Android Eclipse Popup Message with Button
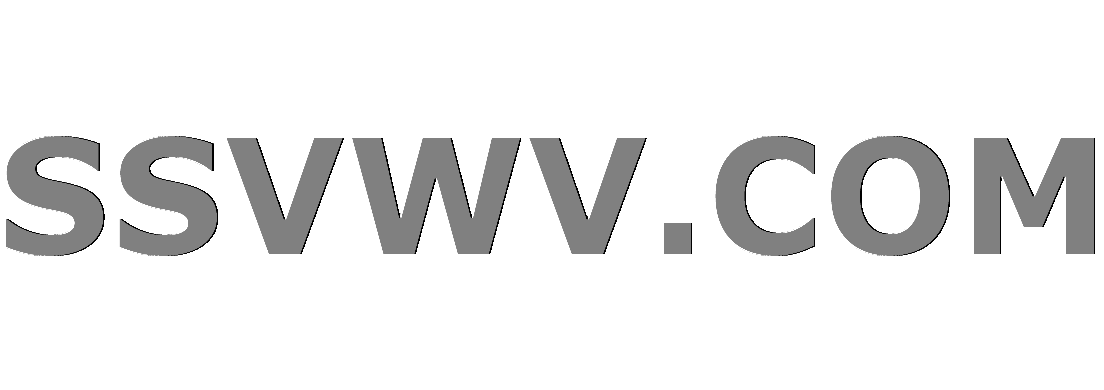
Multi tool use
I would like to make it so, by a click of a button, there is a popup message.
Right now the popup comes as soon as I open the app.
BTW the button I want to trigger the popup is the about button in main.xml
Here is my main.xml (with the layout stuff):
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/main"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:background="#3DE400"
android:orientation="vertical" >
<!-- background originally #d78a00 -->
<TextView
android:id="@+id/title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="60dp"
android:fontFamily="sans-serif-condensed"
android:paddingLeft="10dp"
android:text="Sample App"
android:textColor="#FFF"
android:textSize="60sp" />
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:fontFamily="sans-serif-condensed"
android:paddingLeft="10dp"
android:text="@string/creator"
android:textColor="#FFF"
android:textSize="20dp" />
<Button
android:id="@+id/about"
android:layout_width="123dp"
android:layout_height="wrap_content"
android:layout_marginTop="50dp"
android:background="@android:color/transparent"
android:fontFamily="sans-serif-condensed"
android:gravity="left"
android:paddingLeft="10dp"
android:text="@string/about"
android:textColor="#FFF"
android:textSize="40dp"
android:onClick="show" />
</LinearLayout>
Here is my MainActivity.java:
package com.pranavsanghvi.sampleappv4;
import android.os.Bundle;
import android.app.Activity;
import android.app.AlertDialog;
import android.view.Menu;
import android.widget.Toast;
import android.content.DialogInterface;
import android.view.View;
import android.widget.Button;
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
AlertDialog.Builder alert = new AlertDialog.Builder(MainActivity.this);
alert.setTitle("About");
alert.setMessage("Sample About");
alert.setPositiveButton("Yes", new DialogInterface.OnClickListener() {
public void onClick (DialogInterface dialog, int id) {
Toast.makeText (MainActivity.this, "Success", Toast.LENGTH_SHORT) .show();
}
});
alert.setNegativeButton("No", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
Toast.makeText(MainActivity.this, "Fail", Toast.LENGTH_SHORT) .show();
}
});
alert.show();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}
java

add a comment |
I would like to make it so, by a click of a button, there is a popup message.
Right now the popup comes as soon as I open the app.
BTW the button I want to trigger the popup is the about button in main.xml
Here is my main.xml (with the layout stuff):
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/main"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:background="#3DE400"
android:orientation="vertical" >
<!-- background originally #d78a00 -->
<TextView
android:id="@+id/title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="60dp"
android:fontFamily="sans-serif-condensed"
android:paddingLeft="10dp"
android:text="Sample App"
android:textColor="#FFF"
android:textSize="60sp" />
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:fontFamily="sans-serif-condensed"
android:paddingLeft="10dp"
android:text="@string/creator"
android:textColor="#FFF"
android:textSize="20dp" />
<Button
android:id="@+id/about"
android:layout_width="123dp"
android:layout_height="wrap_content"
android:layout_marginTop="50dp"
android:background="@android:color/transparent"
android:fontFamily="sans-serif-condensed"
android:gravity="left"
android:paddingLeft="10dp"
android:text="@string/about"
android:textColor="#FFF"
android:textSize="40dp"
android:onClick="show" />
</LinearLayout>
Here is my MainActivity.java:
package com.pranavsanghvi.sampleappv4;
import android.os.Bundle;
import android.app.Activity;
import android.app.AlertDialog;
import android.view.Menu;
import android.widget.Toast;
import android.content.DialogInterface;
import android.view.View;
import android.widget.Button;
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
AlertDialog.Builder alert = new AlertDialog.Builder(MainActivity.this);
alert.setTitle("About");
alert.setMessage("Sample About");
alert.setPositiveButton("Yes", new DialogInterface.OnClickListener() {
public void onClick (DialogInterface dialog, int id) {
Toast.makeText (MainActivity.this, "Success", Toast.LENGTH_SHORT) .show();
}
});
alert.setNegativeButton("No", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
Toast.makeText(MainActivity.this, "Fail", Toast.LENGTH_SHORT) .show();
}
});
alert.show();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}
java

add a comment |
I would like to make it so, by a click of a button, there is a popup message.
Right now the popup comes as soon as I open the app.
BTW the button I want to trigger the popup is the about button in main.xml
Here is my main.xml (with the layout stuff):
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/main"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:background="#3DE400"
android:orientation="vertical" >
<!-- background originally #d78a00 -->
<TextView
android:id="@+id/title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="60dp"
android:fontFamily="sans-serif-condensed"
android:paddingLeft="10dp"
android:text="Sample App"
android:textColor="#FFF"
android:textSize="60sp" />
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:fontFamily="sans-serif-condensed"
android:paddingLeft="10dp"
android:text="@string/creator"
android:textColor="#FFF"
android:textSize="20dp" />
<Button
android:id="@+id/about"
android:layout_width="123dp"
android:layout_height="wrap_content"
android:layout_marginTop="50dp"
android:background="@android:color/transparent"
android:fontFamily="sans-serif-condensed"
android:gravity="left"
android:paddingLeft="10dp"
android:text="@string/about"
android:textColor="#FFF"
android:textSize="40dp"
android:onClick="show" />
</LinearLayout>
Here is my MainActivity.java:
package com.pranavsanghvi.sampleappv4;
import android.os.Bundle;
import android.app.Activity;
import android.app.AlertDialog;
import android.view.Menu;
import android.widget.Toast;
import android.content.DialogInterface;
import android.view.View;
import android.widget.Button;
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
AlertDialog.Builder alert = new AlertDialog.Builder(MainActivity.this);
alert.setTitle("About");
alert.setMessage("Sample About");
alert.setPositiveButton("Yes", new DialogInterface.OnClickListener() {
public void onClick (DialogInterface dialog, int id) {
Toast.makeText (MainActivity.this, "Success", Toast.LENGTH_SHORT) .show();
}
});
alert.setNegativeButton("No", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
Toast.makeText(MainActivity.this, "Fail", Toast.LENGTH_SHORT) .show();
}
});
alert.show();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}
java

I would like to make it so, by a click of a button, there is a popup message.
Right now the popup comes as soon as I open the app.
BTW the button I want to trigger the popup is the about button in main.xml
Here is my main.xml (with the layout stuff):
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/main"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:background="#3DE400"
android:orientation="vertical" >
<!-- background originally #d78a00 -->
<TextView
android:id="@+id/title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="60dp"
android:fontFamily="sans-serif-condensed"
android:paddingLeft="10dp"
android:text="Sample App"
android:textColor="#FFF"
android:textSize="60sp" />
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:fontFamily="sans-serif-condensed"
android:paddingLeft="10dp"
android:text="@string/creator"
android:textColor="#FFF"
android:textSize="20dp" />
<Button
android:id="@+id/about"
android:layout_width="123dp"
android:layout_height="wrap_content"
android:layout_marginTop="50dp"
android:background="@android:color/transparent"
android:fontFamily="sans-serif-condensed"
android:gravity="left"
android:paddingLeft="10dp"
android:text="@string/about"
android:textColor="#FFF"
android:textSize="40dp"
android:onClick="show" />
</LinearLayout>
Here is my MainActivity.java:
package com.pranavsanghvi.sampleappv4;
import android.os.Bundle;
import android.app.Activity;
import android.app.AlertDialog;
import android.view.Menu;
import android.widget.Toast;
import android.content.DialogInterface;
import android.view.View;
import android.widget.Button;
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
AlertDialog.Builder alert = new AlertDialog.Builder(MainActivity.this);
alert.setTitle("About");
alert.setMessage("Sample About");
alert.setPositiveButton("Yes", new DialogInterface.OnClickListener() {
public void onClick (DialogInterface dialog, int id) {
Toast.makeText (MainActivity.this, "Success", Toast.LENGTH_SHORT) .show();
}
});
alert.setNegativeButton("No", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
Toast.makeText(MainActivity.this, "Fail", Toast.LENGTH_SHORT) .show();
}
});
alert.show();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}
java

java

asked Jan 19 '14 at 18:48
user3212831user3212831
46116
46116
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
First, declare your alert and button in MainActivity:
public class Mainactivity extends Activity {
private AlertDialog.Builder alert;
private Button btAbout;
//rest of the code
}
Then, in onCreate(), create your alert as you did, except this line:
alert.show(); // <--- remove this line as not to show the alert immediately
Because you've declared global alert, remember to also remove AlertDialog.Builder here, so instead of:
AlertDialog.Builder alert = new AlertDialog.Builder(MainActivity.this);
alert.setTitle("About");
//rest of the code
you should have:
alert = new AlertDialog.Builder(MainActivity.this);
alert.setTitle("About");
//rest of the code
Next, get the handle to your about button:
btAbout = (Button) findViewById(R.id.about);
Set onClickListener to button:
btAbout.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
//when this button is clicked, show the alert
alert.show();
}
});
All of this is in onCreate(). Now when button is clicked, your alert will be displayed.
everything works great except in "alert.show();" in "btAbout.setOnClickListener(new View.OnClickListener() {" the alert has an error that says "Cannot refer to a non-final variable alert inside an inner class defined by another method"
– user3212831
Jan 19 '14 at 19:31
any idea how to fix that?
– user3212831
Jan 19 '14 at 19:32
Have you declaredalert
in MainActivity, not in onCreate()?
– Melquiades
Jan 19 '14 at 19:33
yeah, exactly as you said. but i think the problem is because the alert is being used inside the onclicklistener while it is defined outside in the mainactivity
– user3212831
Jan 19 '14 at 19:36
You need to remove AlertDialog.Builder in front of alert variable in onCreate(), otherwise, global alert is shadowed by the one in onCreate(). See my updated post.
– Melquiades
Jan 19 '14 at 19:38
|
show 2 more comments
If you want to show popup on about button click add the following in onCreate()
Button aboutButton = (Button) findViewById(R.id.about);
aboutButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
alert.show();
}
});
just remove alert.show();
from onCreate();
update :-
Are you getting alert can't be resolved? If so then either make alert global i.e. declare it outside onCreate()
public class MainActivity extends Activity {
AlertDialog.Builder alert;
@Override
protected void onCreate(Bundle savedInstanceState) {
// code
alert = new AlertDialog.Builder(MainActivity.this);
// code
or make it final so that it is
final AlertDialog.Builder alert = new AlertDialog.Builder(MainActivity.this);
also remove alert.show();
which is in onCreate();
when i do that, i have two cannot be resolved's on the alert and on the addNewContact.. how can i fix that?
– user3212831
Jan 19 '14 at 19:00
usealert.show()
only insideaboutbutton
'ssetOnClickListener
– Rohan Kandwal
Jan 19 '14 at 19:21
see my update @user3212831
– Rohan Kandwal
Jan 19 '14 at 20:07
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f21221054%2fandroid-eclipse-popup-message-with-button%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
First, declare your alert and button in MainActivity:
public class Mainactivity extends Activity {
private AlertDialog.Builder alert;
private Button btAbout;
//rest of the code
}
Then, in onCreate(), create your alert as you did, except this line:
alert.show(); // <--- remove this line as not to show the alert immediately
Because you've declared global alert, remember to also remove AlertDialog.Builder here, so instead of:
AlertDialog.Builder alert = new AlertDialog.Builder(MainActivity.this);
alert.setTitle("About");
//rest of the code
you should have:
alert = new AlertDialog.Builder(MainActivity.this);
alert.setTitle("About");
//rest of the code
Next, get the handle to your about button:
btAbout = (Button) findViewById(R.id.about);
Set onClickListener to button:
btAbout.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
//when this button is clicked, show the alert
alert.show();
}
});
All of this is in onCreate(). Now when button is clicked, your alert will be displayed.
everything works great except in "alert.show();" in "btAbout.setOnClickListener(new View.OnClickListener() {" the alert has an error that says "Cannot refer to a non-final variable alert inside an inner class defined by another method"
– user3212831
Jan 19 '14 at 19:31
any idea how to fix that?
– user3212831
Jan 19 '14 at 19:32
Have you declaredalert
in MainActivity, not in onCreate()?
– Melquiades
Jan 19 '14 at 19:33
yeah, exactly as you said. but i think the problem is because the alert is being used inside the onclicklistener while it is defined outside in the mainactivity
– user3212831
Jan 19 '14 at 19:36
You need to remove AlertDialog.Builder in front of alert variable in onCreate(), otherwise, global alert is shadowed by the one in onCreate(). See my updated post.
– Melquiades
Jan 19 '14 at 19:38
|
show 2 more comments
First, declare your alert and button in MainActivity:
public class Mainactivity extends Activity {
private AlertDialog.Builder alert;
private Button btAbout;
//rest of the code
}
Then, in onCreate(), create your alert as you did, except this line:
alert.show(); // <--- remove this line as not to show the alert immediately
Because you've declared global alert, remember to also remove AlertDialog.Builder here, so instead of:
AlertDialog.Builder alert = new AlertDialog.Builder(MainActivity.this);
alert.setTitle("About");
//rest of the code
you should have:
alert = new AlertDialog.Builder(MainActivity.this);
alert.setTitle("About");
//rest of the code
Next, get the handle to your about button:
btAbout = (Button) findViewById(R.id.about);
Set onClickListener to button:
btAbout.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
//when this button is clicked, show the alert
alert.show();
}
});
All of this is in onCreate(). Now when button is clicked, your alert will be displayed.
everything works great except in "alert.show();" in "btAbout.setOnClickListener(new View.OnClickListener() {" the alert has an error that says "Cannot refer to a non-final variable alert inside an inner class defined by another method"
– user3212831
Jan 19 '14 at 19:31
any idea how to fix that?
– user3212831
Jan 19 '14 at 19:32
Have you declaredalert
in MainActivity, not in onCreate()?
– Melquiades
Jan 19 '14 at 19:33
yeah, exactly as you said. but i think the problem is because the alert is being used inside the onclicklistener while it is defined outside in the mainactivity
– user3212831
Jan 19 '14 at 19:36
You need to remove AlertDialog.Builder in front of alert variable in onCreate(), otherwise, global alert is shadowed by the one in onCreate(). See my updated post.
– Melquiades
Jan 19 '14 at 19:38
|
show 2 more comments
First, declare your alert and button in MainActivity:
public class Mainactivity extends Activity {
private AlertDialog.Builder alert;
private Button btAbout;
//rest of the code
}
Then, in onCreate(), create your alert as you did, except this line:
alert.show(); // <--- remove this line as not to show the alert immediately
Because you've declared global alert, remember to also remove AlertDialog.Builder here, so instead of:
AlertDialog.Builder alert = new AlertDialog.Builder(MainActivity.this);
alert.setTitle("About");
//rest of the code
you should have:
alert = new AlertDialog.Builder(MainActivity.this);
alert.setTitle("About");
//rest of the code
Next, get the handle to your about button:
btAbout = (Button) findViewById(R.id.about);
Set onClickListener to button:
btAbout.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
//when this button is clicked, show the alert
alert.show();
}
});
All of this is in onCreate(). Now when button is clicked, your alert will be displayed.
First, declare your alert and button in MainActivity:
public class Mainactivity extends Activity {
private AlertDialog.Builder alert;
private Button btAbout;
//rest of the code
}
Then, in onCreate(), create your alert as you did, except this line:
alert.show(); // <--- remove this line as not to show the alert immediately
Because you've declared global alert, remember to also remove AlertDialog.Builder here, so instead of:
AlertDialog.Builder alert = new AlertDialog.Builder(MainActivity.this);
alert.setTitle("About");
//rest of the code
you should have:
alert = new AlertDialog.Builder(MainActivity.this);
alert.setTitle("About");
//rest of the code
Next, get the handle to your about button:
btAbout = (Button) findViewById(R.id.about);
Set onClickListener to button:
btAbout.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
//when this button is clicked, show the alert
alert.show();
}
});
All of this is in onCreate(). Now when button is clicked, your alert will be displayed.
edited Jan 19 '14 at 19:37
answered Jan 19 '14 at 19:19


MelquiadesMelquiades
7,23112133
7,23112133
everything works great except in "alert.show();" in "btAbout.setOnClickListener(new View.OnClickListener() {" the alert has an error that says "Cannot refer to a non-final variable alert inside an inner class defined by another method"
– user3212831
Jan 19 '14 at 19:31
any idea how to fix that?
– user3212831
Jan 19 '14 at 19:32
Have you declaredalert
in MainActivity, not in onCreate()?
– Melquiades
Jan 19 '14 at 19:33
yeah, exactly as you said. but i think the problem is because the alert is being used inside the onclicklistener while it is defined outside in the mainactivity
– user3212831
Jan 19 '14 at 19:36
You need to remove AlertDialog.Builder in front of alert variable in onCreate(), otherwise, global alert is shadowed by the one in onCreate(). See my updated post.
– Melquiades
Jan 19 '14 at 19:38
|
show 2 more comments
everything works great except in "alert.show();" in "btAbout.setOnClickListener(new View.OnClickListener() {" the alert has an error that says "Cannot refer to a non-final variable alert inside an inner class defined by another method"
– user3212831
Jan 19 '14 at 19:31
any idea how to fix that?
– user3212831
Jan 19 '14 at 19:32
Have you declaredalert
in MainActivity, not in onCreate()?
– Melquiades
Jan 19 '14 at 19:33
yeah, exactly as you said. but i think the problem is because the alert is being used inside the onclicklistener while it is defined outside in the mainactivity
– user3212831
Jan 19 '14 at 19:36
You need to remove AlertDialog.Builder in front of alert variable in onCreate(), otherwise, global alert is shadowed by the one in onCreate(). See my updated post.
– Melquiades
Jan 19 '14 at 19:38
everything works great except in "alert.show();" in "btAbout.setOnClickListener(new View.OnClickListener() {" the alert has an error that says "Cannot refer to a non-final variable alert inside an inner class defined by another method"
– user3212831
Jan 19 '14 at 19:31
everything works great except in "alert.show();" in "btAbout.setOnClickListener(new View.OnClickListener() {" the alert has an error that says "Cannot refer to a non-final variable alert inside an inner class defined by another method"
– user3212831
Jan 19 '14 at 19:31
any idea how to fix that?
– user3212831
Jan 19 '14 at 19:32
any idea how to fix that?
– user3212831
Jan 19 '14 at 19:32
Have you declared
alert
in MainActivity, not in onCreate()?– Melquiades
Jan 19 '14 at 19:33
Have you declared
alert
in MainActivity, not in onCreate()?– Melquiades
Jan 19 '14 at 19:33
yeah, exactly as you said. but i think the problem is because the alert is being used inside the onclicklistener while it is defined outside in the mainactivity
– user3212831
Jan 19 '14 at 19:36
yeah, exactly as you said. but i think the problem is because the alert is being used inside the onclicklistener while it is defined outside in the mainactivity
– user3212831
Jan 19 '14 at 19:36
You need to remove AlertDialog.Builder in front of alert variable in onCreate(), otherwise, global alert is shadowed by the one in onCreate(). See my updated post.
– Melquiades
Jan 19 '14 at 19:38
You need to remove AlertDialog.Builder in front of alert variable in onCreate(), otherwise, global alert is shadowed by the one in onCreate(). See my updated post.
– Melquiades
Jan 19 '14 at 19:38
|
show 2 more comments
If you want to show popup on about button click add the following in onCreate()
Button aboutButton = (Button) findViewById(R.id.about);
aboutButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
alert.show();
}
});
just remove alert.show();
from onCreate();
update :-
Are you getting alert can't be resolved? If so then either make alert global i.e. declare it outside onCreate()
public class MainActivity extends Activity {
AlertDialog.Builder alert;
@Override
protected void onCreate(Bundle savedInstanceState) {
// code
alert = new AlertDialog.Builder(MainActivity.this);
// code
or make it final so that it is
final AlertDialog.Builder alert = new AlertDialog.Builder(MainActivity.this);
also remove alert.show();
which is in onCreate();
when i do that, i have two cannot be resolved's on the alert and on the addNewContact.. how can i fix that?
– user3212831
Jan 19 '14 at 19:00
usealert.show()
only insideaboutbutton
'ssetOnClickListener
– Rohan Kandwal
Jan 19 '14 at 19:21
see my update @user3212831
– Rohan Kandwal
Jan 19 '14 at 20:07
add a comment |
If you want to show popup on about button click add the following in onCreate()
Button aboutButton = (Button) findViewById(R.id.about);
aboutButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
alert.show();
}
});
just remove alert.show();
from onCreate();
update :-
Are you getting alert can't be resolved? If so then either make alert global i.e. declare it outside onCreate()
public class MainActivity extends Activity {
AlertDialog.Builder alert;
@Override
protected void onCreate(Bundle savedInstanceState) {
// code
alert = new AlertDialog.Builder(MainActivity.this);
// code
or make it final so that it is
final AlertDialog.Builder alert = new AlertDialog.Builder(MainActivity.this);
also remove alert.show();
which is in onCreate();
when i do that, i have two cannot be resolved's on the alert and on the addNewContact.. how can i fix that?
– user3212831
Jan 19 '14 at 19:00
usealert.show()
only insideaboutbutton
'ssetOnClickListener
– Rohan Kandwal
Jan 19 '14 at 19:21
see my update @user3212831
– Rohan Kandwal
Jan 19 '14 at 20:07
add a comment |
If you want to show popup on about button click add the following in onCreate()
Button aboutButton = (Button) findViewById(R.id.about);
aboutButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
alert.show();
}
});
just remove alert.show();
from onCreate();
update :-
Are you getting alert can't be resolved? If so then either make alert global i.e. declare it outside onCreate()
public class MainActivity extends Activity {
AlertDialog.Builder alert;
@Override
protected void onCreate(Bundle savedInstanceState) {
// code
alert = new AlertDialog.Builder(MainActivity.this);
// code
or make it final so that it is
final AlertDialog.Builder alert = new AlertDialog.Builder(MainActivity.this);
also remove alert.show();
which is in onCreate();
If you want to show popup on about button click add the following in onCreate()
Button aboutButton = (Button) findViewById(R.id.about);
aboutButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
alert.show();
}
});
just remove alert.show();
from onCreate();
update :-
Are you getting alert can't be resolved? If so then either make alert global i.e. declare it outside onCreate()
public class MainActivity extends Activity {
AlertDialog.Builder alert;
@Override
protected void onCreate(Bundle savedInstanceState) {
// code
alert = new AlertDialog.Builder(MainActivity.this);
// code
or make it final so that it is
final AlertDialog.Builder alert = new AlertDialog.Builder(MainActivity.this);
also remove alert.show();
which is in onCreate();
edited Jan 19 '14 at 19:47
answered Jan 19 '14 at 18:51
Rohan KandwalRohan Kandwal
5,60755087
5,60755087
when i do that, i have two cannot be resolved's on the alert and on the addNewContact.. how can i fix that?
– user3212831
Jan 19 '14 at 19:00
usealert.show()
only insideaboutbutton
'ssetOnClickListener
– Rohan Kandwal
Jan 19 '14 at 19:21
see my update @user3212831
– Rohan Kandwal
Jan 19 '14 at 20:07
add a comment |
when i do that, i have two cannot be resolved's on the alert and on the addNewContact.. how can i fix that?
– user3212831
Jan 19 '14 at 19:00
usealert.show()
only insideaboutbutton
'ssetOnClickListener
– Rohan Kandwal
Jan 19 '14 at 19:21
see my update @user3212831
– Rohan Kandwal
Jan 19 '14 at 20:07
when i do that, i have two cannot be resolved's on the alert and on the addNewContact.. how can i fix that?
– user3212831
Jan 19 '14 at 19:00
when i do that, i have two cannot be resolved's on the alert and on the addNewContact.. how can i fix that?
– user3212831
Jan 19 '14 at 19:00
use
alert.show()
only inside aboutbutton
's setOnClickListener
– Rohan Kandwal
Jan 19 '14 at 19:21
use
alert.show()
only inside aboutbutton
's setOnClickListener
– Rohan Kandwal
Jan 19 '14 at 19:21
see my update @user3212831
– Rohan Kandwal
Jan 19 '14 at 20:07
see my update @user3212831
– Rohan Kandwal
Jan 19 '14 at 20:07
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f21221054%2fandroid-eclipse-popup-message-with-button%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
pCS0o1fBMuIiK3pOU4SfihFckOCtjOwU,6i46xFrjEhLv6ZHnxdMh5eYJ23b3rGemJT7wrK ugav