEYDOWN:
# Then check which `event.key` was pressed.
if event.key == pygame.K_d:
#hammerhood.move_right()
hammerhood.speed_x = 5
elif event.key == pygame.K_a:
#hammerhood.move_left()
hammerhood.speed_x = -5
elif event.key == pygame.K_w:
hammerhood.jump()
elif event.type == pygame.KEYUP:
# Stop moving when the keys are released.
if event.key == pygame.K_d and hammerhood.speed_x > 0:
hammerhood.speed_x = 0
elif event.key == pygame.K_a and hammerhood.speed_x < 0:
hammerhood.speed_x = 0
# Update the game.
hammerhood.update()
fish.teleport()
if hammerhood.xpos == 1280 or hammerhood.xpos == 0:
hammerhood.speed_x = 0
# Draw everything.
ice.draw() # Blit the background to clear the screen.
hammerhood.draw()
fish.draw()
pygame.display.flip()
clock.tick(60) # Limit the frame rate to 60 FPS.
python pygame sprite
add a comment |
I am struggling with finding a way to make the sprite jump in pygame. when i basically run the program now the sprite goes straight to the top of the screen and i just want it to do a normal jump. the jump is in a class and is a function. I have used KEYDOWN
to check when its pressed to move it downwards.
import pygame
import random
import sys
pygame.init()
screen = pygame.display.set_mode((1280, 720))
clock = pygame.time.Clock() # A clock to limit the frame rate.
pygame.display.set_caption("this game")
class Background:
picture = pygame.image.load("C:/images/dunes.jpg").convert()
picture = pygame.transform.scale(picture, (1280, 720))
def __init__(self, x, y):
self.xpos = x
self.ypos = y
def draw(self):
# Blit the picture onto the screen surface.
# `self.picture` not just `picture`.
screen.blit(self.picture, (self.xpos, self.ypos))
class Monster:
picture = pygame.image.load("C:/pics/hammerhood.png").convert_alpha()
picture = pygame.transform.scale(picture, (200, 200))
def __init__(self, x, y):
self.xpos = x
self.ypos = y
# If you want to move continuously you need to set these
# attributes to the desired speed and then add them to
# self.xpos and self.ypos in an update method that should
# be called once each frame.
self.speed_x = 0
self.speed_y = 0
def update(self):
# Call this method each frame to update the positions.
self.xpos += self.speed_x
self.ypos += self.speed_y
# Not necessary anymore.
def move_left(self):
self.xpos -= 5 # -= not = -5 (augmented assignment).
def move_right(self):
self.xpos += 5 # += not = +5 (augmented assignment).
def jump(self): #vvvvvvv this is the part i do not know how to fix
# What do you want to do here?
#for x in range(1, 10):
#self.ypos -= 1 # -= not =-
# pygame.display.show() # There's no show method.
#for x in range(1, 10):
#self.ypos += 1
# pygame.display.show()
def draw(self):
screen.blit(self.picture, (self.xpos, self.ypos))
class Enemy: # Use upper camelcase names for classes.
picture = pygame.image.load("C:/pics/dangler_fish.png").convert_alpha()
picture = pygame.transform.scale(picture, (200, 200))
def __init__(self, x, y):
self.xpos = x
self.ypos = y
def teleport(self):
self.xpos = random.randint(1, 1280)
self.ypos= random.randint(1, 720)
def draw(self):
screen.blit(self.picture, (self.xpos, self.ypos))
# Create the instances before the while loop.
ice = Background(0, 0) # I pass 0, 0 so that it fills the whole screen.
hammerhood = Monster(200, 500)
fish = Enemy(0, 0)
while True:
# Handle events.
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# Check if the `event.type` is KEYDOWN first.
elif event.type == pygame.KEYDOWN:
# Then check which `event.key` was pressed.
if event.key == pygame.K_d:
#hammerhood.move_right()
hammerhood.speed_x = 5
elif event.key == pygame.K_a:
#hammerhood.move_left()
hammerhood.speed_x = -5
elif event.key == pygame.K_w:
hammerhood.jump()
elif event.type == pygame.KEYUP:
# Stop moving when the keys are released.
if event.key == pygame.K_d and hammerhood.speed_x > 0:
hammerhood.speed_x = 0
elif event.key == pygame.K_a and hammerhood.speed_x < 0:
hammerhood.speed_x = 0
# Update the game.
hammerhood.update()
fish.teleport()
if hammerhood.xpos == 1280 or hammerhood.xpos == 0:
hammerhood.speed_x = 0
# Draw everything.
ice.draw() # Blit the background to clear the screen.
hammerhood.draw()
fish.draw()
pygame.display.flip()
clock.tick(60) # Limit the frame rate to 60 FPS.
python pygame sprite
I am struggling with finding a way to make the sprite jump in pygame. when i basically run the program now the sprite goes straight to the top of the screen and i just want it to do a normal jump. the jump is in a class and is a function. I have used KEYDOWN
to check when its pressed to move it downwards.
import pygame
import random
import sys
pygame.init()
screen = pygame.display.set_mode((1280, 720))
clock = pygame.time.Clock() # A clock to limit the frame rate.
pygame.display.set_caption("this game")
class Background:
picture = pygame.image.load("C:/images/dunes.jpg").convert()
picture = pygame.transform.scale(picture, (1280, 720))
def __init__(self, x, y):
self.xpos = x
self.ypos = y
def draw(self):
# Blit the picture onto the screen surface.
# `self.picture` not just `picture`.
screen.blit(self.picture, (self.xpos, self.ypos))
class Monster:
picture = pygame.image.load("C:/pics/hammerhood.png").convert_alpha()
picture = pygame.transform.scale(picture, (200, 200))
def __init__(self, x, y):
self.xpos = x
self.ypos = y
# If you want to move continuously you need to set these
# attributes to the desired speed and then add them to
# self.xpos and self.ypos in an update method that should
# be called once each frame.
self.speed_x = 0
self.speed_y = 0
def update(self):
# Call this method each frame to update the positions.
self.xpos += self.speed_x
self.ypos += self.speed_y
# Not necessary anymore.
def move_left(self):
self.xpos -= 5 # -= not = -5 (augmented assignment).
def move_right(self):
self.xpos += 5 # += not = +5 (augmented assignment).
def jump(self): #vvvvvvv this is the part i do not know how to fix
# What do you want to do here?
#for x in range(1, 10):
#self.ypos -= 1 # -= not =-
# pygame.display.show() # There's no show method.
#for x in range(1, 10):
#self.ypos += 1
# pygame.display.show()
def draw(self):
screen.blit(self.picture, (self.xpos, self.ypos))
class Enemy: # Use upper camelcase names for classes.
picture = pygame.image.load("C:/pics/dangler_fish.png").convert_alpha()
picture = pygame.transform.scale(picture, (200, 200))
def __init__(self, x, y):
self.xpos = x
self.ypos = y
def teleport(self):
self.xpos = random.randint(1, 1280)
self.ypos= random.randint(1, 720)
def draw(self):
screen.blit(self.picture, (self.xpos, self.ypos))
# Create the instances before the while loop.
ice = Background(0, 0) # I pass 0, 0 so that it fills the whole screen.
hammerhood = Monster(200, 500)
fish = Enemy(0, 0)
while True:
# Handle events.
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# Check if the `event.type` is KEYDOWN first.
elif event.type == pygame.KEYDOWN:
# Then check which `event.key` was pressed.
if event.key == pygame.K_d:
#hammerhood.move_right()
hammerhood.speed_x = 5
elif event.key == pygame.K_a:
#hammerhood.move_left()
hammerhood.speed_x = -5
elif event.key == pygame.K_w:
hammerhood.jump()
elif event.type == pygame.KEYUP:
# Stop moving when the keys are released.
if event.key == pygame.K_d and hammerhood.speed_x > 0:
hammerhood.speed_x = 0
elif event.key == pygame.K_a and hammerhood.speed_x < 0:
hammerhood.speed_x = 0
# Update the game.
hammerhood.update()
fish.teleport()
if hammerhood.xpos == 1280 or hammerhood.xpos == 0:
hammerhood.speed_x = 0
# Draw everything.
ice.draw() # Blit the background to clear the screen.
hammerhood.draw()
fish.draw()
pygame.display.flip()
clock.tick(60) # Limit the frame rate to 60 FPS.
python pygame sprite
python pygame sprite
edited Nov 13 '18 at 21:52


skrx
15.6k31834
15.6k31834
asked Nov 13 '18 at 20:44
user10632736
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Just add a GRAVITY
constant and add it to the self.speed_y
each frame to accelerate the player.
GRAVITY = .9 # Define this in the global scope.
# The Monster's update method.
def update(self):
self.speed_y += GRAVITY # Accelerate downwards.
self.xpos += self.speed_x
self.ypos += self.speed_y
# Stop falling at the bottom of the screen.
if self.ypos >= 520:
self.ypos = 520
self.speed_y = 0
self.on_ground = True
Set the self.speed_y
to a negative value when you start to jump:
def jump(self):
if self.on_ground: # This prevents air jumps.
self.on_ground = False
self.speed_y = -25
when it says if self.on_ground is it checking when it was has a Boolean value of true or false
– user10632736
Nov 14 '18 at 20:28
if GRAVITY is .9 (0.9 I am guessing), then if it is misusing 25 that only goes up a small bit, but when i run it it looks like it goes up by about 200 pixels and not 25
– user10632736
Nov 15 '18 at 16:41
TheGRAVITY
andself.speed_y = -25
values are pretty much arbitrary (it looked okay when I tested your game). Setting theself.speed_y
doesn't move the sprite up 25 pixels, it sets the speed that is added to the position every frame. So without theGRAVITY
it would move up 25 pixels all the time, but since theGRAVITY
is added to theself.speed_y
, it accelerates the object a bit more downwards each frame until it starts to fall again.
– skrx
Nov 17 '18 at 11:44
so how does the gravity affect the self.spped_y
– user10632736
Nov 18 '18 at 16:49
The gravity increases the speed each frame. When the speed is positive, the sprite moves downwards, when the speed is negative, the sprite moves upwards.
– skrx
Nov 18 '18 at 16:57
|
show 3 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53289226%2fhow-do-you-create-jumping-in-pygame-for-a-sprite%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Just add a GRAVITY
constant and add it to the self.speed_y
each frame to accelerate the player.
GRAVITY = .9 # Define this in the global scope.
# The Monster's update method.
def update(self):
self.speed_y += GRAVITY # Accelerate downwards.
self.xpos += self.speed_x
self.ypos += self.speed_y
# Stop falling at the bottom of the screen.
if self.ypos >= 520:
self.ypos = 520
self.speed_y = 0
self.on_ground = True
Set the self.speed_y
to a negative value when you start to jump:
def jump(self):
if self.on_ground: # This prevents air jumps.
self.on_ground = False
self.speed_y = -25
when it says if self.on_ground is it checking when it was has a Boolean value of true or false
– user10632736
Nov 14 '18 at 20:28
if GRAVITY is .9 (0.9 I am guessing), then if it is misusing 25 that only goes up a small bit, but when i run it it looks like it goes up by about 200 pixels and not 25
– user10632736
Nov 15 '18 at 16:41
TheGRAVITY
andself.speed_y = -25
values are pretty much arbitrary (it looked okay when I tested your game). Setting theself.speed_y
doesn't move the sprite up 25 pixels, it sets the speed that is added to the position every frame. So without theGRAVITY
it would move up 25 pixels all the time, but since theGRAVITY
is added to theself.speed_y
, it accelerates the object a bit more downwards each frame until it starts to fall again.
– skrx
Nov 17 '18 at 11:44
so how does the gravity affect the self.spped_y
– user10632736
Nov 18 '18 at 16:49
The gravity increases the speed each frame. When the speed is positive, the sprite moves downwards, when the speed is negative, the sprite moves upwards.
– skrx
Nov 18 '18 at 16:57
|
show 3 more comments
Just add a GRAVITY
constant and add it to the self.speed_y
each frame to accelerate the player.
GRAVITY = .9 # Define this in the global scope.
# The Monster's update method.
def update(self):
self.speed_y += GRAVITY # Accelerate downwards.
self.xpos += self.speed_x
self.ypos += self.speed_y
# Stop falling at the bottom of the screen.
if self.ypos >= 520:
self.ypos = 520
self.speed_y = 0
self.on_ground = True
Set the self.speed_y
to a negative value when you start to jump:
def jump(self):
if self.on_ground: # This prevents air jumps.
self.on_ground = False
self.speed_y = -25
when it says if self.on_ground is it checking when it was has a Boolean value of true or false
– user10632736
Nov 14 '18 at 20:28
if GRAVITY is .9 (0.9 I am guessing), then if it is misusing 25 that only goes up a small bit, but when i run it it looks like it goes up by about 200 pixels and not 25
– user10632736
Nov 15 '18 at 16:41
TheGRAVITY
andself.speed_y = -25
values are pretty much arbitrary (it looked okay when I tested your game). Setting theself.speed_y
doesn't move the sprite up 25 pixels, it sets the speed that is added to the position every frame. So without theGRAVITY
it would move up 25 pixels all the time, but since theGRAVITY
is added to theself.speed_y
, it accelerates the object a bit more downwards each frame until it starts to fall again.
– skrx
Nov 17 '18 at 11:44
so how does the gravity affect the self.spped_y
– user10632736
Nov 18 '18 at 16:49
The gravity increases the speed each frame. When the speed is positive, the sprite moves downwards, when the speed is negative, the sprite moves upwards.
– skrx
Nov 18 '18 at 16:57
|
show 3 more comments
Just add a GRAVITY
constant and add it to the self.speed_y
each frame to accelerate the player.
GRAVITY = .9 # Define this in the global scope.
# The Monster's update method.
def update(self):
self.speed_y += GRAVITY # Accelerate downwards.
self.xpos += self.speed_x
self.ypos += self.speed_y
# Stop falling at the bottom of the screen.
if self.ypos >= 520:
self.ypos = 520
self.speed_y = 0
self.on_ground = True
Set the self.speed_y
to a negative value when you start to jump:
def jump(self):
if self.on_ground: # This prevents air jumps.
self.on_ground = False
self.speed_y = -25
Just add a GRAVITY
constant and add it to the self.speed_y
each frame to accelerate the player.
GRAVITY = .9 # Define this in the global scope.
# The Monster's update method.
def update(self):
self.speed_y += GRAVITY # Accelerate downwards.
self.xpos += self.speed_x
self.ypos += self.speed_y
# Stop falling at the bottom of the screen.
if self.ypos >= 520:
self.ypos = 520
self.speed_y = 0
self.on_ground = True
Set the self.speed_y
to a negative value when you start to jump:
def jump(self):
if self.on_ground: # This prevents air jumps.
self.on_ground = False
self.speed_y = -25
edited Nov 13 '18 at 23:27
answered Nov 13 '18 at 22:04


skrxskrx
15.6k31834
15.6k31834
when it says if self.on_ground is it checking when it was has a Boolean value of true or false
– user10632736
Nov 14 '18 at 20:28
if GRAVITY is .9 (0.9 I am guessing), then if it is misusing 25 that only goes up a small bit, but when i run it it looks like it goes up by about 200 pixels and not 25
– user10632736
Nov 15 '18 at 16:41
TheGRAVITY
andself.speed_y = -25
values are pretty much arbitrary (it looked okay when I tested your game). Setting theself.speed_y
doesn't move the sprite up 25 pixels, it sets the speed that is added to the position every frame. So without theGRAVITY
it would move up 25 pixels all the time, but since theGRAVITY
is added to theself.speed_y
, it accelerates the object a bit more downwards each frame until it starts to fall again.
– skrx
Nov 17 '18 at 11:44
so how does the gravity affect the self.spped_y
– user10632736
Nov 18 '18 at 16:49
The gravity increases the speed each frame. When the speed is positive, the sprite moves downwards, when the speed is negative, the sprite moves upwards.
– skrx
Nov 18 '18 at 16:57
|
show 3 more comments
when it says if self.on_ground is it checking when it was has a Boolean value of true or false
– user10632736
Nov 14 '18 at 20:28
if GRAVITY is .9 (0.9 I am guessing), then if it is misusing 25 that only goes up a small bit, but when i run it it looks like it goes up by about 200 pixels and not 25
– user10632736
Nov 15 '18 at 16:41
TheGRAVITY
andself.speed_y = -25
values are pretty much arbitrary (it looked okay when I tested your game). Setting theself.speed_y
doesn't move the sprite up 25 pixels, it sets the speed that is added to the position every frame. So without theGRAVITY
it would move up 25 pixels all the time, but since theGRAVITY
is added to theself.speed_y
, it accelerates the object a bit more downwards each frame until it starts to fall again.
– skrx
Nov 17 '18 at 11:44
so how does the gravity affect the self.spped_y
– user10632736
Nov 18 '18 at 16:49
The gravity increases the speed each frame. When the speed is positive, the sprite moves downwards, when the speed is negative, the sprite moves upwards.
– skrx
Nov 18 '18 at 16:57
when it says if self.on_ground is it checking when it was has a Boolean value of true or false
– user10632736
Nov 14 '18 at 20:28
when it says if self.on_ground is it checking when it was has a Boolean value of true or false
– user10632736
Nov 14 '18 at 20:28
if GRAVITY is .9 (0.9 I am guessing), then if it is misusing 25 that only goes up a small bit, but when i run it it looks like it goes up by about 200 pixels and not 25
– user10632736
Nov 15 '18 at 16:41
if GRAVITY is .9 (0.9 I am guessing), then if it is misusing 25 that only goes up a small bit, but when i run it it looks like it goes up by about 200 pixels and not 25
– user10632736
Nov 15 '18 at 16:41
The
GRAVITY
and self.speed_y = -25
values are pretty much arbitrary (it looked okay when I tested your game). Setting the self.speed_y
doesn't move the sprite up 25 pixels, it sets the speed that is added to the position every frame. So without the GRAVITY
it would move up 25 pixels all the time, but since the GRAVITY
is added to the self.speed_y
, it accelerates the object a bit more downwards each frame until it starts to fall again.– skrx
Nov 17 '18 at 11:44
The
GRAVITY
and self.speed_y = -25
values are pretty much arbitrary (it looked okay when I tested your game). Setting the self.speed_y
doesn't move the sprite up 25 pixels, it sets the speed that is added to the position every frame. So without the GRAVITY
it would move up 25 pixels all the time, but since the GRAVITY
is added to the self.speed_y
, it accelerates the object a bit more downwards each frame until it starts to fall again.– skrx
Nov 17 '18 at 11:44
so how does the gravity affect the self.spped_y
– user10632736
Nov 18 '18 at 16:49
so how does the gravity affect the self.spped_y
– user10632736
Nov 18 '18 at 16:49
The gravity increases the speed each frame. When the speed is positive, the sprite moves downwards, when the speed is negative, the sprite moves upwards.
– skrx
Nov 18 '18 at 16:57
The gravity increases the speed each frame. When the speed is positive, the sprite moves downwards, when the speed is negative, the sprite moves upwards.
– skrx
Nov 18 '18 at 16:57
|
show 3 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53289226%2fhow-do-you-create-jumping-in-pygame-for-a-sprite%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
nHilr2CWn,FPfdr