Selected option is not updated even after making the necessary changes
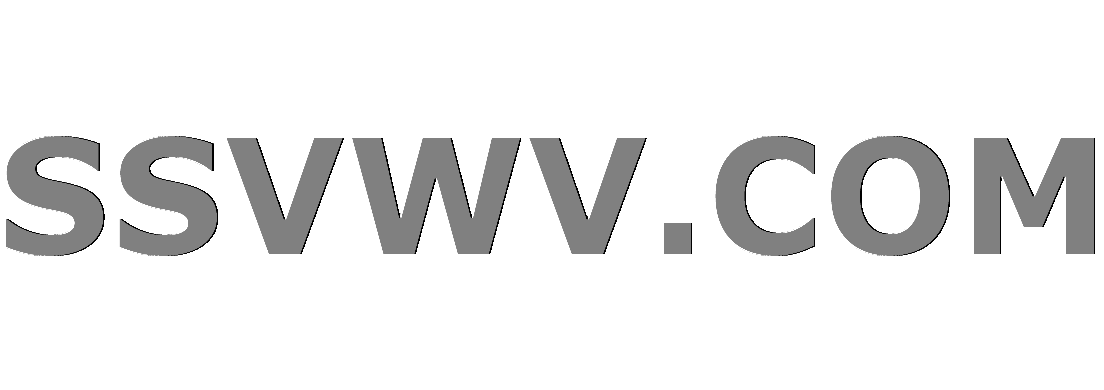
Multi tool use
up vote
1
down vote
favorite
I have a semester list dropdown that when changed (i.e. onChange) should call the following function that updates the list of semesters with respect to the newly selected semester:
function updatesemesterlist() {
var semesterlist = document.getElementById("semesterdropdown")
var updatedsemester = semesterlist.value
while(semesterlist.firstChild) {
semesterlist.removeChild(semesterlist.firstChild)
}
semesterparts = updatedsemester.split(" ")
var season = semesterparts[0]
var year = semesterparts[1]
if (season == "Fall") {
var ny = parseInt(year, 10)
ny += 1
nextyear = ny.toString()
var py = parseInt(year, 10)
py -= 1
prevyear = py.toString()
addnewoption(semesterlist, "Fall" + " " + nextyear)
addnewoption(semesterlist, "Spring" + " " + nextyear)
addnewoption(semesterlist, updatedsemester)
addnewoption(semesterlist, "Spring" + " " + year)
addnewoption(semesterlist, "Fall" + " " + prevyear)
}
else {
var ny = parseInt(year, 10)
ny += 1
nextyear = ny.toString()
var py = parseInt(year, 10)
py -= 1
prevyear = py.toString()
addnewoption(semesterlist, "Spring" + " " + nextyear)
addnewoption(semesterlist, "Fall" + " " + year)
addnewoption(semesterlist, updatedsemester)
addnewoption(semesterlist, "Fall" + " " + prevyear)
addnewoption(semesterlist, "Spring" + " " + prevyear)
}
semesterlist.value = updatedsemester
retrieveclasses(updatedsemester)
}
The function above the calls the retrieveclasses(semester) function, which updates the list of options selected based on data stored in a map structure:
function retrieveclasses(semester) {
classes = semestertoclasses.get(semester)
var table_body = document.getElementById("table of classes").children[1]
while(table_body.firstChild){
table_body.removeChild(table_body.firstChild)
}
console.log(classes)
if(classes != undefined ){
var count = 1
for(var key of Array.from(classes.keys())){
addclass()
var course = document.getElementById("course-select" + (count.toString()))
course.value = key
course.options[course.options.selectedIndex].selected = true
var requirement = document.getElementById("requirement-select" + (count.toString()))
requirement.value = classes.get(key)
requirement.options[requirement.options.selectedIndex].selected = true
count++
}
}
}
For reference, the map is updated with the following function:
function saveclasses(){
var table_body = document.getElementById("table of classes").children[1]
var num_classes = table_body.childElementCount
var semester = document.getElementById("semesterdropdown").value
var courses = table_body.children
var classtorequirement = new Map();
for (var i = 0; i < courses.length; i++){
var data = courses[i].children
classtorequirement.set(data[0].firstChild.children[3].innerHTML,
data[1].firstChild.children[3].innerHTML)
}
semestertoclasses.set(semester, classtorequirement)
}
Where semestertoclasses is initially defined at the top of my javascript file:
var semestertoclasses = new Map();
Now suppose I update the classes I want to take in the 'Fall 2018' semester and then save those courses with the 'Save Courses' button:
Fall 2018 Courses
Then I go to a new semester:
Spring 2019
When I go back to 'Fall 2018', the courses should be updated with the ones that I initially saved (CS 2800 and MATH 2940, as well as the corresponding requirements they fulfill). However, this is not the case:
After going back to Fall 2018
I debugged this issue by ensuring that the selected indices were correct (i.e. by logging options.selectedIndex to the console), and it was indeed correct. But the classes are not updated.
I'm having a hard time exactly figuring out what could cause this issue to occur. I appreciate any suggestions, thank you!
javascript html
add a comment |
up vote
1
down vote
favorite
I have a semester list dropdown that when changed (i.e. onChange) should call the following function that updates the list of semesters with respect to the newly selected semester:
function updatesemesterlist() {
var semesterlist = document.getElementById("semesterdropdown")
var updatedsemester = semesterlist.value
while(semesterlist.firstChild) {
semesterlist.removeChild(semesterlist.firstChild)
}
semesterparts = updatedsemester.split(" ")
var season = semesterparts[0]
var year = semesterparts[1]
if (season == "Fall") {
var ny = parseInt(year, 10)
ny += 1
nextyear = ny.toString()
var py = parseInt(year, 10)
py -= 1
prevyear = py.toString()
addnewoption(semesterlist, "Fall" + " " + nextyear)
addnewoption(semesterlist, "Spring" + " " + nextyear)
addnewoption(semesterlist, updatedsemester)
addnewoption(semesterlist, "Spring" + " " + year)
addnewoption(semesterlist, "Fall" + " " + prevyear)
}
else {
var ny = parseInt(year, 10)
ny += 1
nextyear = ny.toString()
var py = parseInt(year, 10)
py -= 1
prevyear = py.toString()
addnewoption(semesterlist, "Spring" + " " + nextyear)
addnewoption(semesterlist, "Fall" + " " + year)
addnewoption(semesterlist, updatedsemester)
addnewoption(semesterlist, "Fall" + " " + prevyear)
addnewoption(semesterlist, "Spring" + " " + prevyear)
}
semesterlist.value = updatedsemester
retrieveclasses(updatedsemester)
}
The function above the calls the retrieveclasses(semester) function, which updates the list of options selected based on data stored in a map structure:
function retrieveclasses(semester) {
classes = semestertoclasses.get(semester)
var table_body = document.getElementById("table of classes").children[1]
while(table_body.firstChild){
table_body.removeChild(table_body.firstChild)
}
console.log(classes)
if(classes != undefined ){
var count = 1
for(var key of Array.from(classes.keys())){
addclass()
var course = document.getElementById("course-select" + (count.toString()))
course.value = key
course.options[course.options.selectedIndex].selected = true
var requirement = document.getElementById("requirement-select" + (count.toString()))
requirement.value = classes.get(key)
requirement.options[requirement.options.selectedIndex].selected = true
count++
}
}
}
For reference, the map is updated with the following function:
function saveclasses(){
var table_body = document.getElementById("table of classes").children[1]
var num_classes = table_body.childElementCount
var semester = document.getElementById("semesterdropdown").value
var courses = table_body.children
var classtorequirement = new Map();
for (var i = 0; i < courses.length; i++){
var data = courses[i].children
classtorequirement.set(data[0].firstChild.children[3].innerHTML,
data[1].firstChild.children[3].innerHTML)
}
semestertoclasses.set(semester, classtorequirement)
}
Where semestertoclasses is initially defined at the top of my javascript file:
var semestertoclasses = new Map();
Now suppose I update the classes I want to take in the 'Fall 2018' semester and then save those courses with the 'Save Courses' button:
Fall 2018 Courses
Then I go to a new semester:
Spring 2019
When I go back to 'Fall 2018', the courses should be updated with the ones that I initially saved (CS 2800 and MATH 2940, as well as the corresponding requirements they fulfill). However, this is not the case:
After going back to Fall 2018
I debugged this issue by ensuring that the selected indices were correct (i.e. by logging options.selectedIndex to the console), and it was indeed correct. But the classes are not updated.
I'm having a hard time exactly figuring out what could cause this issue to occur. I appreciate any suggestions, thank you!
javascript html
Can you add your code to a jsFiddle?
– Simsteve7
Nov 9 at 22:33
jsfiddle.net/8sfpzL6c
– azdev
Nov 10 at 0:44
@Simsteve7 Please do not request code on external code sites. StackOverflow has snippet functionality which is what is required to use unless SO snippet functionality is not sufficient.
– connexo
Nov 10 at 22:20
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I have a semester list dropdown that when changed (i.e. onChange) should call the following function that updates the list of semesters with respect to the newly selected semester:
function updatesemesterlist() {
var semesterlist = document.getElementById("semesterdropdown")
var updatedsemester = semesterlist.value
while(semesterlist.firstChild) {
semesterlist.removeChild(semesterlist.firstChild)
}
semesterparts = updatedsemester.split(" ")
var season = semesterparts[0]
var year = semesterparts[1]
if (season == "Fall") {
var ny = parseInt(year, 10)
ny += 1
nextyear = ny.toString()
var py = parseInt(year, 10)
py -= 1
prevyear = py.toString()
addnewoption(semesterlist, "Fall" + " " + nextyear)
addnewoption(semesterlist, "Spring" + " " + nextyear)
addnewoption(semesterlist, updatedsemester)
addnewoption(semesterlist, "Spring" + " " + year)
addnewoption(semesterlist, "Fall" + " " + prevyear)
}
else {
var ny = parseInt(year, 10)
ny += 1
nextyear = ny.toString()
var py = parseInt(year, 10)
py -= 1
prevyear = py.toString()
addnewoption(semesterlist, "Spring" + " " + nextyear)
addnewoption(semesterlist, "Fall" + " " + year)
addnewoption(semesterlist, updatedsemester)
addnewoption(semesterlist, "Fall" + " " + prevyear)
addnewoption(semesterlist, "Spring" + " " + prevyear)
}
semesterlist.value = updatedsemester
retrieveclasses(updatedsemester)
}
The function above the calls the retrieveclasses(semester) function, which updates the list of options selected based on data stored in a map structure:
function retrieveclasses(semester) {
classes = semestertoclasses.get(semester)
var table_body = document.getElementById("table of classes").children[1]
while(table_body.firstChild){
table_body.removeChild(table_body.firstChild)
}
console.log(classes)
if(classes != undefined ){
var count = 1
for(var key of Array.from(classes.keys())){
addclass()
var course = document.getElementById("course-select" + (count.toString()))
course.value = key
course.options[course.options.selectedIndex].selected = true
var requirement = document.getElementById("requirement-select" + (count.toString()))
requirement.value = classes.get(key)
requirement.options[requirement.options.selectedIndex].selected = true
count++
}
}
}
For reference, the map is updated with the following function:
function saveclasses(){
var table_body = document.getElementById("table of classes").children[1]
var num_classes = table_body.childElementCount
var semester = document.getElementById("semesterdropdown").value
var courses = table_body.children
var classtorequirement = new Map();
for (var i = 0; i < courses.length; i++){
var data = courses[i].children
classtorequirement.set(data[0].firstChild.children[3].innerHTML,
data[1].firstChild.children[3].innerHTML)
}
semestertoclasses.set(semester, classtorequirement)
}
Where semestertoclasses is initially defined at the top of my javascript file:
var semestertoclasses = new Map();
Now suppose I update the classes I want to take in the 'Fall 2018' semester and then save those courses with the 'Save Courses' button:
Fall 2018 Courses
Then I go to a new semester:
Spring 2019
When I go back to 'Fall 2018', the courses should be updated with the ones that I initially saved (CS 2800 and MATH 2940, as well as the corresponding requirements they fulfill). However, this is not the case:
After going back to Fall 2018
I debugged this issue by ensuring that the selected indices were correct (i.e. by logging options.selectedIndex to the console), and it was indeed correct. But the classes are not updated.
I'm having a hard time exactly figuring out what could cause this issue to occur. I appreciate any suggestions, thank you!
javascript html
I have a semester list dropdown that when changed (i.e. onChange) should call the following function that updates the list of semesters with respect to the newly selected semester:
function updatesemesterlist() {
var semesterlist = document.getElementById("semesterdropdown")
var updatedsemester = semesterlist.value
while(semesterlist.firstChild) {
semesterlist.removeChild(semesterlist.firstChild)
}
semesterparts = updatedsemester.split(" ")
var season = semesterparts[0]
var year = semesterparts[1]
if (season == "Fall") {
var ny = parseInt(year, 10)
ny += 1
nextyear = ny.toString()
var py = parseInt(year, 10)
py -= 1
prevyear = py.toString()
addnewoption(semesterlist, "Fall" + " " + nextyear)
addnewoption(semesterlist, "Spring" + " " + nextyear)
addnewoption(semesterlist, updatedsemester)
addnewoption(semesterlist, "Spring" + " " + year)
addnewoption(semesterlist, "Fall" + " " + prevyear)
}
else {
var ny = parseInt(year, 10)
ny += 1
nextyear = ny.toString()
var py = parseInt(year, 10)
py -= 1
prevyear = py.toString()
addnewoption(semesterlist, "Spring" + " " + nextyear)
addnewoption(semesterlist, "Fall" + " " + year)
addnewoption(semesterlist, updatedsemester)
addnewoption(semesterlist, "Fall" + " " + prevyear)
addnewoption(semesterlist, "Spring" + " " + prevyear)
}
semesterlist.value = updatedsemester
retrieveclasses(updatedsemester)
}
The function above the calls the retrieveclasses(semester) function, which updates the list of options selected based on data stored in a map structure:
function retrieveclasses(semester) {
classes = semestertoclasses.get(semester)
var table_body = document.getElementById("table of classes").children[1]
while(table_body.firstChild){
table_body.removeChild(table_body.firstChild)
}
console.log(classes)
if(classes != undefined ){
var count = 1
for(var key of Array.from(classes.keys())){
addclass()
var course = document.getElementById("course-select" + (count.toString()))
course.value = key
course.options[course.options.selectedIndex].selected = true
var requirement = document.getElementById("requirement-select" + (count.toString()))
requirement.value = classes.get(key)
requirement.options[requirement.options.selectedIndex].selected = true
count++
}
}
}
For reference, the map is updated with the following function:
function saveclasses(){
var table_body = document.getElementById("table of classes").children[1]
var num_classes = table_body.childElementCount
var semester = document.getElementById("semesterdropdown").value
var courses = table_body.children
var classtorequirement = new Map();
for (var i = 0; i < courses.length; i++){
var data = courses[i].children
classtorequirement.set(data[0].firstChild.children[3].innerHTML,
data[1].firstChild.children[3].innerHTML)
}
semestertoclasses.set(semester, classtorequirement)
}
Where semestertoclasses is initially defined at the top of my javascript file:
var semestertoclasses = new Map();
Now suppose I update the classes I want to take in the 'Fall 2018' semester and then save those courses with the 'Save Courses' button:
Fall 2018 Courses
Then I go to a new semester:
Spring 2019
When I go back to 'Fall 2018', the courses should be updated with the ones that I initially saved (CS 2800 and MATH 2940, as well as the corresponding requirements they fulfill). However, this is not the case:
After going back to Fall 2018
I debugged this issue by ensuring that the selected indices were correct (i.e. by logging options.selectedIndex to the console), and it was indeed correct. But the classes are not updated.
I'm having a hard time exactly figuring out what could cause this issue to occur. I appreciate any suggestions, thank you!
javascript html
javascript html
edited Nov 10 at 22:16
marc_s
565k12610921244
565k12610921244
asked Nov 9 at 22:16


azdev
61
61
Can you add your code to a jsFiddle?
– Simsteve7
Nov 9 at 22:33
jsfiddle.net/8sfpzL6c
– azdev
Nov 10 at 0:44
@Simsteve7 Please do not request code on external code sites. StackOverflow has snippet functionality which is what is required to use unless SO snippet functionality is not sufficient.
– connexo
Nov 10 at 22:20
add a comment |
Can you add your code to a jsFiddle?
– Simsteve7
Nov 9 at 22:33
jsfiddle.net/8sfpzL6c
– azdev
Nov 10 at 0:44
@Simsteve7 Please do not request code on external code sites. StackOverflow has snippet functionality which is what is required to use unless SO snippet functionality is not sufficient.
– connexo
Nov 10 at 22:20
Can you add your code to a jsFiddle?
– Simsteve7
Nov 9 at 22:33
Can you add your code to a jsFiddle?
– Simsteve7
Nov 9 at 22:33
jsfiddle.net/8sfpzL6c
– azdev
Nov 10 at 0:44
jsfiddle.net/8sfpzL6c
– azdev
Nov 10 at 0:44
@Simsteve7 Please do not request code on external code sites. StackOverflow has snippet functionality which is what is required to use unless SO snippet functionality is not sufficient.
– connexo
Nov 10 at 22:20
@Simsteve7 Please do not request code on external code sites. StackOverflow has snippet functionality which is what is required to use unless SO snippet functionality is not sufficient.
– connexo
Nov 10 at 22:20
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53233963%2fselected-option-is-not-updated-even-after-making-the-necessary-changes%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Uf9Gf,N cVFlYgj7p44ZH4zQ ui,47LOoq,v7BofQw,fy1ZoYeEQQZHFrqKXt9oPvEDLYCx,YYd3Ub,X1L,VLcNeEhMoVkMdC3O,G4Rog
Can you add your code to a jsFiddle?
– Simsteve7
Nov 9 at 22:33
jsfiddle.net/8sfpzL6c
– azdev
Nov 10 at 0:44
@Simsteve7 Please do not request code on external code sites. StackOverflow has snippet functionality which is what is required to use unless SO snippet functionality is not sufficient.
– connexo
Nov 10 at 22:20