update defaultdict count according to the occurrence of tuples in two lists
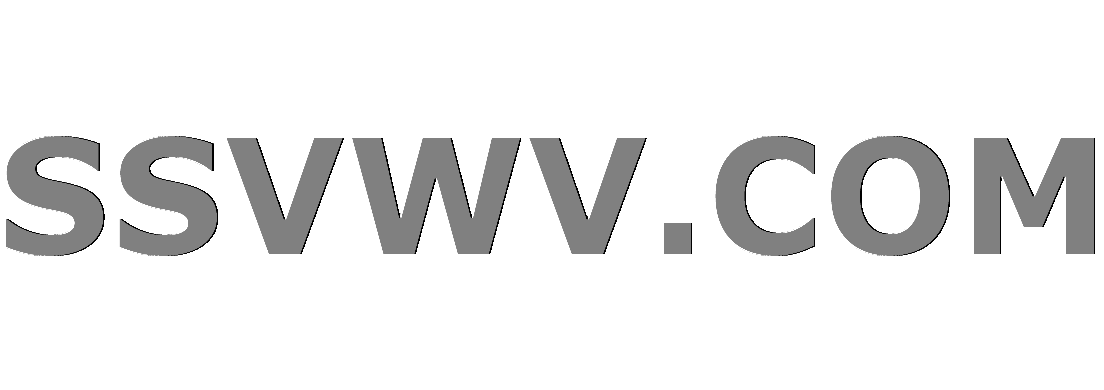
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I have two lists and would like to create a dictionary to record the occurrence of tuples.
My current code:
tup_to_find_test = [('good', 'pea'), ('leaf', 'sweet')]
self_per_list_test = [('leaf', 'liquid'), ('leaf', 'sweet'), ('leaf', 'sweet'),('good', 'pea'),('good', 'pea'),('good', 'pea')]
from collections import defaultdict
tup_dict_test = defaultdict(int)
for tup_to_find_test in self_per_list_test:
tup_dict_test[tup_to_find_test]+=1
My result is:
defaultdict(int, {('leaf', 'liquid'): 1, ('leaf', 'sweet'): 1, ('good', 'pea'): 3})
My desired result is:
('leaf', 'liquid'): 0, ('leaf', 'sweet'): 2, ('good', 'pea'): 3})
I do not know why the count of ('leaf', 'liquid')
is 1. Isn't the default integer of defaultdict(int)
zero? Why I got 1 for the ('leaf', 'liquid')
tuple?
python list dictionary tuples counter
add a comment |
I have two lists and would like to create a dictionary to record the occurrence of tuples.
My current code:
tup_to_find_test = [('good', 'pea'), ('leaf', 'sweet')]
self_per_list_test = [('leaf', 'liquid'), ('leaf', 'sweet'), ('leaf', 'sweet'),('good', 'pea'),('good', 'pea'),('good', 'pea')]
from collections import defaultdict
tup_dict_test = defaultdict(int)
for tup_to_find_test in self_per_list_test:
tup_dict_test[tup_to_find_test]+=1
My result is:
defaultdict(int, {('leaf', 'liquid'): 1, ('leaf', 'sweet'): 1, ('good', 'pea'): 3})
My desired result is:
('leaf', 'liquid'): 0, ('leaf', 'sweet'): 2, ('good', 'pea'): 3})
I do not know why the count of ('leaf', 'liquid')
is 1. Isn't the default integer of defaultdict(int)
zero? Why I got 1 for the ('leaf', 'liquid')
tuple?
python list dictionary tuples counter
1
You have('leaf', 'liquid')
inself_per_list_test
once, so its count is 1
– roeen30
Nov 16 '18 at 21:58
add a comment |
I have two lists and would like to create a dictionary to record the occurrence of tuples.
My current code:
tup_to_find_test = [('good', 'pea'), ('leaf', 'sweet')]
self_per_list_test = [('leaf', 'liquid'), ('leaf', 'sweet'), ('leaf', 'sweet'),('good', 'pea'),('good', 'pea'),('good', 'pea')]
from collections import defaultdict
tup_dict_test = defaultdict(int)
for tup_to_find_test in self_per_list_test:
tup_dict_test[tup_to_find_test]+=1
My result is:
defaultdict(int, {('leaf', 'liquid'): 1, ('leaf', 'sweet'): 1, ('good', 'pea'): 3})
My desired result is:
('leaf', 'liquid'): 0, ('leaf', 'sweet'): 2, ('good', 'pea'): 3})
I do not know why the count of ('leaf', 'liquid')
is 1. Isn't the default integer of defaultdict(int)
zero? Why I got 1 for the ('leaf', 'liquid')
tuple?
python list dictionary tuples counter
I have two lists and would like to create a dictionary to record the occurrence of tuples.
My current code:
tup_to_find_test = [('good', 'pea'), ('leaf', 'sweet')]
self_per_list_test = [('leaf', 'liquid'), ('leaf', 'sweet'), ('leaf', 'sweet'),('good', 'pea'),('good', 'pea'),('good', 'pea')]
from collections import defaultdict
tup_dict_test = defaultdict(int)
for tup_to_find_test in self_per_list_test:
tup_dict_test[tup_to_find_test]+=1
My result is:
defaultdict(int, {('leaf', 'liquid'): 1, ('leaf', 'sweet'): 1, ('good', 'pea'): 3})
My desired result is:
('leaf', 'liquid'): 0, ('leaf', 'sweet'): 2, ('good', 'pea'): 3})
I do not know why the count of ('leaf', 'liquid')
is 1. Isn't the default integer of defaultdict(int)
zero? Why I got 1 for the ('leaf', 'liquid')
tuple?
python list dictionary tuples counter
python list dictionary tuples counter
edited Nov 19 '18 at 15:23


jpp
103k2167117
103k2167117
asked Nov 16 '18 at 21:49
AbbeyAbbey
1107
1107
1
You have('leaf', 'liquid')
inself_per_list_test
once, so its count is 1
– roeen30
Nov 16 '18 at 21:58
add a comment |
1
You have('leaf', 'liquid')
inself_per_list_test
once, so its count is 1
– roeen30
Nov 16 '18 at 21:58
1
1
You have
('leaf', 'liquid')
in self_per_list_test
once, so its count is 1– roeen30
Nov 16 '18 at 21:58
You have
('leaf', 'liquid')
in self_per_list_test
once, so its count is 1– roeen30
Nov 16 '18 at 21:58
add a comment |
3 Answers
3
active
oldest
votes
This line isn't doing what you think it is:
for tup_to_find_test in self_per_list_test:
# ...
Here you are iterating a list elementwise, in this case the elements of self_per_list_test
. There's no filtering taking place. As your for
loop iterates, tup_to_find_test
successively represents ('leaf', 'liquid')
, ('leaf', 'sweet')
, etc. The fact the name is the same as a variable you've defined earlier only serves to confuse.
Instead, you can use a ternary statement to differentiate operations:
for item in self_per_list_test:
tup_dict_test[item] += 1 if item in tup_to_find_test else 0
print(tup_dict_test)
defaultdict(int, {('leaf', 'liquid'): 0, ('leaf', 'sweet'): 2, ('good', 'pea'): 3})
collections.Counter
is more idiomatic with Python. It's good practice to use set
for O(1) lookup within a dictionary comprehension.
from collections import Counter
tup_to_find_set = set(tup_to_find_test)
counts = Counter(self_per_list_test)
tup_dict_test = {k: v if k in tup_to_find_set else 0 for k, v in counts.items()}
print(tup_dict_test)
{('leaf', 'liquid'): 0, ('leaf', 'sweet'): 2, ('good', 'pea'): 3}
This is one line fashion of @BernardL solution right? It is very neat and smart! Thanks!!!
– Abbey
Nov 16 '18 at 22:19
1
@Abbey, Yep, if you want to stick withdefaultdict
, it is indeed a one-liner. It's just advisable to use hashing viaset
to make it efficient.
– jpp
Nov 16 '18 at 22:20
add a comment |
Isn't the default integer of
defaultdict(int)
zero?
Yes.
Why I got 1 for the ('leaf', 'liquid') tuple?
You wrote:
tup_dict_test[tup_to_find_test]+=1
That is, find the current value—which creates a new one set to zero—then add one to it and store the result back. The resulting value is 1.
add a comment |
Without reinventing the wheel. You can use counter
from the wonderful collections
standard module library for this.
from collections import Counter
tup_to_find_test = [('good', 'pea'), ('leaf', 'sweet')]
self_per_list_test = [('leaf', 'liquid'), ('leaf', 'sweet'), ('leaf', 'sweet'),('good', 'pea'),('good', 'pea'),('good', 'pea')]
c = Counter(self_per_list_test)
for key in c:
if key not in tup_to_find_test:
c[key] = 0
print(c)
>>Counter({('good', 'pea'): 3, ('leaf', 'sweet'): 2, ('leaf', 'liquid'): 0})
Here we create a counter based on self_per_list_test
and updates the counts to zero if it is not found in tup_to_find_test
. Hope this is a more intuitive method in solving your problem.
A quick question. Compared todefaultdict
, is theCounter
faster than usingdefaultdict
?
– Abbey
Nov 16 '18 at 22:03
1
Well, you cannot comparedefaultdict
to aCounter
,defaultdict
calls a factory function to supply missing values. ACounter
is optimized to count hashable values. In this use case, if your purpose is just to count, I would go withCounter
.
– BernardL
Nov 16 '18 at 22:06
I tried both of them on my dataset. You are right. As my goal is to simply count the frequency of tuple, I should go forCounter
. Thanks for your smart/straightforward solution! (I think I overthink about my question)
– Abbey
Nov 16 '18 at 22:16
1
Yeah hoped it helped. If you found any of the answers here useful and alas, please accept the best answer here that helped you solved your problem.
– BernardL
Nov 16 '18 at 22:20
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53345897%2fupdate-defaultdict-count-according-to-the-occurrence-of-tuples-in-two-lists%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
This line isn't doing what you think it is:
for tup_to_find_test in self_per_list_test:
# ...
Here you are iterating a list elementwise, in this case the elements of self_per_list_test
. There's no filtering taking place. As your for
loop iterates, tup_to_find_test
successively represents ('leaf', 'liquid')
, ('leaf', 'sweet')
, etc. The fact the name is the same as a variable you've defined earlier only serves to confuse.
Instead, you can use a ternary statement to differentiate operations:
for item in self_per_list_test:
tup_dict_test[item] += 1 if item in tup_to_find_test else 0
print(tup_dict_test)
defaultdict(int, {('leaf', 'liquid'): 0, ('leaf', 'sweet'): 2, ('good', 'pea'): 3})
collections.Counter
is more idiomatic with Python. It's good practice to use set
for O(1) lookup within a dictionary comprehension.
from collections import Counter
tup_to_find_set = set(tup_to_find_test)
counts = Counter(self_per_list_test)
tup_dict_test = {k: v if k in tup_to_find_set else 0 for k, v in counts.items()}
print(tup_dict_test)
{('leaf', 'liquid'): 0, ('leaf', 'sweet'): 2, ('good', 'pea'): 3}
This is one line fashion of @BernardL solution right? It is very neat and smart! Thanks!!!
– Abbey
Nov 16 '18 at 22:19
1
@Abbey, Yep, if you want to stick withdefaultdict
, it is indeed a one-liner. It's just advisable to use hashing viaset
to make it efficient.
– jpp
Nov 16 '18 at 22:20
add a comment |
This line isn't doing what you think it is:
for tup_to_find_test in self_per_list_test:
# ...
Here you are iterating a list elementwise, in this case the elements of self_per_list_test
. There's no filtering taking place. As your for
loop iterates, tup_to_find_test
successively represents ('leaf', 'liquid')
, ('leaf', 'sweet')
, etc. The fact the name is the same as a variable you've defined earlier only serves to confuse.
Instead, you can use a ternary statement to differentiate operations:
for item in self_per_list_test:
tup_dict_test[item] += 1 if item in tup_to_find_test else 0
print(tup_dict_test)
defaultdict(int, {('leaf', 'liquid'): 0, ('leaf', 'sweet'): 2, ('good', 'pea'): 3})
collections.Counter
is more idiomatic with Python. It's good practice to use set
for O(1) lookup within a dictionary comprehension.
from collections import Counter
tup_to_find_set = set(tup_to_find_test)
counts = Counter(self_per_list_test)
tup_dict_test = {k: v if k in tup_to_find_set else 0 for k, v in counts.items()}
print(tup_dict_test)
{('leaf', 'liquid'): 0, ('leaf', 'sweet'): 2, ('good', 'pea'): 3}
This is one line fashion of @BernardL solution right? It is very neat and smart! Thanks!!!
– Abbey
Nov 16 '18 at 22:19
1
@Abbey, Yep, if you want to stick withdefaultdict
, it is indeed a one-liner. It's just advisable to use hashing viaset
to make it efficient.
– jpp
Nov 16 '18 at 22:20
add a comment |
This line isn't doing what you think it is:
for tup_to_find_test in self_per_list_test:
# ...
Here you are iterating a list elementwise, in this case the elements of self_per_list_test
. There's no filtering taking place. As your for
loop iterates, tup_to_find_test
successively represents ('leaf', 'liquid')
, ('leaf', 'sweet')
, etc. The fact the name is the same as a variable you've defined earlier only serves to confuse.
Instead, you can use a ternary statement to differentiate operations:
for item in self_per_list_test:
tup_dict_test[item] += 1 if item in tup_to_find_test else 0
print(tup_dict_test)
defaultdict(int, {('leaf', 'liquid'): 0, ('leaf', 'sweet'): 2, ('good', 'pea'): 3})
collections.Counter
is more idiomatic with Python. It's good practice to use set
for O(1) lookup within a dictionary comprehension.
from collections import Counter
tup_to_find_set = set(tup_to_find_test)
counts = Counter(self_per_list_test)
tup_dict_test = {k: v if k in tup_to_find_set else 0 for k, v in counts.items()}
print(tup_dict_test)
{('leaf', 'liquid'): 0, ('leaf', 'sweet'): 2, ('good', 'pea'): 3}
This line isn't doing what you think it is:
for tup_to_find_test in self_per_list_test:
# ...
Here you are iterating a list elementwise, in this case the elements of self_per_list_test
. There's no filtering taking place. As your for
loop iterates, tup_to_find_test
successively represents ('leaf', 'liquid')
, ('leaf', 'sweet')
, etc. The fact the name is the same as a variable you've defined earlier only serves to confuse.
Instead, you can use a ternary statement to differentiate operations:
for item in self_per_list_test:
tup_dict_test[item] += 1 if item in tup_to_find_test else 0
print(tup_dict_test)
defaultdict(int, {('leaf', 'liquid'): 0, ('leaf', 'sweet'): 2, ('good', 'pea'): 3})
collections.Counter
is more idiomatic with Python. It's good practice to use set
for O(1) lookup within a dictionary comprehension.
from collections import Counter
tup_to_find_set = set(tup_to_find_test)
counts = Counter(self_per_list_test)
tup_dict_test = {k: v if k in tup_to_find_set else 0 for k, v in counts.items()}
print(tup_dict_test)
{('leaf', 'liquid'): 0, ('leaf', 'sweet'): 2, ('good', 'pea'): 3}
edited Nov 16 '18 at 22:05
answered Nov 16 '18 at 22:00


jppjpp
103k2167117
103k2167117
This is one line fashion of @BernardL solution right? It is very neat and smart! Thanks!!!
– Abbey
Nov 16 '18 at 22:19
1
@Abbey, Yep, if you want to stick withdefaultdict
, it is indeed a one-liner. It's just advisable to use hashing viaset
to make it efficient.
– jpp
Nov 16 '18 at 22:20
add a comment |
This is one line fashion of @BernardL solution right? It is very neat and smart! Thanks!!!
– Abbey
Nov 16 '18 at 22:19
1
@Abbey, Yep, if you want to stick withdefaultdict
, it is indeed a one-liner. It's just advisable to use hashing viaset
to make it efficient.
– jpp
Nov 16 '18 at 22:20
This is one line fashion of @BernardL solution right? It is very neat and smart! Thanks!!!
– Abbey
Nov 16 '18 at 22:19
This is one line fashion of @BernardL solution right? It is very neat and smart! Thanks!!!
– Abbey
Nov 16 '18 at 22:19
1
1
@Abbey, Yep, if you want to stick with
defaultdict
, it is indeed a one-liner. It's just advisable to use hashing via set
to make it efficient.– jpp
Nov 16 '18 at 22:20
@Abbey, Yep, if you want to stick with
defaultdict
, it is indeed a one-liner. It's just advisable to use hashing via set
to make it efficient.– jpp
Nov 16 '18 at 22:20
add a comment |
Isn't the default integer of
defaultdict(int)
zero?
Yes.
Why I got 1 for the ('leaf', 'liquid') tuple?
You wrote:
tup_dict_test[tup_to_find_test]+=1
That is, find the current value—which creates a new one set to zero—then add one to it and store the result back. The resulting value is 1.
add a comment |
Isn't the default integer of
defaultdict(int)
zero?
Yes.
Why I got 1 for the ('leaf', 'liquid') tuple?
You wrote:
tup_dict_test[tup_to_find_test]+=1
That is, find the current value—which creates a new one set to zero—then add one to it and store the result back. The resulting value is 1.
add a comment |
Isn't the default integer of
defaultdict(int)
zero?
Yes.
Why I got 1 for the ('leaf', 'liquid') tuple?
You wrote:
tup_dict_test[tup_to_find_test]+=1
That is, find the current value—which creates a new one set to zero—then add one to it and store the result back. The resulting value is 1.
Isn't the default integer of
defaultdict(int)
zero?
Yes.
Why I got 1 for the ('leaf', 'liquid') tuple?
You wrote:
tup_dict_test[tup_to_find_test]+=1
That is, find the current value—which creates a new one set to zero—then add one to it and store the result back. The resulting value is 1.
answered Nov 16 '18 at 21:58
torektorek
201k19251335
201k19251335
add a comment |
add a comment |
Without reinventing the wheel. You can use counter
from the wonderful collections
standard module library for this.
from collections import Counter
tup_to_find_test = [('good', 'pea'), ('leaf', 'sweet')]
self_per_list_test = [('leaf', 'liquid'), ('leaf', 'sweet'), ('leaf', 'sweet'),('good', 'pea'),('good', 'pea'),('good', 'pea')]
c = Counter(self_per_list_test)
for key in c:
if key not in tup_to_find_test:
c[key] = 0
print(c)
>>Counter({('good', 'pea'): 3, ('leaf', 'sweet'): 2, ('leaf', 'liquid'): 0})
Here we create a counter based on self_per_list_test
and updates the counts to zero if it is not found in tup_to_find_test
. Hope this is a more intuitive method in solving your problem.
A quick question. Compared todefaultdict
, is theCounter
faster than usingdefaultdict
?
– Abbey
Nov 16 '18 at 22:03
1
Well, you cannot comparedefaultdict
to aCounter
,defaultdict
calls a factory function to supply missing values. ACounter
is optimized to count hashable values. In this use case, if your purpose is just to count, I would go withCounter
.
– BernardL
Nov 16 '18 at 22:06
I tried both of them on my dataset. You are right. As my goal is to simply count the frequency of tuple, I should go forCounter
. Thanks for your smart/straightforward solution! (I think I overthink about my question)
– Abbey
Nov 16 '18 at 22:16
1
Yeah hoped it helped. If you found any of the answers here useful and alas, please accept the best answer here that helped you solved your problem.
– BernardL
Nov 16 '18 at 22:20
add a comment |
Without reinventing the wheel. You can use counter
from the wonderful collections
standard module library for this.
from collections import Counter
tup_to_find_test = [('good', 'pea'), ('leaf', 'sweet')]
self_per_list_test = [('leaf', 'liquid'), ('leaf', 'sweet'), ('leaf', 'sweet'),('good', 'pea'),('good', 'pea'),('good', 'pea')]
c = Counter(self_per_list_test)
for key in c:
if key not in tup_to_find_test:
c[key] = 0
print(c)
>>Counter({('good', 'pea'): 3, ('leaf', 'sweet'): 2, ('leaf', 'liquid'): 0})
Here we create a counter based on self_per_list_test
and updates the counts to zero if it is not found in tup_to_find_test
. Hope this is a more intuitive method in solving your problem.
A quick question. Compared todefaultdict
, is theCounter
faster than usingdefaultdict
?
– Abbey
Nov 16 '18 at 22:03
1
Well, you cannot comparedefaultdict
to aCounter
,defaultdict
calls a factory function to supply missing values. ACounter
is optimized to count hashable values. In this use case, if your purpose is just to count, I would go withCounter
.
– BernardL
Nov 16 '18 at 22:06
I tried both of them on my dataset. You are right. As my goal is to simply count the frequency of tuple, I should go forCounter
. Thanks for your smart/straightforward solution! (I think I overthink about my question)
– Abbey
Nov 16 '18 at 22:16
1
Yeah hoped it helped. If you found any of the answers here useful and alas, please accept the best answer here that helped you solved your problem.
– BernardL
Nov 16 '18 at 22:20
add a comment |
Without reinventing the wheel. You can use counter
from the wonderful collections
standard module library for this.
from collections import Counter
tup_to_find_test = [('good', 'pea'), ('leaf', 'sweet')]
self_per_list_test = [('leaf', 'liquid'), ('leaf', 'sweet'), ('leaf', 'sweet'),('good', 'pea'),('good', 'pea'),('good', 'pea')]
c = Counter(self_per_list_test)
for key in c:
if key not in tup_to_find_test:
c[key] = 0
print(c)
>>Counter({('good', 'pea'): 3, ('leaf', 'sweet'): 2, ('leaf', 'liquid'): 0})
Here we create a counter based on self_per_list_test
and updates the counts to zero if it is not found in tup_to_find_test
. Hope this is a more intuitive method in solving your problem.
Without reinventing the wheel. You can use counter
from the wonderful collections
standard module library for this.
from collections import Counter
tup_to_find_test = [('good', 'pea'), ('leaf', 'sweet')]
self_per_list_test = [('leaf', 'liquid'), ('leaf', 'sweet'), ('leaf', 'sweet'),('good', 'pea'),('good', 'pea'),('good', 'pea')]
c = Counter(self_per_list_test)
for key in c:
if key not in tup_to_find_test:
c[key] = 0
print(c)
>>Counter({('good', 'pea'): 3, ('leaf', 'sweet'): 2, ('leaf', 'liquid'): 0})
Here we create a counter based on self_per_list_test
and updates the counts to zero if it is not found in tup_to_find_test
. Hope this is a more intuitive method in solving your problem.
answered Nov 16 '18 at 22:01


BernardLBernardL
2,42411232
2,42411232
A quick question. Compared todefaultdict
, is theCounter
faster than usingdefaultdict
?
– Abbey
Nov 16 '18 at 22:03
1
Well, you cannot comparedefaultdict
to aCounter
,defaultdict
calls a factory function to supply missing values. ACounter
is optimized to count hashable values. In this use case, if your purpose is just to count, I would go withCounter
.
– BernardL
Nov 16 '18 at 22:06
I tried both of them on my dataset. You are right. As my goal is to simply count the frequency of tuple, I should go forCounter
. Thanks for your smart/straightforward solution! (I think I overthink about my question)
– Abbey
Nov 16 '18 at 22:16
1
Yeah hoped it helped. If you found any of the answers here useful and alas, please accept the best answer here that helped you solved your problem.
– BernardL
Nov 16 '18 at 22:20
add a comment |
A quick question. Compared todefaultdict
, is theCounter
faster than usingdefaultdict
?
– Abbey
Nov 16 '18 at 22:03
1
Well, you cannot comparedefaultdict
to aCounter
,defaultdict
calls a factory function to supply missing values. ACounter
is optimized to count hashable values. In this use case, if your purpose is just to count, I would go withCounter
.
– BernardL
Nov 16 '18 at 22:06
I tried both of them on my dataset. You are right. As my goal is to simply count the frequency of tuple, I should go forCounter
. Thanks for your smart/straightforward solution! (I think I overthink about my question)
– Abbey
Nov 16 '18 at 22:16
1
Yeah hoped it helped. If you found any of the answers here useful and alas, please accept the best answer here that helped you solved your problem.
– BernardL
Nov 16 '18 at 22:20
A quick question. Compared to
defaultdict
, is the Counter
faster than using defaultdict
?– Abbey
Nov 16 '18 at 22:03
A quick question. Compared to
defaultdict
, is the Counter
faster than using defaultdict
?– Abbey
Nov 16 '18 at 22:03
1
1
Well, you cannot compare
defaultdict
to a Counter
, defaultdict
calls a factory function to supply missing values. A Counter
is optimized to count hashable values. In this use case, if your purpose is just to count, I would go with Counter
.– BernardL
Nov 16 '18 at 22:06
Well, you cannot compare
defaultdict
to a Counter
, defaultdict
calls a factory function to supply missing values. A Counter
is optimized to count hashable values. In this use case, if your purpose is just to count, I would go with Counter
.– BernardL
Nov 16 '18 at 22:06
I tried both of them on my dataset. You are right. As my goal is to simply count the frequency of tuple, I should go for
Counter
. Thanks for your smart/straightforward solution! (I think I overthink about my question)– Abbey
Nov 16 '18 at 22:16
I tried both of them on my dataset. You are right. As my goal is to simply count the frequency of tuple, I should go for
Counter
. Thanks for your smart/straightforward solution! (I think I overthink about my question)– Abbey
Nov 16 '18 at 22:16
1
1
Yeah hoped it helped. If you found any of the answers here useful and alas, please accept the best answer here that helped you solved your problem.
– BernardL
Nov 16 '18 at 22:20
Yeah hoped it helped. If you found any of the answers here useful and alas, please accept the best answer here that helped you solved your problem.
– BernardL
Nov 16 '18 at 22:20
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53345897%2fupdate-defaultdict-count-according-to-the-occurrence-of-tuples-in-two-lists%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
H,GTFDK,zK2Dn2njSykYgZMsyoqMcLd4IaGQ5 rq2Ca7XZNZbs
1
You have
('leaf', 'liquid')
inself_per_list_test
once, so its count is 1– roeen30
Nov 16 '18 at 21:58