Using initial labels causes exception in OpenCV kmeans
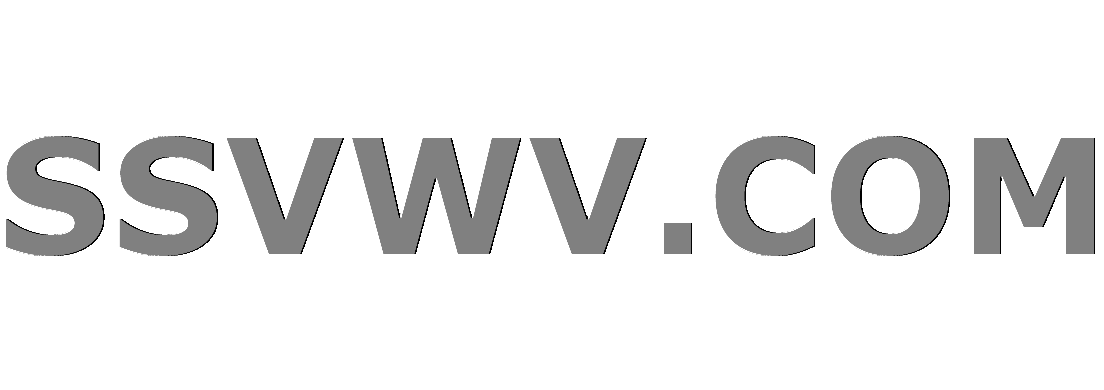
Multi tool use
up vote
0
down vote
favorite
I'm using OpenCV kmeans alghoritm to compute some kind of histogram.
In the first stage, I'm taking some feautres extracted from my train data, and I'm putting it into one of cv::kmeans functions params.
The output of cv::kmeans gives me two different outputs.
- labels computed for my data
- centers, calcualted during executing cv::kmeans
In the next stage, I would like to compute labels for my test data, but using some data computed in the previous stage, but if I set KMEANS_USE_INITIAL_LABELS flag and pass labels computed in previous stage it produces an exception with message:
error: (-215:Assertion failed) (unsigned)_labels.at(i) < (unsigned)K in function 'kmeans'
Here is my wrapper method for cv::kmeans
void Kmeans::fit_predict(cv::InputArray in, cv::InputOutputArray out)
{
// in is and input, my sample/s descriptors
// out -> computed labels; in first stage this array is empty
int flag = cv::KMEANS_PP_CENTERS;
if (!out.empty()) {
flag = cv::KMEANS_USE_INITIAL_LABELS;
cv::Mat m;
out.copyTo(m);
std::cout << m; // M has appropriate values, each value is < K(clusters amount)
}
cv::kmeans(in, _clusters_count, out, _term_criteria, _attempts, flag, _centers);
}
How I'm calling kmeans method(which is a part of my Kmeans class)
auto kmeans = std::make_unique<Kmeans>();
cv::Mat my_train_data .......initializing etc...
cv::Mat labels;
std::vector<Sample> test_samples ........initializng etc....
kmeans->fit_predict(my_train_data, labels);
for (auto& s : test_samples) {
cv::Mat test_labels = labels.clone();
kmeans->fit_predict(s->getDescriptors(), test_labels);
/// Process outputs etc...
}
Here are parameters with I'm calling cv::kmeans
in -> defined above
_clusters_count -> it is property of my class, it is 100
out -> defined above
_term_criteria -> stored as property of my class, it has value:
cv::TermCriteria(cv::TermCriteria::MAX_ITER + cv::TermCriteria::EPS, 300, 0.0001)flag -> _attempts -> property of my class, it is 20
_centers -> property of my class, it has default value: cv::noArray()
Why I'm getting this error?
c++ opencv machine-learning
add a comment |
up vote
0
down vote
favorite
I'm using OpenCV kmeans alghoritm to compute some kind of histogram.
In the first stage, I'm taking some feautres extracted from my train data, and I'm putting it into one of cv::kmeans functions params.
The output of cv::kmeans gives me two different outputs.
- labels computed for my data
- centers, calcualted during executing cv::kmeans
In the next stage, I would like to compute labels for my test data, but using some data computed in the previous stage, but if I set KMEANS_USE_INITIAL_LABELS flag and pass labels computed in previous stage it produces an exception with message:
error: (-215:Assertion failed) (unsigned)_labels.at(i) < (unsigned)K in function 'kmeans'
Here is my wrapper method for cv::kmeans
void Kmeans::fit_predict(cv::InputArray in, cv::InputOutputArray out)
{
// in is and input, my sample/s descriptors
// out -> computed labels; in first stage this array is empty
int flag = cv::KMEANS_PP_CENTERS;
if (!out.empty()) {
flag = cv::KMEANS_USE_INITIAL_LABELS;
cv::Mat m;
out.copyTo(m);
std::cout << m; // M has appropriate values, each value is < K(clusters amount)
}
cv::kmeans(in, _clusters_count, out, _term_criteria, _attempts, flag, _centers);
}
How I'm calling kmeans method(which is a part of my Kmeans class)
auto kmeans = std::make_unique<Kmeans>();
cv::Mat my_train_data .......initializing etc...
cv::Mat labels;
std::vector<Sample> test_samples ........initializng etc....
kmeans->fit_predict(my_train_data, labels);
for (auto& s : test_samples) {
cv::Mat test_labels = labels.clone();
kmeans->fit_predict(s->getDescriptors(), test_labels);
/// Process outputs etc...
}
Here are parameters with I'm calling cv::kmeans
in -> defined above
_clusters_count -> it is property of my class, it is 100
out -> defined above
_term_criteria -> stored as property of my class, it has value:
cv::TermCriteria(cv::TermCriteria::MAX_ITER + cv::TermCriteria::EPS, 300, 0.0001)flag -> _attempts -> property of my class, it is 20
_centers -> property of my class, it has default value: cv::noArray()
Why I'm getting this error?
c++ opencv machine-learning
Please, provide a Minimal, Complete, and Verifiable example (i.e. something that we can just compile without having to add anything) that will let us reproduce this problem. What version of OpenCV is this happening with?
– Dan Mašek
Nov 10 at 22:38
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm using OpenCV kmeans alghoritm to compute some kind of histogram.
In the first stage, I'm taking some feautres extracted from my train data, and I'm putting it into one of cv::kmeans functions params.
The output of cv::kmeans gives me two different outputs.
- labels computed for my data
- centers, calcualted during executing cv::kmeans
In the next stage, I would like to compute labels for my test data, but using some data computed in the previous stage, but if I set KMEANS_USE_INITIAL_LABELS flag and pass labels computed in previous stage it produces an exception with message:
error: (-215:Assertion failed) (unsigned)_labels.at(i) < (unsigned)K in function 'kmeans'
Here is my wrapper method for cv::kmeans
void Kmeans::fit_predict(cv::InputArray in, cv::InputOutputArray out)
{
// in is and input, my sample/s descriptors
// out -> computed labels; in first stage this array is empty
int flag = cv::KMEANS_PP_CENTERS;
if (!out.empty()) {
flag = cv::KMEANS_USE_INITIAL_LABELS;
cv::Mat m;
out.copyTo(m);
std::cout << m; // M has appropriate values, each value is < K(clusters amount)
}
cv::kmeans(in, _clusters_count, out, _term_criteria, _attempts, flag, _centers);
}
How I'm calling kmeans method(which is a part of my Kmeans class)
auto kmeans = std::make_unique<Kmeans>();
cv::Mat my_train_data .......initializing etc...
cv::Mat labels;
std::vector<Sample> test_samples ........initializng etc....
kmeans->fit_predict(my_train_data, labels);
for (auto& s : test_samples) {
cv::Mat test_labels = labels.clone();
kmeans->fit_predict(s->getDescriptors(), test_labels);
/// Process outputs etc...
}
Here are parameters with I'm calling cv::kmeans
in -> defined above
_clusters_count -> it is property of my class, it is 100
out -> defined above
_term_criteria -> stored as property of my class, it has value:
cv::TermCriteria(cv::TermCriteria::MAX_ITER + cv::TermCriteria::EPS, 300, 0.0001)flag -> _attempts -> property of my class, it is 20
_centers -> property of my class, it has default value: cv::noArray()
Why I'm getting this error?
c++ opencv machine-learning
I'm using OpenCV kmeans alghoritm to compute some kind of histogram.
In the first stage, I'm taking some feautres extracted from my train data, and I'm putting it into one of cv::kmeans functions params.
The output of cv::kmeans gives me two different outputs.
- labels computed for my data
- centers, calcualted during executing cv::kmeans
In the next stage, I would like to compute labels for my test data, but using some data computed in the previous stage, but if I set KMEANS_USE_INITIAL_LABELS flag and pass labels computed in previous stage it produces an exception with message:
error: (-215:Assertion failed) (unsigned)_labels.at(i) < (unsigned)K in function 'kmeans'
Here is my wrapper method for cv::kmeans
void Kmeans::fit_predict(cv::InputArray in, cv::InputOutputArray out)
{
// in is and input, my sample/s descriptors
// out -> computed labels; in first stage this array is empty
int flag = cv::KMEANS_PP_CENTERS;
if (!out.empty()) {
flag = cv::KMEANS_USE_INITIAL_LABELS;
cv::Mat m;
out.copyTo(m);
std::cout << m; // M has appropriate values, each value is < K(clusters amount)
}
cv::kmeans(in, _clusters_count, out, _term_criteria, _attempts, flag, _centers);
}
How I'm calling kmeans method(which is a part of my Kmeans class)
auto kmeans = std::make_unique<Kmeans>();
cv::Mat my_train_data .......initializing etc...
cv::Mat labels;
std::vector<Sample> test_samples ........initializng etc....
kmeans->fit_predict(my_train_data, labels);
for (auto& s : test_samples) {
cv::Mat test_labels = labels.clone();
kmeans->fit_predict(s->getDescriptors(), test_labels);
/// Process outputs etc...
}
Here are parameters with I'm calling cv::kmeans
in -> defined above
_clusters_count -> it is property of my class, it is 100
out -> defined above
_term_criteria -> stored as property of my class, it has value:
cv::TermCriteria(cv::TermCriteria::MAX_ITER + cv::TermCriteria::EPS, 300, 0.0001)flag -> _attempts -> property of my class, it is 20
_centers -> property of my class, it has default value: cv::noArray()
Why I'm getting this error?
c++ opencv machine-learning
c++ opencv machine-learning
asked Nov 10 at 22:05
bielu000
19415
19415
Please, provide a Minimal, Complete, and Verifiable example (i.e. something that we can just compile without having to add anything) that will let us reproduce this problem. What version of OpenCV is this happening with?
– Dan Mašek
Nov 10 at 22:38
add a comment |
Please, provide a Minimal, Complete, and Verifiable example (i.e. something that we can just compile without having to add anything) that will let us reproduce this problem. What version of OpenCV is this happening with?
– Dan Mašek
Nov 10 at 22:38
Please, provide a Minimal, Complete, and Verifiable example (i.e. something that we can just compile without having to add anything) that will let us reproduce this problem. What version of OpenCV is this happening with?
– Dan Mašek
Nov 10 at 22:38
Please, provide a Minimal, Complete, and Verifiable example (i.e. something that we can just compile without having to add anything) that will let us reproduce this problem. What version of OpenCV is this happening with?
– Dan Mašek
Nov 10 at 22:38
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53243878%2fusing-initial-labels-causes-exception-in-opencv-kmeans%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
v4F,CUeu7Jcx47NEzhD1A,nz,6 jXF,hIVc3
Please, provide a Minimal, Complete, and Verifiable example (i.e. something that we can just compile without having to add anything) that will let us reproduce this problem. What version of OpenCV is this happening with?
– Dan Mašek
Nov 10 at 22:38