Adding Properties to existing Javascript Objects from Object Constructors
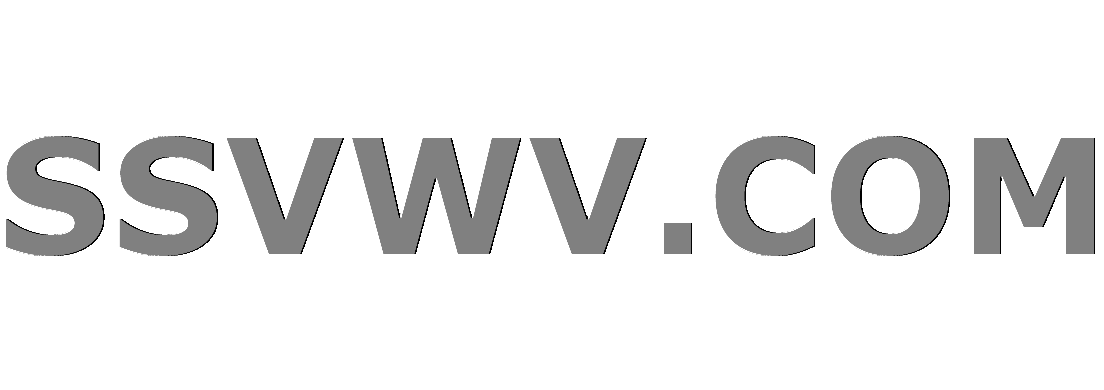
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
When creating an object constructor in Javascript, I understand that it's necessary to prepend property names with the 'this' keyword.
function funcConstruct(first,last,age) {
this.firstName = first;
this.lastName = last;
this.age = age;
this.fullNameAge = function() {
return this.lastName + ', ' + this.firstName + ', ' + this.age
};
};
var johnDoe = new funcConstruct('John', 'Doe', 52);
console.log(johnDoe.fullNameAge());
Doe, John, 52
If you wish to add additional properties to the object later, do you need to use the 'this' keyword, and if so, where does it go in the syntax?
javascript constructor
add a comment |
When creating an object constructor in Javascript, I understand that it's necessary to prepend property names with the 'this' keyword.
function funcConstruct(first,last,age) {
this.firstName = first;
this.lastName = last;
this.age = age;
this.fullNameAge = function() {
return this.lastName + ', ' + this.firstName + ', ' + this.age
};
};
var johnDoe = new funcConstruct('John', 'Doe', 52);
console.log(johnDoe.fullNameAge());
Doe, John, 52
If you wish to add additional properties to the object later, do you need to use the 'this' keyword, and if so, where does it go in the syntax?
javascript constructor
johnDoe.foo = 'bar';
. Also, while comma is not syntactically invalid, it is not good practice to end statements with it. Use a semicolon instead.
– Patrick Roberts
Nov 16 '18 at 21:44
Whether you need to usethis
depends on what exactly you mean by "later" and how that code will be called.
– Bergi
Nov 16 '18 at 22:16
Thanks Robert -- I swapped in semicolons.
– efw
Nov 16 '18 at 23:45
add a comment |
When creating an object constructor in Javascript, I understand that it's necessary to prepend property names with the 'this' keyword.
function funcConstruct(first,last,age) {
this.firstName = first;
this.lastName = last;
this.age = age;
this.fullNameAge = function() {
return this.lastName + ', ' + this.firstName + ', ' + this.age
};
};
var johnDoe = new funcConstruct('John', 'Doe', 52);
console.log(johnDoe.fullNameAge());
Doe, John, 52
If you wish to add additional properties to the object later, do you need to use the 'this' keyword, and if so, where does it go in the syntax?
javascript constructor
When creating an object constructor in Javascript, I understand that it's necessary to prepend property names with the 'this' keyword.
function funcConstruct(first,last,age) {
this.firstName = first;
this.lastName = last;
this.age = age;
this.fullNameAge = function() {
return this.lastName + ', ' + this.firstName + ', ' + this.age
};
};
var johnDoe = new funcConstruct('John', 'Doe', 52);
console.log(johnDoe.fullNameAge());
Doe, John, 52
If you wish to add additional properties to the object later, do you need to use the 'this' keyword, and if so, where does it go in the syntax?
javascript constructor
javascript constructor
edited Nov 16 '18 at 23:52
efw
asked Nov 16 '18 at 21:41
efwefw
110110
110110
johnDoe.foo = 'bar';
. Also, while comma is not syntactically invalid, it is not good practice to end statements with it. Use a semicolon instead.
– Patrick Roberts
Nov 16 '18 at 21:44
Whether you need to usethis
depends on what exactly you mean by "later" and how that code will be called.
– Bergi
Nov 16 '18 at 22:16
Thanks Robert -- I swapped in semicolons.
– efw
Nov 16 '18 at 23:45
add a comment |
johnDoe.foo = 'bar';
. Also, while comma is not syntactically invalid, it is not good practice to end statements with it. Use a semicolon instead.
– Patrick Roberts
Nov 16 '18 at 21:44
Whether you need to usethis
depends on what exactly you mean by "later" and how that code will be called.
– Bergi
Nov 16 '18 at 22:16
Thanks Robert -- I swapped in semicolons.
– efw
Nov 16 '18 at 23:45
johnDoe.foo = 'bar';
. Also, while comma is not syntactically invalid, it is not good practice to end statements with it. Use a semicolon instead.– Patrick Roberts
Nov 16 '18 at 21:44
johnDoe.foo = 'bar';
. Also, while comma is not syntactically invalid, it is not good practice to end statements with it. Use a semicolon instead.– Patrick Roberts
Nov 16 '18 at 21:44
Whether you need to use
this
depends on what exactly you mean by "later" and how that code will be called.– Bergi
Nov 16 '18 at 22:16
Whether you need to use
this
depends on what exactly you mean by "later" and how that code will be called.– Bergi
Nov 16 '18 at 22:16
Thanks Robert -- I swapped in semicolons.
– efw
Nov 16 '18 at 23:45
Thanks Robert -- I swapped in semicolons.
– efw
Nov 16 '18 at 23:45
add a comment |
1 Answer
1
active
oldest
votes
You can add additional properties after the fact:
function funcConstruct(first,last,age) {
this.firstName = first,
this.lastName = last,
this.age = age,
this.fullNameAge = function() {
return this.lastName + ', ' + this.firstName + ', ' + this.age
}
};
var johnDoe = new funcConstruct('John', 'Doe', 52);
johnDoe.foo = "bar";
You are using the function constructor pattern, but note that there are other javascript object encapsulation patterns you can use depending on your use case some are better than others. In my opinion, I would use the function constructor pattern only if I was using pre ES6, if using >=ES6 (ES2015) I would use a class, and only if I needed a bunch of instances of the object. Otherwise, I would rather use the revealing module pattern if only a single instance of the object is required. Use fun
No use re-inventing the wheel, the accepted answer and some other highly voted answers to this question give a good summary. This video explains some of the confusion around using this
in javascript and provides an opinion that it is probably best to avoid this
where possible.
Okay that's just uncanny...
– Patrick Roberts
Nov 16 '18 at 21:45
Should note there are other ways to do it as well such as setter in constructor
– charlietfl
Nov 16 '18 at 21:53
1
Thanks for the answers. I'm new to OOP. I understand there are other patterns for creating objects, and good reasons for avoiding this one, but I just wanted to make sure I understood how it worked on its most basic level.
– efw
Nov 16 '18 at 23:42
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53345814%2fadding-properties-to-existing-javascript-objects-from-object-constructors%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can add additional properties after the fact:
function funcConstruct(first,last,age) {
this.firstName = first,
this.lastName = last,
this.age = age,
this.fullNameAge = function() {
return this.lastName + ', ' + this.firstName + ', ' + this.age
}
};
var johnDoe = new funcConstruct('John', 'Doe', 52);
johnDoe.foo = "bar";
You are using the function constructor pattern, but note that there are other javascript object encapsulation patterns you can use depending on your use case some are better than others. In my opinion, I would use the function constructor pattern only if I was using pre ES6, if using >=ES6 (ES2015) I would use a class, and only if I needed a bunch of instances of the object. Otherwise, I would rather use the revealing module pattern if only a single instance of the object is required. Use fun
No use re-inventing the wheel, the accepted answer and some other highly voted answers to this question give a good summary. This video explains some of the confusion around using this
in javascript and provides an opinion that it is probably best to avoid this
where possible.
Okay that's just uncanny...
– Patrick Roberts
Nov 16 '18 at 21:45
Should note there are other ways to do it as well such as setter in constructor
– charlietfl
Nov 16 '18 at 21:53
1
Thanks for the answers. I'm new to OOP. I understand there are other patterns for creating objects, and good reasons for avoiding this one, but I just wanted to make sure I understood how it worked on its most basic level.
– efw
Nov 16 '18 at 23:42
add a comment |
You can add additional properties after the fact:
function funcConstruct(first,last,age) {
this.firstName = first,
this.lastName = last,
this.age = age,
this.fullNameAge = function() {
return this.lastName + ', ' + this.firstName + ', ' + this.age
}
};
var johnDoe = new funcConstruct('John', 'Doe', 52);
johnDoe.foo = "bar";
You are using the function constructor pattern, but note that there are other javascript object encapsulation patterns you can use depending on your use case some are better than others. In my opinion, I would use the function constructor pattern only if I was using pre ES6, if using >=ES6 (ES2015) I would use a class, and only if I needed a bunch of instances of the object. Otherwise, I would rather use the revealing module pattern if only a single instance of the object is required. Use fun
No use re-inventing the wheel, the accepted answer and some other highly voted answers to this question give a good summary. This video explains some of the confusion around using this
in javascript and provides an opinion that it is probably best to avoid this
where possible.
Okay that's just uncanny...
– Patrick Roberts
Nov 16 '18 at 21:45
Should note there are other ways to do it as well such as setter in constructor
– charlietfl
Nov 16 '18 at 21:53
1
Thanks for the answers. I'm new to OOP. I understand there are other patterns for creating objects, and good reasons for avoiding this one, but I just wanted to make sure I understood how it worked on its most basic level.
– efw
Nov 16 '18 at 23:42
add a comment |
You can add additional properties after the fact:
function funcConstruct(first,last,age) {
this.firstName = first,
this.lastName = last,
this.age = age,
this.fullNameAge = function() {
return this.lastName + ', ' + this.firstName + ', ' + this.age
}
};
var johnDoe = new funcConstruct('John', 'Doe', 52);
johnDoe.foo = "bar";
You are using the function constructor pattern, but note that there are other javascript object encapsulation patterns you can use depending on your use case some are better than others. In my opinion, I would use the function constructor pattern only if I was using pre ES6, if using >=ES6 (ES2015) I would use a class, and only if I needed a bunch of instances of the object. Otherwise, I would rather use the revealing module pattern if only a single instance of the object is required. Use fun
No use re-inventing the wheel, the accepted answer and some other highly voted answers to this question give a good summary. This video explains some of the confusion around using this
in javascript and provides an opinion that it is probably best to avoid this
where possible.
You can add additional properties after the fact:
function funcConstruct(first,last,age) {
this.firstName = first,
this.lastName = last,
this.age = age,
this.fullNameAge = function() {
return this.lastName + ', ' + this.firstName + ', ' + this.age
}
};
var johnDoe = new funcConstruct('John', 'Doe', 52);
johnDoe.foo = "bar";
You are using the function constructor pattern, but note that there are other javascript object encapsulation patterns you can use depending on your use case some are better than others. In my opinion, I would use the function constructor pattern only if I was using pre ES6, if using >=ES6 (ES2015) I would use a class, and only if I needed a bunch of instances of the object. Otherwise, I would rather use the revealing module pattern if only a single instance of the object is required. Use fun
No use re-inventing the wheel, the accepted answer and some other highly voted answers to this question give a good summary. This video explains some of the confusion around using this
in javascript and provides an opinion that it is probably best to avoid this
where possible.
edited Nov 16 '18 at 22:08
answered Nov 16 '18 at 21:44


joshweirjoshweir
1,95621137
1,95621137
Okay that's just uncanny...
– Patrick Roberts
Nov 16 '18 at 21:45
Should note there are other ways to do it as well such as setter in constructor
– charlietfl
Nov 16 '18 at 21:53
1
Thanks for the answers. I'm new to OOP. I understand there are other patterns for creating objects, and good reasons for avoiding this one, but I just wanted to make sure I understood how it worked on its most basic level.
– efw
Nov 16 '18 at 23:42
add a comment |
Okay that's just uncanny...
– Patrick Roberts
Nov 16 '18 at 21:45
Should note there are other ways to do it as well such as setter in constructor
– charlietfl
Nov 16 '18 at 21:53
1
Thanks for the answers. I'm new to OOP. I understand there are other patterns for creating objects, and good reasons for avoiding this one, but I just wanted to make sure I understood how it worked on its most basic level.
– efw
Nov 16 '18 at 23:42
Okay that's just uncanny...
– Patrick Roberts
Nov 16 '18 at 21:45
Okay that's just uncanny...
– Patrick Roberts
Nov 16 '18 at 21:45
Should note there are other ways to do it as well such as setter in constructor
– charlietfl
Nov 16 '18 at 21:53
Should note there are other ways to do it as well such as setter in constructor
– charlietfl
Nov 16 '18 at 21:53
1
1
Thanks for the answers. I'm new to OOP. I understand there are other patterns for creating objects, and good reasons for avoiding this one, but I just wanted to make sure I understood how it worked on its most basic level.
– efw
Nov 16 '18 at 23:42
Thanks for the answers. I'm new to OOP. I understand there are other patterns for creating objects, and good reasons for avoiding this one, but I just wanted to make sure I understood how it worked on its most basic level.
– efw
Nov 16 '18 at 23:42
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53345814%2fadding-properties-to-existing-javascript-objects-from-object-constructors%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
3JWo309d4fd31T1hH
johnDoe.foo = 'bar';
. Also, while comma is not syntactically invalid, it is not good practice to end statements with it. Use a semicolon instead.– Patrick Roberts
Nov 16 '18 at 21:44
Whether you need to use
this
depends on what exactly you mean by "later" and how that code will be called.– Bergi
Nov 16 '18 at 22:16
Thanks Robert -- I swapped in semicolons.
– efw
Nov 16 '18 at 23:45