how to extract logic from views
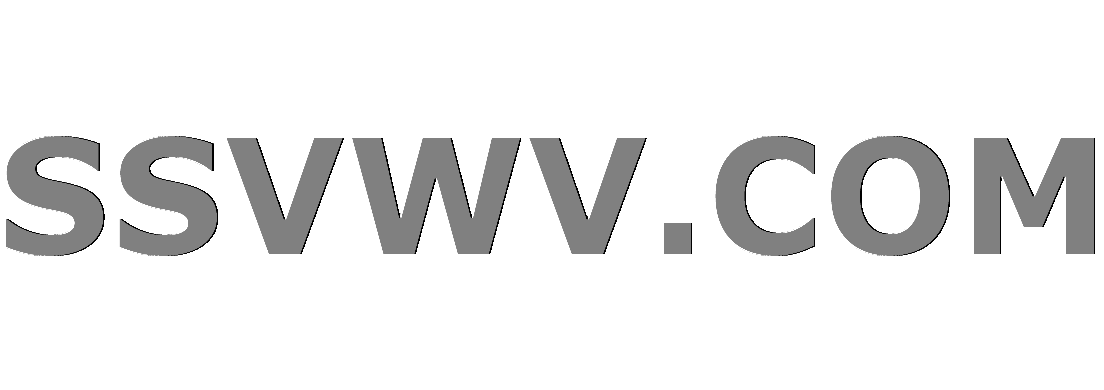
Multi tool use
In my app I can search consumptions
by dates
I have an active record model Consumption which belongs_to :users
and a model ConsumptionSearch
consumption.rb
class Consumption < ApplicationRecord
belongs_to :user
end
consumption_search.rb
class ConsumptionSearch
attr_reader :date_from, :date_to
def initialize(params)
params ||= {}
@date_from = parsed_date(params[:date_from],Time.now.beginning_of_month.to_date.to_s)
@date_to = parsed_date(params[:date_to], (Date.today + 1).to_s)
end
def date_range
Consumption.where('created_at BETWEEN ? AND ?', @date_from, @date_to)
end
private
def parsed_date(date_string, default)
Date.parse(date_string)
rescue ArgumentError, TypeError
default
end
end
In the consumptions_controller
in the index
action I can retrieve the wanted consumptions by date
class ConsumptionsController < ApplicationController
def index
@search = ConsumptionSearch.new(params[:search])
@consumptions = @search.date_range
@consumptions = @consumptions.order('created_at ASC').where(user_id: current_user.id)
end
end
consumptions schema may help:
create_table "consumptions", force: :cascade do |t|
t.float "total_price"
t.float "kilometers"
t.string "shop"
t.float "liter_price"
t.float "total_liters"
t.datetime "created_at", null: false
t.datetime "updated_at", null: false
t.integer "user_id"
t.float "difference", default: 0.0
end
So in my consumptions/view/index.html.erb
I just have to iterrate over @consumptions
to display what I need.
The question is:
How can I make it more "ruby way", I have too much logic in the view... Where and how should I extract the logic? thx
<%= price = (@consumptions.map { |c| c.total_price }.sum - @consumptions.last.total_price).round(2) %>
<%= total_km = (@consumptions.map { |c| c.difference }.sum).round.abs %>
<%= (price / total_km).round(4) %>
<%= (price / total_km * 100).round(2) %>
ruby-on-rails ruby ruby-on-rails-5
add a comment |
In my app I can search consumptions
by dates
I have an active record model Consumption which belongs_to :users
and a model ConsumptionSearch
consumption.rb
class Consumption < ApplicationRecord
belongs_to :user
end
consumption_search.rb
class ConsumptionSearch
attr_reader :date_from, :date_to
def initialize(params)
params ||= {}
@date_from = parsed_date(params[:date_from],Time.now.beginning_of_month.to_date.to_s)
@date_to = parsed_date(params[:date_to], (Date.today + 1).to_s)
end
def date_range
Consumption.where('created_at BETWEEN ? AND ?', @date_from, @date_to)
end
private
def parsed_date(date_string, default)
Date.parse(date_string)
rescue ArgumentError, TypeError
default
end
end
In the consumptions_controller
in the index
action I can retrieve the wanted consumptions by date
class ConsumptionsController < ApplicationController
def index
@search = ConsumptionSearch.new(params[:search])
@consumptions = @search.date_range
@consumptions = @consumptions.order('created_at ASC').where(user_id: current_user.id)
end
end
consumptions schema may help:
create_table "consumptions", force: :cascade do |t|
t.float "total_price"
t.float "kilometers"
t.string "shop"
t.float "liter_price"
t.float "total_liters"
t.datetime "created_at", null: false
t.datetime "updated_at", null: false
t.integer "user_id"
t.float "difference", default: 0.0
end
So in my consumptions/view/index.html.erb
I just have to iterrate over @consumptions
to display what I need.
The question is:
How can I make it more "ruby way", I have too much logic in the view... Where and how should I extract the logic? thx
<%= price = (@consumptions.map { |c| c.total_price }.sum - @consumptions.last.total_price).round(2) %>
<%= total_km = (@consumptions.map { |c| c.difference }.sum).round.abs %>
<%= (price / total_km).round(4) %>
<%= (price / total_km * 100).round(2) %>
ruby-on-rails ruby ruby-on-rails-5
Hint: Helper methods or methods on the model that can help compute things.
– tadman
Nov 12 at 16:51
add a comment |
In my app I can search consumptions
by dates
I have an active record model Consumption which belongs_to :users
and a model ConsumptionSearch
consumption.rb
class Consumption < ApplicationRecord
belongs_to :user
end
consumption_search.rb
class ConsumptionSearch
attr_reader :date_from, :date_to
def initialize(params)
params ||= {}
@date_from = parsed_date(params[:date_from],Time.now.beginning_of_month.to_date.to_s)
@date_to = parsed_date(params[:date_to], (Date.today + 1).to_s)
end
def date_range
Consumption.where('created_at BETWEEN ? AND ?', @date_from, @date_to)
end
private
def parsed_date(date_string, default)
Date.parse(date_string)
rescue ArgumentError, TypeError
default
end
end
In the consumptions_controller
in the index
action I can retrieve the wanted consumptions by date
class ConsumptionsController < ApplicationController
def index
@search = ConsumptionSearch.new(params[:search])
@consumptions = @search.date_range
@consumptions = @consumptions.order('created_at ASC').where(user_id: current_user.id)
end
end
consumptions schema may help:
create_table "consumptions", force: :cascade do |t|
t.float "total_price"
t.float "kilometers"
t.string "shop"
t.float "liter_price"
t.float "total_liters"
t.datetime "created_at", null: false
t.datetime "updated_at", null: false
t.integer "user_id"
t.float "difference", default: 0.0
end
So in my consumptions/view/index.html.erb
I just have to iterrate over @consumptions
to display what I need.
The question is:
How can I make it more "ruby way", I have too much logic in the view... Where and how should I extract the logic? thx
<%= price = (@consumptions.map { |c| c.total_price }.sum - @consumptions.last.total_price).round(2) %>
<%= total_km = (@consumptions.map { |c| c.difference }.sum).round.abs %>
<%= (price / total_km).round(4) %>
<%= (price / total_km * 100).round(2) %>
ruby-on-rails ruby ruby-on-rails-5
In my app I can search consumptions
by dates
I have an active record model Consumption which belongs_to :users
and a model ConsumptionSearch
consumption.rb
class Consumption < ApplicationRecord
belongs_to :user
end
consumption_search.rb
class ConsumptionSearch
attr_reader :date_from, :date_to
def initialize(params)
params ||= {}
@date_from = parsed_date(params[:date_from],Time.now.beginning_of_month.to_date.to_s)
@date_to = parsed_date(params[:date_to], (Date.today + 1).to_s)
end
def date_range
Consumption.where('created_at BETWEEN ? AND ?', @date_from, @date_to)
end
private
def parsed_date(date_string, default)
Date.parse(date_string)
rescue ArgumentError, TypeError
default
end
end
In the consumptions_controller
in the index
action I can retrieve the wanted consumptions by date
class ConsumptionsController < ApplicationController
def index
@search = ConsumptionSearch.new(params[:search])
@consumptions = @search.date_range
@consumptions = @consumptions.order('created_at ASC').where(user_id: current_user.id)
end
end
consumptions schema may help:
create_table "consumptions", force: :cascade do |t|
t.float "total_price"
t.float "kilometers"
t.string "shop"
t.float "liter_price"
t.float "total_liters"
t.datetime "created_at", null: false
t.datetime "updated_at", null: false
t.integer "user_id"
t.float "difference", default: 0.0
end
So in my consumptions/view/index.html.erb
I just have to iterrate over @consumptions
to display what I need.
The question is:
How can I make it more "ruby way", I have too much logic in the view... Where and how should I extract the logic? thx
<%= price = (@consumptions.map { |c| c.total_price }.sum - @consumptions.last.total_price).round(2) %>
<%= total_km = (@consumptions.map { |c| c.difference }.sum).round.abs %>
<%= (price / total_km).round(4) %>
<%= (price / total_km * 100).round(2) %>
ruby-on-rails ruby ruby-on-rails-5
ruby-on-rails ruby ruby-on-rails-5
asked Nov 12 at 16:44


NEL
767
767
Hint: Helper methods or methods on the model that can help compute things.
– tadman
Nov 12 at 16:51
add a comment |
Hint: Helper methods or methods on the model that can help compute things.
– tadman
Nov 12 at 16:51
Hint: Helper methods or methods on the model that can help compute things.
– tadman
Nov 12 at 16:51
Hint: Helper methods or methods on the model that can help compute things.
– tadman
Nov 12 at 16:51
add a comment |
2 Answers
2
active
oldest
votes
You can create helper: app/helpers/consumptions_helper.rb
module ConsumptionsHelper
def some_logic
...
end
end
and use it in the view
<%= some_logic %>
Please check RoR doc here
https://api.rubyonrails.org/classes/ActionController/Helpers.html
add a comment |
Why not add the logic to your ConsumptionSearch
class? You are already abstracting logic to it. And, you are already accessing it in the view. So, perhaps something like:
class ConsumptionSearch
attr_reader :date_from, :date_to
def initialize(params)
params ||= {}
@date_from = parsed_date(params[:date_from],Time.now.beginning_of_month.to_date.to_s)
@date_to = parsed_date(params[:date_to], (Date.today + 1).to_s)
end
def date_range
Consumption.where('created_at BETWEEN ? AND ?', @date_from, @date_to)
end
def price
...
end
def total_km
...
end
def price_per_km(round_to)
(price/total_km).round(round_to)
end
private
def parsed_date(date_string, default)
Date.parse(date_string)
rescue ArgumentError, TypeError
default
end
end
And then in view:
<%= @consumptions.price %>
<%= @consumptions.total_km %>
<%= @consumptions.price_per_km(4) %>
<%= @consumptions.price_per_km(2) %>
Personally, helpers
are not my favorite thing. But, I realize that some people use them regularly and with great success. I'm just odd that way.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53266564%2fhow-to-extract-logic-from-views%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can create helper: app/helpers/consumptions_helper.rb
module ConsumptionsHelper
def some_logic
...
end
end
and use it in the view
<%= some_logic %>
Please check RoR doc here
https://api.rubyonrails.org/classes/ActionController/Helpers.html
add a comment |
You can create helper: app/helpers/consumptions_helper.rb
module ConsumptionsHelper
def some_logic
...
end
end
and use it in the view
<%= some_logic %>
Please check RoR doc here
https://api.rubyonrails.org/classes/ActionController/Helpers.html
add a comment |
You can create helper: app/helpers/consumptions_helper.rb
module ConsumptionsHelper
def some_logic
...
end
end
and use it in the view
<%= some_logic %>
Please check RoR doc here
https://api.rubyonrails.org/classes/ActionController/Helpers.html
You can create helper: app/helpers/consumptions_helper.rb
module ConsumptionsHelper
def some_logic
...
end
end
and use it in the view
<%= some_logic %>
Please check RoR doc here
https://api.rubyonrails.org/classes/ActionController/Helpers.html
answered Nov 12 at 17:14
Maksim P
414
414
add a comment |
add a comment |
Why not add the logic to your ConsumptionSearch
class? You are already abstracting logic to it. And, you are already accessing it in the view. So, perhaps something like:
class ConsumptionSearch
attr_reader :date_from, :date_to
def initialize(params)
params ||= {}
@date_from = parsed_date(params[:date_from],Time.now.beginning_of_month.to_date.to_s)
@date_to = parsed_date(params[:date_to], (Date.today + 1).to_s)
end
def date_range
Consumption.where('created_at BETWEEN ? AND ?', @date_from, @date_to)
end
def price
...
end
def total_km
...
end
def price_per_km(round_to)
(price/total_km).round(round_to)
end
private
def parsed_date(date_string, default)
Date.parse(date_string)
rescue ArgumentError, TypeError
default
end
end
And then in view:
<%= @consumptions.price %>
<%= @consumptions.total_km %>
<%= @consumptions.price_per_km(4) %>
<%= @consumptions.price_per_km(2) %>
Personally, helpers
are not my favorite thing. But, I realize that some people use them regularly and with great success. I'm just odd that way.
add a comment |
Why not add the logic to your ConsumptionSearch
class? You are already abstracting logic to it. And, you are already accessing it in the view. So, perhaps something like:
class ConsumptionSearch
attr_reader :date_from, :date_to
def initialize(params)
params ||= {}
@date_from = parsed_date(params[:date_from],Time.now.beginning_of_month.to_date.to_s)
@date_to = parsed_date(params[:date_to], (Date.today + 1).to_s)
end
def date_range
Consumption.where('created_at BETWEEN ? AND ?', @date_from, @date_to)
end
def price
...
end
def total_km
...
end
def price_per_km(round_to)
(price/total_km).round(round_to)
end
private
def parsed_date(date_string, default)
Date.parse(date_string)
rescue ArgumentError, TypeError
default
end
end
And then in view:
<%= @consumptions.price %>
<%= @consumptions.total_km %>
<%= @consumptions.price_per_km(4) %>
<%= @consumptions.price_per_km(2) %>
Personally, helpers
are not my favorite thing. But, I realize that some people use them regularly and with great success. I'm just odd that way.
add a comment |
Why not add the logic to your ConsumptionSearch
class? You are already abstracting logic to it. And, you are already accessing it in the view. So, perhaps something like:
class ConsumptionSearch
attr_reader :date_from, :date_to
def initialize(params)
params ||= {}
@date_from = parsed_date(params[:date_from],Time.now.beginning_of_month.to_date.to_s)
@date_to = parsed_date(params[:date_to], (Date.today + 1).to_s)
end
def date_range
Consumption.where('created_at BETWEEN ? AND ?', @date_from, @date_to)
end
def price
...
end
def total_km
...
end
def price_per_km(round_to)
(price/total_km).round(round_to)
end
private
def parsed_date(date_string, default)
Date.parse(date_string)
rescue ArgumentError, TypeError
default
end
end
And then in view:
<%= @consumptions.price %>
<%= @consumptions.total_km %>
<%= @consumptions.price_per_km(4) %>
<%= @consumptions.price_per_km(2) %>
Personally, helpers
are not my favorite thing. But, I realize that some people use them regularly and with great success. I'm just odd that way.
Why not add the logic to your ConsumptionSearch
class? You are already abstracting logic to it. And, you are already accessing it in the view. So, perhaps something like:
class ConsumptionSearch
attr_reader :date_from, :date_to
def initialize(params)
params ||= {}
@date_from = parsed_date(params[:date_from],Time.now.beginning_of_month.to_date.to_s)
@date_to = parsed_date(params[:date_to], (Date.today + 1).to_s)
end
def date_range
Consumption.where('created_at BETWEEN ? AND ?', @date_from, @date_to)
end
def price
...
end
def total_km
...
end
def price_per_km(round_to)
(price/total_km).round(round_to)
end
private
def parsed_date(date_string, default)
Date.parse(date_string)
rescue ArgumentError, TypeError
default
end
end
And then in view:
<%= @consumptions.price %>
<%= @consumptions.total_km %>
<%= @consumptions.price_per_km(4) %>
<%= @consumptions.price_per_km(2) %>
Personally, helpers
are not my favorite thing. But, I realize that some people use them regularly and with great success. I'm just odd that way.
answered Nov 12 at 17:24
jvillian
12.2k52135
12.2k52135
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53266564%2fhow-to-extract-logic-from-views%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
fYL1w633j9Zf Q3wdivET84J4cxOCU,gW,0NhsMf,NYNGvm,KhCe EmwqCVFXhd,RjJ,iTVzOkYfBOwRcjAS75WVxyzDckBbt HKwQ9YIk7
Hint: Helper methods or methods on the model that can help compute things.
– tadman
Nov 12 at 16:51