I'm having hard time fetching data using request.POST.get()
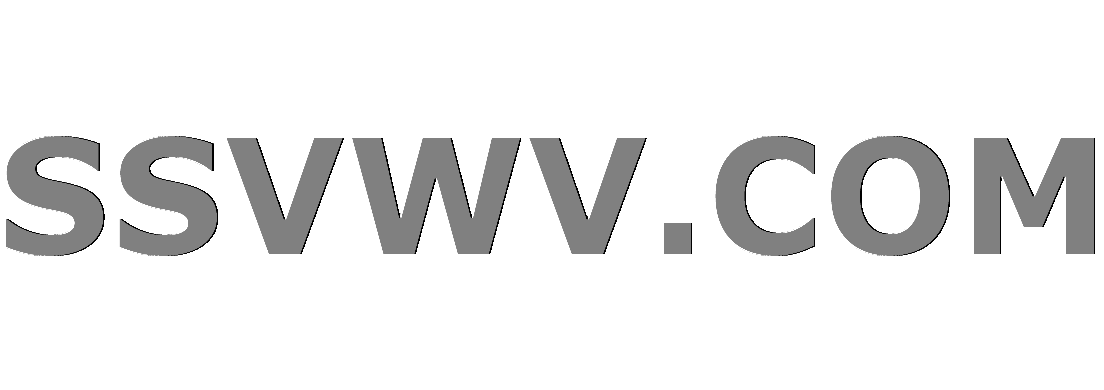
Multi tool use
I'm currently making a very basic dummy social networking site to learn Django, and I'm having trouble with request.POST.get() which is not fetching anything. As this way, I'm unable to have user inputs in my view.py. which means my site can't register any user. Please help me out as all of my further learning depends upon fetching user input. My coding is like (i know it's very basic and could look messy or somewhat outdated, sorry for that):
models.py:
class User(models.Model):
first_name= models.CharField(max_length=200)
last_name = models.CharField(max_length=200)
email = models.CharField(max_length=250)
password = models.CharField(max_length=250)
gender = models.CharField(max_length=250)
flag = models.IntegerField(default=0)
views.py:
def register_user(request):
user = User()
user.first_name = request.POST.get('firstName')
user.last_name = request.POST.get('lastName')
user.email = request.POST.get('email')
user.password = request.POST.get('password')
user.gender = request.POST.get('gender')
user.save()
return redirect('/login/')
urls.py
from django.conf.urls import url
from . import views
app_name = 'signup'
urlpatterns = [
url('^$', views.signup, name='signup'),
url('^login/$', views.register_user, name='register_user'),
]
signup/index.html:
<form action="{% url 'signup:register_user' %}" method="POST">
<div class="name">
<table class="nameTable">
<tr class="nameRow">
<td>
<p class="nameCSS">First Name</p>
</td>
<td>
<p class="lastName nameCSS">Last Name</p>
</td>
</tr>
<tr class="nameRow">
<td>
<input type="text" class="input" name="firstName">
</td>
<td>
<input type="text" class="input lastName" name="lastName">
</td>
</tr>
</table>
</div>
<div>
<p class="text">Email</p>
</div>
<div>
<input type="email" class="input largeInput" name="email">
</div>
<div>
<p class="text">Password</p>
</div>
<div>
<input type="password" class="input largeInput" name="password">
</div>
<div class="signUpDiv">
<button class="signUpButton" type="submit">Sign Up</button>
</div>
(there's a lot more in index.html, but for better short post's sake I've included just a little of its code and yes, I'm not using built-in forms class to make this form, but I've made my own template which this form coding is in. Hope I could do this and the problem isn't there)
When this code is run, no data entry is made. Whereas, if I run this method from shell using static values, it works fine. Thanks in advance
django
|
show 2 more comments
I'm currently making a very basic dummy social networking site to learn Django, and I'm having trouble with request.POST.get() which is not fetching anything. As this way, I'm unable to have user inputs in my view.py. which means my site can't register any user. Please help me out as all of my further learning depends upon fetching user input. My coding is like (i know it's very basic and could look messy or somewhat outdated, sorry for that):
models.py:
class User(models.Model):
first_name= models.CharField(max_length=200)
last_name = models.CharField(max_length=200)
email = models.CharField(max_length=250)
password = models.CharField(max_length=250)
gender = models.CharField(max_length=250)
flag = models.IntegerField(default=0)
views.py:
def register_user(request):
user = User()
user.first_name = request.POST.get('firstName')
user.last_name = request.POST.get('lastName')
user.email = request.POST.get('email')
user.password = request.POST.get('password')
user.gender = request.POST.get('gender')
user.save()
return redirect('/login/')
urls.py
from django.conf.urls import url
from . import views
app_name = 'signup'
urlpatterns = [
url('^$', views.signup, name='signup'),
url('^login/$', views.register_user, name='register_user'),
]
signup/index.html:
<form action="{% url 'signup:register_user' %}" method="POST">
<div class="name">
<table class="nameTable">
<tr class="nameRow">
<td>
<p class="nameCSS">First Name</p>
</td>
<td>
<p class="lastName nameCSS">Last Name</p>
</td>
</tr>
<tr class="nameRow">
<td>
<input type="text" class="input" name="firstName">
</td>
<td>
<input type="text" class="input lastName" name="lastName">
</td>
</tr>
</table>
</div>
<div>
<p class="text">Email</p>
</div>
<div>
<input type="email" class="input largeInput" name="email">
</div>
<div>
<p class="text">Password</p>
</div>
<div>
<input type="password" class="input largeInput" name="password">
</div>
<div class="signUpDiv">
<button class="signUpButton" type="submit">Sign Up</button>
</div>
(there's a lot more in index.html, but for better short post's sake I've included just a little of its code and yes, I'm not using built-in forms class to make this form, but I've made my own template which this form coding is in. Hope I could do this and the problem isn't there)
When this code is run, no data entry is made. Whereas, if I run this method from shell using static values, it works fine. Thanks in advance
django
are you using a form for this?
– RHSmith159
Nov 12 at 16:49
1
There's not nearly enough information here. Are you actually sending data in a POST? You'll need to show how you are doing that; presumably a template with a form, in which case you need to post that template, the URLs, and the view that displays the form in the first place.
– Daniel Roseman
Nov 12 at 17:01
Use modelform docs.djangoproject.com/fr/2.1/topics/forms/modelforms and form.is_valid to get & validate your inputs
– Bast
Nov 12 at 17:10
Great tuto here => simpleisbetterthancomplex.com/tutorial/2017/02/18/…
– Bast
Nov 12 at 17:11
When you say - no data is entered, do you get any errors? (off-course you did not described how you are trying to call your register_user function). use try/catch to capture errors.
– Sopan
Nov 12 at 17:12
|
show 2 more comments
I'm currently making a very basic dummy social networking site to learn Django, and I'm having trouble with request.POST.get() which is not fetching anything. As this way, I'm unable to have user inputs in my view.py. which means my site can't register any user. Please help me out as all of my further learning depends upon fetching user input. My coding is like (i know it's very basic and could look messy or somewhat outdated, sorry for that):
models.py:
class User(models.Model):
first_name= models.CharField(max_length=200)
last_name = models.CharField(max_length=200)
email = models.CharField(max_length=250)
password = models.CharField(max_length=250)
gender = models.CharField(max_length=250)
flag = models.IntegerField(default=0)
views.py:
def register_user(request):
user = User()
user.first_name = request.POST.get('firstName')
user.last_name = request.POST.get('lastName')
user.email = request.POST.get('email')
user.password = request.POST.get('password')
user.gender = request.POST.get('gender')
user.save()
return redirect('/login/')
urls.py
from django.conf.urls import url
from . import views
app_name = 'signup'
urlpatterns = [
url('^$', views.signup, name='signup'),
url('^login/$', views.register_user, name='register_user'),
]
signup/index.html:
<form action="{% url 'signup:register_user' %}" method="POST">
<div class="name">
<table class="nameTable">
<tr class="nameRow">
<td>
<p class="nameCSS">First Name</p>
</td>
<td>
<p class="lastName nameCSS">Last Name</p>
</td>
</tr>
<tr class="nameRow">
<td>
<input type="text" class="input" name="firstName">
</td>
<td>
<input type="text" class="input lastName" name="lastName">
</td>
</tr>
</table>
</div>
<div>
<p class="text">Email</p>
</div>
<div>
<input type="email" class="input largeInput" name="email">
</div>
<div>
<p class="text">Password</p>
</div>
<div>
<input type="password" class="input largeInput" name="password">
</div>
<div class="signUpDiv">
<button class="signUpButton" type="submit">Sign Up</button>
</div>
(there's a lot more in index.html, but for better short post's sake I've included just a little of its code and yes, I'm not using built-in forms class to make this form, but I've made my own template which this form coding is in. Hope I could do this and the problem isn't there)
When this code is run, no data entry is made. Whereas, if I run this method from shell using static values, it works fine. Thanks in advance
django
I'm currently making a very basic dummy social networking site to learn Django, and I'm having trouble with request.POST.get() which is not fetching anything. As this way, I'm unable to have user inputs in my view.py. which means my site can't register any user. Please help me out as all of my further learning depends upon fetching user input. My coding is like (i know it's very basic and could look messy or somewhat outdated, sorry for that):
models.py:
class User(models.Model):
first_name= models.CharField(max_length=200)
last_name = models.CharField(max_length=200)
email = models.CharField(max_length=250)
password = models.CharField(max_length=250)
gender = models.CharField(max_length=250)
flag = models.IntegerField(default=0)
views.py:
def register_user(request):
user = User()
user.first_name = request.POST.get('firstName')
user.last_name = request.POST.get('lastName')
user.email = request.POST.get('email')
user.password = request.POST.get('password')
user.gender = request.POST.get('gender')
user.save()
return redirect('/login/')
urls.py
from django.conf.urls import url
from . import views
app_name = 'signup'
urlpatterns = [
url('^$', views.signup, name='signup'),
url('^login/$', views.register_user, name='register_user'),
]
signup/index.html:
<form action="{% url 'signup:register_user' %}" method="POST">
<div class="name">
<table class="nameTable">
<tr class="nameRow">
<td>
<p class="nameCSS">First Name</p>
</td>
<td>
<p class="lastName nameCSS">Last Name</p>
</td>
</tr>
<tr class="nameRow">
<td>
<input type="text" class="input" name="firstName">
</td>
<td>
<input type="text" class="input lastName" name="lastName">
</td>
</tr>
</table>
</div>
<div>
<p class="text">Email</p>
</div>
<div>
<input type="email" class="input largeInput" name="email">
</div>
<div>
<p class="text">Password</p>
</div>
<div>
<input type="password" class="input largeInput" name="password">
</div>
<div class="signUpDiv">
<button class="signUpButton" type="submit">Sign Up</button>
</div>
(there's a lot more in index.html, but for better short post's sake I've included just a little of its code and yes, I'm not using built-in forms class to make this form, but I've made my own template which this form coding is in. Hope I could do this and the problem isn't there)
When this code is run, no data entry is made. Whereas, if I run this method from shell using static values, it works fine. Thanks in advance
django
django
edited Nov 12 at 17:46
asked Nov 12 at 16:45


Abhishek Kwatra
12
12
are you using a form for this?
– RHSmith159
Nov 12 at 16:49
1
There's not nearly enough information here. Are you actually sending data in a POST? You'll need to show how you are doing that; presumably a template with a form, in which case you need to post that template, the URLs, and the view that displays the form in the first place.
– Daniel Roseman
Nov 12 at 17:01
Use modelform docs.djangoproject.com/fr/2.1/topics/forms/modelforms and form.is_valid to get & validate your inputs
– Bast
Nov 12 at 17:10
Great tuto here => simpleisbetterthancomplex.com/tutorial/2017/02/18/…
– Bast
Nov 12 at 17:11
When you say - no data is entered, do you get any errors? (off-course you did not described how you are trying to call your register_user function). use try/catch to capture errors.
– Sopan
Nov 12 at 17:12
|
show 2 more comments
are you using a form for this?
– RHSmith159
Nov 12 at 16:49
1
There's not nearly enough information here. Are you actually sending data in a POST? You'll need to show how you are doing that; presumably a template with a form, in which case you need to post that template, the URLs, and the view that displays the form in the first place.
– Daniel Roseman
Nov 12 at 17:01
Use modelform docs.djangoproject.com/fr/2.1/topics/forms/modelforms and form.is_valid to get & validate your inputs
– Bast
Nov 12 at 17:10
Great tuto here => simpleisbetterthancomplex.com/tutorial/2017/02/18/…
– Bast
Nov 12 at 17:11
When you say - no data is entered, do you get any errors? (off-course you did not described how you are trying to call your register_user function). use try/catch to capture errors.
– Sopan
Nov 12 at 17:12
are you using a form for this?
– RHSmith159
Nov 12 at 16:49
are you using a form for this?
– RHSmith159
Nov 12 at 16:49
1
1
There's not nearly enough information here. Are you actually sending data in a POST? You'll need to show how you are doing that; presumably a template with a form, in which case you need to post that template, the URLs, and the view that displays the form in the first place.
– Daniel Roseman
Nov 12 at 17:01
There's not nearly enough information here. Are you actually sending data in a POST? You'll need to show how you are doing that; presumably a template with a form, in which case you need to post that template, the URLs, and the view that displays the form in the first place.
– Daniel Roseman
Nov 12 at 17:01
Use modelform docs.djangoproject.com/fr/2.1/topics/forms/modelforms and form.is_valid to get & validate your inputs
– Bast
Nov 12 at 17:10
Use modelform docs.djangoproject.com/fr/2.1/topics/forms/modelforms and form.is_valid to get & validate your inputs
– Bast
Nov 12 at 17:10
Great tuto here => simpleisbetterthancomplex.com/tutorial/2017/02/18/…
– Bast
Nov 12 at 17:11
Great tuto here => simpleisbetterthancomplex.com/tutorial/2017/02/18/…
– Bast
Nov 12 at 17:11
When you say - no data is entered, do you get any errors? (off-course you did not described how you are trying to call your register_user function). use try/catch to capture errors.
– Sopan
Nov 12 at 17:12
When you say - no data is entered, do you get any errors? (off-course you did not described how you are trying to call your register_user function). use try/catch to capture errors.
– Sopan
Nov 12 at 17:12
|
show 2 more comments
1 Answer
1
active
oldest
votes
Ideally, you should be using ModelForm for this scenario but I tried executing your code and it worked with slight changes.
Here are the changes as per my opinion:
You forgot to put {% csrf_token %} in your template which will lead to the forbidden error during the submission of the form
I have updated the template code with the csrf_token.
<form action="{% url 'register_user' %}" method="POST">
{% csrf_token %}
<div class="name">
<table class="nameTable">
<tr class="nameRow">
<td>
<p class="nameCSS">First Name</p>
</td>
<td>
<p class="lastName nameCSS">Last Name</p>
</td>
</tr>
<tr class="nameRow">
<td>
<input type="text" class="input" name="firstName">
</td>
<td>
<input type="text" class="input lastName" name="lastName">
</td>
</tr>
</table>
</div>
<div>
<p class="text">Email</p>
</div>
<div>
<input type="email" class="input largeInput" name="email">
</div>
<div>
<p class="text">Password</p>
</div>
<div>
<input type="password" class="input largeInput" name="password">
</div>
<div class="signUpDiv">
<button class="signUpButton" type="submit">Sign Up</button>
</div>
You can use just one view to handle GET and POST request
I have updated the views just for the sake of this example, however, make sure that you are encrypting the password before saving it (use Django's default user model) and use
user.set_password('PASSWORD_HERE')
Updated the code for views.py
def register_user(request):
if request.POST:
user = User()
user.first_name = request.POST.get('firstName')
user.last_name = request.POST.get('lastName')
user.email = request.POST.get('email')
user.password = request.POST.get('password')
user.gender = request.POST.get('gender')
user.save()
else:
return render(request, "YOUR_TEMPLATE_NAME_HERE")
Also make sure that your url name is correct i.e register_user
I hope this helps:)
it's still not working. i don't know what's wrong with my code, but it doesn't work
– Abhishek Kwatra
Dec 4 at 7:14
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53266578%2fim-having-hard-time-fetching-data-using-request-post-get%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Ideally, you should be using ModelForm for this scenario but I tried executing your code and it worked with slight changes.
Here are the changes as per my opinion:
You forgot to put {% csrf_token %} in your template which will lead to the forbidden error during the submission of the form
I have updated the template code with the csrf_token.
<form action="{% url 'register_user' %}" method="POST">
{% csrf_token %}
<div class="name">
<table class="nameTable">
<tr class="nameRow">
<td>
<p class="nameCSS">First Name</p>
</td>
<td>
<p class="lastName nameCSS">Last Name</p>
</td>
</tr>
<tr class="nameRow">
<td>
<input type="text" class="input" name="firstName">
</td>
<td>
<input type="text" class="input lastName" name="lastName">
</td>
</tr>
</table>
</div>
<div>
<p class="text">Email</p>
</div>
<div>
<input type="email" class="input largeInput" name="email">
</div>
<div>
<p class="text">Password</p>
</div>
<div>
<input type="password" class="input largeInput" name="password">
</div>
<div class="signUpDiv">
<button class="signUpButton" type="submit">Sign Up</button>
</div>
You can use just one view to handle GET and POST request
I have updated the views just for the sake of this example, however, make sure that you are encrypting the password before saving it (use Django's default user model) and use
user.set_password('PASSWORD_HERE')
Updated the code for views.py
def register_user(request):
if request.POST:
user = User()
user.first_name = request.POST.get('firstName')
user.last_name = request.POST.get('lastName')
user.email = request.POST.get('email')
user.password = request.POST.get('password')
user.gender = request.POST.get('gender')
user.save()
else:
return render(request, "YOUR_TEMPLATE_NAME_HERE")
Also make sure that your url name is correct i.e register_user
I hope this helps:)
it's still not working. i don't know what's wrong with my code, but it doesn't work
– Abhishek Kwatra
Dec 4 at 7:14
add a comment |
Ideally, you should be using ModelForm for this scenario but I tried executing your code and it worked with slight changes.
Here are the changes as per my opinion:
You forgot to put {% csrf_token %} in your template which will lead to the forbidden error during the submission of the form
I have updated the template code with the csrf_token.
<form action="{% url 'register_user' %}" method="POST">
{% csrf_token %}
<div class="name">
<table class="nameTable">
<tr class="nameRow">
<td>
<p class="nameCSS">First Name</p>
</td>
<td>
<p class="lastName nameCSS">Last Name</p>
</td>
</tr>
<tr class="nameRow">
<td>
<input type="text" class="input" name="firstName">
</td>
<td>
<input type="text" class="input lastName" name="lastName">
</td>
</tr>
</table>
</div>
<div>
<p class="text">Email</p>
</div>
<div>
<input type="email" class="input largeInput" name="email">
</div>
<div>
<p class="text">Password</p>
</div>
<div>
<input type="password" class="input largeInput" name="password">
</div>
<div class="signUpDiv">
<button class="signUpButton" type="submit">Sign Up</button>
</div>
You can use just one view to handle GET and POST request
I have updated the views just for the sake of this example, however, make sure that you are encrypting the password before saving it (use Django's default user model) and use
user.set_password('PASSWORD_HERE')
Updated the code for views.py
def register_user(request):
if request.POST:
user = User()
user.first_name = request.POST.get('firstName')
user.last_name = request.POST.get('lastName')
user.email = request.POST.get('email')
user.password = request.POST.get('password')
user.gender = request.POST.get('gender')
user.save()
else:
return render(request, "YOUR_TEMPLATE_NAME_HERE")
Also make sure that your url name is correct i.e register_user
I hope this helps:)
it's still not working. i don't know what's wrong with my code, but it doesn't work
– Abhishek Kwatra
Dec 4 at 7:14
add a comment |
Ideally, you should be using ModelForm for this scenario but I tried executing your code and it worked with slight changes.
Here are the changes as per my opinion:
You forgot to put {% csrf_token %} in your template which will lead to the forbidden error during the submission of the form
I have updated the template code with the csrf_token.
<form action="{% url 'register_user' %}" method="POST">
{% csrf_token %}
<div class="name">
<table class="nameTable">
<tr class="nameRow">
<td>
<p class="nameCSS">First Name</p>
</td>
<td>
<p class="lastName nameCSS">Last Name</p>
</td>
</tr>
<tr class="nameRow">
<td>
<input type="text" class="input" name="firstName">
</td>
<td>
<input type="text" class="input lastName" name="lastName">
</td>
</tr>
</table>
</div>
<div>
<p class="text">Email</p>
</div>
<div>
<input type="email" class="input largeInput" name="email">
</div>
<div>
<p class="text">Password</p>
</div>
<div>
<input type="password" class="input largeInput" name="password">
</div>
<div class="signUpDiv">
<button class="signUpButton" type="submit">Sign Up</button>
</div>
You can use just one view to handle GET and POST request
I have updated the views just for the sake of this example, however, make sure that you are encrypting the password before saving it (use Django's default user model) and use
user.set_password('PASSWORD_HERE')
Updated the code for views.py
def register_user(request):
if request.POST:
user = User()
user.first_name = request.POST.get('firstName')
user.last_name = request.POST.get('lastName')
user.email = request.POST.get('email')
user.password = request.POST.get('password')
user.gender = request.POST.get('gender')
user.save()
else:
return render(request, "YOUR_TEMPLATE_NAME_HERE")
Also make sure that your url name is correct i.e register_user
I hope this helps:)
Ideally, you should be using ModelForm for this scenario but I tried executing your code and it worked with slight changes.
Here are the changes as per my opinion:
You forgot to put {% csrf_token %} in your template which will lead to the forbidden error during the submission of the form
I have updated the template code with the csrf_token.
<form action="{% url 'register_user' %}" method="POST">
{% csrf_token %}
<div class="name">
<table class="nameTable">
<tr class="nameRow">
<td>
<p class="nameCSS">First Name</p>
</td>
<td>
<p class="lastName nameCSS">Last Name</p>
</td>
</tr>
<tr class="nameRow">
<td>
<input type="text" class="input" name="firstName">
</td>
<td>
<input type="text" class="input lastName" name="lastName">
</td>
</tr>
</table>
</div>
<div>
<p class="text">Email</p>
</div>
<div>
<input type="email" class="input largeInput" name="email">
</div>
<div>
<p class="text">Password</p>
</div>
<div>
<input type="password" class="input largeInput" name="password">
</div>
<div class="signUpDiv">
<button class="signUpButton" type="submit">Sign Up</button>
</div>
You can use just one view to handle GET and POST request
I have updated the views just for the sake of this example, however, make sure that you are encrypting the password before saving it (use Django's default user model) and use
user.set_password('PASSWORD_HERE')
Updated the code for views.py
def register_user(request):
if request.POST:
user = User()
user.first_name = request.POST.get('firstName')
user.last_name = request.POST.get('lastName')
user.email = request.POST.get('email')
user.password = request.POST.get('password')
user.gender = request.POST.get('gender')
user.save()
else:
return render(request, "YOUR_TEMPLATE_NAME_HERE")
Also make sure that your url name is correct i.e register_user
I hope this helps:)
answered Nov 12 at 18:12
Himanshu Nachane
262
262
it's still not working. i don't know what's wrong with my code, but it doesn't work
– Abhishek Kwatra
Dec 4 at 7:14
add a comment |
it's still not working. i don't know what's wrong with my code, but it doesn't work
– Abhishek Kwatra
Dec 4 at 7:14
it's still not working. i don't know what's wrong with my code, but it doesn't work
– Abhishek Kwatra
Dec 4 at 7:14
it's still not working. i don't know what's wrong with my code, but it doesn't work
– Abhishek Kwatra
Dec 4 at 7:14
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53266578%2fim-having-hard-time-fetching-data-using-request-post-get%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
hvtx4fFEkm8shHyf,73zIm1Xulmz,cQTq VOBNF8YKyZRQKM1Km9bQ,0QI KisxkkrG8Z7FU2wYG tBC5wQE9m,i3tze9
are you using a form for this?
– RHSmith159
Nov 12 at 16:49
1
There's not nearly enough information here. Are you actually sending data in a POST? You'll need to show how you are doing that; presumably a template with a form, in which case you need to post that template, the URLs, and the view that displays the form in the first place.
– Daniel Roseman
Nov 12 at 17:01
Use modelform docs.djangoproject.com/fr/2.1/topics/forms/modelforms and form.is_valid to get & validate your inputs
– Bast
Nov 12 at 17:10
Great tuto here => simpleisbetterthancomplex.com/tutorial/2017/02/18/…
– Bast
Nov 12 at 17:11
When you say - no data is entered, do you get any errors? (off-course you did not described how you are trying to call your register_user function). use try/catch to capture errors.
– Sopan
Nov 12 at 17:12