Why does WPF Style to show validation errors in ToolTip work for a TextBox but fails for a ComboBox?
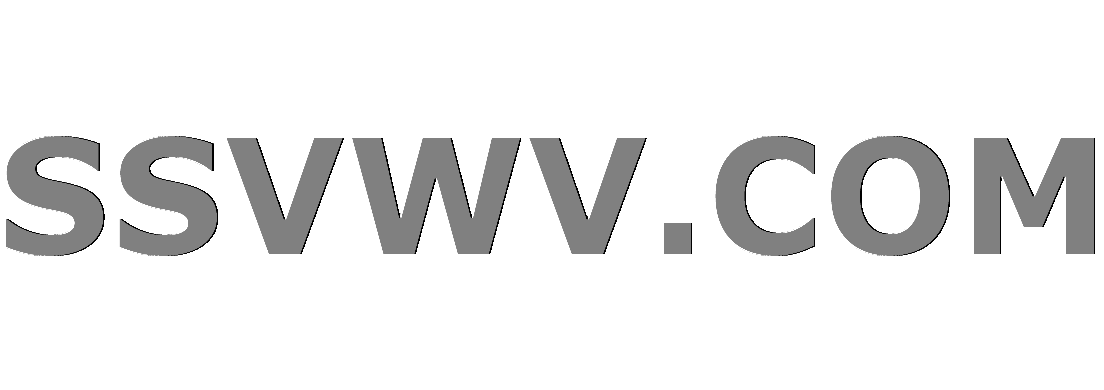
Multi tool use
I am using a typical Style to display validation errors as a tooltip from IErrorDataInfo for a textbox as shown below and it works fine.
<Style TargetType="{x:Type TextBox}">
<Style.Triggers>
<Trigger Property="Validation.HasError" Value="true">
<Setter Property="ToolTip"
Value="{Binding RelativeSource={RelativeSource Self},
Path=(Validation.Errors)[0].ErrorContent}"/>
</Trigger>
</Style.Triggers>
</Style>
But when i try to do the same thing for a ComboBox like this it fails
<Style TargetType="{x:Type ComboBox}">
<Style.Triggers>
<Trigger Property="Validation.HasError" Value="true">
<Setter Property="ToolTip"
Value="{Binding RelativeSource={RelativeSource Self},
Path=(Validation.Errors)[0].ErrorContent}"/>
</Trigger>
</Style.Triggers>
</Style>
The error I get in the output window is:
System.Windows.Data Error: 17 : Cannot get 'Item' value (type 'ValidationError') from '(Validation.Errors)' (type 'ReadOnlyObservableCollection`1'). BindingExpression:Path=(0)[0].ErrorContent; DataItem='ComboBox' (Name='ownerComboBox'); target element is 'ComboBox' (Name='ownerComboBox'); target property is 'ToolTip' (type 'Object') ArgumentOutOfRangeException:'System.ArgumentOutOfRangeException: Specified argument was out of the range of valid values.Parameter name: index'
Oddly it also attempts to make invalid Database changes when I close the window if I change any ComboBox values (This is also when the binding error occurs)!!!
Cannot insert the value NULL into column 'EmpFirstName', table 'OITaskManager.dbo.Employees'; column does not allow nulls. INSERT fails.
The statement has been terminated.
Simply by commenting the style out everyting works perfectly. How do I fix this?
Just in case anyone needs it one of the comboBox' xaml follows:
<ComboBox ItemsSource="{Binding Path=Employees}"
SelectedValuePath="EmpID"
SelectedValue="{Binding Path=SelectedIssue.Employee2.EmpID,
Mode=OneWay, ValidatesOnDataErrors=True}"
ItemTemplate="{StaticResource LastNameFirstComboBoxTemplate}"
Height="28" Name="ownerComboBox" Width="120" Margin="2"
SelectionChanged="ownerComboBox_SelectionChanged" />
<DataTemplate x:Key="LastNameFirstComboBoxTemplate">
<TextBlock>
<TextBlock.Text>
<MultiBinding StringFormat="{}{1}, {0}" >
<Binding Path="EmpFirstName" />
<Binding Path="EmpLastName" />
</MultiBinding>
</TextBlock.Text>
</TextBlock>
</DataTemplate>
SelectionChanged: (I do plan to implement commanding before long but, as this is my first WPF project I have not gone full MVVM yet. I am trying to take things in small-medium sized bites)
// This is done this way to maintain the DataContext Integrity
// and avoid an error due to an Object being "Not New" in Linq-to-SQL
private void ownerComboBox_SelectionChanged(object sender,
SelectionChangedEventArgs e)
{
Employee currentEmpl = ownerComboBox.SelectedItem as Employee;
if (currentEmpl != null &&
currentEmpl != statusBoardViewModel.SelectedIssue.Employee2)
{
statusBoardViewModel.SelectedIssue.Employee2 = currentEmpl;
}
}
c# wpf validation data-binding styles
add a comment |
I am using a typical Style to display validation errors as a tooltip from IErrorDataInfo for a textbox as shown below and it works fine.
<Style TargetType="{x:Type TextBox}">
<Style.Triggers>
<Trigger Property="Validation.HasError" Value="true">
<Setter Property="ToolTip"
Value="{Binding RelativeSource={RelativeSource Self},
Path=(Validation.Errors)[0].ErrorContent}"/>
</Trigger>
</Style.Triggers>
</Style>
But when i try to do the same thing for a ComboBox like this it fails
<Style TargetType="{x:Type ComboBox}">
<Style.Triggers>
<Trigger Property="Validation.HasError" Value="true">
<Setter Property="ToolTip"
Value="{Binding RelativeSource={RelativeSource Self},
Path=(Validation.Errors)[0].ErrorContent}"/>
</Trigger>
</Style.Triggers>
</Style>
The error I get in the output window is:
System.Windows.Data Error: 17 : Cannot get 'Item' value (type 'ValidationError') from '(Validation.Errors)' (type 'ReadOnlyObservableCollection`1'). BindingExpression:Path=(0)[0].ErrorContent; DataItem='ComboBox' (Name='ownerComboBox'); target element is 'ComboBox' (Name='ownerComboBox'); target property is 'ToolTip' (type 'Object') ArgumentOutOfRangeException:'System.ArgumentOutOfRangeException: Specified argument was out of the range of valid values.Parameter name: index'
Oddly it also attempts to make invalid Database changes when I close the window if I change any ComboBox values (This is also when the binding error occurs)!!!
Cannot insert the value NULL into column 'EmpFirstName', table 'OITaskManager.dbo.Employees'; column does not allow nulls. INSERT fails.
The statement has been terminated.
Simply by commenting the style out everyting works perfectly. How do I fix this?
Just in case anyone needs it one of the comboBox' xaml follows:
<ComboBox ItemsSource="{Binding Path=Employees}"
SelectedValuePath="EmpID"
SelectedValue="{Binding Path=SelectedIssue.Employee2.EmpID,
Mode=OneWay, ValidatesOnDataErrors=True}"
ItemTemplate="{StaticResource LastNameFirstComboBoxTemplate}"
Height="28" Name="ownerComboBox" Width="120" Margin="2"
SelectionChanged="ownerComboBox_SelectionChanged" />
<DataTemplate x:Key="LastNameFirstComboBoxTemplate">
<TextBlock>
<TextBlock.Text>
<MultiBinding StringFormat="{}{1}, {0}" >
<Binding Path="EmpFirstName" />
<Binding Path="EmpLastName" />
</MultiBinding>
</TextBlock.Text>
</TextBlock>
</DataTemplate>
SelectionChanged: (I do plan to implement commanding before long but, as this is my first WPF project I have not gone full MVVM yet. I am trying to take things in small-medium sized bites)
// This is done this way to maintain the DataContext Integrity
// and avoid an error due to an Object being "Not New" in Linq-to-SQL
private void ownerComboBox_SelectionChanged(object sender,
SelectionChangedEventArgs e)
{
Employee currentEmpl = ownerComboBox.SelectedItem as Employee;
if (currentEmpl != null &&
currentEmpl != statusBoardViewModel.SelectedIssue.Employee2)
{
statusBoardViewModel.SelectedIssue.Employee2 = currentEmpl;
}
}
c# wpf validation data-binding styles
Well it has been a week with no responses on a question I had assumed was somthing silly on my part. Does anyone have a suggestion on where to research or for additional information for me to post on my problem?
– Mike B
Feb 21 '10 at 1:48
add a comment |
I am using a typical Style to display validation errors as a tooltip from IErrorDataInfo for a textbox as shown below and it works fine.
<Style TargetType="{x:Type TextBox}">
<Style.Triggers>
<Trigger Property="Validation.HasError" Value="true">
<Setter Property="ToolTip"
Value="{Binding RelativeSource={RelativeSource Self},
Path=(Validation.Errors)[0].ErrorContent}"/>
</Trigger>
</Style.Triggers>
</Style>
But when i try to do the same thing for a ComboBox like this it fails
<Style TargetType="{x:Type ComboBox}">
<Style.Triggers>
<Trigger Property="Validation.HasError" Value="true">
<Setter Property="ToolTip"
Value="{Binding RelativeSource={RelativeSource Self},
Path=(Validation.Errors)[0].ErrorContent}"/>
</Trigger>
</Style.Triggers>
</Style>
The error I get in the output window is:
System.Windows.Data Error: 17 : Cannot get 'Item' value (type 'ValidationError') from '(Validation.Errors)' (type 'ReadOnlyObservableCollection`1'). BindingExpression:Path=(0)[0].ErrorContent; DataItem='ComboBox' (Name='ownerComboBox'); target element is 'ComboBox' (Name='ownerComboBox'); target property is 'ToolTip' (type 'Object') ArgumentOutOfRangeException:'System.ArgumentOutOfRangeException: Specified argument was out of the range of valid values.Parameter name: index'
Oddly it also attempts to make invalid Database changes when I close the window if I change any ComboBox values (This is also when the binding error occurs)!!!
Cannot insert the value NULL into column 'EmpFirstName', table 'OITaskManager.dbo.Employees'; column does not allow nulls. INSERT fails.
The statement has been terminated.
Simply by commenting the style out everyting works perfectly. How do I fix this?
Just in case anyone needs it one of the comboBox' xaml follows:
<ComboBox ItemsSource="{Binding Path=Employees}"
SelectedValuePath="EmpID"
SelectedValue="{Binding Path=SelectedIssue.Employee2.EmpID,
Mode=OneWay, ValidatesOnDataErrors=True}"
ItemTemplate="{StaticResource LastNameFirstComboBoxTemplate}"
Height="28" Name="ownerComboBox" Width="120" Margin="2"
SelectionChanged="ownerComboBox_SelectionChanged" />
<DataTemplate x:Key="LastNameFirstComboBoxTemplate">
<TextBlock>
<TextBlock.Text>
<MultiBinding StringFormat="{}{1}, {0}" >
<Binding Path="EmpFirstName" />
<Binding Path="EmpLastName" />
</MultiBinding>
</TextBlock.Text>
</TextBlock>
</DataTemplate>
SelectionChanged: (I do plan to implement commanding before long but, as this is my first WPF project I have not gone full MVVM yet. I am trying to take things in small-medium sized bites)
// This is done this way to maintain the DataContext Integrity
// and avoid an error due to an Object being "Not New" in Linq-to-SQL
private void ownerComboBox_SelectionChanged(object sender,
SelectionChangedEventArgs e)
{
Employee currentEmpl = ownerComboBox.SelectedItem as Employee;
if (currentEmpl != null &&
currentEmpl != statusBoardViewModel.SelectedIssue.Employee2)
{
statusBoardViewModel.SelectedIssue.Employee2 = currentEmpl;
}
}
c# wpf validation data-binding styles
I am using a typical Style to display validation errors as a tooltip from IErrorDataInfo for a textbox as shown below and it works fine.
<Style TargetType="{x:Type TextBox}">
<Style.Triggers>
<Trigger Property="Validation.HasError" Value="true">
<Setter Property="ToolTip"
Value="{Binding RelativeSource={RelativeSource Self},
Path=(Validation.Errors)[0].ErrorContent}"/>
</Trigger>
</Style.Triggers>
</Style>
But when i try to do the same thing for a ComboBox like this it fails
<Style TargetType="{x:Type ComboBox}">
<Style.Triggers>
<Trigger Property="Validation.HasError" Value="true">
<Setter Property="ToolTip"
Value="{Binding RelativeSource={RelativeSource Self},
Path=(Validation.Errors)[0].ErrorContent}"/>
</Trigger>
</Style.Triggers>
</Style>
The error I get in the output window is:
System.Windows.Data Error: 17 : Cannot get 'Item' value (type 'ValidationError') from '(Validation.Errors)' (type 'ReadOnlyObservableCollection`1'). BindingExpression:Path=(0)[0].ErrorContent; DataItem='ComboBox' (Name='ownerComboBox'); target element is 'ComboBox' (Name='ownerComboBox'); target property is 'ToolTip' (type 'Object') ArgumentOutOfRangeException:'System.ArgumentOutOfRangeException: Specified argument was out of the range of valid values.Parameter name: index'
Oddly it also attempts to make invalid Database changes when I close the window if I change any ComboBox values (This is also when the binding error occurs)!!!
Cannot insert the value NULL into column 'EmpFirstName', table 'OITaskManager.dbo.Employees'; column does not allow nulls. INSERT fails.
The statement has been terminated.
Simply by commenting the style out everyting works perfectly. How do I fix this?
Just in case anyone needs it one of the comboBox' xaml follows:
<ComboBox ItemsSource="{Binding Path=Employees}"
SelectedValuePath="EmpID"
SelectedValue="{Binding Path=SelectedIssue.Employee2.EmpID,
Mode=OneWay, ValidatesOnDataErrors=True}"
ItemTemplate="{StaticResource LastNameFirstComboBoxTemplate}"
Height="28" Name="ownerComboBox" Width="120" Margin="2"
SelectionChanged="ownerComboBox_SelectionChanged" />
<DataTemplate x:Key="LastNameFirstComboBoxTemplate">
<TextBlock>
<TextBlock.Text>
<MultiBinding StringFormat="{}{1}, {0}" >
<Binding Path="EmpFirstName" />
<Binding Path="EmpLastName" />
</MultiBinding>
</TextBlock.Text>
</TextBlock>
</DataTemplate>
SelectionChanged: (I do plan to implement commanding before long but, as this is my first WPF project I have not gone full MVVM yet. I am trying to take things in small-medium sized bites)
// This is done this way to maintain the DataContext Integrity
// and avoid an error due to an Object being "Not New" in Linq-to-SQL
private void ownerComboBox_SelectionChanged(object sender,
SelectionChangedEventArgs e)
{
Employee currentEmpl = ownerComboBox.SelectedItem as Employee;
if (currentEmpl != null &&
currentEmpl != statusBoardViewModel.SelectedIssue.Employee2)
{
statusBoardViewModel.SelectedIssue.Employee2 = currentEmpl;
}
}
c# wpf validation data-binding styles
c# wpf validation data-binding styles
edited Feb 14 '10 at 9:43
asked Feb 14 '10 at 9:20
Mike B
1,44232546
1,44232546
Well it has been a week with no responses on a question I had assumed was somthing silly on my part. Does anyone have a suggestion on where to research or for additional information for me to post on my problem?
– Mike B
Feb 21 '10 at 1:48
add a comment |
Well it has been a week with no responses on a question I had assumed was somthing silly on my part. Does anyone have a suggestion on where to research or for additional information for me to post on my problem?
– Mike B
Feb 21 '10 at 1:48
Well it has been a week with no responses on a question I had assumed was somthing silly on my part. Does anyone have a suggestion on where to research or for additional information for me to post on my problem?
– Mike B
Feb 21 '10 at 1:48
Well it has been a week with no responses on a question I had assumed was somthing silly on my part. Does anyone have a suggestion on where to research or for additional information for me to post on my problem?
– Mike B
Feb 21 '10 at 1:48
add a comment |
6 Answers
6
active
oldest
votes
Your getting this error because when you validation finds that there are no issues, the Errors collection returns with no items, and the following binding logic fails:
Path=(Validation.Errors)[0].ErrorContent}"
you are accessing the validation collection by a specific index. I'm currently working on a DataTemplate Suggestion for replacing this text.
I love that Microsoft listed this in their standard example of a validation template.
update so replace the code above with the following, and the binding logic will know how to handle the empty validationresult collection:
Path=(Validation.Errors).CurrentItem.ErrorContent}"
(following xaml was added as a comment)
<ControlTemplate x:Key="ValidationErrorTemplate" TargetType="Control">
<StackPanel Orientation="Horizontal">
<TextBlock Foreground="Red" FontSize="24" Text="*"
ToolTip="{Binding .CurrentItem}">
</TextBlock>
<AdornedElementPlaceholder>
</AdornedElementPlaceholder>
</StackPanel>
</ControlTemplate>
I appreciate your answer on this. unfortunatly I have had to take a bit of a break on coding so it may be a few weeks before I can delve into this.
– Mike B
Nov 3 '10 at 5:18
1
No problem! just trying to answer questions on stack overflow pushes my own knowledge on the subject ;)
– Nathan Tregillus
Dec 27 '10 at 23:18
Thanks for this correction! I had the same problem and now the ugly messages in the output window disappeared.
– Slauma
Feb 4 '11 at 11:46
Exactly! Thanks for the edit Will ;)
– Nathan Tregillus
May 13 '11 at 20:09
2
@NathanTregillus Excellent tip. On the ControlTemplate, I had to useToolTip="{Binding Path=/ErrorContent}"
otherwise I just got a class name inside the tooltip.
– mdisibio
Dec 20 '12 at 5:03
|
show 7 more comments
I think this is the best way:
Path=(Validation.Errors)/ErrorContent
/
is actually equal to CurrentItem
by @Nathan
In my case, CurrentItem
is a no go.
This question was in 2010. Things have changed since then
– Mike B
Apr 25 at 14:42
Sure, the correct answer should be updated also.
– Altiano Gerung
Apr 26 at 5:35
add a comment |
Try the converter for converting to a multi-line string as described here
add a comment |
I've seen the code you're using posted in multiple places, but it seems odd to me that
Path=(Validation.Errors)[0].ErrorContent}
doesn't raise any red flags. But I'm also new to WPF and perhaps there is some secret to making that work in every case.
Rather than attempting to index a possibly empty collection with an array index, add a converter that returns the first error in the list.
add a comment |
In my case, I was getting this exception when I tried to apply @Nation Tregillus' solution:
Cannot resolve property 'CurrentItem' in data context of type
'System.Collections.ObjectModel.ReadOnlyObservableCollection'
So I went with @Altiano Gerung's solution instead, where my code ended up being:
<ControlTemplate x:Key="ValidationErrorTemplate">
<DockPanel Margin="5,0,36,0">
<StackPanel Orientation="Horizontal" VerticalAlignment="Top" DockPanel.Dock="Right"
Margin="5,0,36,0"
ToolTip="{Binding ElementName=ErrorAdorner, Path=AdornedElement.(Validation.Errors)/ErrorContent}"
ToolTipService.ShowDuration="300000"
ToolTipService.InitialShowDelay="0"
ToolTipService.BetweenShowDelay="0"
ToolTipService.VerticalOffset="-75"
>
add a comment |
CurrentItem did not work for me either But
@Nathtan's answer worked for my situation where I have a custom textBox resource. Thanks @Nathan I spent an hour on this.
<Style.Triggers>
<Trigger Property="Validation.HasError" Value="true">
<Setter Property="ToolTip"
Value="{Binding RelativeSource={x:Static RelativeSource.Self},
Path=(Validation.Errors)/ErrorContent}" />
</Trigger>
</Style.Triggers>
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f2260616%2fwhy-does-wpf-style-to-show-validation-errors-in-tooltip-work-for-a-textbox-but-f%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
6 Answers
6
active
oldest
votes
6 Answers
6
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your getting this error because when you validation finds that there are no issues, the Errors collection returns with no items, and the following binding logic fails:
Path=(Validation.Errors)[0].ErrorContent}"
you are accessing the validation collection by a specific index. I'm currently working on a DataTemplate Suggestion for replacing this text.
I love that Microsoft listed this in their standard example of a validation template.
update so replace the code above with the following, and the binding logic will know how to handle the empty validationresult collection:
Path=(Validation.Errors).CurrentItem.ErrorContent}"
(following xaml was added as a comment)
<ControlTemplate x:Key="ValidationErrorTemplate" TargetType="Control">
<StackPanel Orientation="Horizontal">
<TextBlock Foreground="Red" FontSize="24" Text="*"
ToolTip="{Binding .CurrentItem}">
</TextBlock>
<AdornedElementPlaceholder>
</AdornedElementPlaceholder>
</StackPanel>
</ControlTemplate>
I appreciate your answer on this. unfortunatly I have had to take a bit of a break on coding so it may be a few weeks before I can delve into this.
– Mike B
Nov 3 '10 at 5:18
1
No problem! just trying to answer questions on stack overflow pushes my own knowledge on the subject ;)
– Nathan Tregillus
Dec 27 '10 at 23:18
Thanks for this correction! I had the same problem and now the ugly messages in the output window disappeared.
– Slauma
Feb 4 '11 at 11:46
Exactly! Thanks for the edit Will ;)
– Nathan Tregillus
May 13 '11 at 20:09
2
@NathanTregillus Excellent tip. On the ControlTemplate, I had to useToolTip="{Binding Path=/ErrorContent}"
otherwise I just got a class name inside the tooltip.
– mdisibio
Dec 20 '12 at 5:03
|
show 7 more comments
Your getting this error because when you validation finds that there are no issues, the Errors collection returns with no items, and the following binding logic fails:
Path=(Validation.Errors)[0].ErrorContent}"
you are accessing the validation collection by a specific index. I'm currently working on a DataTemplate Suggestion for replacing this text.
I love that Microsoft listed this in their standard example of a validation template.
update so replace the code above with the following, and the binding logic will know how to handle the empty validationresult collection:
Path=(Validation.Errors).CurrentItem.ErrorContent}"
(following xaml was added as a comment)
<ControlTemplate x:Key="ValidationErrorTemplate" TargetType="Control">
<StackPanel Orientation="Horizontal">
<TextBlock Foreground="Red" FontSize="24" Text="*"
ToolTip="{Binding .CurrentItem}">
</TextBlock>
<AdornedElementPlaceholder>
</AdornedElementPlaceholder>
</StackPanel>
</ControlTemplate>
I appreciate your answer on this. unfortunatly I have had to take a bit of a break on coding so it may be a few weeks before I can delve into this.
– Mike B
Nov 3 '10 at 5:18
1
No problem! just trying to answer questions on stack overflow pushes my own knowledge on the subject ;)
– Nathan Tregillus
Dec 27 '10 at 23:18
Thanks for this correction! I had the same problem and now the ugly messages in the output window disappeared.
– Slauma
Feb 4 '11 at 11:46
Exactly! Thanks for the edit Will ;)
– Nathan Tregillus
May 13 '11 at 20:09
2
@NathanTregillus Excellent tip. On the ControlTemplate, I had to useToolTip="{Binding Path=/ErrorContent}"
otherwise I just got a class name inside the tooltip.
– mdisibio
Dec 20 '12 at 5:03
|
show 7 more comments
Your getting this error because when you validation finds that there are no issues, the Errors collection returns with no items, and the following binding logic fails:
Path=(Validation.Errors)[0].ErrorContent}"
you are accessing the validation collection by a specific index. I'm currently working on a DataTemplate Suggestion for replacing this text.
I love that Microsoft listed this in their standard example of a validation template.
update so replace the code above with the following, and the binding logic will know how to handle the empty validationresult collection:
Path=(Validation.Errors).CurrentItem.ErrorContent}"
(following xaml was added as a comment)
<ControlTemplate x:Key="ValidationErrorTemplate" TargetType="Control">
<StackPanel Orientation="Horizontal">
<TextBlock Foreground="Red" FontSize="24" Text="*"
ToolTip="{Binding .CurrentItem}">
</TextBlock>
<AdornedElementPlaceholder>
</AdornedElementPlaceholder>
</StackPanel>
</ControlTemplate>
Your getting this error because when you validation finds that there are no issues, the Errors collection returns with no items, and the following binding logic fails:
Path=(Validation.Errors)[0].ErrorContent}"
you are accessing the validation collection by a specific index. I'm currently working on a DataTemplate Suggestion for replacing this text.
I love that Microsoft listed this in their standard example of a validation template.
update so replace the code above with the following, and the binding logic will know how to handle the empty validationresult collection:
Path=(Validation.Errors).CurrentItem.ErrorContent}"
(following xaml was added as a comment)
<ControlTemplate x:Key="ValidationErrorTemplate" TargetType="Control">
<StackPanel Orientation="Horizontal">
<TextBlock Foreground="Red" FontSize="24" Text="*"
ToolTip="{Binding .CurrentItem}">
</TextBlock>
<AdornedElementPlaceholder>
</AdornedElementPlaceholder>
</StackPanel>
</ControlTemplate>
edited Apr 13 '16 at 1:07


Noctis
9,52533062
9,52533062
answered Nov 2 '10 at 14:39
Nathan Tregillus
3,54123358
3,54123358
I appreciate your answer on this. unfortunatly I have had to take a bit of a break on coding so it may be a few weeks before I can delve into this.
– Mike B
Nov 3 '10 at 5:18
1
No problem! just trying to answer questions on stack overflow pushes my own knowledge on the subject ;)
– Nathan Tregillus
Dec 27 '10 at 23:18
Thanks for this correction! I had the same problem and now the ugly messages in the output window disappeared.
– Slauma
Feb 4 '11 at 11:46
Exactly! Thanks for the edit Will ;)
– Nathan Tregillus
May 13 '11 at 20:09
2
@NathanTregillus Excellent tip. On the ControlTemplate, I had to useToolTip="{Binding Path=/ErrorContent}"
otherwise I just got a class name inside the tooltip.
– mdisibio
Dec 20 '12 at 5:03
|
show 7 more comments
I appreciate your answer on this. unfortunatly I have had to take a bit of a break on coding so it may be a few weeks before I can delve into this.
– Mike B
Nov 3 '10 at 5:18
1
No problem! just trying to answer questions on stack overflow pushes my own knowledge on the subject ;)
– Nathan Tregillus
Dec 27 '10 at 23:18
Thanks for this correction! I had the same problem and now the ugly messages in the output window disappeared.
– Slauma
Feb 4 '11 at 11:46
Exactly! Thanks for the edit Will ;)
– Nathan Tregillus
May 13 '11 at 20:09
2
@NathanTregillus Excellent tip. On the ControlTemplate, I had to useToolTip="{Binding Path=/ErrorContent}"
otherwise I just got a class name inside the tooltip.
– mdisibio
Dec 20 '12 at 5:03
I appreciate your answer on this. unfortunatly I have had to take a bit of a break on coding so it may be a few weeks before I can delve into this.
– Mike B
Nov 3 '10 at 5:18
I appreciate your answer on this. unfortunatly I have had to take a bit of a break on coding so it may be a few weeks before I can delve into this.
– Mike B
Nov 3 '10 at 5:18
1
1
No problem! just trying to answer questions on stack overflow pushes my own knowledge on the subject ;)
– Nathan Tregillus
Dec 27 '10 at 23:18
No problem! just trying to answer questions on stack overflow pushes my own knowledge on the subject ;)
– Nathan Tregillus
Dec 27 '10 at 23:18
Thanks for this correction! I had the same problem and now the ugly messages in the output window disappeared.
– Slauma
Feb 4 '11 at 11:46
Thanks for this correction! I had the same problem and now the ugly messages in the output window disappeared.
– Slauma
Feb 4 '11 at 11:46
Exactly! Thanks for the edit Will ;)
– Nathan Tregillus
May 13 '11 at 20:09
Exactly! Thanks for the edit Will ;)
– Nathan Tregillus
May 13 '11 at 20:09
2
2
@NathanTregillus Excellent tip. On the ControlTemplate, I had to use
ToolTip="{Binding Path=/ErrorContent}"
otherwise I just got a class name inside the tooltip.– mdisibio
Dec 20 '12 at 5:03
@NathanTregillus Excellent tip. On the ControlTemplate, I had to use
ToolTip="{Binding Path=/ErrorContent}"
otherwise I just got a class name inside the tooltip.– mdisibio
Dec 20 '12 at 5:03
|
show 7 more comments
I think this is the best way:
Path=(Validation.Errors)/ErrorContent
/
is actually equal to CurrentItem
by @Nathan
In my case, CurrentItem
is a no go.
This question was in 2010. Things have changed since then
– Mike B
Apr 25 at 14:42
Sure, the correct answer should be updated also.
– Altiano Gerung
Apr 26 at 5:35
add a comment |
I think this is the best way:
Path=(Validation.Errors)/ErrorContent
/
is actually equal to CurrentItem
by @Nathan
In my case, CurrentItem
is a no go.
This question was in 2010. Things have changed since then
– Mike B
Apr 25 at 14:42
Sure, the correct answer should be updated also.
– Altiano Gerung
Apr 26 at 5:35
add a comment |
I think this is the best way:
Path=(Validation.Errors)/ErrorContent
/
is actually equal to CurrentItem
by @Nathan
In my case, CurrentItem
is a no go.
I think this is the best way:
Path=(Validation.Errors)/ErrorContent
/
is actually equal to CurrentItem
by @Nathan
In my case, CurrentItem
is a no go.
answered Apr 25 at 4:08
Altiano Gerung
3572622
3572622
This question was in 2010. Things have changed since then
– Mike B
Apr 25 at 14:42
Sure, the correct answer should be updated also.
– Altiano Gerung
Apr 26 at 5:35
add a comment |
This question was in 2010. Things have changed since then
– Mike B
Apr 25 at 14:42
Sure, the correct answer should be updated also.
– Altiano Gerung
Apr 26 at 5:35
This question was in 2010. Things have changed since then
– Mike B
Apr 25 at 14:42
This question was in 2010. Things have changed since then
– Mike B
Apr 25 at 14:42
Sure, the correct answer should be updated also.
– Altiano Gerung
Apr 26 at 5:35
Sure, the correct answer should be updated also.
– Altiano Gerung
Apr 26 at 5:35
add a comment |
Try the converter for converting to a multi-line string as described here
add a comment |
Try the converter for converting to a multi-line string as described here
add a comment |
Try the converter for converting to a multi-line string as described here
Try the converter for converting to a multi-line string as described here
answered Apr 20 '10 at 18:46
djamwal
212
212
add a comment |
add a comment |
I've seen the code you're using posted in multiple places, but it seems odd to me that
Path=(Validation.Errors)[0].ErrorContent}
doesn't raise any red flags. But I'm also new to WPF and perhaps there is some secret to making that work in every case.
Rather than attempting to index a possibly empty collection with an array index, add a converter that returns the first error in the list.
add a comment |
I've seen the code you're using posted in multiple places, but it seems odd to me that
Path=(Validation.Errors)[0].ErrorContent}
doesn't raise any red flags. But I'm also new to WPF and perhaps there is some secret to making that work in every case.
Rather than attempting to index a possibly empty collection with an array index, add a converter that returns the first error in the list.
add a comment |
I've seen the code you're using posted in multiple places, but it seems odd to me that
Path=(Validation.Errors)[0].ErrorContent}
doesn't raise any red flags. But I'm also new to WPF and perhaps there is some secret to making that work in every case.
Rather than attempting to index a possibly empty collection with an array index, add a converter that returns the first error in the list.
I've seen the code you're using posted in multiple places, but it seems odd to me that
Path=(Validation.Errors)[0].ErrorContent}
doesn't raise any red flags. But I'm also new to WPF and perhaps there is some secret to making that work in every case.
Rather than attempting to index a possibly empty collection with an array index, add a converter that returns the first error in the list.
edited May 3 '14 at 7:48


Noctis
9,52533062
9,52533062
answered Jun 21 '10 at 16:33
David
111
111
add a comment |
add a comment |
In my case, I was getting this exception when I tried to apply @Nation Tregillus' solution:
Cannot resolve property 'CurrentItem' in data context of type
'System.Collections.ObjectModel.ReadOnlyObservableCollection'
So I went with @Altiano Gerung's solution instead, where my code ended up being:
<ControlTemplate x:Key="ValidationErrorTemplate">
<DockPanel Margin="5,0,36,0">
<StackPanel Orientation="Horizontal" VerticalAlignment="Top" DockPanel.Dock="Right"
Margin="5,0,36,0"
ToolTip="{Binding ElementName=ErrorAdorner, Path=AdornedElement.(Validation.Errors)/ErrorContent}"
ToolTipService.ShowDuration="300000"
ToolTipService.InitialShowDelay="0"
ToolTipService.BetweenShowDelay="0"
ToolTipService.VerticalOffset="-75"
>
add a comment |
In my case, I was getting this exception when I tried to apply @Nation Tregillus' solution:
Cannot resolve property 'CurrentItem' in data context of type
'System.Collections.ObjectModel.ReadOnlyObservableCollection'
So I went with @Altiano Gerung's solution instead, where my code ended up being:
<ControlTemplate x:Key="ValidationErrorTemplate">
<DockPanel Margin="5,0,36,0">
<StackPanel Orientation="Horizontal" VerticalAlignment="Top" DockPanel.Dock="Right"
Margin="5,0,36,0"
ToolTip="{Binding ElementName=ErrorAdorner, Path=AdornedElement.(Validation.Errors)/ErrorContent}"
ToolTipService.ShowDuration="300000"
ToolTipService.InitialShowDelay="0"
ToolTipService.BetweenShowDelay="0"
ToolTipService.VerticalOffset="-75"
>
add a comment |
In my case, I was getting this exception when I tried to apply @Nation Tregillus' solution:
Cannot resolve property 'CurrentItem' in data context of type
'System.Collections.ObjectModel.ReadOnlyObservableCollection'
So I went with @Altiano Gerung's solution instead, where my code ended up being:
<ControlTemplate x:Key="ValidationErrorTemplate">
<DockPanel Margin="5,0,36,0">
<StackPanel Orientation="Horizontal" VerticalAlignment="Top" DockPanel.Dock="Right"
Margin="5,0,36,0"
ToolTip="{Binding ElementName=ErrorAdorner, Path=AdornedElement.(Validation.Errors)/ErrorContent}"
ToolTipService.ShowDuration="300000"
ToolTipService.InitialShowDelay="0"
ToolTipService.BetweenShowDelay="0"
ToolTipService.VerticalOffset="-75"
>
In my case, I was getting this exception when I tried to apply @Nation Tregillus' solution:
Cannot resolve property 'CurrentItem' in data context of type
'System.Collections.ObjectModel.ReadOnlyObservableCollection'
So I went with @Altiano Gerung's solution instead, where my code ended up being:
<ControlTemplate x:Key="ValidationErrorTemplate">
<DockPanel Margin="5,0,36,0">
<StackPanel Orientation="Horizontal" VerticalAlignment="Top" DockPanel.Dock="Right"
Margin="5,0,36,0"
ToolTip="{Binding ElementName=ErrorAdorner, Path=AdornedElement.(Validation.Errors)/ErrorContent}"
ToolTipService.ShowDuration="300000"
ToolTipService.InitialShowDelay="0"
ToolTipService.BetweenShowDelay="0"
ToolTipService.VerticalOffset="-75"
>
answered Nov 12 at 16:36
user8128167
2,54753449
2,54753449
add a comment |
add a comment |
CurrentItem did not work for me either But
@Nathtan's answer worked for my situation where I have a custom textBox resource. Thanks @Nathan I spent an hour on this.
<Style.Triggers>
<Trigger Property="Validation.HasError" Value="true">
<Setter Property="ToolTip"
Value="{Binding RelativeSource={x:Static RelativeSource.Self},
Path=(Validation.Errors)/ErrorContent}" />
</Trigger>
</Style.Triggers>
add a comment |
CurrentItem did not work for me either But
@Nathtan's answer worked for my situation where I have a custom textBox resource. Thanks @Nathan I spent an hour on this.
<Style.Triggers>
<Trigger Property="Validation.HasError" Value="true">
<Setter Property="ToolTip"
Value="{Binding RelativeSource={x:Static RelativeSource.Self},
Path=(Validation.Errors)/ErrorContent}" />
</Trigger>
</Style.Triggers>
add a comment |
CurrentItem did not work for me either But
@Nathtan's answer worked for my situation where I have a custom textBox resource. Thanks @Nathan I spent an hour on this.
<Style.Triggers>
<Trigger Property="Validation.HasError" Value="true">
<Setter Property="ToolTip"
Value="{Binding RelativeSource={x:Static RelativeSource.Self},
Path=(Validation.Errors)/ErrorContent}" />
</Trigger>
</Style.Triggers>
CurrentItem did not work for me either But
@Nathtan's answer worked for my situation where I have a custom textBox resource. Thanks @Nathan I spent an hour on this.
<Style.Triggers>
<Trigger Property="Validation.HasError" Value="true">
<Setter Property="ToolTip"
Value="{Binding RelativeSource={x:Static RelativeSource.Self},
Path=(Validation.Errors)/ErrorContent}" />
</Trigger>
</Style.Triggers>
answered Nov 29 at 21:02
bbedson
84
84
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f2260616%2fwhy-does-wpf-style-to-show-validation-errors-in-tooltip-work-for-a-textbox-but-f%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
r,AIGF,E6qNIfplQyuP Fw5Ny 5LdXJ4g DKO0I4 xPY9,fROy4dG yk,C
Well it has been a week with no responses on a question I had assumed was somthing silly on my part. Does anyone have a suggestion on where to research or for additional information for me to post on my problem?
– Mike B
Feb 21 '10 at 1:48